STM32L476G-Discovery BSP User Manual
|
stm32l476g_discovery.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l476g_discovery.c 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief This file provides a set of firmware functions to manage Leds, 00008 * push-button and joystick of STM32L476G-Discovery board (MB1184) 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Includes ------------------------------------------------------------------*/ 00040 #include "stm32l476g_discovery.h" 00041 00042 /** @addtogroup BSP 00043 * @{ 00044 */ 00045 00046 /** @defgroup STM32L476G_DISCOVERY STM32L476G-DISCOVERY 00047 * @{ 00048 */ 00049 00050 /** @defgroup STM32L476G_DISCOVERY_Common STM32L476G-DISCOVERY Common 00051 * @{ 00052 */ 00053 00054 /** @defgroup STM32L476G_DISCOVERY_Private_TypesDefinitions Private Types Definitions 00055 * @brief This file provides firmware functions to manage Leds, push-buttons, 00056 * COM ports, SD card on SPI and temperature sensor (TS751) available on 00057 * STM32L476G-DISCOVERY discoveryuation board from STMicroelectronics. 00058 * @{ 00059 */ 00060 00061 /** 00062 * @} 00063 */ 00064 00065 /** @defgroup STM32L476G_DISCOVERY_Private_Defines Private Defines 00066 * @{ 00067 */ 00068 00069 /** 00070 * @brief STM32L476G DISCOVERY BSP Driver version number $VERSION$ 00071 */ 00072 #define __STM32L476G_DISCOVERY_BSP_VERSION_MAIN (0x00) /*!< [31:24] main version */ 00073 #define __STM32L476G_DISCOVERY_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00074 #define __STM32L476G_DISCOVERY_BSP_VERSION_SUB2 (0x00) /*!< [15:8] sub2 version */ 00075 #define __STM32L476G_DISCOVERY_BSP_VERSION_RC (0x01) /*!< [7:0] release candidate */ 00076 #define __STM32L476G_DISCOVERY_BSP_VERSION ((__STM32L476G_DISCOVERY_BSP_VERSION_MAIN << 24)\ 00077 |(__STM32L476G_DISCOVERY_BSP_VERSION_SUB1 << 16)\ 00078 |(__STM32L476G_DISCOVERY_BSP_VERSION_SUB2 << 8 )\ 00079 |(__STM32L476G_DISCOVERY_BSP_VERSION_RC)) 00080 /** 00081 * @} 00082 */ 00083 00084 00085 /** @defgroup STM32L476G_DISCOVERY_Private_Macros Private Macros 00086 * @{ 00087 */ 00088 00089 /** 00090 * @} 00091 */ 00092 00093 00094 /** @defgroup STM32L476G_DISCOVERY_Exported_Variables Exported Variables 00095 * @{ 00096 */ 00097 00098 /** 00099 * @brief LED variables 00100 */ 00101 #if defined (USE_STM32L476G_DISCO_REVC) || defined (USE_STM32L476G_DISCO_REVB) 00102 GPIO_TypeDef* LED_PORT[LEDn] = {LED4_GPIO_PORT, 00103 LED5_GPIO_PORT}; 00104 00105 const uint16_t LED_PIN[LEDn] = {LED4_PIN, 00106 LED5_PIN}; 00107 #elif defined (USE_STM32L476G_DISCO_REVA) 00108 GPIO_TypeDef* LED_PORT[LEDn] = {LED3_GPIO_PORT, 00109 LED4_GPIO_PORT}; 00110 00111 const uint16_t LED_PIN[LEDn] = {LED3_PIN, 00112 LED4_PIN}; 00113 #endif 00114 00115 00116 /** 00117 * @brief JOYSTICK variables 00118 */ 00119 GPIO_TypeDef* JOY_PORT[JOYn] = {SEL_JOY_GPIO_PORT, 00120 DOWN_JOY_GPIO_PORT, 00121 LEFT_JOY_GPIO_PORT, 00122 RIGHT_JOY_GPIO_PORT, 00123 UP_JOY_GPIO_PORT}; 00124 00125 const uint16_t JOY_PIN[JOYn] = {SEL_JOY_PIN, 00126 LEFT_JOY_PIN, 00127 RIGHT_JOY_PIN, 00128 DOWN_JOY_PIN, 00129 UP_JOY_PIN}; 00130 00131 const uint8_t JOY_IRQn[JOYn] = {SEL_JOY_EXTI_IRQn, 00132 LEFT_JOY_EXTI_IRQn, 00133 RIGHT_JOY_EXTI_IRQn, 00134 DOWN_JOY_EXTI_IRQn, 00135 UP_JOY_EXTI_IRQn}; 00136 00137 /** 00138 * @brief BUS variables 00139 */ 00140 #if defined(HAL_I2C_MODULE_ENABLED) 00141 uint32_t I2c1Timeout = DISCOVERY_I2C2_TIMEOUT_MAX; /*<! Value of Timeout when I2C1 communication fails */ 00142 uint32_t I2c2Timeout = DISCOVERY_I2C2_TIMEOUT_MAX; /*<! Value of Timeout when I2C2 communication fails */ 00143 static I2C_HandleTypeDef I2c1Handle; 00144 static I2C_HandleTypeDef I2c2Handle; 00145 #endif /* HAL_I2C_MODULE_ENABLED */ 00146 00147 #if defined(HAL_SPI_MODULE_ENABLED) 00148 00149 /* LL definition */ 00150 #define __SPI_DIRECTION_2LINES(__HANDLE__) do{\ 00151 CLEAR_BIT((__HANDLE__)->Instance->CR1, SPI_CR1_RXONLY | SPI_CR1_BIDIMODE | SPI_CR1_BIDIOE);\ 00152 }while(0); 00153 00154 #define __SPI_DIRECTION_2LINES_RXONLY(__HANDLE__) do{\ 00155 CLEAR_BIT((__HANDLE__)->Instance->CR1, SPI_CR1_RXONLY | SPI_CR1_BIDIMODE | SPI_CR1_BIDIOE);\ 00156 SET_BIT((__HANDLE__)->Instance->CR1, SPI_CR1_RXONLY);\ 00157 }while(0); 00158 00159 #define __SPI_DIRECTION_1LINE_TX(__HANDLE__) do{\ 00160 CLEAR_BIT((__HANDLE__)->Instance->CR1, SPI_CR1_RXONLY | SPI_CR1_BIDIMODE | SPI_CR1_BIDIOE);\ 00161 SET_BIT((__HANDLE__)->Instance->CR1, SPI_CR1_BIDIMODE | SPI_CR1_BIDIOE);\ 00162 }while(0); 00163 00164 #define __SPI_DIRECTION_1LINE_RX(__HANDLE__) do {\ 00165 CLEAR_BIT((__HANDLE__)->Instance->CR1, SPI_CR1_RXONLY | SPI_CR1_BIDIMODE | SPI_CR1_BIDIOE);\ 00166 SET_BIT((__HANDLE__)->Instance->CR1, SPI_CR1_BIDIMODE);\ 00167 } while(0); 00168 00169 00170 uint32_t SpixTimeout = SPIx_TIMEOUT_MAX; /*<! Value of Timeout when SPI communication fails */ 00171 static SPI_HandleTypeDef SpiHandle; 00172 #endif /* HAL_SPI_MODULE_ENABLED */ 00173 00174 /** 00175 * @} 00176 */ 00177 00178 /** @defgroup STM32L476G_DISCOVERY_Private_FunctionPrototypes Private Functions 00179 * @{ 00180 */ 00181 /**************************** Bus functions ************************************/ 00182 /* I2C2 bus function */ 00183 #if defined(HAL_I2C_MODULE_ENABLED) 00184 static void I2C2_Init(void); 00185 static void I2C2_MspInit(I2C_HandleTypeDef *hi2c); 00186 static void I2C2_DeInit(void); 00187 static void I2C2_MspDeInit(I2C_HandleTypeDef *hi2c); 00188 static void I2C2_WriteData(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t Value); 00189 static HAL_StatusTypeDef I2C2_WriteBuffer(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00190 static uint8_t I2C2_ReadData(uint16_t Addr, uint16_t Reg, uint16_t RegSize); 00191 static HAL_StatusTypeDef I2C2_ReadBuffer(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00192 static void I2C2_Error (void); 00193 00194 static void I2C1_Init(void); 00195 static void I2C1_MspInit(I2C_HandleTypeDef *hi2c); 00196 static void I2C1_DeInit(void); 00197 static void I2C1_MspDeInit(I2C_HandleTypeDef *hi2c); 00198 static HAL_StatusTypeDef I2C1_WriteBuffer(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00199 static HAL_StatusTypeDef I2C1_ReadBuffer(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00200 static void I2C1_Error (void); 00201 #endif/* HAL_I2C_MODULE_ENABLED */ 00202 00203 /* SPIx bus function */ 00204 #if defined(HAL_SPI_MODULE_ENABLED) 00205 static void SPIx_Init(void); 00206 static void SPIx_MspInit(SPI_HandleTypeDef *hspi); 00207 static void SPIx_DeInit(void); 00208 static void SPIx_MspDeInit(void); 00209 static uint8_t SPIx_WriteRead(uint8_t Byte); 00210 static void SPIx_Write(uint8_t byte); 00211 static uint8_t SPIx_Read(void); 00212 #endif 00213 00214 /**************************** Link functions ***********************************/ 00215 #if defined(HAL_I2C_MODULE_ENABLED) 00216 /* Link functions for EEPROM peripheral over I2C */ 00217 void EEPROM_I2C_IO_Init(void); 00218 HAL_StatusTypeDef EEPROM_I2C_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00219 HAL_StatusTypeDef EEPROM_I2C_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00220 HAL_StatusTypeDef EEPROM_I2C_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00221 