_BSP_User_Manual
|
stm3210e_eval.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210e_eval.c 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief This file provides a set of firmware functions to manage Leds, 00008 * push-button and COM ports for STM3210E_EVAL 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Includes ------------------------------------------------------------------*/ 00040 #include "stm3210e_eval.h" 00041 00042 /** @addtogroup BSP 00043 * @{ 00044 */ 00045 00046 /** @defgroup STM3210E_EVAL STM3210E-EVAL 00047 * @{ 00048 */ 00049 00050 /** @defgroup STM3210E_EVAL_Common STM3210E-EVAL Common 00051 * @{ 00052 */ 00053 00054 /** @defgroup STM3210E_EVAL_Private_TypesDefinitions Private Types Definitions 00055 * @{ 00056 */ 00057 00058 typedef struct 00059 { 00060 __IO uint16_t REG; 00061 __IO uint16_t RAM; 00062 00063 }LCD_CONTROLLER_TypeDef; 00064 00065 /** 00066 * @} 00067 */ 00068 00069 /** @defgroup STM3210E_EVAL_Private_Defines Private Defines 00070 * @{ 00071 */ 00072 00073 /** 00074 * @brief STM3210E EVAL BSP Driver version number V2.0.0 00075 */ 00076 #define __STM3210E_EVAL_BSP_VERSION_MAIN (0x02) /*!< [31:24] main version */ 00077 #define __STM3210E_EVAL_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00078 #define __STM3210E_EVAL_BSP_VERSION_SUB2 (0x00) /*!< [15:8] sub2 version */ 00079 #define __STM3210E_EVAL_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00080 #define __STM3210E_EVAL_BSP_VERSION ((__STM3210E_EVAL_BSP_VERSION_MAIN << 24)\ 00081 |(__STM3210E_EVAL_BSP_VERSION_SUB1 << 16)\ 00082 |(__STM3210E_EVAL_BSP_VERSION_SUB2 << 8 )\ 00083 |(__STM3210E_EVAL_BSP_VERSION_RC)) 00084 00085 00086 /* Note: LCD /CS is CE4 - Bank 4 of NOR/SRAM Bank 1~4 */ 00087 #define TFT_LCD_BASE FSMC_BANK1_4 00088 #define TFT_LCD ((LCD_CONTROLLER_TypeDef *) TFT_LCD_BASE) 00089 00090 /** 00091 * @} 00092 */ 00093 00094 00095 /** @defgroup STM3210E_EVAL_Private_Variables Private Variables 00096 * @{ 00097 */ 00098 /** 00099 * @brief LED variables 00100 */ 00101 GPIO_TypeDef* LED_PORT[LEDn] = {LED1_GPIO_PORT, 00102 LED2_GPIO_PORT, 00103 LED3_GPIO_PORT, 00104 LED4_GPIO_PORT}; 00105 00106 const uint16_t LED_PIN[LEDn] = {LED1_PIN, 00107 LED2_PIN, 00108 LED3_PIN, 00109 LED4_PIN}; 00110 00111 /** 00112 * @brief BUTTON variables 00113 */ 00114 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {WAKEUP_BUTTON_GPIO_PORT, 00115 TAMPER_BUTTON_GPIO_PORT, 00116 KEY_BUTTON_GPIO_PORT, 00117 SEL_JOY_GPIO_PORT, 00118 LEFT_JOY_GPIO_PORT, 00119 RIGHT_JOY_GPIO_PORT, 00120 DOWN_JOY_GPIO_PORT, 00121 UP_JOY_GPIO_PORT}; 00122 00123 const uint16_t BUTTON_PIN[BUTTONn] = {WAKEUP_BUTTON_PIN, 00124 TAMPER_BUTTON_PIN, 00125 KEY_BUTTON_PIN, 00126 SEL_JOY_PIN, 00127 LEFT_JOY_PIN, 00128 RIGHT_JOY_PIN, 00129 DOWN_JOY_PIN, 00130 UP_JOY_PIN}; 00131 00132 const uint8_t BUTTON_IRQn[BUTTONn] = {WAKEUP_BUTTON_EXTI_IRQn, 00133 TAMPER_BUTTON_EXTI_IRQn, 00134 KEY_BUTTON_EXTI_IRQn, 00135 SEL_JOY_EXTI_IRQn, 00136 LEFT_JOY_EXTI_IRQn, 00137 RIGHT_JOY_EXTI_IRQn, 00138 DOWN_JOY_EXTI_IRQn, 00139 UP_JOY_EXTI_IRQn}; 00140 00141 /** 00142 * @brief JOYSTICK variables 00143 */ 00144 GPIO_TypeDef* JOY_PORT[JOYn] = {SEL_JOY_GPIO_PORT, 00145 LEFT_JOY_GPIO_PORT, 00146 RIGHT_JOY_GPIO_PORT, 00147 DOWN_JOY_GPIO_PORT, 00148 UP_JOY_GPIO_PORT}; 00149 00150 const uint16_t JOY_PIN[JOYn] = {SEL_JOY_PIN, 00151 LEFT_JOY_PIN, 00152 RIGHT_JOY_PIN, 00153 DOWN_JOY_PIN, 00154 UP_JOY_PIN}; 00155 00156 const uint8_t JOY_IRQn[JOYn] = {SEL_JOY_EXTI_IRQn, 00157 LEFT_JOY_EXTI_IRQn, 00158 RIGHT_JOY_EXTI_IRQn, 00159 DOWN_JOY_EXTI_IRQn, 00160 UP_JOY_EXTI_IRQn}; 00161 00162 /** 00163 * @brief COM variables 00164 */ 00165 USART_TypeDef* COM_USART[COMn] = {EVAL_COM1, EVAL_COM2}; 00166 00167 GPIO_TypeDef* COM_TX_PORT[COMn] = {EVAL_COM1_TX_GPIO_PORT, EVAL_COM2_TX_GPIO_PORT}; 00168 00169 GPIO_TypeDef* COM_RX_PORT[COMn] = {EVAL_COM1_RX_GPIO_PORT, EVAL_COM2_RX_GPIO_PORT}; 00170 00171 const uint16_t COM_TX_PIN[COMn] = {EVAL_COM1_TX_PIN, EVAL_COM2_TX_PIN}; 