_BSP_User_Manual
|
stm3210e_eval_serialflash.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210e_eval_serialflash.c 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief This file provides a set of functions needed to manage the SPI M25Pxx 00008 * FLASH memory mounted on STM3210E_EVAL board. 00009 * It implements a high level communication layer for read and write 00010 * from/to this memory. The needed STM32 hardware resources (SPI and 00011 * GPIO) are defined in stm3210e_eval.h file, and the initialization is 00012 * performed in FLASH_SPI_IO_Init() function declared in stm3210e_eval.c 00013 * file. 00014 * You can easily tailor this driver to any other development board, 00015 * by just adapting the defines for hardware resources and 00016 * FLASH_SPI_IO_Init() function. 00017 * 00018 * +---------------------------------------------------------------------+ 00019 * | Pin assignment | 00020 * +---------------------------------------+---------------+-------------+ 00021 * | STM32 SPI Pins | BSP_FLASH | Pin | 00022 * +---------------------------------------+---------------+-------------+ 00023 * | BSP_SERIAL_FLASH_CS_PIN | ChipSelect(/S)| 1 | 00024 * | BSP_SERIAL_FLASH_SPI_MISO_PIN / MISO | DataOut(Q) | 2 | 00025 * | | VCC | 3 (3.3 V)| 00026 * | | GND | 4 (0 V) | 00027 * | BSP_SERIAL_FLASH_SPI_MOSI_PIN / MOSI | DataIn(D) | 5 | 00028 * | BSP_SERIAL_FLASH_SPI_SCK_PIN / SCLK | Clock(C) | 6 | 00029 * | | VCC | 7 (3.3 V)| 00030 * | | VCC | 8 (3.3 V)| 00031 * +---------------------------------------+---------------+-------------+ 00032 ****************************************************************************** 00033 * @attention 00034 * 00035 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00036 * 00037 * Redistribution and use in source and binary forms, with or without modification, 00038 * are permitted provided that the following conditions are met: 00039 * 1. Redistributions of source code must retain the above copyright notice, 00040 * this list of conditions and the following disclaimer. 00041 * 2. Redistributions in binary form must reproduce the above copyright notice, 00042 * this list of conditions and the following disclaimer in the documentation 00043 * and/or other materials provided with the distribution. 00044 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00045 * may be used to endorse or promote products derived from this software 00046 * without specific prior written permission. 00047 * 00048 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00049 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00050 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00051 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00052 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00053 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00054 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00055 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00056 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00057 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00058 * 00059 ****************************************************************************** 00060 */ 00061 00062 /* Includes ------------------------------------------------------------------*/ 00063 #include "stm3210e_eval_serialflash.h" 00064 00065 /** @addtogroup BSP 00066 * @{ 00067 */ 00068 00069 /** @addtogroup STM3210E_EVAL 00070 * @{ 00071 */ 00072 00073 /** @defgroup STM3210E_EVAL_SERIAL_FLASH STM3210E_EVAL Serial FLASH 00074 * @brief This file includes the M25Pxxx SPI FLASH driver of 00075 * STM3210E-EVAL board. 00076 * @{ 00077 */ 00078 00079 00080 00081 /** @defgroup STM3210E_EVAL_SERIAL_FLASH_Exported_Functions Exported_Functions 00082 * @{ 00083 */ 00084 00085 /** 00086 * @brief Initializes peripherals used by the Serial FLASH device. 00087 * @retval FLASH_OK (0x00) if operation is correctly performed, else 00088 * return FLASH_ERROR (0x01). 