STM32F429I-Discovery BSP User Manual
|
Functions | |
uint8_t | BSP_LCD_Init (void) |
Initializes the LCD. | |
uint32_t | BSP_LCD_GetXSize (void) |
Gets the LCD X size. | |
uint32_t | BSP_LCD_GetYSize (void) |
Gets the LCD Y size. | |
void | BSP_LCD_LayerDefaultInit (uint16_t LayerIndex, uint32_t FrameBuffer) |
Initializes the LCD layers. | |
void | BSP_LCD_SetTransparency (uint32_t LayerIndex, uint8_t Transparency) |
Configures the Transparency. | |
void | BSP_LCD_SetTransparency_NoReload (uint32_t LayerIndex, uint8_t Transparency) |
Configures the transparency without reloading. | |
void | BSP_LCD_SetLayerAddress (uint32_t LayerIndex, uint32_t Address) |
Sets a LCD layer frame buffer address. | |
void | BSP_LCD_SetLayerAddress_NoReload (uint32_t LayerIndex, uint32_t Address) |
Sets an LCD layer frame buffer address without reloading. | |
void | BSP_LCD_SetColorKeying (uint32_t LayerIndex, uint32_t RGBValue) |
Configures and sets the color Keying. | |
void | BSP_LCD_SetColorKeying_NoReload (uint32_t LayerIndex, uint32_t RGBValue) |
Configures and sets the color keying without reloading. | |
void | BSP_LCD_ResetColorKeying (uint32_t LayerIndex) |
Disables the color Keying. | |
void | BSP_LCD_ResetColorKeying_NoReload (uint32_t LayerIndex) |
Disables the color keying without reloading. | |
void | BSP_LCD_SetLayerWindow (uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) |
Sets the Display window. | |
void | BSP_LCD_SetLayerWindow_NoReload (uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) |
Sets display window without reloading. | |
void | BSP_LCD_SelectLayer (uint32_t LayerIndex) |
Selects the LCD Layer. | |
void | BSP_LCD_SetLayerVisible (uint32_t LayerIndex, FunctionalState state) |
Sets a LCD Layer visible. | |
void | BSP_LCD_SetLayerVisible_NoReload (uint32_t LayerIndex, FunctionalState State) |
Sets an LCD Layer visible without reloading. | |
void | BSP_LCD_Relaod (uint32_t ReloadType) |
Disables the color keying without reloading. | |
void | BSP_LCD_SetTextColor (uint32_t Color) |
Sets the Text color. | |
void | BSP_LCD_SetBackColor (uint32_t Color) |
Sets the Background color. | |
uint32_t | BSP_LCD_GetTextColor (void) |
Gets the LCD Text color. | |
uint32_t | BSP_LCD_GetBackColor (void) |
Gets the LCD Background color. | |
void | BSP_LCD_SetFont (sFONT *pFonts) |
Sets the Text Font. | |
sFONT * | BSP_LCD_GetFont (void) |
Gets the Text Font. | |
uint32_t | BSP_LCD_ReadPixel (uint16_t Xpos, uint16_t Ypos) |
Reads Pixel. | |
void | BSP_LCD_DrawPixel (uint16_t Xpos, uint16_t Ypos, uint32_t pixel) |
Writes Pixel. | |
void | BSP_LCD_Clear (uint32_t Color) |
Clears the hole LCD. | |
void | BSP_LCD_ClearStringLine (uint32_t Line) |
Clears the selected line. | |
void | BSP_LCD_DisplayStringAtLine (uint16_t Line, uint8_t *ptr) |
Displays a maximum of 20 char on the LCD. | |
void | BSP_LCD_DisplayStringAt (uint16_t X, uint16_t Y, uint8_t *pText, Text_AlignModeTypdef mode) |
Displays a maximum of 60 char on the LCD. | |
void | BSP_LCD_DisplayChar (uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) |
Displays one character. | |
void | BSP_LCD_DrawHLine (uint16_t Xpos, uint16_t Ypos, uint16_t Length) |
Displays an horizontal line. | |
void | BSP_LCD_DrawVLine (uint16_t Xpos, uint16_t Ypos, uint16_t Length) |
Displays a vertical line. | |
void | BSP_LCD_DrawLine (uint16_t X1, uint16_t Y1, uint16_t X2, uint16_t Y2) |
