STM32769I_EVAL BSP User Manual
|
stm32f769i_eval_camera.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f769i_eval_camera.c 00004 * @author MCD Application Team 00005 * @version V2.0.1 00006 * @date 06-April-2017 00007 * @brief This file includes the driver for Camera modules mounted on 00008 * STM32F769I-EVAL evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info: ------------------------------------------------------------------ 00040 User NOTES 00041 1. How to use this driver: 00042 -------------------------- 00043 - This driver is used to drive the camera. 00044 - The S5K5CAG component driver MUST be included with this driver. 00045 00046 2. Driver description: 00047 --------------------- 00048 + Initialization steps: 00049 o Initialize the camera using the BSP_CAMERA_Init() function. 00050 o Start the camera capture/snapshot using the CAMERA_Start() function. 00051 o Suspend, resume or stop the camera capture using the following functions: 00052 - BSP_CAMERA_Suspend() 00053 - BSP_CAMERA_Resume() 00054 - BSP_CAMERA_Stop() 00055 00056 + Options 00057 o Increase or decrease on the fly the brightness and/or contrast 00058 using the following function: 00059 - BSP_CAMERA_ContrastBrightnessConfig 00060 o Add a special effect on the fly using the following functions: 00061 - BSP_CAMERA_BlackWhiteConfig() 00062 - BSP_CAMERA_ColorEffectConfig() 00063 00064 ------------------------------------------------------------------------------*/ 00065 00066 /* Dependencies 00067 - stm32f769i_eval.c 00068 - stm32f7xx_hal_dcmi.c 00069 - stm32f7xx_hal_dma.c 00070 - stm32f7xx_hal_gpio.c 00071 - stm32f7xx_hal_cortex.c 00072 - stm32f7xx_hal_rcc_ex.h 00073 - s5k5cag.c 00074 EndDependencies */ 00075 00076 /* Includes ------------------------------------------------------------------*/ 00077 #include "stm32f769i_eval_camera.h" 00078 00079 /** @addtogroup BSP 00080 * @{ 00081 */ 00082 00083 /** @addtogroup STM32F769I_EVAL 00084 * @{ 00085 */ 00086 00087 /** @defgroup STM32F769I_EVAL_CAMERA STM32F769I_EVAL CAMERA 00088 * @{ 00089 */ 00090 00091 /** @defgroup STM32F769I_EVAL_CAMERA_Private_TypesDefinitions STM32F769I Eval Camera Private TypesDef 00092 * @{ 00093 */ 00094 /** 00095 * @} 00096 */ 00097 00098 /** @defgroup STM32F769I_EVAL_CAMERA_Private_Defines STM32F769I Eval Camera Private Defines 00099 * @{ 00100 */ 00101 /** 00102 * @} 00103 */ 00104 00105 /** @defgroup STM32F769I_EVAL_CAMERA_Private_Macros STM32F769I Eval Camera Private Macros 00106 * @{ 00107 */ 00108 /** 00109 * @} 00110 */ 00111 00112 /** @defgroup STM32F769I_EVAL_CAMERA_Imported_Variables STM32F769I Eval Camera Imported Variables 00113 * @{ 00114 */ 00115 /** 00116 * @brief DMA2D handle variable 00117 */ 00118 extern DMA2D_HandleTypeDef hdma2d_eval; 00119 /** 00120 * @} 00121 */ 00122 00123 /** @defgroup STM32F769I_EVAL_CAMERA_Private_Variables STM32F769I Eval Camera Private Variables 00124 * @{ 00125 */ 00126 DCMI_HandleTypeDef hDcmiEval; 00127 CAMERA_DrvTypeDef *CameraDrv; 00128 00129 /* Camera current resolution naming (QQVGA, VGA, ...) */ 00130 uint32_t CameraCurrentResolution; 00131 00132 /* Camera image rotation on LCD Displayed frame buffer */ 00133 uint32_t CameraRotation = CAMERA_ROTATION_INVALID; 00134 00135 static uint32_t CameraHwAddress; 00136 00137 /** 00138 * @} 00139 */ 00140 00141 /** @defgroup STM32F769I_EVAL_CAMERA_Private_FunctionPrototypes STM32F769I Eval Camera Private Prototypes 00142 * @{ 00143 */ 00144 static uint32_t GetSize(uint32_t Resolution); 00145 /** 00146 * @} 00147 */ 00148 00149 /** @defgroup STM32F769I_EVAL_CAMERA_Public_Functions STM32F769I Eval Camera Public Functions 00150 * @{ 00151 */ 00152 00153 /** 00154 * @brief Set Camera image rotation on LCD Displayed frame buffer. 