STM32F429I-Discovery BSP User Manual
|
stm32f429i_discovery_lcd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f429i_discovery_lcd.c 00004 * @author MCD Application Team 00005 * @version V2.1.5 00006 * @date 27-January-2017 00007 * @brief This file includes the LCD driver for ILI9341 Liquid Crystal 00008 * Display Modules of STM32F429I-Discovery kit (MB1075). 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive directly an LCD TFT using LTDC controller. 00044 - This driver select dynamically the mounted LCD, ILI9341 240x320 LCD mounted 00045 on MB1075B discovery board, and use the adequate timing and setting for 00046 the specified LCD using device ID of the ILI9341 mounted on MB1075B discovery board 00047 00048 2. Driver description: 00049 --------------------- 00050 + Initialization steps : 00051 o Initialize the LCD using the LCD_Init() function. 00052 o Apply the Layer configuration using LCD_LayerDefaultInit() function 00053 o Select the LCD layer to be used using LCD_SelectLayer() function. 00054 o Enable the LCD display using LCD_DisplayOn() function. 00055 00056 + Options 00057 o Configure and enable the color keying functionality using LCD_SetColorKeying() 00058 function. 00059 o Modify in the fly the transparency and/or the frame buffer address 00060 using the following functions : 00061 - LCD_SetTransparency() 00062 - LCD_SetLayerAddress() 00063 00064 + Display on LCD 00065 o Clear the hole LCD using LCD_Clear() function or only one specified string 00066 line using LCD_ClearStringLine() function. 00067 o Display a character on the specified line and column using LCD_DisplayChar() 00068 function or a complete string line using LCD_DisplayStringAtLine() function. 00069 o Display a string line on the specified position (x,y in pixel) and align mode 00070 using LCD_DisplayStringAtLine() function. 00071 o Draw and fill a basic shapes (dot, line, rectangle, circle, ellipse, .. bitmap) 00072 on LCD using the available set of functions 00073 00074 ------------------------------------------------------------------------------*/ 00075 00076 /* Includes ------------------------------------------------------------------*/ 00077 #include "stm32f429i_discovery_lcd.h" 00078 #include "../../../Utilities/Fonts/fonts.h" 00079 #include "../../../Utilities/Fonts/font24.c" 00080 #include "../../../Utilities/Fonts/font20.c" 00081 #include "../../../Utilities/Fonts/font16.c" 00082 #include "../../../Utilities/Fonts/font12.c" 00083 #include "../../../Utilities/Fonts/font8.c" 00084 00085 /** @addtogroup BSP 00086 * @{ 00087 */ 00088 00089 /** @addtogroup STM32F429I_DISCOVERY 00090 * @{ 00091 */ 00092 00093 /** @defgroup STM32F429I_DISCOVERY_LCD STM32F429I DISCOVERY LCD 00094 * @brief This file includes the LCD driver for (ILI9341) 00095 * @{ 00096 */ 00097 00098 /** @defgroup STM32F429I_DISCOVERY_LCD_Private_TypesDefinitions STM32F429I DISCOVERY LCD Private TypesDefinitions 00099 * @{ 00100 */ 00101 /** 00102 * @} 00103 */ 00104 00105 /** @defgroup STM32F429I_DISCOVERY_LCD_Private_Defines STM32F429I DISCOVERY LCD Private Defines 00106 * @{ 00107 */ 00108 #define POLY_X(Z) ((int32_t)((Points + Z)->X)) 00109 #define POLY_Y(Z) ((int32_t)((Points + Z)->Y)) 00110 /** 00111 * @} 00112 */ 00113 00114 /** @defgroup STM32F429I_DISCOVERY_LCD_Private_Macros STM32F429I DISCOVERY LCD Private Macros 00115 * @{ 00116 */ 00117 #define ABS(X) ((X) > 0 ? (X) : -(X)) 00118 /** 00119 * @} 00120 */ 00121 00122 /** @defgroup STM32F429I_DISCOVERY_LCD_Private_Variables STM32F429I DISCOVERY LCD Private Variables 00123 * @{ 00124 */ 00125 LTDC_HandleTypeDef LtdcHandler; 00126 static DMA2D_HandleTypeDef Dma2dHandler; 00127 static RCC_PeriphCLKInitTypeDef PeriphClkInitStruct; 00128 00129 /* Default LCD configuration with LCD Layer 1 */ 00130 static uint32_t ActiveLayer = 0; 00131 static LCD_DrawPropTypeDef DrawProp[MAX_LAYER_NUMBER]; 00132 LCD_DrvTypeDef *LcdDrv; 00133 /** 00134 * @} 00135 */ 00136 00137 /** @defgroup STM32F429I_DISCOVERY_LCD_Private_FunctionPrototypes STM32F429I DISCOVERY LCD Private FunctionPrototypes 00138 * @{ 00139 */ 00140 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c); 00141 static void FillBuffer(uint32_t LayerIndex, void *pDst, uint32_t xSize, uint32_t ySize, uint32_t OffLine, uint32_t ColorIndex); 00142 static void ConvertLineToARGB8888(void *pSrc, void *pDst, uint32_t xSize, uint32_t ColorMode); 00143 /** 00144 * @} 00145 */ 00146 00147 /** @defgroup STM32F429I_DISCOVERY_LCD_Private_Functions STM32F429I DISCOVERY LCD Private Functions 00148 * @{ 00149 */ 00150 00151 /** 00152 * @brief Initializes the LCD. 00153 * @retval LCD state 00154 */ 00155 uint8_t BSP_LCD_Init(void) 00156 { 00157 /* On STM32F429I-DISCO, it is not possible to read ILI9341 ID because */ 00158 /* PIN EXTC is not connected to VDD and then LCD_READ_ID4 is not accessible. */ 00159 /* In this case, ReadID function is bypassed.*/ 00160 /*if(ili9341_drv.ReadID() == ILI9341_ID)*/ 00161 00162 /* LTDC Configuration ----------------------------------------------------*/ 00163 LtdcHandler.Instance = LTDC; 00164 00165 /* Timing configuration (Typical configuration from ILI9341 datasheet) 00166 HSYNC=10 (9+1) 00167 HBP=20 (29-10+1) 00168 ActiveW=240 (269-20-10+1) 00169 HFP=10 (279-240-20-10+1) 00170 00171 VSYNC=2 (1+1) 00172 VBP=2 (3-2+1) 00173 ActiveH=320 (323-2-2+1) 00174 VFP=4 (327-320-2-2+1) 00175 */ 00176 00177 /* Configure horizontal synchronization width */ 00178 LtdcHandler.Init.HorizontalSync = ILI9341_HSYNC; 00179 /* Configure vertical synchronization height */ 00180 LtdcHandler.Init.VerticalSync = ILI9341_VSYNC; 00181 /* Configure accumulated horizontal back porch */ 00182 LtdcHandler.Init.AccumulatedHBP = ILI9341_HBP; 00183 /* Configure accumulated vertical back porch */ 