STM324x9I_EVAL BSP User Manual
|
stm324x9i_eval_audio.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm324x9i_eval_audio.h 00004 * @author MCD Application Team 00005 * @version V2.2.2 00006 * @date 13-January-2016 00007 * @brief This file contains the common defines and functions prototypes for 00008 * the stm324x9i_eval_audio.c driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM324x9I_EVAL_AUDIO_H 00041 #define __STM324x9I_EVAL_AUDIO_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 /* Include audio component Driver */ 00049 #include "../Components/wm8994/wm8994.h" 00050 #include "stm324x9i_eval.h" 00051 #include "../../../Middlewares/ST/STM32_Audio/Addons/PDM/pdm_filter.h" 00052 00053 /** @addtogroup BSP 00054 * @{ 00055 */ 00056 00057 /** @addtogroup STM324x9I_EVAL 00058 * @{ 00059 */ 00060 00061 /** @addtogroup STM324x9I_EVAL_AUDIO 00062 * @{ 00063 */ 00064 00065 /** @defgroup STM324x9I_EVAL_AUDIO_Exported_Types STM324x9I EVAL AUDIO Exported Types 00066 * @{ 00067 */ 00068 /** 00069 * @} 00070 */ 00071 00072 /** @defgroup STM324x9I_EVAL_AUDIO_Exported_Constants STM324x9I EVAL AUDIO Exported Constants 00073 * @{ 00074 */ 00075 00076 /*------------------------------------------------------------------------------ 00077 USER SAI defines parameters 00078 -----------------------------------------------------------------------------*/ 00079 /** @defgroup CODEC_AudioFrame_SLOT_TDMMode CODEC AudioFrame SLOT TDMMode 00080 * @brief In W8994 codec the Audio frame contains 4 slots : TDM Mode 00081 * TDM format : 00082 * +------------------|------------------|--------------------|-------------------+ 00083 * | CODEC_SLOT0 Left | CODEC_SLOT1 Left | CODEC_SLOT0 Right | CODEC_SLOT1 Right | 00084 * +------------------------------------------------------------------------------+ 00085 * @{ 00086 */ 00087 /* To have 2 separate audio stream in Both headphone and speaker the 4 slot must be activated */ 00088 #define CODEC_AUDIOFRAME_SLOT_0123 SAI_SLOTACTIVE_0 | SAI_SLOTACTIVE_1 | SAI_SLOTACTIVE_2 | SAI_SLOTACTIVE_3 00089 /* To have an audio stream in headphone only SAI Slot 0 and Slot 2 must be activated */ 00090 #define CODEC_AUDIOFRAME_SLOT_02 SAI_SLOTACTIVE_0 | SAI_SLOTACTIVE_2 00091 /* To have an audio stream in speaker only SAI Slot 1 and Slot 3 must be activated */ 00092 #define CODEC_AUDIOFRAME_SLOT_13 SAI_SLOTACTIVE_1 | SAI_SLOTACTIVE_3 00093 /** 00094 * @} 00095 */ 00096 00097 /* SAI peripheral configuration defines */ 00098 #define AUDIO_SAIx SAI1_Block_B 00099 #define AUDIO_SAIx_CLK_ENABLE() __SAI1_CLK_ENABLE() 00100 #define AUDIO_SAIx_MCLK_SCK_SD_FS_AF GPIO_AF6_SAI1 00101 00102 #define AUDIO_SAIx_MCLK_SCK_SD_FS_ENABLE() __GPIOF_CLK_ENABLE() 00103 #define AUDIO_SAIx_FS_PIN GPIO_PIN_9 00104 #define AUDIO_SAIx_SCK_PIN GPIO_PIN_8 00105 #define AUDIO_SAIx_SD_PIN GPIO_PIN_6 00106 #define AUDIO_SAIx_MCK_PIN GPIO_PIN_7 00107 #define AUDIO_SAIx_MCLK_SCK_SD_FS_GPIO_PORT GPIOF 00108 00109 00110 /* SAI DMA Stream definitions */ 00111 #define AUDIO_SAIx_DMAx_CLK_ENABLE() __DMA2_CLK_ENABLE() 00112 #define AUDIO_SAIx_DMAx_STREAM DMA2_Stream5 00113 #define AUDIO_SAIx_DMAx_CHANNEL DMA_CHANNEL_0 00114 #define AUDIO_SAIx_DMAx_IRQ DMA2_Stream5_IRQn 00115 #define AUDIO_SAIx_DMAx_PERIPH_DATA_SIZE DMA_PDATAALIGN_HALFWORD 00116 #define AUDIO_SAIx_DMAx_MEM_DATA_SIZE DMA_MDATAALIGN_HALFWORD 00117 #define DMA_MAX_SZE 0xFFFF 00118 00119 #define AUDIO_SAIx_DMAx_IRQHandler DMA2_Stream5_IRQHandler 00120 00121 /* Select the interrupt