STM32L073Z_EVAL BSP User Manual
|
stm32l073z_eval_eeprom.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l073z_eval_eeprom.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of functions needed to manage a M24LR64 00006 * I2C EEPROM memory. 00007 * 00008 * =================================================================== 00009 * Notes: 00010 * - This driver is intended for STM32L073x families devices only. 00011 * - The I2C EEPROM memory (M24LR64) is available on separate daughter 00012 * board ANT7-M24LR-A, which is provided with the STM32L073Z 00013 * EVAL board. 00014 * =================================================================== 00015 * 00016 * It implements a high level communication layer for read and write 00017 * from/to this memory. The needed STM32L073x hardware resources (I2C and 00018 * GPIO) are defined in stm32l073z_eval.h file, and the initialization is 00019 * performed in EEPROM_IO_Init() function declared in stm32l073z_eval.c 00020 * file. 00021 * You can easily tailor this driver to any other development board, 00022 * by just adapting the defines for hardware resources and 00023 * EEPROM_IO_Init() function. 00024 * 00025 * @note In this driver, basic read and write functions 00026 * (BSP_EEPROM_ReadBuffer() and BSP_EEPROM_WriteBuffer()) 00027 * use Polling mode to perform the data transfer to/from EEPROM memories. 00028 * +-----------------------------------------------------------------+ 00029 * | Pin assignment for M24LR64 EEPROM | 00030 * +---------------------------------------+-----------+-------------+ 00031 * | STM32L073x I2C Pins | sEE | Pin | 00032 * +---------------------------------------+-----------+-------------+ 00033 * | . | E0(GND) | 1 (0V) | 00034 * | . | AC0 | 2 | 00035 * | . | AC1 | 3 | 00036 * | . | VSS | 4 (0V) | 00037 * | sEE_I2C_SDA_PIN/ SDA | SDA | 5 | 00038 * | sEE_I2C_SCL_PIN/ SCL | SCL | 6 | 00039 * | . | E1(GND) | 7 (0V) | 00040 * | . | VDD | 8 (3.3V) | 00041 * +---------------------------------------+-----------+-------------+ 00042 * 00043 ****************************************************************************** 00044 * @attention 00045 * 00046 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00047 * 00048 * Redistribution and use in source and binary forms, with or without modification, 00049 * are permitted provided that the following conditions are met: 00050 * 1. Redistributions of source code must retain the above copyright notice, 00051 * this list of conditions and the following disclaimer. 00052 * 2. Redistributions in binary form must reproduce the above copyright notice, 00053 * this list of conditions and the following disclaimer in the documentation 00054 * and/or other materials provided with the distribution. 00055 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00056 * may be used to endorse or promote products derived from this software 00057 * without specific prior written permission. 00058 * 00059 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00060 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00061 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00062 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00063 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00064 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00065 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00066 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00067 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00068 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00069 * 00070 ****************************************************************************** 00071 */ 00072 /* Includes ------------------------------------------------------------------*/ 00073 #include "stm32l073z_eval_eeprom.h" 00074 00075 /** @addtogroup BSP 00076 * @{ 00077 */ 00078 00079 /** @addtogroup STM32_EVAL 00080 * @{ 00081 */ 00082 00083 /** @addtogroup STM32L073Z_EVAL 00084 * @{ 00085 */ 00086 00087 /** @addtogroup STM32L073Z_EVAL_EEPROM 00088 * @brief This file includes the I2C EEPROM driver of STM32L073Z-EVAL board. 