00172 00173 const uint16_t COM_RX_PIN[COMn] = {EVAL_COM1_RX_PIN, EVAL_COM2_RX_PIN}; 00174 00175 /** 00176 * @brief BUS variables 00177 */ 00178 #ifdef HAL_SPI_MODULE_ENABLED 00179 uint32_t SpixTimeout = EVAL_SPIx_TIMEOUT_MAX; /*<! Value of Timeout when SPI communication fails */ 00180 static SPI_HandleTypeDef heval_Spi; 00181 #endif /* HAL_SPI_MODULE_ENABLED */ 00182 00183 #ifdef HAL_I2C_MODULE_ENABLED 00184 uint32_t I2cxTimeout = EVAL_I2Cx_TIMEOUT_MAX; /*<! Value of Timeout when I2C communication fails */ 00185 I2C_HandleTypeDef heval_I2c; 00186 #endif /* HAL_I2C_MODULE_ENABLED */ 00187 00188 /** 00189 * @} 00190 */ 00191 00192 #if defined(HAL_SRAM_MODULE_ENABLED) 00193 00194 static void FSMC_BANK1NORSRAM4_WriteData(uint16_t Data); 00195 static void FSMC_BANK1NORSRAM4_WriteReg(uint8_t Reg); 00196 static uint16_t FSMC_BANK1NORSRAM4_ReadData(uint8_t Reg); 00197 static void FSMC_BANK1NORSRAM4_Init(void); 00198 static void FSMC_BANK1NORSRAM4_MspInit(void); 00199 00200 /* LCD IO functions */ 00201 void LCD_IO_Init(void); 00202 void LCD_IO_WriteData(uint16_t RegValue); 00203 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size); 00204 void LCD_IO_WriteReg(uint8_t Reg); 00205 uint16_t LCD_IO_ReadData(uint16_t Reg); 00206 void LCD_Delay (uint32_t delay); 00207 #endif /*HAL_SRAM_MODULE_ENABLED*/ 00208 00209 /* I2Cx bus function */ 00210 #ifdef HAL_I2C_MODULE_ENABLED 00211 /* Link function for I2C EEPROM peripheral */ 00212 static void I2Cx_Init(void); 00213 static void I2Cx_WriteData(uint16_t Addr, uint8_t Reg, uint8_t Value); 00214 static HAL_StatusTypeDef I2Cx_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00215 static uint8_t I2Cx_ReadData(uint16_t Addr, uint8_t Reg); 00216 static HAL_StatusTypeDef I2Cx_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00217 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00218 static void I2Cx_Error (void); 00219 static void I2Cx_MspInit(I2C_HandleTypeDef *hi2c); 00220 00221 /* Link functions for Temperature Sensor peripheral */ 00222 void TSENSOR_IO_Init(void); 00223 void TSENSOR_IO_Write(uint16_t DevAddress, uint8_t* pBuffer, uint8_t WriteAddr, uint16_t Length); 00224 void TSENSOR_IO_Read(uint16_t DevAddress, uint8_t* pBuffer, uint8_t ReadAddr, uint16_t Length); 00225 uint16_t TSENSOR_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00226 00227 /* Link function for Audio peripheral */ 00228 void AUDIO_IO_Init(void); 00229 void AUDIO_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00230 uint8_t AUDIO_IO_Read(uint8_t Addr, uint8_t Reg); 00231 00232 #endif /* HAL_I2C_MODULE_ENABLED */ 00233 00234 #ifdef HAL_SPI_MODULE_ENABLED 00235 /* SPIx bus function */ 00236 static HAL_StatusTypeDef SPIx_Init(void); 00237 static uint8_t SPIx_Write(uint8_t Value); 00238 static uint8_t SPIx_Read(void); 00239 static void SPIx_Error (void); 00240 static void SPIx_MspInit(SPI_HandleTypeDef *hspi); 00241 00242 /* Link function for EEPROM peripheral over SPI */ 00243 HAL_StatusTypeDef FLASH_SPI_IO_Init(void); 00244 uint8_t FLASH_SPI_IO_WriteByte(uint8_t Data); 00245 uint8_t FLASH_SPI_IO_ReadByte(void); 00246 HAL_StatusTypeDef FLASH_SPI_IO_ReadData(uint32_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00247 void FLASH_SPI_IO_WriteEnable(void); 00248 HAL_StatusTypeDef FLASH_SPI_IO_WaitForWriteEnd(void); 00249 uint32_t FLASH_SPI_IO_ReadID(void); 00250 #endif /* HAL_SPI_MODULE_ENABLED */ 00251 00252 /** @defgroup STM3210E_EVAL_Exported_Functions Exported Functions 00253 * @{ 00254 */ 00255 00256 /** 00257 * @brief This method returns the STM3210E EVAL BSP Driver revision 00258 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00259 */ 00260 uint32_t BSP_GetVersion(void) 00261 { 00262 return __STM3210E_EVAL_BSP_VERSION; 00263 } 00264 00265 /** 00266 * @brief Configures LED GPIO. 00267 * @param Led: Specifies the Led to be configured. 