STM32L476G-Discovery BSP User Manual
|
stm32l476g_discovery_glass_lcd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l476g_discovery_glass_lcd.c 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief This file provides a set of functions needed to manage the 00008 * LCD Glass driver for the STM32L476G-Discovery board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Includes ------------------------------------------------------------------*/ 00040 #include "stm32l476g_discovery_glass_lcd.h" 00041 00042 /** @addtogroup BSP 00043 * @{ 00044 */ 00045 00046 /** @addtogroup STM32L476G_DISCOVERY 00047 * @{ 00048 */ 00049 00050 /** @defgroup STM32L476G_DISCOVERY_GLASS_LCD STM32L476G-DISCOVERY GLASS LCD 00051 * @brief This file includes the LCD Glass driver for LCD Module of 00052 * STM32L476G-DISCOVERY board. 00053 * @{ 00054 */ 00055 00056 /* Private constants ---------------------------------------------------------*/ 00057 00058 /** @defgroup STM32L476G_DISCOVERY_GLASS_LCD_Private_Constants Private Constants 00059 * @{ 00060 */ 00061 #define ASCII_CHAR_0 0x30 /* 0 */ 00062 #define ASCII_CHAR_AT_SYMBOL 0x40 /* @ */ 00063 #define ASCII_CHAR_LEFT_OPEN_BRACKET 0x5B /* [ */ 00064 #define ASCII_CHAR_APOSTROPHE 0x60 /* ` */ 00065 #define ASCII_CHAR_LEFT_OPEN_BRACE 0x7B /* ( */ 00066 /** 00067 * @} 00068 */ 00069 00070 /* Private variables ---------------------------------------------------------*/ 00071 00072 /** @defgroup STM32L476G_DISCOVERY_GLASS_LCD_Private_Variables Private Variables 00073 * @{ 00074 */ 00075 00076 /* this variable can be used for accelerate the scrolling exit when push user button */ 00077 __IO uint8_t bLCDGlass_KeyPressed = 0; 00078 00079 /** 00080 @verbatim 00081 ================================================================================ 00082 GLASS LCD MAPPING 00083 ================================================================================ 00084 LCD allows to display informations on six 14-segment digits and 4 bars: 00085 00086 1 2 3 4 5 6 00087 ----- ----- ----- ----- ----- ----- 00088 |\|/| o |\|/| o |\|/| o |\|/| o |\|/| |\|/| BAR3 00089 -- -- -- -- -- -- -- -- -- -- -- -- BAR2 00090 |/|\| o |/|\| o |/|\| o |/|\| o |/|\| |/|\| BAR1 00091 ----- * ----- * ----- * ----- * ----- ----- BAR0 00092 00093 LCD segment mapping: 00094 -------------------- 00095 -----A----- _ 00096 |\ | /| COL |_| 00097 F H J K B 00098 | \ | / | _ 00099 --G-- --M-- COL |_| 00100 | / | \ | 00101 E Q P N C 00102 |/ | \| _ 00103 -----D----- DP |_| 00104 00105 An LCD character coding is based on the following matrix: 00106 COM 0 1 2 3 00107 SEG(n) { E , D , P , N } 00108 SEG(n+1) { M , C , COL , DP } 00109 SEG(23-n-1) { B , A , K , J } 00110 SEG(23-n) { G , F , Q , H } 00111 with n positive odd number. 00112 00113 The character 'A' for example is: 00114 ------------------------------- 00115 LSB { 1 , 0 , 0 , 0 } 00116 { 1 , 1 , 0 , 0 } 00117 { 1 , 1 , 0 , 0 } 00118 MSB { 1 , 1 , 0 , 0 } 00119 ------------------- 00120 'A' = F E 0 0 hexa 00121 00122 @endverbatim 00123 */ 00124 00125 LCD_HandleTypeDef LCDHandle; 00126 00127 /* Constant table for cap characters 'A' --> 'Z' */ 00128 const uint16_t CapLetterMap[26]= 00129 { 00130 /* A B C D E F G H I */ 00131 0xFE00, 0x6714, 0x1D00, 0x4714, 0x9D00, 0x9C00, 0x3F00, 0xFA00, 0x0014, 00132 /* J K L M N O P Q R */ 00133 0x5300, 0x9841, 0x1900, 0x5A48, 0x5A09, 0x5F00, 0xFC00, 0x5F01, 0xFC01, 00134 /* S T U V W X Y Z */ 00135 0xAF00, 0x0414, 0x5b00, 0x18C0, 0x5A81, 0x00C9, 0x0058, 0x05C0 00136 }; 00137 00138 /* Constant table for number '0' --> '9' */ 00139 const uint16_t NumberMap[10]= 00140 { 00141 /* 0 1 2 3 4 5 6 7 8 9 */ 00142 0x5F00,0x4200,0xF500,0x6700,0xEa00,0xAF00,0xBF00,0x04600,0xFF00,0xEF00 00143 }; 00144 00145 uint32_t Digit[4]; /* Digit frame buffer */ 00146 00147 /* LCD BAR status: To save the bar setting after writing in LCD RAM memory */ 00148 uint8_t LCDBar = BATTERYLEVEL_FULL; 00149 00150 /** 00151 * @} 00152 */ 00153 00154 /** @defgroup STM32L476G_DISCOVERY_LCD_Private_Functions Private Functions 00155 * @{ 00156 */ 00157 static void Convert(uint8_t* Char, Point_Typedef Point, DoublePoint_Typedef Colon); 00158 static void WriteChar(uint8_t* ch, Point_Typedef Point, DoublePoint_Typedef Colon, DigitPosition_Typedef Position); 00159 static void LCD_MspInit(LCD_HandleTypeDef *hlcd); 00160 static void LCD_MspDeInit(LCD_HandleTypeDef *hlcd); 00161 00162 /** 00163 * @} 00164 */ 00165 00166 /** @addtogroup STM32L476G_DISCOVERY_LCD_Exported_Functions 00167 * @{ 00168 */ 00169 00170 /** 00171 * @brief Initialize the LCD GLASS relative GPIO port IOs and LCD peripheral. 