STM32H743I_EVAL BSP User Manual
|
stm32h743i_eval_lcd.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32h743i_eval_lcd.h 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 21-April-2017 00007 * @brief This file contains the common defines and functions prototypes for 00008 * the stm32h743i_eval_lcd.c driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM32H743I_EVAL_LCD_H 00041 #define __STM32H743I_EVAL_LCD_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 /* Include LCD component Driver */ 00049 /* LCD integrated within MB1063 */ 00050 #include "../Components/ampire640480/ampire640480.h" 00051 /* LCD integrated within MB1046 */ 00052 #include "../Components/ampire480272/ampire480272.h" 00053 00054 /* Include IOExpander(STMPE811) component Driver */ 00055 #include "../Components/stmpe811/stmpe811.h" 00056 00057 /* Include SDRAM Driver */ 00058 #include "stm32h743i_eval_sdram.h" 00059 00060 #include "stm32h743i_eval.h" 00061 #include "../../../Utilities/Fonts/fonts.h" 00062 00063 /** @addtogroup BSP 00064 * @{ 00065 */ 00066 00067 /** @addtogroup STM32H743I_EVAL 00068 * @{ 00069 */ 00070 00071 /** @defgroup STM32H743I_EVAL_LCD LCD 00072 * @{ 00073 */ 00074 00075 /** @defgroup STM32H743I_EVAL_LCD_Exported_Types LCD Exported Types 00076 * @{ 00077 */ 00078 typedef struct 00079 { 00080 uint32_t TextColor; 00081 uint32_t BackColor; 00082 sFONT *pFont; 00083 }LCD_DrawPropTypeDef; 00084 00085 typedef struct 00086 { 00087 int16_t X; 00088 int16_t Y; 00089 }Point, * pPoint; 00090 00091 /** 00092 * @brief Line mode structures definition 00093 */ 00094 typedef enum 00095 { 00096 CENTER_MODE = 0x01, /* Center mode */ 00097 RIGHT_MODE = 0x02, /* Right mode */ 00098 LEFT_MODE = 0x03 /* Left mode */ 00099 }Text_AlignModeTypdef; 00100 00101 /** 00102 * @} 00103 */ 00104 00105 /** @defgroup STM32H743I_EVAL_LCD_Exported_Constants LCD Exported Constants 00106 * @{ 00107 */ 00108 #define MAX_LAYER_NUMBER ((uint32_t)2) 00109 00110 #define LCD_LayerCfgTypeDef LTDC_LayerCfgTypeDef 00111 00112 #define LTDC_ACTIVE_LAYER ((uint32_t)1) /* Layer 1 */ 00113 /** 00114 * @brief LCD status structure definition 00115 */ 00116 #define LCD_OK ((uint8_t)0x00) 00117 #define LCD_ERROR ((uint8_t)0x01) 00118 #define LCD_TIMEOUT ((uint8_t)0x02) 00119 00120 /** 00121 * @brief LCD FB_StartAddress 00122 */ 00123 #define LCD_FB_START_ADDRESS ((uint32_t)0xD0000000) 00124 00125 00126 /** 00127 * @brief LCD Layer_Number 00128 */ 00129 00130 /* The programmed LTDC pixel clock depends on the vertical refresh rate of the panel 60Hz => 25.16MHz and 00131 the LCD/SDRAM bandwidth affected by the several access on the bus and the number of used layers. 00132 when only one layer is enabled "LCD_MAX_PCLK" can be used and when two layers are enabled simultaneously 00133 or/and there is several access on the bus "LCD_MIN_PCLK" parameter is recommended */ 00134 #define LCD_MAX_PCLK ((uint8_t)0x00) 00135 #define LCD_MIN_PCLK ((uint8_t)0x01) 00136 00137 00138 /** 00139 * @brief LCD color 00140 */ 00141 #define LCD_COLOR_BLUE ((uint32_t)0xFF0000FF) 00142 #define LCD_COLOR_GREEN ((uint32_t)0xFF00FF00) 00143 #define LCD_COLOR_RED ((uint32_t)0xFFFF0000) 00144 #define LCD_COLOR_CYAN ((uint32_t)0xFF00FFFF) 00145 #define LCD_COLOR_MAGENTA ((uint32_t)0xFFFF00FF) 00146 #define LCD_COLOR_YELLOW ((uint32_t)0xFFFFFF00) 00147 #define LCD_COLOR_LIGHTBLUE ((uint32_t)0xFF8080FF) 00148 #define LCD_COLOR_LIGHTGREEN ((uint32_t)0xFF80FF80) 00149 #define LCD_COLOR_LIGHTRED ((uint32_t)0xFFFF8080) 00150 #define LCD_COLOR_LIGHTCYAN ((uint32_t)0xFF80FFFF) 00151 #define LCD_COLOR_LIGHTMAGENTA ((uint32_t)0xFFFF80FF) 00152 #define LCD_COLOR_LIGHTYELLOW ((uint32_t)0xFFFFFF80) 00153 #define LCD_COLOR_DARKBLUE ((uint32_t)0xFF000080) 00154 #define LCD_COLOR_DARKGREEN ((uint32_t)0xFF008000) 00155 #define LCD_COLOR_DARKRED ((uint32_t)0xFF800000) 00156 #define LCD_COLOR_DARKCYAN ((uint32_t)0xFF008080) 00157 #define LCD_COLOR_DARKMAGENTA ((uint32_t)0xFF800080) 00158 #define LCD_COLOR_DARKYELLOW ((uint32_t)0xFF808000) 00159 #define LCD_COLOR_WHITE ((uint32_t)0xFFFFFFFF) 00160 #define LCD_COLOR_LIGHTGRAY ((uint32_t)0xFFD3D3D3) 00161 #define LCD_COLOR_GRAY ((uint32_t)0xFF808080) 00162 #define LCD_COLOR_DARKGRAY ((uint32_t)0xFF404040) 00163 #define LCD_COLOR_BLACK ((uint32_t)0xFF000000) 00164 #define LCD_COLOR_BROWN ((uint32_t)0xFFA52A2A) 00165 #define LCD_COLOR_ORANGE ((uint32_t)0xFFFFA500) 00166 #define LCD_COLOR_TRANSPARENT ((uint32_t)0xFF000000) 00167 00168 /** 00169 * @brief LCD default font 00170 */ 00171 #define LCD_DEFAULT_FONT Font24 00172 00173 /** 00174 * @brief LCD Reload Types 00175 */ 00176 #define LCD_RELOAD_IMMEDIATE ((uint32_t)LTDC_SRCR_IMR) 00177 #define LCD_RELOAD_VERTICAL_BLANKING ((uint32_t)LTDC_SRCR_VBR) 00178 00179 /** 00180 * @} 00181 */ 00182 00183 /** @defgroup STM32H743I_EVAL_LCD_Exported_Functions LCD Exported Functions 00184 * @{ 00185 */ 00186 uint8_t BSP_LCD_Init(void); 00187 uint8_t BSP_LCD_InitEx(uint32_t PclkConfig); 00188 00189 uint8_t BSP_LCD_DeInit(void); 00190 uint32_t BSP_LCD_GetXSize(void); 00191 uint32_t BSP_LCD_GetYSize(void); 00192 void BSP_LCD_SetXSize(uint32_t imageWidthPixels); 00193 void BSP_LCD_SetYSize(uint32_t imageHeightPixels); 00194 00195 /* Functions using the LTDC controller */ 00196 void BSP_LCD_LayerDefaultInit(uint16_t LayerIndex, uint32_t FrameBuffer); 00197 void BSP_LCD_SetTransparency(uint32_t LayerIndex, uint8_t Transparency); 00198 void BSP_LCD_SetTransparency_NoReload(uint32_t LayerIndex, uint8_t Transparency); 00199 void BSP_LCD_SetLayerAddress(uint32_t LayerIndex, uint32_t Address); 00200 void BSP_LCD_SetLayerAddress_NoReload(uint32_t LayerIndex, uint32_t Address); 00201 void BSP_LCD_SetColorKeying(uint32_t LayerIndex, uint32_t RGBValue); 00202 void BSP_LCD_SetColorKeying_NoReload(uint32_t LayerIndex, uint32_t RGBValue); 00203 void BSP_LCD_ResetColorKeying(uint32_t LayerIndex); 00204 void BSP_LCD_ResetColorKeying_NoReload(uint32_t LayerIndex); 00205 void BSP_LCD_SetLayerWindow(uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00206 void BSP_LCD_SetLayerWindow_NoReload(uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00207 void BSP_LCD_SelectLayer(uint32_t LayerIndex); 00208 void BSP_LCD_SetLayerVisible(uint32_t LayerIndex, FunctionalState State); 00209 void BSP_LCD_SetLayerVisible_NoReload(uint32_t LayerIndex, FunctionalState State); 00210 void BSP_LCD_Relaod(uint32_t ReloadType); 00211 00212 void BSP_LCD_SetTextColor(uint32_t Color); 00213 uint32_t BSP_LCD_GetTextColor(void); 00214 void BSP_LCD_SetBackColor(uint32_t Color); 00215 uint32_t BSP_LCD_GetBackColor(void); 00216 void BSP_LCD_SetFont(sFONT *fonts); 00217 sFONT *BSP_LCD_GetFont(void); 00218 00219 uint32_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos); 00220 void BSP_LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint32_t pixel); 00221 void BSP_LCD_Clear(uint32_t Color); 00222 void BSP_LCD_ClearStringLine(uint32_t Line); 00223 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *ptr); 00224 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Text_AlignModeTypdef Mode); 00225 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii); 00226 00227 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length); 00228 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length); 00229 void BSP_LCD_DrawLine(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2); 00230 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00231 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius); 00232 void BSP_LCD_DrawPolygon(pPoint Points, uint16_t PointCount); 00233 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius); 00234 void BSP_LCD_DrawBitmap(uint32_t Xpos, uint32_t Ypos, uint8_t *pbmp); 00235 00236 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00237 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius); 00238 void BSP_LCD_FillPolygon(pPoint Points, uint16_t PointCount); 00239 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius); 00240 00241 void BSP_LCD_DisplayOff(void); 00242 void BSP_LCD_DisplayOn(void); 00243 00244 void BSP_LCD_SetBrightness(uint8_t BrightnessValue); 00245 00246 /* These functions can be modified in case the current settings 00247 need to be changed for specific application needs */ 00248 void BSP_LCD_MspInit(LTDC_HandleTypeDef *hltdc, void *Params); 00249 void BSP_LCD_MspDeInit(LTDC_HandleTypeDef *hltdc, void *Params); 00250 void BSP_LCD_ClockConfig(LTDC_HandleTypeDef *hltdc, void *Params); 00251 00252 /** 00253 * @} 00254 */ 00255 00256 /** 00257 * @} 00258 */ 00259 00260 /** 00261 * @} 00262 */ 00263 00264 /** 00265 * @} 00266 */ 00267 00268 #ifdef __cplusplus 00269 } 00270 #endif 00271 00272 #endif /* __STM32H743I_EVAL_LCD_H */ 00273 00274 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Aug 23 2017 17:45:13 for STM32H743I_EVAL BSP User Manual by
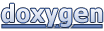