STM32H743I_EVAL BSP User Manual
|
stm32h743i_eval_lcd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32h743i_eval_lcd.c 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 21-April-2017 00007 * @brief This file includes the driver for Liquid Crystal Display (LCD) module 00008 * mounted on STM32H743I-EVAL evaluation board. 00009 @verbatim 00010 How To use this driver: 00011 ----------------------- 00012 - This driver is used to drive directly an LCD TFT using the LTDC controller. 00013 - This driver selects dynamically the mounted LCD, AMPIRE 640x480 LCD mounted 00014 on MB1063 or AMPIRE 480x272 LCD mounted on MB1046 daughter board, 00015 and uses the adequate timing and setting for the specified LCD using 00016 device ID of the STMPE811 mounted on MB1046 daughter board. 00017 00018 Driver description: 00019 ------------------ 00020 + Initialization steps: 00021 o Initialize the LCD using the BSP_LCD_Init() function. 00022 o Apply the Layer configuration using the BSP_LCD_LayerDefaultInit() function. 00023 o Select the LCD layer to be used using the BSP_LCD_SelectLayer() function. 00024 o Enable the LCD display using the BSP_LCD_DisplayOn() function. 00025 00026 + Options 00027 o Configure and enable the colour keying functionality using the 00028 BSP_LCD_SetColorKeying() function. 00029 o Modify in the fly the transparency and/or the frame buffer address 00030 using the following functions: 00031 - BSP_LCD_SetTransparency() 00032 - BSP_LCD_SetLayerAddress() 00033 00034 + Display on LCD 00035 o Clear the whole LCD using BSP_LCD_Clear() function or only one specified string 00036 line using the BSP_LCD_ClearStringLine() function. 00037 o Display a character on the specified line and column using the BSP_LCD_DisplayChar() 00038 function or a complete string line using the BSP_LCD_DisplayStringAtLine() function. 00039 o Display a string line on the specified position (x,y in pixel) and align mode 00040 using the BSP_LCD_DisplayStringAtLine() function. 00041 o Draw and fill a basic shapes (dot, line, rectangle, circle, ellipse, .. bitmap) 00042 on LCD using the available set of functions. 00043 @endverbatim 00044 ****************************************************************************** 00045 * @attention 00046 * 00047 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00048 * 00049 * Redistribution and use in source and binary forms, with or without modification, 00050 * are permitted provided that the following conditions are met: 00051 * 1. Redistributions of source code must retain the above copyright notice, 00052 * this list of conditions and the following disclaimer. 00053 * 2. Redistributions in binary form must reproduce the above copyright notice, 00054 * this list of conditions and the following disclaimer in the documentation 00055 * and/or other materials provided with the distribution. 00056 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00057 * may be used to endorse or promote products derived from this software 00058 * without specific prior written permission. 00059 * 00060 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00061 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00062 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00063 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00064 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00065 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00066 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00067 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00068 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00069 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00070 * 00071 ****************************************************************************** 00072 */ 00073 00074 /* Includes ------------------------------------------------------------------*/ 00075 #include "stm32h743i_eval_lcd.h" 00076 #include "../../../Utilities/Fonts/fonts.h" 00077 #include "../../../Utilities/Fonts/font24.c" 00078 #include "../../../Utilities/Fonts/font20.c" 00079 #include "../../../Utilities/Fonts/font16.c" 00080 #include "../../../Utilities/Fonts/font12.c" 00081 #include "../../../Utilities/Fonts/font8.c" 00082 00083 /** @addtogroup BSP 00084 * @{ 00085 */ 00086 00087 /** @addtogroup STM32H743I_EVAL 00088 * @{ 00089 */ 00090 00091 /** @addtogroup STM32H743I_EVAL_LCD 00092 * @{ 00093 */ 00094 00095 /** @defgroup STM32H743I_EVAL_LCD_Private_TypesDefinitions LCD Private TypesDefinitions 00096 * @{ 00097 */ 00098 /** 00099 * @} 00100 */ 00101 00102 /** @defgroup STM32H743I_EVAL_LCD_Private_Defines LCD Private Defines 00103 * @{ 00104 */ 00105 #define POLY_X(Z) ((int32_t)((Points + Z)->X)) 00106 #define POLY_Y(Z) ((int32_t)((Points + Z)->Y)) 00107 /** 00108 * @} 00109 */ 00110 00111 /** @defgroup STM32H743I_EVAL_LCD_Private_Macros LCD Private Macros 00112 * @{ 00113 */ 00114 #define ABS(X) ((X) > 0 ? (X) : -(X)) 00115 /** 00116 * @} 00117 */ 00118 00119 /** @defgroup STM32H743I_EVAL_LCD_Private_Variables LCD Private Variables 00120 * @{ 00121 */ 00122 DMA2D_HandleTypeDef hdma2d_eval; 00123 LTDC_HandleTypeDef hltdc_eval; 00124 static uint32_t PCLK_profile = LCD_MAX_PCLK; 00125 00126 /* Timer handler declaration */ 00127 static TIM_HandleTypeDef LCD_TimHandle; 00128 00129 /* Default LCD configuration with LCD Layer 1 */ 00130 static uint32_t ActiveLayer = 0; 00131 static LCD_DrawPropTypeDef DrawProp[MAX_LAYER_NUMBER]; 00132 /** 00133 * @} 00134 */ 00135 00136 /** @defgroup STM32H743I_EVAL_LCD_Private_FunctionPrototypes LCD Private FunctionPrototypes 00137 * @{ 00138 */ 00139 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c); 00140 static void FillTriangle(uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3); 00141 static void LL_FillBuffer(uint32_t LayerIndex, void *pDst, uint32_t xSize, uint32_t ySize, uint32_t OffLine, uint32_t ColorIndex); 00142 static void LL_ConvertLineToARGB8888(void * pSrc, void *pDst, uint32_t xSize, uint32_t ColorMode); 00143 static void TIMx_PWM_MspInit(TIM_HandleTypeDef *htim); 00144 static void TIMx_PWM_MspDeInit(TIM_HandleTypeDef *htim); 00145 static void TIMx_PWM_DeInit(TIM_HandleTypeDef *htim); 00146 static void TIMx_PWM_Init(TIM_HandleTypeDef *htim); 00147 00148 /** 00149 * @} 00150 */ 00151 00152 /** @addtogroup STM32H743I_EVAL_LCD_Exported_Functions 00153 * @{ 00154 */ 00155 00156 /** 00157 * @brief Initializes the LCD. 00158 * @retval LCD state 00159 */ 00160 uint8_t BSP_LCD_Init(void) 00161 { 00162 return (BSP_LCD_InitEx(LCD_MAX_PCLK)); 00163 } 00164 00165 /** 00166 * @brief Initializes the LCD. 00167 * @param PclkConfig : pixel clock profile 00168 * @retval LCD state 00169 */ 00170 uint8_t BSP_LCD_InitEx(uint32_t PclkConfig) 00171 { 00172 PCLK_profile = PclkConfig; 00173 /* Select the used LCD */ 00174 /* The AMPIRE 480x272 does not contain an ID register then we check the availability 00175 of AMPIRE 480x640 LCD using device ID of the STMPE811 mounted on MB1046 daughter board */ 00176 if(stmpe811_ts_drv.ReadID(TS_I2C_ADDRESS) == STMPE811_ID) 00177 { 00178 /* The AMPIRE LCD 480x272 is selected */ 00179 /* Timing Configuration */ 00180 hltdc_eval.Init.HorizontalSync = (AMPIRE480272_HSYNC - 1); 00181 hltdc_eval.Init.VerticalSync = (AMPIRE480272_VSYNC - 1); 00182 hltdc_eval.Init.AccumulatedHBP = (AMPIRE480272_HSYNC + AMPIRE480272_HBP - 1); 00183 hltdc_eval.Init.AccumulatedVBP = (AMPIRE480272_VSYNC + AMPIRE480272_VBP - 1); 00184 hltdc_eval.Init.AccumulatedActiveH = (AMPIRE480272_HEIGHT + AMPIRE480272_VSYNC + AMPIRE480272_VBP - 1); 00185 hltdc_eval.Init.AccumulatedActiveW = (AMPIRE480272_WIDTH + AMPIRE480272_HSYNC + AMPIRE480272_HBP - 1); 00186 hltdc_eval.Init.TotalHeigh = (AMPIRE480272_HEIGHT + AMPIRE480272_VSYNC + AMPIRE480272_VBP + AMPIRE480272_VFP - 1); 00187 hltdc_eval.Init.TotalWidth = (AMPIRE480272_WIDTH + AMPIRE480272_HSYNC + AMPIRE480272_HBP + AMPIRE480272_HFP - 1); 00188 00189 /* Initialize the LCD pixel width and pixel height */ 00190 hltdc_eval.LayerCfg->ImageWidth = AMPIRE480272_WIDTH; 00191 hltdc_eval.LayerCfg->ImageHeight = AMPIRE480272_HEIGHT; 00192 } 00193 else 00194 { 00195 /* The LCD AMPIRE 640x480 is selected */ 00196 /* Timing configuration */ 00197 hltdc_eval.Init.HorizontalSync = (AMPIRE640480_HSYNC - 1); 00198 hltdc_eval.Init.VerticalSync = (AMPIRE640480_VSYNC - 1); 00199 hltdc_eval.Init.AccumulatedHBP = (AMPIRE640480_HSYNC + AMPIRE640480_HBP - 1); 00200 hltdc_eval.Init.AccumulatedVBP = (AMPIRE640480_VSYNC + AMPIRE640480_VBP - 1); 00201 hltdc_eval.Init.AccumulatedActiveH = (AMPIRE640480_HEIGHT + AMPIRE640480_VSYNC + AMPIRE640480_VBP - 1); 00202 hltdc_eval.Init.AccumulatedActiveW = (AMPIRE640480_WIDTH + AMPIRE640480_HSYNC + AMPIRE640480_HBP - 1); 00203 hltdc_eval.Init.TotalHeigh = (AMPIRE640480_HEIGHT + AMPIRE640480_VSYNC + AMPIRE640480_VBP + AMPIRE640480_VFP - 1); 00204 hltdc_eval.Init.TotalWidth = (AMPIRE640480_WIDTH + AMPIRE640480_HSYNC + AMPIRE640480_HBP + AMPIRE640480_HFP - 1); 00205 00206 /* Initialize the LCD pixel width and pixel height */ 00207 hltdc_eval.LayerCfg->ImageWidth = AMPIRE640480_WIDTH; 00208 hltdc_eval.LayerCfg->ImageHeight = AMPIRE640480_HEIGHT; 00209 } 00210 00211 /* Background value */ 00212 hltdc_eval.Init.Backcolor.Blue = 0; 00213 hltdc_eval.Init.Backcolor.Green = 0; 00214 hltdc_eval.Init.Backcolor.Red = 0; 00215 00216 /* Polarity */ 00217 hltdc_eval.Init.HSPolarity = LTDC_HSPOLARITY_AL; 00218 hltdc_eval.Init.VSPolarity = LTDC_VSPOLARITY_AL; 00219 hltdc_eval.Init.DEPolarity = LTDC_DEPOLARITY_AL; 00220 hltdc_eval.Init.PCPolarity = LTDC_PCPOLARITY_IPC; 00221 hltdc_eval.Instance = LTDC; 00222 00223 /* LCD clock configuration */ 00224 BSP_LCD_ClockConfig(&hltdc_eval, &PCLK_profile); 00225 00226 if(HAL_LTDC_GetState(&hltdc_eval) == HAL_LTDC_STATE_RESET) 00227 { 00228 /* Initialize the LCD Msp: this __weak function can be rewritten by the application */ 00229 BSP_LCD_MspInit(&hltdc_eval, NULL); 00230 } 00231 HAL_LTDC_Init(&hltdc_eval); 00232 00233 #if !defined(DATA_IN_ExtSDRAM) 00234 /* When DATA_IN_ExtSDRAM define is enabled, the SDRAM will be configured in SystemInit() 00235 function (from system_stm32h7xx.c) before branch to main() routine. In such case, there 00236 is no need to reconfigure the SDRAM within the LCD driver, since it's already initialized. 00237 Otherwise the SDRAM must be configured. */ 00238 BSP_SDRAM_Init(); 00239 #endif 00240 00241 /* Initialize the font */ 00242 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00243 00244 /* Initialize TIM in PWM mode to control brightness */ 00245 TIMx_PWM_Init(&LCD_TimHandle); 00246 00247 return LCD_OK; 00248 } 00249 00250 /** 00251 * @brief DeInitializes the LCD. 00252 * @retval LCD state 00253 */ 00254 uint8_t BSP_LCD_DeInit(void) 00255 { 00256 /* Initialize the hltdc_eval Instance parameter */ 00257 hltdc_eval.Instance = LTDC; 00258 00259 /* Disable LTDC block */ 00260 __HAL_LTDC_DISABLE(&hltdc_eval); 00261 00262 /* DeInit the LTDC */ 00263 HAL_LTDC_DeInit(&hltdc_eval); 00264 00265 /* DeInit the LTDC MSP : this __weak function can be rewritten by the application */ 00266 BSP_LCD_MspDeInit(&hltdc_eval, NULL); 00267 00268 /* DeInit TIM PWM */ 00269 TIMx_PWM_DeInit(&LCD_TimHandle); 00270 00271 return LCD_OK; 00272 } 00273 00274 /** 00275 * @brief Gets the LCD X size. 00276 * @retval Used LCD X size 00277 */ 00278 uint32_t BSP_LCD_GetXSize(void) 00279 { 00280 return hltdc_eval.LayerCfg[ActiveLayer].ImageWidth; 00281 } 00282 00283 /** 00284 * @brief Gets the LCD Y size. 00285 * @retval Used LCD Y size 00286 */ 00287 uint32_t BSP_LCD_GetYSize(void) 00288 { 00289 return hltdc_eval.LayerCfg[ActiveLayer].ImageHeight; 00290 } 00291 00292 /** 00293 * @brief Set the LCD X size. 00294 * @param imageWidthPixels : image width in pixels unit 00295 * @retval None 00296 */ 00297 void BSP_LCD_SetXSize(uint32_t imageWidthPixels) 00298 { 00299 hltdc_eval.LayerCfg[ActiveLayer].ImageWidth = imageWidthPixels; 00300 } 00301 00302 /** 00303 * @brief Set the LCD Y size. 00304 * @param imageHeightPixels : image height in lines unit 00305 * @retval None 00306 */ 00307 void BSP_LCD_SetYSize(uint32_t imageHeightPixels) 00308 { 00309 hltdc_eval.LayerCfg[ActiveLayer].ImageHeight = imageHeightPixels; 00310 } 00311 00312 /** 00313 * @brief Initializes the LCD layers. 00314 * @param LayerIndex: Layer foreground or background 00315 * @param FB_Address: Layer frame buffer 00316 * @retval None 00317 */ 00318 void BSP_LCD_LayerDefaultInit(uint16_t LayerIndex, uint32_t FB_Address) 00319 { 00320 LCD_LayerCfgTypeDef layer_cfg; 00321 00322 /* Layer Init */ 00323 layer_cfg.WindowX0 = 0; 00324 layer_cfg.WindowX1 = BSP_LCD_GetXSize(); 00325 layer_cfg.WindowY0 = 0; 00326 layer_cfg.WindowY1 = BSP_LCD_GetYSize(); 00327 layer_cfg.PixelFormat = LTDC_PIXEL_FORMAT_ARGB8888; 00328 layer_cfg.FBStartAdress = FB_Address; 00329 layer_cfg.Alpha = 255; 00330 layer_cfg.Alpha0 = 0; 00331 layer_cfg.Backcolor.Blue = 0; 00332 layer_cfg.Backcolor.Green = 0; 00333 layer_cfg.Backcolor.Red = 0; 00334 layer_cfg.BlendingFactor1 = LTDC_BLENDING_FACTOR1_PAxCA; 00335 layer_cfg.BlendingFactor2 = LTDC_BLENDING_FACTOR2_PAxCA; 00336 layer_cfg.ImageWidth = BSP_LCD_GetXSize(); 00337 layer_cfg.ImageHeight = BSP_LCD_GetYSize(); 00338 00339 HAL_LTDC_ConfigLayer(&hltdc_eval, &layer_cfg, LayerIndex); 00340 00341 DrawProp[LayerIndex].BackColor = LCD_COLOR_WHITE; 00342 DrawProp[LayerIndex].pFont = &Font24; 00343 DrawProp[LayerIndex].TextColor = LCD_COLOR_BLACK; 00344 } 00345 00346 /** 00347 * @brief Selects the LCD Layer. 00348 * @param LayerIndex: Layer foreground or background 00349 * @retval None 00350 */ 00351 void BSP_LCD_SelectLayer(uint32_t LayerIndex) 00352 { 00353 ActiveLayer = LayerIndex; 00354 } 00355 00356 /** 00357 * @brief Sets an LCD Layer visible 00358 * @param LayerIndex: Visible Layer 00359 * @param State: New state of the specified layer 00360 * This parameter can be one of the following values: 00361 * @arg ENABLE 00362 * @arg DISABLE 00363 * @retval None 00364 */ 00365 void BSP_LCD_SetLayerVisible(uint32_t LayerIndex, FunctionalState State) 00366 { 00367 if(State == ENABLE) 00368 { 00369 __HAL_LTDC_LAYER_ENABLE(&hltdc_eval, LayerIndex); 00370 } 00371 else 00372 { 00373 __HAL_LTDC_LAYER_DISABLE(&hltdc_eval, LayerIndex); 00374 } 00375 __HAL_LTDC_RELOAD_IMMEDIATE_CONFIG(&(hltdc_eval)); 00376 } 00377 00378 /** 00379 * @brief Sets an LCD Layer visible without reloading. 