STM32H743I_EVAL BSP User Manual
|
stm32h743i_eval_io.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32h743i_eval_io.c 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 21-April-2017 00007 * @brief This file provides a set of functions needed to manage the IO pins 00008 * on STM32H743I-EVAL evaluation board. 00009 @verbatim 00010 How To use this driver: 00011 ----------------------- 00012 - This driver is used to drive the IO module of the STM32H743I-EVAL evaluation 00013 board. 00014 - The MFXSTM32L152 IO expander device component driver must be included with this 00015 driver in order to run the IO functionalities commanded by the IO expander (MFX) 00016 device mounted on the evaluation board. 00017 00018 Driver description: 00019 ------------------- 00020 + Initialization steps: 00021 o Initialize the IO module using the BSP_IO_Init() function. This 00022 function includes the MSP layer hardware resources initialization and the 00023 communication layer configuration to start the IO functionalities use. 00024 00025 + IO functionalities use 00026 o The IO pin mode is configured when calling the function BSP_IO_ConfigPin(), you 00027 must specify the desired IO mode by choosing the "IO_ModeTypedef" parameter 00028 predefined value. 00029 o If an IO pin is used in interrupt mode, the function BSP_IO_ITGetStatus() is 00030 needed to get the interrupt status. To clear the IT pending bits, you should 00031 call the function BSP_IO_ITClear() with specifying the IO pending bit to clear. 00032 o The IT is handled using the corresponding external interrupt IRQ handler, 00033 the user IT callback treatment is implemented on the same external interrupt 00034 callback. 00035 o The IRQ_OUT pin (common for all functionalities: JOY, SD, LEDs, etc) can be 00036 configured using the function BSP_IO_ConfigIrqOutPin() 00037 o To get/set an IO pin combination state you can use the functions 00038 BSP_IO_ReadPin()/BSP_IO_WritePin() or the function BSP_IO_TogglePin() to toggle the pin 00039 state. 00040 @endverbatim 00041 ****************************************************************************** 00042 * @attention 00043 * 00044 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00045 * 00046 * Redistribution and use in source and binary forms, with or without modification, 00047 * are permitted provided that the following conditions are met: 00048 * 1. Redistributions of source code must retain the above copyright notice, 00049 * this list of conditions and the following disclaimer. 00050 * 2. Redistributions in binary form must reproduce the above copyright notice, 00051 * this list of conditions and the following disclaimer in the documentation 00052 * and/or other materials provided with the distribution. 00053 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00054 * may be used to endorse or promote products derived from this software 00055 * without specific prior written permission. 00056 * 00057 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00058 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00059 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00060 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00061 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00062 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00063 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00064 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00065 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00066 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00067 * 00068 ****************************************************************************** 00069 */ 00070 00071 /* Includes ------------------------------------------------------------------*/ 00072 #include "stm32h743i_eval_io.h" 00073 00074 /** @addtogroup BSP 00075 * @{ 00076 */ 00077 00078 /** @addtogroup