00172 * @retval None 00173 */ 00174 void BSP_LCD_GLASS_Init(void) 00175 { 00176 LCDHandle.Instance = LCD; 00177 LCDHandle.Init.Prescaler = LCD_PRESCALER_1; 00178 LCDHandle.Init.Divider = LCD_DIVIDER_31; 00179 #if defined (USE_STM32L476G_DISCO_REVC) || defined (USE_STM32L476G_DISCO_REVB) 00180 LCDHandle.Init.Duty = LCD_DUTY_1_4; 00181 #elif defined (USE_STM32L476G_DISCO_REVA) 00182 LCDHandle.Init.Duty = LCD_DUTY_1_8; 00183 #endif 00184 LCDHandle.Init.Bias = LCD_BIAS_1_3; 00185 LCDHandle.Init.VoltageSource = LCD_VOLTAGESOURCE_INTERNAL; 00186 LCDHandle.Init.Contrast = LCD_CONTRASTLEVEL_5; 00187 LCDHandle.Init.DeadTime = LCD_DEADTIME_0; 00188 LCDHandle.Init.PulseOnDuration = LCD_PULSEONDURATION_4; 00189 LCDHandle.Init.HighDrive = LCD_HIGHDRIVE_DISABLE; 00190 LCDHandle.Init.BlinkMode = LCD_BLINKMODE_OFF; 00191 LCDHandle.Init.BlinkFrequency = LCD_BLINKFREQUENCY_DIV32; 00192 LCDHandle.Init.MuxSegment = LCD_MUXSEGMENT_DISABLE; 00193 00194 /* Initialize the LCD */ 00195 LCD_MspInit(&LCDHandle); 00196 HAL_LCD_Init(&LCDHandle); 00197 00198 BSP_LCD_GLASS_Clear(); 00199 } 00200 00201 /** 00202 * @brief DeInitialize the LCD GLASS relative GPIO port IOs and LCD peripheral. 00203 * @retval None 00204 */ 00205 void BSP_LCD_GLASS_DeInit(void) 00206 { 00207 /* De-Initialize the LCD */ 00208 LCD_MspDeInit(&LCDHandle); 00209 HAL_LCD_DeInit(&LCDHandle); 00210 } 00211 00212 00213 /** 00214 * @brief Configure the LCD Blink mode and Blink frequency. 00215 * @param BlinkMode: specifies the LCD blink mode. 00216 * This parameter can be one of the following values: 00217 * @arg LCD_BLINKMODE_OFF: Blink disabled 00218 * @arg LCD_BLINKMODE_SEG0_COM0: Blink enabled on SEG[0], COM[0] (1 pixel) 00219 * @arg LCD_BLINKMODE_SEG0_ALLCOM: Blink enabled on SEG[0], all COM (up to 8 00220 * pixels according to the programmed duty) 00221 * @arg LCD_BLINKMODE_ALLSEG_ALLCOM: Blink enabled on all SEG and all COM 00222 * (all pixels) 00223 * @param BlinkFrequency: specifies the LCD blink frequency. 00224 * @arg LCD_BLINKFREQUENCY_DIV8: The Blink frequency = fLcd/8 00225 * @arg LCD_BLINKFREQUENCY_DIV16: The Blink frequency = fLcd/16 00226 * @arg LCD_BLINKFREQUENCY_DIV32: The Blink frequency = fLcd/32 00227 * @arg LCD_BLINKFREQUENCY_DIV64: The Blink frequency = fLcd/64 00228 * @arg LCD_BLINKFREQUENCY_DIV128: The Blink frequency = fLcd/128 00229 * @arg LCD_BLINKFREQUENCY_DIV256: The Blink frequency = fLcd/256 00230 * @arg LCD_BLINKFREQUENCY_DIV512: The Blink frequency = fLcd/512 00231 * @arg LCD_BLINKFREQUENCY_DIV1024: The Blink frequency = fLcd/1024 00232 * @retval None 00233 */ 00234 void BSP_LCD_GLASS_BlinkConfig(uint32_t BlinkMode, uint32_t BlinkFrequency) 00235 { 00236 __HAL_LCD_BLINK_CONFIG(&LCDHandle, BlinkMode, BlinkFrequency); 00237 } 00238 00239 /** 00240 * @brief Configure the LCD contrast. 00241 * @param Contrast: specifies the LCD contrast value. 00242 * This parameter can be one of the following values: 00243 * @arg LCD_CONTRASTLEVEL_0: Maximum Voltage = 2.60V 00244 * @arg LCD_CONTRASTLEVEL_1: Maximum Voltage = 2.73V 00245 * @arg LCD_CONTRASTLEVEL_2: Maximum Voltage = 2.86V 00246 * @arg LCD_CONTRASTLEVEL_3: Maximum Voltage = 2.99V 00247 * @arg LCD_CONTRASTLEVEL_4: Maximum Voltage = 3.12V 00248 * @arg LCD_CONTRASTLEVEL_5: Maximum Voltage = 3.25V 00249 * @arg LCD_CONTRASTLEVEL_6: Maximum Voltage = 3.38V 00250 * @arg LCD_CONTRASTLEVEL_7: Maximum Voltage = 3.51V 00251 * @retval None 00252 */ 00253 void BSP_LCD_GLASS_Contrast(uint32_t Contrast) 00254 { 00255 __HAL_LCD_CONTRAST_CONFIG(&LCDHandle, Contrast); 00256 } 00257 00258 /** 00259 * @brief Display one or several bar in LCD frame buffer. 