SolrNet.Impl.SolrServer< T > Class Template Reference
Main component to interact with Solr. More...
Public Member Functions | |
SolrServer (ISolrBasicOperations< T > basicServer, IReadOnlyMappingManager mappingManager, IMappingValidator _schemaMappingValidator) | |
SolrQueryResults< T > | Query (ISolrQuery query, QueryOptions options) |
Executes a query. | |
ResponseHeader | Ping () |
Pings the Solr server. It can be used by a load balancer in front of a set of Solr servers to check response time of all the Solr servers in order to do response time based load balancing. See http://wiki.apache.org/solr/SolrConfigXml for more information. | |
SolrQueryResults< T > | Query (string q) |
Executes a query. | |
SolrQueryResults< T > | Query (string q, ICollection< SortOrder > orders) |
Executes a query. | |
SolrQueryResults< T > | Query (string q, QueryOptions options) |
Executes a query. | |
SolrQueryResults< T > | Query (ISolrQuery q) |
Executes a query. | |
SolrQueryResults< T > | Query (ISolrQuery query, ICollection< SortOrder > orders) |
Executes a query. | |
ICollection< KeyValuePair < string, int > > | FacetFieldQuery (SolrFacetFieldQuery facet) |
Executes a facet field query only. | |
ResponseHeader | BuildSpellCheckDictionary () |
Create the dictionary for use by the SolrSpellChecker. In typical applications, one needs to build the dictionary before using it. However, it may not always be necessary as it is possible to setup the spellchecker with a dictionary that already exists. | |
ResponseHeader | AddWithBoost (T doc, double boost) |
Adds / updates a document with index-time boost. | |
ResponseHeader | AddWithBoost (T doc, double boost, AddParameters parameters) |
Adds / updates a document with index-time boost and add parameters. | |
ExtractResponse | Extract (ExtractParameters parameters) |
Adds / updates the extracted content of a richdocument. | |
ResponseHeader | Add (IEnumerable< T > docs) |
Adds / updates several documents at once. | |
ResponseHeader | AddRange (IEnumerable< T > docs) |
Adds / updates several documents at once. | |
ResponseHeader | Add (IEnumerable< T > docs, AddParameters parameters) |
Adds / updates several documents at once. | |
ResponseHeader | AddRange (IEnumerable< T > docs, AddParameters parameters) |
Adds / updates several documents at once. | |
ResponseHeader | AddRangeWithBoost (IEnumerable< KeyValuePair< T, double?>> docs) |
Adds / updates documents with index-time boost. | |
ResponseHeader | AddRangeWithBoost (IEnumerable< KeyValuePair< T, double?>> docs, AddParameters parameters) |
Adds / updates documents with index-time boost and add parameters. | |
ResponseHeader | Delete (IEnumerable< string > ids) |
Deletes several documents by their id (unique key) | |
ResponseHeader | Delete (T doc) |
Deletes a document (requires the document to have a unique key defined with non-null value) | |
ResponseHeader | Delete (IEnumerable< T > docs) |
Deletes several documents (requires the document type to have a unique key defined with non-null value) | |
ResponseHeader | Delete (string id) |
Deletes a document by its id (unique key) | |
ResponseHeader | Commit () |
Commits posted documents, blocking until index changes are flushed to disk and blocking until a new searcher is opened and registered as the main query searcher, making the changes visible. | |
ResponseHeader | Rollback () |
Rollbacks all add/deletes made to the index since the last commit. | |
ResponseHeader | Optimize () |
Commits posts, blocking until index changes are flushed to disk and blocking until a new searcher is opened and registered as the main query searcher, making the changes visible. | |
ResponseHeader | Add (T doc) |
Adds / updates a document. | |
ResponseHeader | Add (T doc, AddParameters parameters) |
Adds / updates a document with parameters. | |
SolrSchema | GetSchema () |
Gets the schema. | |
IEnumerable< ValidationResult > | EnumerateValidationResults () |
SolrDIHStatus | GetDIHStatus (KeyValuePair< string, string > options) |
Gets the DIH Status. | |
SolrMoreLikeThisHandlerResults< T > | MoreLikeThis (SolrMLTQuery query, MoreLikeThisHandlerQueryOptions options) |
Executes a MoreLikeThisHandler query. |
Detailed Description
template<T>
class SolrNet::Impl::SolrServer< T >
Main component to interact with Solr.
- Template Parameters:
-
T Document type
Member Function Documentation
ResponseHeader SolrNet.Impl.SolrServer< T >.Add | ( | IEnumerable< T > | docs ) |
Adds / updates several documents at once.