STM32H743I_EVAL 00079 * @{ 00080 */ 00081 00082 /** @addtogroup STM32H743I_EVAL_IO 00083 * @{ 00084 */ 00085 00086 /** @defgroup STM32H743I_EVAL_IO_Private_Types_Definitions IO Private Types Definitions 00087 * @{ 00088 */ 00089 /** 00090 * @} 00091 */ 00092 00093 /** @defgroup STM32H743I_EVAL_IO_Private_Defines IO Private Defines 00094 * @{ 00095 */ 00096 /** 00097 * @} 00098 */ 00099 00100 /** @defgroup STM32H743I_EVAL_IO_Private_Macros IO Private Macros 00101 * @{ 00102 */ 00103 /** 00104 * @} 00105 */ 00106 00107 /** @defgroup STM32H743I_EVAL_IO_Private_Variables IO Private Variables 00108 * @{ 00109 */ 00110 static IO_DrvTypeDef *IoDrv = NULL; 00111 static uint8_t mfxstm32l152Identifier; 00112 00113 /** 00114 * @} 00115 */ 00116 00117 /** @defgroup STM32H743I_EVAL_IO_Private_Function_Prototypes IO Private Function Prototypes 00118 * @{ 00119 */ 00120 /** 00121 * @} 00122 */ 00123 00124 /** @addtogroup STM32H743I_EVAL_IO_Exported_Functions 00125 * @{ 00126 */ 00127 00128 /** 00129 * @brief Initializes and configures the IO functionalities and configures all 00130 * necessary hardware resources (MFX, ...). 00131 * @note BSP_IO_Init() is using HAL_Delay() function to ensure that MFXSTM32L152 00132 * IO Expander is correctly reset. HAL_Delay() function provides accurate 00133 * delay (in milliseconds) based on variable incremented in SysTick ISR. 00134 * This implies that if BSP_IO_Init() is called from a peripheral ISR process, 00135 * then the SysTick interrupt must have higher priority (numerically lower) 00136 * than the peripheral interrupt. Otherwise the caller ISR process will be blocked. 00137 * @retval IO_OK if all initializations are OK. Other value if error. 00138 */ 00139 uint8_t BSP_IO_Init(void) 00140 { 00141 uint8_t ret = IO_OK; 00142 00143 if (IoDrv == NULL) /* Checks if MFX initialization never done */ 00144 { 00145 /* Read ID and verify the MFX is ready */ 00146 mfxstm32l152Identifier = mfxstm32l152_io_drv.ReadID(IO_I2C_ADDRESS); 00147 if((mfxstm32l152Identifier == MFXSTM32L152_ID_1) || (mfxstm32l152Identifier == MFXSTM32L152_ID_2)) 00148 { 00149 /* Initialize the IO driver structure */ 00150 IoDrv = &mfxstm32l152_io_drv; 00151 00152 /* Initialize MFX */ 00153 IoDrv->Init(IO_I2C_ADDRESS); 00154 IoDrv->Start(IO_I2C_ADDRESS, IO_PIN_ALL); 00155 } 00156 else 00157 { 00158 ret = IO_ERROR; 00159 } 00160 } 00161 else 00162 { 00163 /* MFX initialization already done : do nothing */ 00164 } 00165 00166 return ret; 00167 } 00168 00169 /** 00170 * @brief DeInit allows Mfx Initialization to be executed again 00171 * @note BSP_IO_Init() has no effect if the IoDrv is already initialized 00172 * BSP_IO_DeInit() allows to erase the pointer such to allow init to be effective 00173 * @retval IO_OK 00174 */ 00175 uint8_t BSP_IO_DeInit(void) 00176 { 00177 IoDrv = NULL; 00178 return IO_OK; 00179 } 00180 00181 /** 00182 * @brief Gets the selected pins IT status. 00183 * @param IoPin: Selected pins to check the status. 00184 * This parameter can be any combination of the IO pins. 00185 * @retval IO_OK if read status OK. Other value if error. 00186 */ 00187 uint32_t BSP_IO_ITGetStatus(uint32_t IoPin) 00188 { 00189 /* Return the IO Pin IT status */ 00190 return (IoDrv->ITStatus(IO_I2C_ADDRESS, IoPin)); 00191 } 00192 00193 /** 00194 * @brief Clears all the IO IT pending bits. 00195 * @retval None 00196 */ 00197 void BSP_IO_ITClear(void) 00198 { 00199 /* Clear all IO IT pending bits */ 00200 IoDrv->ClearIT(IO_I2C_ADDRESS, MFXSTM32L152_GPIO_PINS_ALL); 00201 } 00202 00203 /** 00204 * @brief Clear only one or a selection of IO IT pending bits. 00205 * @param IO_Pins_To_Clear : MFX IRQ status IO pin to clear (or combination of several IOs) 00206 */ 00207 void BSP_IO_ITClearPin(uint32_t IO_Pins_To_Clear) 00208 { 00209 /* Clear only the selected list of IO IT pending bits */ 00210 IoDrv->ClearIT(IO_I2C_ADDRESS, IO_Pins_To_Clear); 00211 } 00212 00213 /** 00214 * @brief Configures the IO pin(s) according to IO mode structure value. 