00380 * @param LayerIndex: Visible Layer 00381 * @param State: New state of the specified layer 00382 * This parameter can be one of the following values: 00383 * @arg ENABLE 00384 * @arg DISABLE 00385 * @retval None 00386 */ 00387 void BSP_LCD_SetLayerVisible_NoReload(uint32_t LayerIndex, FunctionalState State) 00388 { 00389 if(State == ENABLE) 00390 { 00391 __HAL_LTDC_LAYER_ENABLE(&hltdc_eval, LayerIndex); 00392 } 00393 else 00394 { 00395 __HAL_LTDC_LAYER_DISABLE(&hltdc_eval, LayerIndex); 00396 } 00397 /* Do not Sets the Reload */ 00398 } 00399 00400 /** 00401 * @brief Configures the transparency. 00402 * @param LayerIndex: Layer foreground or background. 00403 * @param Transparency: Transparency 00404 * This parameter must be a number between Min_Data = 0x00 and Max_Data = 0xFF 00405 * @retval None 00406 */ 00407 void BSP_LCD_SetTransparency(uint32_t LayerIndex, uint8_t Transparency) 00408 { 00409 HAL_LTDC_SetAlpha(&hltdc_eval, Transparency, LayerIndex); 00410 } 00411 00412 /** 00413 * @brief Configures the transparency without reloading. 00414 * @param LayerIndex: Layer foreground or background. 00415 * @param Transparency: Transparency 00416 * This parameter must be a number between Min_Data = 0x00 and Max_Data = 0xFF 00417 * @retval None 00418 */ 00419 void BSP_LCD_SetTransparency_NoReload(uint32_t LayerIndex, uint8_t Transparency) 00420 { 00421 HAL_LTDC_SetAlpha_NoReload(&hltdc_eval, Transparency, LayerIndex); 00422 } 00423 00424 /** 00425 * @brief Sets an LCD layer frame buffer address. 00426 * @param LayerIndex: Layer foreground or background 00427 * @param Address: New LCD frame buffer value 00428 * @retval None 00429 */ 00430 void BSP_LCD_SetLayerAddress(uint32_t LayerIndex, uint32_t Address) 00431 { 00432 HAL_LTDC_SetAddress(&hltdc_eval, Address, LayerIndex); 00433 } 00434 00435 /** 00436 * @brief Sets an LCD layer frame buffer address without reloading. 00437 * @param LayerIndex: Layer foreground or background 00438 * @param Address: New LCD frame buffer value 00439 * @retval None 00440 */ 00441 void BSP_LCD_SetLayerAddress_NoReload(uint32_t LayerIndex, uint32_t Address) 00442 { 00443 HAL_LTDC_SetAddress_NoReload(&hltdc_eval, Address, LayerIndex); 00444 } 00445 00446 /** 00447 * @brief Sets display window. 00448 * @param LayerIndex: Layer index 00449 * @param Xpos: LCD X position 00450 * @param Ypos: LCD Y position 00451 * @param Width: LCD window width 00452 * @param Height: LCD window height 00453 * @retval None 00454 */ 00455 void BSP_LCD_SetLayerWindow(uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00456 { 00457 /* Reconfigure the layer size */ 00458 HAL_LTDC_SetWindowSize(&hltdc_eval, Width, Height, LayerIndex); 00459 00460 /* Reconfigure the layer position */ 00461 HAL_LTDC_SetWindowPosition(&hltdc_eval, Xpos, Ypos, LayerIndex); 00462 } 00463 00464 /** 00465 * @brief Sets display window without reloading. 00466 * @param LayerIndex: Layer index 00467 * @param Xpos: LCD X position 00468 * @param Ypos: LCD Y position 00469 * @param Width: LCD window width 00470 * @param Height: LCD window height 00471 * @retval None 00472 */ 00473 void BSP_LCD_SetLayerWindow_NoReload(uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00474 { 00475 /* Reconfigure the layer size */ 00476 HAL_LTDC_SetWindowSize_NoReload(&hltdc_eval, Width, Height, LayerIndex); 00477 00478 /* Reconfigure the layer position */ 00479 HAL_LTDC_SetWindowPosition_NoReload(&hltdc_eval, Xpos, Ypos, LayerIndex); 00480 } 00481 00482 /** 00483 * @brief Configures and sets the color keying. 00484 * @param LayerIndex: Layer foreground or background 00485 * @param RGBValue: Color reference 00486 * @retval None 00487 */ 00488 void BSP_LCD_SetColorKeying(uint32_t LayerIndex, uint32_t RGBValue) 00489 { 00490 /* Configure and Enable the color Keying for LCD Layer */ 00491 HAL_LTDC_ConfigColorKeying(&hltdc_eval, RGBValue, LayerIndex); 00492 HAL_LTDC_EnableColorKeying(&hltdc_eval, LayerIndex); 00493 } 00494 00495 /** 00496 * @brief Configures and sets the color keying without reloading. 00497 * @param LayerIndex: Layer foreground or background 00498 * @param RGBValue: Color reference 00499 * @retval None 00500 */ 00501 void BSP_LCD_SetColorKeying_NoReload(uint32_t LayerIndex, uint32_t RGBValue) 00502 { 00503 /* Configure and Enable the color Keying for LCD Layer */ 00504 HAL_LTDC_ConfigColorKeying_NoReload(&hltdc_eval, RGBValue, LayerIndex); 00505 HAL_LTDC_EnableColorKeying_NoReload(&hltdc_eval, LayerIndex); 00506 } 00507 00508 /** 00509 * @brief Disables the color keying. 00510 * @param LayerIndex: Layer foreground or background 00511 * @retval None 00512 */ 00513 void BSP_LCD_ResetColorKeying(uint32_t LayerIndex) 00514 { 00515 /* Disable the color Keying for LCD Layer */ 00516 HAL_LTDC_DisableColorKeying(&hltdc_eval, LayerIndex); 00517 } 00518 00519 /** 00520 * @brief Disables the color keying without reloading. 00521 * @param LayerIndex: Layer foreground or background 00522 * @retval None 00523 */ 00524 void BSP_LCD_ResetColorKeying_NoReload(uint32_t LayerIndex) 00525 { 00526 /* Disable the color Keying for LCD Layer */ 00527 HAL_LTDC_DisableColorKeying_NoReload(&hltdc_eval, LayerIndex); 00528 } 00529 00530 /** 00531 * @brief Disables the color keying without reloading. 00532 * @param ReloadType: can be one of the following values 00533 * - LCD_RELOAD_IMMEDIATE 00534 * - LCD_RELOAD_VERTICAL_BLANKING 00535 * @retval None 00536 */ 00537 void BSP_LCD_Relaod(uint32_t ReloadType) 00538 { 00539 HAL_LTDC_Reload (&hltdc_eval, ReloadType); 00540 } 00541 00542 /** 00543 * @brief Sets the LCD text color. 00544 * @param Color: Text color code ARGB(8-8-8-8) 00545 * @retval None 00546 */ 00547 void BSP_LCD_SetTextColor(uint32_t Color) 00548 { 00549 DrawProp[ActiveLayer].TextColor = Color; 00550 } 00551 00552 /** 00553 * @brief Gets the LCD text color. 00554 * @retval Used text color. 00555 */ 00556 uint32_t BSP_LCD_GetTextColor(void) 00557 { 00558 return DrawProp[ActiveLayer].TextColor; 00559 } 00560 00561 /** 00562 * @brief Sets the LCD background color. 00563 * @param Color: Layer background color code ARGB(8-8-8-8) 00564 * @retval None 00565 */ 00566 void BSP_LCD_SetBackColor(uint32_t Color) 00567 { 00568 DrawProp[ActiveLayer].BackColor = Color; 00569 } 00570 00571 /** 00572 * @brief Gets the LCD background color. 00573 * @retval Used background color 00574 */ 00575 uint32_t BSP_LCD_GetBackColor(void) 00576 { 00577 return DrawProp[ActiveLayer].BackColor; 00578 } 00579 00580 /** 00581 * @brief Sets the LCD text font. 00582 * @param fonts: Layer font to be used 00583 * @retval None 00584 */ 00585 void BSP_LCD_SetFont(sFONT *fonts) 00586 { 00587 DrawProp[ActiveLayer].pFont = fonts; 00588 } 00589 00590 /** 00591 * @brief Gets the LCD text font. 00592 * @retval Used layer font 00593 */ 00594 sFONT *BSP_LCD_GetFont(void) 00595 { 00596 return DrawProp[ActiveLayer].pFont; 00597 } 00598 00599 /** 00600 * @brief Reads an LCD pixel. 