00184 LtdcHandler.Init.AccumulatedVBP = ILI9341_VBP; 00185 /* Configure accumulated active width */ 00186 LtdcHandler.Init.AccumulatedActiveW = 269; 00187 /* Configure accumulated active height */ 00188 LtdcHandler.Init.AccumulatedActiveH = 323; 00189 /* Configure total width */ 00190 LtdcHandler.Init.TotalWidth = 279; 00191 /* Configure total height */ 00192 LtdcHandler.Init.TotalHeigh = 327; 00193 00194 /* Configure R,G,B component values for LCD background color */ 00195 LtdcHandler.Init.Backcolor.Red= 0; 00196 LtdcHandler.Init.Backcolor.Blue= 0; 00197 LtdcHandler.Init.Backcolor.Green= 0; 00198 00199 /* LCD clock configuration */ 00200 /* PLLSAI_VCO Input = HSE_VALUE/PLL_M = 1 Mhz */ 00201 /* PLLSAI_VCO Output = PLLSAI_VCO Input * PLLSAIN = 192 Mhz */ 00202 /* PLLLCDCLK = PLLSAI_VCO Output/PLLSAIR = 192/4 = 48 Mhz */ 00203 /* LTDC clock frequency = PLLLCDCLK / LTDC_PLLSAI_DIVR_8 = 48/4 = 6Mhz */ 00204 PeriphClkInitStruct.PeriphClockSelection = RCC_PERIPHCLK_LTDC; 00205 PeriphClkInitStruct.PLLSAI.PLLSAIN = 192; 00206 PeriphClkInitStruct.PLLSAI.PLLSAIR = 4; 00207 PeriphClkInitStruct.PLLSAIDivR = RCC_PLLSAIDIVR_8; 00208 HAL_RCCEx_PeriphCLKConfig(&PeriphClkInitStruct); 00209 00210 /* Polarity */ 00211 LtdcHandler.Init.HSPolarity = LTDC_HSPOLARITY_AL; 00212 LtdcHandler.Init.VSPolarity = LTDC_VSPOLARITY_AL; 00213 LtdcHandler.Init.DEPolarity = LTDC_DEPOLARITY_AL; 00214 LtdcHandler.Init.PCPolarity = LTDC_PCPOLARITY_IPC; 00215 00216 BSP_LCD_MspInit(); 00217 HAL_LTDC_Init(&LtdcHandler); 00218 00219 /* Select the device */ 00220 LcdDrv = &ili9341_drv; 00221 00222 /* LCD Init */ 00223 LcdDrv->Init(); 00224 00225 /* Initialize the SDRAM */ 00226 BSP_SDRAM_Init(); 00227 00228 /* Initialize the font */ 00229 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00230 00231 return LCD_OK; 00232 } 00233 00234 /** 00235 * @brief Gets the LCD X size. 00236 * @retval The used LCD X size 00237 */ 00238 uint32_t BSP_LCD_GetXSize(void) 00239 { 00240 return LcdDrv->GetLcdPixelWidth(); 00241 } 00242 00243 /** 00244 * @brief Gets the LCD Y size. 00245 * @retval The used LCD Y size 00246 */ 00247 uint32_t BSP_LCD_GetYSize(void) 00248 { 00249 return LcdDrv->GetLcdPixelHeight(); 00250 } 00251 00252 /** 00253 * @brief Initializes the LCD layers. 00254 * @param LayerIndex: the layer foreground or background. 00255 * @param FB_Address: the layer frame buffer. 00256 */ 00257 void BSP_LCD_LayerDefaultInit(uint16_t LayerIndex, uint32_t FB_Address) 00258 { 00259 LCD_LayerCfgTypeDef Layercfg; 00260 00261 /* Layer Init */ 00262 Layercfg.WindowX0 = 0; 00263 Layercfg.WindowX1 = BSP_LCD_GetXSize(); 00264 Layercfg.WindowY0 = 0; 00265 Layercfg.WindowY1 = BSP_LCD_GetYSize(); 00266 Layercfg.PixelFormat = LTDC_PIXEL_FORMAT_ARGB8888; 00267 Layercfg.FBStartAdress = FB_Address; 00268 Layercfg.Alpha = 255; 00269 Layercfg.Alpha0 = 0; 00270 Layercfg.Backcolor.Blue = 0; 00271 Layercfg.Backcolor.Green = 0; 00272 Layercfg.Backcolor.Red = 0; 00273 Layercfg.BlendingFactor1 = LTDC_BLENDING_FACTOR1_PAxCA; 00274 Layercfg.BlendingFactor2 = LTDC_BLENDING_FACTOR2_PAxCA; 00275 Layercfg.ImageWidth = BSP_LCD_GetXSize(); 00276 Layercfg.ImageHeight = BSP_LCD_GetYSize(); 00277 00278 HAL_LTDC_ConfigLayer(&LtdcHandler, &Layercfg, LayerIndex); 00279 00280 DrawProp[LayerIndex].BackColor = LCD_COLOR_WHITE; 00281 DrawProp[LayerIndex].pFont = &Font24; 00282 DrawProp[LayerIndex].TextColor = LCD_COLOR_BLACK; 00283 00284 /* Dithering activation */ 00285 HAL_LTDC_EnableDither(&LtdcHandler); 00286 } 00287 00288 /** 00289 * @brief Selects the LCD Layer. 00290 * @param LayerIndex: the Layer foreground or background. 00291 */ 00292 void BSP_LCD_SelectLayer(uint32_t LayerIndex) 00293 { 00294 ActiveLayer = LayerIndex; 00295 } 00296 00297 /** 00298 * @brief Sets a LCD Layer visible. 00299 * @param LayerIndex: the visible Layer. 00300 * @param state: new state of the specified layer. 00301 * This parameter can be: ENABLE or DISABLE. 00302 */ 00303 void BSP_LCD_SetLayerVisible(uint32_t LayerIndex, FunctionalState state) 00304 { 00305 if(state == ENABLE) 00306 { 00307 __HAL_LTDC_LAYER_ENABLE(&LtdcHandler, LayerIndex); 00308 } 00309 else 00310 { 00311 __HAL_LTDC_LAYER_DISABLE(&LtdcHandler, LayerIndex); 00312 } 00313 __HAL_LTDC_RELOAD_CONFIG(&LtdcHandler); 00314 } 00315 00316 /** 00317 * @brief Sets an LCD Layer visible without reloading. 00318 * @param LayerIndex: Visible Layer 00319 * @param State: New state of the specified layer 00320 * This parameter can be one of the following values: 00321 * @arg ENABLE 00322 * @arg DISABLE 00323 * @retval None 00324 */ 00325 void BSP_LCD_SetLayerVisible_NoReload(uint32_t LayerIndex, FunctionalState State) 00326 { 00327 if(State == ENABLE) 00328 { 00329 __HAL_LTDC_LAYER_ENABLE(&LtdcHandler, LayerIndex); 00330 } 00331 else 00332 { 00333 __HAL_LTDC_LAYER_DISABLE(&LtdcHandler, LayerIndex); 00334 } 00335 /* Do not Sets the Reload */ 00336 } 00337 00338 /** 00339 * @brief Configures the Transparency. 00340 * @param LayerIndex: the Layer foreground or background. 00341 * @param Transparency: the Transparency, 00342 * This parameter must range from 0x00 to 0xFF. 00343 */ 00344 void BSP_LCD_SetTransparency(uint32_t LayerIndex, uint8_t Transparency) 00345 { 00346 HAL_LTDC_SetAlpha(&LtdcHandler, Transparency, LayerIndex); 00347 } 00348 00349 /** 00350 * @brief Configures the transparency without reloading. 00351 * @param LayerIndex: Layer foreground or background. 00352 * @param Transparency: Transparency 00353 * This parameter must be a number between Min_Data = 0x00 and Max_Data = 0xFF 00354 * @retval None 00355 */ 00356 void BSP_LCD_SetTransparency_NoReload(uint32_t LayerIndex, uint8_t Transparency) 00357 { 00358 HAL_LTDC_SetAlpha_NoReload(&LtdcHandler, Transparency, LayerIndex); 00359 } 00360 00361 /** 00362 * @brief Sets a LCD layer frame buffer address. 