STM32769I_EVAL BSP User Manual
|
stm32f769i_eval.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f769i_eval.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of firmware functions to manage LEDs, 00006 * push-buttons and COM ports available on STM32F769I-EVAL 00007 * evaluation board(MB1219) from STMicroelectronics. 00008 * 00009 @verbatim 00010 This driver requires the stm32f769i_eval_io.c/.h files to manage the 00011 IO module resources mapped on the MFX IO expander. 00012 These resources are mainly LEDs, Joystick push buttons, SD detect pin, 00013 USB OTG power switch/over current drive pins, Camera plug pin, Audio 00014 INT pin 00015 @endverbatim 00016 ****************************************************************************** 00017 * @attention 00018 * 00019 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00020 * 00021 * Redistribution and use in source and binary forms, with or without modification, 00022 * are permitted provided that the following conditions are met: 00023 * 1. Redistributions of source code must retain the above copyright notice, 00024 * this list of conditions and the following disclaimer. 00025 * 2. Redistributions in binary form must reproduce the above copyright notice, 00026 * this list of conditions and the following disclaimer in the documentation 00027 * and/or other materials provided with the distribution. 00028 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00029 * may be used to endorse or promote products derived from this software 00030 * without specific prior written permission. 00031 * 00032 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00033 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00034 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00035 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00036 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00037 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00038 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00039 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00040 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00041 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00042 * 00043 ****************************************************************************** 00044 */ 00045 00046 /* Dependencies 00047 - stm32f769i_eval_io.c 00048 - stm32f7xx_hal_cortex.c 00049 - stm32f7xx_hal_gpio.c 00050 - stm32f7xx_hal_uart.c 00051 - stm32f7xx_hal_i2c.c 00052 - stm32f7xx_hal_adc.c 00053 EndDependencies */ 00054 00055 /* Includes ------------------------------------------------------------------*/ 00056 #include "stm32f769i_eval.h" 00057 #if defined(USE_IOEXPANDER) 00058 #include "stm32f769i_eval_io.h" 00059 #endif /* USE_IOEXPANDER */ 00060 00061 /** @addtogroup BSP 00062 * @{ 00063 */ 00064 00065 /** @addtogroup STM32F769I_EVAL 00066 * @{ 00067 */ 00068 00069 /** @defgroup STM32F769I_EVAL_LOW_LEVEL STM32F769I_EVAL LOW LEVEL 00070 * @{ 00071 */ 00072 00073 /** @defgroup STM32F769I_EVAL_LOW_LEVEL_Private_TypesDefinitions STM32F769I-EVAL LOW LEVEL Private Types Definitions 00074 * @{ 00075 */ 00076 /** 00077 * @} 00078 */ 00079 00080 /** @defgroup STM32F769I_EVAL_LOW_LEVEL_Private_Defines STM32F769I-EVAL LOW LEVEL Private Defines 00081 * @{ 00082 */ 00083 /** 00084 * @brief STM32F769I EVAL BSP Driver version number V2.0.1 00085 */ 00086 #define __STM32F769I_EVAL_BSP_VERSION_MAIN (0x02) /*!< [31:24] main version */ 00087 #define __STM32F769I_EVAL_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00088 #define __STM32F769I_EVAL_BSP_VERSION_SUB2 (0x01) /*!< [15:8] sub2 version */ 00089 #define __STM32F769I_EVAL_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00090 #define __STM32F769I_EVAL_BSP_VERSION ((__STM32F769I_EVAL_BSP_VERSION_MAIN << 24)\ 00091 |(__STM32F769I_EVAL_BSP_VERSION_SUB1 << 16)\ 00092 |(__STM32F769I_EVAL_BSP_VERSION_SUB2 << 8 )\ 00093 |(__STM32F769I_EVAL_BSP_VERSION_RC)) 00094 /** 00095 * @} 00096 */ 00097 00098 /** @defgroup STM32F769I_EVAL_LOW_LEVEL_Private_Macros STM32F769I-EVAL LOW LEVEL Private Macros 00099 * @{ 00100 */ 00101 /** 00102 * @} 00103 */ 00104 00105 /** @defgroup STM32F769I_EVAL_LOW_LEVEL_Private_Variables STM32F769I-EVAL LOW LEVEL Private Variables 00106 * @{ 00107 */ 00108 GPIO_TypeDef* GPIO_PORT[LEDn] = {LED1_GPIO_PORT, 00109 LED2_GPIO_PORT, 00110 LED3_GPIO_PORT, 00111 LED4_GPIO_PORT}; 00112 00113 const uint32_t GPIO_PIN[LEDn] = {LED1_PIN, 00114 LED2_PIN, 00115 LED3_PIN, 00116 LED4_PIN}; 00117 00118 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {WAKEUP_BUTTON_GPIO_PORT, 00119 TAMPER_BUTTON_GPIO_PORT, 00120 KEY_BUTTON_GPIO_PORT}; 00121 00122 const uint16_t BUTTON_PIN[BUTTONn] = {WAKEUP_BUTTON_PIN, 00123 TAMPER_BUTTON_PIN, 00124 KEY_BUTTON_PIN}; 00125 00126 const uint16_t BUTTON_IRQn[BUTTONn] = {WAKEUP_BUTTON_EXTI_IRQn, 00127 TAMPER_BUTTON_EXTI_IRQn, 00128 KEY_BUTTON_EXTI_IRQn}; 00129 00130 USART_TypeDef* COM_USART[COMn] = {EVAL_COM1}; 00131 00132 GPIO_TypeDef* COM_TX_PORT[COMn] = {EVAL_COM1_TX_GPIO_PORT}; 00133 00134 GPIO_TypeDef* COM_RX_PORT[COMn] = {EVAL_COM1_RX_GPIO_PORT}; 00135 00136 const uint16_t COM_TX_PIN[COMn] = {EVAL_COM1_TX_PIN}; 00137 00138 const uint16_t COM_RX_PIN[COMn] = {EVAL_COM1_RX_PIN}; 00139 00140 const uint16_t COM_TX_AF[COMn] = {EVAL_COM1_TX_AF}; 00141 00142 const uint16_t COM_RX_AF[COMn] = {EVAL_COM1_RX_AF}; 00143 00144 static I2C_HandleTypeDef