STM32L073Z_EVAL BSP User Manual
|
stm32l073z_eval.c
Go to the documentation of this file.
00001 /****************************************************************************** 00002 * @file stm32l073z_eval.c 00003 * @author MCD Application Team 00004 * @brief This file provides: a set of firmware functions to manage Leds, 00005 * push-button and COM ports 00006 ****************************************************************************** 00007 * @attention 00008 * 00009 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00010 * 00011 * Redistribution and use in source and binary forms, with or without modification, 00012 * are permitted provided that the following conditions are met: 00013 * 1. Redistributions of source code must retain the above copyright notice, 00014 * this list of conditions and the following disclaimer. 00015 * 2. Redistributions in binary form must reproduce the above copyright notice, 00016 * this list of conditions and the following disclaimer in the documentation 00017 * and/or other materials provided with the distribution. 00018 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00019 * may be used to endorse or promote products derived from this software 00020 * without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00023 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00024 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00025 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00026 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00027 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00028 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00029 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00030 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00031 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00032 * 00033 ****************************************************************************** 00034 */ 00035 00036 /* Includes ------------------------------------------------------------------*/ 00037 #include "stm32l073z_eval.h" 00038 #include "stm32l073z_eval_io.h" 00039 00040 /** @addtogroup BSP 00041 * @{ 00042 */ 00043 00044 /** @addtogroup STM32L073Z_EVAL 00045 * @{ 00046 */ 00047 00048 /** @defgroup STM32L073Z_EVAL_LOW_LEVEL 00049 * @brief This file provides firmware functions to manage Leds, push-buttons, 00050 * COM ports, SD card on SPI and temperature sensor (LM75) available on 00051 * STM32L073Z-EVAL evaluation board from STMicroelectronics. 00052 * @{ 00053 */ 00054 00055 /** @defgroup STM32L073Z_EVAL_LOW_LEVEL_Private_TypesDefinitions 00056 * @{ 00057 */ 00058 /** 00059 * @} 00060 */ 00061 00062 00063 /** @defgroup STM32L073Z_EVAL_LOW_LEVEL_Private_Defines 00064 * @{ 00065 */ 00066 /* LINK LCD */ 00067 #define START_BYTE 0x70 00068 #define SET_INDEX 0x00 00069 #define READ_STATUS 0x01 00070 #define LCD_WRITE_REG 0x02 00071 #define LCD_READ_REG 0x03 00072 00073 /* LINK SD Card */ 00074 #define SD_DUMMY_BYTE 0xFF 00075 #define SD_NO_RESPONSE_EXPECTED 0x80 00076 00077 /** 00078 * @brief STM32L073Z EVAL BSP Driver version number 00079 */ 00080 #define __STM32L073Z_EVAL_BSP_VERSION_MAIN (0x02) /*!< [31:24] main version */ 00081 #define __STM32L073Z_EVAL_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00082 #define __STM32L073Z_EVAL_BSP_VERSION_SUB2 (0x02) /*!< [15:8] sub2 version */ 00083 #define __STM32L073Z_EVAL_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00084 #define __STM32L073Z_EVAL_BSP_VERSION ((__STM32L073Z_EVAL_BSP_VERSION_MAIN << 24)\ 00085 |(__STM32L073Z_EVAL_BSP_VERSION_SUB1 << 16)\ 00086 |(__STM32L073Z_EVAL_BSP_VERSION_SUB2 << 8 )\ 00087 |(__STM32L073Z_EVAL_BSP_VERSION_RC)) 00088 /** 00089 * @} 00090 */ 00091 00092 00093 /** @defgroup STM32L073Z_EVAL_LOW_LEVEL_Private_Macros 00094 * @{ 00095 */ 00096 /** 00097 * @} 00098 */ 00099 00100 00101 /** @defgroup STM32L073Z_EVAL_LOW_LEVEL_Private_Variables 00102 * @{ 00103 */ 00104 /** 00105 * @brief LED variables 00106 */ 00107 GPIO_TypeDef* LED_PORT[LEDn] = {LED1_GPIO_PORT, 00108 LED2_GPIO_PORT, 00109 LED3_GPIO_PORT, 00110 LED4_GPIO_PORT}; 00111 00112 const uint16_t LED_PIN[LEDn] = {LED1_PIN, 00113 LED2_PIN, 00114 LED3_PIN, 00115 LED4_PIN}; 00116 /** 00117 * @brief BUTTON variables 00118 */ 00119 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {TAMPER_BUTTON_GPIO_PORT}; 00120 const uint16_t BUTTON_PIN[BUTTONn] = {TAMPER_BUTTON_PIN}; 00121 const uint8_t BUTTON_IRQn[BUTTONn] = {TAMPER_BUTTON_EXTI_IRQn}; 00122 00123 00124 /** 00125 * @brief COM variables 00126 */ 00127 #ifdef HAL_UART_MODULE_ENABLED 00128 USART_TypeDef* COM_USART[COMn] = {EVAL_COM1}; 00129 00130 GPIO_TypeDef* COM_TX_PORT[COMn] = {EVAL_COM1_TX_GPIO_PORT}; 00131 00132 GPIO_TypeDef* COM_RX_PORT[COMn] = {EVAL_COM1_RX_GPIO_PORT}; 00133 00134 const uint16_t COM_TX_PIN[COMn] = {EVAL_COM1_TX_PIN}; 00135 00136 const uint16_t COM_RX_PIN[COMn] = {EVAL_COM1_RX_PIN}; 00137 00138 const uint16_t COM_TX_AF[COMn] = {EVAL_COM1_TX_AF}; 00139 00140 const uint16_t COM_RX_AF[COMn] = {EVAL_COM1_RX_AF}; 00141 00142 #endif /*HAL_UART_MODULE_ENABLED*/ 00143 00144 /** 00145 * @brief BUS variables 00146 */ 00147 #if defined(HAL_I2C_MODULE_ENABLED) 00148 uint32_t I2c1Timeout = EVAL_I2C1_TIMEOUT_MAX; /*<! Value of Timeout when I2C1 communication fails */ 00149 I2C_HandleTypeDef heval_I2c1; 00150 #endif /* HAL_I2C_MODULE_ENABLED */ 00151 00152 #if defined(HAL_SPI_MODULE_ENABLED) 00153 uint32_t SpixTimeout = EVAL_SPIx_TIMEOUT_MAX; /*<! Value of Timeout when SPI communication fails */ 00154 static SPI_HandleTypeDef heval_Spi; 00155 #endif /* HAL_SPI_MODULE_ENABLED */ 00156 00157 /** 00158 * @} 00159 */ 00160 