00363 * @param LayerIndex: specifies the Layer foreground or background 00364 * @param Address: new LCD frame buffer value 00365 */ 00366 void BSP_LCD_SetLayerAddress(uint32_t LayerIndex, uint32_t Address) 00367 { 00368 HAL_LTDC_SetAddress(&LtdcHandler, Address, LayerIndex); 00369 } 00370 00371 /** 00372 * @brief Sets an LCD layer frame buffer address without reloading. 00373 * @param LayerIndex: Layer foreground or background 00374 * @param Address: New LCD frame buffer value 00375 * @retval None 00376 */ 00377 void BSP_LCD_SetLayerAddress_NoReload(uint32_t LayerIndex, uint32_t Address) 00378 { 00379 HAL_LTDC_SetAddress_NoReload(&LtdcHandler, Address, LayerIndex); 00380 } 00381 00382 /** 00383 * @brief Sets the Display window. 00384 * @param LayerIndex: layer index 00385 * @param Xpos: LCD X position 00386 * @param Ypos: LCD Y position 00387 * @param Width: LCD window width 00388 * @param Height: LCD window height 00389 */ 00390 void BSP_LCD_SetLayerWindow(uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00391 { 00392 /* reconfigure the layer size */ 00393 HAL_LTDC_SetWindowSize(&LtdcHandler, Width, Height, LayerIndex); 00394 00395 /* reconfigure the layer position */ 00396 HAL_LTDC_SetWindowPosition(&LtdcHandler, Xpos, Ypos, LayerIndex); 00397 } 00398 00399 /** 00400 * @brief Sets display window without reloading. 00401 * @param LayerIndex: Layer index 00402 * @param Xpos: LCD X position 00403 * @param Ypos: LCD Y position 00404 * @param Width: LCD window width 00405 * @param Height: LCD window height 00406 * @retval None 00407 */ 00408 void BSP_LCD_SetLayerWindow_NoReload(uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00409 { 00410 /* Reconfigure the layer size */ 00411 HAL_LTDC_SetWindowSize_NoReload(&LtdcHandler, Width, Height, LayerIndex); 00412 00413 /* Reconfigure the layer position */ 00414 HAL_LTDC_SetWindowPosition_NoReload(&LtdcHandler, Xpos, Ypos, LayerIndex); 00415 } 00416 00417 /** 00418 * @brief Configures and sets the color Keying. 00419 * @param LayerIndex: the Layer foreground or background 00420 * @param RGBValue: the Color reference 00421 */ 00422 void BSP_LCD_SetColorKeying(uint32_t LayerIndex, uint32_t RGBValue) 00423 { 00424 /* Configure and Enable the color Keying for LCD Layer */ 00425 HAL_LTDC_ConfigColorKeying(&LtdcHandler, RGBValue, LayerIndex); 00426 HAL_LTDC_EnableColorKeying(&LtdcHandler, LayerIndex); 00427 } 00428 00429 /** 00430 * @brief Configures and sets the color keying without reloading. 00431 * @param LayerIndex: Layer foreground or background 00432 * @param RGBValue: Color reference 00433 * @retval None 00434 */ 00435 void BSP_LCD_SetColorKeying_NoReload(uint32_t LayerIndex, uint32_t RGBValue) 00436 { 00437 /* Configure and Enable the color Keying for LCD Layer */ 00438 HAL_LTDC_ConfigColorKeying_NoReload(&LtdcHandler, RGBValue, LayerIndex); 00439 HAL_LTDC_EnableColorKeying_NoReload(&LtdcHandler, LayerIndex); 00440 } 00441 00442 /** 00443 * @brief Disables the color Keying. 00444 * @param LayerIndex: the Layer foreground or background 00445 */ 00446 void BSP_LCD_ResetColorKeying(uint32_t LayerIndex) 00447 { 00448 /* Disable the color Keying for LCD Layer */ 00449 HAL_LTDC_DisableColorKeying(&LtdcHandler, LayerIndex); 00450 } 00451 00452 /** 00453 * @brief Disables the color keying without reloading. 00454 * @param LayerIndex: Layer foreground or background 00455 * @retval None 00456 */ 00457 void BSP_LCD_ResetColorKeying_NoReload(uint32_t LayerIndex) 00458 { 00459 /* Disable the color Keying for LCD Layer */ 00460 HAL_LTDC_DisableColorKeying_NoReload(&LtdcHandler, LayerIndex); 00461 } 00462 00463 /** 00464 * @brief Disables the color keying without reloading. 00465 * @param ReloadType: can be one of the following values 00466 * - LCD_RELOAD_IMMEDIATE 00467 * - LCD_RELOAD_VERTICAL_BLANKING 00468 * @retval None 00469 */ 00470 void BSP_LCD_Relaod(uint32_t ReloadType) 00471 { 00472 HAL_LTDC_Relaod (&LtdcHandler, ReloadType); 00473 } 00474 00475 /** 00476 * @brief Gets the LCD Text color. 00477 * @retval Text color 00478 */ 00479 uint32_t BSP_LCD_GetTextColor(void) 00480 { 00481 return DrawProp[ActiveLayer].TextColor; 00482 } 00483 00484 /** 00485 * @brief Gets the LCD Background color. 00486 * @retval Background color 00487 */ 00488 uint32_t BSP_LCD_GetBackColor(void) 00489 { 00490 return DrawProp[ActiveLayer].BackColor; 00491 } 00492 00493 /** 00494 * @brief Sets the Text color. 00495 * @param Color: the Text color code ARGB(8-8-8-8) 00496 */ 00497 void BSP_LCD_SetTextColor(uint32_t Color) 00498 { 00499 DrawProp[ActiveLayer].TextColor = Color; 00500 } 00501 00502 /** 00503 * @brief Sets the Background color. 00504 * @param Color: the layer Background color code ARGB(8-8-8-8) 00505 */ 00506 void BSP_LCD_SetBackColor(uint32_t Color) 00507 { 00508 DrawProp[ActiveLayer].BackColor = Color; 00509 } 00510 00511 /** 00512 * @brief Sets the Text Font. 00513 * @param pFonts: the layer font to be used 00514 */ 00515 void BSP_LCD_SetFont(sFONT *pFonts) 00516 { 00517 DrawProp[ActiveLayer].pFont = pFonts; 00518 } 00519 00520 /** 00521 * @brief Gets the Text Font. 00522 * @retval Layer font 00523 */ 00524 sFONT *BSP_LCD_GetFont(void) 00525 { 00526 return DrawProp[ActiveLayer].pFont; 00527 } 00528 00529 /** 00530 * @brief Reads Pixel. 00531 * @param Xpos: the X position 00532 * @param Ypos: the Y position 00533 * @retval RGB pixel color 00534 */ 00535 uint32_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos) 00536 { 00537 uint32_t ret = 0; 00538 00539 if(LtdcHandler.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_ARGB8888) 00540 { 00541 /* Read data value from SDRAM memory */ 00542 ret = *(__IO uint32_t*) (LtdcHandler.LayerCfg[ActiveLayer].FBStartAdress + (4*(Ypos*BSP_LCD_GetXSize() + Xpos))); 00543 } 00544 else if(LtdcHandler.