Displays an uni-line (between two points). | |
void | BSP_LCD_DrawRect (uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) |
Displays a rectangle. | |
void | BSP_LCD_DrawCircle (uint16_t Xpos, uint16_t Ypos, uint16_t Radius) |
Displays a circle. | |
void | BSP_LCD_DrawPolygon (pPoint Points, uint16_t PointCount) |
Displays an poly-line (between many points). | |
void | BSP_LCD_DrawEllipse (int Xpos, int Ypos, int XRadius, int YRadius) |
Displays an Ellipse. | |
void | BSP_LCD_DrawBitmap (uint32_t X, uint32_t Y, uint8_t *pBmp) |
Displays a bitmap picture loaded in the internal Flash (32 bpp). | |
void | BSP_LCD_FillRect (uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) |
Displays a full rectangle. | |
void | BSP_LCD_FillCircle (uint16_t Xpos, uint16_t Ypos, uint16_t Radius) |
Displays a full circle. | |
void | BSP_LCD_FillTriangle (uint16_t X1, uint16_t X2, uint16_t X3, uint16_t Y1, uint16_t Y2, uint16_t Y3) |
Fill triangle. | |
void | BSP_LCD_FillPolygon (pPoint Points, uint16_t PointCount) |
Displays a full poly-line (between many points). | |
void | BSP_LCD_FillEllipse (int Xpos, int Ypos, int XRadius, int YRadius) |
Draw a full ellipse. | |
void | BSP_LCD_DisplayOff (void) |
Disables the Display. | |
void | BSP_LCD_DisplayOn (void) |
Enables the Display. | |
void | BSP_LCD_MspInit (void) |
Initializes the LTDC MSP. |
Function Documentation
void BSP_LCD_Clear | ( | uint32_t | Color | ) |
Clears the hole LCD.
- Parameters:
-
Color,: the color of the background
Definition at line 569 of file stm32f429i_discovery_lcd.c.
References ActiveLayer, BSP_LCD_GetXSize(), BSP_LCD_GetYSize(), FillBuffer(), and LtdcHandler.
void BSP_LCD_ClearStringLine | ( | uint32_t | Line | ) |
Clears the selected line.
- Parameters:
-
Line,: the line to be cleared
Definition at line 579 of file stm32f429i_discovery_lcd.c.
References ActiveLayer, LCD_DrawPropTypeDef::BackColor, BSP_LCD_FillRect(), BSP_LCD_GetXSize(), BSP_LCD_SetTextColor(), and LCD_DrawPropTypeDef::TextColor.
void BSP_LCD_DisplayChar | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint8_t | Ascii | ||
) |
Displays one character.
- Parameters:
-
Xpos,: start column address Ypos,: the Line where to display the character shape Ascii,: character ascii code, must be between 0x20 and 0x7E
Definition at line 597 of file stm32f429i_discovery_lcd.c.
References ActiveLayer, DrawChar(), and LCD_DrawPropTypeDef::pFont.
Referenced by BSP_LCD_DisplayStringAt().
void BSP_LCD_DisplayOff | ( | void | ) |
Disables the Display.
Definition at line 1214 of file stm32f429i_discovery_lcd.c.
References LcdDrv.
void BSP_LCD_DisplayOn | ( | void | ) |
void BSP_LCD_DisplayStringAt | ( | uint16_t | X, |
uint16_t | Y, | ||
uint8_t * | pText, | ||
Text_AlignModeTypdef | mode | ||
) |
Displays a maximum of 60 char on the LCD.
- Parameters:
-
X,: pointer to x position (in pixel) Y,: pointer to y position (in pixel) pText,: pointer to string to display on LCD mode,: The display mode This parameter can be one of the following values: - CENTER_MODE
- RIGHT_MODE
- LEFT_MODE
Definition at line 614 of file stm32f429i_discovery_lcd.c.
References ActiveLayer, BSP_LCD_DisplayChar(), BSP_LCD_GetXSize(), CENTER_MODE, LEFT_MODE, LCD_DrawPropTypeDef::pFont, and RIGHT_MODE.
Referenced by BSP_LCD_DisplayStringAtLine().
void BSP_LCD_DisplayStringAtLine | ( | uint16_t | Line, |
uint8_t * | ptr | ||
) |
Displays a maximum of 20 char on the LCD.