00215 * @param IoPin: IO pin(s) to be configured. 00216 * This parameter can be one of the following values: 00217 * @arg MFXSTM32L152_GPIO_PIN_x: where x can be from 0 to 23. 00218 * @param IoMode: IO pin mode to configure 00219 * This parameter can be one of the following values: 00220 * @arg IO_MODE_INPUT 00221 * @arg IO_MODE_OUTPUT 00222 * @arg IO_MODE_IT_RISING_EDGE 00223 * @arg IO_MODE_IT_FALLING_EDGE 00224 * @arg IO_MODE_IT_LOW_LEVEL 00225 * @arg IO_MODE_IT_HIGH_LEVEL 00226 * @arg IO_MODE_ANALOG 00227 * @arg IO_MODE_OFF 00228 * @arg IO_MODE_INPUT_PU, 00229 * @arg IO_MODE_INPUT_PD, 00230 * @arg IO_MODE_OUTPUT_OD, 00231 * @arg IO_MODE_OUTPUT_OD_PU, 00232 * @arg IO_MODE_OUTPUT_OD_PD, 00233 * @arg IO_MODE_OUTPUT_PP, 00234 * @arg IO_MODE_OUTPUT_PP_PU, 00235 * @arg IO_MODE_OUTPUT_PP_PD, 00236 * @arg IO_MODE_IT_RISING_EDGE_PU 00237 * @arg IO_MODE_IT_FALLING_EDGE_PU 00238 * @arg IO_MODE_IT_LOW_LEVEL_PU 00239 * @arg IO_MODE_IT_HIGH_LEVEL_PU 00240 * @arg IO_MODE_IT_RISING_EDGE_PD 00241 * @arg IO_MODE_IT_FALLING_EDGE_PD 00242 * @arg IO_MODE_IT_LOW_LEVEL_PD 00243 * @arg IO_MODE_IT_HIGH_LEVEL_PD 00244 * @retval IO_OK if all initializations are OK. Other value if error. 00245 */ 00246 uint8_t BSP_IO_ConfigPin(uint32_t IoPin, IO_ModeTypedef IoMode) 00247 { 00248 /* Configure the selected IO pin(s) mode */ 00249 IoDrv->Config(IO_I2C_ADDRESS, IoPin, IoMode); 00250 00251 return IO_OK; 00252 } 00253 00254 /** 00255 * @brief Sets the IRQ_OUT pin polarity and type 00256 * @param IoIrqOutPinPolarity: High/Low 00257 * @param IoIrqOutPinType: OpenDrain/PushPull 00258 * @retval OK 00259 */ 00260 uint8_t BSP_IO_ConfigIrqOutPin(uint8_t IoIrqOutPinPolarity, uint8_t IoIrqOutPinType) 00261 { 00262 if((mfxstm32l152Identifier == MFXSTM32L152_ID_1) || (mfxstm32l152Identifier == MFXSTM32L152_ID_2)) 00263 { 00264 /* Initialize the IO driver structure */ 00265 mfxstm32l152_SetIrqOutPinPolarity(IO_I2C_ADDRESS, IoIrqOutPinPolarity); 00266 mfxstm32l152_SetIrqOutPinType(IO_I2C_ADDRESS, IoIrqOutPinType); 00267 } 00268 00269 return IO_OK; 00270 } 00271 00272 /** 00273 * @brief Sets the selected pins state. 00274 * @param IoPin: Selected pins to write. 00275 * This parameter can be any combination of the IO pins. 00276 * @param PinState: New pins state to write 00277 * @retval None 00278 */ 00279 void BSP_IO_WritePin(uint32_t IoPin, BSP_IO_PinStateTypeDef PinState) 00280 { 00281 /* Set the Pin state */ 00282 IoDrv->WritePin(IO_I2C_ADDRESS, IoPin, PinState); 00283 } 00284 00285 /** 00286 * @brief Gets the selected pins current state. 00287 * @param IoPin: Selected pins to read. 00288 * This parameter can be any combination of the IO pins. 00289 * @retval The current pins state 00290 */ 00291 uint32_t BSP_IO_ReadPin(uint32_t IoPin) 00292 { 00293 return(IoDrv->ReadPin(IO_I2C_ADDRESS, IoPin)); 00294 } 00295 00296 /** 00297 * @brief Toggles the selected pins state. 00298 * @param IoPin: Selected pins to toggle. 00299 * This parameter can be any combination of the IO pins. 00300 * @note This function is only used to toggle one pin in the same time 00301 * @retval None 00302 */ 00303 void BSP_IO_TogglePin(uint32_t IoPin) 00304 { 00305 /* Toggle the current pin state */ 00306 if(IoDrv->ReadPin(IO_I2C_ADDRESS, IoPin) != 0) /* Set */ 00307 { 00308 IoDrv->WritePin(IO_I2C_ADDRESS, IoPin, 0); /* Reset */ 00309 } 00310 else 00311 { 00312 IoDrv->WritePin(IO_I2C_ADDRESS, IoPin, 1); /* Set */ 00313 } 00314 } 00315 00316 /** 00317 * @} 00318 */ 00319 00320 /** 00321 * @} 00322 */ 00323 00324 /** 00325 * @} 00326 */ 00327 00328 /** 00329 * @} 00330 */ 00331 00332 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Aug 23 2017 17:45:13 for STM32H743I_EVAL BSP User Manual by
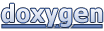