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB888) 00545 { 00546 /* Read data value from SDRAM memory */ 00547 ret = (*(__IO uint32_t*) (LtdcHandler.LayerCfg[ActiveLayer].FBStartAdress + (4*(Ypos*BSP_LCD_GetXSize() + Xpos))) & 0x00FFFFFF); 00548 } 00549 else if((LtdcHandler.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB565) || \ 00550 (LtdcHandler.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_ARGB4444) || \ 00551 (LtdcHandler.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_AL88)) 00552 { 00553 /* Read data value from SDRAM memory */ 00554 ret = *(__IO uint16_t*) (LtdcHandler.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_LCD_GetXSize() + Xpos))); 00555 } 00556 else 00557 { 00558 /* Read data value from SDRAM memory */ 00559 ret = *(__IO uint8_t*) (LtdcHandler.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_LCD_GetXSize() + Xpos))); 00560 } 00561 00562 return ret; 00563 } 00564 00565 /** 00566 * @brief Clears the hole LCD. 00567 * @param Color: the color of the background 00568 */ 00569 void BSP_LCD_Clear(uint32_t Color) 00570 { 00571 /* Clear the LCD */ 00572 FillBuffer(ActiveLayer, (uint32_t *)(LtdcHandler.LayerCfg[ActiveLayer].FBStartAdress), BSP_LCD_GetXSize(), BSP_LCD_GetYSize(), 0, Color); 00573 } 00574 00575 /** 00576 * @brief Clears the selected line. 00577 * @param Line: the line to be cleared 00578 */ 00579 void BSP_LCD_ClearStringLine(uint32_t Line) 00580 { 00581 uint32_t colorbackup = DrawProp[ActiveLayer].TextColor; 00582 DrawProp[ActiveLayer].TextColor = DrawProp[ActiveLayer].BackColor; 00583 00584 /* Draw rectangle with background color */ 00585 BSP_LCD_FillRect(0, (Line * DrawProp[ActiveLayer].pFont->Height), BSP_LCD_GetXSize(), DrawProp[ActiveLayer].pFont->Height); 00586 00587 DrawProp[ActiveLayer].TextColor = colorbackup; 00588 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 00589 } 00590 00591 /** 00592 * @brief Displays one character. 00593 * @param Xpos: start column address 00594 * @param Ypos: the Line where to display the character shape 00595 * @param Ascii: character ascii code, must be between 0x20 and 0x7E 00596 */ 00597 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) 00598 { 00599 DrawChar(Xpos, Ypos, &DrawProp[ActiveLayer].pFont->table[(Ascii-' ') *\ 00600 DrawProp[ActiveLayer].pFont->Height * ((DrawProp[ActiveLayer].pFont->Width + 7) / 8)]); 00601 } 00602 00603 /** 00604 * @brief Displays a maximum of 60 char on the LCD. 00605 * @param X: pointer to x position (in pixel) 00606 * @param Y: pointer to y position (in pixel) 00607 * @param pText: pointer to string to display on LCD 00608 * @param mode: The display mode 00609 * This parameter can be one of the following values: 00610 * @arg CENTER_MODE 00611 * @arg RIGHT_MODE 00612 * @arg LEFT_MODE 00613 */ 00614 void BSP_LCD_DisplayStringAt(uint16_t X, uint16_t Y, uint8_t *pText, Text_AlignModeTypdef mode) 00615 { 00616 uint16_t refcolumn = 1, i = 0; 00617 uint32_t size = 0, xsize = 0; 00618 uint8_t *ptr = pText; 00619 00620 /* Get the text size */ 00621 while (*ptr++) size ++ ; 00622 00623 /* Characters number per line */ 00624 xsize = (BSP_LCD_GetXSize()/DrawProp[ActiveLayer].pFont->Width); 00625 00626 switch (mode) 00627 { 00628 case CENTER_MODE: 00629 { 00630 refcolumn = X+ ((xsize - size)* DrawProp[ActiveLayer].pFont->Width) / 2; 00631 break; 00632 } 00633 case LEFT_MODE: 00634 { 00635 refcolumn = X; 00636 break; 00637 } 00638 case RIGHT_MODE: 00639 { 00640 refcolumn = X + ((xsize - size)*DrawProp[ActiveLayer].pFont->Width); 00641 break; 00642 } 00643 default: 00644 { 00645 refcolumn = X; 00646 break; 00647 } 00648 } 00649 00650 /* Send the string character by character on LCD */ 00651 while ((*pText != 0) & (((BSP_LCD_GetXSize() - (i*DrawProp[ActiveLayer].pFont->Width)) & 0xFFFF) >= DrawProp[ActiveLayer].pFont->Width)) 00652 { 00653 /* Display one character on LCD */ 00654 BSP_LCD_DisplayChar(refcolumn, Y, *pText); 00655 /* Decrement the column position by 16 */ 00656 refcolumn += DrawProp[ActiveLayer].pFont->Width; 00657 /* Point on the next character */ 00658 pText++; 00659 i++; 00660 } 00661 } 00662 00663 /** 00664 * @brief Displays a maximum of 20 char on the LCD. 00665 * @param Line: the Line where to display the character shape 00666 * @param ptr: pointer to string to display on LCD 00667 */ 00668 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *ptr) 00669 { 00670 BSP_LCD_DisplayStringAt(0, LINE(Line), ptr, LEFT_MODE); 00671 } 00672 00673 /** 00674 * @brief Displays an horizontal line. 00675 * @param Xpos: the X position 00676 * @param Ypos: the Y position 00677 * @param Length: line length 00678 */ 00679 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00680 { 00681 uint32_t xaddress = 0; 00682 00683 /* Get the line address */ 00684 xaddress = (LtdcHandler.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_LCD_GetXSize()*Ypos + Xpos); 00685 00686 /* Write line */ 00687 FillBuffer(ActiveLayer, (uint32_t *)xaddress, Length, 1, 0, DrawProp[ActiveLayer].TextColor); 00688 } 00689 00690 /** 00691 * @brief Displays a vertical line. 00692 * @param Xpos: the X position 00693 * @param Ypos: the Y position 00694 * @param Length: line length 00695 */ 00696 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00697 { 00698 uint32_t xaddress = 0; 00699 00700 /* Get the line address */ 00701 xaddress = (LtdcHandler.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_LCD_GetXSize()*Ypos + Xpos); 00702 00703 /* Write line */ 00704 FillBuffer(ActiveLayer, (uint32_t *)xaddress, 1, Length, (BSP_LCD_GetXSize() - 1), DrawProp[ActiveLayer].TextColor); 00705 } 00706 00707 /** 00708 * @brief Displays an uni-line (between two points). 