STM32L073Z_EVAL BSP User Manual
|
Functions | |
uint32_t | BSP_GetVersion (void) |
This method returns the STM32F072B EVAL BSP Driver revision. | |
void | BSP_LED_Init (Led_TypeDef Led) |
Configures LED GPIO. | |
void | BSP_LED_On (Led_TypeDef Led) |
Turns selected LED On. | |
void | BSP_LED_Off (Led_TypeDef Led) |
Turns selected LED Off. | |
void | BSP_LED_Toggle (Led_TypeDef Led) |
Toggles the selected LED. | |
void | BSP_PB_Init (Button_TypeDef Button, ButtonMode_TypeDef Mode) |
Configures Tamper Button GPIO or EXTI Line. | |
uint32_t | BSP_PB_GetState (Button_TypeDef Button) |
Returns the selected button state. | |
uint8_t | BSP_JOY_Init (JOYMode_TypeDef Joy_Mode) |
Configures joystick GPIO and EXTI modes. | |
JOYState_TypeDef | BSP_JOY_GetState (void) |
Returns the current joystick status. | |
void | BSP_COM_Init (COM_TypeDef COM, UART_HandleTypeDef *huart) |
Configures COM port. | |
static void | I2C1_Init (void) |
I2C Bus initialization. | |
static void | I2C1_DeInit (void) |
I2C1 Bus Deinitialization. | |
static void | I2C1_Write (uint8_t Addr, uint8_t Reg, uint8_t Value) |
Writes a single data. | |
static uint8_t | I2C1_Read (uint8_t Addr, uint8_t Reg) |
Reads a single data. | |
static HAL_StatusTypeDef | I2C1_ReadBuffer (uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) |
Reads multiple data on the BUS. | |
static HAL_StatusTypeDef | I2C1_IsDeviceReady (uint16_t DevAddress, uint32_t Trials) |
Checks if target device is ready for communication. | |
static HAL_StatusTypeDef | I2C1_WriteBuffer (uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) |
Write a value in a register of the device through BUS. | |
static void | I2C1_Error (void) |
Manages error callback by re-initializing I2C. | |
static void | I2C1_MspInit (I2C_HandleTypeDef *hi2c) |
I2C MSP Initialization. | |
static void | I2C1_MspDeInit (I2C_HandleTypeDef *hi2c) |
I2C1 MSP DeInitialization. | |
static void | SPIx_Init (void) |
SPIx Bus initialization. | |
static uint32_t | SPIx_Read (void) |
SPI Read 1 bytes from device. | |
static void | SPIx_WriteReadData (const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLegnth) |
SPI Write a byte to device. | |
static void | SPIx_Write (uint8_t Value) |
SPI Write a byte to device. | |
static void | SPIx_Error (void) |
SPI error treatment function. | |
static void | SPIx_MspInit (SPI_HandleTypeDef *hspi) |
SPI MSP Init. | |
void | LCD_IO_Init (void) |
Configures the LCD_SPI interface. | |
void | LCD_IO_WriteMultipleData (uint8_t *pData, uint32_t Size) |
Write register value. | |
void | LCD_IO_WriteReg (uint8_t Reg) |
register address. | |
uint16_t | LCD_IO_ReadData (uint16_t Reg) |
Read register value. | |
void | LCD_Delay (uint32_t Delay) |
Wait for loop in ms. | |
void | SD_IO_Init (void) |
Initializes the SD Card and put it into StandBy State (Ready for data transfer). | |
void | SD_IO_WriteReadData (const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength) |
Write a byte on the SD. | |
uint8_t | SD_IO_WriteByte (uint8_t Data) |
Writes a byte on the SD. | |
void | MFX_IO_Init (void) |
Initializes IOE low level. | |
void | MFX_IO_DeInit (void) |
Deinitializes MFX low level. | |
void | MFX_IO_ITConfig (void) |
Configures IOE low level interrupt. | |
void | MFX_IO_EnableWakeupPin (void) |
Configures MFX wke up pin. | |
void | MFX_IO_Wakeup (void) |
Wakeup MFX. | |
void | MFX_IO_Write (uint16_t Addr, uint8_t Reg, uint8_t Value) |