00268 * This parameter can be one of following parameters: 00269 * @arg LED1 00270 * @arg LED2 00271 * @arg LED3 00272 * @arg LED4 00273 * @retval None 00274 */ 00275 void BSP_LED_Init(Led_TypeDef Led) 00276 { 00277 GPIO_InitTypeDef gpioinitstruct = {0}; 00278 00279 /* Enable the GPIO_LED clock */ 00280 LEDx_GPIO_CLK_ENABLE(Led); 00281 00282 /* Configure the GPIO_LED pin */ 00283 gpioinitstruct.Pin = LED_PIN[Led]; 00284 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 00285 gpioinitstruct.Pull = GPIO_NOPULL; 00286 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00287 00288 HAL_GPIO_Init(LED_PORT[Led], &gpioinitstruct); 00289 00290 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00291 } 00292 00293 /** 00294 * @brief Turns selected LED On. 00295 * @param Led: Specifies the Led to be set on. 00296 * This parameter can be one of following parameters: 00297 * @arg LED1 00298 * @arg LED2 00299 * @arg LED3 00300 * @arg LED4 00301 * @retval None 00302 */ 00303 void BSP_LED_On(Led_TypeDef Led) 00304 { 00305 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00306 } 00307 00308 /** 00309 * @brief Turns selected LED Off. 00310 * @param Led: Specifies the Led to be set off. 00311 * This parameter can be one of following parameters: 00312 * @arg LED1 00313 * @arg LED2 00314 * @arg LED3 00315 * @arg LED4 00316 * @retval None 00317 */ 00318 void BSP_LED_Off(Led_TypeDef Led) 00319 { 00320 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00321 } 00322 00323 /** 00324 * @brief Toggles the selected LED. 00325 * @param Led: Specifies the Led to be toggled. 00326 * This parameter can be one of following parameters: 00327 * @arg LED1 00328 * @arg LED2 00329 * @arg LED3 00330 * @arg LED4 00331 * @retval None 00332 */ 00333 void BSP_LED_Toggle(Led_TypeDef Led) 00334 { 00335 HAL_GPIO_TogglePin(LED_PORT[Led], LED_PIN[Led]); 00336 } 00337 00338 /** 00339 * @brief Configures push button GPIO and EXTI Line. 00340 * @param Button: Button to be configured. 00341 * This parameter can be one of the following values: 00342 * @arg BUTTON_TAMPER: Key/Tamper Push Button 00343 * @arg BUTTON_SEL : Sel Push Button on Joystick 00344 * @arg BUTTON_LEFT : Left Push Button on Joystick 00345 * @arg BUTTON_RIGHT : Right Push Button on Joystick 00346 * @arg BUTTON_DOWN : Down Push Button on Joystick 00347 * @arg BUTTON_UP : Up Push Button on Joystick 00348 * @param Button_Mode: Button mode requested. 00349 * This parameter can be one of the following values: 00350 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00351 * @arg BUTTON_MODE_EVT : Button will be connected to EXTI line 00352 * with event generation capability 00353 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00354 * with interrupt generation capability 00355 * @retval None 00356 */ 00357 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Button_Mode) 00358 { 00359 GPIO_InitTypeDef gpioinitstruct = {0}; 00360 00361 /* Enable the corresponding Push Button clock */ 00362 BUTTONx_GPIO_CLK_ENABLE(Button); 00363 00364 /* Configure Push Button pin as input */ 00365 gpioinitstruct.Pin = BUTTON_PIN[Button]; 00366 gpioinitstruct.Pull = GPIO_PULLDOWN; 00367 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00368 00369 if (Button_Mode == BUTTON_MODE_GPIO) 00370 { 00371 /* Configure Button pin as input */ 00372 gpioinitstruct.Mode = GPIO_MODE_INPUT; 00373 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00374 } 00375 else if (Button_Mode == BUTTON_MODE_EXTI) 00376 { 00377 if(Button != BUTTON_WAKEUP) 00378 { 00379 /* Configure Joystick Button pin as input with External interrupt, falling edge */ 00380 gpioinitstruct.Mode = GPIO_MODE_IT_FALLING; 00381 } 00382 else 00383 { 00384 /* Configure Key Push Button pin as input with External interrupt, rising edge */ 00385 gpioinitstruct.Mode = GPIO_MODE_IT_RISING; 00386 } 00387 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00388 00389 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00390 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0); 00391 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00392 } 00393 else if (Button_Mode == BUTTON_MODE_EVT) 00394 { 00395 if(Button != BUTTON_WAKEUP) 00396 { 00397 /* Configure Joystick Button pin as input with External interrupt, falling edge */ 00398 gpioinitstruct.Mode = GPIO_MODE_EVT_FALLING; 00399 } 00400 else 00401 { 00402 /* Configure Key Push Button pin as input with External interrupt, rising edge */ 00403 gpioinitstruct.Mode = GPIO_MODE_EVT_RISING; 00404 } 00405 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00406 } 00407 } 00408 00409 /** 00410 * @brief Returns the selected button state. 