STM32769I_EVAL BSP User Manual
|
stm32f769i_eval_eeprom.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f769i_eval_eeprom.c 00004 * @author MCD Application Team 00005 * @version V2.0.1 00006 * @date 06-April-2017 00007 * @brief This file provides a set of functions needed to manage an I2C M24LR64 00008 * EEPROM memory. 00009 @verbatim 00010 To be able to use this driver, the switch EE_M24LR64 must be defined 00011 in your toolchain compiler preprocessor 00012 00013 =================================================================== 00014 Notes: 00015 - The I2C EEPROM memory (M24LR64) is available on separate daughter 00016 board ANT7-M24LR-A, which is not provided with the STM32F769I_EVAL 00017 board. 00018 To use this driver you have to connect the ANT7-M24LR-A to CN2 00019 connector of STM32F769I_EVAL board. 00020 =================================================================== 00021 00022 It implements a high level communication layer for read and write 00023 from/to this memory. The needed STM32F7xx hardware resources (I2C and 00024 GPIO) are defined in stm32f769i_eval.h file, and the initialization is 00025 performed in EEPROM_IO_Init() function declared in stm32f769i_eval.c 00026 file. 00027 You can easily tailor this driver to any other development board, 00028 by just adapting the defines for hardware resources and 00029 EEPROM_IO_Init() function. 00030 00031 @note In this driver, basic read and write functions (BSP_EEPROM_ReadBuffer() 00032 and BSP_EEPROM_WritePage()) use DMA mode to perform the data 00033 transfer to/from EEPROM memory. 00034 00035 @note Regarding BSP_EEPROM_WritePage(), it is an optimized function to perform 00036 small write (less than 1 page) BUT the number of bytes (combined to write start address) must not 00037 cross the EEPROM page boundary. This function can only writes into 00038 the boundaries of an EEPROM page. 00039 This function doesn't check on boundaries condition (in this driver 00040 the function BSP_EEPROM_WriteBuffer() which calls BSP_EEPROM_WritePage() is 00041 responsible of checking on Page boundaries). 00042 00043 00044 +-----------------------------------------------------------------+ 00045 | Pin assignment for M24LR64 EEPROM | 00046 +---------------------------------------+-----------+-------------+ 00047 | STM32F7xx I2C Pins | EEPROM | Pin | 00048 +---------------------------------------+-----------+-------------+ 00049 | . | E0(GND) | 1 (0V) | 00050 | . | AC0 | 2 | 00051 | . | AC1 | 3 | 00052 | . | VSS | 4 (0V) | 00053 | SDA | SDA | 5 | 00054 | SCL | SCL | 6 | 00055 | . | E1(GND) | 7 (0V) | 00056 | . | VDD | 8 (3.3V) | 00057 +---------------------------------------+-----------+-------------+ 00058 @endverbatim 00059 ****************************************************************************** 00060 * @attention 00061 * 00062 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00063 * 00064 * Redistribution and use in source and binary forms, with or without modification, 00065 * are permitted provided that the following conditions are met: 00066 * 1. Redistributions of source code must retain the above copyright notice, 00067 * this list of conditions and the following disclaimer. 00068 * 2. Redistributions in binary form must reproduce the above copyright notice, 00069 * this list of conditions and the following disclaimer in the documentation 00070 * and/or other materials provided with the distribution. 00071 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00072 * may be used to endorse or promote products derived from this software 00073 * without specific prior written permission. 