hEvalI2c; 00145 static ADC_HandleTypeDef hEvalADC; 00146 00147 /** 00148 * @} 00149 */ 00150 00151 /** @defgroup STM32F769I_EVAL_LOW_LEVEL_Private_FunctionPrototypes STM32F769I_EVAL LOW LEVEL Private Function Prototypes 00152 * @{ 00153 */ 00154 static void I2Cx_MspInit(void); 00155 static void I2Cx_Init(void); 00156 static void I2Cx_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00157 static uint8_t I2Cx_Read(uint8_t Addr, uint8_t Reg); 00158 static HAL_StatusTypeDef I2Cx_ReadMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00159 static HAL_StatusTypeDef I2Cx_WriteMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00160 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00161 static void I2Cx_Error(uint8_t Addr); 00162 00163 #if defined(USE_IOEXPANDER) 00164 /* IOExpander IO functions */ 00165 void IOE_Init(void); 00166 void IOE_ITConfig(void); 00167 void IOE_Delay(uint32_t Delay); 00168 void IOE_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00169 uint8_t IOE_Read(uint8_t Addr, uint8_t Reg); 00170 uint16_t IOE_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00171 void IOE_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00172 00173 /* MFX IO functions */ 00174 void MFX_IO_Init(void); 00175 void MFX_IO_DeInit(void); 00176 void MFX_IO_ITConfig(void); 00177 void MFX_IO_Delay(uint32_t Delay); 00178 void MFX_IO_Write(uint16_t Addr, uint8_t Reg, uint8_t Value); 00179 uint8_t MFX_IO_Read(uint16_t Addr, uint8_t Reg); 00180 uint16_t MFX_IO_ReadMultiple(uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00181 void MFX_IO_Wakeup(void); 00182 void MFX_IO_EnableWakeupPin(void); 00183 #endif /* USE_IOEXPANDER */ 00184 00185 /* AUDIO IO functions */ 00186 void AUDIO_IO_Init(void); 00187 void AUDIO_IO_DeInit(void); 00188 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value); 00189 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg); 00190 void AUDIO_IO_Delay(uint32_t Delay); 00191 00192 /* CAMERA IO functions */ 00193 void CAMERA_IO_Init(void); 00194 void CAMERA_Delay(uint32_t Delay); 00195 void CAMERA_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value); 00196 uint16_t CAMERA_IO_Read(uint8_t Addr, uint16_t Reg); 00197 00198 /* I2C EEPROM IO function */ 00199 void EEPROM_IO_Init(void); 00200 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00201 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00202 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00203 00204 /* TouchScreen (TS) IO functions */ 00205 void TS_IO_Init(void); 00206 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00207 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg); 00208 uint16_t TS_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00209 void TS_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00210 void TS_IO_Delay(uint32_t Delay); 00211 00212 #if defined(USE_LCD_HDMI) 00213 /* HDMI IO functions */ 00214 void HDMI_IO_Init(void); 00215 void HDMI_IO_Delay(uint32_t Delay); 00216 void HDMI_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00217 uint8_t HDMI_IO_Read(uint8_t Addr, uint8_t Reg); 00218 #endif /* USE_LCD_HDMI */ 00219 00220 void OTM8009A_IO_Delay(uint32_t Delay); 00221 /** 00222 * @} 00223 */ 00224 00225 /** @defgroup STM32F769I_EVAL_LOW_LEVEL_Private_Functions STM32F769I_EVAL LOW LEVEL Private Functions 00226 * @{ 00227 */ 00228 00229 /** 00230 * @brief This method returns the STM32F769I EVAL BSP Driver revision 00231 * @retval version: 0xXYZR (8bits for each decimal, R for RC) 00232 */ 00233 uint32_t BSP_GetVersion(void) 00234 { 00235 return __STM32F769I_EVAL_BSP_VERSION; 00236 } 00237 00238 /** 00239 * @brief Configures LED on GPIO and/or on MFX. 00240 * @param Led: LED to be configured. 00241 * This parameter can be one of the following values: 00242 * @arg LED1 00243 * @arg LED2 00244 * @arg LED3 00245 * @arg LED4 00246 * @retval None 00247 */ 00248 void BSP_LED_Init(Led_TypeDef Led) 00249 { 00250 GPIO_InitTypeDef GPIO_InitStruct; 00251 00252 /* Enable the GPIO_LED clock */ 00253 LEDx_GPIO_CLK_ENABLE(Led); 00254 00255 /* Configure the GPIO_LED pin */ 00256 GPIO_InitStruct.Pin = GPIO_PIN[Led]; 00257 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00258 GPIO_InitStruct.Pull = GPIO_PULLUP; 00259 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00260 00261 HAL_GPIO_Init(GPIO_PORT[Led], &GPIO_InitStruct); 00262 00263 /* By default, turn off LED */ 00264 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_SET); 00265 } 00266 00267 00268 /** 00269 * @brief DeInit LEDs. 00270 * @param Led: LED to be configured. 00271 * This parameter can be one of the following values: 00272 * @arg LED1 00273 * @arg LED2 00274 * @arg LED3 00275 * @arg LED4 00276 * @note Led DeInit does not disable the GPIO clock nor disable the Mfx 00277 * @retval None 00278 */ 00279 void BSP_LED_DeInit(Led_TypeDef Led) 00280 { 00281 /* Turn off LED */ 00282 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00283 /* Configure the GPIO_LED pin */ 00284 HAL_GPIO_DeInit(GPIO_PORT[Led], GPIO_PIN[Led]); 00285 } 00286 00287 /** 00288 * @brief Turns selected LED On. 00289 * @param Led: LED to be set on 00290 * This parameter can be one of the following values: 00291 * @arg LED1 00292 * @arg LED2 00293 * @arg LED3 00294 * @arg LED4 00295 * @retval None 00296 */ 00297 void BSP_LED_On(Led_TypeDef Led) 00298 { 00299 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00300 } 00301 00302 /** 00303 * @brief Turns selected LED Off. 00304 * @param Led: LED to be set off 00305 * This parameter can be one of the following values: 00306 * @arg LED1 00307 * @arg LED2 00308 * @arg LED3 00309 * @arg LED4 00310 * @retval None 00311 */ 00312 void BSP_LED_Off(Led_TypeDef Led) 00313 { 00314 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_SET); 00315 } 00316 00317 /** 00318 * @brief Toggles the selected LED. 00319 * @param Led: LED to be toggled 00320 * This parameter can be one of the following values: 00321 * @arg LED1 00322 * @arg LED2 00323 * @arg LED3 00324 * @arg LED4 00325 * @retval None 00326 */ 00327 void BSP_LED_Toggle(Led_TypeDef Led) 00328 { 00329 HAL_GPIO_TogglePin(GPIO_PORT[Led], GPIO_PIN[Led]); 00330 } 00331 00332 /** 00333 * @brief Configures button GPIO and EXTI Line. 00334 * @param Button: Button to be configured 00335 * This parameter can be one of the following values: 00336 * @arg BUTTON_WAKEUP: Wakeup Push Button 00337 * @arg BUTTON_TAMPER: Tamper Push Button 00338 * @arg BUTTON_KEY: Key Push Button 00339 * @param ButtonMode: Button mode 00340 * This parameter can be one of the following values: 00341 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00342 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00343 * with interrupt generation capability 00344 * @note On STM32F769I-EVAL evaluation board, the three buttons (Wakeup, Tamper 00345 * and key buttons) are mapped on the same push button named "Wakeup/Tamper" 00346 * on the board serigraphy. 00347 * @retval None 00348 */ 00349 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) 00350 { 00351 GPIO_InitTypeDef GPIO_InitStruct; 00352 00353 /* Enable the BUTTON clock */ 00354 BUTTONx_GPIO_CLK_ENABLE(Button); 00355 00356 if(ButtonMode == BUTTON_MODE_GPIO) 00357 { 00358 /* Configure Button pin as input */ 00359 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00360 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00361 GPIO_InitStruct.Pull = GPIO_NOPULL; 00362 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00363 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00364 } 00365 00366 if(ButtonMode == BUTTON_MODE_EXTI) 00367 { 00368 /* Configure Button pin as input with External interrupt */ 00369 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00370 GPIO_InitStruct.Pull = GPIO_NOPULL; 00371 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00372 00373 if(Button != BUTTON_WAKEUP) 00374 { 00375 GPIO_InitStruct.Mode = GPIO_MODE_IT_FALLING; 00376 } 00377 else 00378 { 00379 GPIO_InitStruct.Mode = GPIO_MODE_IT_RISING; 00380 } 00381 00382 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00383 00384 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00385 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x00); 00386 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00387 } 00388 } 00389 00390 /** 00391 * @brief Push Button DeInit. 00392 * @param Button: Button to be configured 00393 * This parameter can be one of the following values: 00394 * @arg BUTTON_WAKEUP: Wakeup Push Button 00395 * @arg BUTTON_TAMPER: Tamper Push Button 00396 * @arg BUTTON_KEY: Key Push Button 00397 * @note On STM32F769I-EVAL evaluation board, the three buttons (Wakeup, Tamper 00398 * and key buttons) are mapped on the same push button named "Wakeup/Tamper" 00399 * on the board serigraphy. 00400 * @note PB DeInit does not disable the GPIO clock 00401 * @retval None 00402 */ 00403 void BSP_PB_DeInit(Button_TypeDef Button) 00404 { 00405 GPIO_InitTypeDef gpio_init_structure; 00406 00407 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00408 HAL_NVIC_DisableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00409 HAL_GPIO_DeInit(BUTTON_PORT[Button], gpio_init_structure.Pin); 00410 } 00411 00412 00413 /** 00414 * @brief Returns the selected button state. 00415 * @param Button: Button to be checked 00416 * This parameter can be one of the following values: 00417 * @arg BUTTON_WAKEUP: Wakeup Push Button 00418 * @arg BUTTON_TAMPER: Tamper Push Button 00419 * @arg BUTTON_KEY: Key Push Button 00420 * @note On STM32F769I-EVAL evaluation board, the three buttons (Wakeup, Tamper 00421 * and key buttons) are mapped on the same push button named "Wakeup/Tamper" 00422 * on the board serigraphy. 00423 * @retval The Button GPIO pin value 00424 */ 00425 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00426 { 00427 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00428 } 00429 00430 /** 00431 * @brief Configures COM port. 00432 * @param COM: COM port to be configured. 00433 * This parameter can be one of the following values: 00434 * @arg COM1 00435 * @arg COM2 00436 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00437 * configuration information for the specified USART peripheral. 