00161 00162 /** @defgroup STM32L073Z_EVAL_LOW_LEVEL_Private_FunctionPrototypes 00163 * @{ 00164 */ 00165 #if defined(HAL_I2C_MODULE_ENABLED) 00166 /* I2C1 bus function */ 00167 /* Link function for I2C EEPROM, TSENSOR & HDMI_SOURCE peripherals */ 00168 static void I2C1_Init(void); 00169 static void I2C1_DeInit(void); 00170 static void I2C1_Error (void); 00171 static void I2C1_MspInit(I2C_HandleTypeDef *hi2c); 00172 static void I2C1_MspDeInit(I2C_HandleTypeDef *hi2c); 00173 static void I2C1_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00174 static uint8_t I2C1_Read(uint8_t Addr, uint8_t Reg); 00175 static HAL_StatusTypeDef I2C1_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00176 static HAL_StatusTypeDef I2C1_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00177 static HAL_StatusTypeDef I2C1_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00178 00179 /* Link functions for EEPROM peripheral */ 00180 void EEPROM_IO_Init(void); 00181 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00182 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00183 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00184 00185 /* Link functions for Temperature Sensor peripheral */ 00186 void TSENSOR_IO_Init(void); 00187 void TSENSOR_IO_Write(uint16_t DevAddress, uint8_t* pBuffer, uint8_t WriteAddr, uint16_t Length); 00188 void TSENSOR_IO_Read(uint16_t DevAddress, uint8_t* pBuffer, uint8_t ReadAddr, uint16_t Length); 00189 uint16_t TSENSOR_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00190 00191 /* IOExpander IO functions */ 00192 void MFX_IO_Init(void); 00193 void MFX_IO_DeInit(void); 00194 void MFX_IO_ITConfig(void); 00195 void MFX_IO_EnableWakeupPin(void); 00196 void MFX_IO_Wakeup(void); 00197 void MFX_IO_Delay(uint32_t Delay); 00198 void MFX_IO_Write(uint16_t Addr, uint8_t Reg, uint8_t Value); 00199 uint8_t MFX_IO_Read(uint16_t Addr, uint8_t Reg); 00200 uint16_t MFX_IO_ReadMultiple(uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00201 /*void MFX_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length);*/ 00202 #endif /* HAL_I2C_MODULE_ENABLED */ 00203 00204 00205 00206 #if defined(HAL_SPI_MODULE_ENABLED) 00207 /* SPIx bus function */ 00208 static void SPIx_Init(void); 00209 static void SPIx_Write(uint8_t Value); 00210 static uint32_t SPIx_Read(void); 00211 static void SPIx_Error (void); 00212 static void SPIx_MspInit(SPI_HandleTypeDef *hspi); 00213 #if defined(HAL_I2C_MODULE_ENABLED) 00214 static void SPIx_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLegnth); 00215 #endif /* HAL_I2C_MODULE_ENABLED */ 00216 00217 /* Link functions for LCD peripheral */ 00218 void LCD_IO_Init(void); 00219 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size); 00220 void LCD_IO_WriteReg(uint8_t Reg); 00221 uint16_t LCD_IO_ReadData(uint16_t RegValue); 00222 void LCD_Delay(uint32_t delay); 00223 00224 /* Link functions for SD Card peripheral */ 00225 void SD_IO_Init(void); 00226 void SD_IO_CSState(uint8_t state); 00227 void SD_IO_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength); 00228 uint8_t SD_IO_WriteByte(uint8_t Data); 00229 #endif /* HAL_SPI_MODULE_ENABLED */ 00230 00231 /** 00232 * @} 00233 */ 00234 00235 /** @defgroup STM32L073Z_EVAL_LOW_LEVEL_Private_Functions 00236 * @{ 00237 */ 00238 00239 /** 00240 * @brief This method returns the STM32F072B EVAL BSP Driver revision 00241 * @param None 00242 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00243 */ 00244 uint32_t BSP_GetVersion(void) 00245 { 00246 return __STM32L073Z_EVAL_BSP_VERSION; 00247 } 00248 00249 /******************************************************************************* 00250 GPIO OPERATIONS 00251 *******************************************************************************/ 00252 #if defined(HAL_GPIO_MODULE_ENABLED) 00253 /** 00254 * @brief Configures LED GPIO. 00255 * @param Led: Specifies the Led to be configured. 00256 * This parameter can be one of following parameters: 00257 * @arg LED1 00258 * @arg LED2 00259 * @arg LED3 00260 * @arg LED4 00261 * @retval None 00262 */ 00263 void BSP_LED_Init(Led_TypeDef Led) 00264 { 00265 GPIO_InitTypeDef GPIO_InitStruct; 00266 00267 /* Enable the GPIO_LED clock */ 00268 LEDx_GPIO_CLK_ENABLE(Led); 00269 00270 /* Configure the GPIO_LED pin */ 00271 GPIO_InitStruct.Pin = LED_PIN[Led]; 00272 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00273 GPIO_InitStruct.Pull = GPIO_PULLUP; 00274 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00275 00276 HAL_GPIO_Init(LED_PORT[Led], &GPIO_InitStruct); 00277 00278 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00279 } 00280 00281 /** 00282 * @brief Turns selected LED On. 00283 * @param Led: Specifies the Led to be set on. 00284 * This parameter can be one of following parameters: 00285 * @arg LED1 00286 * @arg LED2 00287 * @arg LED3 00288 * @arg LED4 00289 * @retval None 00290 */ 00291 void BSP_LED_On(Led_TypeDef Led) 00292 { 00293 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00294 } 00295 00296 /** 00297 * @brief Turns selected LED Off. 00298 * @param Led: Specifies the Led to be set off. 00299 * This parameter can be one of following parameters: 00300 * @arg LED1 00301 * @arg LED2 00302 * @arg LED3 00303 * @arg LED4 00304 * @retval None 00305 */ 00306 void BSP_LED_Off(Led_TypeDef Led) 00307 { 00308 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00309 } 00310 00311 /** 00312 * @brief Toggles the selected LED. 00313 * @param Led: Specifies the Led to be toggled. 