SolrNet.ISolrOperations< T > Interface Template Reference
Consolidating interface, exposes all operations. More...
Public Member Functions | |
ResponseHeader | Commit () |
Commits posted documents, blocking until index changes are flushed to disk and blocking until a new searcher is opened and registered as the main query searcher, making the changes visible. | |
ResponseHeader | Rollback () |
Rollbacks all add/deletes made to the index since the last commit. | |
ResponseHeader | Optimize () |
Optimizes Solr's index. | |
ResponseHeader | Add (T doc) |
Adds / updates a document. | |
ResponseHeader | Add (T doc, AddParameters parameters) |
Adds / updates a document with parameters. | |
ResponseHeader | AddWithBoost (T doc, double boost) |
Adds / updates a document with index-time boost. | |
ResponseHeader | AddWithBoost (T doc, double boost, AddParameters parameters) |
Adds / updates a document with index-time boost and add parameters. | |
ExtractResponse | Extract (ExtractParameters parameters) |
Adds / updates the extracted content of a richdocument. | |
ResponseHeader | Add (IEnumerable< T > docs) |
Adds / updates several documents at once. | |
ResponseHeader | AddRange (IEnumerable< T > docs) |
Adds / updates several documents at once. | |
ResponseHeader | Add (IEnumerable< T > docs, AddParameters parameters) |
Adds / updates several documents at once. | |
ResponseHeader | AddRange (IEnumerable< T > docs, AddParameters parameters) |
Adds / updates several documents at once. | |
ResponseHeader | AddWithBoost (IEnumerable< KeyValuePair< T, double?>> docs) |
Adds / updates documents with index-time boost. | |
ResponseHeader | AddRangeWithBoost (IEnumerable< KeyValuePair< T, double?>> docs) |
Adds / updates documents with index-time boost. | |
ResponseHeader | AddWithBoost (IEnumerable< KeyValuePair< T, double?>> docs, AddParameters parameters) |
Adds / updates documents with index-time boost and add parameters. | |
ResponseHeader | AddRangeWithBoost (IEnumerable< KeyValuePair< T, double?>> docs, AddParameters parameters) |
Adds / updates documents with index-time boost and add parameters. | |
ResponseHeader | Delete (T doc) |
Deletes a document (requires the document to have a unique key defined with non-null value) | |
ResponseHeader | Delete (IEnumerable< T > docs) |
Deletes several documents (requires the document type to have a unique key defined with non-null value) | |
ResponseHeader | Delete (ISolrQuery q) |
Deletes all documents that match a query. | |
ResponseHeader | Delete (string id) |
Deletes a document by its id (unique key) | |
ResponseHeader | Delete (IEnumerable< string > ids) |
Deletes several documents by their id (unique key) | |
ResponseHeader | Delete (IEnumerable< string > ids, ISolrQuery q) |
Deletes all documents that match the given id's or the query. | |
ResponseHeader | BuildSpellCheckDictionary () |
Create the dictionary for use by the SolrSpellChecker. In typical applications, one needs to build the dictionary before using it. However, it may not always be necessary as it is possible to setup the spellchecker with a dictionary that already exists. | |
IEnumerable< ValidationResult > | EnumerateValidationResults () |
Detailed Description
template<T>
interface SolrNet::ISolrOperations< T >
Consolidating interface, exposes all operations.
- Template Parameters:
-
T Document type
Member Function Documentation
ResponseHeader SolrNet.ISolrOperations< T >.Add | ( | T | doc ) |
Adds / updates a document.
- Parameters:
-
doc document to add/update
- Returns:
Implemented in SolrNet.Impl.SolrServer< T >.
ResponseHeader SolrNet.ISolrOperations< T >.Add | ( | T | doc, |
AddParameters | parameters | ||
) |
Adds / updates a document with parameters.
- Parameters:
-
doc The document to add/update. parameters The add parameters.
- Returns:
Implemented in SolrNet.Impl.SolrServer< T >.
ResponseHeader SolrNet.ISolrOperations< T >.Add | ( | IEnumerable< T > | docs ) |
Adds / updates several documents at once.
- Parameters:
-
docs documents to add/update
- Returns:
Implemented in SolrNet.Impl.SolrServer< T >.
ResponseHeader SolrNet.ISolrOperations< T >.Add | ( | IEnumerable< T > | docs, |
AddParameters | parameters | ||
) |
Adds / updates several documents at once.
- Parameters:
-
docs documents to add/update parameters The add parameters.
- Returns:
Implemented in SolrNet.Impl.SolrServer< T >.
ResponseHeader SolrNet.ISolrOperations< T >.AddRange | ( | IEnumerable< T > | docs ) |
Adds / updates several documents at once.
- Parameters:
-
docs documents to add/update
- Returns:
Implemented in SolrNet.Impl.SolrServer< T >.
ResponseHeader SolrNet.ISolrOperations< T >.AddRange | ( | IEnumerable< T > | docs, |
AddParameters | parameters | ||
) |
Adds / updates several documents at once.
- Parameters:
-
docs documents to add/update parameters The add parameters.
- Returns:
Implemented in SolrNet.Impl.SolrServer< T >.
ResponseHeader SolrNet.ISolrOperations< T >.AddRangeWithBoost | ( | IEnumerable< KeyValuePair< T, double?>> | docs, |
AddParameters | parameters | ||
) |
Adds / updates documents with index-time boost and add parameters.