00709 * @param X1: the point 1 X position 00710 * @param Y1: the point 1 Y position 00711 * @param X2: the point 2 X position 00712 * @param Y2: the point 2 Y position 00713 */ 00714 void BSP_LCD_DrawLine(uint16_t X1, uint16_t Y1, uint16_t X2, uint16_t Y2) 00715 { 00716 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00717 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 00718 curpixel = 0; 00719 00720 deltax = ABS(X2 - X1); /* The difference between the x's */ 00721 deltay = ABS(Y2 - Y1); /* The difference between the y's */ 00722 x = X1; /* Start x off at the first pixel */ 00723 y = Y1; /* Start y off at the first pixel */ 00724 00725 if (X2 >= X1) /* The x-values are increasing */ 00726 { 00727 xinc1 = 1; 00728 xinc2 = 1; 00729 } 00730 else /* The x-values are decreasing */ 00731 { 00732 xinc1 = -1; 00733 xinc2 = -1; 00734 } 00735 00736 if (Y2 >= Y1) /* The y-values are increasing */ 00737 { 00738 yinc1 = 1; 00739 yinc2 = 1; 00740 } 00741 else /* The y-values are decreasing */ 00742 { 00743 yinc1 = -1; 00744 yinc2 = -1; 00745 } 00746 00747 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00748 { 00749 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00750 yinc2 = 0; /* Don't change the y for every iteration */ 00751 den = deltax; 00752 num = deltax / 2; 00753 numadd = deltay; 00754 numpixels = deltax; /* There are more x-values than y-values */ 00755 } 00756 else /* There is at least one y-value for every x-value */ 00757 { 00758 xinc2 = 0; /* Don't change the x for every iteration */ 00759 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00760 den = deltay; 00761 num = deltay / 2; 00762 numadd = deltax; 00763 numpixels = deltay; /* There are more y-values than x-values */ 00764 } 00765 00766 for (curpixel = 0; curpixel <= numpixels; curpixel++) 00767 { 00768 BSP_LCD_DrawPixel(x, y, DrawProp[ActiveLayer].TextColor); /* Draw the current pixel */ 00769 num += numadd; /* Increase the numerator by the top of the fraction */ 00770 if (num >= den) /* Check if numerator >= denominator */ 00771 { 00772 num -= den; /* Calculate the new numerator value */ 00773 x += xinc1; /* Change the x as appropriate */ 00774 y += yinc1; /* Change the y as appropriate */ 00775 } 00776 x += xinc2; /* Change the x as appropriate */ 00777 y += yinc2; /* Change the y as appropriate */ 00778 } 00779 } 00780 00781 /** 00782 * @brief Displays a rectangle. 00783 * @param Xpos: the X position 00784 * @param Ypos: the Y position 00785 * @param Height: display rectangle height 00786 * @param Width: display rectangle width 00787 */ 00788 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00789 { 00790 /* Draw horizontal lines */ 00791 BSP_LCD_DrawHLine(Xpos, Ypos, Width); 00792 BSP_LCD_DrawHLine(Xpos, (Ypos+ Height), Width); 00793 00794 /* Draw vertical lines */ 00795 BSP_LCD_DrawVLine(Xpos, Ypos, Height); 00796 BSP_LCD_DrawVLine((Xpos + Width), Ypos, Height); 00797 } 00798 00799 /** 00800 * @brief Displays a circle. 00801 * @param Xpos: the X position 00802 * @param Ypos: the Y position 00803 * @param Radius: the circle radius 00804 */ 00805 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00806 { 00807 int32_t d;/* Decision Variable */ 00808 uint32_t curx;/* Current X Value */ 00809 uint32_t cury;/* Current Y Value */ 00810 00811 d = 3 - (Radius << 1); 00812 curx = 0; 00813 cury = Radius; 00814 00815 while (curx <= cury) 00816 { 00817 BSP_LCD_DrawPixel((Xpos + curx), (Ypos - cury), DrawProp[ActiveLayer].TextColor); 00818 BSP_LCD_DrawPixel((Xpos - curx), (Ypos - cury), DrawProp[ActiveLayer].TextColor); 00819 BSP_LCD_DrawPixel((Xpos + cury), (Ypos - curx), DrawProp[ActiveLayer].TextColor); 00820 BSP_LCD_DrawPixel((Xpos - cury), (Ypos - curx), DrawProp[ActiveLayer].TextColor); 00821 BSP_LCD_DrawPixel((Xpos + curx), (Ypos + cury), DrawProp[ActiveLayer].TextColor); 00822 BSP_LCD_DrawPixel((Xpos - curx), (Ypos + cury), DrawProp[ActiveLayer].TextColor); 00823 BSP_LCD_DrawPixel((Xpos + cury), (Ypos + curx), DrawProp[ActiveLayer].TextColor); 00824 BSP_LCD_DrawPixel((Xpos - cury), (Ypos + curx), DrawProp[ActiveLayer].TextColor); 00825 00826 if (d < 0) 00827 { 00828 d += (curx << 2) + 6; 00829 } 00830 else 00831 { 00832 d += ((curx - cury) << 2) + 10; 00833 cury--; 00834 } 00835 curx++; 00836 } 00837 } 00838 00839 /** 00840 * @brief Displays an poly-line (between many points). 00841 * @param Points: pointer to the points array 00842 * @param PointCount: Number of points 00843 */ 00844 void BSP_LCD_DrawPolygon(pPoint Points, uint16_t PointCount) 00845 { 00846 int16_t x = 0, y = 0; 00847 00848 if(PointCount < 2) 00849 { 00850 return; 00851 } 00852 00853 BSP_LCD_DrawLine(Points->X, Points->Y, (Points+PointCount-1)->X, (Points+PointCount-1)->Y); 00854 00855 while(--PointCount) 00856 { 00857 x = Points->X; 00858 y = Points->Y; 00859 Points++; 00860 BSP_LCD_DrawLine(x, y, Points->X, Points->Y); 00861 } 00862 } 00863 00864 /** 00865 * @brief Displays an Ellipse. 00866 * @param Xpos: the X position 00867 * @param Ypos: the Y position 00868 * @param XRadius: the X radius of ellipse 00869 * @param YRadius: the Y radius of ellipse 00870 */ 00871 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00872 { 00873 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 00874 float k = 0, rad1 = 0, rad2 = 0; 00875 00876 rad1 = XRadius; 00877 rad2 = YRadius; 00878 00879 k = (float)(rad2/rad1); 00880 00881 do { 00882 BSP_LCD_DrawPixel((Xpos-(uint16_t)(x/k)), (Ypos+y), DrawProp[ActiveLayer].TextColor); 00883 BSP_LCD_DrawPixel((Xpos+(uint16_t)(x/k)), (Ypos+y), DrawProp[ActiveLayer].TextColor); 00884 BSP_LCD_DrawPixel((Xpos+(uint16_t)(x/k)), (Ypos-y), DrawProp[ActiveLayer].TextColor); 00885 BSP_LCD_DrawPixel((Xpos-(uint16_t)(x/k)), (Ypos-y), DrawProp[ActiveLayer].TextColor); 00886 00887 e2 = err; 00888 if (e2 <= x) { 00889 err += ++x*2+1; 00890 if (-y == x && e2 <= y) e2 = 0; 00891 } 00892 if (e2 > y) err += ++y*2+1; 00893 } 00894 while (y <= 0); 00895 } 00896 00897 /** 00898 * @brief Displays a bitmap picture loaded in the internal Flash (32 bpp). 