00314 * This parameter can be one of following parameters: 00315 * @arg LED1 00316 * @arg LED2 00317 * @arg LED3 00318 * @arg LED4 00319 * @retval None 00320 */ 00321 void BSP_LED_Toggle(Led_TypeDef Led) 00322 { 00323 HAL_GPIO_TogglePin(LED_PORT[Led], LED_PIN[Led]); 00324 } 00325 00326 /** 00327 * @brief Configures Tamper Button GPIO or EXTI Line. 00328 * @param Button: Button to be configured 00329 * This parameter can be one of the following values: 00330 * @arg BUTTON_TAMPER: Tamper Push Button 00331 * @param Mode: Button mode 00332 * This parameter can be one of the following values: 00333 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00334 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00335 * with interrupt generation capability 00336 * @retval None 00337 */ 00338 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Mode) 00339 { 00340 GPIO_InitTypeDef GPIO_InitStruct; 00341 00342 /* Enable the Tamper Clock */ 00343 TAMPERx_GPIO_CLK_ENABLE(Button); 00344 00345 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00346 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00347 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00348 00349 if (Mode == BUTTON_MODE_GPIO) 00350 { 00351 /* Configure Button pin as input */ 00352 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00353 00354 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00355 } 00356 00357 if (Mode == BUTTON_MODE_EXTI) 00358 { 00359 /* Configure Button pin as input with External interrupt */ 00360 GPIO_InitStruct.Mode = GPIO_MODE_IT_FALLING; 00361 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00362 00363 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00364 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x03, 0x00); 00365 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00366 } 00367 } 00368 00369 /** 00370 * @brief Returns the selected button state. 00371 * @param Button: Button to be checked. 00372 * This parameter can be one of the following values: 00373 * @arg BUTTON_TAMPER: Tamper Push Button 00374 * @retval The Button GPIO pin value 00375 */ 00376 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00377 { 00378 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00379 } 00380 00381 #endif //HAL_GPIO_MODULE_ENABLED 00382 00383 /******************************************************************************* 00384 JOYSTICK OPERATIONS 00385 Note : The joystick uses I2C interface (through MFX) 00386 *******************************************************************************/ 00387 #if defined(HAL_I2C_MODULE_ENABLED) 00388 /** 00389 * @brief Configures joystick GPIO and EXTI modes. 00390 * @param Joy_Mode: Button mode. 00391 * This parameter can be one of the following values: 00392 * @arg JOY_MODE_GPIO: Joystick pins will be used as simple IOs 00393 * @arg JOY_MODE_EXTI: Joystick pins will be connected to EXTI line 00394 * with interrupt generation capability 00395 * @retval IO_OK: if all initializations are OK. Other value if error. 00396 */ 00397 uint8_t BSP_JOY_Init(JOYMode_TypeDef Joy_Mode) 00398 { 00399 uint8_t ret = 0; 00400 00401 /* Initialize the IO functionalities */ 00402 ret = BSP_IO_Init(); 00403 00404 if (ret == IO_OK) 00405 { 00406 /* Configure joystick pins in IT mode */ 00407 if(Joy_Mode == JOY_MODE_EXTI) 00408 { 00409 /* MFX pins init for Joystick */ 00410 /* Configure IO interrupt acquisition mode */ 00411 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_IT_LOW_LEVEL_PU); 00412 } 00413 else 00414 { 00415 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_INPUT_PU); 00416 } 00417 } 00418 00419 return ret; 00420 } 00421 00422 /** 00423 * @brief Returns the current joystick status. 00424 * @param None 00425 * @retval Code of the joystick key pressed 00426 * This code can be one of the following values: 00427 * @arg JOY_NONE 00428 * @arg JOY_SEL 00429 * @arg JOY_DOWN 00430 * @arg JOY_LEFT 00431 * @arg JOY_RIGHT 00432 * @arg JOY_UP 00433 */ 00434 JOYState_TypeDef BSP_JOY_GetState(void) 00435 { 00436 uint16_t tmp = 0; 00437 00438 /* Read the status joystick pins */ 00439 tmp = BSP_IO_ReadPin(JOY_ALL_PINS); 00440 00441 /* Check the pressed keys */ 00442 if((tmp & JOY_NONE_PIN) == JOY_NONE) 00443 { 00444 return(JOYState_TypeDef) JOY_NONE; 00445 } 00446 else if(!(tmp & JOY_SEL_PIN)) 00447 { 00448 return(JOYState_TypeDef) JOY_SEL; 00449 } 00450 else if(!(tmp & JOY_DOWN_PIN)) 00451 { 00452 return(JOYState_TypeDef) JOY_DOWN; 00453 } 00454 else if(!(tmp & JOY_LEFT_PIN)) 00455 { 00456 return(JOYState_TypeDef) JOY_LEFT; 00457 } 00458 else if(!(tmp & JOY_RIGHT_PIN)) 00459 { 00460 return(JOYState_TypeDef) JOY_RIGHT; 00461 } 00462 else if(!(tmp & JOY_UP_PIN)) 00463 { 00464 return(JOYState_TypeDef) JOY_UP; 00465 } 00466 else 00467 { 00468 return(JOYState_TypeDef) JOY_NONE; 00469 } 00470 } 00471 #endif //HAL_I2C_MODULE_ENABLED 00472 00473 /******************************************************************************* 00474 UART OPERATIONS 00475 *******************************************************************************/ 00476 #if defined(HAL_UART_MODULE_ENABLED) 00477 /** 00478 * @brief Configures COM port. 00479 * @param COM: Specifies the COM port to be configured. 00480 * This parameter can be one of following parameters: 00481 * @arg COM1 00482 * @param huart: pointer to a UART_HandleTypeDef structure that 00483 * contains the configuration information for the specified UART peripheral. 