- Parameters:
-
docs List of docs / boost. If boost is null, no boost is applied parameters The add parameters.
- Returns:
Implemented in SolrNet.Impl.SolrServer< T >.
ResponseHeader SolrNet.ISolrOperations< T >.AddRangeWithBoost | ( | IEnumerable< KeyValuePair< T, double?>> | docs ) |
Adds / updates documents with index-time boost.
- Parameters:
-
docs List of docs / boost. If boost is null, no boost is applied
- Returns:
Implemented in SolrNet.Impl.SolrServer< T >.
ResponseHeader SolrNet.ISolrOperations< T >.AddWithBoost | ( | T | doc, |
double | boost | ||
) |
Adds / updates a document with index-time boost.
- Parameters:
-
doc boost
- Returns:
Implemented in SolrNet.Impl.SolrServer< T >.
ResponseHeader SolrNet.ISolrOperations< T >.AddWithBoost | ( | IEnumerable< KeyValuePair< T, double?>> | docs ) |
Adds / updates documents with index-time boost.
- Parameters:
-
docs List of docs / boost. If boost is null, no boost is applied
- Returns:
ResponseHeader SolrNet.ISolrOperations< T >.AddWithBoost | ( | T | doc, |
double | boost, | ||
AddParameters | parameters | ||
) |
Adds / updates a document with index-time boost and add parameters.
- Parameters:
-
doc The document to add/update. boost The index-time boost to apply. parameters The add parameters.
- Returns:
Implemented in SolrNet.Impl.SolrServer< T >.
ResponseHeader SolrNet.ISolrOperations< T >.AddWithBoost | ( | IEnumerable< KeyValuePair< T, double?>> | docs, |
AddParameters | parameters | ||
) |
Adds / updates documents with index-time boost and add parameters.
- Parameters:
-
docs List of docs / boost. If boost is null, no boost is applied parameters The add parameters.
- Returns:
ResponseHeader SolrNet.ISolrOperations< T >.BuildSpellCheckDictionary | ( | ) |
Create the dictionary for use by the SolrSpellChecker. In typical applications, one needs to build the dictionary before using it. However, it may not always be necessary as it is possible to setup the spellchecker with a dictionary that already exists.
summary> Validates the mapping of the type T against the Solr schema XML document. /summary> returns> A collection of ValidationResult objects containing warnings and error found validating the type's mapping against the Solr schema if any.
Implemented in SolrNet.Impl.SolrServer< T >.
ResponseHeader SolrNet.ISolrOperations< T >.Commit | ( | ) |
Commits posted documents, blocking until index changes are flushed to disk and blocking until a new searcher is opened and registered as the main query searcher, making the changes visible.
Implemented in SolrNet.Impl.SolrServer< T >.
ResponseHeader SolrNet.ISolrOperations< T >.Delete | ( | IEnumerable< string > | ids, |
ISolrQuery | q | ||
) |
Deletes all documents that match the given id's or the query.
- Parameters:
-
ids document unique keys q query to match
- Returns:
ResponseHeader SolrNet.ISolrOperations< T >.Delete | ( | IEnumerable< string > | ids ) |
Deletes several documents by their id (unique key)
- Parameters:
-
ids document unique keys
- Returns:
Implemented in SolrNet.Impl.SolrServer< T >.
ResponseHeader SolrNet.ISolrOperations< T >.Delete | ( | T | doc ) |
Deletes a document (requires the document to have a unique key defined with non-null value)
- Parameters:
-
doc document to delete
- Returns:
- Exceptions:
-
SolrNetException throws if document type doesn't have a unique key or document has null unique key
Implemented in SolrNet.Impl.SolrServer< T >.
ResponseHeader SolrNet.ISolrOperations< T >.Delete | ( | IEnumerable< T > | docs ) |
Deletes several documents (requires the document type to have a unique key defined with non-null value)
- Parameters:
-
docs
- Returns:
- Exceptions:
-
SolrNetException throws if document type doesn't have a unique key or document has null unique key
Implemented in SolrNet.Impl.SolrServer< T >.
ResponseHeader SolrNet.ISolrOperations< T >.Delete | ( | ISolrQuery | q ) |
Deletes all documents that match a query.
- Parameters:
-
q query to match
- Returns:
ResponseHeader SolrNet.ISolrOperations< T >.Delete | ( | string | id ) |
Deletes a document by its id (unique key)
- Parameters:
-
id document key
- Returns:
Implemented in SolrNet.Impl.SolrServer< T >.
ExtractResponse SolrNet.ISolrOperations< T >.Extract | ( | ExtractParameters | parameters ) |
Adds / updates the extracted content of a richdocument.
- Parameters:
-
parameters The extracttion parameters
- Returns:
Implemented in SolrNet.Impl.SolrServer< T >.
ResponseHeader SolrNet.ISolrOperations< T >.Optimize | ( | ) |
Optimizes Solr's index.
Implemented in SolrNet.Impl.SolrServer< T >.
ResponseHeader SolrNet.ISolrOperations< T >.Rollback | ( | ) |
Rollbacks all add/deletes made to the index since the last commit.
- Returns:
Implemented in SolrNet.Impl.SolrServer< T >.
The documentation for this interface was generated from the following file:
- SolrNet/ISolrOperations.cs
Generated on Sun May 3 2015 17:19:06 for SolrNet by
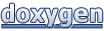