IOE writes single data. | |
uint8_t | MFX_IO_Read (uint16_t Addr, uint8_t Reg) |
IOE reads single data. | |
uint16_t | MFX_IO_ReadMultiple (uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) |
IOE reads multiple data. | |
void | MFX_IO_Delay (uint32_t Delay) |
IOE writes multiple data. | |
void | EEPROM_IO_Init (void) |
Initializes peripherals used by the I2C EEPROM driver. | |
HAL_StatusTypeDef | EEPROM_IO_WriteData (uint16_t DevAddress, uint16_t MemAddress, uint8_t *pBuffer, uint32_t BufferSize) |
Write data to I2C EEPROM driver. | |
HAL_StatusTypeDef | EEPROM_IO_ReadData (uint16_t DevAddress, uint16_t MemAddress, uint8_t *pBuffer, uint32_t BufferSize) |
Read data from I2C EEPROM driver. | |
HAL_StatusTypeDef | EEPROM_IO_IsDeviceReady (uint16_t DevAddress, uint32_t Trials) |
Checks if target device is ready for communication. | |
void | TSENSOR_IO_Init (void) |
Initializes peripherals used by the I2C Temperature Sensor driver. | |
void | TSENSOR_IO_Write (uint16_t DevAddress, uint8_t *pBuffer, uint8_t WriteAddr, uint16_t Length) |
Writes one byte to the TSENSOR. | |
void | TSENSOR_IO_Read (uint16_t DevAddress, uint8_t *pBuffer, uint8_t ReadAddr, uint16_t Length) |
Reads one byte from the TSENSOR. | |
uint16_t | TSENSOR_IO_IsDeviceReady (uint16_t DevAddress, uint32_t Trials) |
Checks if Temperature Sensor is ready for communication. |
Function Documentation
void BSP_COM_Init | ( | COM_TypeDef | COM, |
UART_HandleTypeDef * | huart | ||
) |
Configures COM port.
- Parameters:
-
COM,: Specifies the COM port to be configured. This parameter can be one of following parameters: - COM1
huart,: pointer to a UART_HandleTypeDef structure that contains the configuration information for the specified UART peripheral.
- Return values:
-
None
Definition at line 486 of file stm32l073z_eval.c.
References COM_RX_AF, COM_RX_PIN, COM_RX_PORT, COM_TX_AF, COM_TX_PIN, COM_TX_PORT, COM_USART, COMx_CLK_ENABLE, COMx_RX_GPIO_CLK_ENABLE, and COMx_TX_GPIO_CLK_ENABLE.
uint32_t BSP_GetVersion | ( | void | ) |
This method returns the STM32F072B EVAL BSP Driver revision.
- Parameters:
-
None
- Return values:
-
version : 0xXYZR (8bits for each decimal, R for RC)
Definition at line 244 of file stm32l073z_eval.c.
References __STM32L073Z_EVAL_BSP_VERSION.
JOYState_TypeDef BSP_JOY_GetState | ( | void | ) |
Returns the current joystick status.
- Parameters:
-
None
- Return values:
-
Code of the joystick key pressed This code can be one of the following values: - JOY_NONE
- JOY_SEL
- JOY_DOWN
- JOY_LEFT
- JOY_RIGHT
- JOY_UP
Definition at line 434 of file stm32l073z_eval.c.
References BSP_IO_ReadPin(), JOY_ALL_PINS, JOY_DOWN, JOY_DOWN_PIN, JOY_LEFT, JOY_LEFT_PIN, JOY_NONE, JOY_NONE_PIN, JOY_RIGHT, JOY_RIGHT_PIN, JOY_SEL, JOY_SEL_PIN, JOY_UP, JOY_UP_PIN, and tmp.
uint8_t BSP_JOY_Init | ( | JOYMode_TypeDef | Joy_Mode | ) |
Configures joystick GPIO and EXTI modes.
- Parameters:
-
Joy_Mode,: Button mode. This parameter can be one of the following values: - JOY_MODE_GPIO: Joystick pins will be used as simple IOs
- JOY_MODE_EXTI: Joystick pins will be connected to EXTI line with interrupt generation capability
- Return values:
-
IO_OK,: if all initializations are OK. Other value if error.
Definition at line 397 of file stm32l073z_eval.c.
References BSP_IO_ConfigPin(), BSP_IO_Init(), IO_OK, JOY_ALL_PINS, and JOY_MODE_EXTI.
void BSP_LED_Init | ( | Led_TypeDef | Led | ) |
Configures LED GPIO.