00222 /* Link functions for Audio Codec peripheral */ 00223 void AUDIO_IO_Init(void); 00224 void AUDIO_IO_DeInit(void); 00225 void AUDIO_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00226 uint8_t AUDIO_IO_Read(uint8_t Addr, uint8_t Reg); 00227 void AUDIO_IO_Delay(uint32_t delay); 00228 #endif/* HAL_I2C_MODULE_ENABLED */ 00229 00230 #if defined(HAL_SPI_MODULE_ENABLED) 00231 /* Link function for COMPASS / ACCELERO peripheral */ 00232 void ACCELERO_IO_Init(void); 00233 void ACCELERO_IO_DeInit(void); 00234 void ACCELERO_IO_ITConfig(void); 00235 void ACCELERO_IO_Write(uint8_t RegisterAddr, uint8_t Value); 00236 uint8_t ACCELERO_IO_Read(uint8_t RegisterAddr); 00237 00238 void MAGNETO_IO_Init(void); 00239 void MAGNETO_IO_DeInit(void); 00240 void MAGNETO_IO_ITConfig(void); 00241 void MAGNETO_IO_Write(uint8_t RegisterAddr, uint8_t Value); 00242 uint8_t MAGNETO_IO_Read(uint8_t RegisterAddr); 00243 00244 00245 /* Link functions for GYRO peripheral */ 00246 void GYRO_IO_Init(void); 00247 void GYRO_IO_DeInit(void); 00248 void GYRO_IO_Write(uint8_t* pBuffer, uint8_t WriteAddr, uint16_t NumByteToWrite); 00249 void GYRO_IO_Read(uint8_t* pBuffer, uint8_t ReadAddr, uint16_t NumByteToRead); 00250 00251 #endif 00252 00253 #if defined(HAL_I2C_MODULE_ENABLED) 00254 /* Link functions IOExpander */ 00255 void IOE_Init(void); 00256 void IOE_ITConfig(void); 00257 void IOE_Delay(uint32_t Delay); 00258 void IOE_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00259 uint8_t IOE_Read(uint8_t Addr, uint8_t Reg); 00260 uint16_t IOE_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00261 00262 /* Link functions for IDD measurment */ 00263 void MFX_IO_Init(void); 00264 void MFX_IO_DeInit(void); 00265 void MFX_IO_ITConfig (void); 00266 void MFX_IO_EnableWakeupPin(void); 00267 void MFX_IO_Wakeup(void); 00268 void MFX_IO_Delay(uint32_t delay); 00269 void MFX_IO_Write(uint16_t addr, uint8_t reg, uint8_t value); 00270 uint8_t MFX_IO_Read(uint16_t addr, uint8_t reg); 00271 void MFX_IO_WriteMultiple(uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00272 uint16_t MFX_IO_ReadMultiple(uint16_t addr, uint8_t reg, uint8_t *buffer, uint16_t length); 00273 #endif/* HAL_I2C_MODULE_ENABLED */ 00274 /** 00275 * @} 00276 */ 00277 00278 /** @defgroup STM32L476G_DISCOVERY_Exported_Functions Exported Functions 00279 * @{ 00280 */ 00281 00282 /** 00283 * @brief This method returns the STM32L476 DISCOVERY BSP Driver revision 00284 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00285 */ 00286 uint32_t BSP_GetVersion(void) 00287 { 00288 return __STM32L476G_DISCOVERY_BSP_VERSION; 00289 } 00290 00291 /** 00292 * @brief This method returns the STM32L476 DISCOVERY supply mode 00293 * @retval Code of current supply mode 00294 * This code can be one of following: 00295 * @arg SUPPLY_MODE_EXTERNAL 00296 * @arg SUPPLY_MODE_BATTERY 00297 */ 00298 SupplyMode_TypeDef BSP_SupplyModeDetection(void) 00299 { 00300 SupplyMode_TypeDef supplymode = SUPPLY_MODE_ERROR; 00301 GPIO_InitTypeDef GPIO_InitStruct; 00302 00303 BATTERY_DETECTION_GPIO_CLK_ENABLE(); 00304 00305 /* COMP GPIO pin configuration */ 00306 GPIO_InitStruct.Pin = BATTERY_DETECTION_PIN; 00307 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00308 GPIO_InitStruct.Pull = GPIO_NOPULL; 00309 GPIO_InitStruct.Speed = GPIO_SPEED_HIGH; 00310 HAL_GPIO_Init(BATTERY_DETECTION_GPIO_PORT, &GPIO_InitStruct); 00311 00312 HAL_Delay(400); 00313 if(HAL_GPIO_ReadPin(BATTERY_DETECTION_GPIO_PORT, GPIO_InitStruct.Pin) != GPIO_PIN_RESET) 00314 { 00315 supplymode = SUPPLY_MODE_EXTERNAL; 00316 } 00317 else 00318 { 00319 supplymode = SUPPLY_MODE_BATTERY; 00320 } 00321 00322 HAL_GPIO_DeInit(BATTERY_DETECTION_GPIO_PORT, GPIO_InitStruct.Pin); 00323 00324 return supplymode; 00325 } 00326 00327 #if defined (USE_STM32L476G_DISCO_REVC) || defined (USE_STM32L476G_DISCO_REVB) 00328 /** 00329 * @brief Configures LED GPIOs. 00330 * @param Led: Specifies the Led to be configured. 00331 * This parameter can be one of following parameters: 00332 * @arg LED4 00333 * @arg LED5 00334 * @retval None 00335 */ 00336 #elif defined (USE_STM32L476G_DISCO_REVA) 00337 /** 00338 * @brief Configures LED GPIOs. 00339 * @param Led: Specifies the Led to be configured. 00340 * This parameter can be one of following parameters: 00341 * @arg LED3 00342 * @arg LED4 00343 * @retval None 00344 */ 00345 #endif 00346 void BSP_LED_Init(Led_TypeDef Led) 00347 { 00348 GPIO_InitTypeDef GPIO_InitStructure; 00349 00350 /* Enable the GPIO_LED clock */ 00351 LEDx_GPIO_CLK_ENABLE(Led); 00352 00353 /* Configure the GPIO_LED pin */ 00354 GPIO_InitStructure.Pin = LED_PIN[Led]; 00355 GPIO_InitStructure.Mode = GPIO_MODE_OUTPUT_PP; 00356 GPIO_InitStructure.Pull = GPIO_NOPULL; 00357 GPIO_InitStructure.Speed = GPIO_SPEED_HIGH; 00358 00359 HAL_GPIO_Init(LED_PORT[Led], &GPIO_InitStructure); 00360 00361 HAL_GPIO_WritePin(LED_PORT[Led], GPIO_InitStructure.Pin, GPIO_PIN_RESET); 00362 } 00363 00364 #if defined (USE_STM32L476G_DISCO_REVC) || defined (USE_STM32L476G_DISCO_REVB) 00365 /** 00366 * @brief Unconfigures LED GPIOs. 00367 * @param Led: Specifies the Led to be unconfigured. 00368 * This parameter can be one of following parameters: 00369 * @arg LED4 00370 * @arg LED5 00371 * @retval None 00372 */ 00373 #elif defined (USE_STM32L476G_DISCO_REVA) 00374 /** 00375 * @brief Unconfigures LED GPIOs. 00376 * @param Led: Specifies the Led to be unconfigured. 00377 * This parameter can be one of following parameters: 00378 * @arg LED3 00379 * @arg LED4 00380 * @retval None 00381 */ 00382 #endif 00383 void BSP_LED_DeInit(Led_TypeDef Led) 00384 { 00385 /* Enable the GPIO_LED clock */ 00386 LEDx_GPIO_CLK_ENABLE(Led); 00387 00388 HAL_GPIO_DeInit(LED_PORT[Led], LED_PIN[Led]); 00389 } 00390 00391 #if defined (USE_STM32L476G_DISCO_REVC) || defined (USE_STM32L476G_DISCO_REVB) 00392 /** 00393 * @brief Turns selected LED On. 00394 * @param Led: Specifies the Led to be set on. 00395 * This parameter can be one of following parameters: 00396 * @arg LED4 00397 * @arg LED5 00398 * @retval None 00399 */ 00400 #elif defined (USE_STM32L476G_DISCO_REVA) 00401 /** 00402 * @brief Turns selected LED On. 00403 * @param Led: Specifies the Led to be set on. 00404 * This parameter can be one of following parameters: 00405 * @arg LED3 00406 * @arg LED4 00407 * @retval None 00408 */ 00409 #endif 00410 void BSP_LED_On(Led_TypeDef Led) 00411 { 00412 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00413 } 00414 00415 #if defined (USE_STM32L476G_DISCO_REVC) || defined (USE_STM32L476G_DISCO_REVB) 00416 /** 00417 * @brief Turns selected LED Off. 00418 * @param Led: Specifies the Led to be set off. 00419 * This parameter can be one of following parameters: 00420 * @arg LED4 00421 * @arg LED5 00422 * @retval None 00423 */ 00424 #elif defined (USE_STM32L476G_DISCO_REVA) 00425 /** 00426 * @brief Turns selected LED Off. 00427 * @param Led: Specifies the Led to be set off. 00428 * This parameter can be one of following parameters: 00429 * @arg LED3 00430 * @arg LED4 00431 * @retval None 00432 */ 00433 #endif 00434 void BSP_LED_Off(Led_TypeDef Led) 00435 { 00436 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00437 } 00438 00439 #if defined (USE_STM32L476G_DISCO_REVC) || defined (USE_STM32L476G_DISCO_REVB) 00440 /** 00441 * @brief Toggles the selected LED. 00442 * @param Led: Specifies the Led to be toggled. 