00089 */ 00090 uint8_t BSP_SERIAL_FLASH_Init(void) 00091 { 00092 if(FLASH_SPI_IO_Init() != HAL_OK) 00093 { 00094 return FLASH_ERROR; 00095 } 00096 else 00097 { 00098 return FLASH_OK; 00099 } 00100 } 00101 00102 /** 00103 * @brief Erases the specified FLASH sector. 00104 * @param SectorAddr: address of the sector to erase. 00105 * @retval FLASH_OK (0x00) if operation is correctly performed, else 00106 * return FLASH_ERROR (0x01). 00107 */ 00108 uint8_t BSP_SERIAL_FLASH_EraseSector(uint32_t SectorAddr) 00109 { 00110 /*!< Sector Erase */ 00111 /*!< Select the FLASH and send "Write Enable" instruction */ 00112 FLASH_SPI_IO_WriteEnable(); 00113 00114 /*!< Send Sector Erase instruction */ 00115 FLASH_SPI_IO_WriteByte(FLASH_SPI_CMD_SE); 00116 /*!< Send SectorAddr high nibble address byte */ 00117 FLASH_SPI_IO_WriteByte((SectorAddr & 0xFF0000) >> 16); 00118 /*!< Send SectorAddr medium nibble address byte */ 00119 FLASH_SPI_IO_WriteByte((SectorAddr & 0xFF00) >> 8); 00120 /*!< Send SectorAddr low nibble address byte */ 00121 FLASH_SPI_IO_WriteByte(SectorAddr & 0xFF); 00122 00123 /*!< Wait the end of Flash writing and Deselect the FLASH*/ 00124 if(FLASH_SPI_IO_WaitForWriteEnd()!= HAL_OK) 00125 { 00126 return FLASH_ERROR; 00127 } 00128 else 00129 { 00130 return FLASH_OK; 00131 } 00132 } 00133 00134 /** 00135 * @brief Erases the entire FLASH. 00136 * @retval FLASH_OK (0x00) if operation is correctly performed, else 00137 * return FLASH_ERROR (0x01). 00138 */ 00139 uint8_t BSP_SERIAL_FLASH_EraseBulk(void) 00140 { 00141 /*!< Bulk Erase */ 00142 /*!< Select the FLASH and send "Write Enable" instruction */ 00143 FLASH_SPI_IO_WriteEnable(); 00144 00145 /*!< Send Bulk Erase instruction */ 00146 FLASH_SPI_IO_WriteByte(FLASH_SPI_CMD_BE); 00147 00148 /*!< Wait the end of Flash writing and Deselect the FLASH*/ 00149 if(FLASH_SPI_IO_WaitForWriteEnd()!= HAL_OK) 00150 { 00151 return FLASH_ERROR; 00152 } 00153 else 00154 { 00155 return FLASH_OK; 00156 } 00157 } 00158 00159 /** 00160 * @brief Writes more than one byte to the FLASH with a single WRITE cycle 00161 * (Page WRITE sequence). 00162 * @note The number of byte can't exceed the FLASH page size (FLASH_SPI_PAGESIZE). 00163 * @param pData: pointer to the buffer containing the data to be written 00164 * to the FLASH. 00165 * @param uwStartAddress: FLASH's internal address to write to. 00166 * @param uwDataSize: number of bytes to write to the FLASH, must be equal 00167 * or less than "FLASH_SPI_PAGESIZE" value. 00168 * @retval FLASH_OK (0x00) if operation is correctly performed, else 00169 * return FLASH_ERROR (0x01). 00170 */ 00171 uint8_t BSP_SERIAL_FLASH_WritePage(uint32_t uwStartAddress, uint8_t* pData, uint32_t uwDataSize) 00172 { 00173 /*!< Select the FLASH and send "Write Enable" instruction */ 00174 FLASH_SPI_IO_WriteEnable(); 00175 00176 /*!< Send "Write to Memory " instruction */ 00177 FLASH_SPI_IO_WriteByte(FLASH_SPI_CMD_WRITE); 00178 /*!< Send uwStartAddress high nibble address byte to write to */ 00179 FLASH_SPI_IO_WriteByte((uwStartAddress & 0xFF0000) >> 16); 00180 /*!< Send uwStartAddress medium nibble address byte to write to */ 00181 FLASH_SPI_IO_WriteByte((uwStartAddress & 0xFF00) >> 8); 00182 /*!< Send uwStartAddress low nibble address byte to write to */ 00183 FLASH_SPI_IO_WriteByte(uwStartAddress & 0xFF); 00184 00185 /*!< while there is data to be written on the FLASH */ 00186 while (uwDataSize--) 00187 { 00188 /*!< Send the current byte */ 00189 FLASH_SPI_IO_WriteByte(*pData); 00190 /*!< Point on the next byte to be written */ 00191 pData++; 00192 } 00193 00194 /*!< Wait the end of Flash writing */ 00195 if (FLASH_SPI_IO_WaitForWriteEnd()!= HAL_OK) 00196 { 00197 return FLASH_ERROR; 00198 } 00199 else 00200 { 00201 return FLASH_OK; 00202 } 00203 } 00204 00205 /** 00206 * @brief Writes block of data to the FLASH. In this function, the number of 00207 * WRITE cycles are reduced, using Page WRITE sequence. 00208 * @param pData: pointer to the buffer containing the data to be written 00209 * to the FLASH. 