00074 * 00075 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00076 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00077 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00078 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00079 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00080 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00081 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00082 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00083 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00084 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00085 * 00086 ****************************************************************************** 00087 */ 00088 00089 /* Dependencies 00090 - stm32f769i_eval.c 00091 EndDependencies */ 00092 00093 /* Includes ------------------------------------------------------------------*/ 00094 #include "stm32f769i_eval_eeprom.h" 00095 00096 /** @addtogroup BSP 00097 * @{ 00098 */ 00099 00100 /** @addtogroup STM32F769I_EVAL 00101 * @{ 00102 */ 00103 00104 /** @defgroup STM32F769I_EVAL_EEPROM STM32F769I_EVAL EEPROM 00105 * @brief This file includes the I2C EEPROM driver of STM32F769I-EVAL evaluation board. 00106 * @{ 00107 */ 00108 00109 /** @defgroup STM32F769I_EVAL_EEPROM_Private_Types EEPROM Private Types 00110 * @{ 00111 */ 00112 /** 00113 * @} 00114 */ 00115 00116 /** @defgroup STM32F769I_EVAL_EEPROM_Private_Defines EEPROM Private Defines 00117 * @{ 00118 */ 00119 /** 00120 * @} 00121 */ 00122 00123 /** @defgroup STM32F769I_EVAL_EEPROM_Private_Macros EEPROM Private Macros 00124 * @{ 00125 */ 00126 /** 00127 * @} 00128 */ 00129 00130 /** @defgroup STM32F769I_EVAL_EEPROM_Private_Variables EEPROM Private Variables 00131 * @{ 00132 */ 00133 __IO uint16_t EEPROMAddress = 0; 00134 __IO uint16_t EEPROMDataRead; 00135 __IO uint8_t EEPROMDataWrite; 00136 /** 00137 * @} 00138 */ 00139 00140 /** @defgroup STM32F769I_EVAL_EEPROM_Private_Function_Prototypes EEPROM Private Functions Prototypes 00141 * @{ 00142 */ 00143 /** 00144 * @} 00145 */ 00146 00147 /** @defgroup STM32F769I_EVAL_EEPROM_Private_Functions EEPROM Private Functions 00148 * @{ 00149 */ 00150 00151 /** 00152 * @brief Initializes peripherals used by the I2C EEPROM driver. 00153 * @note There are 2 different versions of M24LR64 (A01 & A02). 00154 * Then try to connect on 1st one (EEPROM_I2C_ADDRESS_A01) 00155 * and if problem, check the 2nd one (EEPROM_I2C_ADDRESS_A02) 00156 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00157 * different from EEPROM_OK (0) 00158 */ 00159 uint32_t BSP_EEPROM_Init(void) 00160 { 00161 /* I2C Initialization */ 00162 EEPROM_IO_Init(); 00163 00164 /* Select the EEPROM address for A01 and check if OK */ 00165 EEPROMAddress = EEPROM_I2C_ADDRESS_A01; 00166 if(EEPROM_IO_IsDeviceReady(EEPROMAddress, EEPROM_MAX_TRIALS) != HAL_OK) 00167 { 00168 /* Select the EEPROM address for A02 and check if OK */ 00169 EEPROMAddress = EEPROM_I2C_ADDRESS_A02; 00170 if(EEPROM_IO_IsDeviceReady(EEPROMAddress, EEPROM_MAX_TRIALS) != HAL_OK) 00171 { 00172 return EEPROM_FAIL; 00173 } 00174 } 00175 return EEPROM_OK; 00176 } 00177 00178 /** 00179 * @brief DeInitializes the EEPROM. 00180 * @retval EEPROM state 00181 */ 00182 uint8_t BSP_EEPROM_DeInit(void) 00183 { 00184 /* I2C won't be disabled because common to other functionalities */ 00185 return EEPROM_OK; 00186 } 00187 00188 /** 00189 * @brief Reads a block of data from the EEPROM. 00190 * @param pBuffer: pointer to the buffer that receives the data read from 00191 * the EEPROM. 00192 * @param ReadAddr: EEPROM's internal address to start reading from. 00193 * @param NumByteToRead: pointer to the variable holding number of bytes to 00194 * be read from the EEPROM. 00195 * 00196 * @note The variable pointed by NumByteToRead is reset to 0 when all the 00197 * data are read from the EEPROM. Application should monitor this 00198 * variable in order know when the transfer is complete. 00199 * 00200 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00201 * different from EEPROM_OK (0) or the timeout user callback. 00202 */ 00203 uint32_t BSP_EEPROM_ReadBuffer(uint8_t* pBuffer, uint16_t ReadAddr, uint16_t* NumByteToRead) 00204 { 00205 uint32_t buffersize = *NumByteToRead; 00206 00207 /* Set the pointer to the Number of data to be read. This pointer will be used 00208 by the DMA Transfer Completer interrupt Handler in order to reset the 00209 variable to 0. User should check on this variable in order to know if the 00210 DMA transfer has been complete or not. */ 00211 EEPROMDataRead = *NumByteToRead; 00212 00213 if(EEPROM_IO_ReadData(EEPROMAddress, ReadAddr, pBuffer, buffersize) != HAL_OK) 00214 { 00215 BSP_EEPROM_TIMEOUT_UserCallback(); 00216 return EEPROM_FAIL; 00217 } 00218 00219 /* If all operations OK, return EEPROM_OK (0) */ 00220 return EEPROM_OK; 00221 } 00222 00223 /** 00224 * @brief Writes more than one byte to the EEPROM with a single WRITE cycle. 