STM32H743I_EVAL BSP User Manual
|
stm32h743i_eval_sdram.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32h743i_eval_sdram.c 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 21-April-2017 00007 * @brief This file includes the SDRAM driver for the IS42S32800G memory 00008 * device mounted on STM32H743I-EVAL evaluation boards. 00009 @verbatim 00010 How To use this driver: 00011 ----------------------- 00012 - This driver is used to drive the IS42S32800G SDRAM external memory mounted 00013 on STM32H743I-EVAL evaluation board. 00014 - This driver does not need a specific component driver for the SDRAM device 00015 to be included with. 00016 00017 Driver description: 00018 ------------------ 00019 + Initialization steps: 00020 o Initialize the SDRAM external memory using the BSP_SDRAM_Init() function. This 00021 function includes the MSP layer hardware resources initialization and the 00022 FMC controller configuration to interface with the external SDRAM memory. 00023 o It contains the SDRAM initialization sequence to program the SDRAM external 00024 device using the function BSP_SDRAM_Initialization_sequence(). Note that this 00025 sequence is standard for all SDRAM devices, but can include some differences 00026 from a device to another. If it is the case, the right sequence should be 00027 implemented separately. 00028 00029 + SDRAM read/write operations 00030 o SDRAM external memory can be accessed with read/write operations once it is 00031 initialized. 00032 Read/write operation can be performed with AHB access using the functions 00033 BSP_SDRAM_ReadData()/BSP_SDRAM_WriteData(), or by MDMA transfer using the functions 00034 BSP_SDRAM_ReadData_DMA()/BSP_SDRAM_WriteData_DMA(). 00035 o The AHB access is performed with 32-bit width transaction, the MDMA transfer 00036 configuration is fixed at single (no burst) word transfer (see the 00037 SDRAM_MspInit() static function). 00038 o User can implement his own functions for read/write access with his desired 00039 configurations. 00040 o If interrupt mode is used for MDMA transfer, the function BSP_SDRAM_MDMA_IRQHandler() 00041 is called in IRQ handler file, to serve the generated interrupt once the MDMA 00042 transfer is complete. 00043 o You can send a command to the SDRAM device in runtime using the function 00044 BSP_SDRAM_Sendcmd(), and giving the desired command as parameter chosen between 00045 the predefined commands of the "FMC_SDRAM_CommandTypeDef" structure. 00046 @endverbatim 00047 ****************************************************************************** 00048 * @attention 00049 * 00050 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00051 * 00052 * Redistribution and use in source and binary forms, with or without modification, 00053 * are permitted provided that the following conditions are met: 00054 * 1. Redistributions of source code must retain the above copyright notice, 00055 * this list of conditions and the following disclaimer. 00056 * 2. Redistributions in binary form must reproduce the above copyright notice, 00057 * this list of conditions and the following disclaimer in the documentation 00058 * and/or other materials provided with the distribution. 00059 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00060 * may be used to endorse or promote products derived from this software 00061 * without specific prior written permission. 