00260 * @param BarId: specifies the LCD GLASS Bar to display 00261 * This parameter can be one of the following values: 00262 * @arg BAR0: LCD GLASS Bar 0 00263 * @arg BAR0: LCD GLASS Bar 1 00264 * @arg BAR0: LCD GLASS Bar 2 00265 * @arg BAR0: LCD GLASS Bar 3 00266 * @retval None 00267 */ 00268 void BSP_LCD_GLASS_DisplayBar(uint32_t BarId) 00269 { 00270 uint32_t position = 0; 00271 00272 /* Check which bar is selected */ 00273 while ((BarId) >> position) 00274 { 00275 /* Check if current bar is selected */ 00276 switch(BarId & (1 << position)) 00277 { 00278 /* Bar 0 */ 00279 case LCD_BAR_0: 00280 /* Set BAR0 */ 00281 HAL_LCD_Write(&LCDHandle, LCD_BAR0_2_COM, ~(LCD_BAR0_SEG), LCD_BAR0_SEG); 00282 break; 00283 00284 /* Bar 1 */ 00285 case LCD_BAR_1: 00286 /* Set BAR1 */ 00287 HAL_LCD_Write(&LCDHandle, LCD_BAR1_3_COM, ~(LCD_BAR1_SEG), LCD_BAR1_SEG); 00288 break; 00289 00290 /* Bar 2 */ 00291 case LCD_BAR_2: 00292 /* Set BAR2 */ 00293 HAL_LCD_Write(&LCDHandle, LCD_BAR0_2_COM, ~(LCD_BAR2_SEG), LCD_BAR2_SEG); 00294 break; 00295 00296 /* Bar 3 */ 00297 case LCD_BAR_3: 00298 /* Set BAR3 */ 00299 HAL_LCD_Write(&LCDHandle, LCD_BAR1_3_COM, ~(LCD_BAR3_SEG), LCD_BAR3_SEG); 00300 break; 00301 00302 default: 00303 break; 00304 } 00305 position++; 00306 } 00307 00308 /* Update the LCD display */ 00309 HAL_LCD_UpdateDisplayRequest(&LCDHandle); 00310 } 00311 00312 /** 00313 * @brief Clear one or several bar in LCD frame buffer. 00314 * @param BarId: specifies the LCD GLASS Bar to display 00315 * This parameter can be combination of one of the following values: 00316 * @arg LCD_BAR_0: LCD GLASS Bar 0 00317 * @arg LCD_BAR_1: LCD GLASS Bar 1 00318 * @arg LCD_BAR_2: LCD GLASS Bar 2 00319 * @arg LCD_BAR_3: LCD GLASS Bar 3 00320 * @retval None 00321 */ 00322 void BSP_LCD_GLASS_ClearBar(uint32_t BarId) 00323 { 00324 uint32_t position = 0; 00325 00326 /* Check which bar is selected */ 00327 while ((BarId) >> position) 00328 { 00329 /* Check if current bar is selected */ 00330 switch(BarId & (1 << position)) 00331 { 00332 /* Bar 0 */ 00333 case LCD_BAR_0: 00334 /* Set BAR0 */ 00335 HAL_LCD_Write(&LCDHandle, LCD_BAR0_2_COM, ~(LCD_BAR0_SEG) , 0); 00336 break; 00337 00338 /* Bar 1 */ 00339 case LCD_BAR_1: 00340 /* Set BAR1 */ 00341 HAL_LCD_Write(&LCDHandle, LCD_BAR1_3_COM, ~(LCD_BAR1_SEG), 0); 00342 break; 00343 00344 /* Bar 2 */ 00345 case LCD_BAR_2: 00346 /* Set BAR2 */ 00347 HAL_LCD_Write(&LCDHandle, LCD_BAR0_2_COM, ~(LCD_BAR2_SEG), 0); 00348 break; 00349 00350 /* Bar 3 */ 00351 case LCD_BAR_3: 00352 /* Set BAR3 */ 00353 HAL_LCD_Write(&LCDHandle, LCD_BAR1_3_COM, ~(LCD_BAR3_SEG), 0); 00354 break; 00355 00356 default: 00357 break; 00358 } 00359 position++; 00360 } 00361 00362 /* Update the LCD display */ 00363 HAL_LCD_UpdateDisplayRequest(&LCDHandle); 00364 } 00365 00366 /** 00367 * @brief Configure the bar level on LCD by writing bar value in LCD frame buffer. 00368 * @param BarLevel: specifies the LCD GLASS Battery Level. 00369 * This parameter can be one of the following values: 00370 * @arg BATTERYLEVEL_OFF: LCD GLASS Battery Empty 00371 * @arg BATTERYLEVEL_1_4: LCD GLASS Battery 1/4 Full 00372 * @arg BATTERYLEVEL_1_2: LCD GLASS Battery 1/2 Full 00373 * @arg BATTERYLEVEL_3_4: LCD GLASS Battery 3/4 Full 00374 * @arg BATTERYLEVEL_FULL: LCD GLASS Battery Full 00375 * @retval None 00376 */ 00377 void BSP_LCD_GLASS_BarLevelConfig(uint8_t BarLevel) 00378 { 00379 switch (BarLevel) 00380 { 00381 /* BATTERYLEVEL_OFF */ 00382 case BATTERYLEVEL_OFF: 00383 /* Set BAR0 & BAR2 off */ 00384 HAL_LCD_Write(&LCDHandle, LCD_BAR0_2_COM, ~(LCD_BAR0_SEG | LCD_BAR2_SEG), 0); 00385 /* Set BAR1 & BAR3 off */ 00386 HAL_LCD_Write(&LCDHandle, LCD_BAR1_3_COM, ~(LCD_BAR1_SEG | LCD_BAR3_SEG), 0); 00387 LCDBar = BATTERYLEVEL_OFF; 00388 break; 00389 00390 /* BARLEVEL 1/4 */ 00391 case BATTERYLEVEL_1_4: 00392 /* Set BAR0 on & BAR2 off */ 00393 HAL_LCD_Write(&LCDHandle, LCD_BAR0_2_COM, ~(LCD_BAR0_SEG | LCD_BAR2_SEG), LCD_BAR0_SEG); 00394 /* Set BAR1 & BAR3 off */ 00395 HAL_LCD_Write(&LCDHandle, LCD_BAR1_3_COM, ~(LCD_BAR1_SEG | LCD_BAR3_SEG), 0); 00396 LCDBar = BATTERYLEVEL_1_4; 00397 break; 00398 00399 /* BARLEVEL 1/2 */ 00400 case BATTERYLEVEL_1_2: 00401 /* Set BAR0 on & BAR2 off */ 00402 HAL_LCD_Write(&LCDHandle, LCD_BAR0_2_COM, ~(LCD_BAR0_SEG | LCD_BAR2_SEG), LCD_BAR0_SEG); 00403 /* Set BAR1 on & BAR3 off */ 00404 HAL_LCD_Write(&LCDHandle, LCD_BAR1_3_COM, ~(LCD_BAR1_SEG | LCD_BAR3_SEG), LCD_BAR1_SEG); 00405 LCDBar = BATTERYLEVEL_1_2; 00406 break; 00407 00408 /* Battery Level 3/4 */ 00409 case BATTERYLEVEL_3_4: 00410 /* Set BAR0 & BAR2 on */ 00411 HAL_LCD_Write(&LCDHandle, LCD_BAR0_2_COM, ~(LCD_BAR0_SEG | LCD_BAR2_SEG), (LCD_BAR0_SEG | LCD_BAR2_SEG)); 00412 /* Set BAR1 on & BAR3 off */ 00413 HAL_LCD_Write(&LCDHandle, LCD_BAR1_3_COM, ~(LCD_BAR1_SEG | LCD_BAR3_SEG), LCD_BAR1_SEG); 00414 LCDBar = BATTERYLEVEL_3_4; 00415 break; 00416 00417 /* BATTERYLEVEL_FULL */ 00418 case BATTERYLEVEL_FULL: 00419 /* Set BAR0 & BAR2 on */ 00420 HAL_LCD_Write(&LCDHandle, LCD_BAR0_2_COM, ~(LCD_BAR0_SEG | LCD_BAR2_SEG), (LCD_BAR0_SEG | LCD_BAR2_SEG)); 00421 /* Set BAR1 on & BAR3 on */ 00422 HAL_LCD_Write(&LCDHandle, LCD_BAR1_3_COM, ~(LCD_BAR1_SEG | LCD_BAR3_SEG), (LCD_BAR1_SEG | LCD_BAR3_SEG)); 00423 LCDBar = BATTERYLEVEL_FULL; 00424 break; 00425 00426 default: 00427 break; 00428 } 00429 00430 /* Update the LCD display */ 00431 HAL_LCD_UpdateDisplayRequest(&LCDHandle); 00432 } 00433 00434 /** 00435 * @brief Write a character in the LCD RAM buffer. 