00411 * @param Button: Button to be checked. 00412 * This parameter can be one of the following values: 00413 * @arg BUTTON_TAMPER: Key/Tamper Push Button 00414 * @retval Button state 00415 */ 00416 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00417 { 00418 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00419 } 00420 00421 /** 00422 * @brief Configures all button of the joystick in GPIO or EXTI modes. 00423 * @param Joy_Mode: Joystick mode. 00424 * This parameter can be one of the following values: 00425 * @arg JOY_MODE_GPIO: Joystick pins will be used as simple IOs 00426 * @arg JOY_MODE_EXTI: Joystick pins will be connected to EXTI line 00427 * with interrupt generation capability 00428 * @retval HAL_OK: if all initializations are OK. Other value if error. 00429 */ 00430 uint8_t BSP_JOY_Init(JOYMode_TypeDef Joy_Mode) 00431 { 00432 JOYState_TypeDef joykey = JOY_NONE; 00433 GPIO_InitTypeDef gpioinitstruct = {0}; 00434 00435 /* Initialized the Joystick. */ 00436 for(joykey = JOY_SEL; joykey < (JOY_SEL+JOYn) ; joykey++) 00437 { 00438 /* Enable the JOY clock */ 00439 JOYx_GPIO_CLK_ENABLE(joykey); 00440 00441 gpioinitstruct.Pin = JOY_PIN[joykey]; 00442 gpioinitstruct.Pull = GPIO_NOPULL; 00443 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00444 00445 if (Joy_Mode == JOY_MODE_GPIO) 00446 { 00447 /* Configure Joy pin as input */ 00448 gpioinitstruct.Mode = GPIO_MODE_INPUT; 00449 HAL_GPIO_Init(JOY_PORT[joykey], &gpioinitstruct); 00450 } 00451 00452 if (Joy_Mode == JOY_MODE_EXTI) 00453 { 00454 /* Configure Joy pin as input with External interrupt */ 00455 gpioinitstruct.Mode = GPIO_MODE_IT_FALLING; 00456 HAL_GPIO_Init(JOY_PORT[joykey], &gpioinitstruct); 00457 00458 /* Enable and set Joy EXTI Interrupt to the lowest priority */ 00459 HAL_NVIC_SetPriority((IRQn_Type)(JOY_IRQn[joykey]), 0x0F, 0); 00460 HAL_NVIC_EnableIRQ((IRQn_Type)(JOY_IRQn[joykey])); 00461 } 00462 } 00463 00464 return HAL_OK; 00465 } 00466 00467 /** 00468 * @brief Returns the current joystick status. 00469 * @retval Code of the joystick key pressed 00470 * This code can be one of the following values: 00471 * @arg JOY_SEL 00472 * @arg JOY_DOWN 00473 * @arg JOY_LEFT 00474 * @arg JOY_RIGHT 00475 * @arg JOY_UP 00476 * @arg JOY_NONE 00477 */ 00478 JOYState_TypeDef BSP_JOY_GetState(void) 00479 { 00480 JOYState_TypeDef joykey = JOY_NONE; 00481 00482 for(joykey = JOY_SEL; joykey < (JOY_SEL+JOYn) ; joykey++) 00483 { 00484 if(HAL_GPIO_ReadPin(JOY_PORT[joykey], JOY_PIN[joykey]) == GPIO_PIN_RESET) 00485 { 00486 /* Return Code Joystick key pressed */ 00487 return joykey; 00488 } 00489 } 00490 00491 /* No Joystick key pressed */ 00492 return JOY_NONE; 00493 } 00494 00495 #ifdef HAL_UART_MODULE_ENABLED 00496 /** 00497 * @brief Configures COM port. 00498 * @param COM: Specifies the COM port to be configured. 00499 * This parameter can be one of following parameters: 00500 * @arg COM1 00501 * @param huart: pointer to a UART_HandleTypeDef structure that 00502 * contains the configuration information for the specified UART peripheral. 