preemption priority for the DMA interrupt */ 00122 #define AUDIO_OUT_IRQ_PREPRIO 5 /* Select the preemption priority level(0 is the highest) */ 00123 00124 /*------------------------------------------------------------------------------ 00125 AUDIO IN CONFIGURATION 00126 ------------------------------------------------------------------------------*/ 00127 /* SPI Configuration defines */ 00128 #define AUDIO_I2Sx SPI3 00129 #define AUDIO_I2Sx_CLK_ENABLE() __SPI3_CLK_ENABLE() 00130 #define AUDIO_I2Sx_SCK_PIN GPIO_PIN_3 00131 #define AUDIO_I2Sx_SCK_GPIO_PORT GPIOB 00132 #define AUDIO_I2Sx_SCK_GPIO_CLK_ENABLE() __GPIOB_CLK_ENABLE() 00133 #define AUDIO_I2Sx_SCK_AF GPIO_AF6_SPI3 00134 00135 #define AUDIO_I2Sx_SD_PIN GPIO_PIN_6 00136 #define AUDIO_I2Sx_SD_GPIO_PORT GPIOD 00137 #define AUDIO_I2Sx_SD_GPIO_CLK_ENABLE() __GPIOD_CLK_ENABLE() 00138 #define AUDIO_I2Sx_SD_AF GPIO_AF5_I2S3ext 00139 00140 /* I2S DMA Stream Rx definitions */ 00141 #define AUDIO_I2Sx_DMAx_CLK_ENABLE() __DMA1_CLK_ENABLE() 00142 #define AUDIO_I2Sx_DMAx_STREAM DMA1_Stream2 00143 #define AUDIO_I2Sx_DMAx_CHANNEL DMA_CHANNEL_0 00144 #define AUDIO_I2Sx_DMAx_IRQ DMA1_Stream2_IRQn 00145 #define AUDIO_I2Sx_DMAx_PERIPH_DATA_SIZE DMA_PDATAALIGN_HALFWORD 00146 #define AUDIO_I2Sx_DMAx_MEM_DATA_SIZE DMA_MDATAALIGN_HALFWORD 00147 00148 #define AUDIO_I2Sx_DMAx_IRQHandler DMA1_Stream2_IRQHandler 00149 00150 /* Select the interrupt preemption priority and subpriority for the IT/DMA interrupt */ 00151 #define AUDIO_IN_IRQ_PREPRIO 6 /* Select the preemption priority level(0 is the highest) */ 00152 00153 00154 /* Two channels are used: 00155 - one channel as input which is connected to I2S SCK in stereo mode 00156 - one channel as output which divides the frequency on the input 00157 */ 00158 00159 #define AUDIO_TIMx_CLK_ENABLE() __TIM3_CLK_ENABLE() 00160 #define AUDIO_TIMx_CLK_DISABLE() __TIM3_CLK_DISABLE() 00161 #define AUDIO_TIMx TIM3 00162 #define AUDIO_TIMx_IN_CHANNEL TIM_CHANNEL_1 00163 #define AUDIO_TIMx_OUT_CHANNEL TIM_CHANNEL_2 /* Select channel 2 as output */ 00164 #define AUDIO_TIMx_GPIO_CLK_ENABLE() __GPIOC_CLK_ENABLE() 00165 #define AUDIO_TIMx_GPIO GPIOC 00166 #define AUDIO_TIMx_IN_GPIO_PIN GPIO_PIN_6 00167 #define AUDIO_TIMx_OUT_GPIO_PIN GPIO_PIN_7 00168 #define AUDIO_TIMx_AF GPIO_AF2_TIM3 00169 00170 /*------------------------------------------------------------------------------ 00171 CONFIGURATION: Audio Driver Configuration parameters 00172 ------------------------------------------------------------------------------*/ 00173 00174 #define AUDIODATA_SIZE 2 /* 16-bits audio data size */ 00175 00176 /* Audio status definition */ 00177 #define AUDIO_OK 0 00178 #define AUDIO_ERROR 1 00179 #define AUDIO_TIMEOUT 2 00180 00181 /* AudioFreq * DataSize (2 bytes) * NumChannels (Stereo: 2) */ 00182 #define DEFAULT_AUDIO_IN_FREQ I2S_AUDIOFREQ_16K 00183 #define DEFAULT_AUDIO_IN_BIT_RESOLUTION 16 00184 #define DEFAULT_AUDIO_IN_CHANNEL_NBR 2 /* Mono = 1, Stereo = 2 */ 00185 #define DEFAULT_AUDIO_IN_VOLUME 64 00186 00187 /* PDM buffer input size */ 00188 #define INTERNAL_BUFF_SIZE 128*DEFAULT_AUDIO_IN_FREQ/16000*DEFAULT_AUDIO_IN_CHANNEL_NBR 00189 /* PCM buffer output size */ 00190 #define PCM_OUT_SIZE DEFAULT_AUDIO_IN_FREQ/1000*2 00191 #define CHANNEL_DEMUX_MASK 0x55 00192 00193 /*------------------------------------------------------------------------------ 00194 OPTIONAL Configuration defines parameters 00195 ------------------------------------------------------------------------------*/ 