00443 * This parameter can be one of following parameters: 00444 * @arg LED4 00445 * @arg LED5 00446 * @retval None 00447 */ 00448 #elif defined (USE_STM32L476G_DISCO_REVA) 00449 /** 00450 * @brief Toggles the selected LED. 00451 * @param Led: Specifies the Led to be toggled. 00452 * This parameter can be one of following parameters: 00453 * @arg LED3 00454 * @arg LED4 00455 * @retval None 00456 */ 00457 #endif 00458 void BSP_LED_Toggle(Led_TypeDef Led) 00459 { 00460 HAL_GPIO_TogglePin(LED_PORT[Led], LED_PIN[Led]); 00461 } 00462 00463 /** 00464 * @brief Configures all buttons of the joystick in GPIO or EXTI modes. 00465 * @param Joy_Mode: Joystick mode. 00466 * This parameter can be one of the following values: 00467 * @arg JOY_MODE_GPIO: Joystick pins will be used as simple IOs 00468 * @arg JOY_MODE_EXTI: Joystick pins will be connected to EXTI line 00469 * with interrupt generation capability 00470 * @retval HAL_OK: if all initializations are OK. Other value if error. 00471 */ 00472 uint8_t BSP_JOY_Init(JOYMode_TypeDef Joy_Mode) 00473 { 00474 JOYState_TypeDef joykey; 00475 GPIO_InitTypeDef GPIO_InitStruct; 00476 00477 /* Initialized the Joystick. */ 00478 for(joykey = JOY_SEL; joykey < (JOY_SEL + JOYn) ; joykey++) 00479 { 00480 /* Enable the JOY clock */ 00481 JOYx_GPIO_CLK_ENABLE(joykey); 00482 00483 GPIO_InitStruct.Pin = JOY_PIN[joykey]; 00484 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00485 GPIO_InitStruct.Speed = GPIO_SPEED_HIGH; 00486 00487 if (Joy_Mode == JOY_MODE_GPIO) 00488 { 00489 /* Configure Joy pin as input */ 00490 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00491 HAL_GPIO_Init(JOY_PORT[joykey], &GPIO_InitStruct); 00492 } 00493 else if (Joy_Mode == JOY_MODE_EXTI) 00494 { 00495 /* Configure Joy pin as input with External interrupt */ 00496 GPIO_InitStruct.Mode = GPIO_MODE_IT_FALLING; 00497 HAL_GPIO_Init(JOY_PORT[joykey], &GPIO_InitStruct); 00498 00499 /* Enable and set Joy EXTI Interrupt to the lowest priority */ 00500 HAL_NVIC_SetPriority((IRQn_Type)(JOY_IRQn[joykey]), 0x0F, 0x00); 00501 HAL_NVIC_EnableIRQ((IRQn_Type)(JOY_IRQn[joykey])); 00502 } 00503 } 00504 00505 return HAL_OK; 00506 } 00507 00508 /** 00509 * @brief Unonfigures all GPIOs used as buttons of the joystick. 00510 * @retval None. 00511 */ 00512 void BSP_JOY_DeInit(void) 00513 { 00514 JOYState_TypeDef joykey; 00515 00516 /* Initialized the Joystick. */ 00517 for(joykey = JOY_SEL; joykey < (JOY_SEL + JOYn) ; joykey++) 00518 { 00519 /* Enable the JOY clock */ 00520 JOYx_GPIO_CLK_ENABLE(joykey); 00521 00522 HAL_GPIO_DeInit(JOY_PORT[joykey], JOY_PIN[joykey]); 00523 } 00524 } 00525 00526 /** 00527 * @brief Returns the current joystick status. 00528 * @retval Code of the joystick key pressed 00529 * This code can be one of the following values: 00530 * @arg JOY_NONE 00531 * @arg JOY_SEL 00532 * @arg JOY_DOWN 00533 * @arg JOY_LEFT 00534 * @arg JOY_RIGHT 00535 * @arg JOY_UP 00536 */ 00537 JOYState_TypeDef BSP_JOY_GetState(void) 00538 { 00539 JOYState_TypeDef joykey; 00540 00541 for (joykey = JOY_SEL; joykey < (JOY_SEL + JOYn) ; joykey++) 00542 { 00543 if (HAL_GPIO_ReadPin(JOY_PORT[joykey], JOY_PIN[joykey]) == GPIO_PIN_SET) 00544 { 00545 /* Return Code Joystick key pressed */ 00546 return joykey; 00547 } 00548 } 00549 00550 /* No Joystick key pressed */ 00551 return JOY_NONE; 00552 } 00553 00554 /** 00555 * @} 00556 */ 00557 00558 /** @defgroup STM32L476G_DISCOVERY_BusOperations_Functions Bus Operations Functions 00559 * @{ 00560 */ 00561 00562 /******************************************************************************* 00563 BUS OPERATIONS 00564 *******************************************************************************/ 00565 #if defined(HAL_SPI_MODULE_ENABLED) 00566 /******************************* SPI Routines**********************************/ 00567 /** 00568 * @brief SPIx Bus initialization 00569 * @retval None 00570 */ 00571 static void SPIx_Init(void) 00572 { 00573 if(HAL_SPI_GetState(&SpiHandle) == HAL_SPI_STATE_RESET) 00574 { 00575 /* SPI Config */ 00576 SpiHandle.Instance = DISCOVERY_SPIx; 00577 /* SPI baudrate is set to 10 MHz (PCLK2/SPI_BaudRatePrescaler = 80/8 = 10 MHz) 00578 to verify these constraints: 00579 lsm303c SPI interface max baudrate is 10MHz for write/read 00580 PCLK2 frequency is set to 80 MHz 00581 */ 00582 SpiHandle.Init.BaudRatePrescaler = SPI_BAUDRATEPRESCALER_8; 00583 SpiHandle.Init.Direction = SPI_DIRECTION_2LINES; 00584 SpiHandle.Init.CLKPhase = SPI_PHASE_1EDGE; 00585 SpiHandle.Init.CLKPolarity = SPI_POLARITY_LOW; 00586 SpiHandle.Init.CRCCalculation = SPI_CRCCALCULATION_DISABLE; 00587 SpiHandle.Init.CRCPolynomial = 7; 00588 SpiHandle.Init.DataSize = SPI_DATASIZE_8BIT; 00589 SpiHandle.Init.FirstBit = SPI_FIRSTBIT_MSB; 00590 SpiHandle.Init.NSS = SPI_NSS_SOFT; 00591 SpiHandle.Init.TIMode = SPI_TIMODE_DISABLE; 00592 SpiHandle.Init.Mode = SPI_MODE_MASTER; 00593 00594 SPIx_MspInit(&SpiHandle); 00595 HAL_SPI_Init(&SpiHandle); 00596 } 00597 } 00598 00599 /** 00600 * @brief SPI MSP Init 00601 * @param hspi: SPI handle 00602 * @retval None 00603 */ 00604 static void SPIx_MspInit(SPI_HandleTypeDef *hspi) 00605 { 00606 GPIO_InitTypeDef GPIO_InitStructure; 00607 00608 /* Enable SPIx clock */ 00609 DISCOVERY_SPIx_CLOCK_ENABLE(); 00610 00611 /* enable SPIx gpio clock */ 00612 DISCOVERY_SPIx_GPIO_CLK_ENABLE(); 00613 00614 /* configure SPIx SCK, MOSI and MISO */ 00615 GPIO_InitStructure.Pin = (DISCOVERY_SPIx_SCK_PIN | DISCOVERY_SPIx_MOSI_PIN | DISCOVERY_SPIx_MISO_PIN); 00616 GPIO_InitStructure.Mode = GPIO_MODE_AF_PP; 00617 GPIO_InitStructure.Pull = GPIO_NOPULL; // GPIO_PULLDOWN; 00618 GPIO_InitStructure.Speed = GPIO_SPEED_FAST; 00619 GPIO_InitStructure.Alternate = DISCOVERY_SPIx_AF; 00620 HAL_GPIO_Init(DISCOVERY_SPIx_GPIO_PORT, &GPIO_InitStructure); 00621 } 00622 00623 /** 00624 * @brief SPIx Bus Deinitialization 00625 * @retval None 00626 */ 00627 void SPIx_DeInit(void) 00628 { 00629 if(HAL_SPI_GetState(&SpiHandle) != HAL_SPI_STATE_RESET) 00630 { 00631 /* SPI Deinit */ 00632 HAL_SPI_DeInit(&SpiHandle); 00633 SPIx_MspDeInit(); 00634 } 00635 } 00636 00637 /** 00638 * @brief SPI MSP DeInit 00639 * @retval None 00640 */ 00641 static void SPIx_MspDeInit(void) 00642 { 00643 /* enable SPIx gpio clock */ 00644 DISCOVERY_SPIx_GPIO_CLK_ENABLE(); 00645 00646 /* Unconfigure SPIx SCK, MOSI and MISO */ 00647 HAL_GPIO_DeInit(DISCOVERY_SPIx_GPIO_PORT, (DISCOVERY_SPIx_SCK_PIN | DISCOVERY_SPIx_MOSI_PIN | DISCOVERY_SPIx_MISO_PIN)); 00648 00649 DISCOVERY_SPIx_GPIO_FORCE_RESET(); 00650 DISCOVERY_SPIx_GPIO_RELEASE_RESET(); 00651 00652 /* Disable SPIx clock */ 00653 DISCOVERY_SPIx_CLOCK_DISABLE(); 00654 } 00655 00656 /** 00657 * @brief Sends a Byte through the SPI interface and return the Byte received 00658 * from the SPI bus. 00659 * @param Byte : Byte send. 00660 * @retval none. 00661 */ 00662 static uint8_t SPIx_WriteRead(uint8_t Byte) 00663 { 00664 uint8_t receivedbyte; 00665 00666 /* Enable the SPI */ 00667 __HAL_SPI_ENABLE(&SpiHandle); 00668 /* check TXE flag */ 00669 while((SpiHandle.Instance->SR & SPI_FLAG_TXE) != SPI_FLAG_TXE); 00670 00671 /* Write the data */ 00672 *((__IO uint8_t*)&SpiHandle.Instance->DR) = Byte; 00673 00674 while((SpiHandle.Instance->SR & SPI_FLAG_RXNE) != SPI_FLAG_RXNE); 00675 receivedbyte = *((__IO uint8_t*)&SpiHandle.Instance->DR); 00676 00677 /* Wait BSY flag */ 00678 while((SpiHandle.Instance->SR & SPI_FLAG_FTLVL) != SPI_FTLVL_EMPTY); 00679 while((SpiHandle.Instance->SR & SPI_FLAG_BSY) == SPI_FLAG_BSY); 00680 00681 /* disable the SPI */ 00682 __HAL_SPI_DISABLE(&SpiHandle); 00683 00684 return receivedbyte; 00685 } 00686 00687 /** 00688 * @brief Sends a Byte through the SPI interface. 