00438 * @retval None 00439 */ 00440 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef *huart) 00441 { 00442 GPIO_InitTypeDef gpio_init_structure; 00443 00444 /* Enable GPIO clock */ 00445 EVAL_COMx_TX_GPIO_CLK_ENABLE(COM); 00446 EVAL_COMx_RX_GPIO_CLK_ENABLE(COM); 00447 00448 /* Enable USART clock */ 00449 EVAL_COMx_CLK_ENABLE(COM); 00450 00451 /* Configure USART Tx as alternate function */ 00452 gpio_init_structure.Pin = COM_TX_PIN[COM]; 00453 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00454 gpio_init_structure.Speed = GPIO_SPEED_FREQ_HIGH; 00455 gpio_init_structure.Pull = GPIO_PULLUP; 00456 gpio_init_structure.Alternate = COM_TX_AF[COM]; 00457 HAL_GPIO_Init(COM_TX_PORT[COM], &gpio_init_structure); 00458 00459 /* Configure USART Rx as alternate function */ 00460 gpio_init_structure.Pin = COM_RX_PIN[COM]; 00461 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00462 gpio_init_structure.Alternate = COM_RX_AF[COM]; 00463 HAL_GPIO_Init(COM_RX_PORT[COM], &gpio_init_structure); 00464 00465 /* USART configuration */ 00466 huart->Instance = COM_USART[COM]; 00467 HAL_UART_Init(huart); 00468 } 00469 00470 /** 00471 * @brief DeInit COM port. 00472 * @param COM: COM port to be configured. 00473 * This parameter can be one of the following values: 00474 * @arg COM1 00475 * @arg COM2 00476 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00477 * configuration information for the specified USART peripheral. 00478 * @retval None 00479 */ 00480 void BSP_COM_DeInit(COM_TypeDef COM, UART_HandleTypeDef *huart) 00481 { 00482 /* USART configuration */ 00483 huart->Instance = COM_USART[COM]; 00484 HAL_UART_DeInit(huart); 00485 00486 /* Enable USART clock */ 00487 EVAL_COMx_CLK_DISABLE(COM); 00488 00489 /* DeInit GPIO pins can be done in the application 00490 (by surcharging this __weak function) */ 00491 00492 /* GPIO pins clock, DMA clock can be shut down in the application 00493 by surcharging this __weak function */ 00494 } 00495 00496 /** 00497 * @brief Init Potentiometer. 00498 * @retval None 00499 */ 00500 void BSP_POTENTIOMETER_Init(void) 00501 { 00502 GPIO_InitTypeDef GPIO_InitStruct; 00503 ADC_ChannelConfTypeDef ADC_Config; 00504 00505 /* ADC an GPIO Periph clock enable */ 00506 ADCx_CLK_ENABLE(); 00507 ADCx_CHANNEL_GPIO_CLK_ENABLE(); 00508 00509 /* ADC Channel GPIO pin configuration */ 00510 GPIO_InitStruct.Pin = ADCx_CHANNEL_PIN; 00511 GPIO_InitStruct.Mode = GPIO_MODE_ANALOG; 00512 GPIO_InitStruct.Pull = GPIO_NOPULL; 00513 HAL_GPIO_Init(ADCx_CHANNEL_GPIO_PORT, &GPIO_InitStruct); 00514 00515 /* Configure the ADC peripheral */ 00516 hEvalADC.Instance = ADCx; 00517 00518 HAL_ADC_DeInit(&hEvalADC); 00519 00520 hEvalADC.Init.ClockPrescaler = ADC_CLOCKPRESCALER_PCLK_DIV4; /* Asynchronous clock mode, input ADC clock not divided */ 00521 hEvalADC.Init.Resolution = ADC_RESOLUTION_12B; /* 12-bit resolution for converted data */ 00522 hEvalADC.Init.DataAlign = ADC_DATAALIGN_RIGHT; /* Right-alignment for converted data */ 00523 hEvalADC.Init.ScanConvMode = DISABLE; /* Sequencer disabled (ADC conversion on only 1 channel: channel set on rank 1) */ 00524 hEvalADC.Init.EOCSelection = DISABLE; /* EOC flag picked-up to indicate conversion end */ 00525 hEvalADC.Init.ContinuousConvMode = DISABLE; /* Continuous mode disabled to have only 1 conversion at each conversion trig */ 00526 hEvalADC.Init.NbrOfConversion = 1; /* Parameter discarded because sequencer is disabled */ 00527 hEvalADC.Init.DiscontinuousConvMode = DISABLE; /* Parameter discarded because sequencer is disabled */ 00528 hEvalADC.Init.NbrOfDiscConversion = 0; /* Parameter discarded because sequencer is disabled */ 00529 hEvalADC.Init.ExternalTrigConv = ADC_EXTERNALTRIGCONV_T1_CC1; /* Software start to trig the 1st conversion manually, without external event */ 00530 hEvalADC.Init.ExternalTrigConvEdge = ADC_EXTERNALTRIGCONVEDGE_NONE; /* Parameter discarded because software trigger chosen */ 00531 hEvalADC.Init.DMAContinuousRequests = DISABLE; /* DMA one-shot mode selected */ 00532 00533 00534 HAL_ADC_Init(&hEvalADC); 00535 00536 /* Configure ADC regular channel */ 00537 ADC_Config.Channel = ADCx_CHANNEL; /* Sampled channel number */ 00538 ADC_Config.Rank = 1; /* Rank of sampled channel number ADCx_CHANNEL */ 00539 ADC_Config.SamplingTime = ADC_SAMPLETIME_3CYCLES; /* Sampling time (number of clock cycles unit) */ 00540 ADC_Config.Offset = 0; /* Parameter discarded because offset correction is disabled */ 00541 00542 HAL_ADC_ConfigChannel(&hEvalADC, &ADC_Config); 00543 } 00544 00545 /** 00546 * @brief Get Potentiometer level in 12 bits. 00547 * @retval Potentiometer level(0..0xFFF) / 0xFFFFFFFF : Error 00548 */ 00549 uint32_t BSP_POTENTIOMETER_GetLevel(void) 00550 { 00551 if(HAL_ADC_Start(&hEvalADC) == HAL_OK) 00552 { 00553 if(HAL_ADC_PollForConversion(&hEvalADC, ADCx_POLL_TIMEOUT)== HAL_OK) 00554 { 00555 return (HAL_ADC_GetValue(&hEvalADC)); 00556 } 00557 } 00558 return 0xFFFFFFFF; 00559 } 00560 00561 #if defined(USE_IOEXPANDER) 00562 /** 00563 * @brief Configures joystick GPIO and EXTI modes. 00564 * @param JoyMode: Button mode. 00565 * This parameter can be one of the following values: 00566 * @arg JOY_MODE_GPIO: Joystick pins will be used as simple IOs 00567 * @arg JOY_MODE_EXTI: Joystick pins will be connected to EXTI line 00568 * with interrupt generation capability 00569 * @retval IO_OK: if all initializations are OK. Other value if error. 00570 */ 00571 uint8_t BSP_JOY_Init(JOYMode_TypeDef JoyMode) 00572 { 00573 uint8_t ret = 0; 00574 00575 /* Initialize the IO functionalities */ 00576 ret = BSP_IO_Init(); 00577 00578 /* Configure joystick pins in IT mode */ 00579 if(JoyMode == JOY_MODE_EXTI) 00580 { 00581 /* Configure IO interrupt acquisition mode */ 00582 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_IT_LOW_LEVEL_PU); 00583 } 00584 else 00585 { 00586 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_INPUT_PU); 00587 } 00588 00589 return ret; 00590 } 00591 00592 00593 /** 00594 * @brief DeInit joystick GPIOs. 