00062 * 00063 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00064 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00065 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00066 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00067 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00068 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00069 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00070 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00071 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00072 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00073 * 00074 ****************************************************************************** 00075 */ 00076 00077 /* Includes ------------------------------------------------------------------*/ 00078 #include "stm32h743i_eval_sdram.h" 00079 00080 /** @addtogroup BSP 00081 * @{ 00082 */ 00083 00084 /** @addtogroup STM32H743I_EVAL 00085 * @{ 00086 */ 00087 00088 /** @addtogroup STM32H743I_EVAL_SDRAM 00089 * @{ 00090 */ 00091 00092 /** @defgroup STM32H743I_EVAL_SDRAM_Private_Types_Definitions SDRAM Private Types Definitions 00093 * @{ 00094 */ 00095 /** 00096 * @} 00097 */ 00098 00099 /** @defgroup STM32H743I_EVAL_SDRAM_Private_Defines SDRAM Private Defines 00100 * @{ 00101 */ 00102 /** 00103 * @} 00104 */ 00105 00106 /** @defgroup STM32H743I_EVAL_SDRAM_Private_Macros SDRAM Private Macros 00107 * @{ 00108 */ 00109 /** 00110 * @} 00111 */ 00112 00113 /** @defgroup STM32H743I_EVAL_SDRAM_Private_Variables SDRAM Private Variables 00114 * @{ 00115 */ 00116 SDRAM_HandleTypeDef sdramHandle; 00117 static FMC_SDRAM_TimingTypeDef Timing; 00118 static FMC_SDRAM_CommandTypeDef Command; 00119 /** 00120 * @} 00121 */ 00122 00123 /** @defgroup STM32H743I_EVAL_SDRAM_Private_Function_Prototypes SDRAM Private Function Prototypes 00124 * @{ 00125 */ 00126 /** 00127 * @} 00128 */ 00129 00130 /** @addtogroup STM32H743I_EVAL_SDRAM_Exported_Functions 00131 * @{ 00132 */ 00133 00134 /** 00135 * @brief Initializes the SDRAM device. 00136 * @retval SDRAM status 00137 */ 00138 uint8_t BSP_SDRAM_Init(void) 00139 { 00140 static uint8_t sdramstatus = SDRAM_ERROR; 00141 /* SDRAM device configuration */ 00142 sdramHandle.Instance = FMC_SDRAM_DEVICE; 00143 00144 /* Timing configuration for 100Mhz as SDRAM clock frequency (System clock is up to 200Mhz) */ 00145 Timing.LoadToActiveDelay = 2; 00146 Timing.ExitSelfRefreshDelay = 7; 00147 Timing.SelfRefreshTime = 4; 00148 Timing.RowCycleDelay = 7; 00149 Timing.WriteRecoveryTime = 2; 00150 Timing.RPDelay = 2; 00151 Timing.RCDDelay = 2; 00152 00153 sdramHandle.Init.SDBank = FMC_SDRAM_BANK2; 00154 sdramHandle.Init.ColumnBitsNumber = FMC_SDRAM_COLUMN_BITS_NUM_9; 00155 sdramHandle.Init.RowBitsNumber = FMC_SDRAM_ROW_BITS_NUM_12; 00156 sdramHandle.Init.MemoryDataWidth = SDRAM_MEMORY_WIDTH; 00157 sdramHandle.Init.InternalBankNumber = FMC_SDRAM_INTERN_BANKS_NUM_4; 00158 sdramHandle.Init.CASLatency = FMC_SDRAM_CAS_LATENCY_3; 00159 sdramHandle.Init.WriteProtection = FMC_SDRAM_WRITE_PROTECTION_DISABLE; 00160 sdramHandle.Init.SDClockPeriod = SDCLOCK_PERIOD; 00161 sdramHandle.Init.ReadBurst = FMC_SDRAM_RBURST_ENABLE; 00162 sdramHandle.Init.ReadPipeDelay = FMC_SDRAM_RPIPE_DELAY_0; 00163 00164 /* SDRAM controller initialization */ 00165 00166 BSP_SDRAM_MspInit(&sdramHandle, NULL); /* __weak function can be rewritten by the application */ 00167 00168 if(HAL_SDRAM_Init(&sdramHandle, &Timing) != HAL_OK) 00169 { 00170 sdramstatus = SDRAM_ERROR; 00171 } 00172 else 00173 { 00174 /* SDRAM initialization sequence */ 00175 BSP_SDRAM_Initialization_sequence(REFRESH_COUNT); 00176 sdramstatus = SDRAM_OK; 00177 } 00178 00179 return sdramstatus; 00180 } 00181 00182 /** 00183 * @brief DeInitializes the SDRAM device. 