- Parameters:
-
Line,: the Line where to display the character shape ptr,: pointer to string to display on LCD
Definition at line 668 of file stm32f429i_discovery_lcd.c.
References BSP_LCD_DisplayStringAt(), and LEFT_MODE.
void BSP_LCD_DrawBitmap | ( | uint32_t | X, |
uint32_t | Y, | ||
uint8_t * | pBmp | ||
) |
Displays a bitmap picture loaded in the internal Flash (32 bpp).
- Parameters:
-
X,: the bmp x position in the LCD Y,: the bmp Y position in the LCD pBmp,: Bmp picture address in the internal Flash
Definition at line 903 of file stm32f429i_discovery_lcd.c.
References ActiveLayer, BSP_LCD_GetXSize(), ConvertLineToARGB8888(), and LtdcHandler.
void BSP_LCD_DrawCircle | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Radius | ||
) |
Displays a circle.
- Parameters:
-
Xpos,: the X position Ypos,: the Y position Radius,: the circle radius
Definition at line 805 of file stm32f429i_discovery_lcd.c.
References ActiveLayer, and BSP_LCD_DrawPixel().
Referenced by BSP_LCD_FillCircle().
void BSP_LCD_DrawEllipse | ( | int | Xpos, |
int | Ypos, | ||
int | XRadius, | ||
int | YRadius | ||
) |
Displays an Ellipse.
- Parameters:
-
Xpos,: the X position Ypos,: the Y position XRadius,: the X radius of ellipse YRadius,: the Y radius of ellipse
Definition at line 871 of file stm32f429i_discovery_lcd.c.
References ActiveLayer, and BSP_LCD_DrawPixel().
void BSP_LCD_DrawHLine | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Length | ||
) |
Displays an horizontal line.
- Parameters:
-
Xpos,: the X position Ypos,: the Y position Length,: line length
Definition at line 679 of file stm32f429i_discovery_lcd.c.
References ActiveLayer, BSP_LCD_GetXSize(), FillBuffer(), and LtdcHandler.
Referenced by BSP_LCD_DrawRect(), BSP_LCD_FillCircle(), and BSP_LCD_FillEllipse().
void BSP_LCD_DrawLine | ( | uint16_t | X1, |
uint16_t | Y1, | ||
uint16_t | X2, | ||
uint16_t | Y2 | ||
) |
Displays an uni-line (between two points).
- Parameters:
-
X1,: the point 1 X position Y1,: the point 1 Y position X2,: the point 2 X position Y2,: the point 2 Y position
Definition at line 714 of file stm32f429i_discovery_lcd.c.
References ABS, ActiveLayer, and BSP_LCD_DrawPixel().
Referenced by BSP_LCD_DrawPolygon(), and BSP_LCD_FillTriangle().
void BSP_LCD_DrawPixel | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint32_t | RGB_Code | ||
) |
Writes Pixel.
- Parameters:
-
Xpos,: the X position Ypos,: the Y position RGB_Code,: the pixel color in ARGB mode (8-8-8-8)
Definition at line 1313 of file stm32f429i_discovery_lcd.c.
References ActiveLayer, BSP_LCD_GetXSize(), and LtdcHandler.
Referenced by BSP_LCD_DrawCircle(), BSP_LCD_DrawEllipse(), BSP_LCD_DrawLine(), and DrawChar().
void BSP_LCD_DrawPolygon | ( | pPoint | Points, |
uint16_t | PointCount | ||
) |
Displays an poly-line (between many points).
- Parameters:
-
Points,: pointer to the points array PointCount,: Number of points
Definition at line 844 of file stm32f429i_discovery_lcd.c.
References BSP_LCD_DrawLine(), Point::X, and Point::Y.
void BSP_LCD_DrawRect | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Width, | ||
uint16_t | Height | ||
) |
Displays a rectangle.
- Parameters:
-
Xpos,: the X position Ypos,: the Y position Height,: display rectangle height Width,: display rectangle width
Definition at line 788 of file stm32f429i_discovery_lcd.c.
References BSP_LCD_DrawHLine(), and BSP_LCD_DrawVLine().
void BSP_LCD_DrawVLine | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Length | ||
) |
Displays a vertical line.
- Parameters:
-
Xpos,: the X position Ypos,: the Y position Length,: line length
Definition at line 696 of file stm32f429i_discovery_lcd.c.
References ActiveLayer, BSP_LCD_GetXSize(), FillBuffer(), and LtdcHandler.