00484 * @retval None 00485 */ 00486 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef* huart) 00487 { 00488 GPIO_InitTypeDef GPIO_InitStruct; 00489 00490 /* Enable GPIO clock */ 00491 COMx_TX_GPIO_CLK_ENABLE(COM); 00492 COMx_RX_GPIO_CLK_ENABLE(COM); 00493 00494 /* Enable USART clock */ 00495 COMx_CLK_ENABLE(COM); 00496 00497 /* Configure USART Tx as alternate function push-pull */ 00498 GPIO_InitStruct.Pin = COM_TX_PIN[COM]; 00499 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00500 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00501 GPIO_InitStruct.Pull = GPIO_PULLUP; 00502 GPIO_InitStruct.Alternate = COM_TX_AF[COM]; 00503 HAL_GPIO_Init(COM_TX_PORT[COM], &GPIO_InitStruct); 00504 00505 /* Configure USART Rx as alternate function push-pull */ 00506 GPIO_InitStruct.Pin = COM_RX_PIN[COM]; 00507 GPIO_InitStruct.Alternate = COM_RX_AF[COM]; 00508 HAL_GPIO_Init(COM_RX_PORT[COM], &GPIO_InitStruct); 00509 00510 /* USART configuration */ 00511 huart->Instance = COM_USART[COM]; 00512 HAL_UART_Init(huart); 00513 } 00514 #endif /* HAL_UART_MODULE_ENABLED */ 00515 00516 /******************************************************************************* 00517 BUS OPERATIONS 00518 *******************************************************************************/ 00519 #if defined(HAL_I2C_MODULE_ENABLED) 00520 /******************************* I2C Routines *********************************/ 00521 00522 /** 00523 * @brief I2C Bus initialization 00524 * @param None 00525 * @retval None 00526 */ 00527 static void I2C1_Init(void) 00528 { 00529 if(HAL_I2C_GetState(&heval_I2c1) == HAL_I2C_STATE_RESET) 00530 { 00531 heval_I2c1.Instance = EVAL_I2C1; 00532 heval_I2c1.Init.Timing = I2C1_TIMING; 00533 heval_I2c1.Init.OwnAddress1 = 0; 00534 heval_I2c1.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00535 heval_I2c1.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00536 heval_I2c1.Init.OwnAddress2 = 0; 00537 heval_I2c1.Init.OwnAddress2Masks = I2C_OA2_NOMASK; 00538 heval_I2c1.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00539 heval_I2c1.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00540 00541 /* Init the I2C */ 00542 I2C1_MspInit(&heval_I2c1); 00543 HAL_I2C_Init(&heval_I2c1); 00544 } 00545 } 00546 00547 /** 00548 * @brief I2C1 Bus Deinitialization 00549 * @retval None 00550 */ 00551 static void I2C1_DeInit(void) 00552 { 00553 if(HAL_I2C_GetState(&heval_I2c1) != HAL_I2C_STATE_RESET) 00554 { 00555 /* DeInit the I2C */ 00556 HAL_I2C_DeInit(&heval_I2c1); 00557 I2C1_MspDeInit(&heval_I2c1); 00558 } 00559 } 00560 /** 00561 * @brief Writes a single data. 00562 * @param Addr: I2C address 00563 * @param Reg: Register address 00564 * @param Value: Data to be written 00565 * @retval None 00566 */ 00567 static void I2C1_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00568 { 00569 HAL_StatusTypeDef status = HAL_OK; 00570 00571 status = HAL_I2C_Mem_Write(&heval_I2c1, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, 100); 00572 00573 /* Check the communication status */ 00574 if(status != HAL_OK) 00575 { 00576 /* Execute user timeout callback */ 00577 I2C1_Error(); 00578 } 00579 } 00580 00581 /** 00582 * @brief Reads a single data. 00583 * @param Addr: I2C address 00584 * @param Reg: Register address 00585 * @retval Read data 00586 */ 00587 static uint8_t I2C1_Read(uint8_t Addr, uint8_t Reg) 00588 { 00589 HAL_StatusTypeDef status = HAL_OK; 00590 uint8_t Value = 0; 00591 00592 status = HAL_I2C_Mem_Read(&heval_I2c1, Addr, Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, 1000); 00593 00594 /* Check the communication status */ 00595 if(status != HAL_OK) 00596 { 00597 /* Execute user timeout callback */ 00598 I2C1_Error(); 00599 } 00600 return Value; 00601 } 00602 00603 00604 00605 /** 00606 * @brief Reads multiple data on the BUS. 00607 * @param Addr : I2C Address 00608 * @param Reg : Reg Address 00609 * @param RegSize : The target register size (can be 8BIT or 16BIT) 00610 * @param pBuffer : pointer to read data buffer 00611 * @param Length : length of the data 00612 * @retval 0 if no problems to read multiple data 00613 */ 00614 static HAL_StatusTypeDef I2C1_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00615 { 00616 HAL_StatusTypeDef status = HAL_OK; 00617 00618 status = HAL_I2C_Mem_Read(&heval_I2c1, Addr, Reg, RegSize, pBuffer, Length, I2c1Timeout); 00619 00620 /* Check the communication status */ 00621 if(status != HAL_OK) 00622 { 00623 /* Re-Initiaize the BUS */ 00624 I2C1_Error(); 00625 } 00626 return status; 00627 } 00628 00629 /** 00630 * @brief Checks if target device is ready for communication. 00631 * @note This function is used with Memory devices 00632 * @param DevAddress: Target device address 00633 * @param Trials: Number of trials 00634 * @retval HAL status 00635 */ 00636 static HAL_StatusTypeDef I2C1_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00637 { 00638 return (HAL_I2C_IsDeviceReady(&heval_I2c1, DevAddress, Trials, I2c1Timeout)); 00639 } 00640 00641 /** 00642 * @brief Write a value in a register of the device through BUS. 00643 * @param Addr: Device address on BUS Bus. 00644 * @param Reg: The target register address to write 00645 * @param RegSize: The target register size (can be 8BIT or 16BIT) 00646 * @param pBuffer: The target register value to be written 00647 * @param Length: buffer size to be written 00648 * @retval None 00649 */ 00650 static HAL_StatusTypeDef I2C1_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00651 { 00652 HAL_StatusTypeDef status = HAL_OK; 00653 00654 status = HAL_I2C_Mem_Write(&heval_I2c1, Addr, Reg, RegSize, pBuffer, Length, I2c1Timeout); 00655 00656 /* Check the communication status */ 00657 if(status != HAL_OK) 00658 { 00659 /* Re-Initiaize the BUS */ 00660 I2C1_Error(); 00661 } 00662 return status; 00663 } 00664 00665 /** 00666 * @brief Manages error callback by re-initializing I2C. 00667 * @param None 00668 * @retval None 00669 */ 00670 static void I2C1_Error(void) 00671 { 00672 /* De-initialize the I2C communication BUS */ 00673 HAL_I2C_DeInit(&heval_I2c1); 00674 00675 /* Re-Initiaize the I2C communication BUS */ 00676 I2C1_Init(); 00677 } 00678 00679 /** 00680 * @brief I2C MSP Initialization 00681 * @param hi2c: I2C handle 00682 * @retval None 00683 */ 00684 static void I2C1_MspInit(I2C_HandleTypeDef *hi2c) 00685 { 00686 GPIO_InitTypeDef GPIO_InitStruct; 00687 RCC_PeriphCLKInitTypeDef RCC_PeriphCLKInitStruct; 00688 00689 /*##-1- Set source clock to SYSCLK for I2C1 ################################################*/ 00690 RCC_PeriphCLKInitStruct.PeriphClockSelection = RCC_PERIPHCLK_I2C1; 00691 RCC_PeriphCLKInitStruct.I2c1ClockSelection = RCC_I2C1CLKSOURCE_SYSCLK; 00692 HAL_RCCEx_PeriphCLKConfig(&RCC_PeriphCLKInitStruct); 00693 00694 /*##-2- Configure the GPIOs ################################################*/ 00695 00696 /* Enable GPIO clock */ 00697 EVAL_I2C1_GPIO_CLK_ENABLE(); 00698 00699 /* Configure I2C SCL & SDA as alternate function */ 00700 GPIO_InitStruct.Pin = (EVAL_I2C1_SCL_PIN| EVAL_I2C1_SDA_PIN); 00701 GPIO_InitStruct.Mode = GPIO_MODE_AF_OD; 00702 GPIO_InitStruct.Pull = GPIO_NOPULL; 00703 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00704 GPIO_InitStruct.Alternate = EVAL_I2C1_SCL_SDA_AF; 00705 HAL_GPIO_Init(EVAL_I2C1_GPIO_PORT, &GPIO_InitStruct); 00706 00707 /*##-3- Configure the Eval I2C peripheral #######################################*/ 00708 /* Enable I2C clock */ 00709 EVAL_I2C1_CLK_ENABLE(); 00710 00711 /* Force the I2C peripheral clock reset */ 00712 EVAL_I2C1_FORCE_RESET(); 00713 00714 /* Release the I2C peripheral clock reset */ 00715 EVAL_I2C1_RELEASE_RESET(); 00716 } 00717 00718 /** 00719 * @brief I2C1 MSP DeInitialization 00720 * @param hi2c: I2C2 handle 00721 * @retval None 00722 */ 00723 static void I2C1_MspDeInit(I2C_HandleTypeDef *hi2c) 00724 { 00725 00726 /*##-1- Unconfigure the GPIOs ################################################*/ 00727 /* Enable GPIO clock */ 00728 EVAL_I2C1_GPIO_CLK_ENABLE(); 00729 00730 /* Configure I2C Rx/Tx as alternate function */ 00731 HAL_GPIO_DeInit(EVAL_I2C1_GPIO_PORT, (EVAL_I2C1_SCL_PIN| EVAL_I2C1_SDA_PIN)); 00732 00733 /*##-2- Unconfigure the Discovery I2C2 peripheral ############################*/ 00734 /* Force and release I2C Peripheral */ 00735 EVAL_I2C1_FORCE_RESET(); 00736 EVAL_I2C1_RELEASE_RESET(); 00737 00738 /* Disable Discovery I2C1 clock */ 00739 EVAL_I2C1_RELEASE_RESET(); 00740 } 00741 00742 #endif /*HAL_I2C_MODULE_ENABLED*/ 00743 00744 #if defined(HAL_SPI_MODULE_ENABLED) 00745 /******************************* SPI Routines *********************************/ 00746 00747 /** 00748 * @brief SPIx Bus initialization 00749 * @param None 00750 * @retval None 00751 */ 00752 static void SPIx_Init(void) 00753 { 00754 if(HAL_SPI_GetState(&heval_Spi) == HAL_SPI_STATE_RESET) 00755 { 00756 /* SPI Config */ 00757 heval_Spi.Instance = EVAL_SPIx; 00758 /* SPI baudrate is set to 16 MHz (PCLK2/SPI_BaudRatePrescaler = 32/2 = 16 MHz) 00759 to verify these constraints: 00760 HX8347D LCD SPI interface max baudrate is 50MHz for write and 6.66MHz for read 00761 PCLK1 frequency is set to 32 MHz 00762 - SD card SPI interface max baudrate is 25MHz for write/read 00763 */ 00764 heval_Spi.Init.BaudRatePrescaler = SPI_BAUDRATEPRESCALER_2; 00765 heval_Spi.Init.Direction = SPI_DIRECTION_2LINES; 00766 heval_Spi.Init.CLKPhase = SPI_PHASE_2EDGE; 00767 heval_Spi.Init.CLKPolarity = SPI_POLARITY_HIGH; 00768 heval_Spi.Init.CRCCalculation = SPI_CRCCALCULATION_DISABLE; 00769 heval_Spi.Init.CRCPolynomial = 7; 00770 heval_Spi.Init.DataSize = SPI_DATASIZE_8BIT; 00771 heval_Spi.Init.FirstBit = SPI_FIRSTBIT_MSB; 00772 heval_Spi.Init.NSS = SPI_NSS_SOFT; 00773 heval_Spi.Init.TIMode = SPI_TIMODE_DISABLE; 00774 heval_Spi.Init.Mode = SPI_MODE_MASTER; 00775 00776 SPIx_MspInit(&heval_Spi); 00777 HAL_SPI_Init(&heval_Spi); 00778 } 00779 } 00780 00781 /** 00782 * @brief SPI Read 1 bytes from device 00783 * @param None 00784 * @retval Read data 00785 */ 00786 static uint32_t SPIx_Read(void) 00787 { 00788 HAL_StatusTypeDef status = HAL_OK; 00789 uint8_t readvalue = 0x0; 00790 uint8_t writevalue = 0xff; 00791 00792 status = HAL_SPI_TransmitReceive(&heval_Spi, &writevalue, &readvalue, 1, SpixTimeout); 00793 00794 /* Check the communication status */ 00795 if(status != HAL_OK) 00796 { 00797 /* Execute user timeout callback */ 00798 SPIx_Error(); 00799 } 00800 00801 return (uint32_t) readvalue; 00802 } 00803 00804 /** 00805 * @brief SPI Write a byte to device 00806 * @param Value: value to be written 00807 * @retval None 00808 */ 00809 #if defined(HAL_I2C_MODULE_ENABLED) 00810 static void SPIx_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLegnth) 00811 { 00812 HAL_StatusTypeDef status = HAL_OK; 00813 00814 status = HAL_SPI_TransmitReceive(&heval_Spi, (uint8_t*) DataIn, DataOut, DataLegnth, SpixTimeout); 00815 00816 /* Check the communication status */ 00817 if(status != HAL_OK) 00818 { 00819 /* Execute user timeout callback */ 00820 SPIx_Error(); 00821 } 00822 } 00823 #endif //HAL_I2C_MODULE_ENABLED 00824 /** 00825 * @brief SPI Write a byte to device 00826 * @param Value: value to be written 00827 * @retval None 00828 */ 00829 static void SPIx_Write(uint8_t Value) 00830 { 00831 HAL_StatusTypeDef status = HAL_OK; 00832 uint8_t data; 00833 00834 status = HAL_SPI_TransmitReceive(&heval_Spi, (uint8_t*) &Value, &data, 1, SpixTimeout); 00835 00836 /* Check the communication status */ 00837 if(status != HAL_OK) 00838 { 00839 /* Execute user timeout callback */ 00840 SPIx_Error(); 