00184 * @retval SDRAM status 00185 */ 00186 uint8_t BSP_SDRAM_DeInit(void) 00187 { 00188 static uint8_t sdramstatus = SDRAM_ERROR; 00189 /* SDRAM device de-initialization */ 00190 sdramHandle.Instance = FMC_SDRAM_DEVICE; 00191 00192 if(HAL_SDRAM_DeInit(&sdramHandle) != HAL_OK) 00193 { 00194 sdramstatus = SDRAM_ERROR; 00195 } 00196 else 00197 { 00198 sdramstatus = SDRAM_OK; 00199 } 00200 00201 /* SDRAM controller de-initialization */ 00202 BSP_SDRAM_MspDeInit(&sdramHandle, NULL); 00203 00204 return sdramstatus; 00205 } 00206 00207 /** 00208 * @brief Programs the SDRAM device. 00209 * @param RefreshCount: SDRAM refresh counter value 00210 * @retval None 00211 */ 00212 void BSP_SDRAM_Initialization_sequence(uint32_t RefreshCount) 00213 { 00214 __IO uint32_t tmpmrd = 0; 00215 00216 /* Step 1: Configure a clock configuration enable command */ 00217 Command.CommandMode = FMC_SDRAM_CMD_CLK_ENABLE; 00218 Command.CommandTarget = FMC_SDRAM_CMD_TARGET_BANK2; 00219 Command.AutoRefreshNumber = 1; 00220 Command.ModeRegisterDefinition = 0; 00221 00222 /* Send the command */ 00223 HAL_SDRAM_SendCommand(&sdramHandle, &Command, SDRAM_TIMEOUT); 00224 00225 /* Step 2: Insert 100 us minimum delay */ 00226 /* Inserted delay is equal to 1 ms due to systick time base unit (ms) */ 00227 HAL_Delay(1); 00228 00229 /* Step 3: Configure a PALL (precharge all) command */ 00230 Command.CommandMode = FMC_SDRAM_CMD_PALL; 00231 Command.CommandTarget = FMC_SDRAM_CMD_TARGET_BANK2; 00232 Command.AutoRefreshNumber = 1; 00233 Command.ModeRegisterDefinition = 0; 00234 00235 /* Send the command */ 00236 HAL_SDRAM_SendCommand(&sdramHandle, &Command, SDRAM_TIMEOUT); 00237 00238 /* Step 4: Configure an Auto Refresh command */ 00239 Command.CommandMode = FMC_SDRAM_CMD_AUTOREFRESH_MODE; 00240 Command.CommandTarget = FMC_SDRAM_CMD_TARGET_BANK2; 00241 Command.AutoRefreshNumber = 8; 00242 Command.ModeRegisterDefinition = 0; 00243 00244 /* Send the command */ 00245 HAL_SDRAM_SendCommand(&sdramHandle, &Command, SDRAM_TIMEOUT); 00246 00247 /* Step 5: Program the external memory mode register */ 00248 tmpmrd = (uint32_t)SDRAM_MODEREG_BURST_LENGTH_1 |\ 00249 SDRAM_MODEREG_BURST_TYPE_SEQUENTIAL |\ 00250 SDRAM_MODEREG_CAS_LATENCY_3 |\ 00251 SDRAM_MODEREG_OPERATING_MODE_STANDARD |\ 00252 SDRAM_MODEREG_WRITEBURST_MODE_SINGLE; 00253 00254 Command.CommandMode = FMC_SDRAM_CMD_LOAD_MODE; 00255 Command.CommandTarget = FMC_SDRAM_CMD_TARGET_BANK2; 00256 Command.AutoRefreshNumber = 1; 00257 Command.ModeRegisterDefinition = tmpmrd; 00258 00259 /* Send the command */ 00260 HAL_SDRAM_SendCommand(&sdramHandle, &Command, SDRAM_TIMEOUT); 00261 00262 /* Step 6: Set the refresh rate counter */ 00263 /* Set the device refresh rate */ 00264 HAL_SDRAM_ProgramRefreshRate(&sdramHandle, RefreshCount); 00265 } 00266 00267 /** 00268 * @brief Reads an amount of data from the SDRAM memory in polling mode. 00269 * @param uwStartAddress: Read start address 00270 * @param pData: Pointer to data to be read 00271 * @param uwDataSize: Size of read data from the memory 00272 * @retval SDRAM status 00273 */ 00274 uint8_t BSP_SDRAM_ReadData(uint32_t uwStartAddress, uint32_t *pData, uint32_t uwDataSize) 00275 { 00276 if(HAL_SDRAM_Read_32b(&sdramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00277 { 00278 return SDRAM_ERROR; 00279 } 00280 else 00281 { 00282 return SDRAM_OK; 00283 } 00284 } 00285 00286 /** 00287 * @brief Reads an amount