Referenced by BSP_LCD_DrawRect().
void BSP_LCD_FillCircle | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Radius | ||
) |
Displays a full circle.
- Parameters:
-
Xpos,: the X position Ypos,: the Y position Radius,: the circle radius
Definition at line 983 of file stm32f429i_discovery_lcd.c.
References ActiveLayer, BSP_LCD_DrawCircle(), BSP_LCD_DrawHLine(), and BSP_LCD_SetTextColor().
void BSP_LCD_FillEllipse | ( | int | Xpos, |
int | Ypos, | ||
int | XRadius, | ||
int | YRadius | ||
) |
Draw a full ellipse.
- Parameters:
-
Xpos,: the X position Ypos,: the Y position XRadius,: X radius of ellipse YRadius,: Y radius of ellipse.
Definition at line 1175 of file stm32f429i_discovery_lcd.c.
References BSP_LCD_DrawHLine().
void BSP_LCD_FillPolygon | ( | pPoint | Points, |
uint16_t | PointCount | ||
) |
Displays a full poly-line (between many points).
- Parameters:
-
Points,: pointer to the points array PointCount,: Number of points
Definition at line 1107 of file stm32f429i_discovery_lcd.c.
References BSP_LCD_FillTriangle(), POLY_X, POLY_Y, Point::X, and Point::Y.
void BSP_LCD_FillRect | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Width, | ||
uint16_t | Height | ||
) |
Displays a full rectangle.
- Parameters:
-
Xpos,: the X position Ypos,: the Y position Height,: rectangle height Width,: rectangle width
Definition at line 963 of file stm32f429i_discovery_lcd.c.
References ActiveLayer, BSP_LCD_GetXSize(), BSP_LCD_SetTextColor(), FillBuffer(), and LtdcHandler.
Referenced by BSP_LCD_ClearStringLine().
void BSP_LCD_FillTriangle | ( | uint16_t | X1, |
uint16_t | X2, | ||
uint16_t | X3, | ||
uint16_t | Y1, | ||
uint16_t | Y2, | ||
uint16_t | Y3 | ||
) |
Fill triangle.
- Parameters:
-
X1,: the point 1 x position Y1,: the point 1 y position X2,: the point 2 x position Y2,: the point 2 y position X3,: the point 3 x position Y3,: the point 3 y position
Definition at line 1034 of file stm32f429i_discovery_lcd.c.
References ABS, and BSP_LCD_DrawLine().
Referenced by BSP_LCD_FillPolygon().
uint32_t BSP_LCD_GetBackColor | ( | void | ) |
Gets the LCD Background color.
- Return values:
-
Background color
Definition at line 488 of file stm32f429i_discovery_lcd.c.
References ActiveLayer, and LCD_DrawPropTypeDef::BackColor.
sFONT* BSP_LCD_GetFont | ( | void | ) |
Gets the Text Font.
- Return values:
-
Layer font
Definition at line 524 of file stm32f429i_discovery_lcd.c.
References ActiveLayer, and LCD_DrawPropTypeDef::pFont.
uint32_t BSP_LCD_GetTextColor | ( | void | ) |
Gets the LCD Text color.
- Return values:
-
Text color
Definition at line 479 of file stm32f429i_discovery_lcd.c.
References ActiveLayer, and LCD_DrawPropTypeDef::TextColor.
uint32_t BSP_LCD_GetXSize | ( | void | ) |
Gets the LCD X size.
- Return values:
-
The used LCD X size
Definition at line 238 of file stm32f429i_discovery_lcd.c.
References LcdDrv.
Referenced by BSP_LCD_Clear(), BSP_LCD_ClearStringLine(), BSP_LCD_DisplayStringAt(), BSP_LCD_DrawBitmap(), BSP_LCD_DrawHLine(), BSP_LCD_DrawPixel(), BSP_LCD_DrawVLine(), BSP_LCD_FillRect(), BSP_LCD_LayerDefaultInit(), and BSP_LCD_ReadPixel().
uint32_t BSP_LCD_GetYSize | ( | void | ) |
Gets the LCD Y size.
- Return values:
-
The used LCD Y size
Definition at line 247 of file stm32f429i_discovery_lcd.c.
References LcdDrv.
Referenced by BSP_LCD_Clear(), and BSP_LCD_LayerDefaultInit().
uint8_t BSP_LCD_Init | ( | void | ) |
Initializes the LCD.