00503 * @retval None 00504 */ 00505 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef* huart) 00506 { 00507 GPIO_InitTypeDef gpioinitstruct = {0}; 00508 00509 /* Enable GPIO clock */ 00510 COMx_TX_GPIO_CLK_ENABLE(COM); 00511 COMx_RX_GPIO_CLK_ENABLE(COM); 00512 00513 /* Enable USART clock */ 00514 COMx_CLK_ENABLE(COM); 00515 00516 /* Configure USART Tx as alternate function push-pull */ 00517 gpioinitstruct.Pin = COM_TX_PIN[COM]; 00518 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00519 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00520 gpioinitstruct.Pull = GPIO_PULLUP; 00521 HAL_GPIO_Init(COM_TX_PORT[COM], &gpioinitstruct); 00522 00523 /* Configure USART Rx as alternate function push-pull */ 00524 gpioinitstruct.Mode = GPIO_MODE_INPUT; 00525 gpioinitstruct.Pin = COM_RX_PIN[COM]; 00526 HAL_GPIO_Init(COM_RX_PORT[COM], &gpioinitstruct); 00527 00528 /* USART configuration */ 00529 huart->Instance = COM_USART[COM]; 00530 HAL_UART_Init(huart); 00531 } 00532 #endif /* HAL_UART_MODULE_ENABLED */ 00533 00534 /** 00535 * @} 00536 */ 00537 00538 /** @defgroup STM3210E_EVAL_BusOperations_Functions Bus Operations Functions 00539 * @{ 00540 */ 00541 00542 /******************************************************************************* 00543 BUS OPERATIONS 00544 *******************************************************************************/ 00545 00546 /*************************** FSMC Routines ************************************/ 00547 #if defined(HAL_SRAM_MODULE_ENABLED) 00548 /** 00549 * @brief Initializes FSMC_BANK4 MSP. 00550 * @retval None 00551 */ 00552 static void FSMC_BANK1NORSRAM4_MspInit(void) 00553 { 00554 GPIO_InitTypeDef gpioinitstruct = {0}; 00555 00556 /* Enable FMC clock */ 00557 __HAL_RCC_FSMC_CLK_ENABLE(); 00558 00559 /* Enable GPIOs clock */ 00560 __HAL_RCC_GPIOD_CLK_ENABLE(); 00561 __HAL_RCC_GPIOE_CLK_ENABLE(); 00562 __HAL_RCC_GPIOF_CLK_ENABLE(); 00563 __HAL_RCC_GPIOG_CLK_ENABLE(); 00564 __HAL_RCC_AFIO_CLK_ENABLE(); 00565 00566 /* Common GPIO configuration */ 00567 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00568 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00569 00570 /* Set PD.00(D2), PD.01(D3), PD.04(NOE), PD.05(NWE), PD.08(D13), PD.09(D14), 00571 PD.10(D15), PD.14(D0), PD.15(D1) as alternate function push pull */ 00572 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_4 | GPIO_PIN_5 | 00573 GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_14 | 00574 GPIO_PIN_15; 00575 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00576 00577 /* Set PE.07(D4), PE.08(D5), PE.09(D6), PE.10(D7), PE.11(D8), PE.12(D9), PE.13(D10), 00578 PE.14(D11), PE.15(D12) as alternate function push pull */ 00579 gpioinitstruct.Pin = GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | 00580 GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | 00581 GPIO_PIN_15; 00582 HAL_GPIO_Init(GPIOE, &gpioinitstruct); 00583 00584 /* Set PF.00(A0 (RS)) as alternate function push pull */ 00585 gpioinitstruct.Pin = GPIO_PIN_0; 00586 HAL_GPIO_Init(GPIOF, &gpioinitstruct); 00587 00588 /* Set PG.12(NE4 (LCD/CS)) as alternate function push pull - CE3(LCD /CS) */ 00589 gpioinitstruct.Pin = GPIO_PIN_12; 00590 HAL_GPIO_Init(GPIOG, &gpioinitstruct); 00591 } 00592 00593 /** 00594 * @brief Initializes LCD IO. 00595 * @retval None 00596 */ 00597 static void FSMC_BANK1NORSRAM4_Init(void) 00598 { 00599 SRAM_HandleTypeDef hsram; 00600 FSMC_NORSRAM_TimingTypeDef sramtiming = {0}; 00601 00602 /*** Configure the SRAM Bank 4 ***/ 00603 /* Configure IPs */ 00604 hsram.Instance = FSMC_NORSRAM_DEVICE; 00605 hsram.Extended = FSMC_NORSRAM_EXTENDED_DEVICE; 00606 00607 sramtiming.AddressSetupTime = 1; 00608 sramtiming.AddressHoldTime = 1; 00609 sramtiming.DataSetupTime = 2; 00610 sramtiming.BusTurnAroundDuration = 1; 00611 sramtiming.CLKDivision = 2; 00612 sramtiming.DataLatency = 2; 00613 sramtiming.AccessMode = FSMC_ACCESS_MODE_A; 00614 00615 /* Color LCD configuration 00616 LCD configured as follow: 00617 - Data/Address MUX = Disable 00618 - Memory Type = SRAM 00619 - Data Width = 16bit 00620 - Write Operation = Enable 00621 - Extended Mode = Enable 00622 - Asynchronous Wait = Disable */ 00623 hsram.Init.NSBank = FSMC_NORSRAM_BANK4; 00624 hsram.Init.DataAddressMux = FSMC_DATA_ADDRESS_MUX_DISABLE; 00625 hsram.Init.MemoryType = FSMC_MEMORY_TYPE_SRAM; 00626 hsram.Init.MemoryDataWidth = FSMC_NORSRAM_MEM_BUS_WIDTH_16; 00627 hsram.Init.BurstAccessMode = FSMC_BURST_ACCESS_MODE_DISABLE; 00628 hsram.Init.WaitSignalPolarity = FSMC_WAIT_SIGNAL_POLARITY_LOW; 00629 hsram.Init.WrapMode = FSMC_WRAP_MODE_DISABLE; 00630 hsram.Init.WaitSignalActive = FSMC_WAIT_TIMING_BEFORE_WS; 00631 hsram.Init.WriteOperation = FSMC_WRITE_OPERATION_ENABLE; 00632 hsram.Init.WaitSignal = FSMC_WAIT_SIGNAL_DISABLE; 00633 hsram.Init.ExtendedMode = FSMC_EXTENDED_MODE_DISABLE; 00634 hsram.Init.AsynchronousWait = FSMC_ASYNCHRONOUS_WAIT_DISABLE; 00635 hsram.Init.WriteBurst = FSMC_WRITE_BURST_DISABLE; 00636 00637 /* Initialize the SRAM controller */ 00638 FSMC_BANK1NORSRAM4_MspInit(); 00639 HAL_SRAM_Init(&hsram, &sramtiming, &sramtiming); 00640 } 00641 00642 /** 00643 * @brief Writes register value. 00644 * @param Data: 00645 * @retval None 00646 */ 00647 static void FSMC_BANK1NORSRAM4_WriteData(uint16_t Data) 00648 { 00649 /* Write 16-bit Data */ 00650 TFT_LCD->RAM = Data; 00651 } 00652 00653 /** 00654 * @brief Writes register address. 00655 * @param Reg: 00656 * @retval None 00657 */ 00658 static void FSMC_BANK1NORSRAM4_WriteReg(uint8_t Reg) 00659 { 00660 /* Write 16-bit Index, then Write Reg */ 00661 TFT_LCD->REG = Reg; 00662 } 00663 00664 /** 00665 * @brief Reads register value. 00666 * @retval Read value 00667 */ 00668 static uint16_t FSMC_BANK1NORSRAM4_ReadData(uint8_t Reg) 00669 { 00670 /* Write 16-bit Index (then Read Reg) */ 00671 TFT_LCD->REG = Reg; 00672 /* Read 16-bit Reg */ 00673 return (TFT_LCD->RAM); 00674 } 00675 #endif /*HAL_SRAM_MODULE_ENABLED*/ 00676 00677 #ifdef HAL_I2C_MODULE_ENABLED 00678 /******************************* I2C Routines**********************************/ 00679 00680 /** 00681 * @brief Eval I2Cx MSP Initialization 00682 * @param hi2c: I2C handle 00683 * @retval None 00684 */ 00685 static void I2Cx_MspInit(I2C_HandleTypeDef *hi2c) 00686 { 00687 GPIO_InitTypeDef gpioinitstruct = {0}; 00688 00689 if (hi2c->Instance == EVAL_I2Cx) 00690 { 00691 /*## Configure the GPIOs ################################################*/ 00692 00693 /* Enable GPIO clock */ 00694 EVAL_I2Cx_SDA_GPIO_CLK_ENABLE(); 00695 EVAL_I2Cx_SCL_GPIO_CLK_ENABLE(); 00696 00697 /* Configure I2C Tx as alternate function */ 00698 gpioinitstruct.Pin = EVAL_I2Cx_SCL_PIN; 00699 gpioinitstruct.Mode = GPIO_MODE_AF_OD; 00700 gpioinitstruct.Pull = GPIO_NOPULL; 00701 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00702 HAL_GPIO_Init(EVAL_I2Cx_SCL_GPIO_PORT, &gpioinitstruct); 00703 00704 /* Configure I2C Rx as alternate function */ 00705 gpioinitstruct.Pin = EVAL_I2Cx_SDA_PIN; 00706 HAL_GPIO_Init(EVAL_I2Cx_SDA_GPIO_PORT, &gpioinitstruct); 00707 00708 00709 /*## Configure the Eval I2Cx peripheral #######################################*/ 00710 /* Enable Eval_I2Cx clock */ 00711 EVAL_I2Cx_CLK_ENABLE(); 00712 00713 /* Add delay related to RCC workaround */ 00714 while (READ_BIT(RCC->APB1ENR, RCC_APB1ENR_I2C1EN) != RCC_APB1ENR_I2C1EN) {}; 00715 00716 /* Force the I2C Periheral Clock Reset */ 00717 EVAL_I2Cx_FORCE_RESET(); 00718 00719 /* Release the I2C Periheral Clock Reset */ 00720 EVAL_I2Cx_RELEASE_RESET(); 00721 00722 /* Enable and set Eval I2Cx Interrupt to the highest priority */ 00723 HAL_NVIC_SetPriority(EVAL_I2Cx_EV_IRQn, 5, 0); 00724 HAL_NVIC_EnableIRQ(EVAL_I2Cx_EV_IRQn); 00725 00726 /* Enable and set Eval I2Cx Interrupt to the highest priority */ 00727 HAL_NVIC_SetPriority(EVAL_I2Cx_ER_IRQn, 5, 0); 00728 HAL_NVIC_EnableIRQ(EVAL_I2Cx_ER_IRQn); 00729 } 00730 } 00731 00732 /** 00733 * @brief Eval I2Cx Bus initialization 00734 * @retval None 00735 */ 00736 static void I2Cx_Init(void) 00737 { 00738 if(HAL_I2C_GetState(&heval_I2c) == HAL_I2C_STATE_RESET) 00739 { 00740 heval_I2c.Instance = EVAL_I2Cx; 00741 heval_I2c.Init.ClockSpeed = BSP_I2C_SPEED; 00742 heval_I2c.Init.DutyCycle = I2C_DUTYCYCLE_2; 00743 heval_I2c.Init.OwnAddress1 = 0; 00744 heval_I2c.