00155 * @param rotation : uint32_t rotation of camera image in preview buffer sent to LCD 00156 * need to be of type Camera_ImageRotationTypeDef 00157 * @retval Camera status 00158 */ 00159 uint8_t BSP_CAMERA_SetRotation(uint32_t rotation) 00160 { 00161 uint8_t status = CAMERA_ERROR; 00162 00163 if(rotation < CAMERA_ROTATION_INVALID) 00164 { 00165 /* Set Camera image rotation on LCD Displayed frame buffer */ 00166 CameraRotation = rotation; 00167 status = CAMERA_OK; 00168 } 00169 00170 return status; 00171 } 00172 00173 /** 00174 * @brief Get Camera image rotation on LCD Displayed frame buffer. 00175 * @retval rotation : uint32_t value of type Camera_ImageRotationTypeDef 00176 */ 00177 uint32_t BSP_CAMERA_GetRotation(void) 00178 { 00179 return(CameraRotation); 00180 } 00181 00182 /** 00183 * @brief Initializes the camera. 00184 * @param Resolution : camera sensor requested resolution (x, y) : standard resolution 00185 * naming QQVGA, QVGA, VGA ... 00186 * @retval Camera status 00187 */ 00188 uint8_t BSP_CAMERA_Init(uint32_t Resolution) 00189 { 00190 DCMI_HandleTypeDef *phdcmi; 00191 uint8_t status = CAMERA_ERROR; 00192 00193 /* Get the DCMI handle structure */ 00194 phdcmi = &hDcmiEval; 00195 00196 /*** Configures the DCMI to interface with the camera module ***/ 00197 /* DCMI configuration */ 00198 phdcmi->Init.CaptureRate = DCMI_CR_ALL_FRAME; 00199 phdcmi->Init.HSPolarity = DCMI_HSPOLARITY_HIGH; 00200 phdcmi->Init.SynchroMode = DCMI_SYNCHRO_HARDWARE; 00201 phdcmi->Init.VSPolarity = DCMI_VSPOLARITY_HIGH; 00202 phdcmi->Init.ExtendedDataMode = DCMI_EXTEND_DATA_8B; 00203 phdcmi->Init.PCKPolarity = DCMI_PCKPOLARITY_RISING; 00204 00205 phdcmi->Instance = DCMI; 00206 00207 /* Configure IO functionalities for CAMERA detect pin */ 00208 BSP_IO_Init(); 00209 /* Apply Camera Module hardware reset */ 00210 BSP_CAMERA_HwReset(); 00211 00212 /* Check if the CAMERA Module is plugged on board */ 00213 if(BSP_IO_ReadPin(CAM_PLUG_PIN) == BSP_IO_PIN_SET) 00214 { 00215 status = CAMERA_NOT_DETECTED; 00216 return status; /* Exit with error */ 00217 } 00218 00219 /* Read ID of Camera module via I2C */ 00220 if (s5k5cag_ReadID(CAMERA_I2C_ADDRESS) == S5K5CAG_ID) 00221 { 00222 /* Initialize the camera driver structure */ 00223 CameraDrv = &s5k5cag_drv; 00224 CameraHwAddress = CAMERA_I2C_ADDRESS; 00225 00226 /* DCMI Initialization */ 00227 BSP_CAMERA_MspInit(&hDcmiEval, NULL); 00228 HAL_DCMI_Init(phdcmi); 00229 00230 /* Camera Module Initialization via I2C to the wanted 'Resolution' */ 00231 CameraDrv->Init(CameraHwAddress, Resolution); 00232 00233 CameraCurrentResolution = Resolution; 00234 00235 /* Return CAMERA_OK status */ 00236 status = CAMERA_OK; 00237 } 00238 else 00239 { 00240 /* Return CAMERA_NOT_SUPPORTED status */ 00241 status = CAMERA_NOT_SUPPORTED; 00242 } 00243 00244 return status; 00245 } 00246 00247 00248 /** 00249 * @brief DeInitializes the camera. 