00841 } 00842 } 00843 00844 /** 00845 * @brief SPI error treatment function 00846 * @param None 00847 * @retval None 00848 */ 00849 static void SPIx_Error (void) 00850 { 00851 /* De-initialize the SPI communication BUS */ 00852 HAL_SPI_DeInit(&heval_Spi); 00853 00854 /* Re- Initiaize the SPI communication BUS */ 00855 SPIx_Init(); 00856 } 00857 00858 /** 00859 * @brief SPI MSP Init 00860 * @param hspi: SPI handle 00861 * @retval None 00862 */ 00863 static void SPIx_MspInit(SPI_HandleTypeDef *hspi) 00864 { 00865 GPIO_InitTypeDef GPIO_InitStruct; 00866 00867 /* Enable SPI clock */ 00868 EVAL_SPIx_CLK_ENABLE(); 00869 00870 /* enable EVAL_SPI gpio clocks */ 00871 EVAL_SPIx_SCK_GPIO_CLK_ENABLE(); 00872 EVAL_SPIx_MISO_GPIO_CLK_ENABLE(); 00873 EVAL_SPIx_MOSI_GPIO_CLK_ENABLE(); 00874 EVAL_SPIx_MOSI_DIR_GPIO_CLK_ENABLE(); 00875 00876 /* configure SPI SCK */ 00877 GPIO_InitStruct.Pin = EVAL_SPIx_SCK_PIN; 00878 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00879 GPIO_InitStruct.Pull = GPIO_NOPULL; 00880 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00881 GPIO_InitStruct.Alternate = EVAL_SPIx_SCK_AF; 00882 HAL_GPIO_Init(EVAL_SPIx_SCK_GPIO_PORT, &GPIO_InitStruct); 00883 00884 /* configure SPI MOSI */ 00885 GPIO_InitStruct.Pin = EVAL_SPIx_MOSI_PIN; 00886 GPIO_InitStruct.Alternate = EVAL_SPIx_MOSI_AF; 00887 HAL_GPIO_Init(EVAL_SPIx_MOSI_GPIO_PORT, &GPIO_InitStruct); 00888 00889 /* configure SPI MISO */ 00890 GPIO_InitStruct.Pin = EVAL_SPIx_MISO_PIN; 00891 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00892 GPIO_InitStruct.Alternate = EVAL_SPIx_MISO_AF; 00893 HAL_GPIO_Init(EVAL_SPIx_MISO_GPIO_PORT, &GPIO_InitStruct); 00894 00895 /* Set PB.2 as Out PP, as direction pin for MOSI */ 00896 GPIO_InitStruct.Pin = EVAL_SPIx_MOSI_DIR_PIN; 00897 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_MEDIUM ; 00898 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00899 GPIO_InitStruct.Pull = GPIO_NOPULL; 00900 HAL_GPIO_Init(EVAL_SPIx_MOSI_DIR_GPIO_PORT, &GPIO_InitStruct); 00901 00902 /* MOSI DIRECTION as output */ 00903 HAL_GPIO_WritePin(EVAL_SPIx_MOSI_DIR_GPIO_PORT, EVAL_SPIx_MOSI_DIR_PIN, GPIO_PIN_SET); 00904 00905 /* Force the SPI peripheral clock reset */ 00906 EVAL_SPIx_FORCE_RESET(); 00907 00908 /* Release the SPI peripheral clock reset */ 00909 EVAL_SPIx_RELEASE_RESET(); 00910 } 00911 00912 #endif /*HAL_SPI_MODULE_ENABLED*/ 00913 00914 /****************************************************************************** 00915 LINK OPERATIONS 00916 *******************************************************************************/ 00917 00918 #if defined(HAL_SPI_MODULE_ENABLED) 00919 /********************************* LINK LCD ***********************************/ 00920 00921 /** 00922 * @brief Configures the LCD_SPI interface. 00923 * @param None 00924 * @retval None 00925 */ 00926 void LCD_IO_Init(void) 00927 { 00928 GPIO_InitTypeDef GPIO_InitStruct; 00929 00930 /* Configure the LCD Control pins ------------------------------------------*/ 00931 LCD_NCS_GPIO_CLK_ENABLE(); 00932 00933 /* Configure NCS in Output Push-Pull mode */ 00934 GPIO_InitStruct.Pin = LCD_NCS_PIN; 00935 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00936 GPIO_InitStruct.Pull = GPIO_PULLUP; 00937 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW ; 00938 HAL_GPIO_Init(LCD_NCS_GPIO_PORT, &GPIO_InitStruct); 00939 00940 /* Set or Reset the control line */ 00941 LCD_CS_LOW(); 00942 LCD_CS_HIGH(); 00943 00944 SPIx_Init(); 00945 } 00946 00947 /** 00948 * @brief Write register value. 00949 * @param pData Pointer on the register value 00950 * @param Size Size of byte to transmit to the register 00951 * @retval None 00952 */ 00953 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size) 00954 { 00955 uint32_t counter = 0; 00956 __IO uint32_t data = 0; 00957 00958 /* Reset LCD control line(/CS) and Send data */ 00959 LCD_CS_LOW(); 00960 00961 /* Send Start Byte */ 00962 SPIx_Write(START_BYTE | LCD_WRITE_REG); 00963 00964 if (Size == 1) 00965 { 00966 /* Only 1 byte to be sent to LCD - general interface can be used */ 00967 /* Send Data */ 00968 SPIx_Write(*pData); 00969 } 00970 else 00971 { 00972 for (counter = Size; counter != 0; counter--) 00973 { 00974 while(((heval_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 00975 { 00976 } 00977 /* Need to invert bytes for LCD*/ 00978 *((__IO uint8_t*)&heval_Spi.Instance->DR) = *(pData+1); 00979 00980 while(((heval_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 00981 { 00982 } 00983 *((__IO uint8_t*)&heval_Spi.Instance->DR) = *pData; 00984 counter--; 00985 pData += 2; 00986 } 00987 00988 /* Wait until the bus is ready before releasing Chip select */ 00989 while(((heval_Spi.Instance->SR) & SPI_FLAG_BSY) != RESET) 00990 { 00991 } 00992 } 00993 00994 /* Empty the Rx fifo */ 00995 data = *(&heval_Spi.Instance->DR); 00996 UNUSED(data); /* Remove GNU warning */ 00997 00998 /* Reset LCD control line(/CS) and Send data */ 00999 LCD_CS_HIGH(); 01000 } 01001 01002 /** 01003 * @brief register address. 01004 * @param Reg 01005 * @retval None 01006 */ 01007 void LCD_IO_WriteReg(uint8_t Reg) 01008 { 01009 /* Reset LCD control line(/CS) and Send command */ 01010 LCD_CS_LOW(); 01011 01012 /* Send Start Byte */ 01013 SPIx_Write(START_BYTE | SET_INDEX); 01014 01015 /* Write 16-bit Reg Index (High Byte is 0) */ 01016 SPIx_Write(0x00); 01017 SPIx_Write(Reg); 01018 01019 /* Deselect : Chip Select high */ 01020 LCD_CS_HIGH(); 01021 } 01022 01023 /** 01024 * @brief Read register value. 