HttpWebAdapters.IHttpWebRequest Interface Reference
Public Member Functions | |
IHttpWebResponse | GetResponse () |
Stream | GetRequestStream () |
Gets a Stream object to use to write request data. | |
void | Abort () |
Cancels a request to an Internet resource. | |
void | AddRange (int from, int to) |
Adds a byte range header to the request for a specified range. | |
void | AddRange (int range) |
Adds a byte range header to a request for a specific range from the beginning or end of the requested data. | |
void | AddRange (string rangeSpecifier, int from, int to) |
Adds a range header to a request for a specified range. | |
void | AddRange (string rangeSpecifier, int range) |
Adds a range header to a request for a specific range from the beginning or end of the requested data. | |
IAsyncResult | BeginGetResponse (AsyncCallback callback, object state) |
IHttpWebResponse | EndGetResponse (IAsyncResult result) |
IAsyncResult | BeginGetRequestStream (AsyncCallback callback, object state) |
Stream | EndGetRequestStream (IAsyncResult result) |
Properties | |
HttpWebRequestMethod | Method [get, set] |
bool | AllowAutoRedirect [get, set] |
Gets or sets a value that indicates whether the request should follow redirection responses. | |
bool | AllowWriteStreamBuffering [get, set] |
Gets or sets a value that indicates whether to buffer the data sent to the Internet resource. | |
bool | HaveResponse [get] |
Gets a value that indicates whether a response has been received from an Internet resource. | |
bool | KeepAlive [get, set] |
Gets or sets a value that indicates whether to make a persistent connection to the Internet resource. | |
bool | Pipelined [get, set] |
Gets or sets a value that indicates whether to pipeline the request to the Internet resource. | |
bool | PreAuthenticate [get, set] |
Gets or sets a value that indicates whether to send an authenticate header with the request. | |
bool | UnsafeAuthenticatedConnectionSharing [get, set] |
Gets or sets a value that indicates whether to allow high-speed NTLM-authenticated connection sharing. | |
bool | SendChunked [get, set] |
Gets or sets a value that indicates whether to send data in segments to the Internet resource. | |
DecompressionMethods | AutomaticDecompression [get, set] |
Gets or sets the type of decompression that is used. | |
int | MaximumResponseHeadersLength [get, set] |
Gets or sets the maximum allowed length of the response headers. | |
X509CertificateCollection | ClientCertificates [get, set] |
Gets or sets the collection of security certificates that are associated with this request. | |
CookieContainer | CookieContainer [get, set] |
Gets or sets the cookies associated with the request. | |
Uri | RequestUri [get] |
Gets the original Uniform Resource Identifier (URI) of the request. | |
long | ContentLength [get, set] |
Gets or sets the Content-length HTTP header. | |
int | Timeout [get, set] |
Gets or sets the time-out value for the M:System.Net.HttpWebRequest.GetResponse and M:System.Net.HttpWebRequest.GetRequestStream methods. | |
int | ReadWriteTimeout [get, set] |
Gets or sets a time-out when writing to or reading from a stream. | |
Uri | Address [get] |
Gets the Uniform Resource Identifier (URI) of the Internet resource that actually responds to the request. | |
ServicePoint | ServicePoint [get] |
Gets the service point to use for the request. | |
int | MaximumAutomaticRedirections [get, set] |
Gets or sets the maximum number of redirects that the request follows. | |
ICredentials | Credentials [get, set] |
Gets or sets authentication information for the request. | |
bool | UseDefaultCredentials [get, set] |
Gets or sets a T:System.Boolean value that controls whether default credentials are sent with requests. | |
string | ConnectionGroupName [get, set] |
Gets or sets the name of the connection group for the request. | |
WebHeaderCollection | Headers [get, set] |
Specifies a collection of the name/value pairs that make up the HTTP headers. | |
IWebProxy | Proxy [get, set] |
Gets or sets proxy information for the request. | |
Version | ProtocolVersion [get, set] |
Gets or sets the version of HTTP to use for the request. | |
string | ContentType [get, set] |
Gets or sets the value of the Content-type HTTP header. | |
string | MediaType [get, set] |
Gets or sets the media type of the request. | |
string | TransferEncoding [get, set] |
Gets or sets the value of the Transfer-encoding HTTP header. | |
string | Connection [get, set] |
Gets or sets the value of the Connection HTTP header. | |
string | Accept [get, set] |
Gets or sets the value of the Accept HTTP header. | |
string | Referer [get, set] |
Gets or sets the value of the Referer HTTP header. | |
string | UserAgent [get, set] |
Gets or sets the value of the User-agent HTTP header. | |
string | Expect [get, set] |
Gets or sets the value of the Expect HTTP header. | |
DateTime | IfModifiedSince [get, set] |
Gets or sets the value of the If-Modified-Since HTTP header. |
Member Function Documentation
void HttpWebAdapters.IHttpWebRequest.Abort | ( | ) |
Cancels a request to an Internet resource.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
void HttpWebAdapters.IHttpWebRequest.AddRange | ( | int | from, |
int | to | ||
) |
Adds a byte range header to the request for a specified range.