00601 * @param Xpos: X position 00602 * @param Ypos: Y position 00603 * @retval RGB pixel color 00604 */ 00605 uint32_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos) 00606 { 00607 uint32_t ret = 0; 00608 00609 if(hltdc_eval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_ARGB8888) 00610 { 00611 /* Read data value from SDRAM memory */ 00612 ret = *(__IO uint32_t*) (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (4*(Ypos*BSP_LCD_GetXSize() + Xpos))); 00613 } 00614 else if(hltdc_eval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB888) 00615 { 00616 /* Read data value from SDRAM memory */ 00617 ret = (*(__IO uint32_t*) (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (4*(Ypos*BSP_LCD_GetXSize() + Xpos))) & 0x00FFFFFF); 00618 } 00619 else if((hltdc_eval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB565) || \ 00620 (hltdc_eval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_ARGB4444) || \ 00621 (hltdc_eval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_AL88)) 00622 { 00623 /* Read data value from SDRAM memory */ 00624 ret = *(__IO uint16_t*) (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_LCD_GetXSize() + Xpos))); 00625 } 00626 else 00627 { 00628 /* Read data value from SDRAM memory */ 00629 ret = *(__IO uint8_t*) (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_LCD_GetXSize() + Xpos))); 00630 } 00631 00632 return ret; 00633 } 00634 00635 /** 00636 * @brief Clears the hole LCD. 00637 * @param Color: Color of the background 00638 * @retval None 00639 */ 00640 void BSP_LCD_Clear(uint32_t Color) 00641 { 00642 /* Clear the LCD */ 00643 LL_FillBuffer(ActiveLayer, (uint32_t *)(hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress), BSP_LCD_GetXSize(), BSP_LCD_GetYSize(), 0, Color); 00644 } 00645 00646 /** 00647 * @brief Clears the selected line. 00648 * @param Line: Line to be cleared 00649 * @retval None 00650 */ 00651 void BSP_LCD_ClearStringLine(uint32_t Line) 00652 { 00653 uint32_t color_backup = DrawProp[ActiveLayer].TextColor; 00654 DrawProp[ActiveLayer].TextColor = DrawProp[ActiveLayer].BackColor; 00655 00656 /* Draw rectangle with background color */ 00657 BSP_LCD_FillRect(0, (Line * DrawProp[ActiveLayer].pFont->Height), BSP_LCD_GetXSize(), DrawProp[ActiveLayer].pFont->Height); 00658 00659 DrawProp[ActiveLayer].TextColor = color_backup; 00660 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 00661 } 00662 00663 /** 00664 * @brief Displays one character. 00665 * @param Xpos: Start column address 00666 * @param Ypos: Line where to display the character shape. 00667 * @param Ascii: Character ascii code 00668 * This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E 00669 * @retval None 00670 */ 00671 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) 00672 { 00673 DrawChar(Xpos, Ypos, &DrawProp[ActiveLayer].pFont->table[(Ascii-' ') *\ 00674 DrawProp[ActiveLayer].pFont->Height * ((DrawProp[ActiveLayer].pFont->Width + 7) / 8)]); 00675 } 00676 00677 /** 00678 * @brief Displays characters on the LCD. 00679 * @param Xpos: X position (in pixel) 00680 * @param Ypos: Y position (in pixel) 00681 * @param Text: Pointer to string to display on LCD 00682 * @param Mode: Display mode 00683 * This parameter can be one of the following values: 00684 * @arg CENTER_MODE 00685 * @arg RIGHT_MODE 00686 * @arg LEFT_MODE 00687 * @retval None 00688 */ 00689 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Text_AlignModeTypdef Mode) 00690 { 00691 uint16_t ref_column = 1, i = 0; 00692 uint32_t size = 0, xsize = 0; 00693 uint8_t *ptr = Text; 00694 00695 /* Get the text size */ 00696 while (*ptr++) size ++ ; 00697 00698 /* Characters number per line */ 00699 xsize = (BSP_LCD_GetXSize()/DrawProp[ActiveLayer].pFont->Width); 00700 00701 switch (Mode) 00702 { 00703 case CENTER_MODE: 00704 { 00705 ref_column = Xpos + ((xsize - size)* DrawProp[ActiveLayer].pFont->Width) / 2; 00706 break; 00707 } 00708 case LEFT_MODE: 00709 { 00710 ref_column = Xpos; 00711 break; 00712 } 00713 case RIGHT_MODE: 00714 { 00715 ref_column = - Xpos + ((xsize - size)*DrawProp[ActiveLayer].pFont->Width); 00716 break; 00717 } 00718 default: 00719 { 00720 ref_column = Xpos; 00721 break; 00722 } 00723 } 00724 00725 /* Check that the Start column is located in the screen */ 00726 if ((ref_column < 1) || (ref_column >= 0x8000)) 00727 { 00728 ref_column = 1; 00729 } 00730 00731 /* Send the string character by character on LCD */ 00732 while ((*Text != 0) & (((BSP_LCD_GetXSize() - (i*DrawProp[ActiveLayer].pFont->Width)) & 0xFFFF) >= DrawProp[ActiveLayer].pFont->Width)) 00733 { 00734 /* Display one character on LCD */ 00735 BSP_LCD_DisplayChar(ref_column, Ypos, *Text); 00736 /* Decrement the column position by 16 */ 00737 ref_column += DrawProp[ActiveLayer].pFont->Width; 00738 /* Point on the next character */ 00739 Text++; 00740 i++; 00741 } 00742 } 00743 00744 /** 00745 * @brief Displays a maximum of 60 characters on the LCD. 00746 * @param Line: Line where to display the character shape 00747 * @param ptr: Pointer to string to display on LCD 00748 * @retval None 00749 */ 00750 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *ptr) 00751 { 00752 BSP_LCD_DisplayStringAt(0, LINE(Line), ptr, LEFT_MODE); 00753 } 00754 00755 /** 00756 * @brief Draws an horizontal line. 00757 * @param Xpos: X position 00758 * @param Ypos: Y position 00759 * @param Length: Line length 00760 * @retval None 00761 */ 00762 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00763 { 00764 uint32_t Xaddress = 0; 00765 00766 /* Get the line address */ 00767 Xaddress = (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_LCD_GetXSize()*Ypos + Xpos); 00768 00769 /* Write line */ 00770 LL_FillBuffer(ActiveLayer, (uint32_t *)Xaddress, Length, 1, 0, DrawProp[ActiveLayer].TextColor); 00771 } 00772 00773 /** 00774 * @brief Draws a vertical line. 00775 * @param Xpos: X position 00776 * @param Ypos: Y position 00777 * @param Length: Line length 00778 * @retval None 00779 */ 00780 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00781 { 00782 uint32_t Xaddress = 0; 00783 00784 /* Get the line address */ 00785 Xaddress = (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_LCD_GetXSize()*Ypos + Xpos); 00786 00787 /* Write line */ 00788 LL_FillBuffer(ActiveLayer, (uint32_t *)Xaddress, 1, Length, (BSP_LCD_GetXSize() - 1), DrawProp[ActiveLayer].TextColor); 00789 } 00790 00791 /** 00792 * @brief Draws an uni-line (between two points). 00793 * @param x1: Point 1 X position 00794 * @param y1: Point 1 Y position 00795 * @param x2: Point 2 X position 00796 * @param y2: Point 2 Y position 00797 * @retval None 00798 */ 00799 void BSP_LCD_DrawLine(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2) 00800 { 00801 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00802 yinc1 = 0, yinc2 = 0, den = 0, num = 0, num_add = 0, num_pixels = 0, 00803 curpixel = 0; 00804 00805 deltax = ABS(x2 - x1); /* The difference between the x's */ 00806 deltay = ABS(y2 - y1); /* The difference between the y's */ 00807 x = x1; /* Start x off at the first pixel */ 00808 y = y1; /* Start y off at the first pixel */ 00809 00810 if (x2 >= x1) /* The x-values are increasing */ 00811 { 00812 xinc1 = 1; 00813 xinc2 = 1; 00814 } 00815 else /* The x-values are decreasing */ 00816 { 00817 xinc1 = -1; 00818 xinc2 = -1; 00819 } 00820 00821 if (y2 >= y1) /* The y-values are increasing */ 00822 { 00823 yinc1 = 1; 00824 yinc2 = 1; 00825 } 00826 else /* The y-values are decreasing */ 00827 { 00828 yinc1 = -1; 00829 yinc2 = -1; 00830 } 00831 00832 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00833 { 00834 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00835 yinc2 = 