01025 * @param Reg 01026 * @retval None 01027 */ 01028 uint16_t LCD_IO_ReadData(uint16_t Reg) 01029 { 01030 uint32_t readvalue = 0; 01031 01032 /* Send Reg value to Read */ 01033 LCD_IO_WriteReg(Reg); 01034 01035 /* Reset LCD control line(/CS) and Send command */ 01036 LCD_CS_LOW(); 01037 01038 /* Send Start Byte */ 01039 SPIx_Write(START_BYTE | LCD_READ_REG); 01040 01041 /* Read Upper Byte */ 01042 SPIx_Write(0xFF); 01043 readvalue = SPIx_Read(); 01044 readvalue = readvalue << 8; 01045 readvalue |= SPIx_Read(); 01046 01047 HAL_Delay(10); 01048 01049 /* Deselect : Chip Select high */ 01050 LCD_CS_HIGH(); 01051 return readvalue; 01052 } 01053 01054 /** 01055 * @brief Wait for loop in ms. 01056 * @param Delay in ms. 01057 * @retval None 01058 */ 01059 void LCD_Delay (uint32_t Delay) 01060 { 01061 HAL_Delay(Delay); 01062 } 01063 01064 #endif /*HAL_SPI_MODULE_ENABLED*/ 01065 01066 /******************************************************************************* 01067 SD Card OPERATIONS 01068 *******************************************************************************/ 01069 #if defined(HAL_SPI_MODULE_ENABLED) && defined(HAL_I2C_MODULE_ENABLED) 01070 01071 /** 01072 * @brief Initializes the SD Card and put it into StandBy State (Ready for 01073 * data transfer). 01074 * @param None 01075 * @retval None 01076 */ 01077 void SD_IO_Init(void) 01078 { 01079 GPIO_InitTypeDef GPIO_InitStruct; 01080 uint8_t counter; 01081 01082 /* SD_CS_GPIO and SD_DETECT_GPIO Periph clock enable */ 01083 SD_CS_GPIO_CLK_ENABLE(); 01084 01085 /* Configure SD_CS_PIN pin: SD Card CS pin */ 01086 GPIO_InitStruct.Pin = SD_CS_PIN; 01087 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 01088 GPIO_InitStruct.Pull = GPIO_PULLUP; 01089 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_MEDIUM ; 01090 HAL_GPIO_Init(SD_CS_GPIO_PORT, &GPIO_InitStruct); 01091 01092 /* Configure SD_DETECT_PIN pin: SD Card detect pin (Via MFX) */ 01093 if (BSP_IO_Init() == IO_OK) 01094 { 01095 BSP_IO_ConfigPin(SD_DETECT_PIN, IO_MODE_INPUT_PU); 01096 } 01097 01098 /*------------Put SD in SPI mode--------------*/ 01099 /* SD SPI Config */ 01100 SPIx_Init(); 01101 01102 /* SD chip select high */ 01103 SD_CS_HIGH(); 01104 01105 /* Send dummy byte 0xFF, 10 times with CS high */ 01106 /* Rise CS and MOSI for 80 clocks cycles */ 01107 for (counter = 0; counter <= 9; counter++) 01108 { 01109 /* Send dummy byte 0xFF */ 01110 SD_IO_WriteByte(SD_DUMMY_BYTE); 01111 } 01112 } 01113 01114 01115 void SD_IO_CSState(uint8_t val) 01116 { 01117 if(val == 1) 01118 { 01119 SD_CS_HIGH(); 01120 } 01121 else 01122 { 01123 SD_CS_LOW(); 01124 } 01125 } 01126 01127 /** 01128 * @brief Write a byte on the SD. 01129 * @param Data: byte to send. 01130 * @retval None 01131 */ 01132 void SD_IO_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength) 01133 { 01134 // /* SD chip select low */ 01135 // SD_CS_LOW(); 01136 /* Send the byte */ 01137 SPIx_WriteReadData(DataIn, DataOut, DataLength); 01138 } 01139 01140 /** 01141 * @brief Writes a byte on the SD. 01142 * @param Data: byte to send. 01143 * @retval None 01144 */ 01145 uint8_t SD_IO_WriteByte(uint8_t Data) 01146 { 01147 uint8_t tmp; 01148 // /* SD chip select low */ 01149 // SD_CS_LOW(); 01150 // 01151 /* Send the byte */ 01152 SPIx_WriteReadData(&Data,&tmp,1); 01153 return tmp; 01154 } 01155 01156 #endif /* HAL_SPI_MODULE_ENABLED && HAL_I2C_MODULE_ENABLED*/ 01157 01158 /******************************************************************************* 01159 MFX OPERATIONS 01160 *******************************************************************************/ 01161 #if defined(HAL_I2C_MODULE_ENABLED) 01162 /** 01163 * @brief Initializes IOE low level. 01164 * @param None 01165 * @retval None 01166 */ 01167 void MFX_IO_Init(void) 01168 { 01169 I2C1_Init(); 01170 } 01171 01172 /** 01173 * @brief Deinitializes MFX low level. 01174 * @retval None 01175 */ 01176 void MFX_IO_DeInit(void) 01177 { 01178 I2C1_DeInit(); 01179 } 01180 01181 /** 01182 * @brief Configures IOE low level interrupt. 01183 * @param None 01184 * @retval None 01185 */ 01186 void MFX_IO_ITConfig(void) 01187 { 01188 static uint8_t MFX_IO_IT_Enabled = 0; 01189 GPIO_InitTypeDef GPIO_InitStruct; 01190 01191 if(MFX_IO_IT_Enabled == 0) 01192 { 01193 MFX_IO_IT_Enabled = 1; 01194 /* Enable the GPIO EXTI clock */ 01195 MFX_IRQOUT_CLK_ENABLE(); 01196 __HAL_RCC_SYSCFG_CLK_ENABLE(); 01197 01198 GPIO_InitStruct.Pin = MFX_IRQOUT_PIN; 01199 GPIO_InitStruct.Pull = GPIO_PULLUP; 01200 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 01201 GPIO_InitStruct.Mode = GPIO_MODE_IT_RISING; 01202 HAL_GPIO_Init(MFX_IRQOUT_PORT, &GPIO_InitStruct); 01203 01204 /* Enable and set GPIO EXTI Interrupt to the lowest priority */ 01205 HAL_NVIC_SetPriority((IRQn_Type)(MFX_IRQOUT_EXTI_IRQn), 0x03, 0x00); 01206 HAL_NVIC_EnableIRQ((IRQn_Type)(MFX_IRQOUT_EXTI_IRQn)); 01207 01208 } 01209 } 01210 01211 /** 01212 * @brief Configures MFX wke up pin. 01213 * @retval None 01214 */ 01215 void MFX_IO_EnableWakeupPin(void) 01216 { 01217 GPIO_InitTypeDef GPIO_InitStruct; 01218 01219 /* Enable wakeup gpio clock */ 01220 IDD_WAKEUP_GPIO_CLK_ENABLE(); 01221 01222 /* MFX wakeup pin configuration */ 01223 GPIO_InitStruct.Pin = IDD_WAKEUP_PIN; 01224 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 01225 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 01226 GPIO_InitStruct.Pull = GPIO_NOPULL; 01227 HAL_GPIO_Init(IDD_WAKEUP_GPIO_PORT, &GPIO_InitStruct); 01228 } 01229 01230 /** 01231 * @brief Wakeup MFX. 01232 * @retval None 01233 */ 01234 void MFX_IO_Wakeup(void) 01235 { 01236 /* Set Wakeup pin to high to wakeup Idd measurement component from standby mode */ 01237 HAL_GPIO_WritePin(IDD_WAKEUP_GPIO_PORT, IDD_WAKEUP_PIN, GPIO_PIN_SET); 