- Parameters:
-
to The position at which to stop sending data. from The position at which to start sending data.
- Exceptions:
-
ArgumentException rangeSpecifier is invalid. ArgumentOutOfRangeException from is greater than to-or- from or to is less than 0. InvalidOperationException The range header could not be added.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
void HttpWebAdapters.IHttpWebRequest.AddRange | ( | int | range ) |
Adds a byte range header to a request for a specific range from the beginning or end of the requested data.
- Parameters:
-
range The starting or ending point of the range.
- Exceptions:
-
T:System.ArgumentException rangeSpecifier is invalid. T:System.InvalidOperationException The range header could not be added.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
void HttpWebAdapters.IHttpWebRequest.AddRange | ( | string | rangeSpecifier, |
int | from, | ||
int | to | ||
) |
Adds a range header to a request for a specified range.
- Parameters:
-
from The position at which to start sending data. to The position at which to stop sending data. rangeSpecifier The description of the range.
- Exceptions:
-
T:System.ArgumentException rangeSpecifier is invalid. T:System.ArgumentNullException rangeSpecifier is null. T:System.ArgumentOutOfRangeException from is greater than to-or- from or to is less than 0. T:System.InvalidOperationException The range header could not be added.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
void HttpWebAdapters.IHttpWebRequest.AddRange | ( | string | rangeSpecifier, |
int | range | ||
) |
Adds a range header to a request for a specific range from the beginning or end of the requested data.
- Parameters:
-
range The starting or ending point of the range. rangeSpecifier The description of the range.
- Exceptions:
-
T:System.ArgumentException rangeSpecifier is invalid. T:System.ArgumentNullException rangeSpecifier is null. T:System.InvalidOperationException The range header could not be added.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
Stream HttpWebAdapters.IHttpWebRequest.GetRequestStream | ( | ) |
Gets a Stream object to use to write request data.
- Returns:
- A Stream to use to write request data.
- Exceptions:
-
InvalidOperationException The GetRequestStream method is called more than once.-or- TransferEncoding is set to a value and SendChunked is false. ObjectDisposedException In a .NET Compact Framework application, a request stream with zero content length was not obtained and closed correctly. For more information about handling zero content length requests, see Network Programming in the .NET Compact Framework. WebException Abort was previously called.-or- The time-out period for the request expired.-or- An error occurred while processing the request. NotSupportedException The request cache validator indicated that the response for this request can be served from the cache; however, requests that write data must not use the cache. This exception can occur if you are using a custom cache validator that is incorrectly implemented. ProtocolViolationException The Method property is GET or HEAD.-or- KeepAlive is true, AllowWriteStreamBuffering is false, ContentLength is -1, SendChunked is false, and Method is POST or PUT.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
Property Documentation
string HttpWebAdapters.IHttpWebRequest.Accept [get, set] |
Gets or sets the value of the Accept HTTP header.
- Returns:
- The value of the Accept HTTP header. The default value is null.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
Uri HttpWebAdapters.IHttpWebRequest.Address [get] |
Gets the Uniform Resource Identifier (URI) of the Internet resource that actually responds to the request.
- Returns:
- A T:System.Uri that identifies the Internet resource that actually responds to the request. The default is the URI used by the M:System.Net.WebRequest.Create(System.String) method to initialize the request.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
bool HttpWebAdapters.IHttpWebRequest.AllowAutoRedirect [get, set] |
Gets or sets a value that indicates whether the request should follow redirection responses.
- Returns:
- true if the request should automatically follow redirection responses from the Internet resource; otherwise, false. The default value is true.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
bool HttpWebAdapters.IHttpWebRequest.AllowWriteStreamBuffering [get, set] |
Gets or sets a value that indicates whether to buffer the data sent to the Internet resource.
- Returns:
- true to enable buffering of the data sent to the Internet resource; false to disable buffering. The default is true.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
DecompressionMethods HttpWebAdapters.IHttpWebRequest.AutomaticDecompression [get, set] |
Gets or sets the type of decompression that is used.
