STM32H743I_EVAL BSP User Manual
|
stm32h743i_eval_sd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32h743i_eval_sd.c 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 21-April-2017 00007 * @brief This file includes the uSD card driver mounted on STM32H743I-EVAL 00008 * evaluation boards. 00009 @verbatim 00010 How To use this driver: 00011 ----------------------- 00012 - This driver is used to drive the micro SD external cards mounted on STM32H743I-EVAL 00013 evaluation board. 00014 - This driver does not need a specific component driver for the micro SD device 00015 to be included with. 00016 00017 Driver description: 00018 ------------------ 00019 + Initialization steps: 00020 o Initialize the micro SD card using the BSP_SD_Init() function. This 00021 function includes the MSP layer hardware resources initialization and the 00022 SDIO interface configuration to interface with the external micro SD. It 00023 also includes the micro SD initialization sequence for SDCard1. 00024 o To check the SD card presence you can use the function BSP_SD_IsDetected() which 00025 returns the detection status for SDCard1. The function BSP_SD_IsDetected() returns 00026 the detection status for SDCard1. 00027 o If SD presence detection interrupt mode is desired, you must configure the 00028 SD detection interrupt mode by calling the functions BSP_SD_ITConfig() for 00029 SDCard1. The interrupt is generated as an external interrupt whenever the 00030 micro SD card is plugged/unplugged in/from the evaluation board. The SD detection 00031 is managed by MFX, so the SD detection interrupt has to be treated by MFX_IRQOUT 00032 gpio pin IRQ handler. 00033 o The function BSP_SD_GetCardInfo() are used to get the micro SD card information 00034 which is stored in the structure "HAL_SD_CardInfoTypedef". 00035 00036 + Micro SD card operations 00037 o The micro SD card can be accessed with read/write block(s) operations once 00038 it is ready for access. The access, by default to SDCard1, can be performed whether 00039 using the polling mode by calling the functions BSP_SD_ReadBlocks()/BSP_SD_WriteBlocks(), 00040 or by DMA transfer using the functions BSP_SD_ReadBlocks_DMA()/BSP_SD_WriteBlocks_DMA(). 00041 o The DMA transfer complete is used with interrupt mode. Once the SD transfer 00042 is complete, the SD interrupt is handled using the function BSP_SDMMC1_IRQHandler() 00043 when SDCard1 is used. 00044 o The SD erase block(s) is performed using the functions BSP_SD_Erase() with specifying 00045 the number of blocks to erase. 00046 o The SD runtime status is returned when calling the function BSP_SD_GetStatus(). 00047 00048 @endverbatim 00049 ****************************************************************************** 00050 * @attention 00051 * 00052 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00053 * 00054 * Redistribution and use in source and binary forms, with or without modification, 00055 * are permitted provided that the following conditions are met: 00056 * 1. Redistributions of source code must retain the above copyright notice, 00057 * this list of conditions and the following disclaimer. 00058 * 2. Redistributions in binary form must reproduce the above copyright notice, 00059 * this list of conditions and the following disclaimer in the documentation 00060 * and/or other materials provided with the distribution. 