00089 * @{ 00090 */ 00091 00092 /** @defgroup STM32L073Z_EVAL_EEPROM_Private_Types 00093 * @{ 00094 */ 00095 /** 00096 * @} 00097 */ 00098 00099 00100 /** @defgroup STM32L073Z_EVAL_EEPROM_Private_Defines 00101 * @{ 00102 */ 00103 /** 00104 * @} 00105 */ 00106 00107 00108 /** @defgroup STM32L073Z_EVAL_EEPROM_Private_Macros 00109 * @{ 00110 */ 00111 /** 00112 * @} 00113 */ 00114 00115 00116 /** @defgroup STM32L073Z_EVAL_EEPROM_Private_Variables 00117 * @{ 00118 */ 00119 __IO uint16_t EEPROMAddress = 0; 00120 __IO uint16_t EEPROMPageSize = 0; 00121 /** 00122 * @} 00123 */ 00124 00125 00126 /** @defgroup STM32L073Z_EVAL_EEPROM_Private_Function_Prototypes 00127 * @{ 00128 */ 00129 static uint32_t EEPROM_WritePage(uint8_t* pBuffer, uint16_t WriteAddr, uint32_t* NumByteToWrite); 00130 /** 00131 * @} 00132 */ 00133 00134 00135 /** @defgroup STM32L073Z_EVAL_EEPROM_Private_Functions 00136 * @{ 00137 */ 00138 00139 /** 00140 * @brief Initializes peripherals used by the I2C EEPROM driver. 00141 * @param None 00142 * 00143 * @note There are 2 different versions of EEPROM (A01 & A02). 00144 * Then try to connect on 1st one (EEPROM_I2C_ADDRESS_A01) 00145 * and if problem, check the 2nd one (EEPROM_I2C_ADDRESS_A02) 00146 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00147 * different from EEPROM_OK (0) 00148 */ 00149 uint32_t BSP_EEPROM_Init(void) 00150 { 00151 EEPROM_IO_Init(); 00152 00153 /*Select the EEPROM address for M24LR64 A01 and check if OK*/ 00154 EEPROMAddress = EEPROM_ADDRESS_M24LR64_A01; 00155 EEPROMPageSize = EEPROM_PAGESIZE_M24LR64; 00156 if (EEPROM_IO_IsDeviceReady(EEPROMAddress, EEPROM_MAX_TRIALS) != HAL_OK) 00157 { 00158 /*Select the EEPROM address for M24LR64 A02 and check if OK*/ 00159 EEPROMAddress = EEPROM_ADDRESS_M24LR64_A02; 00160 if (EEPROM_IO_IsDeviceReady(EEPROMAddress, EEPROM_MAX_TRIALS) != HAL_OK) 00161 { 00162 return EEPROM_FAIL; 00163 } 00164 } 00165 00166 return EEPROM_OK; 00167 } 00168 00169 /** 00170 * @brief Reads a block of data from the EEPROM. 00171 * @param pBuffer : pointer to the buffer that receives the data read from 00172 * the EEPROM. 00173 * @param ReadAddr : EEPROM's internal address to start reading from. 00174 * @param NumByteToRead : pointer to the variable holding number of bytes to 00175 * be read from the EEPROM. 00176 * 00177 * @note The variable pointed by NumByteToRead is reset to 0 when all the 00178 * data are read from the EEPROM. Application should monitor this 00179 * variable in order know when the transfer is complete. 00180 * 00181 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00182 * different from EEPROM_OK (0) or the timeout user callback. 00183 */ 00184 uint32_t BSP_EEPROM_ReadBuffer(uint8_t* pBuffer, uint16_t ReadAddr, uint32_t* NumByteToRead) 00185 { 00186 uint32_t buffersize = *NumByteToRead; 00187 00188 if (EEPROM_IO_ReadData(EEPROMAddress, ReadAddr, pBuffer, buffersize) != HAL_OK) 00189 { 00190 return EEPROM_FAIL; 00191 } 00192 00193 /* If all operations OK, return EEPROM_OK (0) */ 00194 return EEPROM_OK; 00195 } 00196 00197 /** 00198 * @brief Writes more than one byte to the EEPROM with a single WRITE cycle. 00199 * 00200 * @note The number of bytes (combined to write start address) must not 00201 * cross the EEPROM page boundary. This function can only write into 00202 * the boundaries of an EEPROM page. 00203 * This function doesn't check on boundaries condition (in this driver 00204 * the function EEPROM_WriteBuffer() which calls EEPROM_WritePage() is 00205 * responsible of checking on Page boundaries). 