00689 * @param Byte : Byte to send. 00690 * @retval none. 00691 */ 00692 static void SPIx_Write(uint8_t Byte) 00693 { 00694 /* Enable the SPI */ 00695 __HAL_SPI_ENABLE(&SpiHandle); 00696 /* check TXE flag */ 00697 while((SpiHandle.Instance->SR & SPI_FLAG_TXE) != SPI_FLAG_TXE); 00698 00699 /* Write the data */ 00700 *((__IO uint8_t*)&SpiHandle.Instance->DR) = Byte; 00701 00702 /* Wait BSY flag */ 00703 while((SpiHandle.Instance->SR & SPI_FLAG_BSY) == SPI_FLAG_BSY); 00704 00705 /* disable the SPI */ 00706 __HAL_SPI_DISABLE(&SpiHandle); 00707 } 00708 00709 #if defined(__ICCARM__) 00710 #pragma optimize=none 00711 #endif 00712 /** 00713 * @brief Receives a Byte from the SPI bus. 00714 * @retval The received byte value 00715 */ 00716 static uint8_t SPIx_Read(void) 00717 { 00718 uint8_t receivedbyte; 00719 00720 __HAL_SPI_ENABLE(&SpiHandle); 00721 __DSB(); 00722 __DSB(); 00723 __DSB(); 00724 __DSB(); 00725 __DSB(); 00726 __DSB(); 00727 __DSB(); 00728 __DSB(); 00729 __HAL_SPI_DISABLE(&SpiHandle); 00730 00731 while((SpiHandle.Instance->SR & SPI_FLAG_RXNE) != SPI_FLAG_RXNE); 00732 /* read the received data */ 00733 receivedbyte = *(__IO uint8_t *)&SpiHandle.Instance->DR; 00734 00735 /* Wait for the BSY flag reset */ 00736 while((SpiHandle.Instance->SR & SPI_FLAG_BSY) == SPI_FLAG_BSY); 00737 00738 00739 return receivedbyte; 00740 } 00741 #endif /* HAL_SPI_MODULE_ENABLED */ 00742 00743 00744 #if defined(HAL_I2C_MODULE_ENABLED) 00745 /******************************* I2C Routines**********************************/ 00746 /** 00747 * @brief Discovery I2C1 Bus initialization 00748 * @retval None 00749 */ 00750 static void I2C1_Init(void) 00751 { 00752 if(HAL_I2C_GetState(&I2c1Handle) == HAL_I2C_STATE_RESET) 00753 { 00754 I2c1Handle.Instance = DISCOVERY_I2C1; 00755 I2c1Handle.Init.Timing = DISCOVERY_I2C1_TIMING; 00756 I2c1Handle.Init.OwnAddress1 = 0; 00757 I2c1Handle.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00758 I2c1Handle.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00759 I2c1Handle.Init.OwnAddress2 = 0; 00760 I2c1Handle.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00761 I2c1Handle.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00762 00763 /* Init the I2C */ 00764 I2C1_MspInit(&I2c1Handle); 00765 HAL_I2C_Init(&I2c1Handle); 00766 } 00767 } 00768 00769 /** 00770 * @brief Discovery I2C1 MSP Initialization 00771 * @param hi2c: I2C handle 00772 * @retval None 00773 */ 00774 static void I2C1_MspInit(I2C_HandleTypeDef *hi2c) 00775 { 00776 GPIO_InitTypeDef GPIO_InitStructure; 00777 RCC_PeriphCLKInitTypeDef RCC_PeriphCLKInitStruct; 00778 00779 /* IOSV bit MUST be set to access GPIO port G[2:15] */ 00780 __HAL_RCC_PWR_CLK_ENABLE(); 00781 HAL_PWREx_EnableVddIO2(); 00782 00783 if (hi2c->Instance == DISCOVERY_I2C1) 00784 { 00785 /*##-1- Configure the Discovery I2C clock source. The clock is derived from the SYSCLK #*/ 00786 RCC_PeriphCLKInitStruct.PeriphClockSelection = RCC_PERIPHCLK_I2C1; 00787 RCC_PeriphCLKInitStruct.I2c1ClockSelection = RCC_I2C1CLKSOURCE_SYSCLK; 00788 HAL_RCCEx_PeriphCLKConfig(&RCC_PeriphCLKInitStruct); 00789 00790 /*##-2- Configure the GPIOs ################################################*/ 00791 /* Enable GPIO clock */ 00792 DISCOVERY_I2C1_SDA_GPIO_CLK_ENABLE(); 00793 DISCOVERY_I2C1_SCL_GPIO_CLK_ENABLE(); 00794 00795 /* Configure I2C Rx/Tx as alternate function */ 00796 GPIO_InitStructure.Pin = DISCOVERY_I2C1_SCL_PIN | DISCOVERY_I2C1_SDA_PIN; 00797 GPIO_InitStructure.Mode = GPIO_MODE_AF_OD; 00798 GPIO_InitStructure.Pull = GPIO_PULLUP; 00799 GPIO_InitStructure.Speed = GPIO_SPEED_HIGH; 00800 GPIO_InitStructure.Alternate = DISCOVERY_I2C1_SCL_SDA_AF; 00801 HAL_GPIO_Init(DISCOVERY_I2C1_SCL_GPIO_PORT, &GPIO_InitStructure); 00802 00803 /*##-3- Configure the Discovery I2C1 peripheral #######################################*/ 00804 /* Enable Discovery I2C1 clock */ 00805 DISCOVERY_I2C1_CLK_ENABLE(); 00806 00807 /* Force and release the I2C Peripheral Clock Reset */ 00808 DISCOVERY_I2C1_FORCE_RESET(); 00809 DISCOVERY_I2C1_RELEASE_RESET(); 00810 00811 /* Enable and set Discovery I2C1 Interrupt to the highest priority */ 00812 HAL_NVIC_SetPriority(DISCOVERY_I2C1_EV_IRQn, 0x00, 0); 00813 HAL_NVIC_EnableIRQ(DISCOVERY_I2C1_EV_IRQn); 00814 00815 /* Enable and set Discovery I2C1 Interrupt to the highest priority */ 00816 HAL_NVIC_SetPriority(DISCOVERY_I2C1_ER_IRQn, 0x00, 0); 00817 HAL_NVIC_EnableIRQ(DISCOVERY_I2C1_ER_IRQn); 00818 } 00819 } 00820 00821 /** 00822 * @brief Discovery I2C1 Bus Deitialization 00823 * @retval None 00824 */ 00825 static void I2C1_DeInit(void) 00826 { 00827 if(HAL_I2C_GetState(&I2c1Handle) != HAL_I2C_STATE_RESET) 00828 { 00829 /* Deinit the I2C */ 00830 HAL_I2C_DeInit(&I2c1Handle); 00831 I2C1_MspDeInit(&I2c1Handle); 00832 } 00833 } 00834 00835 /** 00836 * @brief Discovery I2C1 MSP Deinitialization 00837 * @param hi2c: I2C handle 00838 * @retval None 00839 */ 00840 static void I2C1_MspDeInit(I2C_HandleTypeDef *hi2c) 00841 { 00842 if(hi2c->Instance == DISCOVERY_I2C1) 00843 { 00844 /*##-1- Unconfigure the GPIOs ################################################*/ 00845 /* Enable GPIO clock */ 00846 DISCOVERY_I2C1_SDA_GPIO_CLK_ENABLE(); 00847 DISCOVERY_I2C1_SCL_GPIO_CLK_ENABLE(); 00848 00849 /* Deinit Rx/Tx pins */ 00850 HAL_GPIO_DeInit(DISCOVERY_I2C1_SCL_GPIO_PORT, (DISCOVERY_I2C1_SCL_PIN | DISCOVERY_I2C1_SDA_PIN)); 00851 00852 /*##-2- Unconfigure the Discovery I2C1 peripheral ############################*/ 00853 /* Force & Release the I2C Peripheral Clock Reset */ 00854 DISCOVERY_I2C1_FORCE_RESET(); 00855 DISCOVERY_I2C1_RELEASE_RESET(); 00856 00857 /* Disable Discovery I2C1 clock */ 00858 DISCOVERY_I2C1_CLK_DISABLE(); 00859 00860 /* Disable Discovery I2C1 interrupts */ 00861 HAL_NVIC_DisableIRQ(DISCOVERY_I2C1_EV_IRQn); 00862 HAL_NVIC_DisableIRQ(DISCOVERY_I2C1_ER_IRQn); 00863 00864 __HAL_RCC_PWR_CLK_ENABLE(); 00865 HAL_PWREx_DisableVddIO2(); 00866 } 00867 } 00868 00869 /** 00870 * @brief Write a value in a register of the device through BUS. 00871 * @param Addr: Device address on BUS Bus. 00872 * @param Reg: The target register address to write 00873 * @param RegSize: The target register size (can be 8BIT or 16BIT) 00874 * @param pBuffer: The target register value to be written 00875 * @param Length: buffer size to be written 00876 * @retval None 00877 */ 00878 static HAL_StatusTypeDef I2C1_WriteBuffer(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00879 { 00880 HAL_StatusTypeDef status = HAL_OK; 00881 