01238 01239 /* Wait */ 01240 HAL_Delay(1); 01241 01242 /* Set gpio pin basck to low */ 01243 HAL_GPIO_WritePin(IDD_WAKEUP_GPIO_PORT, IDD_WAKEUP_PIN, GPIO_PIN_RESET); 01244 } 01245 01246 /** 01247 * @brief IOE writes single data. 01248 * @param Addr: I2C address 01249 * @param Reg: Register address 01250 * @param Value: Data to be written 01251 * @retval None 01252 */ 01253 void MFX_IO_Write(uint16_t Addr, uint8_t Reg, uint8_t Value) 01254 { 01255 I2C1_Write((uint8_t)Addr, Reg, Value); 01256 } 01257 01258 /** 01259 * @brief IOE reads single data. 01260 * @param Addr: I2C address 01261 * @param Reg: Register address 01262 * @retval Read data 01263 */ 01264 uint8_t MFX_IO_Read(uint16_t Addr, uint8_t Reg) 01265 { 01266 return I2C1_Read((uint8_t)Addr, Reg); 01267 } 01268 01269 /** 01270 * @brief IOE reads multiple data. 01271 * @param Addr: I2C address 01272 * @param Reg: Register address 01273 * @param Buffer: Pointer to data buffer 01274 * @param Length: Length of the data 01275 * @retval Number of read data 01276 */ 01277 uint16_t MFX_IO_ReadMultiple(uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01278 { 01279 return I2C1_ReadBuffer((uint8_t)Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01280 } 01281 01282 /** 01283 * @brief IOE writes multiple data. 01284 * @param Addr: I2C address 01285 * @param Reg: Register address 01286 * @param Buffer: Pointer to data buffer 01287 * @param Length: Length of the data 01288 * @retval None 01289 */ 01290 /* 01291 void MFX_IO_WriteMultiple(uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01292 { 01293 I2C1_WriteMultiple((uint8_t)Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01294 } 01295 */ 01296 01297 /** 01298 * @brief IOE delay 01299 * @param Delay: Delay in ms 01300 * @retval None 01301 */ 01302 void MFX_IO_Delay(uint32_t Delay) 01303 { 01304 HAL_Delay(Delay); 01305 } 01306 01307 01308 01309 /********************************* LINK I2C EEPROM *****************************/ 01310 /** 01311 * @brief Initializes peripherals used by the I2C EEPROM driver. 01312 * @param None 01313 * @retval None 01314 */ 01315 void EEPROM_IO_Init(void) 01316 { 01317 I2C1_Init(); 01318 } 01319 01320 /** 01321 * @brief Write data to I2C EEPROM driver 01322 * @param DevAddress: Target device address 01323 * @param MemAddress: Internal memory address 01324 * @param pBuffer: Pointer to data buffer 01325 * @param BufferSize: Amount of data to be sent 01326 * @retval HAL status 01327 */ 01328 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01329 { 01330 return (I2C1_WriteBuffer(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01331 } 01332 01333 /** 01334 * @brief Read data from I2C EEPROM driver 01335 * @param DevAddress: Target device address 01336 * @param MemAddress: Internal memory address 01337 * @param pBuffer: Pointer to data buffer 01338 * @param BufferSize: Amount of data to be read 01339 * @retval HAL status 01340 */ 01341 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01342 { 01343 return (I2C1_ReadBuffer(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01344 } 01345 01346 /** 01347 * @brief Checks if target device is ready for communication. 01348 * @note This function is used with Memory devices 01349 * @param DevAddress: Target device address 01350 * @param Trials: Number of trials 01351 * @retval HAL status 01352 */ 01353 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01354 { 01355 return (I2C1_IsDeviceReady(DevAddress, Trials)); 01356 } 01357 01358 /********************************* LINK I2C TEMPERATURE SENSOR *****************************/ 01359 /** 01360 * @brief Initializes peripherals used by the I2C Temperature Sensor driver. 01361 * @param None 01362 * @retval None 01363 */ 01364 void TSENSOR_IO_Init(void) 01365 { 01366 I2C1_Init(); 01367 } 01368 01369 /** 01370 * @brief Writes one byte to the TSENSOR. 01371 * @param DevAddress: Target device address 01372 * @param pBuffer: Pointer to data buffer 01373 * @param WriteAddr: TSENSOR's internal address to write to. 01374 * @param Length: Number of data to write 01375 * @retval None 01376 */ 01377 void TSENSOR_IO_Write(uint16_t DevAddress, uint8_t* pBuffer, uint8_t WriteAddr, uint16_t Length) 01378 { 01379 I2C1_WriteBuffer(DevAddress, WriteAddr, I2C_MEMADD_SIZE_8BIT, pBuffer, Length); 01380 } 01381 01382 /** 01383 * @brief Reads one byte from the TSENSOR. 01384 * @param DevAddress: Target device address 01385 * @param pBuffer : pointer to the buffer that receives the data read from the TSENSOR. 01386 * @param ReadAddr : TSENSOR's internal address to read from. 01387 * @param Length: Number of data to read 01388 * @retval None 01389 */ 01390 void TSENSOR_IO_Read(uint16_t DevAddress, uint8_t* pBuffer, uint8_t ReadAddr, uint16_t Length) 01391 { 01392 I2C1_ReadBuffer(DevAddress, ReadAddr, I2C_MEMADD_SIZE_8BIT, pBuffer, Length); 01393 } 01394 01395 /** 01396 * @brief Checks if Temperature Sensor is ready for communication. 01397 * @param DevAddress: Target device address 01398 * @param Trials: Number of trials 01399 * @retval HAL status 01400 */ 01401 uint16_t TSENSOR_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01402 { 01403 return (I2C1_IsDeviceReady(DevAddress, Trials)); 01404 } 01405 01406 #endif /* HAL_I2C_MODULE_ENABLED */ 01407 01408 /** 01409 * @} 01410 */ 01411 01412 /** 01413 * @} 01414 */ 01415 01416 /** 01417 * @} 01418 */ 01419 01420 /** 01421 * @} 01422 */ 01423 01424 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Mon Aug 28 2017 14:54:25 for STM32L073Z_EVAL BSP User Manual by
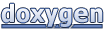