00061 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00062 * may be used to endorse or promote products derived from this software 00063 * without specific prior written permission. 00064 * 00065 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00066 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00067 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00068 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00069 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00070 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00071 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00072 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00073 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00074 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00075 * 00076 ****************************************************************************** 00077 */ 00078 00079 /* Includes ------------------------------------------------------------------*/ 00080 #include "stm32h743i_eval_sd.h" 00081 00082 /** @addtogroup BSP 00083 * @{ 00084 */ 00085 00086 /** @addtogroup STM32H743I_EVAL 00087 * @{ 00088 */ 00089 00090 /** @addtogroup STM32H743I_EVAL_SD 00091 * @{ 00092 */ 00093 00094 00095 /** @defgroup STM32H743I_EVAL_SD_Private_TypesDefinitions SD Private TypesDefinitions 00096 * @{ 00097 */ 00098 /** 00099 * @} 00100 */ 00101 00102 /** @defgroup STM32H743I_EVAL_SD_Private_Defines SD Private Defines 00103 * @{ 00104 */ 00105 /** 00106 * @} 00107 */ 00108 00109 /** @defgroup STM32H743I_EVAL_SD_Private_Macros SD Private Macros 00110 * @{ 00111 */ 00112 /** 00113 * @} 00114 */ 00115 00116 /** @defgroup STM32H743I_EVAL_SD_Private_Variables SD Private Variables 00117 * @{ 00118 */ 00119 SD_HandleTypeDef uSdHandle; 00120 static uint8_t UseExtiModeDetection = 0; 00121 00122 /** 00123 * @} 00124 */ 00125 00126 /** @defgroup STM32H743I_EVAL_SD_Private_FunctionPrototypes SD Private FunctionPrototypes 00127 * @{ 00128 */ 00129 /** 00130 * @} 00131 */ 00132 00133 /** @addtogroup STM32H743I_EVAL_SD_Exported_Functions 00134 * @{ 00135 */ 00136 00137 /** 00138 * @brief Initializes the SD card device. 00139 * @retval SD status 00140 */ 00141 uint8_t BSP_SD_Init(void) 00142 { 00143 uint8_t sd_state = MSD_OK; 00144 00145 /* uSD device interface configuration */ 00146 uSdHandle.Instance = SDMMC1; 00147 00148 uSdHandle.Init.ClockDiv = 1; 00149 uSdHandle.Init.ClockPowerSave = SDMMC_CLOCK_POWER_SAVE_DISABLE; 00150 uSdHandle.Init.ClockEdge = SDMMC_CLOCK_EDGE_RISING; 00151 uSdHandle.Init.HardwareFlowControl = SDMMC_HARDWARE_FLOW_CONTROL_DISABLE; 00152 uSdHandle.Init.BusWide = SDMMC_BUS_WIDE_4B; 00153 00154 /* Initialize IO functionalities (MFX) used by SD detect pin and Transceiver MFXPIN */ 00155 BSP_IO_Init(); 00156 00157 /* Check if the SD card is plugged in the slot */ 00158 BSP_IO_ConfigPin(SD_DETECT_PIN, IO_MODE_INPUT_PU); 00159 00160 /* Initialise Transciver MFXPIN to enable 1.8V Switch mode */ 00161 BSP_IO_ConfigPin(SD_LDO_SEL_PIN, IO_MODE_OUTPUT_PP_PU); 00162 00163 if(BSP_SD_IsDetected() != SD_PRESENT) 00164 { 00165 return MSD_ERROR_SD_NOT_PRESENT; 00166 } 00167 00168 /* Msp SD initialization */ 00169 BSP_SD_MspInit(&uSdHandle, NULL); 00170 00171 /* HAL SD initialization */ 00172 if(HAL_SD_Init(&uSdHandle) != HAL_OK) 00173 { 00174 sd_state = MSD_ERROR; 00175 } 00176 00177 return sd_state; 00178 } 00179 00180 /** 00181 * @brief DeInitializes the SD card device. 