- Parameters:
-
docs documents to add/update
- Returns:
Implements SolrNet.ISolrOperations< T >.
ResponseHeader SolrNet.Impl.SolrServer< T >.Add | ( | IEnumerable< T > | docs, |
AddParameters | parameters | ||
) |
Adds / updates several documents at once.
- Parameters:
-
docs documents to add/update parameters The add parameters.
- Returns:
Implements SolrNet.ISolrOperations< T >.
ResponseHeader SolrNet.Impl.SolrServer< T >.Add | ( | T | doc ) |
Adds / updates a document.
- Parameters:
-
doc document to add/update
- Returns:
Implements SolrNet.ISolrOperations< T >.
ResponseHeader SolrNet.Impl.SolrServer< T >.Add | ( | T | doc, |
AddParameters | parameters | ||
) |
Adds / updates a document with parameters.
- Parameters:
-
doc The document to add/update. parameters The add parameters.
- Returns:
Implements SolrNet.ISolrOperations< T >.
ResponseHeader SolrNet.Impl.SolrServer< T >.AddRange | ( | IEnumerable< T > | docs, |
AddParameters | parameters | ||
) |
Adds / updates several documents at once.
- Parameters:
-
docs documents to add/update parameters The add parameters.
- Returns:
Implements SolrNet.ISolrOperations< T >.
ResponseHeader SolrNet.Impl.SolrServer< T >.AddRange | ( | IEnumerable< T > | docs ) |
Adds / updates several documents at once.
- Parameters:
-
docs documents to add/update
- Returns:
Implements SolrNet.ISolrOperations< T >.
ResponseHeader SolrNet.Impl.SolrServer< T >.AddRangeWithBoost | ( | IEnumerable< KeyValuePair< T, double?>> | docs ) |
Adds / updates documents with index-time boost.
- Parameters:
-
docs List of docs / boost. If boost is null, no boost is applied
- Returns:
Implements SolrNet.ISolrOperations< T >.
ResponseHeader SolrNet.Impl.SolrServer< T >.AddRangeWithBoost | ( | IEnumerable< KeyValuePair< T, double?>> | docs, |
AddParameters | parameters | ||
) |
Adds / updates documents with index-time boost and add parameters.
- Parameters:
-
docs List of docs / boost. If boost is null, no boost is applied parameters The add parameters.
- Returns:
Implements SolrNet.ISolrOperations< T >.
ResponseHeader SolrNet.Impl.SolrServer< T >.AddWithBoost | ( | T | doc, |
double | boost | ||
) |
Adds / updates a document with index-time boost.
- Parameters:
-
doc boost
- Returns:
Implements SolrNet.ISolrOperations< T >.
ResponseHeader SolrNet.Impl.SolrServer< T >.AddWithBoost | ( | T | doc, |
double | boost, | ||
AddParameters | parameters | ||
) |
Adds / updates a document with index-time boost and add parameters.
- Parameters:
-
doc The document to add/update. boost The index-time boost to apply. parameters The add parameters.
- Returns:
Implements SolrNet.ISolrOperations< T >.
ResponseHeader SolrNet.Impl.SolrServer< T >.BuildSpellCheckDictionary | ( | ) |
Create the dictionary for use by the SolrSpellChecker. In typical applications, one needs to build the dictionary before using it. However, it may not always be necessary as it is possible to setup the spellchecker with a dictionary that already exists.
summary> Validates the mapping of the type T against the Solr schema XML document. /summary> returns> A collection of ValidationResult objects containing warnings and error found validating the type's mapping against the Solr schema if any.
Implements SolrNet.ISolrOperations< T >.
ResponseHeader SolrNet.Impl.SolrServer< T >.Commit | ( | ) |
Commits posted documents, blocking until index changes are flushed to disk and blocking until a new searcher is opened and registered as the main query searcher, making the changes visible.
Implements SolrNet.ISolrOperations< T >.
ResponseHeader SolrNet.Impl.SolrServer< T >.Delete | ( | string | id ) |
Deletes a document by its id (unique key)
- Parameters:
-
id document key
- Returns:
Implements SolrNet.ISolrOperations< T >.
ResponseHeader SolrNet.Impl.SolrServer< T >.Delete | ( | IEnumerable< string > | ids ) |
Deletes several documents by their id (unique key)
- Parameters:
-
ids document unique keys
- Returns:
Implements SolrNet.ISolrOperations< T >.
