_BSP_User_Manual
|
Functions | |
uint8_t | BSP_SERIAL_FLASH_Init (void) |
Initializes peripherals used by the Serial FLASH device. | |
uint8_t | BSP_SERIAL_FLASH_EraseSector (uint32_t SectorAddr) |
Erases the specified FLASH sector. | |
uint8_t | BSP_SERIAL_FLASH_EraseBulk (void) |
Erases the entire FLASH. | |
uint8_t | BSP_SERIAL_FLASH_WritePage (uint32_t uwStartAddress, uint8_t *pData, uint32_t uwDataSize) |
Writes more than one byte to the FLASH with a single WRITE cycle (Page WRITE sequence). | |
uint8_t | BSP_SERIAL_FLASH_WriteData (uint32_t uwStartAddress, uint8_t *pData, uint32_t uwDataSize) |
Writes block of data to the FLASH. | |
uint8_t | BSP_SERIAL_FLASH_ReadData (uint32_t uwStartAddress, uint8_t *pData, uint32_t uwDataSize) |
Reads a block of data from the FLASH. | |
uint32_t | BSP_SERIAL_FLASH_ReadID (void) |
Reads FLASH identification. | |
HAL_StatusTypeDef | FLASH_SPI_IO_Init (void) |
Initializes the FLASH SPI and put it into StandBy State (Ready for data transfer). | |
uint8_t | FLASH_SPI_IO_WriteByte (uint8_t Data) |
Write a byte on the FLASH SPI. | |
uint8_t | FLASH_SPI_IO_ReadByte (void) |
Read a byte from the FLASH SPI. | |
HAL_StatusTypeDef | FLASH_SPI_IO_ReadData (uint32_t MemAddress, uint8_t *pBuffer, uint32_t BufferSize) |
Read data from FLASH SPI driver. | |
void | FLASH_SPI_IO_WriteEnable (void) |
Select the FLASH SPI and send "Write Enable" instruction. | |
HAL_StatusTypeDef | FLASH_SPI_IO_WaitForWriteEnd (void) |
Wait response from the FLASH SPI and Deselect the device. | |
uint32_t | FLASH_SPI_IO_ReadID (void) |
Reads FLASH SPI identification. |
Function Documentation
uint8_t BSP_SERIAL_FLASH_EraseBulk | ( | void | ) |
Erases the entire FLASH.
< Bulk Erase
< Select the FLASH and send "Write Enable" instruction
< Send Bulk Erase instruction
< Wait the end of Flash writing and Deselect the FLASH
Definition at line 139 of file stm3210e_eval_serialflash.c.
References FLASH_ERROR, FLASH_OK, FLASH_SPI_CMD_BE, FLASH_SPI_IO_WaitForWriteEnd(), FLASH_SPI_IO_WriteByte(), and FLASH_SPI_IO_WriteEnable().
uint8_t BSP_SERIAL_FLASH_EraseSector | ( | uint32_t | SectorAddr | ) |
Erases the specified FLASH sector.
< Sector Erase
< Select the FLASH and send "Write Enable" instruction
< Send Sector Erase instruction
< Send SectorAddr high nibble address byte
< Send SectorAddr medium nibble address byte
< Send SectorAddr low nibble address byte
< Wait the end of Flash writing and Deselect the FLASH
Definition at line 108 of file stm3210e_eval_serialflash.c.
References FLASH_ERROR, FLASH_OK, FLASH_SPI_CMD_SE, FLASH_SPI_IO_WaitForWriteEnd(), FLASH_SPI_IO_WriteByte(), and FLASH_SPI_IO_WriteEnable().
uint8_t BSP_SERIAL_FLASH_Init | ( | void | ) |
Initializes peripherals used by the Serial FLASH device.
Definition at line 90 of file stm3210e_eval_serialflash.c.
References FLASH_ERROR, FLASH_OK, and FLASH_SPI_IO_Init().
uint8_t BSP_SERIAL_FLASH_ReadData | ( | uint32_t | uwStartAddress, |
uint8_t * | pData, | ||
uint32_t | uwDataSize | ||
) |
Reads a block of data from the FLASH.
Definition at line 302 of file stm3210e_eval_serialflash.c.
References FLASH_ERROR, FLASH_OK, and FLASH_SPI_IO_ReadData().
uint32_t BSP_SERIAL_FLASH_ReadID | ( | void | ) |
Reads FLASH identification.
Definition at line 319 of file stm3210e_eval_serialflash.c.
References FLASH_SPI_IO_ReadID().
uint8_t BSP_SERIAL_FLASH_WriteData | ( | uint32_t | uwStartAddress, |
uint8_t * | pData, | ||
uint32_t | uwDataSize | ||
) |
Writes block of data to the FLASH.
In this function, the number of WRITE cycles are reduced, using Page WRITE sequence.
< uwStartAddress is FLASH_SPI_PAGESIZE aligned
< uwDataSize < FLASH_SPI_PAGESIZE
< uwDataSize > FLASH_SPI_PAGESIZE
< uwStartAddress is not FLASH_SPI_PAGESIZE aligned
< uwDataSize < FLASH_SPI_PAGESIZE
< (uwDataSize + uwStartAddress) > FLASH_SPI_PAGESIZE
< uwDataSize > BSP_SERIAL_FLASH_PAGESIZE
Definition at line 215 of file stm3210e_eval_serialflash.c.