00882 status = HAL_I2C_Mem_Write(&I2c1Handle, Addr, (uint16_t)Reg, RegSize, pBuffer, Length, I2c1Timeout); 00883 00884 /* Check the communication status */ 00885 if(status != HAL_OK) 00886 { 00887 /* Re-Initiaize the BUS */ 00888 I2C1_Error(); 00889 } 00890 return status; 00891 } 00892 00893 /** 00894 * @brief Reads multiple data on the BUS. 00895 * @param Addr: I2C Address 00896 * @param Reg: Reg Address 00897 * @param RegSize : The target register size (can be 8BIT or 16BIT) 00898 * @param pBuffer: pointer to read data buffer 00899 * @param Length: length of the data 00900 * @retval 0 if no problems to read multiple data 00901 */ 00902 static HAL_StatusTypeDef I2C1_ReadBuffer(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00903 { 00904 HAL_StatusTypeDef status = HAL_OK; 00905 00906 status = HAL_I2C_Mem_Read(&I2c1Handle, Addr, (uint16_t)Reg, RegSize, pBuffer, Length, I2c1Timeout); 00907 00908 /* Check the communication status */ 00909 if(status != HAL_OK) 00910 { 00911 /* Re-Initiaize the BUS */ 00912 I2C1_Error(); 00913 } 00914 return status; 00915 } 00916 00917 /** 00918 * @brief Discovery I2C1 error treatment function 00919 * @retval None 00920 */ 00921 static void I2C1_Error (void) 00922 { 00923 /* De-initialize the I2C communication BUS */ 00924 HAL_I2C_DeInit(&I2c1Handle); 00925 00926 /* Re- Initiaize the I2C communication BUS */ 00927 I2C1_Init(); 00928 } 00929 00930 /** 00931 * @brief Discovery I2C2 Bus initialization 00932 * @retval None 00933 */ 00934 static void I2C2_Init(void) 00935 { 00936 if(HAL_I2C_GetState(&I2c2Handle) == HAL_I2C_STATE_RESET) 00937 { 00938 I2c2Handle.Instance = DISCOVERY_I2C2; 00939 I2c2Handle.Init.Timing = DISCOVERY_I2C2_TIMING; 00940 I2c2Handle.Init.OwnAddress1 = 0; 00941 I2c2Handle.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00942 I2c2Handle.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00943 I2c2Handle.Init.OwnAddress2 = 0; 00944 I2c2Handle.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00945 I2c2Handle.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00946 00947 /* Init the I2C */ 00948 I2C2_MspInit(&I2c2Handle); 00949 HAL_I2C_Init(&I2c2Handle); 00950 } 00951 } 00952 00953 /** 00954 * @brief Discovery I2C2 MSP Initialization 00955 * @param hi2c: I2C2 handle 00956 * @retval None 00957 */ 00958 static void I2C2_MspInit(I2C_HandleTypeDef *hi2c) 00959 { 00960 GPIO_InitTypeDef GPIO_InitStructure; 00961 RCC_PeriphCLKInitTypeDef RCC_PeriphCLKInitStruct; 00962 00963 if (hi2c->Instance == DISCOVERY_I2C2) 00964 { 00965 /*##-1- Configure the Discovery I2C2 clock source. The clock is derived from the SYSCLK #*/ 00966 RCC_PeriphCLKInitStruct.PeriphClockSelection = RCC_PERIPHCLK_I2C2; 00967 RCC_PeriphCLKInitStruct.I2c2ClockSelection = RCC_I2C2CLKSOURCE_SYSCLK; 00968 HAL_RCCEx_PeriphCLKConfig(&RCC_PeriphCLKInitStruct); 00969 00970 /*##-2- Configure the GPIOs ################################################*/ 00971 /* Enable GPIO clock */ 00972 DISCOVERY_I2C2_SDA_GPIO_CLK_ENABLE(); 00973 DISCOVERY_I2C2_SCL_GPIO_CLK_ENABLE(); 00974 00975 /* Configure I2C Rx/Tx as alternate function */ 00976 GPIO_InitStructure.Pin = DISCOVERY_I2C2_SCL_PIN | DISCOVERY_I2C2_SDA_PIN; 00977 GPIO_InitStructure.Mode = GPIO_MODE_AF_OD; 00978 GPIO_InitStructure.Pull = GPIO_PULLUP; 00979 GPIO_InitStructure.Speed = GPIO_SPEED_HIGH; 00980 GPIO_InitStructure.Alternate = DISCOVERY_I2C2_SCL_SDA_AF; 00981 HAL_GPIO_Init(DISCOVERY_I2C2_SCL_GPIO_PORT, &GPIO_InitStructure); 00982 00983 /*##-3- Configure the Discovery I2C2 peripheral #############################*/ 00984 /* Enable Discovery_I2C2 clock */ 00985 DISCOVERY_I2C2_CLK_ENABLE(); 00986 00987 /* Force and release the I2C Peripheral Clock Reset */ 00988 DISCOVERY_I2C2_FORCE_RESET(); 00989 DISCOVERY_I2C2_RELEASE_RESET(); 00990 00991 /* Enable and set Discovery I2C2 Interrupt to the highest priority */ 00992 HAL_NVIC_SetPriority(DISCOVERY_I2C2_EV_IRQn, 0x00, 0); 00993 HAL_NVIC_EnableIRQ(DISCOVERY_I2C2_EV_IRQn); 00994 00995 /* Enable and set Discovery I2C2 Interrupt to the highest priority */ 00996 HAL_NVIC_SetPriority(DISCOVERY_I2C2_ER_IRQn, 0x00, 0); 00997 HAL_NVIC_EnableIRQ(DISCOVERY_I2C2_ER_IRQn); 00998 } 00999 } 01000 01001 /** 01002 * @brief Discovery I2C2 Bus Deinitialization 01003 * @retval None 01004 */ 01005 static void I2C2_DeInit(void) 01006 { 01007 if(HAL_I2C_GetState(&I2c2Handle) != HAL_I2C_STATE_RESET) 01008 { 01009 /* DeInit the I2C */ 01010 HAL_I2C_DeInit(&I2c2Handle); 01011 I2C2_MspDeInit(&I2c2Handle); 01012 } 01013 } 01014 01015 /** 01016 * @brief Discovery I2C2 MSP DeInitialization 01017 * @param hi2c: I2C2 handle 01018 * @retval None 01019 */ 01020 static void I2C2_MspDeInit(I2C_HandleTypeDef *hi2c) 01021 { 01022 if (hi2c->Instance == DISCOVERY_I2C2) 01023 { 01024 /*##-1- Unconfigure the GPIOs ################################################*/ 01025 /* Enable GPIO clock */ 01026 DISCOVERY_I2C2_SDA_GPIO_CLK_ENABLE(); 01027 DISCOVERY_I2C2_SCL_GPIO_CLK_ENABLE(); 01028 01029 /* Configure I2C Rx/Tx as alternate function */ 01030 HAL_GPIO_DeInit(DISCOVERY_I2C2_SCL_GPIO_PORT, (DISCOVERY_I2C2_SCL_PIN | DISCOVERY_I2C2_SDA_PIN)); 01031 01032 /*##-2- Unconfigure the Discovery I2C2 peripheral ############################*/ 01033 /* Force and release I2C Peripheral */ 01034 DISCOVERY_I2C2_FORCE_RESET(); 01035 DISCOVERY_I2C2_RELEASE_RESET(); 01036 01037 /* Disable Discovery I2C2 clock */ 01038 DISCOVERY_I2C2_CLK_DISABLE(); 01039 01040 /* Disable Discovery I2C2 interrupts */ 01041 HAL_NVIC_DisableIRQ(DISCOVERY_I2C2_EV_IRQn); 01042 HAL_NVIC_DisableIRQ(DISCOVERY_I2C2_ER_IRQn); 01043 } 01044 } 01045 01046 /** 01047 * @brief Write a value in a register of the device through BUS. 01048 * @param Addr: Device address on BUS Bus. 01049 * @param Reg: The target register address to write 01050 * @param RegSize: The target register size (can be 8BIT or 16BIT) 01051 * @param Value: The target register value to be written 01052 * @retval None 01053 */ 01054 static void I2C2_WriteData(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t Value) 01055 { 01056 HAL_StatusTypeDef status = HAL_OK; 01057 01058 status = HAL_I2C_Mem_Write(&I2c2Handle, Addr, (uint16_t)Reg, RegSize, &Value, 1, I2c2Timeout); 01059 01060 /* Check the communication status */ 01061 if(status != HAL_OK) 01062 { 01063 /* Re-Initiaize the BUS */ 01064 I2C2_Error(); 01065 } 01066 } 01067 01068 /** 01069 * @brief Write a value in a register of the device through BUS. 01070 * @param Addr: Device address on BUS Bus. 01071 * @param Reg: The target register address to write 01072 * @param RegSize: The target register size (can be 8BIT or 16BIT) 01073 * @param pBuffer: The target register value to be written 01074 * @param Length: buffer size to be written 01075 * @retval None 01076 */ 01077 static HAL_StatusTypeDef I2C2_WriteBuffer(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 01078 { 01079 HAL_StatusTypeDef status = HAL_OK; 01080 01081 status = HAL_I2C_Mem_Write(&I2c2Handle, Addr, (uint16_t)Reg, RegSize, pBuffer, Length, I2c2Timeout); 01082 01083 /* Check the communication status */ 01084 if(status != HAL_OK) 01085 { 01086 /* Re-Initiaize the BUS */ 01087 I2C2_Error(); 01088 } 01089 01090 