0; /* Don't change the y for every iteration */ 00836 den = deltax; 00837 num = deltax / 2; 00838 num_add = deltay; 00839 num_pixels = deltax; /* There are more x-values than y-values */ 00840 } 00841 else /* There is at least one y-value for every x-value */ 00842 { 00843 xinc2 = 0; /* Don't change the x for every iteration */ 00844 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00845 den = deltay; 00846 num = deltay / 2; 00847 num_add = deltax; 00848 num_pixels = deltay; /* There are more y-values than x-values */ 00849 } 00850 00851 for (curpixel = 0; curpixel <= num_pixels; curpixel++) 00852 { 00853 BSP_LCD_DrawPixel(x, y, DrawProp[ActiveLayer].TextColor); /* Draw the current pixel */ 00854 num += num_add; /* Increase the numerator by the top of the fraction */ 00855 if (num >= den) /* Check if numerator >= denominator */ 00856 { 00857 num -= den; /* Calculate the new numerator value */ 00858 x += xinc1; /* Change the x as appropriate */ 00859 y += yinc1; /* Change the y as appropriate */ 00860 } 00861 x += xinc2; /* Change the x as appropriate */ 00862 y += yinc2; /* Change the y as appropriate */ 00863 } 00864 } 00865 00866 /** 00867 * @brief Draws a rectangle. 00868 * @param Xpos: X position 00869 * @param Ypos: Y position 00870 * @param Width: Rectangle width 00871 * @param Height: Rectangle height 00872 * @retval None 00873 */ 00874 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00875 { 00876 /* Draw horizontal lines */ 00877 BSP_LCD_DrawHLine(Xpos, Ypos, Width); 00878 BSP_LCD_DrawHLine(Xpos, (Ypos+ Height), Width); 00879 00880 /* Draw vertical lines */ 00881 BSP_LCD_DrawVLine(Xpos, Ypos, Height); 00882 BSP_LCD_DrawVLine((Xpos + Width), Ypos, Height); 00883 } 00884 00885 /** 00886 * @brief Draws a circle. 00887 * @param Xpos: X position 00888 * @param Ypos: Y position 00889 * @param Radius: Circle radius 00890 * @retval None 00891 */ 00892 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00893 { 00894 int32_t decision; /* Decision Variable */ 00895 uint32_t current_x; /* Current X Value */ 00896 uint32_t current_y; /* Current Y Value */ 00897 00898 decision = 3 - (Radius << 1); 00899 current_x = 0; 00900 current_y = Radius; 00901 00902 while (current_x <= current_y) 00903 { 00904 BSP_LCD_DrawPixel((Xpos + current_x), (Ypos - current_y), DrawProp[ActiveLayer].TextColor); 00905 00906 BSP_LCD_DrawPixel((Xpos - current_x), (Ypos - current_y), DrawProp[ActiveLayer].TextColor); 00907 00908 BSP_LCD_DrawPixel((Xpos + current_y), (Ypos - current_x), DrawProp[ActiveLayer].TextColor); 00909 00910 BSP_LCD_DrawPixel((Xpos - current_y), (Ypos - current_x), DrawProp[ActiveLayer].TextColor); 00911 00912 BSP_LCD_DrawPixel((Xpos + current_x), (Ypos + current_y), DrawProp[ActiveLayer].TextColor); 00913 00914 BSP_LCD_DrawPixel((Xpos - current_x), (Ypos + current_y), DrawProp[ActiveLayer].TextColor); 00915 00916 BSP_LCD_DrawPixel((Xpos + current_y), (Ypos + current_x), DrawProp[ActiveLayer].TextColor); 00917 00918 BSP_LCD_DrawPixel((Xpos - current_y), (Ypos + current_x), DrawProp[ActiveLayer].TextColor); 00919 00920 if (decision < 0) 00921 { 00922 decision += (current_x << 2) + 6; 00923 } 00924 else 00925 { 00926 decision += ((current_x - current_y) << 2) + 10; 00927 current_y--; 00928 } 00929 current_x++; 00930 } 00931 } 00932 00933 /** 00934 * @brief Draws an poly-line (between many points). 00935 * @param Points: Pointer to the points array 00936 * @param PointCount: Number of points 00937 * @retval None 00938 */ 00939 void BSP_LCD_DrawPolygon(pPoint Points, uint16_t PointCount) 00940 { 00941 int16_t x = 0, y = 0; 00942 00943 if(PointCount < 2) 00944 { 00945 return; 00946 } 00947 00948 BSP_LCD_DrawLine(Points->X, Points->Y, (Points+PointCount-1)->X, (Points+PointCount-1)->Y); 00949 00950 while(--PointCount) 00951 { 00952 x = Points->X; 00953 y = Points->Y; 00954 Points++; 00955 BSP_LCD_DrawLine(x, y, Points->X, Points->Y); 00956 } 00957 } 00958 00959 /** 00960 * @brief Draws an ellipse on LCD. 00961 * @param Xpos: X position 00962 * @param Ypos: Y position 00963 * @param XRadius: Ellipse X radius 00964 * @param YRadius: Ellipse Y radius 00965 * @retval None 00966 */ 00967 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00968 { 00969 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 00970 float k = 0, rad1 = 0, rad2 = 0; 00971 00972 rad1 = XRadius; 00973 rad2 = YRadius; 00974 00975 k = (float)(rad2/rad1); 00976 00977 do { 00978 BSP_LCD_DrawPixel((Xpos-(uint16_t)(x/k)), (Ypos+y), DrawProp[ActiveLayer].TextColor); 00979 BSP_LCD_DrawPixel((Xpos+(uint16_t)(x/k)), (Ypos+y), DrawProp[ActiveLayer].TextColor); 00980 BSP_LCD_DrawPixel((Xpos+(uint16_t)(x/k)), (Ypos-y), DrawProp[ActiveLayer].TextColor); 00981 BSP_LCD_DrawPixel((Xpos-(uint16_t)(x/k)), (Ypos-y), DrawProp[ActiveLayer].TextColor); 00982 00983 e2 = err; 00984 if (e2 <= x) { 00985 err += ++x*2+1; 00986 if (-y == x && e2 <= y) e2 = 0; 00987 } 00988 if (e2 > y) err += ++y*2+1; 00989 } 00990 while (y <= 0); 00991 } 00992 00993 /** 00994 * @brief Draws a pixel on LCD. 00995 * @param Xpos: X position 00996 * @param Ypos: Y position 00997 * @param RGB_Code: Pixel color in ARGB mode (8-8-8-8) 00998 * @retval None 00999 */ 01000 void BSP_LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint32_t RGB_Code) 01001 { 01002 /* Write data value to all SDRAM memory */ 01003 *(__IO uint32_t*) (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (4*(Ypos*BSP_LCD_GetXSize() + Xpos))) = RGB_Code; 01004 } 01005 01006 /** 01007 * @brief Draws a bitmap picture loaded in the internal Flash (32 bpp). 01008 * @param Xpos: Bmp X position in the LCD 01009 * @param Ypos: Bmp Y position in the LCD 01010 * @param pbmp: Pointer to Bmp picture address in the internal Flash 01011 * @retval None 01012 */ 01013 void BSP_LCD_DrawBitmap(uint32_t Xpos, uint32_t Ypos, uint8_t *pbmp) 01014 { 01015 uint32_t index = 0, width = 0, height = 0, bit_pixel = 0; 01016 uint32_t address; 01017 uint32_t input_color_mode = 0; 01018 01019 /* Get bitmap data address offset */ 01020 index = pbmp[10] + (pbmp[11] << 8) + (pbmp[12] << 16) + (pbmp[13] << 24); 01021 01022 /* Read bitmap width */ 01023 width = pbmp[18] + (pbmp[19] << 8) + (pbmp[20] << 16) + (pbmp[21] << 24); 01024 01025 /* Read bitmap height */ 01026 height = pbmp[22] + (pbmp[23] << 8) + (pbmp[24] << 16) + (pbmp[25] << 24); 01027 01028 /* Read bit/pixel */ 01029 bit_pixel = pbmp[28] + (pbmp[29] << 8); 01030 01031 /* Set the address */ 01032 address = hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (((BSP_LCD_GetXSize()*Ypos) + Xpos)*(4)); 01033 01034 /* Get the layer pixel format */ 01035 if ((bit_pixel/8) == 4) 01036 { 01037 input_color_mode = DMA2D_INPUT_ARGB8888; 01038 } 01039 else if ((bit_pixel/8) == 2) 01040 { 01041 input_color_mode = DMA2D_INPUT_RGB565; 01042 } 01043 else 01044 { 01045 input_color_mode = DMA2D_INPUT_RGB888; 01046 } 01047 01048 /* Bypass the bitmap header */ 01049 pbmp += (index + (width * (height - 1) * (bit_pixel/8))); 01050 01051 /* Convert picture to ARGB8888 pixel format */ 01052 for(index=0; index < height; index++) 01053 { 01054 /* Pixel format conversion */ 01055 LL_ConvertLineToARGB8888((uint32_t *)pbmp, (uint32_t *)address, width, input_color_mode); 01056 01057 /* Increment the source and destination buffers */ 01058 address+= (BSP_LCD_GetXSize()*4); 01059 pbmp -= width*(bit_pixel/8); 01060 } 01061 } 01062 01063 /** 01064 * @brief Draws a full rectangle. 