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00745 heval_I2c.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00746 heval_I2c.Init.OwnAddress2 = 0; 00747 heval_I2c.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00748 heval_I2c.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00749 00750 /* Init the I2C */ 00751 I2Cx_MspInit(&heval_I2c); 00752 HAL_I2C_Init(&heval_I2c); 00753 } 00754 } 00755 00756 /** 00757 * @brief Write a value in a register of the device through BUS. 00758 * @param Addr: Device address on BUS Bus. 00759 * @param Reg: The target register address to write 00760 * @param Value: The target register value to be written 00761 * @retval None 00762 */ 00763 static void I2Cx_WriteData(uint16_t Addr, uint8_t Reg, uint8_t Value) 00764 { 00765 HAL_StatusTypeDef status = HAL_OK; 00766 00767 status = HAL_I2C_Mem_Write(&heval_I2c, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, I2cxTimeout); 00768 00769 /* Check the communication status */ 00770 if(status != HAL_OK) 00771 { 00772 /* Execute user timeout callback */ 00773 I2Cx_Error(); 00774 } 00775 } 00776 00777 /** 00778 * @brief Write a value in a register of the device through BUS. 00779 * @param Addr: Device address on BUS Bus. 00780 * @param Reg: The target register address to write 00781 * @param RegSize: The target register size (can be 8BIT or 16BIT) 00782 * @param pBuffer: The target register value to be written 00783 * @param Length: buffer size to be written 00784 * @retval None 00785 */ 00786 static HAL_StatusTypeDef I2Cx_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00787 { 00788 HAL_StatusTypeDef status = HAL_OK; 00789 00790 status = HAL_I2C_Mem_Write(&heval_I2c, Addr, (uint16_t)Reg, RegSize, pBuffer, Length, I2cxTimeout); 00791 00792 /* Check the communication status */ 00793 if(status != HAL_OK) 00794 { 00795 /* Re-Initiaize the BUS */ 00796 I2Cx_Error(); 00797 } 00798 return status; 00799 } 00800 00801 /** 00802 * @brief Read a value in a register of the device through BUS. 00803 * @param Addr: Device address on BUS Bus. 00804 * @param Reg: The target register address to write 00805 * @retval Data read at register @ 00806 */ 00807 static uint8_t I2Cx_ReadData(uint16_t Addr, uint8_t Reg) 00808 { 00809 HAL_StatusTypeDef status = HAL_OK; 00810 uint8_t value = 0; 00811 00812 status = HAL_I2C_Mem_Read(&heval_I2c, Addr, Reg, I2C_MEMADD_SIZE_8BIT, &value, 1, I2cxTimeout); 00813 00814 /* Check the communication status */ 00815 if(status != HAL_OK) 00816 { 00817 /* Execute user timeout callback */ 00818 I2Cx_Error(); 00819 00820 } 00821 return value; 00822 } 00823 00824 /** 00825 * @brief Reads multiple data on the BUS. 00826 * @param Addr: I2C Address 00827 * @param Reg: Reg Address 00828 * @param RegSize : The target register size (can be 8BIT or 16BIT) 00829 * @param pBuffer: pointer to read data buffer 00830 * @param Length: length of the data 00831 * @retval 0 if no problems to read multiple data 00832 */ 00833 static HAL_StatusTypeDef I2Cx_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00834 { 00835 HAL_StatusTypeDef status = HAL_OK; 00836 00837 status = HAL_I2C_Mem_Read(&heval_I2c, Addr, (uint16_t)Reg, RegSize, pBuffer, Length, I2cxTimeout); 00838 00839 /* Check the communication status */ 00840 if(status != HAL_OK) 00841 { 00842 /* Re-Initiaize the BUS */ 00843 I2Cx_Error(); 00844 } 00845 return status; 00846 } 00847 00848 /** 00849 * @brief Checks if target device is ready for communication. 