return status; 01091 } 01092 01093 /** 01094 * @brief Read a register of the device through BUS 01095 * @param Addr: Device address on BUS 01096 * @param Reg: The target register address to read 01097 * @param RegSize: The target register size (can be 8BIT or 16BIT) 01098 * @retval read register value 01099 */ 01100 static uint8_t I2C2_ReadData(uint16_t Addr, uint16_t Reg, uint16_t RegSize) 01101 { 01102 HAL_StatusTypeDef status = HAL_OK; 01103 uint8_t value = 0x0; 01104 01105 status = HAL_I2C_Mem_Read(&I2c2Handle, Addr, Reg, RegSize, &value, 1, I2c2Timeout); 01106 01107 /* Check the communication status */ 01108 if(status != HAL_OK) 01109 { 01110 /* Re-Initiaize the BUS */ 01111 I2C2_Error(); 01112 } 01113 01114 return value; 01115 } 01116 01117 /** 01118 * @brief Reads multiple data on the BUS. 01119 * @param Addr: I2C Address 01120 * @param Reg: Reg Address 01121 * @param RegSize : The target register size (can be 8BIT or 16BIT) 01122 * @param pBuffer: pointer to read data buffer 01123 * @param Length: length of the data 01124 * @retval 0 if no problems to read multiple data 01125 */ 01126 static HAL_StatusTypeDef I2C2_ReadBuffer(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 01127 { 01128 HAL_StatusTypeDef status = HAL_OK; 01129 01130 status = HAL_I2C_Mem_Read(&I2c2Handle, Addr, (uint16_t)Reg, RegSize, pBuffer, Length, I2c2Timeout); 01131 01132 /* Check the communication status */ 01133 if(status != HAL_OK) 01134 { 01135 /* Re-Initiaize the BUS */ 01136 I2C2_Error(); 01137 } 01138 01139 return status; 01140 } 01141 01142 /** 01143 * @brief Discovery I2C2 error treatment function 01144 * @retval None 01145 */ 01146 static void I2C2_Error (void) 01147 { 01148 /* De-initialize the I2C communication BUS */ 01149 HAL_I2C_DeInit(&I2c2Handle); 01150 01151 /* Re- Initiaize the I2C communication BUS */ 01152 I2C2_Init(); 01153 } 01154 #endif /*HAL_I2C_MODULE_ENABLED*/ 01155 01156 01157 /******************************************************************************* 01158 LINK OPERATIONS 01159 *******************************************************************************/ 01160 #if defined(HAL_SPI_MODULE_ENABLED) 01161 /*********************** LINK ACCELEROMETER ***********************************/ 01162 /** 01163 * @brief Configures COMPASS/ACCELEROMETER io interface. 01164 * @retval None 01165 */ 01166 void ACCELERO_IO_Init(void) 01167 { 01168 GPIO_InitTypeDef GPIO_InitStructure; 01169 01170 /* Enable CS GPIO clock and Configure GPIO PIN for Gyroscope Chip select */ 01171 ACCELERO_CS_GPIO_CLK_ENABLE(); 01172 GPIO_InitStructure.Pin = ACCELERO_CS_PIN; 01173 GPIO_InitStructure.Mode = GPIO_MODE_OUTPUT_PP; 01174 GPIO_InitStructure.Pull = GPIO_NOPULL; 01175 GPIO_InitStructure.Speed = GPIO_SPEED_HIGH; 01176 HAL_GPIO_Init(ACCELERO_CS_GPIO_PORT, &GPIO_InitStructure); 01177 01178 /* Deselect : Chip Select high */ 01179 ACCELERO_CS_HIGH(); 01180 01181 SPIx_Init(); 01182 } 01183 01184 /** 01185 * @brief De-Configures COMPASS/ACCELEROMETER io interface. 01186 * @retval None 01187 */ 01188 void ACCELERO_IO_DeInit(void) 01189 { 01190 GPIO_InitTypeDef GPIO_InitStructure; 01191 01192 /* Enable CS GPIO clock and Configure GPIO PIN for Gyroscope Chip select */ 01193 ACCELERO_CS_GPIO_CLK_ENABLE(); 01194 GPIO_InitStructure.Pin = ACCELERO_CS_PIN; 01195 GPIO_InitStructure.Mode = GPIO_MODE_OUTPUT_PP; 01196 GPIO_InitStructure.Pull = GPIO_NOPULL; 01197 GPIO_InitStructure.Speed = GPIO_SPEED_HIGH; 01198 HAL_GPIO_Init(ACCELERO_CS_GPIO_PORT, &GPIO_InitStructure); 01199 01200 /* Deselect : Chip Select high */ 01201 ACCELERO_CS_HIGH(); 01202 01203 /* Uninitialize SPI bus */ 01204 SPIx_DeInit(); 01205 } 01206 01207 /** 01208 * @brief Configures COMPASS / ACCELERO click IT 01209 * @retval None 01210 */ 01211 void ACCELERO_IO_ITConfig(void) 01212 { 01213 } 01214 01215 /** 01216 * @brief Writes one byte to the COMPASS / ACCELEROMETER. 01217 * @param RegisterAddr specifies the COMPASS / ACCELEROMETER register to be written. 01218 * @param Value : Data to be written 01219 * @retval None 01220 */ 01221 void ACCELERO_IO_Write(uint8_t RegisterAddr, uint8_t Value) 01222 { 01223 ACCELERO_CS_LOW(); 01224 __SPI_DIRECTION_1LINE_TX(&SpiHandle); 01225 /* call SPI Read data bus function */ 01226 SPIx_Write(RegisterAddr); 01227 SPIx_Write(Value); 01228 ACCELERO_CS_HIGH(); 01229 } 01230 01231 /** 01232 * @brief Reads a block of data from the COMPASS / ACCELEROMETER. 01233 * @param RegisterAddr : specifies the COMPASS / ACCELEROMETER internal address register to read from 01234 * @retval ACCELEROMETER register value 01235 */ 01236 uint8_t ACCELERO_IO_Read(uint8_t RegisterAddr) 01237 { 01238 RegisterAddr = RegisterAddr | ((uint8_t)0x80); 01239 ACCELERO_CS_LOW(); 01240 __SPI_DIRECTION_1LINE_TX(&SpiHandle); 01241 SPIx_Write(RegisterAddr); 01242 __SPI_DIRECTION_1LINE_RX(&SpiHandle); 01243 uint8_t val = SPIx_Read(); 01244 ACCELERO_CS_HIGH(); 01245 return val; 01246 } 01247 01248 /********************************* LINK MAGNETO *******************************/ 01249 /** 01250 * @brief Configures COMPASS/MAGNETO SPI interface. 01251 * @retval None 01252 */ 01253 void MAGNETO_IO_Init(void) 01254 { 01255 GPIO_InitTypeDef GPIO_InitStructure; 01256 01257 /* Enable CS GPIO clock and Configure GPIO PIN for Gyroscope Chip select */ 01258 MAGNETO_CS_GPIO_CLK_ENABLE(); 01259 GPIO_InitStructure.Pin = MAGNETO_CS_PIN; 01260 GPIO_InitStructure.Mode = GPIO_MODE_OUTPUT_PP; 01261 GPIO_InitStructure.Pull = GPIO_NOPULL; 01262 GPIO_InitStructure.Speed = GPIO_SPEED_HIGH; 01263 HAL_GPIO_Init(MAGNETO_CS_GPIO_PORT, &GPIO_InitStructure); 01264 01265 /* Deselect : Chip Select high */ 01266 MAGNETO_CS_HIGH(); 01267 01268 SPIx_Init(); 01269 } 01270 01271 /** 01272 * @brief de-Configures COMPASS/MAGNETO SPI interface. 01273 * @retval None 01274 */ 01275 void MAGNETO_IO_DeInit(void) 01276 { 01277 GPIO_InitTypeDef GPIO_InitStructure; 01278 01279 /* Enable CS GPIO clock and Configure GPIO PIN for Gyroscope Chip select */ 01280 MAGNETO_CS_GPIO_CLK_ENABLE(); 01281 GPIO_InitStructure.Pin = MAGNETO_CS_PIN; 01282 GPIO_InitStructure.Mode = GPIO_MODE_OUTPUT_PP; 01283 GPIO_InitStructure.Pull = GPIO_NOPULL; 01284 GPIO_InitStructure.Speed = GPIO_SPEED_HIGH; 01285 HAL_GPIO_Init(MAGNETO_CS_GPIO_PORT, &GPIO_InitStructure); 01286 01287 /* Deselect : Chip Select high */ 01288 MAGNETO_CS_HIGH(); 01289 01290 HAL_GPIO_DeInit(MAGNETO_CS_GPIO_PORT, MAGNETO_INT1_PIN|MAGNETO_DRDY_PIN); 01291 01292 01293 /* Uninitialize SPI bus */ 01294 SPIx_DeInit(); 01295 } 01296 01297 /** 01298 * @brief Writes one byte to the COMPASS/MAGNETO. 01299 * @param RegisterAddr specifies the COMPASS/MAGNETO register to be written. 01300 * @param Value : Data to be written 01301 * @retval None 01302 */ 01303 void MAGNETO_IO_Write(uint8_t RegisterAddr, uint8_t Value) 01304 { 01305 MAGNETO_CS_LOW(); 01306 __SPI_DIRECTION_1LINE_TX(&SpiHandle); 01307 /* call SPI Read data bus function */ 01308 SPIx_Write(RegisterAddr); 01309 SPIx_Write(Value); 01310 MAGNETO_CS_HIGH(); 01311 } 01312 01313 /** 01314 * @brief Reads a block of data from the COMPASS/MAGNETO. 