00899 * @param X: the bmp x position in the LCD 00900 * @param Y: the bmp Y position in the LCD 00901 * @param pBmp: Bmp picture address in the internal Flash 00902 */ 00903 void BSP_LCD_DrawBitmap(uint32_t X, uint32_t Y, uint8_t *pBmp) 00904 { 00905 uint32_t index = 0, width = 0, height = 0, bitpixel = 0; 00906 uint32_t address; 00907 uint32_t inputcolormode = 0; 00908 00909 /* Get bitmap data address offset */ 00910 index = *(__IO uint16_t *) (pBmp + 10); 00911 index |= (*(__IO uint16_t *) (pBmp + 12)) << 16; 00912 00913 /* Read bitmap width */ 00914 width = *(uint16_t *) (pBmp + 18); 00915 width |= (*(uint16_t *) (pBmp + 20)) << 16; 00916 00917 /* Read bitmap height */ 00918 height = *(uint16_t *) (pBmp + 22); 00919 height |= (*(uint16_t *) (pBmp + 24)) << 16; 00920 00921 /* Read bit/pixel */ 00922 bitpixel = *(uint16_t *) (pBmp + 28); 00923 00924 /* Set Address */ 00925 address = LtdcHandler.LayerCfg[ActiveLayer].FBStartAdress + (((BSP_LCD_GetXSize()*Y) + X)*(4)); 00926 00927 /* Get the Layer pixel format */ 00928 if ((bitpixel/8) == 4) 00929 { 00930 inputcolormode = CM_ARGB8888; 00931 } 00932 else if ((bitpixel/8) == 2) 00933 { 00934 inputcolormode = CM_RGB565; 00935 } 00936 else 00937 { 00938 inputcolormode = CM_RGB888; 00939 } 00940 00941 /* bypass the bitmap header */ 00942 pBmp += (index + (width * (height - 1) * (bitpixel/8))); 00943 00944 /* Convert picture to ARGB8888 pixel format */ 00945 for(index=0; index < height; index++) 00946 { 00947 /* Pixel format conversion */ 00948 ConvertLineToARGB8888((uint32_t *)pBmp, (uint32_t *)address, width, inputcolormode); 00949 00950 /* Increment the source and destination buffers */ 00951 address+= ((BSP_LCD_GetXSize() - width + width)*4); 00952 pBmp -= width*(bitpixel/8); 00953 } 00954 } 00955 00956 /** 00957 * @brief Displays a full rectangle. 00958 * @param Xpos: the X position 00959 * @param Ypos: the Y position 00960 * @param Height: rectangle height 00961 * @param Width: rectangle width 00962 */ 00963 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00964 { 00965 uint32_t xaddress = 0; 00966 00967 /* Set the text color */ 00968 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 00969 00970 /* Get the rectangle start address */ 00971 xaddress = (LtdcHandler.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_LCD_GetXSize()*Ypos + Xpos); 00972 00973 /* Fill the rectangle */ 00974 FillBuffer(ActiveLayer, (uint32_t *)xaddress, Width, Height, (BSP_LCD_GetXSize() - Width), DrawProp[ActiveLayer].TextColor); 00975 } 00976 00977 /** 00978 * @brief Displays a full circle. 00979 * @param Xpos: the X position 00980 * @param Ypos: the Y position 00981 * @param Radius: the circle radius 00982 */ 00983 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00984 { 00985 int32_t d; /* Decision Variable */ 00986 uint32_t curx;/* Current X Value */ 00987 uint32_t cury;/* Current Y Value */ 00988 00989 d = 3 - (Radius << 1); 00990 00991 curx = 0; 00992 cury = Radius; 00993 00994 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 00995 00996 while (curx <= cury) 00997 { 00998 if(cury > 0) 00999 { 01000 BSP_LCD_DrawHLine(Xpos - cury, Ypos + curx, 2*cury); 01001 BSP_LCD_DrawHLine(Xpos - cury, Ypos - curx, 2*cury); 01002 } 01003 01004 if(curx > 0) 01005 { 01006 BSP_LCD_DrawHLine(Xpos - curx, Ypos - cury, 2*curx); 01007 BSP_LCD_DrawHLine(Xpos - curx, Ypos + cury, 2*curx); 01008 } 01009 if (d < 0) 01010 { 01011 d += (curx << 2) + 6; 01012 } 01013 else 01014 { 01015 d += ((curx - cury) << 2) + 10; 01016 cury--; 01017 } 01018 curx++; 01019 } 01020 01021 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 01022 BSP_LCD_DrawCircle(Xpos, Ypos, Radius); 01023 } 01024 01025 /** 01026 * @brief Fill triangle. 01027 * @param X1: the point 1 x position 01028 * @param Y1: the point 1 y position 01029 * @param X2: the point 2 x position 01030 * @param Y2: the point 2 y position 01031 * @param X3: the point 3 x position 01032 * @param Y3: the point 3 y position 01033 */ 01034 void BSP_LCD_FillTriangle(uint16_t X1, uint16_t X2, uint16_t X3, uint16_t Y1, uint16_t Y2, uint16_t Y3) 01035 { 01036 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 01037 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 01038 curpixel = 0; 01039 01040 deltax = ABS(X2 - X1); /* The difference between the x's */ 01041 deltay = ABS(Y2 - Y1); /* The difference between the y's */ 01042 x = X1; /* Start x off at the first pixel */ 01043 y = Y1; /* Start y off at the first pixel */ 01044 01045 if (X2 >= X1) /* The x-values are increasing */ 01046 { 01047 xinc1 = 1; 01048 xinc2 = 1; 01049 } 01050 else /* The x-values are decreasing */ 01051 { 01052 xinc1 = -1; 01053 xinc2 = -1; 01054 } 01055 01056 if (Y2 >= Y1) /* The y-values are increasing */ 01057 { 01058 yinc1 = 1; 01059 yinc2 = 1; 01060 } 01061 else /* The y-values are decreasing */ 01062 { 01063 yinc1 = -1; 01064 yinc2 = -1; 01065 } 01066 01067 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 01068 { 01069 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 01070 yinc2 = 0; /* Don't change the y for every iteration */ 01071 den = deltax; 01072 num = deltax / 2; 01073 numadd = deltay; 01074 numpixels = deltax; /* There are more x-values than y-values */ 01075 } 01076 else /* There is at least one y-value for every x-value */ 01077 { 01078 xinc2 = 0; /* Don't change the x for every iteration */ 01079 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 01080 den = deltay; 01081 num = deltay / 2; 01082 numadd = deltax; 01083 numpixels = deltay; /* There are more y-values than x-values */ 01084 } 01085 01086 for (curpixel = 0; curpixel <= numpixels; curpixel++) 01087 { 01088 BSP_LCD_DrawLine(x, y, X3, Y3); 01089 01090 num += numadd; /* Increase the numerator by the top of the fraction */ 01091 if (num >= den) /* Check if numerator >= denominator */ 01092 { 01093 num -= den; /* Calculate the new numerator value */ 01094 x += xinc1; /* Change the x as appropriate */ 01095 y += yinc1; /* Change the y as appropriate */ 01096 } 01097 x += xinc2; /* Change the x as appropriate */ 01098 y += yinc2; /* Change the y as appropriate */ 01099 } 01100 } 01101 01102 /** 01103 * @brief Displays a full poly-line (between many points). 