STM32L476G-Discovery BSP User Manual
|
stm32l476g_discovery_accelerometer.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l476g_discovery_accelerometer.c 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief This file provides a set of functions needed to manage the ACCELEROMETER 00008 * MEMS available on STM32L476G-Discovery Kit. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Includes ------------------------------------------------------------------*/ 00040 #include "stm32l476g_discovery_accelerometer.h" 00041 00042 /** @addtogroup BSP 00043 * @{ 00044 */ 00045 00046 /** @addtogroup STM32L476G_DISCOVERY 00047 * @{ 00048 */ 00049 00050 /** @defgroup STM32L476G_DISCOVERY_ACCELEROMETER STM32L476G-DISCOVERY ACCELEROMETER 00051 * @{ 00052 */ 00053 00054 /* Private typedef -----------------------------------------------------------*/ 00055 /** @defgroup STM32L476G_DISCOVERY_ACCELEROMETER_Private_Types Private Types 00056 * @{ 00057 */ 00058 /** 00059 * @} 00060 */ 00061 00062 /* Private defines ------------------------------------------------------------*/ 00063 /** @defgroup STM32L476G_DISCOVERY_ACCELEROMETER_Private_Constants Private Constants 00064 * @{ 00065 */ 00066 /** 00067 * @} 00068 */ 00069 00070 /* Private macros ------------------------------------------------------------*/ 00071 /** @defgroup STM32L476G_DISCOVERY_ACCELEROMETER_Private_Macros Private Macros 00072 * @{ 00073 */ 00074 /** 00075 * @} 00076 */ 00077 00078 /* Private variables ---------------------------------------------------------*/ 00079 /** @defgroup STM32L476G_DISCOVERY_ACCELEROMETER_Private_Variables Private Variables 00080 * @{ 00081 */ 00082 static ACCELERO_DrvTypeDef *AccelerometerDrv; 00083 00084 /** 00085 * @} 00086 */ 00087 00088 /* Private function prototypes -----------------------------------------------*/ 00089 /** @defgroup STM32L476G_DISCOVERY_ACCELEROMETER_Private_FunctionPrototypes Private Functions 00090 * @{ 00091 */ 00092 /** 00093 * @} 00094 */ 00095 00096 /* Exported functions ---------------------------------------------------------*/ 00097 /** @addtogroup STM32L476G_DISCOVERY_ACCELEROMETER_Exported_Functions 00098 * @{ 00099 */ 00100 00101 /** 00102 * @brief Initialize Accelerometer. 00103 * @retval ACCELERO_OK or ACCELERO_ERROR 00104 */ 00105 uint8_t BSP_ACCELERO_Init(void) 00106 { 00107 uint8_t ret = ACCELERO_ERROR; 00108 uint16_t ctrl = 0x0000; 00109 ACCELERO_InitTypeDef LSM303DLHC_InitStructure; 00110 ACCELERO_FilterConfigTypeDef LSM303DLHC_FilterStructure; 00111 00112 if(Lsm303dlhcDrv.ReadID() == I_AM_LMS303DLHC) 00113 { 00114 /* Initialize the gyroscope driver structure */ 00115 AccelerometerDrv = &Lsm303dlhcDrv; 00116 00117 /* MEMS configuration ------------------------------------------------------*/ 00118 /* Fill the accelerometer structure */ 00119 LSM303DLHC_InitStructure.Power_Mode = LSM303DLHC_NORMAL_MODE; 00120 LSM303DLHC_InitStructure.AccOutput_DataRate = LSM303DLHC_ODR_50_HZ; 00121 LSM303DLHC_InitStructure.Axes_Enable= LSM303DLHC_AXES_ENABLE; 00122 LSM303DLHC_InitStructure.AccFull_Scale = LSM303DLHC_FULLSCALE_2G; 00123 LSM303DLHC_InitStructure.BlockData_Update = LSM303DLHC_BlockUpdate_Continous; 00124 LSM303DLHC_InitStructure.Endianness=LSM303DLHC_BLE_LSB; 00125 LSM303DLHC_InitStructure.High_Resolution=LSM303DLHC_HR_ENABLE; 00126 00127 /* Configure MEMS: data rate, power mode, full scale and axes */ 00128 ctrl |= (LSM303DLHC_InitStructure.Power_Mode | LSM303DLHC_InitStructure.AccOutput_DataRate | \ 00129 LSM303DLHC_InitStructure.Axes_Enable); 00130 00131 ctrl |= ((LSM303DLHC_InitStructure.BlockData_Update | LSM303DLHC_InitStructure.Endianness | \ 00132 LSM303DLHC_InitStructure.AccFull_Scale | LSM303DLHC_InitStructure.High_Resolution) << 8); 00133 00134 /* Configure the accelerometer main parameters */ 00135 AccelerometerDrv->Init(ctrl); 00136 00137 /* Fill the accelerometer LPF structure */ 00138 LSM303DLHC_FilterStructure.HighPassFilter_Mode_Selection =LSM303DLHC_HPM_NORMAL_MODE; 00139 LSM303DLHC_FilterStructure.HighPassFilter_CutOff_Frequency = LSM303DLHC_HPFCF_16; 00140 LSM303DLHC_FilterStructure.HighPassFilter_AOI1 = LSM303DLHC_HPF_AOI1_DISABLE; 00141 LSM303DLHC_FilterStructure.HighPassFilter_AOI2 = LSM303DLHC_HPF_AOI2_DISABLE; 00142 00143 /* Configure MEMS: mode, cutoff frquency, Filter status, Click, AOI1 and AOI2 */ 00144 ctrl = (uint8_t) (LSM303DLHC_FilterStructure.HighPassFilter_Mode_Selection |\ 00145 LSM303DLHC_FilterStructure.HighPassFilter_CutOff_Frequency|\ 00146 LSM303DLHC_FilterStructure.HighPassFilter_AOI1|\ 00147 LSM303DLHC_FilterStructure.HighPassFilter_AOI2); 00148 00149 /* Configure the accelerometer LPF main parameters */ 00150 AccelerometerDrv->FilterConfig(ctrl); 00151 00152 ret = ACCELERO_OK; 00153 } 00154 else 00155 { 00156 ret = ACCELERO_ERROR; 00157 } 00158 00159 return ret; 00160 } 00161 00162 /** 00163 * @brief Reboot memory content of Accelerometer. 00164 * @retval None 00165 */ 00166 void BSP_ACCELERO_Reset(void) 00167 { 00168 if(AccelerometerDrv->Reset != NULL) 00169 { 00170 AccelerometerDrv->Reset(); 00171 } 00172 } 00173 00174 /** 00175 * @brief Get XYZ angular accelerations from the Accelerometer. 00176 * @param pDataXYZ Pointer on 3 angular accelerations table with 00177 * pDataXYZ[0] = X axis, pDataXYZ[1] = Y axis, pDataXYZ[2] = Z axis 00178 * @retval None 00179 */ 00180 void BSP_ACCELERO_GetXYZ(int16_t *pDataXYZ) 00181 { 00182 if(AccelerometerDrv->GetXYZ!= NULL) 00183 { 00184 AccelerometerDrv->GetXYZ(pDataXYZ); 00185 } 00186 } 00187 00188 /** 00189 * @} 00190 */ 00191 00192 /** 00193 * @} 00194 */ 00195 00196 /** 00197 * @} 00198 */ 00199 00200 /** 00201 * @} 00202 */ 00203 00204 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jun 23 2015 17:15:45 for STM32L476G-Discovery BSP User Manual by
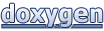