00250 * @retval Camera status 00251 */ 00252 uint8_t BSP_CAMERA_DeInit(void) 00253 { 00254 hDcmiEval.Instance = DCMI; 00255 00256 HAL_DCMI_DeInit(&hDcmiEval); 00257 BSP_CAMERA_MspDeInit(&hDcmiEval, NULL); 00258 return CAMERA_OK; 00259 } 00260 00261 /** 00262 * @brief Starts the camera capture in continuous mode. 00263 * @param buff: pointer to the camera output buffer 00264 */ 00265 void BSP_CAMERA_ContinuousStart(uint8_t *buff) 00266 { 00267 /* Start the camera capture */ 00268 HAL_DCMI_Start_DMA(&hDcmiEval, DCMI_MODE_CONTINUOUS, (uint32_t)buff, GetSize(CameraCurrentResolution)); 00269 } 00270 00271 /** 00272 * @brief Starts the camera capture in snapshot mode. 00273 * @param buff: pointer to the camera output buffer 00274 */ 00275 void BSP_CAMERA_SnapshotStart(uint8_t *buff) 00276 { 00277 /* Start the camera capture */ 00278 HAL_DCMI_Start_DMA(&hDcmiEval, DCMI_MODE_SNAPSHOT, (uint32_t)buff, GetSize(CameraCurrentResolution)); 00279 } 00280 00281 /** 00282 * @brief Suspend the CAMERA capture 00283 */ 00284 void BSP_CAMERA_Suspend(void) 00285 { 00286 /* Suspend the Camera Capture */ 00287 HAL_DCMI_Suspend(&hDcmiEval); 00288 } 00289 00290 /** 00291 * @brief Resume the CAMERA capture 00292 */ 00293 void BSP_CAMERA_Resume(void) 00294 { 00295 /* Start the Camera Capture */ 00296 HAL_DCMI_Resume(&hDcmiEval); 00297 } 00298 00299 /** 00300 * @brief Stop the CAMERA capture 00301 * @retval Camera status 00302 */ 00303 uint8_t BSP_CAMERA_Stop(void) 00304 { 00305 uint8_t status = CAMERA_ERROR; 00306 00307 if(HAL_DCMI_Stop(&hDcmiEval) == HAL_OK) 00308 { 00309 status = CAMERA_OK; 00310 } 00311 00312 /* Set Camera in Power Down */ 00313 BSP_CAMERA_PwrDown(); 00314 00315 return status; 00316 } 00317 00318 /** 00319 * @brief CANERA hardware reset 00320 */ 00321 void BSP_CAMERA_HwReset(void) 00322 { 00323 /* Camera sensor RESET sequence */ 00324 BSP_IO_ConfigPin(RSTI_PIN, IO_MODE_OUTPUT); 00325 BSP_IO_ConfigPin(XSDN_PIN, IO_MODE_OUTPUT); 00326 00327 /* Assert the camera STANDBY pin (active high) */ 00328 BSP_IO_WritePin(XSDN_PIN, BSP_IO_PIN_SET); 00329 00330 /* Assert the camera RSTI pin (active low) */ 00331 BSP_IO_WritePin(RSTI_PIN, BSP_IO_PIN_RESET); 00332 00333 HAL_Delay(100); /* RST and XSDN signals asserted during 100ms */ 00334 00335 /* De-assert the camera STANDBY pin (active high) */ 00336 BSP_IO_WritePin(XSDN_PIN, BSP_IO_PIN_RESET); 00337 00338 HAL_Delay(3); /* RST de-asserted and XSDN asserted during 3ms */ 00339 00340 /* De-assert the camera RSTI pin (active low) */ 00341 BSP_IO_WritePin(RSTI_PIN, BSP_IO_PIN_SET); 00342 00343 HAL_Delay(6); /* RST de-asserted during 3ms */ 00344 } 00345 00346 /** 00347 * @brief CAMERA power down 00348 */ 00349 void BSP_CAMERA_PwrDown(void) 00350 { 00351 /* Camera power down sequence */ 00352 BSP_IO_ConfigPin(RSTI_PIN, IO_MODE_OUTPUT); 00353 BSP_IO_ConfigPin(XSDN_PIN, IO_MODE_OUTPUT); 00354 00355 /* De-assert the camera STANDBY pin (active high) */ 00356 BSP_IO_WritePin(XSDN_PIN, BSP_IO_PIN_RESET); 00357 00358 /* Assert the camera RSTI pin (active low) */ 00359 BSP_IO_WritePin(RSTI_PIN, BSP_IO_PIN_RESET); 00360 } 00361 00362 /** 00363 * @brief Configures the camera contrast and brightness. 