01104 * @param Points: pointer to the points array 01105 * @param PointCount: Number of points 01106 */ 01107 void BSP_LCD_FillPolygon(pPoint Points, uint16_t PointCount) 01108 { 01109 01110 int16_t x = 0, y = 0, x2 = 0, y2 = 0, xcenter = 0, ycenter = 0, xfirst = 0, yfirst = 0, pixelx = 0, pixely = 0, counter = 0; 01111 uint16_t imageleft = 0, imageright = 0, imagetop = 0, imagebottom = 0; 01112 01113 imageleft = imageright = Points->X; 01114 imagetop= imagebottom = Points->Y; 01115 01116 for(counter = 1; counter < PointCount; counter++) 01117 { 01118 pixelx = POLY_X(counter); 01119 if(pixelx < imageleft) 01120 { 01121 imageleft = pixelx; 01122 } 01123 if(pixelx > imageright) 01124 { 01125 imageright = pixelx; 01126 } 01127 01128 pixely = POLY_Y(counter); 01129 if(pixely < imagetop) 01130 { 01131 imagetop = pixely; 01132 } 01133 if(pixely > imagebottom) 01134 { 01135 imagebottom = pixely; 01136 } 01137 } 01138 01139 if(PointCount < 2) 01140 { 01141 return; 01142 } 01143 01144 xcenter = (imageleft + imageright)/2; 01145 ycenter = (imagebottom + imagetop)/2; 01146 01147 xfirst = Points->X; 01148 yfirst = Points->Y; 01149 01150 while(--PointCount) 01151 { 01152 x = Points->X; 01153 y = Points->Y; 01154 Points++; 01155 x2 = Points->X; 01156 y2 = Points->Y; 01157 01158 BSP_LCD_FillTriangle(x, x2, xcenter, y, y2, ycenter); 01159 BSP_LCD_FillTriangle(x, xcenter, x2, y, ycenter, y2); 01160 BSP_LCD_FillTriangle(xcenter, x2, x, ycenter, y2, y); 01161 } 01162 01163 BSP_LCD_FillTriangle(xfirst, x2, xcenter, yfirst, y2, ycenter); 01164 BSP_LCD_FillTriangle(xfirst, xcenter, x2, yfirst, ycenter, y2); 01165 BSP_LCD_FillTriangle(xcenter, x2, xfirst, ycenter, y2, yfirst); 01166 } 01167 01168 /** 01169 * @brief Draw a full ellipse. 01170 * @param Xpos: the X position 01171 * @param Ypos: the Y position 01172 * @param XRadius: X radius of ellipse 01173 * @param YRadius: Y radius of ellipse. 01174 */ 01175 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 01176 { 01177 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 01178 float K = 0, rad1 = 0, rad2 = 0; 01179 01180 rad1 = XRadius; 01181 rad2 = YRadius; 01182 K = (float)(rad2/rad1); 01183 01184 do 01185 { 01186 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/K)), (Ypos+y), (2*(uint16_t)(x/K) + 1)); 01187 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/K)), (Ypos-y), (2*(uint16_t)(x/K) + 1)); 01188 01189 e2 = err; 01190 if (e2 <= x) 01191 { 01192 err += ++x*2+1; 01193 if (-y == x && e2 <= y) e2 = 0; 01194 } 01195 if (e2 > y) err += ++y*2+1; 01196 } 01197 while (y <= 0); 01198 } 01199 01200 /** 01201 * @brief Enables the Display. 01202 */ 01203 void BSP_LCD_DisplayOn(void) 01204 { 01205 if(LcdDrv->DisplayOn != NULL) 01206 { 01207 LcdDrv->DisplayOn(); 01208 } 01209 } 01210 01211 /** 01212 * @brief Disables the Display. 01213 */ 01214 void BSP_LCD_DisplayOff(void) 01215 { 01216 if(LcdDrv->DisplayOff != NULL) 01217 { 01218 LcdDrv->DisplayOff(); 01219 } 01220 } 01221 01222 /******************************************************************************* 01223 LTDC and DMA2D BSP Routines 01224 *******************************************************************************/ 01225 01226 /** 01227 * @brief Initializes the LTDC MSP. 01228 */ 01229 __weak void BSP_LCD_MspInit(void) 01230 { 01231 GPIO_InitTypeDef GPIO_InitStructure; 01232 01233 /* Enable the LTDC and DMA2D Clock */ 01234 __HAL_RCC_LTDC_CLK_ENABLE(); 01235 __HAL_RCC_DMA2D_CLK_ENABLE(); 01236 01237 /* Enable GPIOs clock */ 01238 __HAL_RCC_GPIOA_CLK_ENABLE(); 01239 __HAL_RCC_GPIOB_CLK_ENABLE(); 01240 __HAL_RCC_GPIOC_CLK_ENABLE(); 01241 __HAL_RCC_GPIOD_CLK_ENABLE(); 01242 __HAL_RCC_GPIOF_CLK_ENABLE(); 01243 __HAL_RCC_GPIOG_CLK_ENABLE(); 01244 01245 /* GPIOs Configuration */ 01246 /* 01247 +------------------------+-----------------------+----------------------------+ 01248 + LCD pins assignment + 01249 +------------------------+-----------------------+----------------------------+ 01250 | LCD_TFT R2 <-> PC.10 | LCD_TFT G2 <-> PA.06 | LCD_TFT B2 <-> PD.06 | 01251 | LCD_TFT R3 <-> PB.00 | LCD_TFT G3 <-> PG.10 | LCD_TFT B3 <-> PG.11 | 01252 | LCD_TFT R4 <-> PA.11 | LCD_TFT G4 <-> PB.10 | LCD_TFT B4 <-> PG.12 | 01253 | LCD_TFT R5 <-> PA.12 | LCD_TFT G5 <-> PB.11 | LCD_TFT B5 <-> PA.03 | 01254 | LCD_TFT R6 <-> PB.01 | LCD_TFT G6 <-> PC.07 | LCD_TFT B6 <-> PB.08 | 01255 | LCD_TFT R7 <-> PG.06 | LCD_TFT G7 <-> PD.03 | LCD_TFT B7 <-> PB.09 | 01256 ------------------------------------------------------------------------------- 01257 | LCD_TFT HSYNC <-> PC.06 | LCDTFT VSYNC <-> PA.04 | 01258 | LCD_TFT CLK <-> PG.07 | LCD_TFT DE <-> PF.10 | 01259 ----------------------------------------------------- 01260 */ 01261 01262 /* GPIOA configuration */ 01263 GPIO_InitStructure.Pin = GPIO_PIN_3 | GPIO_PIN_4 | GPIO_PIN_6 | 01264 GPIO_PIN_11 | GPIO_PIN_12; 01265 GPIO_InitStructure.Mode = GPIO_MODE_AF_PP; 01266 GPIO_InitStructure.Pull = GPIO_NOPULL; 01267 GPIO_InitStructure.Speed = GPIO_SPEED_FAST; 01268 GPIO_InitStructure.Alternate= GPIO_AF14_LTDC; 01269 