00436 * @param ch: The character to display. 00437 * @param Point: A point to add in front of char. 00438 * This parameter can be one of the following values: 00439 * @arg POINT_OFF: No point to add in front of char. 00440 * @arg POINT_ON: Add a point in front of char. 00441 * @param Colon: Flag indicating if a colon character has to be added in front 00442 * of displayed character. 00443 * This parameter can be one of the following values: 00444 * @arg DOUBLEPOINT_OFF: No colon to add in back of char. 00445 * @arg DOUBLEPOINT_ON: Add an colon in back of char. 00446 * @param Position: Position in the LCD of the character to write. 00447 * This parameter can be any value in range [1:6]. 00448 * @retval None 00449 * @note Required preconditions: The LCD should be cleared before to start the 00450 * write operation. 00451 */ 00452 void BSP_LCD_GLASS_DisplayChar(uint8_t* ch, Point_Typedef Point, DoublePoint_Typedef Colon, DigitPosition_Typedef Position) 00453 { 00454 WriteChar(ch, Point, Colon, Position); 00455 00456 /* Update the LCD display */ 00457 HAL_LCD_UpdateDisplayRequest(&LCDHandle); 00458 } 00459 00460 /** 00461 * @brief Write a character string in the LCD RAM buffer. 00462 * @param ptr: Pointer to string to display on the LCD Glass. 00463 * @retval None 00464 */ 00465 void BSP_LCD_GLASS_DisplayString(uint8_t* ptr) 00466 { 00467 DigitPosition_Typedef position = LCD_DIGIT_POSITION_1; 00468 00469 /* Send the string character by character on lCD */ 00470 while ((*ptr != 0) & (position <= LCD_DIGIT_POSITION_6)) 00471 { 00472 /* Write one character on LCD */ 00473 WriteChar(ptr, POINT_OFF, DOUBLEPOINT_OFF, position); 00474 00475 /* Point on the next character */ 00476 ptr++; 00477 00478 /* Increment the character counter */ 00479 position++; 00480 } 00481 /* Update the LCD display */ 00482 HAL_LCD_UpdateDisplayRequest(&LCDHandle); 00483 } 00484 00485 /** 00486 * @brief Write a character string with decimal point in the LCD RAM buffer. 00487 * @param ptr: Pointer to string to display on the LCD Glass. 00488 * @retval None 00489 * @note Required preconditions: Char is ASCCI value "ORed" with decimal point or Colon flag 00490 */ 00491 void BSP_LCD_GLASS_DisplayStrDeci(uint16_t* ptr) 00492 { 00493 DigitPosition_Typedef index = LCD_DIGIT_POSITION_1; 00494 uint8_t tmpchar = 0; 00495 00496 /* Send the string character by character on lCD */ 00497 while((*ptr != 0) & (index <= LCD_DIGIT_POSITION_6)) 00498 { 00499 tmpchar = (*ptr) & 0x00FF; 00500 00501 switch((*ptr) & 0xF000) 00502 { 00503 case DOT: 00504 /* Write one character on LCD with decimal point */ 00505 WriteChar(&tmpchar, POINT_ON, DOUBLEPOINT_OFF, index); 00506 break; 00507 case DOUBLE_DOT: 00508 /* Write one character on LCD with decimal point */ 00509 WriteChar(&tmpchar, POINT_OFF, DOUBLEPOINT_ON, index); 00510 break; 00511 default: 00512 WriteChar(&tmpchar, POINT_OFF, DOUBLEPOINT_OFF, index); 00513 break; 00514 }/* Point on the next character */ 00515 ptr++; 00516 00517 /* Increment the character counter */ 00518 index++; 00519 } 00520 /* Update the LCD display */ 00521 HAL_LCD_UpdateDisplayRequest(&LCDHandle); 00522 } 00523 00524 /** 00525 * @brief Clear the whole LCD RAM buffer. 00526 * @retval None 00527 */ 00528 void BSP_LCD_GLASS_Clear(void) 00529 { 00530 HAL_LCD_Clear(&LCDHandle); 00531 } 00532 00533 /** 00534 * @brief Display a string in scrolling mode 00535 * @param ptr: Pointer to string to display on the LCD Glass. 00536 * @param nScroll: Specifies how many time the message will be scrolled 00537 * @param ScrollSpeed : Specifies the speed of the scroll, low value gives 00538 * higher speed 00539 * @retval None 00540 * @note Required preconditions: The LCD should be cleared before to start the 00541 * write operation. 