00364 * @param contrast_level: Contrast level 00365 * This parameter can be one of the following values: 00366 * @arg CAMERA_CONTRAST_LEVEL4: for contrast +2 00367 * @arg CAMERA_CONTRAST_LEVEL3: for contrast +1 00368 * @arg CAMERA_CONTRAST_LEVEL2: for contrast 0 00369 * @arg CAMERA_CONTRAST_LEVEL1: for contrast -1 00370 * @arg CAMERA_CONTRAST_LEVEL0: for contrast -2 00371 * @param brightness_level: Contrast level 00372 * This parameter can be one of the following values: 00373 * @arg CAMERA_BRIGHTNESS_LEVEL4: for brightness +2 00374 * @arg CAMERA_BRIGHTNESS_LEVEL3: for brightness +1 00375 * @arg CAMERA_BRIGHTNESS_LEVEL2: for brightness 0 00376 * @arg CAMERA_BRIGHTNESS_LEVEL1: for brightness -1 00377 * @arg CAMERA_BRIGHTNESS_LEVEL0: for brightness -2 00378 */ 00379 void BSP_CAMERA_ContrastBrightnessConfig(uint32_t contrast_level, uint32_t brightness_level) 00380 { 00381 if(CameraDrv->Config != NULL) 00382 { 00383 CameraDrv->Config(CameraHwAddress, CAMERA_CONTRAST_BRIGHTNESS, contrast_level, brightness_level); 00384 } 00385 } 00386 00387 /** 00388 * @brief Configures the camera white balance. 00389 * @param Mode: black_white mode 00390 * This parameter can be one of the following values: 00391 * @arg CAMERA_BLACK_WHITE_BW 00392 * @arg CAMERA_BLACK_WHITE_NEGATIVE 00393 * @arg CAMERA_BLACK_WHITE_BW_NEGATIVE 00394 * @arg CAMERA_BLACK_WHITE_NORMAL 00395 */ 00396 void BSP_CAMERA_BlackWhiteConfig(uint32_t Mode) 00397 { 00398 if(CameraDrv->Config != NULL) 00399 { 00400 CameraDrv->Config(CameraHwAddress, CAMERA_BLACK_WHITE, Mode, 0); 00401 } 00402 } 00403 00404 /** 00405 * @brief Configures the camera color effect. 00406 * @param Effect: Color effect 00407 * This parameter can be one of the following values: 00408 * @arg CAMERA_COLOR_EFFECT_NONE 00409 * @arg CAMERA_COLOR_EFFECT_BLUE 00410 * @arg CAMERA_COLOR_EFFECT_GREEN 00411 * @arg CAMERA_COLOR_EFFECT_RED 00412 * @arg CAMERA_COLOR_EFFECT_ANTIQUE 00413 */ 00414 void BSP_CAMERA_ColorEffectConfig(uint32_t Effect) 00415 { 00416 if(CameraDrv->Config != NULL) 00417 { 00418 CameraDrv->Config(CameraHwAddress, CAMERA_COLOR_EFFECT, Effect, 0); 00419 } 00420 } 00421 00422 /** 00423 * @brief Get the capture size in pixels unit. 00424 * @param Resolution: the current resolution. 00425 * @retval capture size in pixels unit. 00426 */ 00427 static uint32_t GetSize(uint32_t Resolution) 00428 { 00429 uint32_t size = 0; 00430 00431 /* Get capture size */ 00432 switch (Resolution) 00433 { 00434 case CAMERA_R160x120: 00435 { 00436 size = 0x2580; 00437 } 00438 break; 00439 case CAMERA_R320x240: 00440 { 00441 size = 0x9600; 00442 } 00443 break; 00444 case CAMERA_R480x272: 00445 { 00446 size = 0xFF00; 00447 } 00448 break; 00449 case CAMERA_R640x480: 00450 { 00451 size = 0x25800; 00452 } 00453 break; 00454 default: 00455 { 00456 break; 00457 } 00458 } 00459 00460 return size; 00461 } 00462 00463 /** 00464 * @brief Initializes the DCMI MSP. 00465 * @param hdcmi: HDMI handle 00466 * @param Params : pointer on additional configuration parameters, can be NULL. 00467 */ 00468 __weak void BSP_CAMERA_MspInit(DCMI_HandleTypeDef *hdcmi, void *Params) 00469 { 00470 static DMA_HandleTypeDef hdma_eval; 00471 GPIO_InitTypeDef gpio_init_structure; 00472 00473 /*** Enable peripherals and GPIO clocks ***/ 00474 /* Enable DCMI clock */ 00475 __HAL_RCC_DCMI_CLK_ENABLE(); 00476 00477 /* Enable DMA2 clock */ 00478 __HAL_RCC_DMA2_CLK_ENABLE(); 