01065 * @param Xpos: X position 01066 * @param Ypos: Y position 01067 * @param Width: Rectangle width 01068 * @param Height: Rectangle height 01069 * @retval None 01070 */ 01071 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 01072 { 01073 uint32_t x_address = 0; 01074 01075 /* Set the text color */ 01076 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 01077 01078 /* Get the rectangle start address */ 01079 x_address = (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_LCD_GetXSize()*Ypos + Xpos); 01080 01081 /* Fill the rectangle */ 01082 LL_FillBuffer(ActiveLayer, (uint32_t *)x_address, Width, Height, (BSP_LCD_GetXSize() - Width), DrawProp[ActiveLayer].TextColor); 01083 } 01084 01085 /** 01086 * @brief Draws a full circle. 01087 * @param Xpos: X position 01088 * @param Ypos: Y position 01089 * @param Radius: Circle radius 01090 * @retval None 01091 */ 01092 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 01093 { 01094 int32_t decision; /* Decision Variable */ 01095 uint32_t current_x; /* Current X Value */ 01096 uint32_t current_y; /* Current Y Value */ 01097 01098 decision = 3 - (Radius << 1); 01099 01100 current_x = 0; 01101 current_y = Radius; 01102 01103 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 01104 01105 while (current_x <= current_y) 01106 { 01107 if(current_y > 0) 01108 { 01109 BSP_LCD_DrawHLine(Xpos - current_y, Ypos + current_x, 2*current_y); 01110 BSP_LCD_DrawHLine(Xpos - current_y, Ypos - current_x, 2*current_y); 01111 } 01112 01113 if(current_x > 0) 01114 { 01115 BSP_LCD_DrawHLine(Xpos - current_x, Ypos - current_y, 2*current_x); 01116 BSP_LCD_DrawHLine(Xpos - current_x, Ypos + current_y, 2*current_x); 01117 } 01118 if (decision < 0) 01119 { 01120 decision += (current_x << 2) + 6; 01121 } 01122 else 01123 { 01124 decision += ((current_x - current_y) << 2) + 10; 01125 current_y--; 01126 } 01127 current_x++; 01128 } 01129 01130 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 01131 BSP_LCD_DrawCircle(Xpos, Ypos, Radius); 01132 } 01133 01134 /** 01135 * @brief Draws a full poly-line (between many points). 01136 * @param Points: Pointer to the points array 01137 * @param PointCount: Number of points 01138 * @retval None 01139 */ 01140 void BSP_LCD_FillPolygon(pPoint Points, uint16_t PointCount) 01141 { 01142 int16_t X = 0, Y = 0, X2 = 0, Y2 = 0, X_center = 0, Y_center = 0, X_first = 0, Y_first = 0, pixelX = 0, pixelY = 0, counter = 0; 01143 uint16_t image_left = 0, image_right = 0, image_top = 0, image_bottom = 0; 01144 01145 image_left = image_right = Points->X; 01146 image_top= image_bottom = Points->Y; 01147 01148 for(counter = 1; counter < PointCount; counter++) 01149 { 01150 pixelX = POLY_X(counter); 01151 if(pixelX < image_left) 01152 { 01153 image_left = pixelX; 01154 } 01155 if(pixelX > image_right) 01156 { 01157 image_right = pixelX; 01158 } 01159 01160 pixelY = POLY_Y(counter); 01161 if(pixelY < image_top) 01162 { 01163 image_top = pixelY; 01164 } 01165 if(pixelY > image_bottom) 01166 { 01167 image_bottom = pixelY; 01168 } 01169 } 01170 01171 if(PointCount < 2) 01172 { 01173 return; 01174 } 01175 01176 X_center = (image_left + image_right)/2; 01177 Y_center = (image_bottom + image_top)/2; 01178 01179 X_first = Points->X; 01180 Y_first = Points->Y; 01181 01182 while(--PointCount) 01183 { 01184 X = Points->X; 01185 Y = Points->Y; 01186 Points++; 01187 X2 = Points->X; 01188 Y2 = Points->Y; 01189 01190 FillTriangle(X, X2, X_center, Y, Y2, Y_center); 01191 FillTriangle(X, X_center, X2, Y, Y_center, Y2); 01192 FillTriangle(X_center, X2, X, Y_center, Y2, Y); 01193 } 01194 01195 FillTriangle(X_first, X2, X_center, Y_first, Y2, Y_center); 01196 FillTriangle(X_first, X_center, X2, Y_first, Y_center, Y2); 01197 FillTriangle(X_center, X2, X_first, Y_center, Y2, Y_first); 01198 } 01199 01200 /** 01201 * @brief Draws a full ellipse. 01202 * @param Xpos: X position 01203 * @param Ypos: Y position 01204 * @param XRadius: Ellipse X radius 01205 * @param YRadius: Ellipse Y radius 01206 * @retval None 01207 */ 01208 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 01209 { 01210 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 01211 float k = 0, rad1 = 0, rad2 = 0; 01212 01213 rad1 = XRadius; 01214 rad2 = YRadius; 01215 01216 k = (float)(rad2/rad1); 01217 01218 do 01219 { 01220 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/k)), (Ypos+y), (2*(uint16_t)(x/k) + 1)); 01221 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/k)), (Ypos-y), (2*(uint16_t)(x/k) + 1)); 01222 01223 e2 = err; 01224 if (e2 <= x) 01225 { 01226 err += ++x*2+1; 01227 if (-y == x && e2 <= y) e2 = 0; 01228 } 01229 if (e2 > y) err += ++y*2+1; 01230 } 01231 while (y <= 0); 01232 } 01233 01234 /** 01235 * @brief Enables the display. 01236 * @retval None 01237 */ 01238 void BSP_LCD_DisplayOn(void) 01239 { 01240 /* Display On */ 01241 __HAL_LTDC_ENABLE(&hltdc_eval); 01242 } 01243 01244 /** 01245 * @brief Disables the display. 01246 * @retval None 01247 */ 01248 void BSP_LCD_DisplayOff(void) 01249 { 01250 /* Display Off */ 01251 __HAL_LTDC_DISABLE(&hltdc_eval); 01252 } 01253 01254 /** 01255 * @brief Initializes the LTDC MSP. 01256 * @param hltdc: LTDC handle 01257 * @param Params: Pointer to void 01258 * @retval None 01259 */ 01260 __weak void BSP_LCD_MspInit(LTDC_HandleTypeDef *hltdc, void *Params) 01261 { 01262 GPIO_InitTypeDef gpio_init_structure; 01263 01264 /* Enable the LTDC and DMA2D clocks */ 01265 __HAL_RCC_LTDC_CLK_ENABLE(); 01266 __HAL_RCC_DMA2D_CLK_ENABLE(); 01267 01268 /* Enable GPIOs clock */ 01269 __HAL_RCC_GPIOI_CLK_ENABLE(); 01270 __HAL_RCC_GPIOJ_CLK_ENABLE(); 01271 __HAL_RCC_GPIOK_CLK_ENABLE(); 01272 01273 /*** LTDC Pins configuration ***/ 01274 /* GPIOI configuration */ 01275 gpio_init_structure.Pin = GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 01276 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 01277 gpio_init_structure.Pull = GPIO_NOPULL; 01278 gpio_init_structure.Speed = GPIO_SPEED_FREQ_HIGH; 01279 gpio_init_structure.Alternate = GPIO_AF14_LTDC; 01280 HAL_GPIO_Init(GPIOI, &gpio_init_structure); 01281 01282 /* GPIOJ configuration */ 01283 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | \ 01284 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6 | GPIO_PIN_7 | \ 01285 GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | \ 01286 GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 01287 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 01288 gpio_init_structure.Pull = GPIO_NOPULL; 01289 gpio_init_structure.Speed = GPIO_SPEED_FREQ_HIGH; 01290 gpio_init_structure.Alternate = GPIO_AF14_LTDC; 01291 HAL_GPIO_Init(GPIOJ, &gpio_init_structure); 01292 01293 /* GPIOK configuration */ 01294 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | \ 01295 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6 | GPIO_PIN_7; 01296 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 01297 gpio_init_structure.Pull = GPIO_NOPULL; 01298 gpio_init_structure.Speed = GPIO_SPEED_FREQ_HIGH; 01299 gpio_init_structure.Alternate = GPIO_AF14_LTDC; 01300 