of data from the SDRAM memory in DMA mode. 00288 * @param uwStartAddress: Read start address 00289 * @param pData: Pointer to data to be read 00290 * @param uwDataSize: Size of read data from the memory 00291 * @retval SDRAM status 00292 */ 00293 uint8_t BSP_SDRAM_ReadData_DMA(uint32_t uwStartAddress, uint32_t *pData, uint32_t uwDataSize) 00294 { 00295 if(HAL_SDRAM_Read_DMA(&sdramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00296 { 00297 return SDRAM_ERROR; 00298 } 00299 else 00300 { 00301 return SDRAM_OK; 00302 } 00303 } 00304 00305 /** 00306 * @brief Writes an amount of data to the SDRAM memory in polling mode. 00307 * @param uwStartAddress: Write start address 00308 * @param pData: Pointer to data to be written 00309 * @param uwDataSize: Size of written data from the memory 00310 * @retval SDRAM status 00311 */ 00312 uint8_t BSP_SDRAM_WriteData(uint32_t uwStartAddress, uint32_t *pData, uint32_t uwDataSize) 00313 { 00314 if(HAL_SDRAM_Write_32b(&sdramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00315 { 00316 return SDRAM_ERROR; 00317 } 00318 else 00319 { 00320 return SDRAM_OK; 00321 } 00322 } 00323 00324 /** 00325 * @brief Writes an amount of data to the SDRAM memory in DMA mode. 00326 * @param uwStartAddress: Write start address 00327 * @param pData: Pointer to data to be written 00328 * @param uwDataSize: Size of written data from the memory 00329 * @retval SDRAM status 00330 */ 00331 uint8_t BSP_SDRAM_WriteData_DMA(uint32_t uwStartAddress, uint32_t *pData, uint32_t uwDataSize) 00332 { 00333 if(HAL_SDRAM_Write_DMA(&sdramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00334 { 00335 return SDRAM_ERROR; 00336 } 00337 else 00338 { 00339 return SDRAM_OK; 00340 } 00341 } 00342 00343 /** 00344 * @brief Sends command to the SDRAM bank. 00345 * @param SdramCmd: Pointer to SDRAM command structure 00346 * @retval SDRAM status 00347 */ 00348 uint8_t BSP_SDRAM_Sendcmd(FMC_SDRAM_CommandTypeDef *SdramCmd) 00349 { 00350 if(HAL_SDRAM_SendCommand(&sdramHandle, SdramCmd, SDRAM_TIMEOUT) != HAL_OK) 00351 { 00352 return SDRAM_ERROR; 00353 } 00354 else 00355 { 00356 return SDRAM_OK; 00357 } 00358 } 00359 00360 /** 00361 * @brief Initializes SDRAM MSP. 00362 * @param hsdram: SDRAM handle 00363 * @param Params: Pointer to void 00364 * @retval None 00365 */ 00366 __weak void BSP_SDRAM_MspInit(SDRAM_HandleTypeDef *hsdram, void *Params) 00367 { 00368 static MDMA_HandleTypeDef mdma_handle; 00369 GPIO_InitTypeDef gpio_init_structure; 00370 00371 /* Enable FMC clock */ 00372 __HAL_RCC_FMC_CLK_ENABLE(); 00373 00374 /* Enable chosen MDMAx clock */ 00375 __MDMAx_CLK_ENABLE(); 00376 00377 /* Enable GPIOs clock */ 00378 __HAL_RCC_GPIOD_CLK_ENABLE(); 00379 __HAL_RCC_GPIOE_CLK_ENABLE(); 00380 __HAL_RCC_GPIOF_CLK_ENABLE(); 00381 __HAL_RCC_GPIOG_CLK_ENABLE(); 00382 __HAL_RCC_GPIOH_CLK_ENABLE(); 00383 __HAL_RCC_GPIOI_CLK_ENABLE(); 00384 00385 /* Common GPIO configuration */ 00386 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00387 gpio_init_structure.Pull = GPIO_PULLUP; 00388 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00389 gpio_init_structure.Alternate = GPIO_AF12_FMC; 00390 00391 /* GPIOD configuration */ 00392 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_8| GPIO_PIN_9 | GPIO_PIN_10 |\ 00393 GPIO_PIN_14 | GPIO_PIN_15; 00394 00395 00396 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00397 00398 /* GPIOE configuration */ 00399 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_7| GPIO_PIN_8 | GPIO_PIN_9 |\ 00400 GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 |\ 00401 GPIO_PIN_15; 00402 00403 HAL_GPIO_Init(GPIOE, &gpio_init_structure); 00404 00405 /* GPIOF configuration */ 00406 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2| GPIO_PIN_3 | GPIO_PIN_4 |\ 00407 GPIO_PIN_5 | GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 |\ 00408 GPIO_PIN_15; 00409 00410 HAL_GPIO_Init(GPIOF, &gpio_init_structure); 00411 00412 /* GPIOG configuration */ 00413 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 |\ 00414 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_8 | GPIO_PIN_15; 00415 HAL_GPIO_Init(GPIOG, &gpio_init_structure); 00416 00417 /* GPIOH configuration */ 00418 gpio_init_structure.Pin = GPIO_PIN_5 | GPIO_PIN_6 | GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 |\ 00419 GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 |\ 00420 GPIO_PIN_15; 00421 HAL_GPIO_Init(GPIOH, &gpio_init_structure); 00422 00423 /* GPIOI configuration */ 00424 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | GPIO_PIN_4 |\ 00425 GPIO_PIN_5 | GPIO_PIN_6 | GPIO_PIN_7 | GPIO_PIN_9 | GPIO_PIN_10; 00426 HAL_GPIO_Init(GPIOI, &gpio_init_structure); 00427 00428 /* Configure common MDMA parameters */ 00429 mdma_handle.Init.Request = MDMA_REQUEST_SW; 00430 mdma_handle.Init.TransferTriggerMode = MDMA_BLOCK_TRANSFER; 00431 mdma_handle.Init.Priority = MDMA_PRIORITY_HIGH; 00432 mdma_handle.Init.Endianness = MDMA_LITTLE_ENDIANNESS_PRESERVE; 00433 mdma_handle.Init.SourceInc = MDMA_SRC_INC_WORD; 00434 mdma_handle.Init.DestinationInc = MDMA_DEST_INC_WORD; 00435 mdma_handle.Init.SourceDataSize = MDMA_SRC_DATASIZE_WORD; 00436 mdma_handle.Init.DestDataSize = MDMA_DEST_DATASIZE_WORD; 00437 mdma_handle.Init.DataAlignment = MDMA_DATAALIGN_PACKENABLE; 00438 mdma_handle.Init.SourceBurst = MDMA_SOURCE_BURST_SINGLE; 00439 mdma_handle.Init.DestBurst = MDMA_DEST_BURST_SINGLE; 00440 mdma_handle.Init.BufferTransferLength = 128; 00441 mdma_handle.Init.SourceBlockAddressOffset = 0; 00442 mdma_handle.Init.DestBlockAddressOffset = 0; 00443 00444 mdma_handle.Instance = SDRAM_MDMAx_CHANNEL; 00445 00446 /* Associate the DMA handle */ 00447 __HAL_LINKDMA(hsdram, hmdma, mdma_handle); 00448 00449 /* Deinitialize the stream for new transfer */ 00450 HAL_MDMA_DeInit(&mdma_handle); 00451 00452 /* Configure the DMA stream */ 00453 HAL_MDMA_Init(&mdma_handle); 00454 00455 /* NVIC configuration for DMA transfer complete interrupt */ 00456 HAL_NVIC_SetPriority(SDRAM_MDMAx_IRQn, 0x0F, 0); 00457 HAL_NVIC_EnableIRQ(SDRAM_MDMAx_IRQn); 00458 } 00459 00460 /** 00461 * @brief DeInitializes SDRAM MSP. 00462 * @param hsdram: SDRAM handle 00463 * @param Params: Pointer to void 00464 * @retval None 00465 */ 00466 __weak void BSP_SDRAM_MspDeInit(SDRAM_HandleTypeDef *hsdram, void *Params) 00467 { 00468 static MDMA_HandleTypeDef mdma_handle; 00469 00470 /* Disable NVIC configuration for DMA interrupt */ 00471 HAL_NVIC_DisableIRQ(SDRAM_MDMAx_IRQn); 00472 00473 /* Deinitialize the stream for new transfer */ 00474 mdma_handle.Instance = SDRAM_MDMAx_CHANNEL; 00475 HAL_MDMA_DeInit(&mdma_handle); 00476 00477 /* GPIO pins clock, FMC clock and MDMA clock can be shut down in the applications 00478 by surcharging this __weak function */ 00479 } 00480 00481 /** 00482 * @} 00483 */ 00484 00485 /** 00486 * @} 00487 */ 00488 00489 /** 00490 * @} 00491 */ 00492 00493 /** 00494 * @} 00495 */ 00496 00497 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Aug 23 2017 17:45:13 for STM32H743I_EVAL BSP User Manual by
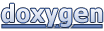