- Return values:
-
LCD state
Definition at line 155 of file stm32f429i_discovery_lcd.c.
References BSP_LCD_MspInit(), BSP_LCD_SetFont(), BSP_SDRAM_Init(), LCD_DEFAULT_FONT, LCD_OK, LcdDrv, LtdcHandler, and PeriphClkInitStruct.
void BSP_LCD_LayerDefaultInit | ( | uint16_t | LayerIndex, |
uint32_t | FB_Address | ||
) |
Initializes the LCD layers.
- Parameters:
-
LayerIndex,: the layer foreground or background. FB_Address,: the layer frame buffer.
Definition at line 257 of file stm32f429i_discovery_lcd.c.
References LCD_DrawPropTypeDef::BackColor, BSP_LCD_GetXSize(), BSP_LCD_GetYSize(), LCD_COLOR_BLACK, LCD_COLOR_WHITE, LCD_LayerCfgTypeDef, LtdcHandler, LCD_DrawPropTypeDef::pFont, and LCD_DrawPropTypeDef::TextColor.
void BSP_LCD_MspInit | ( | void | ) |
Initializes the LTDC MSP.
Definition at line 1229 of file stm32f429i_discovery_lcd.c.
Referenced by BSP_LCD_Init().
uint32_t BSP_LCD_ReadPixel | ( | uint16_t | Xpos, |
uint16_t | Ypos | ||
) |
Reads Pixel.
- Parameters:
-
Xpos,: the X position Ypos,: the Y position
- Return values:
-
RGB pixel color
Definition at line 535 of file stm32f429i_discovery_lcd.c.
References ActiveLayer, BSP_LCD_GetXSize(), and LtdcHandler.
void BSP_LCD_Relaod | ( | uint32_t | ReloadType | ) |
Disables the color keying without reloading.
- Parameters:
-
ReloadType,: can be one of the following values - LCD_RELOAD_IMMEDIATE
- LCD_RELOAD_VERTICAL_BLANKING
- Return values:
-
None
Definition at line 470 of file stm32f429i_discovery_lcd.c.
References LtdcHandler.
void BSP_LCD_ResetColorKeying | ( | uint32_t | LayerIndex | ) |
Disables the color Keying.
- Parameters:
-
LayerIndex,: the Layer foreground or background
Definition at line 446 of file stm32f429i_discovery_lcd.c.
References LtdcHandler.
void BSP_LCD_ResetColorKeying_NoReload | ( | uint32_t | LayerIndex | ) |
Disables the color keying without reloading.
- Parameters:
-
LayerIndex,: Layer foreground or background
- Return values:
-
None
Definition at line 457 of file stm32f429i_discovery_lcd.c.
References LtdcHandler.
void BSP_LCD_SelectLayer | ( | uint32_t | LayerIndex | ) |
Selects the LCD Layer.
- Parameters:
-
LayerIndex,: the Layer foreground or background.
Definition at line 292 of file stm32f429i_discovery_lcd.c.
References ActiveLayer.
void BSP_LCD_SetBackColor | ( | uint32_t | Color | ) |
Sets the Background color.
- Parameters:
-
Color,: the layer Background color code ARGB(8-8-8-8)
Definition at line 506 of file stm32f429i_discovery_lcd.c.
References ActiveLayer, and LCD_DrawPropTypeDef::BackColor.
void BSP_LCD_SetColorKeying | ( | uint32_t | LayerIndex, |
uint32_t | RGBValue | ||
) |
Configures and sets the color Keying.
- Parameters:
-
LayerIndex,: the Layer foreground or background RGBValue,: the Color reference
Definition at line 422 of file stm32f429i_discovery_lcd.c.
References LtdcHandler.
void BSP_LCD_SetColorKeying_NoReload | ( | uint32_t | LayerIndex, |
uint32_t | RGBValue | ||
) |
Configures and sets the color keying without reloading.
- Parameters:
-
LayerIndex,: Layer foreground or background RGBValue,: Color reference
- Return values:
-
None
Definition at line 435 of file stm32f429i_discovery_lcd.c.
References LtdcHandler.
void BSP_LCD_SetFont | ( | sFONT * | pFonts | ) |
Sets the Text Font.
- Parameters:
-
pFonts,: the layer font to be used
Definition at line 515 of file stm32f429i_discovery_lcd.c.