00206 * 00207 * @param pBuffer : pointer to the buffer containing the data to be written to 00208 * the EEPROM. 00209 * @param WriteAddr : EEPROM's internal address to write to. 00210 * @param NumByteToWrite : pointer to the variable holding number of bytes to 00211 * be written into the EEPROM. 00212 * 00213 * @note The variable pointed by NumByteToWrite is reset to 0 when all the 00214 * data are written to the EEPROM. Application should monitor this 00215 * variable in order know when the transfer is complete. 00216 * 00217 * @note This function just configure the communication and enable the DMA 00218 * channel to transfer data. Meanwhile, the user application may perform 00219 * other tasks in parallel. 00220 * 00221 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00222 * different from EEPROM_OK (0) or the timeout user callback. 00223 */ 00224 static uint32_t EEPROM_WritePage(uint8_t* pBuffer, uint16_t WriteAddr, uint32_t* NumByteToWrite) 00225 { 00226 uint32_t buffersize = *NumByteToWrite; 00227 00228 if (EEPROM_IO_WriteData(EEPROMAddress, WriteAddr, pBuffer, buffersize) != HAL_OK) 00229 { 00230 return EEPROM_FAIL; 00231 } 00232 00233 /* Wait for EEPROM Standby state */ 00234 if (BSP_EEPROM_WaitEepromStandbyState() != EEPROM_OK) 00235 { 00236 return EEPROM_FAIL; 00237 } 00238 00239 /* If all operations OK, return EEPROM_OK (0) */ 00240 return EEPROM_OK; 00241 } 00242 00243 /** 00244 * @brief Writes buffer of data to the I2C EEPROM. 00245 * @param pBuffer : pointer to the buffer containing the data to be written 00246 * to the EEPROM. 00247 * @param WriteAddr : EEPROM's internal address to write to. 00248 * @param NumByteToWrite : number of bytes to write to the EEPROM. 00249 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00250 * different from EEPROM_OK (0) or the timeout user callback. 00251 */ 00252 uint32_t BSP_EEPROM_WriteBuffer(uint8_t* pBuffer, uint16_t WriteAddr, uint32_t NumByteToWrite) 00253 { 00254 uint16_t numofpage = 0, numofsingle = 0, count = 0; 00255 uint16_t addr = 0; 00256 uint32_t dataindex = 0; 00257 uint32_t status = EEPROM_OK; 00258 00259 addr = WriteAddr % EEPROMPageSize; 00260 count = EEPROMPageSize - addr; 00261 numofpage = NumByteToWrite / EEPROMPageSize; 00262 numofsingle = NumByteToWrite % EEPROMPageSize; 00263 00264 /*!< If WriteAddr is EEPROM_PAGESIZE aligned */ 00265 if(addr == 0) 00266 { 00267 /*!< If NumByteToWrite < EEPROM_PAGESIZE */ 00268 if(numofpage == 0) 00269 { 00270 /* Store the number of data to be written */ 00271 dataindex = numofsingle; 00272 /* Start writing data */ 00273 status = EEPROM_WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00274 if (status != EEPROM_OK) 00275 { 00276 return status; 00277 } 00278 } 00279 /*!< If NumByteToWrite > EEPROM_PAGESIZE */ 00280 else 00281 { 00282 while(numofpage--) 00283 { 00284 /* Store the number of data to be written */ 00285 dataindex = EEPROMPageSize; 00286 status = EEPROM_WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00287 if (status != EEPROM_OK) 00288 { 00289 return status; 00290 } 00291 00292 WriteAddr += EEPROMPageSize; 00293 pBuffer += EEPROMPageSize; 00294 } 00295 00296 if(numofsingle!=0) 00297 { 00298 /* Store the number of data to be written */ 00299 dataindex = numofsingle; 00300 status = EEPROM_WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00301 if (status != EEPROM_OK) 00302 { 00303 return status; 00304 } 00305 } 00306 } 00307 } 00308 /*!< If WriteAddr is not EEPROM_PAGESIZE aligned */ 00309 else 00310 { 00311 /*!< If NumByteToWrite < EEPROM_PAGESIZE */ 00312 if(numofpage== 0) 00313 { 00314 /*!< If the number of data to be written is more than the remaining space 00315 in the current page: */ 00316 if (NumByteToWrite > count) 00317 { 00318 /* Store the number of data to be written */ 00319 dataindex = count; 00320 /*!