01315 * @param RegisterAddr : specifies the COMPASS/MAGNETO internal address register to read from 01316 * @retval ACCELEROMETER register value 01317 */ 01318 uint8_t MAGNETO_IO_Read(uint8_t RegisterAddr) 01319 { 01320 MAGNETO_CS_LOW(); 01321 __SPI_DIRECTION_1LINE_TX(&SpiHandle); 01322 SPIx_Write(RegisterAddr | 0x80); 01323 __SPI_DIRECTION_1LINE_RX(&SpiHandle); 01324 uint8_t val = SPIx_Read(); 01325 MAGNETO_CS_HIGH(); 01326 return val; 01327 } 01328 01329 /********************************* LINK GYRO *****************************/ 01330 /** 01331 * @brief Configures the GYRO SPI interface. 01332 * @retval None 01333 */ 01334 void GYRO_IO_Init(void) 01335 { 01336 GPIO_InitTypeDef GPIO_InitStructure; 01337 01338 01339 /* Case GYRO not used in the demonstration software except being set in 01340 low power mode. 01341 To avoid access conflicts with accelerometer and magnetometer, 01342 initialize XL_CS and MAG_CS pins then deselect these I/O */ 01343 ACCELERO_CS_GPIO_CLK_ENABLE(); 01344 GPIO_InitStructure.Pin = ACCELERO_CS_PIN; 01345 GPIO_InitStructure.Mode = GPIO_MODE_OUTPUT_PP; 01346 GPIO_InitStructure.Pull = GPIO_NOPULL; 01347 GPIO_InitStructure.Speed = GPIO_SPEED_HIGH; 01348 HAL_GPIO_Init(ACCELERO_CS_GPIO_PORT, &GPIO_InitStructure); 01349 01350 /* Deselect : Chip Select high */ 01351 ACCELERO_CS_HIGH(); 01352 01353 /* Enable CS GPIO clock and Configure GPIO PIN for Gyroscope Chip select */ 01354 MAGNETO_CS_GPIO_CLK_ENABLE(); 01355 GPIO_InitStructure.Pin = MAGNETO_CS_PIN; 01356 GPIO_InitStructure.Mode = GPIO_MODE_OUTPUT_PP; 01357 GPIO_InitStructure.Pull = GPIO_NOPULL; 01358 GPIO_InitStructure.Speed = GPIO_SPEED_HIGH; 01359 HAL_GPIO_Init(MAGNETO_CS_GPIO_PORT, &GPIO_InitStructure); 01360 01361 /* Deselect : Chip Select high */ 01362 MAGNETO_CS_HIGH(); 01363 01364 01365 /* Configure the Gyroscope Control pins ---------------------------------*/ 01366 /* Enable CS GPIO clock and Configure GPIO PIN for Gyroscope Chip select */ 01367 GYRO_CS_GPIO_CLK_ENABLE(); 01368 GPIO_InitStructure.Pin = GYRO_CS_PIN; 01369 GPIO_InitStructure.Mode = GPIO_MODE_OUTPUT_PP; 01370 GPIO_InitStructure.Pull = GPIO_NOPULL; 01371 GPIO_InitStructure.Speed = GPIO_SPEED_HIGH; 01372 HAL_GPIO_Init(GYRO_CS_GPIO_PORT, &GPIO_InitStructure); 01373 01374 /* Deselect : Chip Select high */ 01375 GYRO_CS_HIGH(); 01376 01377 /* Enable INT1, INT2 GPIO clock and Configure GPIO PINs to detect Interrupts */ 01378 GYRO_INT1_GPIO_CLK_ENABLE(); 01379 GPIO_InitStructure.Pin = GYRO_INT1_PIN; 01380 GPIO_InitStructure.Mode = GPIO_MODE_INPUT; 01381 GPIO_InitStructure.Speed = GPIO_SPEED_HIGH; 01382 GPIO_InitStructure.Pull= GPIO_NOPULL; 01383 HAL_GPIO_Init(GYRO_INT1_GPIO_PORT, &GPIO_InitStructure); 01384 01385 GYRO_INT2_GPIO_CLK_ENABLE(); 01386 GPIO_InitStructure.Pin = GYRO_INT2_PIN; 01387 HAL_GPIO_Init(GYRO_INT2_GPIO_PORT, &GPIO_InitStructure); 01388 01389 SPIx_Init(); 01390 01391 } 01392 01393 01394 /** 01395 * @brief de-Configures GYRO SPI interface. 01396 * @retval None 01397 */ 01398 void GYRO_IO_DeInit(void) 01399 { 01400 GPIO_InitTypeDef GPIO_InitStructure; 01401 /* Enable CS GPIO clock */ 01402 GYRO_CS_GPIO_CLK_ENABLE(); 01403 01404 GPIO_InitStructure.Pin = GYRO_CS_PIN; 01405 GPIO_InitStructure.Mode = GPIO_MODE_OUTPUT_PP; 01406 GPIO_InitStructure.Pull = GPIO_NOPULL; 01407 GPIO_InitStructure.Speed = GPIO_SPEED_HIGH; 01408 HAL_GPIO_Init(GYRO_CS_GPIO_PORT, &GPIO_InitStructure); 01409 01410 /* Deselect : Chip Select high */ 01411 GYRO_CS_HIGH(); 01412 01413 GYRO_INT1_GPIO_CLK_ENABLE(); 01414 GYRO_INT2_GPIO_CLK_ENABLE(); 01415 01416 /* Uninitialize the INT1/INT2 Pins */ 01417 HAL_GPIO_DeInit(GYRO_INT1_GPIO_PORT, GYRO_INT1_PIN); 01418 HAL_GPIO_DeInit(GYRO_INT2_GPIO_PORT, GYRO_INT2_PIN); 01419 01420 /* Uninitialize SPI bus */ 01421 SPIx_DeInit(); 01422 } 01423 01424 /** 01425 * @brief Writes one byte to the GYRO. 01426 * @param pBuffer : pointer to the buffer containing the data to be written to the GYRO. 01427 * @param WriteAddr : GYRO's internal address to write to. 01428 * @param NumByteToWrite: Number of bytes to write. 01429 * @retval None 01430 */ 01431 void GYRO_IO_Write(uint8_t* pBuffer, uint8_t WriteAddr, uint16_t NumByteToWrite) 01432 { 01433 /* Configure the MS bit: 01434 - When 0, the address will remain unchanged in multiple read/write commands. 01435 - When 1, the address will be auto incremented in multiple read/write commands. 01436 */ 01437 if(NumByteToWrite > 0x01) 01438 { 01439 WriteAddr |= (uint8_t)MULTIPLEBYTE_CMD; 01440 } 01441 /* Set chip select Low at the start of the transmission */ 01442 GYRO_CS_LOW(); 01443 __SPI_DIRECTION_2LINES(&SpiHandle); 01444 01445 /* Send the Address of the indexed register */ 01446 SPIx_WriteRead(WriteAddr); 01447 01448 /* Send the data that will be written into the device (MSB First) */ 01449 while(NumByteToWrite >= 0x01) 01450 { 01451 SPIx_WriteRead(*pBuffer); 01452 NumByteToWrite--; 01453 pBuffer++; 01454 } 01455 01456 /* Set chip select High at the end of the transmission */ 01457 GYRO_CS_HIGH(); 01458 } 01459 01460 /** 01461 * @brief Reads a block of data from the GYROSCOPE. 01462 * @param pBuffer : pointer to the buffer that receives the data read from the GYROSCOPE. 01463 * @param ReadAddr : GYROSCOPE's internal address to read from. 01464 * @param NumByteToRead : number of bytes to read from the GYROSCOPE. 01465 * @retval None 01466 */ 01467 void GYRO_IO_Read(uint8_t* pBuffer, uint8_t ReadAddr, uint16_t NumByteToRead) 01468 { 01469 if(NumByteToRead > 0x01) 01470 { 01471 ReadAddr |= (uint8_t)(READWRITE_CMD | MULTIPLEBYTE_CMD); 01472 } 01473 else 01474 { 01475 ReadAddr |= (uint8_t)READWRITE_CMD; 01476 } 01477 /* Set chip select Low at the start of the transmission */ 01478 GYRO_CS_LOW(); 01479 __SPI_DIRECTION_2LINES(&SpiHandle); 01480 /* Send the Address of the indexed register */ 01481 SPIx_WriteRead(ReadAddr); 01482 01483 /* Receive the data that will be read from the device (MSB First) */ 01484 while(NumByteToRead > 0x00) 01485 { 01486 /* Send dummy byte (0x00) to generate the SPI clock to GYROSCOPE (Slave device) */ 01487 *pBuffer = SPIx_WriteRead(0x00); 01488 NumByteToRead--; 01489 pBuffer++; 01490 } 01491 01492 /* Set chip select High at the end of the transmission */ 01493 GYRO_CS_HIGH(); 01494 } 01495 #endif /* HAL_SPI_MODULE_ENABLED */ 01496 01497 #if defined(HAL_I2C_MODULE_ENABLED) 01498 /********************************* LINK MFX ***********************************/ 01499 /** 01500 * @brief Initializes MFX low level. 01501 * @retval None 01502 */ 01503 void MFX_IO_Init(void) 01504 { 01505 /* I2C2 init */ 01506 I2C2_Init(); 01507 } 01508 /** 01509 * @brief Deinitializes MFX low level. 01510 * @retval None 01511 */ 01512 void MFX_IO_DeInit(void) 01513 { 01514 GPIO_InitTypeDef GPIO_InitStruct; 01515 01516 /* Enable wakeup gpio clock */ 01517 IDD_WAKEUP_GPIO_CLK_ENABLE(); 01518 01519 /* MFX wakeup pin configuration */ 01520 GPIO_InitStruct.Pin = IDD_WAKEUP_PIN; 01521 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 01522 GPIO_InitStruct.Speed = GPIO_SPEED_LOW; 01523 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 01524 HAL_GPIO_Init(IDD_WAKEUP_GPIO_PORT, &GPIO_InitStruct); 01525 01526 /* DeInit interrupt pin : disable IRQ before to avoid spurious interrupt */ 01527 HAL_NVIC_DisableIRQ((IRQn_Type)(IDD_INT_EXTI_IRQn)); 01528 IDD_INT_GPIO_CLK_ENABLE(); 01529 HAL_GPIO_DeInit(IDD_INT_GPIO_PORT, IDD_INT_PIN); 01530 01531 /* I2C2 Deinit */ 01532 I2C2_DeInit(); 01533 } 01534 01535 /** 01536 * @brief Configures MFX low level interrupt. 