References ActiveLayer, and LCD_DrawPropTypeDef::pFont.
Referenced by BSP_LCD_Init().
void BSP_LCD_SetLayerAddress | ( | uint32_t | LayerIndex, |
uint32_t | Address | ||
) |
Sets a LCD layer frame buffer address.
- Parameters:
-
LayerIndex,: specifies the Layer foreground or background Address,: new LCD frame buffer value
Definition at line 366 of file stm32f429i_discovery_lcd.c.
References LtdcHandler.
void BSP_LCD_SetLayerAddress_NoReload | ( | uint32_t | LayerIndex, |
uint32_t | Address | ||
) |
Sets an LCD layer frame buffer address without reloading.
- Parameters:
-
LayerIndex,: Layer foreground or background Address,: New LCD frame buffer value
- Return values:
-
None
Definition at line 377 of file stm32f429i_discovery_lcd.c.
References LtdcHandler.
void BSP_LCD_SetLayerVisible | ( | uint32_t | LayerIndex, |
FunctionalState | state | ||
) |
Sets a LCD Layer visible.
- Parameters:
-
LayerIndex,: the visible Layer. state,: new state of the specified layer. This parameter can be: ENABLE or DISABLE.
Definition at line 303 of file stm32f429i_discovery_lcd.c.
References LtdcHandler.
void BSP_LCD_SetLayerVisible_NoReload | ( | uint32_t | LayerIndex, |
FunctionalState | State | ||
) |
Sets an LCD Layer visible without reloading.
- Parameters:
-
LayerIndex,: Visible Layer State,: New state of the specified layer This parameter can be one of the following values: - ENABLE
- DISABLE
- Return values:
-
None
Definition at line 325 of file stm32f429i_discovery_lcd.c.
References LtdcHandler.
void BSP_LCD_SetLayerWindow | ( | uint16_t | LayerIndex, |
uint16_t | Xpos, | ||
uint16_t | Ypos, | ||
uint16_t | Width, | ||
uint16_t | Height | ||
) |
Sets the Display window.
- Parameters:
-
LayerIndex,: layer index Xpos,: LCD X position Ypos,: LCD Y position Width,: LCD window width Height,: LCD window height
Definition at line 390 of file stm32f429i_discovery_lcd.c.
References LtdcHandler.
void BSP_LCD_SetLayerWindow_NoReload | ( | uint16_t | LayerIndex, |
uint16_t | Xpos, | ||
uint16_t | Ypos, | ||
uint16_t | Width, | ||
uint16_t | Height | ||
) |
Sets display window without reloading.
- Parameters:
-
LayerIndex,: Layer index Xpos,: LCD X position Ypos,: LCD Y position Width,: LCD window width Height,: LCD window height
- Return values:
-
None
Definition at line 408 of file stm32f429i_discovery_lcd.c.
References LtdcHandler.
void BSP_LCD_SetTextColor | ( | uint32_t | Color | ) |
Sets the Text color.
- Parameters:
-
Color,: the Text color code ARGB(8-8-8-8)
Definition at line 497 of file stm32f429i_discovery_lcd.c.
References ActiveLayer, and LCD_DrawPropTypeDef::TextColor.
Referenced by BSP_LCD_ClearStringLine(), BSP_LCD_FillCircle(), and BSP_LCD_FillRect().
void BSP_LCD_SetTransparency | ( | uint32_t | LayerIndex, |
uint8_t | Transparency | ||
) |
Configures the Transparency.
- Parameters:
-
LayerIndex,: the Layer foreground or background. Transparency,: the Transparency, This parameter must range from 0x00 to 0xFF.
Definition at line 344 of file stm32f429i_discovery_lcd.c.
References LtdcHandler.
void BSP_LCD_SetTransparency_NoReload | ( | uint32_t | LayerIndex, |
uint8_t | Transparency | ||
) |
Configures the transparency without reloading.
- Parameters:
-
LayerIndex,: Layer foreground or background. Transparency,: Transparency This parameter must be a number between Min_Data = 0x00 and Max_Data = 0xFF
- Return values:
-
None
Definition at line 356 of file stm32f429i_discovery_lcd.c.
References LtdcHandler.
Generated on Fri Feb 17 2017 12:10:39 for STM32F429I-Discovery BSP User Manual by
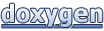