00196 00197 /* Delay for the Codec to be correctly reset */ 00198 #define CODEC_RESET_DELAY 5 00199 00200 /** 00201 * @} 00202 */ 00203 00204 /** @defgroup STM324x9I_EVAL_AUDIO_Exported_Variables STM324x9I EVAL AUDIO Exported Variables 00205 * @{ 00206 */ 00207 extern __IO uint16_t AudioInVolume; 00208 /** 00209 * @} 00210 */ 00211 00212 /** @defgroup STM324x9I_EVAL_AUDIO_Exported_Macros STM324x9I EVAL AUDIO Exported Macros 00213 * @{ 00214 */ 00215 #define DMA_MAX(x) (((x) <= DMA_MAX_SZE)? (x):DMA_MAX_SZE) 00216 /** 00217 * @} 00218 */ 00219 00220 /** @defgroup STM324x9I_EVAL_AUDIO_OUT_Exported_Functions STM324x9I EVAL AUDIO OUT Exported Functions 00221 * @{ 00222 */ 00223 uint8_t BSP_AUDIO_OUT_Init(uint16_t OutputDevice, uint8_t Volume, uint32_t AudioFreq); 00224 uint8_t BSP_AUDIO_OUT_Play(uint16_t* pBuffer, uint32_t Size); 00225 void BSP_AUDIO_OUT_ChangeBuffer(uint16_t *pData, uint16_t Size); 00226 uint8_t BSP_AUDIO_OUT_Pause(void); 00227 uint8_t BSP_AUDIO_OUT_Resume(void); 00228 uint8_t BSP_AUDIO_OUT_Stop(uint32_t Option); 00229 uint8_t BSP_AUDIO_OUT_SetVolume(uint8_t Volume); 00230 void BSP_AUDIO_OUT_SetFrequency(uint32_t AudioFreq); 00231 void BSP_AUDIO_OUT_SetAudioFrameSlot(uint32_t AudioFrameSlot); 00232 uint8_t BSP_AUDIO_OUT_SetMute(uint32_t Cmd); 00233 uint8_t BSP_AUDIO_OUT_SetOutputMode(uint8_t Output); 00234 00235 /* User Callbacks: user has to implement these functions in his code if they are needed. */ 00236 /* This function is called when the requested data has been completely transferred.*/ 00237 void BSP_AUDIO_OUT_TransferComplete_CallBack(void); 00238 00239 /* This function is called when half of the requested buffer has been transferred. */ 00240 void BSP_AUDIO_OUT_HalfTransfer_CallBack(void); 00241 00242 /* This function is called when an Interrupt due to transfer error on or peripheral 00243 error occurs. */ 00244 void BSP_AUDIO_OUT_Error_CallBack(void); 00245 00246 /** 00247 * @} 00248 */ 00249 00250 /** @defgroup STM324x9I_EVAL_AUDIO_IN_Exported_Functions STM324x9I EVAL AUDIO IN Exported Functions 00251 * @{ 00252 */ 00253 uint8_t BSP_AUDIO_IN_Init(uint32_t AudioFreq, uint32_t BitRes, uint32_t ChnlNbr); 00254 uint8_t BSP_AUDIO_IN_Record(uint16_t *pData, uint32_t Size); 00255 uint8_t BSP_AUDIO_IN_Stop(void); 00256 uint8_t BSP_AUDIO_IN_Pause(void); 00257 uint8_t BSP_AUDIO_IN_Resume(void); 00258 uint8_t BSP_AUDIO_IN_SetVolume(uint8_t Volume); 00259 uint8_t BSP_AUDIO_IN_PDMToPCM(uint16_t* PDMBuf, uint16_t* PCMBuf); 00260 /* User Callbacks: user has to implement these functions in his code if they are needed. */ 00261 /* This function should be implemented by the user application. 00262 It is called into this driver when the current buffer is filled to prepare the next 00263 buffer pointer and its size. */ 00264 void BSP_AUDIO_IN_TransferComplete_CallBack(void); 00265 void BSP_AUDIO_IN_HalfTransfer_CallBack(void); 00266 00267 /* This function is called when an Interrupt due to transfer error on or peripheral 00268 error occurs. */ 00269 void BSP_AUDIO_IN_Error_Callback(void); 00270 00271 /** 00272 * @} 00273 */ 00274 00275 /** 00276 * @} 00277 */ 00278 00279 /** 00280 * @} 00281 */ 00282 00283 /** 00284 * @} 00285 */ 00286 00287 #ifdef __cplusplus 00288 } 00289 #endif 00290 00291 #endif /* __STM324x9I_EVAL_AUDIO_H */ 00292 00293 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jan 13 2016 15:52:54 for STM324x9I_EVAL BSP User Manual by
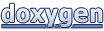