01537 * @retval None 01538 */ 01539 void MFX_IO_ITConfig(void) 01540 { 01541 GPIO_InitTypeDef GPIO_InitStruct; 01542 01543 /* Enable the GPIO clock */ 01544 IDD_INT_GPIO_CLK_ENABLE(); 01545 01546 /* MFX_OUT_IRQ (normally used for EXTI_WKUP) */ 01547 GPIO_InitStruct.Pin = IDD_INT_PIN; 01548 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 01549 GPIO_InitStruct.Speed = GPIO_SPEED_HIGH; 01550 GPIO_InitStruct.Mode = GPIO_MODE_IT_RISING; 01551 HAL_GPIO_Init(IDD_INT_GPIO_PORT, &GPIO_InitStruct); 01552 01553 /* Enable and set GPIO EXTI Interrupt to the lowest priority */ 01554 HAL_NVIC_SetPriority((IRQn_Type)(IDD_INT_EXTI_IRQn), 0x0F, 0x0F); 01555 HAL_NVIC_EnableIRQ((IRQn_Type)(IDD_INT_EXTI_IRQn)); 01556 } 01557 01558 /** 01559 * @brief Configures MFX wke up pin. 01560 * @retval None 01561 */ 01562 void MFX_IO_EnableWakeupPin(void) 01563 { 01564 GPIO_InitTypeDef GPIO_InitStruct; 01565 01566 /* Enable wakeup gpio clock */ 01567 IDD_WAKEUP_GPIO_CLK_ENABLE(); 01568 01569 /* MFX wakeup pin configuration */ 01570 GPIO_InitStruct.Pin = IDD_WAKEUP_PIN; 01571 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 01572 GPIO_InitStruct.Speed = GPIO_SPEED_FAST; 01573 GPIO_InitStruct.Pull = GPIO_NOPULL; 01574 HAL_GPIO_Init(IDD_WAKEUP_GPIO_PORT, &GPIO_InitStruct); 01575 } 01576 01577 /** 01578 * @brief Wakeup MFX. 01579 * @retval None 01580 */ 01581 void MFX_IO_Wakeup(void) 01582 { 01583 /* Set Wakeup pin to high to wakeup Idd measurement component from standby mode */ 01584 HAL_GPIO_WritePin(IDD_WAKEUP_GPIO_PORT, IDD_WAKEUP_PIN, GPIO_PIN_SET); 01585 01586 /* Wait */ 01587 HAL_Delay(1); 01588 01589 /* Set gpio pin basck to low */ 01590 HAL_GPIO_WritePin(IDD_WAKEUP_GPIO_PORT, IDD_WAKEUP_PIN, GPIO_PIN_RESET); 01591 } 01592 01593 /** 01594 * @brief MFX writes single data. 01595 * @param Addr: I2C address 01596 * @param Reg: Register address 01597 * @param Value: Data to be written 01598 * @retval None 01599 */ 01600 void MFX_IO_Write(uint16_t Addr, uint8_t Reg, uint8_t Value) 01601 { 01602 I2C2_WriteData(Addr, Reg, I2C_MEMADD_SIZE_8BIT, Value); 01603 } 01604 01605 /** 01606 * @brief MFX reads single data. 01607 * @param Addr: I2C address 01608 * @param Reg: Register address 01609 * @retval Read data 01610 */ 01611 uint8_t MFX_IO_Read(uint16_t Addr, uint8_t Reg) 01612 { 01613 return I2C2_ReadData(Addr, Reg, I2C_MEMADD_SIZE_8BIT); 01614 } 01615 01616 /** 01617 * @brief MFX reads multiple data. 01618 * @param Addr: I2C address 01619 * @param Reg: Register address 01620 * @param Buffer: Pointer to data buffer 01621 * @param Length: Length of the data 01622 * @retval Number of read data 01623 */ 01624 uint16_t MFX_IO_ReadMultiple(uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01625 { 01626 return I2C2_ReadBuffer(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01627 } 01628 01629 /** 01630 * @brief MFX writes multiple data. 01631 * @param Addr: I2C address 01632 * @param Reg: Register address 01633 * @param Buffer: Pointer to data buffer 01634 * @param Length: Length of the data 01635 * @retval None 01636 */ 01637 void MFX_IO_WriteMultiple(uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01638 { 01639 I2C2_WriteBuffer(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01640 } 01641 01642 /** 01643 * @brief MFX delay 01644 * @param Delay: Delay in ms 01645 * @retval None 01646 */ 01647 void MFX_IO_Delay(uint32_t Delay) 01648 { 01649 HAL_Delay(Delay); 01650 } 01651 01652 01653 /********************************* LINK AUDIO *********************************/ 01654 /** 01655 * @brief Initializes Audio low level. 01656 * @retval None 01657 */ 01658 void AUDIO_IO_Init(void) 01659 { 01660 GPIO_InitTypeDef GPIO_InitStruct; 01661 01662 /* Enable Reset GPIO Clock */ 01663 AUDIO_RESET_GPIO_CLK_ENABLE(); 01664 01665 /* Audio reset pin configuration */ 01666 GPIO_InitStruct.Pin = AUDIO_RESET_PIN; 01667 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 01668 GPIO_InitStruct.Speed = GPIO_SPEED_FAST; 01669 GPIO_InitStruct.Pull = GPIO_NOPULL; 01670 HAL_GPIO_Init(AUDIO_RESET_GPIO, &GPIO_InitStruct); 01671 01672 /* I2C bus init */ 01673 I2C1_Init(); 01674 01675 /* Power Down the codec */ 01676 CODEC_AUDIO_POWER_OFF(); 01677 01678 /* wait for a delay to insure registers erasing */ 01679 HAL_Delay(5); 01680 01681 /* Power on the codec */ 01682 CODEC_AUDIO_POWER_ON(); 01683 01684 /* wait for a delay to insure registers erasing */ 01685 HAL_Delay(5); 01686 } 01687 01688 /** 01689 * @brief Deinitializes Audio low level. 01690 * @retval None 01691 */ 01692 void AUDIO_IO_DeInit(void) /* TO DO */ 01693 { 01694 GPIO_InitTypeDef GPIO_InitStruct; 01695 01696 /***********************************************************************/ 01697 /* In case of battery-supplied powered, there is no audio codec-based 01698 features available. Set audio codec I/O default setting */ 01699 /***********************************************************************/ 01700 __HAL_RCC_GPIOE_CLK_ENABLE(); 01701 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP ; 01702 GPIO_InitStruct.Pin = (GPIO_PIN_2 | GPIO_PIN_3 | GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6); 01703 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 01704 GPIO_InitStruct.Speed = GPIO_SPEED_HIGH; 01705 HAL_GPIO_Init(GPIOE, &GPIO_InitStruct); 01706 HAL_GPIO_WritePin(GPIOE, GPIO_PIN_2, GPIO_PIN_RESET); 01707 HAL_GPIO_WritePin(GPIOE, GPIO_PIN_3, GPIO_PIN_RESET); 01708 HAL_GPIO_WritePin(GPIOE, GPIO_PIN_4, GPIO_PIN_RESET); 01709 HAL_GPIO_WritePin(GPIOE, GPIO_PIN_5, GPIO_PIN_RESET); 01710 HAL_GPIO_WritePin(GPIOE, GPIO_PIN_6, GPIO_PIN_RESET); 01711 01712 /* I2C bus Deinit */ 01713 I2C1_DeInit(); 01714 } 01715 01716 /** 01717 * @brief Writes a single data. 01718 * @param Addr: I2C address 01719 * @param Reg: Reg address 01720 * @param Value: Data to be written 01721 * @retval None 01722 */ 01723 void AUDIO_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 01724 { 01725 I2C1_WriteBuffer(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1); 01726 } 01727 01728 /** 01729 * @brief Reads a single data. 01730 * @param Addr: I2C address 01731 * @param Reg: Reg address 01732 * @retval Data to be read 01733 */ 01734 uint8_t AUDIO_IO_Read(uint8_t Addr, uint8_t Reg) 01735 { 01736 uint8_t Read_Value = 0; 01737 01738 I2C1_ReadBuffer((uint16_t) Addr, (uint16_t) Reg, I2C_MEMADD_SIZE_8BIT, &Read_Value, 1); 01739 01740 return Read_Value; 01741 } 01742 01743 /** 01744 * @brief AUDIO Codec delay 01745 * @param Delay: Delay in ms 01746 * @retval None 01747 */ 01748 void AUDIO_IO_Delay(uint32_t Delay) 01749 { 01750 HAL_Delay(Delay); 01751 } 01752 #endif /* HAL_I2C_MODULE_ENABLED */ 01753 01754 /** 01755 * @} 01756 */ 01757 01758 /** 01759 * @} 01760 */ 01761 01762 /** 01763 * @} 01764 */ 01765 01766 /** 01767 * @} 01768 */ 01769 01770 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jun 23 2015 17:15:45 for STM32L476G-Discovery BSP User Manual by
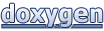