HAL_GPIO_Init(GPIOA, &GPIO_InitStructure); 01270 01271 /* GPIOB configuration */ 01272 GPIO_InitStructure.Pin = GPIO_PIN_8 | \ 01273 GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11; 01274 HAL_GPIO_Init(GPIOB, &GPIO_InitStructure); 01275 01276 /* GPIOC configuration */ 01277 GPIO_InitStructure.Pin = GPIO_PIN_6 | GPIO_PIN_7 | GPIO_PIN_10; 01278 HAL_GPIO_Init(GPIOC, &GPIO_InitStructure); 01279 01280 /* GPIOD configuration */ 01281 GPIO_InitStructure.Pin = GPIO_PIN_3 | GPIO_PIN_6; 01282 HAL_GPIO_Init(GPIOD, &GPIO_InitStructure); 01283 01284 /* GPIOF configuration */ 01285 GPIO_InitStructure.Pin = GPIO_PIN_10; 01286 HAL_GPIO_Init(GPIOF, &GPIO_InitStructure); 01287 01288 /* GPIOG configuration */ 01289 GPIO_InitStructure.Pin = GPIO_PIN_6 | GPIO_PIN_7 | \ 01290 GPIO_PIN_11; 01291 HAL_GPIO_Init(GPIOG, &GPIO_InitStructure); 01292 01293 /* GPIOB configuration */ 01294 GPIO_InitStructure.Pin = GPIO_PIN_0 | GPIO_PIN_1; 01295 GPIO_InitStructure.Alternate= GPIO_AF9_LTDC; 01296 HAL_GPIO_Init(GPIOB, &GPIO_InitStructure); 01297 01298 /* GPIOG configuration */ 01299 GPIO_InitStructure.Pin = GPIO_PIN_10 | GPIO_PIN_12; 01300 HAL_GPIO_Init(GPIOG, &GPIO_InitStructure); 01301 } 01302 01303 /******************************************************************************* 01304 Static Functions 01305 *******************************************************************************/ 01306 01307 /** 01308 * @brief Writes Pixel. 01309 * @param Xpos: the X position 01310 * @param Ypos: the Y position 01311 * @param RGB_Code: the pixel color in ARGB mode (8-8-8-8) 01312 */ 01313 void BSP_LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint32_t RGB_Code) 01314 { 01315 /* Write data value to all SDRAM memory */ 01316 *(__IO uint32_t*) (LtdcHandler.LayerCfg[ActiveLayer].FBStartAdress + (4*(Ypos*BSP_LCD_GetXSize() + Xpos))) = RGB_Code; 01317 } 01318 01319 /** 01320 * @brief Draws a character on LCD. 01321 * @param Xpos: the Line where to display the character shape 01322 * @param Ypos: start column address 01323 * @param c: pointer to the character data 01324 */ 01325 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c) 01326 { 01327 uint32_t i = 0, j = 0; 01328 uint16_t height, width; 01329 uint8_t offset; 01330 uint8_t *pchar; 01331 uint32_t line=0; 01332 01333 height = DrawProp[ActiveLayer].pFont->Height; 01334 width = DrawProp[ActiveLayer].pFont->Width; 01335 01336 offset = 8 *((width + 7)/8) - width ; 01337 01338 for(i = 0; i < height; i++) 01339 { 01340 pchar = ((uint8_t *)c + (width + 7)/8 * i); 01341 01342 switch(((width + 7)/8)) 01343 { 01344 case 1: 01345 line = pchar[0]; 01346 break; 01347 01348 case 2: 01349 line = (pchar[0]<< 8) | pchar[1]; 01350 break; 01351 01352 case 3: 01353 default: 01354 line = (pchar[0]<< 16) | (pchar[1]<< 8) | pchar[2]; 01355 break; 01356 } 01357 01358 for (j = 0; j < width; j++) 01359 { 01360 if(line & (1 << (width- j + offset- 1))) 01361 { 01362 BSP_LCD_DrawPixel((Xpos + j), Ypos, DrawProp[ActiveLayer].TextColor); 01363 } 01364 else 01365 { 01366 BSP_LCD_DrawPixel((Xpos + j), Ypos, DrawProp[ActiveLayer].BackColor); 01367 } 01368 } 01369 Ypos++; 01370 } 01371 } 01372 01373 /** 01374 * @brief Fills buffer. 01375 * @param LayerIndex: layer index 01376 * @param pDst: output color 01377 * @param xSize: buffer width 01378 * @param ySize: buffer height 01379 * @param OffLine: offset 01380 * @param ColorIndex: color Index 01381 */ 01382 static void FillBuffer(uint32_t LayerIndex, void * pDst, uint32_t xSize, uint32_t ySize, uint32_t OffLine, uint32_t ColorIndex) 01383 { 01384 01385 /* Register to memory mode with ARGB8888 as color Mode */ 01386 Dma2dHandler.Init.Mode = DMA2D_R2M; 01387 Dma2dHandler.Init.ColorMode = DMA2D_ARGB8888; 01388 Dma2dHandler.Init.OutputOffset = OffLine; 01389 01390 Dma2dHandler.Instance = DMA2D; 01391 01392 /* DMA2D Initialization */ 01393 if(HAL_DMA2D_Init(&Dma2dHandler) == HAL_OK) 01394 { 01395 if(HAL_DMA2D_ConfigLayer(&Dma2dHandler, LayerIndex) == HAL_OK) 01396 { 01397 if (HAL_DMA2D_Start(&Dma2dHandler, ColorIndex, (uint32_t)pDst, xSize, ySize) == HAL_OK) 01398 { 01399 /* Polling For DMA transfer */ 01400 HAL_DMA2D_PollForTransfer(&Dma2dHandler, 10); 01401 } 01402 } 01403 } 01404 } 01405 01406 /** 01407 * @brief Converts Line to ARGB8888 pixel format. 01408 * @param pSrc: pointer to source buffer 01409 * @param pDst: output color 01410 * @param xSize: buffer width 01411 * @param ColorMode: input color mode 01412 */ 01413 static void ConvertLineToARGB8888(void * pSrc, void * pDst, uint32_t xSize, uint32_t ColorMode) 01414 { 01415 /* Configure the DMA2D Mode, Color Mode and output offset */ 01416 Dma2dHandler.Init.Mode = DMA2D_M2M_PFC; 01417 Dma2dHandler.Init.ColorMode = DMA2D_ARGB8888; 01418 Dma2dHandler.Init.OutputOffset = 0; 01419 01420 /* Foreground Configuration */ 01421 Dma2dHandler.LayerCfg[1].AlphaMode = DMA2D_NO_MODIF_ALPHA; 01422 Dma2dHandler.LayerCfg[1].InputAlpha = 0xFF; 01423 Dma2dHandler.LayerCfg[1].InputColorMode = ColorMode; 01424 Dma2dHandler.LayerCfg[1].InputOffset = 0; 01425 01426 Dma2dHandler.Instance = DMA2D; 01427 01428 /* DMA2D Initialization */ 01429 if(HAL_DMA2D_Init(&Dma2dHandler) == HAL_OK) 01430 { 01431 if(HAL_DMA2D_ConfigLayer(&Dma2dHandler, 1) == HAL_OK) 01432 { 01433 if (HAL_DMA2D_Start(&Dma2dHandler, (uint32_t)pSrc, (uint32_t)pDst, xSize, 1) == HAL_OK) 01434 { 01435 /* Polling For DMA transfer */ 01436 HAL_DMA2D_PollForTransfer(&Dma2dHandler, 10); 01437 } 01438 } 01439 } 01440 } 01441 01442 /** 01443 * @} 01444 */ 01445 01446 /** 01447 * @} 01448 */ 01449 01450 /** 01451 * @} 01452 */ 01453 01454 /** 01455 * @} 01456 */ 01457 01458 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Feb 17 2017 12:10:38 for STM32F429I-Discovery BSP User Manual by
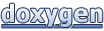