< Write the data contained in same page */ 00321 status = EEPROM_WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00322 if (status != EEPROM_OK) 00323 { 00324 return status; 00325 } 00326 00327 /* Store the number of data to be written */ 00328 dataindex = (NumByteToWrite - count); 00329 /*!< Write the remaining data in the following page */ 00330 status = EEPROM_WritePage((uint8_t*)(pBuffer + count), (WriteAddr + count), (uint32_t*)(&dataindex)); 00331 if (status != EEPROM_OK) 00332 { 00333 return status; 00334 } 00335 } 00336 else 00337 { 00338 /* Store the number of data to be written */ 00339 dataindex = numofsingle; 00340 status = EEPROM_WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00341 if (status != EEPROM_OK) 00342 { 00343 return status; 00344 } 00345 } 00346 } 00347 /*!< If NumByteToWrite > EEPROM_PAGESIZE */ 00348 else 00349 { 00350 NumByteToWrite -= count; 00351 numofpage = NumByteToWrite / EEPROMPageSize; 00352 numofsingle = NumByteToWrite % EEPROMPageSize; 00353 00354 if(count != 0) 00355 { 00356 /* Store the number of data to be written */ 00357 dataindex = count; 00358 status = EEPROM_WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00359 if (status != EEPROM_OK) 00360 { 00361 return status; 00362 } 00363 WriteAddr += count; 00364 pBuffer += count; 00365 } 00366 00367 while(numofpage--) 00368 { 00369 /* Store the number of data to be written */ 00370 dataindex = EEPROMPageSize; 00371 status = EEPROM_WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00372 if (status != EEPROM_OK) 00373 { 00374 return status; 00375 } 00376 WriteAddr += EEPROMPageSize; 00377 pBuffer += EEPROMPageSize; 00378 } 00379 if(numofsingle != 0) 00380 { 00381 /* Store the number of data to be written */ 00382 dataindex = numofsingle; 00383 status = EEPROM_WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00384 if (status != EEPROM_OK) 00385 { 00386 return status; 00387 } 00388 } 00389 } 00390 } 00391 00392 /* If all operations OK, return EEPROM_OK (0) */ 00393 return EEPROM_OK; 00394 } 00395 00396 /** 00397 * @brief Wait for EEPROM Standby state. 00398 * 00399 * @note This function allows to wait and check that EEPROM has finished the 00400 * last operation. It is mostly used after Write operation: after receiving 00401 * the buffer to be written, the EEPROM may need additional time to actually 00402 * perform the write operation. During this time, it doesn't answer to 00403 * I2C packets addressed to it. Once the write operation is complete 00404 * the EEPROM responds to its address. 00405 * 00406 * @param None 00407 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00408 * different from EEPROM_OK (0) or the timeout user callback. 00409 */ 00410 uint32_t BSP_EEPROM_WaitEepromStandbyState(void) 00411 { 00412 /* Check if the maximum allowed number of trials has bee reached */ 00413 if (EEPROM_IO_IsDeviceReady(EEPROMAddress, EEPROM_MAX_TRIALS) != HAL_OK) 00414 { 00415 /* If the maximum number of trials has been reached, exit the function */ 00416 BSP_EEPROM_TIMEOUT_UserCallback(); 00417 return EEPROM_TIMEOUT; 00418 } 00419 return EEPROM_OK; 00420 } 00421 00422 /** 00423 * @brief Basic management of the timeout situation. 00424 * @param None. 00425 * @retval None. 00426 */ 00427 __weak void BSP_EEPROM_TIMEOUT_UserCallback(void) 00428 { 00429 } 00430 00431 /** 00432 * @} 00433 */ 00434 00435 /** 00436 * @} 00437 */ 00438 00439 /** 00440 * @} 00441 */ 00442 00443 /** 00444 * @} 00445 */ 00446 00447 /** 00448 * @} 00449 */ 00450 00451 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Mon Aug 28 2017 14:54:25 for STM32L073Z_EVAL BSP User Manual by
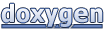