00182 * @retval SD status 00183 */ 00184 uint8_t BSP_SD_DeInit(void) 00185 { 00186 uint8_t sd_state = MSD_OK; 00187 00188 uSdHandle.Instance = SDMMC1; 00189 00190 /* Set back Mfx pin to INPUT mode in case it was in exti */ 00191 UseExtiModeDetection = 0; 00192 00193 /* HAL SD deinitialization */ 00194 if(HAL_SD_DeInit(&uSdHandle) != HAL_OK) 00195 { 00196 sd_state = MSD_ERROR; 00197 } 00198 00199 /* Msp SD deinitialization */ 00200 uSdHandle.Instance = SDMMC1; 00201 BSP_SD_MspDeInit(&uSdHandle, NULL); 00202 00203 return sd_state; 00204 } 00205 00206 /** 00207 * @brief Configures Interrupt mode for SD1 detection pin. 00208 * @retval Returns 0 00209 */ 00210 uint8_t BSP_SD_ITConfig(void) 00211 { 00212 /* Configure Interrupt mode for SD detection pin */ 00213 /* Note: disabling exti mode can be done calling BSP_SD_DeInit() */ 00214 UseExtiModeDetection = 1; 00215 BSP_SD_IsDetected(); 00216 00217 return 0; 00218 } 00219 00220 /** 00221 * @brief Detects if SD card is correctly plugged in the memory slot or not. 00222 * @retval Returns if SD is detected or not 00223 */ 00224 uint8_t BSP_SD_IsDetected(void) 00225 { 00226 __IO uint8_t status = SD_PRESENT; 00227 00228 00229 /* Check SD card detect pin */ 00230 if((BSP_IO_ReadPin(SD_DETECT_PIN)&SD_DETECT_PIN) == SD_DETECT_PIN) 00231 { 00232 status = SD_NOT_PRESENT; 00233 } 00234 00235 if (UseExtiModeDetection) 00236 { 00237 if (status == SD_PRESENT) 00238 BSP_IO_ConfigPin(SD_DETECT_PIN, IO_MODE_IT_RISING_EDGE_PU); 00239 else 00240 BSP_IO_ConfigPin(SD_DETECT_PIN, IO_MODE_IT_FALLING_EDGE_PU); 00241 } 00242 00243 return status; 00244 } 00245 00246 00247 /** 00248 * @brief Reads block(s) from a specified address in an SD card, in polling mode. 00249 * @param pData: Pointer to the buffer that will contain the data to transmit 00250 * @param ReadAddr: Address from where data is to be read 00251 * @param NumOfBlocks: Number of SD blocks to read 00252 * @param Timeout: Timeout for read operation 00253 * @retval SD status 00254 */ 00255 uint8_t BSP_SD_ReadBlocks(uint32_t *pData, uint32_t ReadAddr, uint32_t NumOfBlocks, uint32_t Timeout) 00256 { 00257 00258 if( HAL_SD_ReadBlocks(&uSdHandle, (uint8_t *)pData, ReadAddr, NumOfBlocks, Timeout) == HAL_OK) 00259 { 00260 return MSD_OK; 00261 } 00262 else 00263 { 00264 return MSD_ERROR; 00265 } 00266 00267 } 00268 00269 /** 00270 * @brief Writes block(s) to a specified address in an SD card, in polling mode. 00271 * @param pData: Pointer to the buffer that will contain the data to transmit 00272 * @param WriteAddr: Address from where data is to be written 00273 * @param NumOfBlocks: Number of SD blocks to write 00274 * @param Timeout: Timeout for write operation 00275 * @retval SD status 00276 */ 00277 uint8_t BSP_SD_WriteBlocks(uint32_t *pData, uint32_t WriteAddr, uint32_t NumOfBlocks, uint32_t Timeout) 00278 { 00279 00280 if( HAL_SD_WriteBlocks(&uSdHandle, (uint8_t *)pData, WriteAddr, NumOfBlocks, Timeout) == HAL_OK) 00281 { 00282 return MSD_OK; 00283 } 00284 else 00285 { 00286 return MSD_ERROR; 00287 } 00288 } 00289 00290 /** 00291 * @brief Reads block(s) from a specified address in an SD card, in DMA mode. 00292 * @param pData: Pointer to the buffer that will contain the data to transmit 00293 * @param ReadAddr: Address from where data is to be read 00294 * @param NumOfBlocks: Number of SD blocks to read 00295 * @retval SD status 00296 */ 00297 uint8_t BSP_SD_ReadBlocks_DMA(uint32_t *pData, uint32_t ReadAddr, uint32_t NumOfBlocks) 00298 { 00299 00300 if( HAL_SD_ReadBlocks_DMA(&uSdHandle, (uint8_t *)pData, ReadAddr, NumOfBlocks) == HAL_OK) 00301 { 00302 return MSD_OK; 00303 } 00304 else 00305 { 00306 return MSD_ERROR; 00307 } 00308 } 00309 00310 /** 00311 * @brief Writes block(s) to a specified address in an SD card, in DMA mode. 00312 * @param pData: Pointer to the buffer that will contain the data to transmit 00313 * @param WriteAddr: Address from where data is to be written 00314 * @param NumOfBlocks: Number of SD blocks to write 00315 * @retval SD status 00316 */ 00317 uint8_t BSP_SD_WriteBlocks_DMA(uint32_t *pData, uint32_t WriteAddr, uint32_t NumOfBlocks) 00318 { 00319 00320 if( HAL_SD_WriteBlocks_DMA(&uSdHandle, (uint8_t *)pData, WriteAddr, NumOfBlocks) == HAL_OK) 00321 { 00322 return MSD_OK; 00323 } 00324 else 00325 { 00326 return MSD_ERROR; 00327 } 00328 00329 } 00330 00331 /** 00332 * @brief Erases the specified memory area of the given SD card. 00333 * @param StartAddr: Start byte address 00334 * @param EndAddr: End byte address 00335 * @retval SD status 00336 */ 00337 uint8_t BSP_SD_Erase(uint32_t StartAddr, uint32_t EndAddr) 00338 { 00339 00340 if( HAL_SD_Erase(&uSdHandle, StartAddr, EndAddr) == HAL_OK) 00341 { 00342 return MSD_OK; 00343 } 00344 else 00345 { 00346 return MSD_ERROR; 00347 } 00348 } 00349 00350 /** 00351 * @brief Initializes the SD MSP. 00352 * @param hsd: SD handle 00353 * @param Params: Pointer to void 00354 * @retval None 00355 */ 00356 __weak void BSP_SD_MspInit(SD_HandleTypeDef *hsd, void *Params) 00357 { 00358 00359 GPIO_InitTypeDef gpio_init_structure; 00360 00361 /* Enable SDIO clock */ 00362 __HAL_RCC_SDMMC1_CLK_ENABLE(); 00363 00364 /* Enable GPIOs clock */ 00365 __HAL_RCC_GPIOB_CLK_ENABLE(); 00366 __HAL_RCC_GPIOC_CLK_ENABLE(); 00367 __HAL_RCC_GPIOD_CLK_ENABLE(); 00368 00369 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00370 gpio_init_structure.Pull = GPIO_NOPULL; 00371 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00372 00373 /* D0(PC8), D1(PC9), D2(PC10), D3(PC11), CK(PC12), CMD(PD2) */ 00374 /* Common GPIO configuration */ 00375 gpio_init_structure.Alternate = GPIO_AF12_SDIO1; 00376 00377 /* GPIOC configuration */ 00378 gpio_init_structure.Pin = GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12; 00379 HAL_GPIO_Init(GPIOC, &gpio_init_structure); 00380 00381 /* GPIOD configuration */ 00382 gpio_init_structure.Pin = GPIO_PIN_2; 00383 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00384 00385 /* D0DIR(PC6), D123DIR(PC7) */ 00386 gpio_init_structure.Alternate = GPIO_AF8_SDIO1; 00387 /* GPIOC configuration */ 00388 gpio_init_structure.Pin = GPIO_PIN_6 | GPIO_PIN_7; 00389 HAL_GPIO_Init(GPIOC, &gpio_init_structure); 00390 00391 /* CKIN(PB8), CDIR(PB9) */ 00392 gpio_init_structure.Alternate = GPIO_AF7_SDIO1; 00393 /* GPIOB configuration */ 00394 gpio_init_structure.Pin = GPIO_PIN_8 | GPIO_PIN_9; 00395 HAL_GPIO_Init(GPIOB, &gpio_init_structure); 00396 00397 __HAL_RCC_SDMMC1_FORCE_RESET(); 00398 __HAL_RCC_SDMMC1_RELEASE_RESET(); 00399 00400 /* NVIC configuration for SDIO interrupts */ 00401 HAL_NVIC_SetPriority(SDMMC1_IRQn, 5, 0); 00402 HAL_NVIC_EnableIRQ(SDMMC1_IRQn); 00403 00404 00405 } 00406 00407 /** 00408 * @brief DeInitializes the SD MSP. 00409 * @param hsd: SD handle 00410 * @param Params: Pointer to void 00411 * @retval None 00412 */ 00413 __weak void BSP_SD_MspDeInit(SD_HandleTypeDef *hsd, void *Params) 00414 { 00415 /* Disable NVIC for SDIO interrupts */ 00416 HAL_NVIC_DisableIRQ(SDMMC1_IRQn); 00417 00418 /* DeInit GPIO pins can be done in the application 00419 (by surcharging this __weak function) */ 00420 00421 /* Disable SDMMC1 clock */ 00422 __HAL_RCC_SDMMC1_CLK_DISABLE(); 00423 00424 /* GPIO pins clock and DMA clocks can be shut down in the application 00425 by surcharging this __weak function */ 00426 } 00427 00428 /** 00429 * @brief Gets the current SD card data status. 