00479 00480 /* Enable GPIO clocks */ 00481 __HAL_RCC_GPIOA_CLK_ENABLE(); 00482 __HAL_RCC_GPIOB_CLK_ENABLE(); 00483 __HAL_RCC_GPIOC_CLK_ENABLE(); 00484 __HAL_RCC_GPIOD_CLK_ENABLE(); 00485 __HAL_RCC_GPIOE_CLK_ENABLE(); 00486 00487 /*** Configure the GPIO ***/ 00488 /* Configure DCMI GPIO as alternate function */ 00489 gpio_init_structure.Pin = GPIO_PIN_4 | GPIO_PIN_6; 00490 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00491 gpio_init_structure.Pull = GPIO_PULLUP; 00492 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00493 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00494 HAL_GPIO_Init(GPIOA, &gpio_init_structure); 00495 00496 gpio_init_structure.Pin = GPIO_PIN_7; 00497 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00498 gpio_init_structure.Pull = GPIO_PULLUP; 00499 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00500 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00501 HAL_GPIO_Init(GPIOB, &gpio_init_structure); 00502 00503 gpio_init_structure.Pin = GPIO_PIN_6 | GPIO_PIN_7 | GPIO_PIN_8 |\ 00504 GPIO_PIN_9 | GPIO_PIN_11; 00505 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00506 gpio_init_structure.Pull = GPIO_PULLUP; 00507 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00508 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00509 HAL_GPIO_Init(GPIOC, &gpio_init_structure); 00510 00511 gpio_init_structure.Pin = GPIO_PIN_3 | GPIO_PIN_6; 00512 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00513 gpio_init_structure.Pull = GPIO_PULLUP; 00514 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00515 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00516 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00517 00518 gpio_init_structure.Pin = GPIO_PIN_5 | GPIO_PIN_6; 00519 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00520 gpio_init_structure.Pull = GPIO_PULLUP; 00521 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00522 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00523 HAL_GPIO_Init(GPIOE, &gpio_init_structure); 00524 00525 /*** Configure the DMA ***/ 00526 /* Set the parameters to be configured */ 00527 hdma_eval.Init.Channel = DMA_CHANNEL_1; 00528 hdma_eval.Init.Direction = DMA_PERIPH_TO_MEMORY; 00529 hdma_eval.Init.PeriphInc = DMA_PINC_DISABLE; 00530 hdma_eval.Init.MemInc = DMA_MINC_ENABLE; 00531 hdma_eval.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00532 hdma_eval.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00533 hdma_eval.Init.Mode = DMA_CIRCULAR; 00534 hdma_eval.Init.Priority = DMA_PRIORITY_HIGH; 00535 hdma_eval.Init.FIFOMode = DMA_FIFOMODE_DISABLE; 00536 hdma_eval.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00537 hdma_eval.Init.MemBurst = DMA_MBURST_SINGLE; 00538 hdma_eval.Init.PeriphBurst = DMA_PBURST_SINGLE; 00539 00540 hdma_eval.Instance = DMA2_Stream1; 00541 00542 /* Associate the initialized DMA handle to the DCMI handle */ 00543 __HAL_LINKDMA(hdcmi, DMA_Handle, hdma_eval); 00544 00545 /*** Configure the NVIC for DCMI and DMA ***/ 00546 /* NVIC configuration for DCMI transfer complete interrupt */ 00547 HAL_NVIC_SetPriority(DCMI_IRQn, 0x0F, 0); 00548 HAL_NVIC_EnableIRQ(DCMI_IRQn); 00549 00550 /* NVIC configuration for DMA2D transfer complete interrupt */ 00551 HAL_NVIC_SetPriority(DMA2_Stream1_IRQn, 0x0F, 0); 00552 