00210 * @param uwStartAddress: FLASH's internal address to write to. 00211 * @param uwDataSize: number of bytes to write to the FLASH. 00212 * @retval FLASH_OK (0x00) if operation is correctly performed, else 00213 * return FLASH_ERROR (0x01). 00214 */ 00215 uint8_t BSP_SERIAL_FLASH_WriteData(uint32_t uwStartAddress, uint8_t* pData, uint32_t uwDataSize) 00216 { 00217 uint8_t NumOfPage = 0, NumOfSingle = 0, Addr = 0, count = 0, temp = 0, status = 0; 00218 00219 Addr = uwStartAddress % FLASH_SPI_PAGESIZE; 00220 count = FLASH_SPI_PAGESIZE - Addr; 00221 NumOfPage = uwDataSize / FLASH_SPI_PAGESIZE; 00222 NumOfSingle = uwDataSize % FLASH_SPI_PAGESIZE; 00223 00224 if (Addr == 0) /*!< uwStartAddress is FLASH_SPI_PAGESIZE aligned */ 00225 { 00226 if (NumOfPage == 0) /*!< uwDataSize < FLASH_SPI_PAGESIZE */ 00227 { 00228 status = BSP_SERIAL_FLASH_WritePage(uwStartAddress, pData, uwDataSize); 00229 } 00230 else /*!< uwDataSize > FLASH_SPI_PAGESIZE */ 00231 { 00232 while (NumOfPage--) 00233 { 00234 status = BSP_SERIAL_FLASH_WritePage(uwStartAddress, pData, FLASH_SPI_PAGESIZE); 00235 uwStartAddress += FLASH_SPI_PAGESIZE; 00236 pData += FLASH_SPI_PAGESIZE; 00237 } 00238 00239 status = BSP_SERIAL_FLASH_WritePage(uwStartAddress, pData, NumOfSingle); 00240 } 00241 } 00242 else /*!< uwStartAddress is not FLASH_SPI_PAGESIZE aligned */ 00243 { 00244 if (NumOfPage == 0) /*!< uwDataSize < FLASH_SPI_PAGESIZE */ 00245 { 00246 if (NumOfSingle > count) /*!< (uwDataSize + uwStartAddress) > FLASH_SPI_PAGESIZE */ 00247 { 00248 temp = NumOfSingle - count; 00249 00250 status = BSP_SERIAL_FLASH_WritePage(uwStartAddress, pData, count); 00251 uwStartAddress += count; 00252 pData += count; 00253 00254 status = BSP_SERIAL_FLASH_WritePage(uwStartAddress, pData, temp); 00255 } 00256 else 00257 { 00258 status = BSP_SERIAL_FLASH_WritePage(uwStartAddress, pData, uwDataSize); 00259 } 00260 } 00261 else /*!< uwDataSize > BSP_SERIAL_FLASH_PAGESIZE */ 00262 { 00263 uwDataSize -= count; 00264 NumOfPage = uwDataSize / FLASH_SPI_PAGESIZE; 00265 NumOfSingle = uwDataSize % FLASH_SPI_PAGESIZE; 00266 00267 status = BSP_SERIAL_FLASH_WritePage(uwStartAddress, pData, count); 00268 uwStartAddress += count; 00269 pData += count; 00270 00271 while (NumOfPage--) 00272 { 00273 status = BSP_SERIAL_FLASH_WritePage(uwStartAddress, pData, FLASH_SPI_PAGESIZE); 00274 uwStartAddress += FLASH_SPI_PAGESIZE; 00275 pData += FLASH_SPI_PAGESIZE; 00276 } 00277 00278 if (NumOfSingle != 0) 00279 { 00280 status = BSP_SERIAL_FLASH_WritePage(uwStartAddress, pData, NumOfSingle); 00281 } 00282 } 00283 } 00284 if (status!= HAL_OK) 00285 { 00286 return FLASH_ERROR; 00287 } 00288 else 00289 { 00290 return FLASH_OK; 00291 } 00292 } 00293 00294 /** 00295 * @brief Reads a block of data from the FLASH. 00296 * @param pData: pointer to the buffer that receives the data read from the FLASH. 00297 * @param uwStartAddress: FLASH's internal address to read from. 00298 * @param uwDataSize: number of bytes to read from the FLASH. 00299 * @retval FLASH_OK (0x00) if operation is correctly performed, else 00300 * return FLASH_ERROR (0x01). 00301 */ 00302 uint8_t BSP_SERIAL_FLASH_ReadData(uint32_t uwStartAddress, uint8_t* pData, uint32_t uwDataSize) 00303 { 00304 if(FLASH_SPI_IO_ReadData(uwStartAddress, pData, uwDataSize)!= HAL_OK) 00305 { 00306 return FLASH_ERROR; 00307 } 00308 else 00309 { 00310 return FLASH_OK; 00311 } 00312 } 00313 00314 00315 /** 00316 * @brief Reads FLASH identification. 00317 * @retval FLASH identification 00318 */ 00319 uint32_t BSP_SERIAL_FLASH_ReadID(void) 00320 { 00321 return(FLASH_SPI_IO_ReadID()); 00322 } 00323 00324 00325 /** 00326 * @} 00327 */ 00328 00329 /** 00330 * @} 00331 */ 00332 00333 /** 00334 * @} 00335 */ 00336 00337 /** 00338 * @} 00339 */ 00340 00341 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Dec 11 2014 16:16:37 for _BSP_User_Manual by
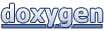