00430 * @retval Data transfer state. 00431 * This value can be one of the following values: 00432 * @arg SD_TRANSFER_OK: No data transfer is acting 00433 * @arg SD_TRANSFER_BUSY: Data transfer is acting 00434 */ 00435 uint8_t BSP_SD_GetCardState(void) 00436 { 00437 return((HAL_SD_GetCardState(&uSdHandle) == HAL_SD_CARD_TRANSFER ) ? SD_TRANSFER_OK : SD_TRANSFER_BUSY); 00438 } 00439 00440 00441 /** 00442 * @brief Get SD information about specific SD card. 00443 * @param CardInfo: Pointer to HAL_SD_CardInfoTypedef structure 00444 * @retval None 00445 */ 00446 void BSP_SD_GetCardInfo(BSP_SD_CardInfo *CardInfo) 00447 { 00448 HAL_SD_GetCardInfo(&uSdHandle, CardInfo); 00449 } 00450 00451 00452 /** 00453 * @brief BSP SD Abort callbacks 00454 * @retval None 00455 */ 00456 __weak void BSP_SD_AbortCallback(void) 00457 { 00458 00459 } 00460 00461 /** 00462 * @brief BSP Tx Transfer completed callbacks 00463 * @retval None 00464 */ 00465 __weak void BSP_SD_WriteCpltCallback(void) 00466 { 00467 00468 } 00469 00470 /** 00471 * @brief BSP Rx Transfer completed callbacks 00472 * @retval None 00473 */ 00474 __weak void BSP_SD_ReadCpltCallback(void) 00475 { 00476 00477 } 00478 00479 00480 /** 00481 * @brief BSP Error callbacks 00482 * @retval None 00483 */ 00484 __weak void BSP_SD_ErrorCallback(void) 00485 { 00486 00487 } 00488 00489 00490 00491 00492 /** 00493 * @brief BSP SD Transceiver 1.8V Mode Callback. 00494 */ 00495 __weak void BSP_SD_DriveTransciver_1_8V_Callback(FlagStatus status) 00496 { 00497 if(status == SET) 00498 { 00499 BSP_IO_WritePin(IO_PIN_13, BSP_IO_PIN_SET); 00500 } 00501 else 00502 { 00503 BSP_IO_WritePin(IO_PIN_13, BSP_IO_PIN_RESET); 00504 } 00505 } 00506 00507 /** 00508 * @} 00509 */ 00510 00511 /** 00512 * @brief SD Abort callbacks 00513 * @param hsd: SD handle 00514 * @retval None 00515 */ 00516 void HAL_SD_AbortCallback(SD_HandleTypeDef *hsd) 00517 { 00518 BSP_SD_AbortCallback(); 00519 } 00520 00521 00522 /** 00523 * @brief Tx Transfer completed callbacks 00524 * @param hsd: SD handle 00525 * @retval None 00526 */ 00527 void HAL_SD_TxCpltCallback(SD_HandleTypeDef *hsd) 00528 { 00529 BSP_SD_WriteCpltCallback(); 00530 } 00531 00532 /** 00533 * @brief Rx Transfer completed callbacks 00534 * @param hsd: SD handle 00535 * @retval None 00536 */ 00537 void HAL_SD_RxCpltCallback(SD_HandleTypeDef *hsd) 00538 { 00539 BSP_SD_ReadCpltCallback(); 00540 } 00541 00542 /** 00543 * @brief Error callbacks 00544 * @param hsd: SD handle 00545 * @retval None 00546 */ 00547 void HAL_SD_ErrorCallback(SD_HandleTypeDef *hsd) 00548 { 00549 BSP_SD_ErrorCallback(); 00550 } 00551 00552 /** 00553 * @brief Enable the SD Transceiver 1.8V Mode Callback. 00554 */ 00555 void HAL_SD_DriveTransciver_1_8V_Callback(FlagStatus status) 00556 { 00557 BSP_SD_DriveTransciver_1_8V_Callback(status); 00558 } 00559 00560 /** 00561 * @} 00562 */ 00563 00564 /** 00565 * @} 00566 */ 00567 00568 /** 00569 * @} 00570 */ 00571 00572 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Aug 23 2017 17:45:13 for STM32H743I_EVAL BSP User Manual by
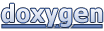