- Returns:
- A T:System.Net.DecompressionMethods object that indicates the type of decompression that is used.
- Exceptions:
-
T:System.InvalidOperationException The object's current state does not allow this property to be set.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
X509CertificateCollection HttpWebAdapters.IHttpWebRequest.ClientCertificates [get, set] |
Gets or sets the collection of security certificates that are associated with this request.
- Returns:
- The T:System.Security.Cryptography.X509Certificates.X509CertificateCollection that contains the security certificates associated with this request.
- Exceptions:
-
T:System.ArgumentNullException The value specified for a set operation is null.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
string HttpWebAdapters.IHttpWebRequest.Connection [get, set] |
Gets or sets the value of the Connection HTTP header.
- Returns:
- The value of the Connection HTTP header. The default value is null.
- Exceptions:
-
T:System.ArgumentException The value of P:System.Net.HttpWebRequest.Connection is set to Keep-alive or Close.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
string HttpWebAdapters.IHttpWebRequest.ConnectionGroupName [get, set] |
Gets or sets the name of the connection group for the request.
- Returns:
- The name of the connection group for this request. The default value is null.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
long HttpWebAdapters.IHttpWebRequest.ContentLength [get, set] |
Gets or sets the Content-length HTTP header.
- Returns:
- The number of bytes of data to send to the Internet resource. The default is -1, which indicates the property has not been set and that there is no request data to send.
- Exceptions:
-
T:System.ArgumentOutOfRangeException The new P:System.Net.HttpWebRequest.ContentLength value is less than 0. T:System.InvalidOperationException The request has been started by calling the M:System.Net.HttpWebRequest.GetRequestStream, M:System.Net.HttpWebRequest.BeginGetRequestStream(System.AsyncCallback,System.Object), M:System.Net.HttpWebRequest.GetResponse, or M:System.Net.HttpWebRequest.BeginGetResponse(System.AsyncCallback,System.Object) method.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
string HttpWebAdapters.IHttpWebRequest.ContentType [get, set] |
Gets or sets the value of the Content-type HTTP header.
- Returns:
- The value of the Content-type HTTP header. The default value is null.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
CookieContainer HttpWebAdapters.IHttpWebRequest.CookieContainer [get, set] |
Gets or sets the cookies associated with the request.
- Returns:
- A T:System.Net.CookieContainer that contains the cookies associated with this request.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
ICredentials HttpWebAdapters.IHttpWebRequest.Credentials [get, set] |
Gets or sets authentication information for the request.
- Returns:
- An T:System.Net.ICredentials that contains the authentication credentials associated with the request. The default is null.
<PermissionSet><IPermission class="System.Security.Permissions.SecurityPermission, mscorlib, Version=2.0.3600.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" version="1" flags="UnmanagedCode, ControlEvidence"></PermissionSet>
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
string HttpWebAdapters.IHttpWebRequest.Expect [get, set] |
Gets or sets the value of the Expect HTTP header.
- Returns:
- The contents of the Expect HTTP header. The default value is null.The value for this property is stored in T:System.Net.WebHeaderCollection. If WebHeaderCollection is set, the property value is lost.
- Exceptions:
-
T:System.ArgumentException Expect is set to a string that contains "100-continue" as a substring.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
bool HttpWebAdapters.IHttpWebRequest.HaveResponse [get] |
Gets a value that indicates whether a response has been received from an Internet resource.
- Returns:
- true if a response has been received; otherwise, false.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
WebHeaderCollection HttpWebAdapters.IHttpWebRequest.Headers [get, set] |
Specifies a collection of the name/value pairs that make up the HTTP headers.
- Returns:
- A T:System.Net.WebHeaderCollection that contains the name/value pairs that make up the headers for the HTTP request.
- Exceptions:
-
T:System.InvalidOperationException The request has been started by calling the M:System.Net.HttpWebRequest.GetRequestStream, M:System.Net.HttpWebRequest.BeginGetRequestStream(System.AsyncCallback,System.Object), M:System.Net.HttpWebRequest.GetResponse, or M:System.Net.HttpWebRequest.BeginGetResponse(System.AsyncCallback,System.Object) method.
<PermissionSet><IPermission class="System.Security.Permissions.SecurityPermission, mscorlib, Version=2.0.3600.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" version="1" flags="UnmanagedCode, ControlEvidence"></PermissionSet>
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
DateTime HttpWebAdapters.IHttpWebRequest.IfModifiedSince [get, set] |
Gets or sets the value of the If-Modified-Since HTTP header.