00542 */ 00543 void BSP_LCD_GLASS_ScrollSentence(uint8_t* ptr, uint16_t nScroll, uint16_t ScrollSpeed) 00544 { 00545 uint8_t repetition = 0, nbrchar = 0, sizestr = 0; 00546 uint8_t* ptr1; 00547 uint8_t str[6] = ""; 00548 00549 /* Reset interrupt variable in case key was press before entering function */ 00550 bLCDGlass_KeyPressed = 0; 00551 00552 if(ptr == 0) 00553 { 00554 return; 00555 } 00556 00557 /* To calculate end of string */ 00558 for(ptr1 = ptr, sizestr = 0; *ptr1 != 0; sizestr++, ptr1++); 00559 00560 ptr1 = ptr; 00561 00562 BSP_LCD_GLASS_DisplayString(str); 00563 HAL_Delay(ScrollSpeed); 00564 00565 /* To shift the string for scrolling display*/ 00566 for (repetition = 0; repetition < nScroll; repetition++) 00567 { 00568 for(nbrchar = 0; nbrchar < sizestr; nbrchar++) 00569 { 00570 *(str) =* (ptr1+((nbrchar+1)%sizestr)); 00571 *(str+1) =* (ptr1+((nbrchar+2)%sizestr)); 00572 *(str+2) =* (ptr1+((nbrchar+3)%sizestr)); 00573 *(str+3) =* (ptr1+((nbrchar+4)%sizestr)); 00574 *(str+4) =* (ptr1+((nbrchar+5)%sizestr)); 00575 *(str+5) =* (ptr1+((nbrchar+6)%sizestr)); 00576 BSP_LCD_GLASS_Clear(); 00577 BSP_LCD_GLASS_DisplayString(str); 00578 00579 /* user button pressed stop the scrolling sentence */ 00580 if(bLCDGlass_KeyPressed) 00581 { 00582 bLCDGlass_KeyPressed = 0; 00583 return; 00584 } 00585 HAL_Delay(ScrollSpeed); 00586 } 00587 } 00588 } 00589 00590 /** 00591 * @} 00592 */ 00593 00594 /** @addtogroup STM32L476G_DISCOVERY_LCD_Private_Functions 00595 * @{ 00596 */ 00597 00598 /** 00599 * @brief Initialize the LCD MSP. 00600 * @param hlcd: LCD handle 00601 * @retval None 00602 */ 00603 static void LCD_MspInit(LCD_HandleTypeDef *hlcd) 00604 { 00605 GPIO_InitTypeDef gpioinitstruct = {0}; 00606 RCC_OscInitTypeDef oscinitstruct = {0}; 00607 RCC_PeriphCLKInitTypeDef periphclkstruct = {0}; 00608 00609 /*##-1- Enable PWR peripheral Clock #######################################*/ 00610 __HAL_RCC_PWR_CLK_ENABLE(); 00611 00612 /*##-2- Configure LSE as RTC clock soucre ###################################*/ 00613 oscinitstruct.OscillatorType = RCC_OSCILLATORTYPE_LSE; 00614 oscinitstruct.PLL.PLLState = RCC_PLL_NONE; 00615 oscinitstruct.LSEState = RCC_LSE_ON; 00616 if(HAL_RCC_OscConfig(&oscinitstruct) != HAL_OK) 00617 { 00618 while(1); 00619 } 00620 00621 /*##-3- Select LSE as RTC clock source.##########################*/ 00622 /* Backup domain management is done in RCC function */ 00623 periphclkstruct.PeriphClockSelection = RCC_PERIPHCLK_RTC; 00624 periphclkstruct.RTCClockSelection = RCC_RTCCLKSOURCE_LSE; 00625 HAL_RCCEx_PeriphCLKConfig(&periphclkstruct); 00626 00627 /*##-4- Enable LCD GPIO Clocks #############################################*/ 00628 __HAL_RCC_GPIOA_CLK_ENABLE(); 00629 __HAL_RCC_GPIOB_CLK_ENABLE(); 00630 __HAL_RCC_GPIOC_CLK_ENABLE(); 00631 __HAL_RCC_GPIOD_CLK_ENABLE(); 00632 00633 00634 /*##-5- Configure peripheral GPIO ##########################################*/ 00635 /* Configure Output for LCD */ 00636 /* Port A */ 00637 gpioinitstruct.Pin = LCD_GPIO_BANKA_PINS; 00638 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00639 gpioinitstruct.Pull = GPIO_NOPULL; 00640 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00641 gpioinitstruct.Alternate = GPIO_AF11_LCD; 00642 HAL_GPIO_Init(GPIOA, &gpioinitstruct); 00643 00644 /* Port B */ 00645 gpioinitstruct.Pin = LCD_GPIO_BANKB_PINS; 00646 HAL_GPIO_Init(GPIOB, &gpioinitstruct); 00647 00648 /* Port C*/ 00649 gpioinitstruct.Pin = LCD_GPIO_BANKC_PINS; 00650 HAL_GPIO_Init(GPIOC, &gpioinitstruct); 00651 00652 /* Port D */ 00653 gpioinitstruct.Pin = LCD_GPIO_BANKD_PINS; 00654 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00655 00656 /* Wait for the external capacitor Cext which is connected to the VLCD pin is charged 00657 (approximately 2ms for Cext=1uF) */ 00658 HAL_Delay(2); 00659 00660 /*##-6- Enable LCD peripheral Clock ########################################*/ 00661 __HAL_RCC_LCD_CLK_ENABLE(); 00662 } 00663 00664 /** 00665 * @brief DeInitialize the LCD MSP. 00666 * @param hlcd: LCD handle 00667 * @retval None 00668 */ 00669 static void LCD_MspDeInit(LCD_HandleTypeDef *hlcd) 00670 { 00671 uint32_t gpiopin = 0; 00672 00673 /*##-1- Enable LCD GPIO Clocks #############################################*/ 00674 __HAL_RCC_GPIOA_CLK_ENABLE(); 00675 __HAL_RCC_GPIOB_CLK_ENABLE(); 00676 __HAL_RCC_GPIOC_CLK_ENABLE(); 00677 __HAL_RCC_GPIOD_CLK_ENABLE(); 00678 00679 /*##-1- Configure peripheral