- Parameters:
-
Led,: Specifies the Led to be configured. This parameter can be one of following parameters: - LED1
- LED2
- LED3
- LED4
- Return values:
-
None
Definition at line 263 of file stm32l073z_eval.c.
References LED_PIN, LED_PORT, and LEDx_GPIO_CLK_ENABLE.
void BSP_LED_Off | ( | Led_TypeDef | Led | ) |
Turns selected LED Off.
- Parameters:
-
Led,: Specifies the Led to be set off. This parameter can be one of following parameters: - LED1
- LED2
- LED3
- LED4
- Return values:
-
None
Definition at line 306 of file stm32l073z_eval.c.
void BSP_LED_On | ( | Led_TypeDef | Led | ) |
Turns selected LED On.
- Parameters:
-
Led,: Specifies the Led to be set on. This parameter can be one of following parameters: - LED1
- LED2
- LED3
- LED4
- Return values:
-
None
Definition at line 291 of file stm32l073z_eval.c.
void BSP_LED_Toggle | ( | Led_TypeDef | Led | ) |
Toggles the selected LED.
- Parameters:
-
Led,: Specifies the Led to be toggled. This parameter can be one of following parameters: - LED1
- LED2
- LED3
- LED4
- Return values:
-
None
Definition at line 321 of file stm32l073z_eval.c.
uint32_t BSP_PB_GetState | ( | Button_TypeDef | Button | ) |
Returns the selected button state.
- Parameters:
-
Button,: Button to be checked. This parameter can be one of the following values: - BUTTON_TAMPER: Tamper Push Button
- Return values:
-
The Button GPIO pin value
Definition at line 376 of file stm32l073z_eval.c.
References BUTTON_PIN, and BUTTON_PORT.
void BSP_PB_Init | ( | Button_TypeDef | Button, |
ButtonMode_TypeDef | Mode | ||
) |
Configures Tamper Button GPIO or EXTI Line.
- Parameters:
-
Button,: Button to be configured This parameter can be one of the following values: - BUTTON_TAMPER: Tamper Push Button
Mode,: Button mode This parameter can be one of the following values: - BUTTON_MODE_GPIO: Button will be used as simple IO
- BUTTON_MODE_EXTI: Button will be connected to EXTI line with interrupt generation capability
- Return values:
-
None
Definition at line 338 of file stm32l073z_eval.c.
References BUTTON_IRQn, BUTTON_MODE_EXTI, BUTTON_MODE_GPIO, BUTTON_PIN, BUTTON_PORT, and TAMPERx_GPIO_CLK_ENABLE.
void EEPROM_IO_Init | ( | void | ) |
Initializes peripherals used by the I2C EEPROM driver.
- Parameters:
-
None
- Return values:
-
None
Definition at line 1315 of file stm32l073z_eval.c.
References I2C1_Init().
Referenced by BSP_EEPROM_Init().
HAL_StatusTypeDef EEPROM_IO_IsDeviceReady | ( | uint16_t | DevAddress, |
uint32_t | Trials | ||
) |
Checks if target device is ready for communication.
- Note:
- This function is used with Memory devices
- Parameters:
-
DevAddress,: Target device address Trials,: Number of trials
- Return values:
-
HAL status
Definition at line 1353 of file stm32l073z_eval.c.
References I2C1_IsDeviceReady().
Referenced by BSP_EEPROM_Init(), and BSP_EEPROM_WaitEepromStandbyState().
HAL_StatusTypeDef EEPROM_IO_ReadData | ( | uint16_t | DevAddress, |
uint16_t | MemAddress, | ||
uint8_t * | pBuffer, | ||
uint32_t | BufferSize | ||
) |
Read data from I2C EEPROM driver.
- Parameters:
-
DevAddress,: Target device address MemAddress,: Internal memory address pBuffer,: Pointer to data buffer BufferSize,: Amount of data to be read
- Return values:
-
HAL status
Definition at line 1341 of file stm32l073z_eval.c.
References I2C1_ReadBuffer().
Referenced by BSP_EEPROM_ReadBuffer().
HAL_StatusTypeDef EEPROM_IO_WriteData | ( | uint16_t | DevAddress, |
uint16_t | MemAddress, | ||
uint8_t * | pBuffer, | ||
uint32_t | BufferSize | ||
) |
Write data to I2C EEPROM driver.