HAL_NVIC_EnableIRQ(DMA2_Stream1_IRQn); 00553 00554 /* Configure the DMA stream */ 00555 HAL_DMA_Init(hdcmi->DMA_Handle); 00556 } 00557 00558 /** 00559 * @brief DeInitializes the DCMI MSP. 00560 * @param hdcmi: HDMI handle 00561 * @param Params : pointer on additional configuration parameters, can be NULL. 00562 */ 00563 __weak void BSP_CAMERA_MspDeInit(DCMI_HandleTypeDef *hdcmi, void *Params) 00564 { 00565 /* Disable NVIC for DCMI transfer complete interrupt */ 00566 HAL_NVIC_DisableIRQ(DCMI_IRQn); 00567 00568 /* Disable NVIC for DMA2 transfer complete interrupt */ 00569 HAL_NVIC_DisableIRQ(DMA2_Stream1_IRQn); 00570 00571 /* Configure the DMA stream */ 00572 HAL_DMA_DeInit(hdcmi->DMA_Handle); 00573 00574 /* Disable DCMI clock */ 00575 __HAL_RCC_DCMI_CLK_DISABLE(); 00576 00577 /* GPIO pins clock and DMA clock can be shut down in the application 00578 by surcharging this __weak function */ 00579 } 00580 00581 /** 00582 * @brief Line event callback 00583 * @param hdcmi: pointer to the DCMI handle 00584 */ 00585 void HAL_DCMI_LineEventCallback(DCMI_HandleTypeDef *hdcmi) 00586 { 00587 BSP_CAMERA_LineEventCallback(); 00588 } 00589 00590 /** 00591 * @brief Line Event callback. 00592 */ 00593 __weak void BSP_CAMERA_LineEventCallback(void) 00594 { 00595 /* NOTE : This function Should not be modified, when the callback is needed, 00596 the HAL_DCMI_LineEventCallback could be implemented in the user file 00597 */ 00598 } 00599 00600 /** 00601 * @brief VSYNC event callback 00602 * @param hdcmi: pointer to the DCMI handle 00603 */ 00604 void HAL_DCMI_VsyncEventCallback(DCMI_HandleTypeDef *hdcmi) 00605 { 00606 BSP_CAMERA_VsyncEventCallback(); 00607 } 00608 00609 /** 00610 * @brief VSYNC Event callback. 00611 */ 00612 __weak void BSP_CAMERA_VsyncEventCallback(void) 00613 { 00614 /* NOTE : This function Should not be modified, when the callback is needed, 00615 the HAL_DCMI_VsyncEventCallback could be implemented in the user file 00616 */ 00617 } 00618 00619 /** 00620 * @brief Frame event callback 00621 * @param hdcmi: pointer to the DCMI handle 00622 */ 00623 void HAL_DCMI_FrameEventCallback(DCMI_HandleTypeDef *hdcmi) 00624 { 00625 BSP_CAMERA_FrameEventCallback(); 00626 } 00627 00628 /** 00629 * @brief Frame Event callback. 00630 */ 00631 __weak void BSP_CAMERA_FrameEventCallback(void) 00632 { 00633 /* NOTE : This function Should not be modified, when the callback is needed, 00634 the HAL_DCMI_FrameEventCallback could be implemented in the user file 00635 */ 00636 } 00637 00638 /** 00639 * @brief Error callback 00640 * @param hdcmi: pointer to the DCMI handle 00641 */ 00642 void HAL_DCMI_ErrorCallback(DCMI_HandleTypeDef *hdcmi) 00643 { 00644 BSP_CAMERA_ErrorCallback(); 00645 } 00646 00647 /** 00648 * @brief Error callback. 00649 */ 00650 __weak void BSP_CAMERA_ErrorCallback(void) 00651 { 00652 /* NOTE : This function Should not be modified, when the callback is needed, 00653 the HAL_DCMI_ErrorCallback could be implemented in the user file 00654 */ 00655 } 00656 00657 /** 00658 * @} 00659 */ 00660 00661 /** 00662 * @} 00663 */ 00664 00665 /** 00666 * @} 00667 */ 00668 00669 /** 00670 * @} 00671 */ 00672 00673 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu May 25 2017 11:03:11 for STM32769I_EVAL BSP User Manual by
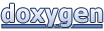