HAL_GPIO_Init(GPIOK, &gpio_init_structure); 01301 } 01302 01303 /** 01304 * @brief DeInitializes BSP_LCD MSP. 01305 * @param hltdc: LTDC handle 01306 * @param Params: Pointer to void 01307 * @retval None 01308 */ 01309 __weak void BSP_LCD_MspDeInit(LTDC_HandleTypeDef *hltdc, void *Params) 01310 { 01311 GPIO_InitTypeDef gpio_init_structure; 01312 01313 /* Disable LTDC block */ 01314 __HAL_LTDC_DISABLE(hltdc); 01315 01316 /* LTDC Pins deactivation */ 01317 /* GPIOI deactivation */ 01318 gpio_init_structure.Pin = GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 01319 HAL_GPIO_DeInit(GPIOI, gpio_init_structure.Pin); 01320 /* GPIOJ deactivation */ 01321 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | \ 01322 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6 | GPIO_PIN_7 | \ 01323 GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | \ 01324 GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 01325 HAL_GPIO_DeInit(GPIOJ, gpio_init_structure.Pin); 01326 /* GPIOK deactivation */ 01327 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | \ 01328 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6 | GPIO_PIN_7; 01329 HAL_GPIO_DeInit(GPIOK, gpio_init_structure.Pin); 01330 01331 /* Disable LTDC clock */ 01332 __HAL_RCC_LTDC_CLK_DISABLE(); 01333 01334 /* GPIO pins clock can be shut down in the application 01335 by surcharging this __weak function */ 01336 } 01337 01338 /** 01339 * @brief Clock Config. 01340 * @param hltdc: LTDC handle 01341 * @param Params: Pointer to void 01342 * @note This API is called by BSP_LCD_Init() 01343 * Being __weak it can be overwritten by the application 01344 * @retval None 01345 */ 01346 __weak void BSP_LCD_ClockConfig(LTDC_HandleTypeDef *hltdc, void *Params) 01347 { 01348 static RCC_PeriphCLKInitTypeDef periph_clk_init_struct; 01349 01350 if(stmpe811_ts_drv.ReadID(TS_I2C_ADDRESS) == STMPE811_ID) 01351 { 01352 /* AMPIRE480272 LCD clock configuration */ 01353 /* LCD clock configuration */ 01354 /* PLL3_VCO Input = HSE_VALUE/PLL3M = 5 Mhz */ 01355 /* PLL3_VCO Output = PLL3_VCO Input * PLL3N = 800 Mhz */ 01356 /* PLLLCDCLK = PLL3_VCO Output/PLL3R = 800/83 = 9.63 Mhz */ 01357 /* LTDC clock frequency = PLLLCDCLK = 9.63 Mhz */ 01358 periph_clk_init_struct.PeriphClockSelection = RCC_PERIPHCLK_LTDC; 01359 periph_clk_init_struct.PLL3.PLL3M = 5; 01360 periph_clk_init_struct.PLL3.PLL3N = 160; 01361 periph_clk_init_struct.PLL3.PLL3P = 2; 01362 periph_clk_init_struct.PLL3.PLL3Q = 2; 01363 periph_clk_init_struct.PLL3.PLL3R = 83; 01364 HAL_RCCEx_PeriphCLKConfig(&periph_clk_init_struct); 01365 } 01366 else 01367 { 01368 /* The programmed LTDC pixel clock depends on the vertical refresh rate of the panel 60Hz => 25.16MHz and 01369 the LCD/SDRAM bandwidth affected by the several access on the bus and the number of used layers. 01370 */ 01371 if(*(uint32_t *)Params == LCD_MAX_PCLK) 01372 { 01373 /* In case of single layer the bandwidth is arround 160MBytesPerSec ==> theorical PCLK of 40MHz */ 01374 /* AMPIRE640480 typical PCLK is 25 MHz so the PLL3R is configured to provide this clock */ 01375 /* AMPIRE640480 LCD clock configuration */ 01376 /* PLL3_VCO Input = HSE_VALUE/PLL3M = 5 Mhz */ 01377 /* PLL3_VCO Output = PLL3_VCO Input * PLL3N = 800 Mhz */ 01378 /* PLLLCDCLK = PLL3_VCO Output/PLL3R = 800/32 = 25Mhz */ 01379 /* LTDC clock frequency = PLLLCDCLK = 25 Mhz */ 01380 periph_clk_init_struct.PLL3.PLL3R = 32; 01381 } 01382 else 01383 { 01384 /* In case of double layers the bandwidth is arround 80MBytesPerSec => 20MHz (<25MHz) */ 01385 /* so the PLL3R is configured to provide this clock */ 01386 /* AMPIRE640480 LCD clock configuration */ 01387 /* PLL3_VCO Input = HSE_VALUE/PLL3M = 5 Mhz */ 01388 /* PLL3_VCO Output = PLL3_VCO Input * PLL3N = 800 Mhz */ 01389 /* PLLLCDCLK = PLL3_VCO Output/PLL3R = 800/40 = 20Mhz */ 01390 /* LTDC clock frequency = PLLLCDCLK = 20 Mhz */ 01391 periph_clk_init_struct.PLL3.PLL3R = 40; 01392 } 01393 periph_clk_init_struct.PeriphClockSelection = RCC_PERIPHCLK_LTDC; 01394 periph_clk_init_struct.PLL3.PLL3M = 5; 01395 periph_clk_init_struct.PLL3.PLL3N = 160; 01396 periph_clk_init_struct.PLL3.PLL3P = 2; 01397 periph_clk_init_struct.PLL3.PLL3Q = 2; 01398 HAL_RCCEx_PeriphCLKConfig(&periph_clk_init_struct); 01399 } 01400 } 01401 01402 /** 01403 * @brief Set the brightness value 01404 * @param BrightnessValue: [00: Min (black), 100 Max] 01405 */ 01406 void BSP_LCD_SetBrightness(uint8_t BrightnessValue) 01407 { 01408 /* Timer Configuration */ 01409 TIM_OC_InitTypeDef LCD_TIM_Config; 01410 01411 /* Stop PWM Timer channel */ 01412 HAL_TIM_PWM_Stop(&LCD_TimHandle, LCD_TIMx_CHANNEL); 01413 01414 /* Common configuration for all channels */ 01415 LCD_TIM_Config.OCMode = TIM_OCMODE_PWM1; 01416 LCD_TIM_Config.OCPolarity = TIM_OCPOLARITY_HIGH; 01417 LCD_TIM_Config.OCFastMode = TIM_OCFAST_DISABLE; 01418 LCD_TIM_Config.OCNPolarity = TIM_OCNPOLARITY_HIGH; 01419 LCD_TIM_Config.OCNIdleState = TIM_OCNIDLESTATE_RESET; 01420 LCD_TIM_Config.OCIdleState = TIM_OCIDLESTATE_RESET; 01421 01422 /* Set the pulse value for channel */ 01423 LCD_TIM_Config.Pulse = (uint32_t)((LCD_TIMX_PERIOD_VALUE * BrightnessValue) / 100); 01424 01425 HAL_TIM_PWM_ConfigChannel(&LCD_TimHandle, &LCD_TIM_Config, LCD_TIMx_CHANNEL); 01426 01427 /* Start PWM Timer channel */ 01428 HAL_TIM_PWM_Start(&LCD_TimHandle, LCD_TIMx_CHANNEL); 01429 } 01430 01431 /** 01432 * @} 01433 */ 01434 01435 /******************************************************************************* 01436 Static Functions 01437 *******************************************************************************/ 01438 01439 /** @defgroup STM32H743I_EVAL_LCD_Private_Functions LCD Private Functions 01440 * @{ 01441 */ 01442 01443 /** 01444 * @brief Draws a character on LCD. 01445 * @param Xpos: Line where to display the character shape 01446 * @param Ypos: Start column address 01447 * @param c: Pointer to the character data 01448 * @retval None 01449 */ 01450 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c) 01451 { 01452 uint32_t i = 0, j = 0; 01453 uint16_t height, width; 01454 uint8_t offset; 01455 uint8_t *pchar; 01456 uint32_t line; 01457 01458 height = DrawProp[ActiveLayer].pFont->Height; 01459 width = DrawProp[ActiveLayer].pFont->Width; 01460 01461 offset = 8 *((width + 7)/8) - width ; 01462 01463 for(i = 0; i < height; i++) 01464 { 01465 pchar = ((uint8_t *)c + (width + 7)/8 * i); 01466 01467 switch(((width + 7)/8)) 01468 { 01469 01470 case 1: 01471 line = pchar[0]; 01472 break; 01473 01474 case 2: 01475 line = (pchar[0]<< 8) | pchar[1]; 01476 break; 01477 01478 case 3: 01479 default: 01480 line = (pchar[0]<< 16) | (pchar[1]<< 8) | pchar[2]; 01481 break; 01482 } 01483 01484 for (j = 0; j < width; j++) 01485 { 01486 if(line & (1 << (width- j + offset- 1))) 01487 { 01488 BSP_LCD_DrawPixel((Xpos + j), Ypos, DrawProp[ActiveLayer].TextColor); 01489 } 01490 else 01491 { 01492 BSP_LCD_DrawPixel((Xpos + j), Ypos, DrawProp[ActiveLayer].BackColor); 01493 } 01494 } 01495 Ypos++; 01496 } 01497 } 01498 01499 /** 01500 * @brief Fills a triangle (between 3 points). 