- Parameters:
-
DevAddress,: Target device address MemAddress,: Internal memory address pBuffer,: Pointer to data buffer BufferSize,: Amount of data to be sent
- Return values:
-
HAL status
Definition at line 1328 of file stm32l073z_eval.c.
References I2C1_WriteBuffer().
Referenced by EEPROM_WritePage().
static void I2C1_DeInit | ( | void | ) | [static] |
I2C1 Bus Deinitialization.
- Return values:
-
None
Definition at line 551 of file stm32l073z_eval.c.
References heval_I2c1, and I2C1_MspDeInit().
Referenced by MFX_IO_DeInit().
static void I2C1_Error | ( | void | ) | [static] |
Manages error callback by re-initializing I2C.
- Parameters:
-
None
- Return values:
-
None
Definition at line 670 of file stm32l073z_eval.c.
References heval_I2c1, and I2C1_Init().
Referenced by I2C1_Read(), I2C1_ReadBuffer(), I2C1_Write(), and I2C1_WriteBuffer().
static void I2C1_Init | ( | void | ) | [static] |
I2C Bus initialization.
- Parameters:
-
None
- Return values:
-
None
Definition at line 527 of file stm32l073z_eval.c.
References EVAL_I2C1, heval_I2c1, I2C1_MspInit(), and I2C1_TIMING.
Referenced by EEPROM_IO_Init(), I2C1_Error(), MFX_IO_Init(), and TSENSOR_IO_Init().
static HAL_StatusTypeDef I2C1_IsDeviceReady | ( | uint16_t | DevAddress, |
uint32_t | Trials | ||
) | [static] |
Checks if target device is ready for communication.
- Note:
- This function is used with Memory devices
- Parameters:
-
DevAddress,: Target device address Trials,: Number of trials
- Return values:
-
HAL status
Definition at line 636 of file stm32l073z_eval.c.
References heval_I2c1, and I2c1Timeout.
Referenced by EEPROM_IO_IsDeviceReady(), and TSENSOR_IO_IsDeviceReady().
static void I2C1_MspDeInit | ( | I2C_HandleTypeDef * | hi2c | ) | [static] |
I2C1 MSP DeInitialization.
- Parameters:
-
hi2c,: I2C2 handle
- Return values:
-
None
Definition at line 723 of file stm32l073z_eval.c.
References EVAL_I2C1_FORCE_RESET, EVAL_I2C1_GPIO_CLK_ENABLE, EVAL_I2C1_GPIO_PORT, EVAL_I2C1_RELEASE_RESET, EVAL_I2C1_SCL_PIN, and EVAL_I2C1_SDA_PIN.
Referenced by I2C1_DeInit().
static void I2C1_MspInit | ( | I2C_HandleTypeDef * | hi2c | ) | [static] |
I2C MSP Initialization.
- Parameters:
-
hi2c,: I2C handle
- Return values:
-
None
Definition at line 684 of file stm32l073z_eval.c.
References EVAL_I2C1_CLK_ENABLE, EVAL_I2C1_FORCE_RESET, EVAL_I2C1_GPIO_CLK_ENABLE, EVAL_I2C1_GPIO_PORT, EVAL_I2C1_RELEASE_RESET, EVAL_I2C1_SCL_PIN, EVAL_I2C1_SCL_SDA_AF, and EVAL_I2C1_SDA_PIN.
Referenced by I2C1_Init().
static uint8_t I2C1_Read | ( | uint8_t | Addr, |
uint8_t | Reg | ||
) | [static] |
Reads a single data.
- Parameters:
-
Addr,: I2C address Reg,: Register address
- Return values:
-
Read data
Definition at line 587 of file stm32l073z_eval.c.
References heval_I2c1, and I2C1_Error().
Referenced by MFX_IO_Read().
static HAL_StatusTypeDef I2C1_ReadBuffer | ( | uint16_t | Addr, |
uint8_t | Reg, | ||
uint16_t | RegSize, | ||
uint8_t * | pBuffer, | ||
uint16_t | Length | ||
) | [static] |
Reads multiple data on the BUS.
- Parameters:
-
Addr : I2C Address Reg : Reg Address RegSize : The target register size (can be 8BIT or 16BIT) pBuffer : pointer to read data buffer Length : length of the data
- Return values:
-
0 if no problems to read multiple data
Definition at line 614 of file stm32l073z_eval.c.
References heval_I2c1, I2C1_Error(), and I2c1Timeout.