00225 * 00226 * @note The number of bytes (combined to write start address) must not 00227 * cross the EEPROM page boundary. This function can only write into 00228 * the boundaries of an EEPROM page. 00229 * This function doesn't check on boundaries condition (in this driver 00230 * the function BSP_EEPROM_WriteBuffer() which calls BSP_EEPROM_WritePage() is 00231 * responsible of checking on Page boundaries). 00232 * 00233 * @param pBuffer: pointer to the buffer containing the data to be written to 00234 * the EEPROM. 00235 * @param WriteAddr: EEPROM's internal address to write to. 00236 * @param NumByteToWrite: pointer to the variable holding number of bytes to 00237 * be written into the EEPROM. 00238 * 00239 * @note The variable pointed by NumByteToWrite is reset to 0 when all the 00240 * data are written to the EEPROM. Application should monitor this 00241 * variable in order know when the transfer is complete. 00242 * 00243 * @note This function just configure the communication and enable the DMA 00244 * channel to transfer data. Meanwhile, the user application may perform 00245 * other tasks in parallel. 00246 * 00247 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00248 * different from EEPROM_OK (0) or the timeout user callback. 00249 */ 00250 uint32_t BSP_EEPROM_WritePage(uint8_t* pBuffer, uint16_t WriteAddr, uint8_t* NumByteToWrite) 00251 { 00252 uint32_t buffersize = *NumByteToWrite; 00253 uint32_t status = EEPROM_OK; 00254 00255 /* Set the pointer to the Number of data to be written. This pointer will be used 00256 by the DMA Transfer Completer interrupt Handler in order to reset the 00257 variable to 0. User should check on this variable in order to know if the 00258 DMA transfer has been complete or not. */ 00259 EEPROMDataWrite = *NumByteToWrite; 00260 00261 if(EEPROM_IO_WriteData(EEPROMAddress, WriteAddr, pBuffer, buffersize) != HAL_OK) 00262 { 00263 BSP_EEPROM_TIMEOUT_UserCallback(); 00264 status = EEPROM_FAIL; 00265 } 00266 00267 if(BSP_EEPROM_WaitEepromStandbyState() != EEPROM_OK) 00268 { 00269 return EEPROM_FAIL; 00270 } 00271 00272 /* If all operations OK, return EEPROM_OK (0) */ 00273 return status; 00274 } 00275 00276 /** 00277 * @brief Writes buffer of data to the I2C EEPROM. 00278 * @param pBuffer: pointer to the buffer containing the data to be written 00279 * to the EEPROM. 00280 * @param WriteAddr: EEPROM's internal address to write to. 00281 * @param NumByteToWrite: number of bytes to write to the EEPROM. 00282 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00283 * different from EEPROM_OK (0) or the timeout user callback. 00284 */ 00285 uint32_t BSP_EEPROM_WriteBuffer(uint8_t *pBuffer, uint16_t WriteAddr, uint16_t NumByteToWrite) 00286 { 00287 uint16_t numofpage = 0, numofsingle = 0, count = 0; 00288 uint16_t addr = 0; 00289 uint8_t dataindex = 0; 00290 uint32_t status = EEPROM_OK; 00291 00292 addr = WriteAddr % EEPROM_PAGESIZE; 00293 count = EEPROM_PAGESIZE - addr; 00294 numofpage = NumByteToWrite / EEPROM_PAGESIZE; 00295 numofsingle = NumByteToWrite % EEPROM_PAGESIZE; 00296 00297 /* If WriteAddr is EEPROM_PAGESIZE aligned */ 00298 if(addr == 0) 00299 { 00300 /* If NumByteToWrite < EEPROM_PAGESIZE */ 00301 if(numofpage == 0) 00302 { 00303 /* Store the number of data to be written */ 00304 dataindex = numofsingle; 00305 /* Start writing data */ 00306 status = BSP_EEPROM_WritePage(pBuffer, WriteAddr, (uint8_t*)(&dataindex)); 00307 if(status != EEPROM_OK) 00308 { 00309 return status; 00310 } 00311 } 00312 /* If NumByteToWrite > EEPROM_PAGESIZE */ 00313 else 00314 { 00315 while(numofpage--) 00316 { 00317 /* Store the number of data to be written */ 00318 dataindex = EEPROM_PAGESIZE; 00319 status = BSP_EEPROM_WritePage(pBuffer, WriteAddr, (uint8_t*)(&dataindex)); 00320 if(status != EEPROM_OK) 00321 { 00322 return status; 00323 } 00324 00325 WriteAddr += EEPROM_PAGESIZE; 00326 pBuffer += EEPROM_PAGESIZE; 00327 } 00328 00329 if(numofsingle!