STM32L073Z_EVAL BSP User Manual
|
Go to the source code of this file.
Defines | |
#define | START_BYTE 0x70 |
#define | SET_INDEX 0x00 |
#define | READ_STATUS 0x01 |
#define | LCD_WRITE_REG 0x02 |
#define | LCD_READ_REG 0x03 |
#define | SD_DUMMY_BYTE 0xFF |
#define | SD_NO_RESPONSE_EXPECTED 0x80 |
#define | __STM32L073Z_EVAL_BSP_VERSION_MAIN (0x02) |
STM32L073Z EVAL BSP Driver version number. | |
#define | __STM32L073Z_EVAL_BSP_VERSION_SUB1 (0x00) |
#define | __STM32L073Z_EVAL_BSP_VERSION_SUB2 (0x02) |
#define | __STM32L073Z_EVAL_BSP_VERSION_RC (0x00) |
#define | __STM32L073Z_EVAL_BSP_VERSION |
Functions | |
static void | I2C1_Init (void) |
I2C Bus initialization. | |
static void | I2C1_DeInit (void) |
I2C1 Bus Deinitialization. | |
static void | I2C1_Error (void) |
Manages error callback by re-initializing I2C. | |
static void | I2C1_MspInit (I2C_HandleTypeDef *hi2c) |
I2C MSP Initialization. | |
static void | I2C1_MspDeInit (I2C_HandleTypeDef *hi2c) |
I2C1 MSP DeInitialization. | |
static void | I2C1_Write (uint8_t Addr, uint8_t Reg, uint8_t Value) |
Writes a single data. | |
static uint8_t | I2C1_Read (uint8_t Addr, uint8_t Reg) |
Reads a single data. | |
static HAL_StatusTypeDef | I2C1_WriteBuffer (uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) |
Write a value in a register of the device through BUS. | |
static HAL_StatusTypeDef | I2C1_ReadBuffer (uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) |
Reads multiple data on the BUS. | |
static HAL_StatusTypeDef | I2C1_IsDeviceReady (uint16_t DevAddress, uint32_t Trials) |
Checks if target device is ready for communication. | |
void | EEPROM_IO_Init (void) |
Initializes peripherals used by the I2C EEPROM driver. | |
HAL_StatusTypeDef | EEPROM_IO_WriteData (uint16_t DevAddress, uint16_t MemAddress, uint8_t *pBuffer, uint32_t BufferSize) |
Write data to I2C EEPROM driver. | |
HAL_StatusTypeDef | EEPROM_IO_ReadData (uint16_t DevAddress, uint16_t MemAddress, uint8_t *pBuffer, uint32_t BufferSize) |
Read data from I2C EEPROM driver. | |
HAL_StatusTypeDef | EEPROM_IO_IsDeviceReady (uint16_t DevAddress, uint32_t Trials) |
Checks if target device is ready for communication. | |
void | TSENSOR_IO_Init (void) |
Initializes peripherals used by the I2C Temperature Sensor driver. | |
void | TSENSOR_IO_Write (uint16_t DevAddress, uint8_t *pBuffer, uint8_t WriteAddr, uint16_t Length) |
Writes one byte to the TSENSOR. | |
void | TSENSOR_IO_Read (uint16_t DevAddress, uint8_t *pBuffer, uint8_t ReadAddr, uint16_t Length) |
Reads one byte from the TSENSOR. | |
uint16_t | TSENSOR_IO_IsDeviceReady (uint16_t DevAddress, uint32_t Trials) |
Checks if Temperature Sensor is ready for communication. | |
void | MFX_IO_Init (void) |
Initializes IOE low level. | |
void | MFX_IO_DeInit (void) |
Deinitializes MFX low level. | |
void | MFX_IO_ITConfig (void) |
Configures IOE low level interrupt. | |
void | MFX_IO_EnableWakeupPin (void) |
Configures MFX wke up pin. | |
void | MFX_IO_Wakeup (void) |
Wakeup MFX. | |
void | MFX_IO_Delay (uint32_t Delay) |
IOE writes multiple data. | |
void | MFX_IO_Write (uint16_t Addr, uint8_t Reg, uint8_t Value) |
IOE writes single data. | |
uint8_t | MFX_IO_Read (uint16_t Addr, uint8_t Reg) |