Referenced by EEPROM_IO_ReadData(), MFX_IO_ReadMultiple(), and TSENSOR_IO_Read().
static void I2C1_Write | ( | uint8_t | Addr, |
uint8_t | Reg, | ||
uint8_t | Value | ||
) | [static] |
Writes a single data.
- Parameters:
-
Addr,: I2C address Reg,: Register address Value,: Data to be written
- Return values:
-
None
Definition at line 567 of file stm32l073z_eval.c.
References heval_I2c1, and I2C1_Error().
Referenced by MFX_IO_Write().
static HAL_StatusTypeDef I2C1_WriteBuffer | ( | uint16_t | Addr, |
uint8_t | Reg, | ||
uint16_t | RegSize, | ||
uint8_t * | pBuffer, | ||
uint16_t | Length | ||
) | [static] |
Write a value in a register of the device through BUS.
- Parameters:
-
Addr,: Device address on BUS Bus. Reg,: The target register address to write RegSize,: The target register size (can be 8BIT or 16BIT) pBuffer,: The target register value to be written Length,: buffer size to be written
- Return values:
-
None
Definition at line 650 of file stm32l073z_eval.c.
References heval_I2c1, I2C1_Error(), and I2c1Timeout.
Referenced by EEPROM_IO_WriteData(), and TSENSOR_IO_Write().
void LCD_Delay | ( | uint32_t | Delay | ) |
Wait for loop in ms.
- Parameters:
-
Delay in ms.
- Return values:
-
None
Definition at line 1059 of file stm32l073z_eval.c.
void LCD_IO_Init | ( | void | ) |
Configures the LCD_SPI interface.
- Parameters:
-
None
- Return values:
-
None
Definition at line 926 of file stm32l073z_eval.c.
References LCD_CS_HIGH, LCD_CS_LOW, LCD_NCS_GPIO_CLK_ENABLE, LCD_NCS_GPIO_PORT, LCD_NCS_PIN, and SPIx_Init().
uint16_t LCD_IO_ReadData | ( | uint16_t | Reg | ) |
Read register value.
- Parameters:
-
Reg
- Return values:
-
None
Definition at line 1028 of file stm32l073z_eval.c.
References LCD_CS_HIGH, LCD_CS_LOW, LCD_IO_WriteReg(), LCD_READ_REG, SPIx_Read(), SPIx_Write(), and START_BYTE.
void LCD_IO_WriteMultipleData | ( | uint8_t * | pData, |
uint32_t | Size | ||
) |
Write register value.
- Parameters:
-
pData Pointer on the register value Size Size of byte to transmit to the register
- Return values:
-
None
Definition at line 953 of file stm32l073z_eval.c.
References heval_Spi, LCD_CS_HIGH, LCD_CS_LOW, LCD_WRITE_REG, SPIx_Write(), and START_BYTE.
void LCD_IO_WriteReg | ( | uint8_t | Reg | ) |
register address.
- Parameters:
-
Reg
- Return values:
-
None
Definition at line 1007 of file stm32l073z_eval.c.
References LCD_CS_HIGH, LCD_CS_LOW, SET_INDEX, SPIx_Write(), and START_BYTE.
Referenced by LCD_IO_ReadData().
void MFX_IO_DeInit | ( | void | ) |
Deinitializes MFX low level.
- Return values:
-
None
Definition at line 1176 of file stm32l073z_eval.c.
References I2C1_DeInit().
void MFX_IO_Delay | ( | uint32_t | Delay | ) |
IOE writes multiple data.
- Parameters:
-
Addr,: I2C address Reg,: Register address Buffer,: Pointer to data buffer Length,: Length of the data
- Return values:
-
None IOE delay
- Parameters:
-
Delay,: Delay in ms
- Return values:
-
None
Definition at line 1302 of file stm32l073z_eval.c.
void MFX_IO_EnableWakeupPin | ( | void | ) |
Configures MFX wke up pin.
- Return values:
-
None
Definition at line 1215 of file stm32l073z_eval.c.
References IDD_WAKEUP_GPIO_CLK_ENABLE, IDD_WAKEUP_GPIO_PORT, and IDD_WAKEUP_PIN.
void MFX_IO_Init | ( | void | ) |
Initializes IOE low level.
- Parameters:
-
None
- Return values:
-
None
Definition at line 1167 of file stm32l073z_eval.c.