=0) 00330 { 00331 /* Store the number of data to be written */ 00332 dataindex = numofsingle; 00333 status = BSP_EEPROM_WritePage(pBuffer, WriteAddr, (uint8_t*)(&dataindex)); 00334 if(status != EEPROM_OK) 00335 { 00336 return status; 00337 } 00338 } 00339 } 00340 } 00341 /* If WriteAddr is not EEPROM_PAGESIZE aligned */ 00342 else 00343 { 00344 /* If NumByteToWrite < EEPROM_PAGESIZE */ 00345 if(numofpage== 0) 00346 { 00347 /* If the number of data to be written is more than the remaining space 00348 in the current page: */ 00349 if(NumByteToWrite > count) 00350 { 00351 /* Store the number of data to be written */ 00352 dataindex = count; 00353 /* Write the data contained in same page */ 00354 status = BSP_EEPROM_WritePage(pBuffer, WriteAddr, (uint8_t*)(&dataindex)); 00355 if(status != EEPROM_OK) 00356 { 00357 return status; 00358 } 00359 00360 /* Store the number of data to be written */ 00361 dataindex = (NumByteToWrite - count); 00362 /* Write the remaining data in the following page */ 00363 status = BSP_EEPROM_WritePage((uint8_t*)(pBuffer + count), (WriteAddr + count), (uint8_t*)(&dataindex)); 00364 if(status != EEPROM_OK) 00365 { 00366 return status; 00367 } 00368 } 00369 else 00370 { 00371 /* Store the number of data to be written */ 00372 dataindex = numofsingle; 00373 status = BSP_EEPROM_WritePage(pBuffer, WriteAddr, (uint8_t*)(&dataindex)); 00374 if(status != EEPROM_OK) 00375 { 00376 return status; 00377 } 00378 } 00379 } 00380 /* If NumByteToWrite > EEPROM_PAGESIZE */ 00381 else 00382 { 00383 NumByteToWrite -= count; 00384 numofpage = NumByteToWrite / EEPROM_PAGESIZE; 00385 numofsingle = NumByteToWrite % EEPROM_PAGESIZE; 00386 00387 if(count != 0) 00388 { 00389 /* Store the number of data to be written */ 00390 dataindex = count; 00391 status = BSP_EEPROM_WritePage(pBuffer, WriteAddr, (uint8_t*)(&dataindex)); 00392 if(status != EEPROM_OK) 00393 { 00394 return status; 00395 } 00396 WriteAddr += count; 00397 pBuffer += count; 00398 } 00399 00400 while(numofpage--) 00401 { 00402 /* Store the number of data to be written */ 00403 dataindex = EEPROM_PAGESIZE; 00404 status = BSP_EEPROM_WritePage(pBuffer, WriteAddr, (uint8_t*)(&dataindex)); 00405 if(status != EEPROM_OK) 00406 { 00407 return status; 00408 } 00409 WriteAddr += EEPROM_PAGESIZE; 00410 pBuffer += EEPROM_PAGESIZE; 00411 } 00412 if(numofsingle != 0) 00413 { 00414 /* Store the number of data to be written */ 00415 dataindex = numofsingle; 00416 status = BSP_EEPROM_WritePage(pBuffer, WriteAddr, (uint8_t*)(&dataindex)); 00417 if(status != EEPROM_OK) 00418 { 00419 return status; 00420 } 00421 } 00422 } 00423 } 00424 00425 /* If all operations OK, return EEPROM_OK (0) */ 00426 return EEPROM_OK; 00427 } 00428 00429 /** 00430 * @brief Wait for EEPROM Standby state. 00431 * 00432 * @note This function allows to wait and check that EEPROM has finished the 00433 * last operation. It is mostly used after Write operation: after receiving 00434 * the buffer to be written, the EEPROM may need additional time to actually 00435 * perform the write operation. During this time, it doesn't answer to 00436 * I2C packets addressed to it. Once the write operation is complete 00437 * the EEPROM responds to its address. 00438 * 00439 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00440 * different from EEPROM_OK (0) or the timeout user callback. 00441 */ 00442 uint32_t BSP_EEPROM_WaitEepromStandbyState(void) 00443 { 00444 /* Check if the maximum allowed number of trials has bee reached */ 00445 if(EEPROM_IO_IsDeviceReady(EEPROMAddress, EEPROM_MAX_TRIALS) != HAL_OK) 00446 { 00447 /* If the maximum number of trials has been reached, exit the function */ 00448 BSP_EEPROM_TIMEOUT_UserCallback(); 00449 return EEPROM_TIMEOUT; 00450 } 00451 return EEPROM_OK; 00452 } 00453 00454 /** 00455 * @brief Basic management of the timeout situation. 00456 * @retval None 00457 */ 00458 __weak void BSP_EEPROM_TIMEOUT_UserCallback(void) 00459 { 00460 } 00461 00462 /** 00463 * @} 00464 */ 00465 00466 /** 00467 * @} 00468 */ 00469 00470 /** 00471 * @} 00472 */ 00473 00474 /** 00475 * @} 00476 */ 00477 00478 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu May 25 2017 11:03:11 for STM32769I_EVAL BSP User Manual by
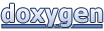