GPIO ##########################################*/ 00680 /* Configure Output for LCD */ 00681 /* Port A */ 00682 gpiopin = LCD_GPIO_BANKA_PINS; 00683 HAL_GPIO_DeInit(GPIOA, gpiopin); 00684 00685 /* Port B */ 00686 gpiopin = LCD_GPIO_BANKB_PINS; 00687 HAL_GPIO_DeInit(GPIOB, gpiopin); 00688 00689 /* Port C*/ 00690 gpiopin = LCD_GPIO_BANKC_PINS; 00691 HAL_GPIO_DeInit(GPIOC, gpiopin); 00692 00693 /* Port D */ 00694 gpiopin = LCD_GPIO_BANKD_PINS; 00695 HAL_GPIO_DeInit(GPIOD, gpiopin); 00696 00697 /*##-5- Enable LCD peripheral Clock ########################################*/ 00698 __HAL_RCC_LCD_CLK_DISABLE(); 00699 } 00700 00701 /** 00702 * @brief Convert an ascii char to the a LCD digit. 00703 * @param Char: a char to display. 00704 * @param Point: a point to add in front of char 00705 * This parameter can be: POINT_OFF or POINT_ON 00706 * @param Colon : flag indicating if a colon character has to be added in front 00707 * of displayed character. 00708 * This parameter can be: DOUBLEPOINT_OFF or DOUBLEPOINT_ON. 00709 * @retval None 00710 */ 00711 static void Convert(uint8_t* Char, Point_Typedef Point, DoublePoint_Typedef Colon) 00712 { 00713 uint16_t ch = 0 ; 00714 uint8_t loop = 0, index = 0; 00715 00716 switch (*Char) 00717 { 00718 case ' ' : 00719 ch = 0x00; 00720 break; 00721 00722 case '*': 00723 ch = C_STAR; 00724 break; 00725 00726 case '(' : 00727 ch = C_OPENPARMAP; 00728 break; 00729 00730 case ')' : 00731 ch = C_CLOSEPARMAP; 00732 break; 00733 00734 case 'd' : 00735 ch = C_DMAP; 00736 break; 00737 00738 case 'm' : 00739 ch = C_MMAP; 00740 break; 00741 00742 case 'n' : 00743 ch = C_NMAP; 00744 break; 00745 00746 case '�' : 00747 ch = C_UMAP; 00748 break; 00749 00750 case '-' : 00751 ch = C_MINUS; 00752 break; 00753 00754 case '+' : 00755 ch = C_PLUS; 00756 break; 00757 00758 case '/' : 00759 ch = C_SLATCH; 00760 break; 00761 00762 case '�' : 00763 ch = C_PERCENT_1; 00764 break; 00765 case '%' : 00766 ch = C_PERCENT_2; 00767 break; 00768 case 255 : 00769 ch = C_FULL; 00770 break ; 00771 00772 case '0': 00773 case '1': 00774 case '2': 00775 case '3': 00776 case '4': 00777 case '5': 00778 case '6': 00779 case '7': 00780 case '8': 00781 case '9': 00782 ch = NumberMap[*Char - ASCII_CHAR_0]; 00783 break; 00784 00785 default: 00786 /* The character Char is one letter in upper case*/ 00787 if ( (*Char < ASCII_CHAR_LEFT_OPEN_BRACKET) && (*Char > ASCII_CHAR_AT_SYMBOL) ) 00788 { 00789 ch = CapLetterMap[*Char - 'A']; 00790 } 00791 /* The character Char is one letter in lower case*/ 00792 if ( (*Char < ASCII_CHAR_LEFT_OPEN_BRACE) && ( *Char > ASCII_CHAR_APOSTROPHE) ) 00793 { 00794 ch = CapLetterMap[*Char - 'a']; 00795 } 00796 break; 00797 } 00798 00799 /* Set the digital point can be displayed if the point is on */ 00800 if (Point == POINT_ON) 00801 { 00802 ch |= 0x0002; 00803 } 00804 00805 /* Set the "COL" segment in the character that can be displayed if the colon is on */ 00806 if (Colon == DOUBLEPOINT_ON) 00807 { 00808 ch |= 0x0020; 00809 } 00810 00811 for (loop = 12,index=0 ;index < 4; loop -= 4,index++) 00812 { 00813 Digit[index] = (ch >> loop) & 0x0f; /*To isolate the less significant digit */ 00814 } 00815 } 00816 00817 /** 00818 * @brief Write a character in the LCD frame buffer. 00819 * @param ch: the character to display. 00820 * @param Point: a point to add in front of char 00821 * This parameter can be: POINT_OFF or POINT_ON 00822 * @param Colon: flag indicating if a colon character has to be added in front 00823 * of displayed character. 00824 * This parameter can be: DOUBLEPOINT_OFF or DOUBLEPOINT_ON. 00825 * @param Position: position in the LCD of the character to write [1:6] 00826 * @retval None 00827 */ 00828 static void WriteChar(uint8_t* ch, Point_Typedef Point, DoublePoint_Typedef Colon, DigitPosition_Typedef Position) 00829 { 00830 uint32_t data =0x00; 00831 /* To convert displayed character in segment in array digit */ 00832 Convert(ch, (Point_Typedef)Point, (DoublePoint_Typedef)Colon); 00833 00834 switch (Position) 00835 { 00836 /* Position 1 on LCD (Digit1)*/ 00837 case LCD_DIGIT_POSITION_1: 00838 data = ((Digit[0] & 0x1) << LCD_SEG0_SHIFT) | (((Digit[0] & 0x2) >> 1) << LCD_SEG1_SHIFT) 00839 | (((Digit[0] & 0x4) >> 2) << LCD_SEG22_SHIFT) | (((Digit[0] & 0x8) >> 3) << LCD_SEG23_SHIFT); 00840 HAL_LCD_Write(&LCDHandle, LCD_DIGIT1_COM0, LCD_DIGIT1_COM0_SEG_MASK, data); /* 1G 1B 1M 1E */ 