00595 * @note JOY DeInit does not disable the MFX, just set the MFX pins in Off mode 00596 * @retval None. 00597 */ 00598 void BSP_JOY_DeInit() 00599 { 00600 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_OFF); 00601 } 00602 00603 /** 00604 * @brief Returns the current joystick status. 00605 * @retval Code of the joystick key pressed 00606 * This code can be one of the following values: 00607 * @arg JOY_NONE 00608 * @arg JOY_SEL 00609 * @arg JOY_DOWN 00610 * @arg JOY_LEFT 00611 * @arg JOY_RIGHT 00612 * @arg JOY_UP 00613 */ 00614 JOYState_TypeDef BSP_JOY_GetState(void) 00615 { 00616 uint16_t pin_status = 0; 00617 00618 /* Read the status joystick pins */ 00619 pin_status = BSP_IO_ReadPin(JOY_ALL_PINS); 00620 00621 /* Check the pressed keys */ 00622 if((pin_status & JOY_NONE_PIN) == JOY_NONE) 00623 { 00624 return(JOYState_TypeDef) JOY_NONE; 00625 } 00626 else if(!(pin_status & JOY_SEL_PIN)) 00627 { 00628 return(JOYState_TypeDef) JOY_SEL; 00629 } 00630 else if(!(pin_status & JOY_DOWN_PIN)) 00631 { 00632 return(JOYState_TypeDef) JOY_DOWN; 00633 } 00634 else if(!(pin_status & JOY_LEFT_PIN)) 00635 { 00636 return(JOYState_TypeDef) JOY_LEFT; 00637 } 00638 else if(!(pin_status & JOY_RIGHT_PIN)) 00639 { 00640 return(JOYState_TypeDef) JOY_RIGHT; 00641 } 00642 else if(!(pin_status & JOY_UP_PIN)) 00643 { 00644 return(JOYState_TypeDef) JOY_UP; 00645 } 00646 else 00647 { 00648 return(JOYState_TypeDef) JOY_NONE; 00649 } 00650 } 00651 00652 #endif /* USE_IOEXPANDER */ 00653 00654 /******************************************************************************* 00655 BUS OPERATIONS 00656 *******************************************************************************/ 00657 00658 /******************************* I2C Routines *********************************/ 00659 /** 00660 * @brief Initializes I2C MSP. 00661 * @retval None 00662 */ 00663 static void I2Cx_MspInit(void) 00664 { 00665 GPIO_InitTypeDef gpio_init_structure; 00666 00667 /*** Configure the GPIOs ***/ 00668 /* Enable GPIO clock */ 00669 EVAL_I2Cx_SCL_SDA_GPIO_CLK_ENABLE(); 00670 00671 /* Configure I2C Tx as alternate function */ 00672 gpio_init_structure.Pin = EVAL_I2Cx_SCL_PIN; 00673 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00674 gpio_init_structure.Pull = GPIO_NOPULL; 00675 gpio_init_structure.Speed = GPIO_SPEED_FREQ_HIGH; 00676 gpio_init_structure.Alternate = EVAL_I2Cx_SCL_SDA_AF; 00677 HAL_GPIO_Init(EVAL_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00678 00679 /* Configure I2C Rx as alternate function */ 00680 gpio_init_structure.Pin = EVAL_I2Cx_SDA_PIN; 00681 HAL_GPIO_Init(EVAL_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00682 00683 /*** Configure the I2C peripheral ***/ 00684 /* Enable I2C clock */ 00685 EVAL_I2Cx_CLK_ENABLE(); 00686 00687 /* Force the I2C peripheral clock reset */ 00688 EVAL_I2Cx_FORCE_RESET(); 00689 00690 /* Release the I2C peripheral clock reset */ 00691 EVAL_I2Cx_RELEASE_RESET(); 00692 00693 /* Enable and set I2Cx Interrupt to a lower priority */ 00694 HAL_NVIC_SetPriority(EVAL_I2Cx_EV_IRQn, 0x0F, 0); 00695 HAL_NVIC_EnableIRQ(EVAL_I2Cx_EV_IRQn); 00696 00697 /* Enable and set I2Cx Interrupt to a lower priority */ 00698 HAL_NVIC_SetPriority(EVAL_I2Cx_ER_IRQn, 0x0F, 0); 00699 HAL_NVIC_EnableIRQ(EVAL_I2Cx_ER_IRQn); 00700 } 00701 00702 /** 00703 * @brief Initializes I2C HAL. 00704 * @retval None 00705 */ 00706 static void I2Cx_Init(void) 00707 { 00708 if(HAL_I2C_GetState(&hEvalI2c) == HAL_I2C_STATE_RESET) 00709 { 00710 hEvalI2c.Instance = EVAL_I2Cx; 00711 hEvalI2c.Init.Timing = EVAL_I2Cx_TIMING; 00712 hEvalI2c.Init.OwnAddress1 = 0; 00713 hEvalI2c.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00714 hEvalI2c.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00715 hEvalI2c.Init.OwnAddress2 = 0; 00716 hEvalI2c.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00717 hEvalI2c.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00718 00719 /* Init the I2C */ 00720 I2Cx_MspInit(); 00721 HAL_I2C_Init(&hEvalI2c); 00722 } 00723 } 00724 00725 /** 00726 * @brief Writes a single data. 00727 * @param Addr: I2C address 00728 * @param Reg: Register address 00729 * @param Value: Data to be written 00730 * @retval None 00731 */ 00732 static void I2Cx_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00733 { 00734 HAL_StatusTypeDef status = HAL_OK; 00735 00736 status = HAL_I2C_Mem_Write(&hEvalI2c, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, 100); 00737 00738 /* Check the communication status */ 00739 if(status != HAL_OK) 00740 { 00741 /* Execute user timeout callback */ 00742 I2Cx_Error(Addr); 00743 } 00744 } 00745 00746 /** 00747 * @brief Reads a single data. 00748 * @param Addr: I2C address 00749 * @param Reg: Register address 00750 * @retval Read data 00751 */ 00752 static uint8_t I2Cx_Read(uint8_t Addr, uint8_t Reg) 00753 { 00754 HAL_StatusTypeDef status = HAL_OK; 00755 uint8_t Value = 0; 00756 00757 status = HAL_I2C_Mem_Read(&hEvalI2c, Addr, Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, 1000); 00758 00759 /* Check the communication status */ 00760 if(status != HAL_OK) 00761 { 00762 /* Execute user timeout callback */ 00763 I2Cx_Error(Addr); 00764 } 00765 return Value; 00766 } 00767 00768 /** 00769 * @brief Reads multiple data. 00770 * @param Addr: I2C address 00771 * @param Reg: Reg address 00772 * @param MemAddress: internal memory address 00773 * @param Buffer: Pointer to data buffer 00774 * @param Length: Length of the data 00775 * @retval Number of read data 00776 */ 00777 static HAL_StatusTypeDef I2Cx_ReadMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00778 { 00779 HAL_StatusTypeDef status = HAL_OK; 00780 00781 status = HAL_I2C_Mem_Read(&hEvalI2c, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00782 00783 /* Check the communication status */ 00784 if(status != HAL_OK) 00785 { 00786 /* I2C error occurred */ 00787 I2Cx_Error(Addr); 00788 } 00789 return status; 00790 } 00791 00792 /** 00793 * @brief Writes a value in a register of the device through BUS in using DMA mode. 00794 * @param Addr: Device address on BUS Bus. 00795 * @param Reg: The target register address to write 00796 * @param MemAddress: internal memory address 00797 * @param Buffer: The target register value to be written 00798 * @param Length: buffer size to be written 00799 * @retval HAL status 00800 */ 00801 static HAL_StatusTypeDef I2Cx_WriteMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00802 { 00803 HAL_StatusTypeDef status = HAL_OK; 00804 00805 status = HAL_I2C_Mem_Write(&hEvalI2c, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00806 00807 /* Check the communication status */ 00808 if(status != HAL_OK) 00809 { 00810 /* Re-Initiaize the I2C Bus */ 00811 I2Cx_Error(Addr); 00812 } 00813 return status; 00814 } 00815 00816 /** 00817 * @brief Checks if target device is ready for communication. 00818 * @note This function is used with Memory devices 00819 * @param DevAddress: Target device address 00820 * @param Trials: Number of trials 00821 * @retval HAL status 00822 */ 00823 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00824 { 00825 return (HAL_I2C_IsDeviceReady(&hEvalI2c, DevAddress, Trials, 1000)); 00826 } 00827 00828 /** 00829 * @brief Manages error callback by re-initializing I2C. 00830 * @param Addr: I2C Address 00831 * @retval None 00832 */ 00833 static void I2Cx_Error(uint8_t Addr) 00834 { 00835 /* De-initialize the I2C communication bus */ 00836 HAL_I2C_DeInit(&hEvalI2c); 00837 00838 /* Re-Initialize the I2C communication bus */ 00839 I2Cx_Init(); 00840 } 00841 00842 /******************************************************************************* 00843 LINK OPERATIONS 00844 *******************************************************************************/ 00845 00846 /********************************* LINK IOE ***********************************/ 00847 #if defined(USE_IOEXPANDER) 00848 /** 00849 * @brief Initializes IOE low level. 00850 * @retval None 00851 */ 00852 void IOE_Init(void) 00853 { 00854 I2Cx_Init(); 00855 } 00856 00857 /** 00858 * @brief Configures IOE low level interrupt. 00859 * @retval None 00860 */ 00861 void IOE_ITConfig(void) 00862 { 00863 /* IO expander IT config done by BSP_TS_ITConfig function */ 00864 } 00865 00866 /** 00867 * @brief IOE writes single data. 00868 * @param Addr: I2C address 00869 * @param Reg: Register address 00870 * @param Value: Data to be written 00871 * @retval None 00872 */ 00873 void IOE_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00874 { 00875 I2Cx_Write(Addr, Reg, Value); 00876 } 00877 00878 /** 00879 * @brief IOE reads single data. 00880 * @param Addr: I2C address 00881 * @param Reg: Register address 00882 * @retval Read data 00883 */ 00884 uint8_t IOE_Read(uint8_t Addr, uint8_t Reg) 00885 { 00886 return I2Cx_Read(Addr, Reg); 00887 } 00888 00889 /** 00890 * @brief IOE reads multiple data. 00891 * @param Addr: I2C address 00892 * @param Reg: Register address 00893 * @param Buffer: Pointer to data buffer 00894 * @param Length: Length of the data 00895 * @retval Number of read data 00896 */ 00897 uint16_t IOE_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 00898 { 00899 return I2Cx_ReadMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 00900 } 00901 00902 /** 00903 * @brief IOE writes multiple data. 00904 * @param Addr: I2C address 00905 * @param Reg: Register address 00906 * @param Buffer: Pointer to data buffer 00907 * @param Length: Length of the data 00908 * @retval None 00909 */ 00910 void IOE_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 00911 { 00912 I2Cx_WriteMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 00913 } 00914 00915 /** 00916 * @brief IOE delay 00917 * @param Delay: Delay in ms 00918 * @retval None 00919 */ 00920 void IOE_Delay(uint32_t Delay) 00921 { 00922 HAL_Delay(Delay); 00923 } 00924 #endif /* USE_IOEXPANDER */ 00925 00926 /********************************* LINK MFX ***********************************/ 00927 00928 #if defined(USE_IOEXPANDER) 00929 /** 00930 * @brief Initializes MFX low level. 00931 * @retval None 00932 */ 00933 void MFX_IO_Init(void) 00934 { 00935 I2Cx_Init(); 00936 } 00937 00938 /** 00939 * @brief DeInitializes MFX low level. 00940 * @retval None 00941 */ 00942 void MFX_IO_DeInit(void) 00943 { 00944 } 00945 00946 /** 00947 * @brief Configures MFX low level interrupt. 