STM32303C_EVAL BSP User Manual
|
stm32303c_eval_tsensor.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32303c_eval_tsensor.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of functions needed to manage the I2C TS751 00006 * temperature sensor mounted on STM32303C-EVAL board . 00007 * It implements a high level communication layer for read and write 00008 * from/to this sensor. The needed STM323F30x hardware resources (I2C and 00009 * GPIO) are defined in stm32303c_eval.h file, and the initialization is 00010 * performed in TSENSOR_IO_Init() function declared in stm32303c_eval.c 00011 * file. 00012 * You can easily tailor this driver to any other development board, 00013 * by just adapting the defines for hardware resources and 00014 * TSENSOR_IO_Init() function. 00015 * 00016 * +--------------------------------------------------------------------+ 00017 * | Pin assignment | 00018 * +----------------------------------------+--------------+------------+ 00019 * | STM32F30x I2C Pins | STTS751 | Pin | 00020 * +----------------------------------------+--------------+------------+ 00021 * | . | Addr/Therm | 1 | 00022 * | . | GND | 2 (0V) | 00023 * | . | VDD | 3 (3.3V)| 00024 * | TSENSOR_I2C_SCL_PIN/ SCL | SCL | 4 | 00025 * | TSENSOR_I2C_SMBUSALERT_PIN/ SMBUS ALERT| SMBUS ALERT| 5 | 00026 * | TSENSOR_I2C_SDA_PIN/ SDA | SDA | 6 | 00027 * +----------------------------------------+--------------+------------+ 00028 ****************************************************************************** 00029 * @attention 00030 * 00031 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00032 * 00033 * Redistribution and use in source and binary forms, with or without modification, 00034 * are permitted provided that the following conditions are met: 00035 * 1. Redistributions of source code must retain the above copyright notice, 00036 * this list of conditions and the following disclaimer. 00037 * 2. Redistributions in binary form must reproduce the above copyright notice, 00038 * this list of conditions and the following disclaimer in the documentation 00039 * and/or other materials provided with the distribution. 00040 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00041 * may be used to endorse or promote products derived from this software 00042 * without specific prior written permission. 00043 * 00044 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00045 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00046 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00047 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00048 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00049 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00050 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00051 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00052 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00053 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00054 * 00055 ****************************************************************************** 00056 */ 00057 00058 /* Includes ------------------------------------------------------------------*/ 00059 #include "stm32303c_eval_tsensor.h" 00060 00061 /** @addtogroup BSP 00062 * @{ 00063 */ 00064 00065 /** @addtogroup STM32303C_EVAL 00066 * @{ 00067 */ 00068 00069 /** @addtogroup STM32303C_EVAL_TSENSOR 00070 * @brief This file includes the TS751 Temperature Sensor driver of 00071 * STM32303C-EVAL boards. 00072 * @{ 00073 */ 00074 00075 /** @addtogroup STM32303C_EVAL_TSENSOR_Private_Variables 00076 * @{ 00077 */ 00078 static TSENSOR_DrvTypeDef *tsensor_drv; 00079 /** 00080 * @} 00081 */ 00082 00083 /** @addtogroup STM32303C_EVAL_TSENSOR_Private_Functions 00084 * @{ 00085 */ 00086 00087 /** 00088 * @brief Initializes peripherals used by the I2C Temperature Sensor driver. 00089 * @retval TSENSOR status 00090 */ 00091 uint32_t BSP_TSENSOR_Init(void) 00092 { 00093 uint8_t ret = TSENSOR_ERROR; 00094 TSENSOR_InitTypeDef STTS751_InitStructure; 00095 00096 /* Temperature Sensor Initialization */ 00097 if(Stts751Drv.IsReady(TSENSOR_I2C_ADDRESS, TSENSOR_MAX_TRIALS) == HAL_OK) 00098 { 00099 /* Initialize the temperature sensor driver structure */ 00100 tsensor_drv = &Stts751Drv; 00101 00102 /* Configure Temperature Sensor : Conversion 12 bits in continuous mode at one conversion per second */ 00103 /* Alert outside range Limit Temperature 12� <-> 24�c */ 00104 STTS751_InitStructure.AlertMode = STTS751_ALERT_ENABLE; 00105 STTS751_InitStructure.ConversionMode = STTS751_CONTINUOUS_MODE; 00106 STTS751_InitStructure.ConversionResolution = STTS751_CONV_12BITS; 00107 STTS751_InitStructure.ConversionRate = STTS751_ONE_PER_SECOND; 00108 STTS751_InitStructure.TemperatureLimitHigh = 24; 00109 STTS751_InitStructure.TemperatureLimitLow = 12; 00110 00111 /* TSENSOR Init */ 00112 tsensor_drv->Init(TSENSOR_I2C_ADDRESS, &STTS751_InitStructure); 00113 00114 ret = TSENSOR_OK; 00115 } 00116 else 00117 { 00118 ret = TSENSOR_ERROR; 00119 } 00120 00121 return ret; 00122 } 00123 00124 /** 00125 * @brief Returns the Temperature Sensor status. 00126 * @retval The Temperature Sensor status. 00127 */ 00128 uint8_t BSP_TSENSOR_ReadStatus(void) 00129 { 00130 return (tsensor_drv->ReadStatus(TSENSOR_I2C_ADDRESS)); 00131 } 00132 00133 /** 00134 * @brief Read Temperature register of TS751. 00135 * @retval STTS751 measured temperature value. 00136 */ 00137 uint16_t BSP_TSENSOR_ReadTemp(void) 00138 { 00139 return tsensor_drv->ReadTemp(TSENSOR_I2C_ADDRESS); 00140 00141 } 00142 00143 /** 00144 * @} 00145 */ 00146 00147 /** 00148 * @} 00149 */ 00150 00151 /** 00152 * @} 00153 */ 00154 00155 /** 00156 * @} 00157 */ 00158 00159 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed May 31 2017 10:00:44 for STM32303C_EVAL BSP User Manual by
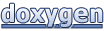