00841 00842 data = ((Digit[1] & 0x1) << LCD_SEG0_SHIFT) | (((Digit[1] & 0x2) >> 1) << LCD_SEG1_SHIFT) 00843 | (((Digit[1] & 0x4) >> 2) << LCD_SEG22_SHIFT) | (((Digit[1] & 0x8) >> 3) << LCD_SEG23_SHIFT); 00844 HAL_LCD_Write(&LCDHandle, LCD_DIGIT1_COM1, LCD_DIGIT1_COM1_SEG_MASK, data) ; /* 1F 1A 1C 1D */ 00845 00846 data = ((Digit[2] & 0x1) << LCD_SEG0_SHIFT) | (((Digit[2] & 0x2) >> 1) << LCD_SEG1_SHIFT) 00847 | (((Digit[2] & 0x4) >> 2) << LCD_SEG22_SHIFT) | (((Digit[2] & 0x8) >> 3) << LCD_SEG23_SHIFT); 00848 HAL_LCD_Write(&LCDHandle, LCD_DIGIT1_COM2, LCD_DIGIT1_COM2_SEG_MASK, data) ; /* 1Q 1K 1Col 1P */ 00849 00850 data = ((Digit[3] & 0x1) << LCD_SEG0_SHIFT) | (((Digit[3] & 0x2) >> 1) << LCD_SEG1_SHIFT) 00851 | (((Digit[3] & 0x4) >> 2) << LCD_SEG22_SHIFT) | (((Digit[3] & 0x8) >> 3) << LCD_SEG23_SHIFT); 00852 HAL_LCD_Write(&LCDHandle, LCD_DIGIT1_COM3, LCD_DIGIT1_COM3_SEG_MASK, data) ; /* 1H 1J 1DP 1N */ 00853 break; 00854 00855 /* Position 2 on LCD (Digit2)*/ 00856 case LCD_DIGIT_POSITION_2: 00857 data = ((Digit[0] & 0x1) << LCD_SEG2_SHIFT) | (((Digit[0] & 0x2) >> 1) << LCD_SEG3_SHIFT) 00858 | (((Digit[0] & 0x4) >> 2) << LCD_SEG20_SHIFT) | (((Digit[0] & 0x8) >> 3) << LCD_SEG21_SHIFT); 00859 HAL_LCD_Write(&LCDHandle, LCD_DIGIT2_COM0, LCD_DIGIT2_COM0_SEG_MASK, data); /* 1G 1B 1M 1E */ 00860 00861 data = ((Digit[1] & 0x1) << LCD_SEG2_SHIFT) | (((Digit[1] & 0x2) >> 1) << LCD_SEG3_SHIFT) 00862 | (((Digit[1] & 0x4) >> 2) << LCD_SEG20_SHIFT) | (((Digit[1] & 0x8) >> 3) << LCD_SEG21_SHIFT); 00863 HAL_LCD_Write(&LCDHandle, LCD_DIGIT2_COM1, LCD_DIGIT2_COM1_SEG_MASK, data) ; /* 1F 1A 1C 1D */ 00864 00865 data = ((Digit[2] & 0x1) << LCD_SEG2_SHIFT) | (((Digit[2] & 0x2) >> 1) << LCD_SEG3_SHIFT) 00866 | (((Digit[2] & 0x4) >> 2) << LCD_SEG20_SHIFT) | (((Digit[2] & 0x8) >> 3) << LCD_SEG21_SHIFT); 00867 HAL_LCD_Write(&LCDHandle, LCD_DIGIT2_COM2, LCD_DIGIT2_COM2_SEG_MASK, data) ; /* 1Q 1K 1Col 1P */ 00868 00869 data = ((Digit[3] & 0x1) << LCD_SEG2_SHIFT) | (((Digit[3] & 0x2) >> 1) << LCD_SEG3_SHIFT) 00870 | (((Digit[3] & 0x4) >> 2) << LCD_SEG20_SHIFT) | (((Digit[3] & 0x8) >> 3) << LCD_SEG21_SHIFT); 00871 HAL_LCD_Write(&LCDHandle, LCD_DIGIT2_COM3, LCD_DIGIT2_COM3_SEG_MASK, data) ; /* 1H 1J 1DP 1N */ 00872 break; 00873 00874 /* Position 3 on LCD (Digit3)*/ 00875 case LCD_DIGIT_POSITION_3: 00876 data = ((Digit[0] & 0x1) << LCD_SEG4_SHIFT) | (((Digit[0] & 0x2) >> 1) << LCD_SEG5_SHIFT) 00877 | (((Digit[0] & 0x4) >> 2) << LCD_SEG18_SHIFT) | (((Digit[0] & 0x8) >> 3) << LCD_SEG19_SHIFT); 00878 HAL_LCD_Write(&LCDHandle, LCD_DIGIT3_COM0, LCD_DIGIT3_COM0_SEG_MASK, data); /* 1G 1B 1M 1E */ 00879 00880 data = ((Digit[1] & 0x1) << LCD_SEG4_SHIFT) | (((Digit[1] & 0x2) >> 1) << LCD_SEG5_SHIFT) 00881 | (((Digit[1] & 0x4) >> 2) << LCD_SEG18_SHIFT) | (((Digit[1] & 0x8) >> 3) << LCD_SEG19_SHIFT); 00882 HAL_LCD_Write(&LCDHandle, LCD_DIGIT3_COM1, LCD_DIGIT3_COM1_SEG_MASK, data) ; /* 1F 1A 1C 1D */ 00883 00884 data = ((Digit[2] & 0x1) << LCD_SEG4_SHIFT) | (((Digit[2] & 0x2) >> 1) << LCD_SEG5_SHIFT) 00885 | (((Digit[2] & 0x4) >> 2) << LCD_SEG18_SHIFT) | (((Digit[2] & 0x8) >> 3) << LCD_SEG19_SHIFT); 00886 HAL_LCD_Write(&LCDHandle, LCD_DIGIT3_COM2, LCD_DIGIT3_COM2_SEG_MASK, data) ; /* 1Q 1K 1Col 1P */ 00887 00888 data = ((Digit[3] & 0x1) << LCD_SEG4_SHIFT) | (((Digit[3] & 0x2) >> 1) << LCD_SEG5_SHIFT) 00889 | (((Digit[3] & 0x4) >> 2) << LCD_SEG18_SHIFT) | (((Digit[3] & 0x8) >> 3) << LCD_SEG19_SHIFT); 00890 HAL_LCD_Write(&LCDHandle, LCD_DIGIT3_COM3, LCD_DIGIT3_COM3_SEG_MASK, data) ; /* 1H 1J 1DP 1N */ 00891 break; 00892 00893 /* Position 4 on LCD (Digit4)*/ 00894 case LCD_DIGIT_POSITION_4: 00895 data = ((Digit[0] & 0x1) << LCD_SEG6_SHIFT) | (((Digit[0] & 0x8) >> 3) << LCD_SEG17_SHIFT); 00896 HAL_LCD_Write(&LCDHandle, LCD_DIGIT4_COM0, LCD_DIGIT4_COM0_SEG_MASK, data); /* 1G 1B 1M 1E */ 00897 00898 data = (((Digit[0] & 0x2) >> 1) << LCD_SEG7_SHIFT) | (((Digit[0] & 0x4) >> 2) << LCD_SEG16_SHIFT); 00899 HAL_LCD_Write(&LCDHandle, LCD_DIGIT4_COM0_1, LCD_DIGIT4_COM0_1_SEG_MASK, data); /* 1G 1B 1M 1E */ 00900 00901 data = ((Digit[1] & 0x1) << LCD_SEG6_SHIFT) | (((Digit[1] & 0x8) >> 3) << LCD_SEG17_SHIFT); 00902 HAL_LCD_Write(&LCDHandle, LCD_DIGIT4_COM1, LCD_DIGIT4_COM1_SEG_MASK, data) ; /* 1F 1A 1C 1D */ 00903 00904 data = (((Digit[1] & 0x2) >> 1) << LCD_SEG7_SHIFT) | (((Digit[1] & 0x4) >> 2) << LCD_SEG16_SHIFT); 00905 