References I2C1_Init().
void MFX_IO_ITConfig | ( | void | ) |
Configures IOE low level interrupt.
- Parameters:
-
None
- Return values:
-
None
Definition at line 1186 of file stm32l073z_eval.c.
References MFX_IRQOUT_CLK_ENABLE, MFX_IRQOUT_EXTI_IRQn, MFX_IRQOUT_PIN, and MFX_IRQOUT_PORT.
uint8_t MFX_IO_Read | ( | uint16_t | Addr, |
uint8_t | Reg | ||
) |
IOE reads single data.
- Parameters:
-
Addr,: I2C address Reg,: Register address
- Return values:
-
Read data
Definition at line 1264 of file stm32l073z_eval.c.
References I2C1_Read().
uint16_t MFX_IO_ReadMultiple | ( | uint16_t | Addr, |
uint8_t | Reg, | ||
uint8_t * | Buffer, | ||
uint16_t | Length | ||
) |
IOE reads multiple data.
- Parameters:
-
Addr,: I2C address Reg,: Register address Buffer,: Pointer to data buffer Length,: Length of the data
- Return values:
-
Number of read data
Definition at line 1277 of file stm32l073z_eval.c.
References I2C1_ReadBuffer().
void MFX_IO_Wakeup | ( | void | ) |
Wakeup MFX.
- Return values:
-
None
Definition at line 1234 of file stm32l073z_eval.c.
References IDD_WAKEUP_GPIO_PORT, and IDD_WAKEUP_PIN.
void MFX_IO_Write | ( | uint16_t | Addr, |
uint8_t | Reg, | ||
uint8_t | Value | ||
) |
IOE writes single data.
- Parameters:
-
Addr,: I2C address Reg,: Register address Value,: Data to be written
- Return values:
-
None
Definition at line 1253 of file stm32l073z_eval.c.
References I2C1_Write().
void SD_IO_Init | ( | void | ) |
Initializes the SD Card and put it into StandBy State (Ready for data transfer).
- Parameters:
-
None
- Return values:
-
None
Definition at line 1077 of file stm32l073z_eval.c.
References BSP_IO_ConfigPin(), BSP_IO_Init(), IO_OK, SD_CS_GPIO_CLK_ENABLE, SD_CS_GPIO_PORT, SD_CS_HIGH, SD_CS_PIN, SD_DETECT_PIN, SD_DUMMY_BYTE, SD_IO_WriteByte(), and SPIx_Init().
Referenced by BSP_SD_Init().
uint8_t SD_IO_WriteByte | ( | uint8_t | Data | ) |
Writes a byte on the SD.
- Parameters:
-
Data,: byte to send.
- Return values:
-
None
Definition at line 1145 of file stm32l073z_eval.c.
References SPIx_WriteReadData(), and tmp.
Referenced by BSP_SD_Erase(), BSP_SD_GetStatus(), BSP_SD_ReadBlocks(), BSP_SD_WriteBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), SD_GetDataResponse(), SD_GoIdleState(), SD_IO_Init(), SD_ReadData(), SD_SendCmd(), and SD_WaitData().
void SD_IO_WriteReadData | ( | const uint8_t * | DataIn, |
uint8_t * | DataOut, | ||
uint16_t | DataLength | ||
) |
Write a byte on the SD.
- Parameters:
-
Data,: byte to send.
- Return values:
-
None
Definition at line 1132 of file stm32l073z_eval.c.
References SPIx_WriteReadData().
Referenced by BSP_SD_ReadBlocks(), BSP_SD_WriteBlocks(), and SD_SendCmd().
static void SPIx_Error | ( | void | ) | [static] |
SPI error treatment function.
- Parameters:
-
None
- Return values:
-
None
Definition at line 849 of file stm32l073z_eval.c.
References heval_Spi, and SPIx_Init().
Referenced by SPIx_Read(), SPIx_Write(), and SPIx_WriteReadData().
static void SPIx_Init | ( | void | ) | [static] |
SPIx Bus initialization.
- Parameters:
-
None
- Return values:
-
None
Definition at line 752 of file stm32l073z_eval.c.
References EVAL_SPIx, heval_Spi, and SPIx_MspInit().
Referenced by LCD_IO_Init(), SD_IO_Init(), and SPIx_Error().
static void SPIx_MspInit | ( | SPI_HandleTypeDef * | hspi | ) | [static] |
SPI MSP Init.