- Returns:
- A T:System.DateTime that contains the contents of the If-Modified-Since HTTP header. The default value is the current date and time.
<PermissionSet><IPermission class="System.Security.Permissions.SecurityPermission, mscorlib, Version=2.0.3600.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" version="1" flags="UnmanagedCode, ControlEvidence"></PermissionSet>
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
bool HttpWebAdapters.IHttpWebRequest.KeepAlive [get, set] |
Gets or sets a value that indicates whether to make a persistent connection to the Internet resource.
- Returns:
- true if the request to the Internet resource should contain a Connection HTTP header with the value Keep-alive; otherwise, false. The default is true.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
int HttpWebAdapters.IHttpWebRequest.MaximumAutomaticRedirections [get, set] |
Gets or sets the maximum number of redirects that the request follows.
- Returns:
- The maximum number of redirection responses that the request follows. The default value is 50.
- Exceptions:
-
T:System.ArgumentException The value is set to 0 or less.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
int HttpWebAdapters.IHttpWebRequest.MaximumResponseHeadersLength [get, set] |
Gets or sets the maximum allowed length of the response headers.
- Returns:
- The length, in kilobytes (1024 bytes), of the response headers.
- Exceptions:
-
T:System.InvalidOperationException The property is set after the request has already been submitted. T:System.ArgumentOutOfRangeException The value is less than 0 and is not equal to -1.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
string HttpWebAdapters.IHttpWebRequest.MediaType [get, set] |
Gets or sets the media type of the request.
- Returns:
- The media type of the request. The default value is null.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
bool HttpWebAdapters.IHttpWebRequest.Pipelined [get, set] |
Gets or sets a value that indicates whether to pipeline the request to the Internet resource.
- Returns:
- true if the request should be pipelined; otherwise, false. The default is true.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
bool HttpWebAdapters.IHttpWebRequest.PreAuthenticate [get, set] |
Gets or sets a value that indicates whether to send an authenticate header with the request.
- Returns:
- true to send a WWW-authenticate HTTP header with requests after authentication has taken place; otherwise, false. The default is false.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
Version HttpWebAdapters.IHttpWebRequest.ProtocolVersion [get, set] |
Gets or sets the version of HTTP to use for the request.
- Returns:
- The HTTP version to use for the request. The default is F:System.Net.HttpVersion.Version11.
- Exceptions:
-
T:System.ArgumentException The HTTP version is set to a value other than 1.0 or 1.1.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
IWebProxy HttpWebAdapters.IHttpWebRequest.Proxy [get, set] |
Gets or sets proxy information for the request.
- Returns:
- The T:System.Net.IWebProxy object to use to proxy the request. The default value is set by calling the P:System.Net.GlobalProxySelection.Select property.
- Exceptions:
-
T:System.Security.SecurityException The caller does not have permission for the requested operation. T:System.ArgumentNullException P:System.Net.HttpWebRequest.Proxy is set to null. T:System.InvalidOperationException The request has been started by calling M:System.Net.HttpWebRequest.GetRequestStream, M:System.Net.HttpWebRequest.BeginGetRequestStream(System.AsyncCallback,System.Object), M:System.Net.HttpWebRequest.GetResponse, or M:System.Net.HttpWebRequest.BeginGetResponse(System.AsyncCallback,System.Object).
<PermissionSet><IPermission class="System.Security.Permissions.EnvironmentPermission, mscorlib, Version=2.0.3600.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" version="1" unrestricted="true"><IPermission class="System.Security.Permissions.FileIOPermission, mscorlib, Version=2.0.3600.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" version="1" unrestricted="true"><IPermission class="System.Security.Permissions.SecurityPermission, mscorlib, Version=2.0.3600.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" version="1" flags="UnmanagedCode, ControlEvidence"><IPermission class="System.Net.WebPermission, System, Version=2.0.3600.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" version="1" unrestricted="true"></PermissionSet>
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
int HttpWebAdapters.IHttpWebRequest.ReadWriteTimeout [get, set] |
Gets or sets a time-out when writing to or reading from a stream.
- Returns:
- The number of milliseconds before the writing or reading times out. The default value is 300,000 milliseconds (5 minutes).
- Exceptions:
-
T:System.ArgumentOutOfRangeException The value specified for a set operation is less than or equal to zero and is not equal to F:System.Threading.Timeout.Infinite T:System.InvalidOperationException The request has already been sent.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
string HttpWebAdapters.IHttpWebRequest.Referer [get, set] |
Gets or sets the value of the Referer HTTP header.
