STM32446E_EVAL BSP User Manual
|
stm32446e_eval_qspi.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32446e_eval_qspi.h 00004 * @author MCD Application Team 00005 * @version V1.1.1 00006 * @date 13-January-2016 00007 * @brief This file contains the common defines and functions prototypes for 00008 * the stm32446e_eval_qspi.c driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /** @addtogroup BSP 00040 * @{ 00041 */ 00042 00043 /** @addtogroup STM32446E_EVAL 00044 * @{ 00045 */ 00046 00047 /* Define to prevent recursive inclusion -------------------------------------*/ 00048 #ifndef __STM32446E_EVAL_QSPI_H 00049 #define __STM32446E_EVAL_QSPI_H 00050 00051 #ifdef __cplusplus 00052 extern "C" { 00053 #endif 00054 00055 /* Includes ------------------------------------------------------------------*/ 00056 #include "stm32f4xx_hal.h" 00057 #include "../Components/n25q256a/n25q256a.h" 00058 00059 /** @defgroup STM32446E_EVAL_QSPI STM32446E EVAL QSPI 00060 * @{ 00061 */ 00062 00063 00064 /* Exported constants --------------------------------------------------------*/ 00065 /** @defgroup STM32446E_EVAL_QSPI_Exported_Constants STM32446E EVAL QSPI Exported Constants 00066 * @{ 00067 */ 00068 /* QSPI Error codes */ 00069 #define QSPI_OK ((uint8_t)0x00) 00070 #define QSPI_ERROR ((uint8_t)0x01) 00071 #define QSPI_BUSY ((uint8_t)0x02) 00072 #define QSPI_NOT_SUPPORTED ((uint8_t)0x04) 00073 #define QSPI_SUSPENDED ((uint8_t)0x08) 00074 00075 00076 /* Definition for QSPI clock resources */ 00077 #define QSPI_CLK_ENABLE() __HAL_RCC_QSPI_CLK_ENABLE() 00078 #define QSPI_CLK_DISABLE() __HAL_RCC_QSPI_CLK_DISABLE() 00079 #define QSPI_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOG_CLK_ENABLE() 00080 #define QSPI_CS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOG_CLK_DISABLE() 00081 #if !defined(USE_STM32446E_EVAL_REVA) 00082 #define QSPI_CLK_GPIO_CLK_ENABLE() __HAL_RCC_GPIOD_CLK_ENABLE() 00083 #define QSPI_CLK_GPIO_CLK_DISABLE() __HAL_RCC_GPIOD_CLK_DISABLE() 00084 #else 00085 #define QSPI_CLK_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00086 #define QSPI_CLK_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00087 #endif // !USE_STM32446E_EVAL_REVA 00088 #define QSPI_Dx_GPIO_CLK_ENABLE() __HAL_RCC_GPIOF_CLK_ENABLE() 00089 #define QSPI_Dx_GPIO_CLK_DISABLE() __HAL_RCC_GPIOF_CLK_DISABLE() 00090 00091 #define QSPI_FORCE_RESET() __HAL_RCC_QSPI_FORCE_RESET() 00092 #define QSPI_RELEASE_RESET() __HAL_RCC_QSPI_RELEASE_RESET() 00093 00094 /* Definition for QSPI Pins */ 00095 #define QSPI_CS_PIN GPIO_PIN_6 00096 #define QSPI_CS_GPIO_PORT GPIOG 00097 #if !defined(USE_STM32446E_EVAL_REVA) 00098 #define QSPI_CLK_PIN GPIO_PIN_3 00099 #define QSPI_CLK_GPIO_PORT GPIOD 00100 #else 00101 #define QSPI_CLK_PIN GPIO_PIN_2 00102 #define QSPI_CLK_GPIO_PORT GPIOB 00103 #endif // !USE_STM32446E_EVAL_REVA 00104 #define QSPI_D0_PIN GPIO_PIN_8 00105 #define QSPI_D0_GPIO_PORT GPIOF 00106 #define QSPI_D1_PIN GPIO_PIN_9 00107 #define QSPI_D1_GPIO_PORT GPIOF 00108 #define QSPI_D2_PIN GPIO_PIN_7 00109 #define QSPI_D2_GPIO_PORT GPIOF 00110 #define QSPI_D3_PIN GPIO_PIN_6 00111 #define QSPI_D3_GPIO_PORT GPIOF 00112 00113 00114 /** 00115 * @} 00116 */ 00117 00118 /* Exported types ------------------------------------------------------------*/ 00119 /** @defgroup STM32446E_EVAL_QSPI_Exported_Types STM32446E EVAL QSPI Exported Types 00120 * @{ 00121 */ 00122 /* QSPI Info */ 00123 typedef struct { 00124 uint32_t FlashSize; /*!< Size of the flash */ 00125 uint32_t EraseSectorSize; /*!< Size of sectors for the erase operation */ 00126 uint32_t EraseSectorsNumber; /*!< Number of sectors for the erase operation */ 00127 uint32_t ProgPageSize; /*!< Size of pages for the program operation */ 00128 uint32_t ProgPagesNumber; /*!< Number of pages for the program operation */ 00129 } QSPI_Info; 00130 00131 /** 00132 * @} 00133 */ 00134 00135 00136 /* Exported functions --------------------------------------------------------*/ 00137 /** @defgroup STM32446E_EVAL_QSPI_Exported_Functions STM32446E EVAL QSPI Exported Functions 00138 * @{ 00139 */ 00140 uint8_t BSP_QSPI_Init (void); 00141 uint8_t BSP_QSPI_DeInit (void); 00142 uint8_t BSP_QSPI_Read (uint8_t* pData, uint32_t ReadAddr, uint32_t Size); 00143 uint8_t BSP_QSPI_Write (uint8_t* pData, uint32_t WriteAddr, uint32_t Size); 00144 uint8_t BSP_QSPI_Erase_Block(uint32_t BlockAddress); 00145 uint8_t BSP_QSPI_Erase_Chip (void); 00146 uint8_t BSP_QSPI_GetStatus (void); 00147 uint8_t BSP_QSPI_GetInfo (QSPI_Info* pInfo); 00148 uint8_t BSP_QSPI_MemoryMappedMode(void); 00149 00150 /* These function can be modified in case the current settings (e.g. DMA stream) 00151 need to be changed for specific application needs */ 00152 void BSP_QSPI_MspInit(QSPI_HandleTypeDef *hqspi, void *Params); 00153 void BSP_QSPI_MspDeInit(QSPI_HandleTypeDef *hqspi, void *Params); 00154 00155 /** 00156 * @} 00157 */ 00158 00159 /** 00160 * @} 00161 */ 00162 00163 #ifdef __cplusplus 00164 } 00165 #endif 00166 00167 #endif /* __STM32446E_EVAL_QSPI_H */ 00168 /** 00169 * @} 00170 */ 00171 00172 /** 00173 * @} 00174 */ 00175 00176 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Jan 15 2016 10:06:21 for STM32446E_EVAL BSP User Manual by
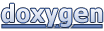