- Parameters:
-
hspi,: SPI handle
- Return values:
-
None
Definition at line 863 of file stm32l073z_eval.c.
References EVAL_SPIx_CLK_ENABLE, EVAL_SPIx_FORCE_RESET, EVAL_SPIx_MISO_AF, EVAL_SPIx_MISO_GPIO_CLK_ENABLE, EVAL_SPIx_MISO_GPIO_PORT, EVAL_SPIx_MISO_PIN, EVAL_SPIx_MOSI_AF, EVAL_SPIx_MOSI_DIR_GPIO_CLK_ENABLE, EVAL_SPIx_MOSI_DIR_GPIO_PORT, EVAL_SPIx_MOSI_DIR_PIN, EVAL_SPIx_MOSI_GPIO_CLK_ENABLE, EVAL_SPIx_MOSI_GPIO_PORT, EVAL_SPIx_MOSI_PIN, EVAL_SPIx_RELEASE_RESET, EVAL_SPIx_SCK_AF, EVAL_SPIx_SCK_GPIO_CLK_ENABLE, EVAL_SPIx_SCK_GPIO_PORT, and EVAL_SPIx_SCK_PIN.
Referenced by SPIx_Init().
static uint32_t SPIx_Read | ( | void | ) | [static] |
SPI Read 1 bytes from device.
- Parameters:
-
None
- Return values:
-
Read data
Definition at line 786 of file stm32l073z_eval.c.
References heval_Spi, SPIx_Error(), and SpixTimeout.
Referenced by LCD_IO_ReadData().
static void SPIx_Write | ( | uint8_t | Value | ) | [static] |
SPI Write a byte to device.
- Parameters:
-
Value,: value to be written
- Return values:
-
None
Definition at line 829 of file stm32l073z_eval.c.
References heval_Spi, SPIx_Error(), and SpixTimeout.
Referenced by LCD_IO_ReadData(), LCD_IO_WriteMultipleData(), and LCD_IO_WriteReg().
static void SPIx_WriteReadData | ( | const uint8_t * | DataIn, |
uint8_t * | DataOut, | ||
uint16_t | DataLegnth | ||
) | [static] |
SPI Write a byte to device.
- Parameters:
-
Value,: value to be written
- Return values:
-
None
Definition at line 810 of file stm32l073z_eval.c.
References heval_Spi, SPIx_Error(), and SpixTimeout.
Referenced by SD_IO_WriteByte(), and SD_IO_WriteReadData().
void TSENSOR_IO_Init | ( | void | ) |
Initializes peripherals used by the I2C Temperature Sensor driver.
- Parameters:
-
None
- Return values:
-
None
Definition at line 1364 of file stm32l073z_eval.c.
References I2C1_Init().
uint16_t TSENSOR_IO_IsDeviceReady | ( | uint16_t | DevAddress, |
uint32_t | Trials | ||
) |
Checks if Temperature Sensor is ready for communication.
- Parameters:
-
DevAddress,: Target device address Trials,: Number of trials
- Return values:
-
HAL status
Definition at line 1401 of file stm32l073z_eval.c.
References I2C1_IsDeviceReady().
void TSENSOR_IO_Read | ( | uint16_t | DevAddress, |
uint8_t * | pBuffer, | ||
uint8_t | ReadAddr, | ||
uint16_t | Length | ||
) |
Reads one byte from the TSENSOR.
- Parameters:
-
DevAddress,: Target device address pBuffer : pointer to the buffer that receives the data read from the TSENSOR. ReadAddr : TSENSOR's internal address to read from. Length,: Number of data to read
- Return values:
-
None
Definition at line 1390 of file stm32l073z_eval.c.
References I2C1_ReadBuffer().
void TSENSOR_IO_Write | ( | uint16_t | DevAddress, |
uint8_t * | pBuffer, | ||
uint8_t | WriteAddr, | ||
uint16_t | Length | ||
) |
Writes one byte to the TSENSOR.
- Parameters:
-
DevAddress,: Target device address pBuffer,: Pointer to data buffer WriteAddr,: TSENSOR's internal address to write to. Length,: Number of data to write
- Return values:
-
None
Definition at line 1377 of file stm32l073z_eval.c.
References I2C1_WriteBuffer().
Generated on Mon Aug 28 2017 14:54:25 for STM32L073Z_EVAL BSP User Manual by
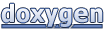