IOE reads single data. | |
uint16_t | MFX_IO_ReadMultiple (uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) |
IOE reads multiple data. | |
static void | SPIx_Init (void) |
SPIx Bus initialization. | |
static void | SPIx_Write (uint8_t Value) |
SPI Write a byte to device. | |
static uint32_t | SPIx_Read (void) |
SPI Read 1 bytes from device. | |
static void | SPIx_Error (void) |
SPI error treatment function. | |
static void | SPIx_MspInit (SPI_HandleTypeDef *hspi) |
SPI MSP Init. | |
static void | SPIx_WriteReadData (const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLegnth) |
SPI Write a byte to device. | |
void | LCD_IO_Init (void) |
Configures the LCD_SPI interface. | |
void | LCD_IO_WriteMultipleData (uint8_t *pData, uint32_t Size) |
Write register value. | |
void | LCD_IO_WriteReg (uint8_t Reg) |
register address. | |
uint16_t | LCD_IO_ReadData (uint16_t Reg) |
Read register value. | |
void | LCD_Delay (uint32_t Delay) |
Wait for loop in ms. | |
void | SD_IO_Init (void) |
Initializes the SD Card and put it into StandBy State (Ready for data transfer). | |
void | SD_IO_CSState (uint8_t state) |
void | SD_IO_WriteReadData (const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength) |
Write a byte on the SD. | |
uint8_t | SD_IO_WriteByte (uint8_t Data) |
Writes a byte on the SD. | |
uint32_t | BSP_GetVersion (void) |
This method returns the STM32F072B EVAL BSP Driver revision. | |
void | BSP_LED_Init (Led_TypeDef Led) |
Configures LED GPIO. | |
void | BSP_LED_On (Led_TypeDef Led) |
Turns selected LED On. | |
void | BSP_LED_Off (Led_TypeDef Led) |
Turns selected LED Off. | |
void | BSP_LED_Toggle (Led_TypeDef Led) |
Toggles the selected LED. | |
void | BSP_PB_Init (Button_TypeDef Button, ButtonMode_TypeDef Mode) |
Configures Tamper Button GPIO or EXTI Line. | |
uint32_t | BSP_PB_GetState (Button_TypeDef Button) |
Returns the selected button state. | |
uint8_t | BSP_JOY_Init (JOYMode_TypeDef Joy_Mode) |
Configures joystick GPIO and EXTI modes. | |
JOYState_TypeDef | BSP_JOY_GetState (void) |
Returns the current joystick status. | |
void | BSP_COM_Init (COM_TypeDef COM, UART_HandleTypeDef *huart) |
Configures COM port. | |
Variables | |
GPIO_TypeDef * | LED_PORT [LEDn] |
LED variables. | |
const uint16_t | LED_PIN [LEDn] |
GPIO_TypeDef * | BUTTON_PORT [BUTTONn] = {TAMPER_BUTTON_GPIO_PORT} |
BUTTON variables. | |
const uint16_t | BUTTON_PIN [BUTTONn] = {TAMPER_BUTTON_PIN} |
const uint8_t | BUTTON_IRQn [BUTTONn] = {TAMPER_BUTTON_EXTI_IRQn} |
USART_TypeDef * | COM_USART [COMn] = {EVAL_COM1} |
COM variables. | |
GPIO_TypeDef * | COM_TX_PORT [COMn] = {EVAL_COM1_TX_GPIO_PORT} |
GPIO_TypeDef * | COM_RX_PORT [COMn] = {EVAL_COM1_RX_GPIO_PORT} |
const uint16_t | COM_TX_PIN [COMn] = {EVAL_COM1_TX_PIN} |
const uint16_t | COM_RX_PIN [COMn] = {EVAL_COM1_RX_PIN} |
const uint16_t | COM_TX_AF [COMn] = {EVAL_COM1_TX_AF} |
const uint16_t | COM_RX_AF [COMn] = {EVAL_COM1_RX_AF} |
uint32_t | I2c1Timeout = EVAL_I2C1_TIMEOUT_MAX |
BUS variables. | |
I2C_HandleTypeDef | heval_I2c1 |
uint32_t | SpixTimeout = EVAL_SPIx_TIMEOUT_MAX |
static SPI_HandleTypeDef | heval_Spi |
Generated on Mon Aug 28 2017 14:54:25 for STM32L073Z_EVAL BSP User Manual by
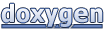