01501 * @param x1: Point 1 X position 01502 * @param y1: Point 1 Y position 01503 * @param x2: Point 2 X position 01504 * @param y2: Point 2 Y position 01505 * @param x3: Point 3 X position 01506 * @param y3: Point 3 Y position 01507 * @retval None 01508 */ 01509 static void FillTriangle(uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3) 01510 { 01511 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 01512 yinc1 = 0, yinc2 = 0, den = 0, num = 0, num_add = 0, num_pixels = 0, 01513 curpixel = 0; 01514 01515 deltax = ABS(x2 - x1); /* The difference between the x's */ 01516 deltay = ABS(y2 - y1); /* The difference between the y's */ 01517 x = x1; /* Start x off at the first pixel */ 01518 y = y1; /* Start y off at the first pixel */ 01519 01520 if (x2 >= x1) /* The x-values are increasing */ 01521 { 01522 xinc1 = 1; 01523 xinc2 = 1; 01524 } 01525 else /* The x-values are decreasing */ 01526 { 01527 xinc1 = -1; 01528 xinc2 = -1; 01529 } 01530 01531 if (y2 >= y1) /* The y-values are increasing */ 01532 { 01533 yinc1 = 1; 01534 yinc2 = 1; 01535 } 01536 else /* The y-values are decreasing */ 01537 { 01538 yinc1 = -1; 01539 yinc2 = -1; 01540 } 01541 01542 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 01543 { 01544 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 01545 yinc2 = 0; /* Don't change the y for every iteration */ 01546 den = deltax; 01547 num = deltax / 2; 01548 num_add = deltay; 01549 num_pixels = deltax; /* There are more x-values than y-values */ 01550 } 01551 else /* There is at least one y-value for every x-value */ 01552 { 01553 xinc2 = 0; /* Don't change the x for every iteration */ 01554 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 01555 den = deltay; 01556 num = deltay / 2; 01557 num_add = deltax; 01558 num_pixels = deltay; /* There are more y-values than x-values */ 01559 } 01560 01561 for (curpixel = 0; curpixel <= num_pixels; curpixel++) 01562 { 01563 BSP_LCD_DrawLine(x, y, x3, y3); 01564 01565 num += num_add; /* Increase the numerator by the top of the fraction */ 01566 if (num >= den) /* Check if numerator >= denominator */ 01567 { 01568 num -= den; /* Calculate the new numerator value */ 01569 x += xinc1; /* Change the x as appropriate */ 01570 y += yinc1; /* Change the y as appropriate */ 01571 } 01572 x += xinc2; /* Change the x as appropriate */ 01573 y += yinc2; /* Change the y as appropriate */ 01574 } 01575 } 01576 01577 /** 01578 * @brief Fills a buffer. 01579 * @param LayerIndex: Layer index 01580 * @param pDst: Pointer to destination buffer 01581 * @param xSize: Buffer width 01582 * @param ySize: Buffer height 01583 * @param OffLine: Offset 01584 * @param ColorIndex: Color index 01585 * @retval None 01586 */ 01587 static void LL_FillBuffer(uint32_t LayerIndex, void *pDst, uint32_t xSize, uint32_t ySize, uint32_t OffLine, uint32_t ColorIndex) 01588 { 01589 /* Register to memory mode with ARGB8888 as color Mode */ 01590 hdma2d_eval.Init.Mode = DMA2D_R2M; 01591 hdma2d_eval.Init.ColorMode = DMA2D_OUTPUT_ARGB8888; 01592 hdma2d_eval.Init.OutputOffset = OffLine; 01593 01594 hdma2d_eval.Instance = DMA2D; 01595 01596 /* DMA2D Initialization */ 01597 if(HAL_DMA2D_Init(&hdma2d_eval) == HAL_OK) 01598 { 01599 if(HAL_DMA2D_ConfigLayer(&hdma2d_eval, 1) == HAL_OK) 01600 { 01601 if (HAL_DMA2D_Start(&hdma2d_eval, ColorIndex, (uint32_t)pDst, xSize, ySize) == HAL_OK) 01602 { 01603 /* Polling For DMA transfer */ 01604 HAL_DMA2D_PollForTransfer(&hdma2d_eval, 10); 01605 } 01606 } 01607 } 01608 } 01609 01610 /** 01611 * @brief Converts a line to an ARGB8888 pixel format. 01612 * @param pSrc: Pointer to source buffer 01613 * @param pDst: Output color 01614 * @param xSize: Buffer width 01615 * @param ColorMode: Input color mode 01616 * @retval None 01617 */ 01618 static void LL_ConvertLineToARGB8888(void *pSrc, void *pDst, uint32_t xSize, uint32_t ColorMode) 01619 { 01620 /* Configure the DMA2D Mode, Color Mode and output offset */ 01621 hdma2d_eval.Init.Mode = DMA2D_M2M_PFC; 01622 hdma2d_eval.Init.ColorMode = DMA2D_OUTPUT_ARGB8888; 01623 hdma2d_eval.Init.OutputOffset = 0; 01624 01625 /* Foreground Configuration */ 01626 hdma2d_eval.LayerCfg[1].AlphaMode = DMA2D_NO_MODIF_ALPHA; 01627 hdma2d_eval.LayerCfg[1].InputAlpha = 0xFF; 01628 hdma2d_eval.LayerCfg[1].InputColorMode = ColorMode; 01629 hdma2d_eval.LayerCfg[1].InputOffset = 0; 01630 01631 hdma2d_eval.Instance = DMA2D; 01632 01633 /* DMA2D Initialization */ 01634 if(HAL_DMA2D_Init(&hdma2d_eval) == HAL_OK) 01635 { 01636 if(HAL_DMA2D_ConfigLayer(&hdma2d_eval, 1) == HAL_OK) 01637 { 01638 if (HAL_DMA2D_Start(&hdma2d_eval, (uint32_t)pSrc, (uint32_t)pDst, xSize, 1) == HAL_OK) 01639 { 01640 /* Polling For DMA transfer */ 01641 HAL_DMA2D_PollForTransfer(&hdma2d_eval, 10); 01642 } 01643 } 01644 } 01645 } 01646 01647 /** 01648 * @brief Initializes TIM MSP. 01649 * @param htim: TIM handle 01650 * @retval None 01651 */ 01652 static void TIMx_PWM_MspInit(TIM_HandleTypeDef *htim) 01653 { 01654 GPIO_InitTypeDef GPIO_InitStruct; 01655 01656 __HAL_RCC_GPIOA_CLK_ENABLE(); 01657 01658 /* TIMx Peripheral clock enable */ 01659 LCD_TIMx_CLK_ENABLE(); 01660 01661 /* Timer channel configuration */ 01662 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 01663 GPIO_InitStruct.Pull = GPIO_PULLUP; 01664 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 01665 GPIO_InitStruct.Alternate = LCD_TIMx_CHANNEL_AF; 01666 GPIO_InitStruct.Pin = GPIO_PIN_6; /* BL_CTRL */ 01667 01668 HAL_GPIO_Init(GPIOA, &GPIO_InitStruct); 01669 } 01670 01671 /** 01672 * @brief De-Initializes TIM MSP. 01673 * @param htim: TIM handle 01674 * @retval None 01675 */ 01676 static void TIMx_PWM_MspDeInit(TIM_HandleTypeDef *htim) 01677 { 01678 GPIO_InitTypeDef GPIO_InitStruct; 01679 01680 /* TIMx Peripheral clock enable */ 01681 LCD_TIMx_CLK_DISABLE(); 01682 01683 /* Timer channel configuration */ 01684 GPIO_InitStruct.Pin = GPIO_PIN_6; /* BL_CTRL */ 01685 HAL_GPIO_DeInit(GPIOA, GPIO_InitStruct.Pin); 01686 } 01687 01688 /** 01689 * @brief Initializes TIM in PWM mode 01690 * @param htim: TIM handle 01691 * @retval None 01692 */ 01693 static void TIMx_PWM_Init(TIM_HandleTypeDef *htim) 01694 { 01695 /* Timer_Clock = 2 x APB2_clock = 200 MHz */ 01696 /* PWM_freq = Timer_Clock /(Period x (Prescaler + 1))*/ 01697 /* PWM_freq = 200 MHz /(50000 x 5) = 800 Hz*/ 01698 01699 htim->Instance = LCD_TIMx; 01700 HAL_TIM_PWM_DeInit(htim); 01701 01702 TIMx_PWM_MspInit(htim); 01703 01704 htim->Init.Prescaler = LCD_TIMX_PRESCALER_VALUE; 01705 htim->Init.Period = LCD_TIMX_PERIOD_VALUE; 01706 htim->Init.ClockDivision = 0; 01707 htim->Init.CounterMode = TIM_COUNTERMODE_UP; 01708 htim->Init.RepetitionCounter = 0; 01709 01710 HAL_TIM_PWM_Init(htim); 01711 } 01712 01713 /** 01714 * @brief De-Initializes TIM in PWM mode 01715 * @param htim: TIM handle 01716 * @retval None 01717 */ 01718 static void TIMx_PWM_DeInit(TIM_HandleTypeDef *htim) 01719 { 01720 htim->Instance = LCD_TIMx; 01721 /* Timer de-intialization */ 01722 HAL_TIM_PWM_DeInit(htim); 01723 01724 /* Timer Msp de-intialization */ 01725 TIMx_PWM_MspDeInit(htim); 01726 } 01727 01728 01729 /** 01730 * @} 01731 */ 01732 01733 /** 01734 * @} 01735 */ 01736 01737 /** 01738 * @} 01739 */ 01740 01741 /** 01742 * @} 01743 */ 01744 01745 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 01746
Generated on Wed Aug 23 2017 17:45:13 for STM32H743I_EVAL BSP User Manual by
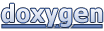