- Returns:
- The value of the Referer HTTP header. The default value is null.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
Uri HttpWebAdapters.IHttpWebRequest.RequestUri [get] |
Gets the original Uniform Resource Identifier (URI) of the request.
- Returns:
- A T:System.Uri that contains the URI of the Internet resource passed to the M:System.Net.WebRequest.Create(System.String) method.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
bool HttpWebAdapters.IHttpWebRequest.SendChunked [get, set] |
Gets or sets a value that indicates whether to send data in segments to the Internet resource.
- Returns:
- true to send data to the Internet resource in segments; otherwise, false. The default value is false.
- Exceptions:
-
T:System.InvalidOperationException The request has been started by calling the M:System.Net.HttpWebRequest.GetRequestStream, M:System.Net.HttpWebRequest.BeginGetRequestStream(System.AsyncCallback,System.Object), M:System.Net.HttpWebRequest.GetResponse, or M:System.Net.HttpWebRequest.BeginGetResponse(System.AsyncCallback,System.Object) method.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
ServicePoint HttpWebAdapters.IHttpWebRequest.ServicePoint [get] |
Gets the service point to use for the request.
- Returns:
- A T:System.Net.ServicePoint that represents the network connection to the Internet resource.
<PermissionSet><IPermission class="System.Security.Permissions.EnvironmentPermission, mscorlib, Version=2.0.3600.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" version="1" unrestricted="true"><IPermission class="System.Security.Permissions.FileIOPermission, mscorlib, Version=2.0.3600.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" version="1" unrestricted="true"><IPermission class="System.Security.Permissions.SecurityPermission, mscorlib, Version=2.0.3600.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" version="1" flags="UnmanagedCode, ControlEvidence"></PermissionSet>
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
int HttpWebAdapters.IHttpWebRequest.Timeout [get, set] |
Gets or sets the time-out value for the M:System.Net.HttpWebRequest.GetResponse and M:System.Net.HttpWebRequest.GetRequestStream methods.
- Returns:
- The number of milliseconds to wait before the request times out. The default is 100,000 milliseconds (100 seconds).
- Exceptions:
-
T:System.ArgumentOutOfRangeException The value specified is less than zero and is not F:System.Threading.Timeout.Infinite.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
string HttpWebAdapters.IHttpWebRequest.TransferEncoding [get, set] |
Gets or sets the value of the Transfer-encoding HTTP header.
- Returns:
- The value of the Transfer-encoding HTTP header. The default value is null.
- Exceptions:
-
T:System.InvalidOperationException P:System.Net.HttpWebRequest.TransferEncoding is set when P:System.Net.HttpWebRequest.SendChunked is false. T:System.ArgumentException P:System.Net.HttpWebRequest.TransferEncoding is set to the value "Chunked".
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
bool HttpWebAdapters.IHttpWebRequest.UnsafeAuthenticatedConnectionSharing [get, set] |
Gets or sets a value that indicates whether to allow high-speed NTLM-authenticated connection sharing.
- Returns:
- true to keep the authenticated connection open; otherwise, false.
<PermissionSet><IPermission class="System.Net.WebPermission, System, Version=2.0.3600.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" version="1" unrestricted="true"></PermissionSet>
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
bool HttpWebAdapters.IHttpWebRequest.UseDefaultCredentials [get, set] |
Gets or sets a T:System.Boolean value that controls whether default credentials are sent with requests.
- Returns:
- true if the default credentials are used; otherwise false. The default value is false.
- Exceptions:
-
T:System.InvalidOperationException You attempted to set this property after the request was sent.
<PermissionSet><IPermission class="System.Security.Permissions.EnvironmentPermission, mscorlib, Version=2.0.3600.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" version="1" read="USERNAME"></PermissionSet>
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
string HttpWebAdapters.IHttpWebRequest.UserAgent [get, set] |
Gets or sets the value of the User-agent HTTP header.
- Returns:
- The value of the User-agent HTTP header. The default value is null.The value for this property is stored in T:System.Net.WebHeaderCollection. If WebHeaderCollection is set, the property value is lost.
Implemented in HttpWebAdapters.Adapters.HttpWebRequestAdapter.
The documentation for this interface was generated from the following file:
- HttpWebAdapters/IHttpWebRequest.cs
Generated on Sun May 3 2015 17:19:05 for SolrNet by
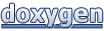