References BSP_SERIAL_FLASH_WritePage(), FLASH_ERROR, FLASH_OK, and FLASH_SPI_PAGESIZE.
uint8_t BSP_SERIAL_FLASH_WritePage | ( | uint32_t | uwStartAddress, |
uint8_t * | pData, | ||
uint32_t | uwDataSize | ||
) |
Writes more than one byte to the FLASH with a single WRITE cycle (Page WRITE sequence).
- Note:
- The number of byte can't exceed the FLASH page size (FLASH_SPI_PAGESIZE).
< Select the FLASH and send "Write Enable" instruction
< Send "Write to Memory " instruction
< Send uwStartAddress high nibble address byte to write to
< Send uwStartAddress medium nibble address byte to write to
< Send uwStartAddress low nibble address byte to write to
< while there is data to be written on the FLASH
< Send the current byte
< Point on the next byte to be written
Definition at line 171 of file stm3210e_eval_serialflash.c.
References FLASH_ERROR, FLASH_OK, FLASH_SPI_CMD_WRITE, FLASH_SPI_IO_WaitForWriteEnd(), FLASH_SPI_IO_WriteByte(), and FLASH_SPI_IO_WriteEnable().
Referenced by BSP_SERIAL_FLASH_WriteData().
HAL_StatusTypeDef FLASH_SPI_IO_Init | ( | void | ) |
Initializes the FLASH SPI and put it into StandBy State (Ready for data transfer).
Definition at line 1084 of file stm3210e_eval.c.
References FLASH_SPI_CS_GPIO_CLK_ENABLE, FLASH_SPI_CS_GPIO_PORT, FLASH_SPI_CS_HIGH, FLASH_SPI_CS_PIN, and SPIx_Init().
Referenced by BSP_SERIAL_FLASH_Init().
uint8_t FLASH_SPI_IO_ReadByte | ( | void | ) |
Read a byte from the FLASH SPI.
Definition at line 1124 of file stm3210e_eval.c.
References SPIx_Read().
HAL_StatusTypeDef FLASH_SPI_IO_ReadData | ( | uint32_t | MemAddress, |
uint8_t * | pBuffer, | ||
uint32_t | BufferSize | ||
) |
Read data from FLASH SPI driver.
< Select the FLASH: Chip Select low
< Send "Read from Memory " instruction
< Send ReadAddr high nibble address byte to read from
< Send ReadAddr medium nibble address byte to read from
< Send ReadAddr low nibble address byte to read from
< while there is data to be read
< Read a byte from the FLASH
< Point to the next location where the byte read will be saved
Definition at line 1142 of file stm3210e_eval.c.
References FLASH_SPI_CMD_READ, FLASH_SPI_CS_HIGH, FLASH_SPI_CS_LOW, FLASH_SPI_DUMMY_BYTE, and SPIx_Write().
Referenced by BSP_SERIAL_FLASH_ReadData().
uint32_t FLASH_SPI_IO_ReadID | ( | void | ) |
Reads FLASH SPI identification.
< Select the FLASH: Chip Select low
< Send "RDID " instruction
< Read a byte from the FLASH
< Read a byte from the FLASH
< Read a byte from the FLASH
< Deselect the FLASH: Chip Select high
Definition at line 1227 of file stm3210e_eval.c.
References FLASH_SPI_CS_HIGH, FLASH_SPI_CS_LOW, FLASH_SPI_DUMMY_BYTE, and SPIx_Write().
Referenced by BSP_SERIAL_FLASH_ReadID().
HAL_StatusTypeDef FLASH_SPI_IO_WaitForWriteEnd | ( | void | ) |
Wait response from the FLASH SPI and Deselect the device.
< Select the FLASH: Chip Select low
< Select the FLASH: Chip Select low
< Send "Read Status Register" instruction
< Loop as long as the memory is busy with a write cycle
< Send a dummy byte to generate the clock needed by the FLASH and put the value of the status register in FLASH_Status variable
< Deselect the FLASH: Chip Select high
Definition at line 1194 of file stm3210e_eval.c.
References FLASH_SPI_CMD_RDSR, FLASH_SPI_CS_HIGH, FLASH_SPI_CS_LOW, FLASH_SPI_DUMMY_BYTE, FLASH_SPI_WIP_FLAG, and SPIx_Write().
Referenced by BSP_SERIAL_FLASH_EraseBulk(), BSP_SERIAL_FLASH_EraseSector(), and BSP_SERIAL_FLASH_WritePage().
uint8_t FLASH_SPI_IO_WriteByte | ( | uint8_t | Data | ) |
Write a byte on the FLASH SPI.
Definition at line 1114 of file stm3210e_eval.c.
References SPIx_Write().
Referenced by BSP_SERIAL_FLASH_EraseBulk(), BSP_SERIAL_FLASH_EraseSector(), and BSP_SERIAL_FLASH_WritePage().
void FLASH_SPI_IO_WriteEnable | ( | void | ) |
Select the FLASH SPI and send "Write Enable" instruction.
< Select the FLASH: Chip Select low
< Send "Write Enable" instruction
< Select the FLASH: Chip Select low
< Select the FLASH: Chip Select low
Definition at line 1175 of file stm3210e_eval.c.
References FLASH_SPI_CMD_WREN, FLASH_SPI_CS_HIGH, FLASH_SPI_CS_LOW, and SPIx_Write().
Referenced by BSP_SERIAL_FLASH_EraseBulk(), BSP_SERIAL_FLASH_EraseSector(), and BSP_SERIAL_FLASH_WritePage().
Generated on Thu Dec 11 2014 16:16:37 for _BSP_User_Manual by
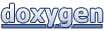