00948 * @retval None 00949 */ 00950 void MFX_IO_ITConfig(void) 00951 { 00952 static uint8_t mfx_io_it_enabled = 0; 00953 GPIO_InitTypeDef gpio_init_structure; 00954 00955 if(mfx_io_it_enabled == 0) 00956 { 00957 mfx_io_it_enabled = 1; 00958 /* Enable the GPIO EXTI clock */ 00959 __HAL_RCC_GPIOI_CLK_ENABLE(); 00960 __HAL_RCC_SYSCFG_CLK_ENABLE(); 00961 00962 gpio_init_structure.Pin = GPIO_PIN_8; 00963 gpio_init_structure.Pull = GPIO_NOPULL; 00964 gpio_init_structure.Speed = GPIO_SPEED_FREQ_LOW; 00965 gpio_init_structure.Mode = GPIO_MODE_IT_RISING; 00966 HAL_GPIO_Init(GPIOI, &gpio_init_structure); 00967 00968 /* Enable and set GPIO EXTI Interrupt to the lowest priority */ 00969 HAL_NVIC_SetPriority((IRQn_Type)(EXTI9_5_IRQn), 0x0F, 0x0F); 00970 HAL_NVIC_EnableIRQ((IRQn_Type)(EXTI9_5_IRQn)); 00971 } 00972 } 00973 00974 /** 00975 * @brief MFX writes single data. 00976 * @param Addr: I2C address 00977 * @param Reg: Register address 00978 * @param Value: Data to be written 00979 * @retval None 00980 */ 00981 void MFX_IO_Write(uint16_t Addr, uint8_t Reg, uint8_t Value) 00982 { 00983 I2Cx_Write((uint8_t) Addr, Reg, Value); 00984 } 00985 00986 /** 00987 * @brief MFX reads single data. 00988 * @param Addr: I2C address 00989 * @param Reg: Register address 00990 * @retval Read data 00991 */ 00992 uint8_t MFX_IO_Read(uint16_t Addr, uint8_t Reg) 00993 { 00994 return I2Cx_Read((uint8_t) Addr, Reg); 00995 } 00996 00997 /** 00998 * @brief MFX reads multiple data. 00999 * @param Addr: I2C address 01000 * @param Reg: Register address 01001 * @param Buffer: Pointer to data buffer 01002 * @param Length: Length of the data 01003 * @retval Number of read data 01004 */ 01005 uint16_t MFX_IO_ReadMultiple(uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01006 { 01007 return I2Cx_ReadMultiple((uint8_t)Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01008 } 01009 01010 /** 01011 * @brief MFX delay 01012 * @param Delay: Delay in ms 01013 * @retval None 01014 */ 01015 void MFX_IO_Delay(uint32_t Delay) 01016 { 01017 HAL_Delay(Delay); 01018 } 01019 01020 /** 01021 * @brief Used by Lx family but requested for MFX component compatibility. 01022 * @retval None 01023 */ 01024 void MFX_IO_Wakeup(void) 01025 { 01026 } 01027 01028 /** 01029 * @brief Used by Lx family but requested for MXF component compatibility. 01030 * @retval None 01031 */ 01032 void MFX_IO_EnableWakeupPin(void) 01033 { 01034 } 01035 01036 #endif /* USE_IOEXPANDER */ 01037 01038 /********************************* LINK AUDIO *********************************/ 01039 01040 /** 01041 * @brief Initializes Audio low level. 01042 * @retval None 01043 */ 01044 void AUDIO_IO_Init(void) 01045 { 01046 I2Cx_Init(); 01047 } 01048 01049 /** 01050 * @brief Deinitializes Audio low level. 01051 * @retval None 01052 */ 01053 void AUDIO_IO_DeInit(void) 01054 { 01055 } 01056 01057 /** 01058 * @brief Writes a single data. 01059 * @param Addr: I2C address 01060 * @param Reg: Reg address 01061 * @param Value: Data to be written 01062 * @retval None 01063 */ 01064 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value) 01065 { 01066 uint16_t tmp = Value; 01067 01068 Value = ((uint16_t)(tmp >> 8) & 0x00FF); 01069 01070 Value |= ((uint16_t)(tmp << 8)& 0xFF00); 01071 01072 I2Cx_WriteMultiple(Addr, Reg, I2C_MEMADD_SIZE_16BIT,(uint8_t*)&Value, 2); 01073 } 01074 01075 /** 01076 * @brief Reads a single data. 01077 * @param Addr: I2C address 01078 * @param Reg: Reg address 01079 * @retval Data to be read 01080 */ 01081 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg) 01082 { 01083 uint16_t read_value = 0, tmp = 0; 01084 01085 I2Cx_ReadMultiple(Addr, Reg, I2C_MEMADD_SIZE_16BIT, (uint8_t*)&read_value, 2); 01086 01087 tmp = ((uint16_t)(read_value >> 8) & 0x00FF); 01088 01089 tmp |= ((uint16_t)(read_value << 8)& 0xFF00); 01090 01091 read_value = tmp; 01092 01093 return read_value; 01094 } 01095 01096 /** 01097 * @brief AUDIO Codec delay 01098 * @param Delay: Delay in ms 01099 * @retval None 01100 */ 01101 void AUDIO_IO_Delay(uint32_t Delay) 01102 { 01103 HAL_Delay(Delay); 01104 } 01105 01106 /********************************* LINK CAMERA ********************************/ 01107 01108 /** 01109 * @brief Initializes Camera low level. 01110 * @retval None 01111 */ 01112 void CAMERA_IO_Init(void) 01113 { 01114 I2Cx_Init(); 01115 } 01116 01117 /** 01118 * @brief Camera writes single data. 01119 * @param Addr: I2C address 01120 * @param Reg: Register address 01121 * @param Value: Data to be written 01122 * @retval None 01123 */ 01124 void CAMERA_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value) 01125 { 01126 uint16_t tmp = Value; 01127 /* For S5K5CAG sensor, 16 bits accesses are used */ 01128 Value = ((uint16_t)(tmp >> 8) & 0x00FF); 01129 Value |= ((uint16_t)(tmp << 8)& 0xFF00); 01130 I2Cx_WriteMultiple(Addr, Reg, I2C_MEMADD_SIZE_16BIT,(uint8_t*)&Value, 2); 01131 } 01132 01133 /** 01134 * @brief Camera reads single data. 01135 * @param Addr: I2C address 01136 * @param Reg: Register address 01137 * @retval Read data 01138 */ 01139 uint16_t CAMERA_IO_Read(uint8_t Addr, uint16_t Reg) 01140 { 01141 uint16_t read_value = 0, tmp = 0; 01142 /* For S5K5CAG sensor, 16 bits accesses are used */ 01143 I2Cx_ReadMultiple(Addr, Reg, I2C_MEMADD_SIZE_16BIT, (uint8_t*)&read_value, 2); 01144 tmp = ((uint16_t)(read_value >> 8) & 0x00FF); 01145 tmp |= ((uint16_t)(read_value << 8)& 0xFF00); 01146 read_value = tmp; 01147 return read_value; 01148 } 01149 01150 /** 01151 * @brief Camera delay 01152 * @param Delay: Delay in ms 01153 * @retval None 01154 */ 01155 void CAMERA_Delay(uint32_t Delay) 01156 { 01157 HAL_Delay(Delay); 01158 } 01159 01160 /******************************** LINK I2C EEPROM *****************************/ 01161 01162 /** 01163 * @brief Initializes peripherals used by the I2C EEPROM driver. 