ResponseHeader SolrNet.Impl.SolrServer< T >.Delete | ( | T | doc ) |
Deletes a document (requires the document to have a unique key defined with non-null value)
- Parameters:
-
doc document to delete
- Returns:
- Exceptions:
-
SolrNetException throws if document type doesn't have a unique key or document has null unique key
Implements SolrNet.ISolrOperations< T >.
ResponseHeader SolrNet.Impl.SolrServer< T >.Delete | ( | IEnumerable< T > | docs ) |
Deletes several documents (requires the document type to have a unique key defined with non-null value)
- Parameters:
-
docs
- Returns:
- Exceptions:
-
SolrNetException throws if document type doesn't have a unique key or document has null unique key
Implements SolrNet.ISolrOperations< T >.
ExtractResponse SolrNet.Impl.SolrServer< T >.Extract | ( | ExtractParameters | parameters ) |
Adds / updates the extracted content of a richdocument.
- Parameters:
-
parameters The extracttion parameters
- Returns:
Implements SolrNet.ISolrOperations< T >.
ICollection<KeyValuePair<string, int> > SolrNet.Impl.SolrServer< T >.FacetFieldQuery | ( | SolrFacetFieldQuery | facet ) |
Executes a facet field query only.
- Parameters:
-
facet
- Returns:
Implements SolrNet.ISolrReadOnlyOperations< T >.
SolrDIHStatus SolrNet.Impl.SolrServer< T >.GetDIHStatus | ( | KeyValuePair< string, string > | options ) |
Gets the DIH Status.
- Parameters:
-
options command options
- Returns:
- A XmlDocument containing the DIH Status XML.
Implements SolrNet.ISolrBasicReadOnlyOperations< T >.
SolrSchema SolrNet.Impl.SolrServer< T >.GetSchema | ( | ) |
SolrMoreLikeThisHandlerResults<T> SolrNet.Impl.SolrServer< T >.MoreLikeThis | ( | SolrMLTQuery | query, |
MoreLikeThisHandlerQueryOptions | options | ||
) |
Executes a MoreLikeThisHandler query.
- Parameters:
-
query options
- Returns:
Implements SolrNet.ISolrBasicReadOnlyOperations< T >.
ResponseHeader SolrNet.Impl.SolrServer< T >.Optimize | ( | ) |
Commits posts, blocking until index changes are flushed to disk and blocking until a new searcher is opened and registered as the main query searcher, making the changes visible.
Implements SolrNet.ISolrOperations< T >.
ResponseHeader SolrNet.Impl.SolrServer< T >.Ping | ( | ) |
Pings the Solr server. It can be used by a load balancer in front of a set of Solr servers to check response time of all the Solr servers in order to do response time based load balancing. See http://wiki.apache.org/solr/SolrConfigXml for more information.
Implements SolrNet.ISolrBasicReadOnlyOperations< T >.
SolrQueryResults<T> SolrNet.Impl.SolrServer< T >.Query | ( | ISolrQuery | query, |
QueryOptions | options | ||
) |
Executes a query.
- Parameters:
-
query options
- Returns:
Implements SolrNet.ISolrBasicReadOnlyOperations< T >.
SolrQueryResults<T> SolrNet.Impl.SolrServer< T >.Query | ( | ISolrQuery | q ) |
SolrQueryResults<T> SolrNet.Impl.SolrServer< T >.Query | ( | string | q ) |
Executes a query.
- Parameters:
-
q query to execute
- Returns:
- query results
Implements SolrNet.ISolrReadOnlyOperations< T >.
SolrQueryResults<T> SolrNet.Impl.SolrServer< T >.Query | ( | string | q, |
QueryOptions | options | ||
) |
SolrQueryResults<T> SolrNet.Impl.SolrServer< T >.Query | ( | ISolrQuery | query, |
ICollection< SortOrder > | orders | ||
) |
Executes a query.
- Parameters:
-
query orders
- Returns:
Implements SolrNet.ISolrReadOnlyOperations< T >.
SolrQueryResults<T> SolrNet.Impl.SolrServer< T >.Query | ( | string | q, |
ICollection< SortOrder > | orders | ||
) |
ResponseHeader SolrNet.Impl.SolrServer< T >.Rollback | ( | ) |
Rollbacks all add/deletes made to the index since the last commit.
- Returns:
Implements SolrNet.ISolrOperations< T >.
The documentation for this class was generated from the following file:
- SolrNet/Impl/SolrServer.cs
Generated on Sun May 3 2015 17:19:09 for SolrNet by
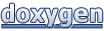