HAL_LCD_Write(&LCDHandle, LCD_DIGIT4_COM1_1, LCD_DIGIT4_COM1_1_SEG_MASK, data) ; /* 1F 1A 1C 1D */ 00906 00907 data = ((Digit[2] & 0x1) << LCD_SEG6_SHIFT) | (((Digit[2] & 0x8) >> 3) << LCD_SEG17_SHIFT); 00908 HAL_LCD_Write(&LCDHandle, LCD_DIGIT4_COM2, LCD_DIGIT4_COM2_SEG_MASK, data) ; /* 1Q 1K 1Col 1P */ 00909 00910 data = (((Digit[2] & 0x2) >> 1) << LCD_SEG7_SHIFT) | (((Digit[2] & 0x4) >> 2) << LCD_SEG16_SHIFT); 00911 HAL_LCD_Write(&LCDHandle, LCD_DIGIT4_COM2_1, LCD_DIGIT4_COM2_1_SEG_MASK, data) ; /* 1Q 1K 1Col 1P */ 00912 00913 data = ((Digit[3] & 0x1) << LCD_SEG6_SHIFT) | (((Digit[3] & 0x8) >> 3) << LCD_SEG17_SHIFT); 00914 HAL_LCD_Write(&LCDHandle, LCD_DIGIT4_COM3, LCD_DIGIT4_COM3_SEG_MASK, data) ; /* 1H 1J 1DP 1N */ 00915 00916 data = (((Digit[3] & 0x2) >> 1) << LCD_SEG7_SHIFT) | (((Digit[3] & 0x4) >> 2) << LCD_SEG16_SHIFT); 00917 HAL_LCD_Write(&LCDHandle, LCD_DIGIT4_COM3_1, LCD_DIGIT4_COM3_1_SEG_MASK, data) ; /* 1H 1J 1DP 1N */ 00918 break; 00919 00920 /* Position 5 on LCD (Digit5)*/ 00921 case LCD_DIGIT_POSITION_5: 00922 data = (((Digit[0] & 0x2) >> 1) << LCD_SEG9_SHIFT) | (((Digit[0] & 0x4) >> 2) << LCD_SEG14_SHIFT); 00923 HAL_LCD_Write(&LCDHandle, LCD_DIGIT5_COM0, LCD_DIGIT5_COM0_SEG_MASK, data); /* 1G 1B 1M 1E */ 00924 00925 data = ((Digit[0] & 0x1) << LCD_SEG8_SHIFT) | (((Digit[0] & 0x8) >> 3) << LCD_SEG15_SHIFT); 00926 HAL_LCD_Write(&LCDHandle, LCD_DIGIT5_COM0_1, LCD_DIGIT5_COM0_1_SEG_MASK, data); /* 1G 1B 1M 1E */ 00927 00928 data = (((Digit[1] & 0x2) >> 1) << LCD_SEG9_SHIFT) | (((Digit[1] & 0x4) >> 2) << LCD_SEG14_SHIFT); 00929 HAL_LCD_Write(&LCDHandle, LCD_DIGIT5_COM1, LCD_DIGIT5_COM1_SEG_MASK, data) ; /* 1F 1A 1C 1D */ 00930 00931 data = ((Digit[1] & 0x1) << LCD_SEG8_SHIFT) | (((Digit[1] & 0x8) >> 3) << LCD_SEG15_SHIFT); 00932 HAL_LCD_Write(&LCDHandle, LCD_DIGIT5_COM1_1, LCD_DIGIT5_COM1_1_SEG_MASK, data) ; /* 1F 1A 1C 1D */ 00933 00934 data = (((Digit[2] & 0x2) >> 1) << LCD_SEG9_SHIFT) | (((Digit[2] & 0x4) >> 2) << LCD_SEG14_SHIFT); 00935 HAL_LCD_Write(&LCDHandle, LCD_DIGIT5_COM2, LCD_DIGIT5_COM2_SEG_MASK, data) ; /* 1Q 1K 1Col 1P */ 00936 00937 data = ((Digit[2] & 0x1) << LCD_SEG8_SHIFT) | (((Digit[2] & 0x8) >> 3) << LCD_SEG15_SHIFT); 00938 HAL_LCD_Write(&LCDHandle, LCD_DIGIT5_COM2_1, LCD_DIGIT5_COM2_1_SEG_MASK, data) ; /* 1Q 1K 1Col 1P */ 00939 00940 data = (((Digit[3] & 0x2) >> 1) << LCD_SEG9_SHIFT) | (((Digit[3] & 0x4) >> 2) << LCD_SEG14_SHIFT); 00941 HAL_LCD_Write(&LCDHandle, LCD_DIGIT5_COM3, LCD_DIGIT5_COM3_SEG_MASK, data) ; /* 1H 1J 1DP 1N */ 00942 00943 data = ((Digit[3] & 0x1) << LCD_SEG8_SHIFT) | (((Digit[3] & 0x8) >> 3) << LCD_SEG15_SHIFT); 00944 HAL_LCD_Write(&LCDHandle, LCD_DIGIT5_COM3_1, LCD_DIGIT5_COM3_1_SEG_MASK, data) ; /* 1H 1J 1DP 1N */ 00945 break; 00946 00947 /* Position 6 on LCD (Digit6)*/ 00948 case LCD_DIGIT_POSITION_6: 00949 data = ((Digit[0] & 0x1) << LCD_SEG10_SHIFT) | (((Digit[0] & 0x2) >> 1) << LCD_SEG11_SHIFT) 00950 | (((Digit[0] & 0x4) >> 2) << LCD_SEG12_SHIFT) | (((Digit[0] & 0x8) >> 3) << LCD_SEG13_SHIFT); 00951 HAL_LCD_Write(&LCDHandle, LCD_DIGIT6_COM0, LCD_DIGIT6_COM0_SEG_MASK, data); /* 1G 1B 1M 1E */ 00952 00953 data = ((Digit[1] & 0x1) << LCD_SEG10_SHIFT) | (((Digit[1] & 0x2) >> 1) << LCD_SEG11_SHIFT) 00954 | (((Digit[1] & 0x4) >> 2) << LCD_SEG12_SHIFT) | (((Digit[1] & 0x8) >> 3) << LCD_SEG13_SHIFT); 00955 HAL_LCD_Write(&LCDHandle, LCD_DIGIT6_COM1, LCD_DIGIT6_COM1_SEG_MASK, data) ; /* 1F 1A 1C 1D */ 00956 00957 data = ((Digit[2] & 0x1) << LCD_SEG10_SHIFT) | (((Digit[2] & 0x2) >> 1) << LCD_SEG11_SHIFT) 00958 | (((Digit[2] & 0x4) >> 2) << LCD_SEG12_SHIFT) | (((Digit[2] & 0x8) >> 3) << LCD_SEG13_SHIFT); 00959 HAL_LCD_Write(&LCDHandle, LCD_DIGIT6_COM2, LCD_DIGIT6_COM2_SEG_MASK, data) ; /* 1Q 1K 1Col 1P */ 00960 00961 data = ((Digit[3] & 0x1) << LCD_SEG10_SHIFT) | (((Digit[3] & 0x2) >> 1) << LCD_SEG11_SHIFT) 00962 | (((Digit[3] & 0x4) >> 2) << LCD_SEG12_SHIFT) | (((Digit[3] & 0x8) >> 3) << LCD_SEG13_SHIFT); 00963 HAL_LCD_Write(&LCDHandle, LCD_DIGIT6_COM3, LCD_DIGIT6_COM3_SEG_MASK, data) ; /* 1H 1J 1DP 1N */ 00964 break; 00965 00966 default: 00967 break; 00968 } 00969 } 00970 00971 /** 00972 * @} 00973 */ 00974 00975 /** 00976 * @} 00977 */ 00978 00979 /** 00980 * @} 00981 */ 00982 00983 /** 00984 * @} 00985 */ 00986 00987 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jun 23 2015 17:15:45 for STM32L476G-Discovery BSP User Manual by
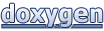