01164 * @retval None 01165 */ 01166 void EEPROM_IO_Init(void) 01167 { 01168 I2Cx_Init(); 01169 } 01170 01171 /** 01172 * @brief Write data to I2C EEPROM driver in using DMA channel. 01173 * @param DevAddress: Target device address 01174 * @param MemAddress: Internal memory address 01175 * @param pBuffer: Pointer to data buffer 01176 * @param BufferSize: Amount of data to be sent 01177 * @retval HAL status 01178 */ 01179 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01180 { 01181 return (I2Cx_WriteMultiple(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01182 } 01183 01184 /** 01185 * @brief Read data from I2C EEPROM driver in using DMA channel. 01186 * @param DevAddress: Target device address 01187 * @param MemAddress: Internal memory address 01188 * @param pBuffer: Pointer to data buffer 01189 * @param BufferSize: Amount of data to be read 01190 * @retval HAL status 01191 */ 01192 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01193 { 01194 return (I2Cx_ReadMultiple(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01195 } 01196 01197 /** 01198 * @brief Checks if target device is ready for communication. 01199 * @note This function is used with Memory devices 01200 * @param DevAddress: Target device address 01201 * @param Trials: Number of trials 01202 * @retval HAL status 01203 */ 01204 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01205 { 01206 return (I2Cx_IsDeviceReady(DevAddress, Trials)); 01207 } 01208 01209 /******************************** LINK TS (TouchScreen) *****************************/ 01210 01211 /** 01212 * @brief Initialize I2C communication 01213 * channel from MCU to TouchScreen (TS). 01214 */ 01215 void TS_IO_Init(void) 01216 { 01217 I2Cx_Init(); 01218 } 01219 01220 /** 01221 * @brief Writes single data with I2C communication 01222 * channel from MCU to TouchScreen. 01223 * @param Addr: I2C address 01224 * @param Reg: Register address 01225 * @param Value: Data to be written 01226 */ 01227 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 01228 { 01229 I2Cx_Write(Addr, Reg, Value); 01230 } 01231 01232 /** 01233 * @brief Reads single data with I2C communication 01234 * channel from TouchScreen. 01235 * @param Addr: I2C address 01236 * @param Reg: Register address 01237 * @retval Read data 01238 */ 01239 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg) 01240 { 01241 return I2Cx_Read(Addr, Reg); 01242 } 01243 01244 /** 01245 * @brief Reads multiple data with I2C communication 01246 * channel from TouchScreen. 01247 * @param Addr: I2C address 01248 * @param Reg: Register address 01249 * @param Buffer: Pointer to data buffer 01250 * @param Length: Length of the data 01251 * @retval Number of read data 01252 */ 01253 uint16_t TS_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01254 { 01255 return I2Cx_ReadMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01256 } 01257 01258 /** 01259 * @brief Writes multiple data with I2C communication 01260 * channel from MCU to TouchScreen. 01261 * @param Addr: I2C address 01262 * @param Reg: Register address 01263 * @param Buffer: Pointer to data buffer 01264 * @param Length: Length of the data 01265 * @retval None 01266 */ 01267 void TS_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01268 { 01269 I2Cx_WriteMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01270 } 01271 01272 /** 01273 * @brief Delay function used in TouchScreen low level driver. 01274 * @param Delay: Delay in ms 01275 * @retval None 01276 */ 01277 void TS_IO_Delay(uint32_t Delay) 01278 { 01279 HAL_Delay(Delay); 01280 } 01281 01282 /**************************** LINK OTM8009A (Display driver) ******************/ 01283 /** 01284 * @brief OTM8009A delay 01285 * @param Delay: Delay in ms 01286 */ 01287 void OTM8009A_IO_Delay(uint32_t Delay) 01288 { 01289 HAL_Delay(Delay); 01290 } 01291 01292 #if defined(USE_LCD_HDMI) 01293 /**************************** LINK ADV7533 DSI-HDMI (Display driver) **********/ 01294 /** 01295 * @brief Initializes HDMI IO low level. 01296 * @retval None 01297 */ 01298 void HDMI_IO_Init(void) 01299 { 01300 I2Cx_Init(); 01301 } 01302 01303 /** 01304 * @brief HDMI writes single data. 01305 * @param Addr: I2C address 01306 * @param Reg: Register address 01307 * @param Value: Data to be written 01308 * @retval None 01309 */ 01310 void HDMI_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 01311 { 01312 I2Cx_Write(Addr, Reg, Value); 01313 } 01314 01315 /** 01316 * @brief Reads single data with I2C communication 01317 * channel from HDMI bridge. 01318 * @param Addr: I2C address 01319 * @param Reg: Register address 01320 * @retval Read data 01321 */ 01322 uint8_t HDMI_IO_Read(uint8_t Addr, uint8_t Reg) 01323 { 01324 return I2Cx_Read(Addr, Reg); 01325 } 01326 01327 /** 01328 * @brief HDMI delay 01329 * @param Delay: Delay in ms 01330 * @retval None 01331 */ 01332 void HDMI_IO_Delay(uint32_t Delay) 01333 { 01334 HAL_Delay(Delay); 01335 } 01336 #endif /* USE_LCD_HDMI */ 01337 01338 /** 01339 * @} 01340 */ 01341 01342 /** 01343 * @} 01344 */ 01345 01346 /** 01347 * @} 01348 */ 01349 01350 /** 01351 * @} 01352 */ 01353 01354 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu May 25 2017 11:03:11 for STM32769I_EVAL BSP User Manual by
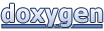