00850 * @note This function is used with Memory devices 00851 * @param DevAddress: Target device address 00852 * @param Trials: Number of trials 00853 * @retval HAL status 00854 */ 00855 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00856 { 00857 return (HAL_I2C_IsDeviceReady(&heval_I2c, DevAddress, Trials, I2cxTimeout)); 00858 } 00859 00860 00861 /** 00862 * @brief Eval I2Cx error treatment function 00863 * @retval None 00864 */ 00865 static void I2Cx_Error (void) 00866 { 00867 /* De-initialize the I2C communication BUS */ 00868 HAL_I2C_DeInit(&heval_I2c); 00869 00870 /* Re- Initiaize the I2C communication BUS */ 00871 I2Cx_Init(); 00872 } 00873 00874 #endif /* HAL_I2C_MODULE_ENABLED */ 00875 00876 /******************************* SPI Routines**********************************/ 00877 #ifdef HAL_SPI_MODULE_ENABLED 00878 /** 00879 * @brief Initializes SPI MSP. 00880 * @retval None 00881 */ 00882 static void SPIx_MspInit(SPI_HandleTypeDef *hspi) 00883 { 00884 GPIO_InitTypeDef gpioinitstruct = {0}; 00885 00886 /*** Configure the GPIOs ***/ 00887 /* Enable GPIO clock */ 00888 EVAL_SPIx_SCK_GPIO_CLK_ENABLE(); 00889 EVAL_SPIx_MISO_MOSI_GPIO_CLK_ENABLE(); 00890 00891 /* configure SPI SCK */ 00892 gpioinitstruct.Pin = EVAL_SPIx_SCK_PIN; 00893 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00894 gpioinitstruct.Pull = GPIO_NOPULL; 00895 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00896 HAL_GPIO_Init(EVAL_SPIx_SCK_GPIO_PORT, &gpioinitstruct); 00897 00898 /* configure SPI MISO and MOSI */ 00899 gpioinitstruct.Pin = (EVAL_SPIx_MISO_PIN | EVAL_SPIx_MOSI_PIN); 00900 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00901 gpioinitstruct.Pull = GPIO_NOPULL; 00902 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00903 HAL_GPIO_Init(EVAL_SPIx_MISO_MOSI_GPIO_PORT, &gpioinitstruct); 00904 00905 /*** Configure the SPI peripheral ***/ 00906 /* Enable SPI clock */ 00907 EVAL_SPIx_CLK_ENABLE(); 00908 } 00909 00910 /** 00911 * @brief Initializes SPI HAL. 00912 * @retval None 00913 */ 00914 HAL_StatusTypeDef SPIx_Init(void) 00915 { 00916 /* DeInitializes the SPI peripheral */ 00917 heval_Spi.Instance = EVAL_SPIx; 00918 HAL_SPI_DeInit(&heval_Spi); 00919 00920 /* SPI Config */ 00921 /* SPI baudrate is set to 36 MHz (PCLK2/SPI_BaudRatePrescaler = 72/2 = 36 MHz) */ 00922 heval_Spi.Init.BaudRatePrescaler = SPI_BAUDRATEPRESCALER_2; 00923 heval_Spi.Init.Direction = SPI_DIRECTION_2LINES; 00924 heval_Spi.Init.CLKPhase = SPI_PHASE_1EDGE; 00925 heval_Spi.Init.CLKPolarity = SPI_POLARITY_LOW; 00926 heval_Spi.Init.CRCCalculation = SPI_CRCCALCULATION_DISABLE; 00927 heval_Spi.Init.CRCPolynomial = 7; 00928 heval_Spi.Init.DataSize = SPI_DATASIZE_8BIT; 00929 heval_Spi.Init.FirstBit = SPI_FIRSTBIT_MSB; 00930 heval_Spi.Init.NSS = SPI_NSS_SOFT; 00931 heval_Spi.Init.TIMode = SPI_TIMODE_DISABLE; 00932 heval_Spi.Init.Mode = SPI_MODE_MASTER; 00933 00934 SPIx_MspInit(&heval_Spi); 00935 00936 return (HAL_SPI_Init(&heval_Spi)); 00937 } 00938 00939 00940 /** 00941 * @brief SPI Write a byte to device 00942 * @param WriteValue to be written 00943 * @retval The value of the received byte. 00944 */ 00945 static uint8_t SPIx_Write(uint8_t WriteValue) 00946 { 00947 HAL_StatusTypeDef status = HAL_OK; 00948 uint8_t ReadValue = 0; 00949 00950 status = HAL_SPI_TransmitReceive(&heval_Spi, (uint8_t*) &WriteValue, (uint8_t*) &ReadValue, 1, SpixTimeout); 00951 00952 /* Check the communication status */ 00953 if(status != HAL_OK) 00954 { 00955 /* Execute user timeout callback */ 00956 SPIx_Error(); 00957 } 00958 00959 return ReadValue; 00960 } 00961 00962 00963 /** 00964 * @brief SPI Read 1 byte from device 00965 * @retval Read data 00966 */ 00967 static uint8_t SPIx_Read(void) 00968 { 00969 return (SPIx_Write(FLASH_SPI_DUMMY_BYTE)); 00970 } 00971 00972 00973 /** 00974 * @brief SPI error treatment function 00975 * @retval None 00976 */ 00977 static void SPIx_Error (void) 00978 { 00979 /* De-initialize the SPI communication BUS */ 00980 HAL_SPI_DeInit(&heval_Spi); 00981 00982 /* Re- Initiaize the SPI communication BUS */ 00983 SPIx_Init(); 00984 } 00985 #endif /* HAL_SPI_MODULE_ENABLED */ 00986 00987 /** 00988 * @} 00989 */ 00990 00991 /** @defgroup STM3210E_EVAL_LinkOperations_Functions Link Operations Functions 00992 * @{ 00993 */ 00994 00995 /******************************************************************************* 00996 LINK OPERATIONS 00997 *******************************************************************************/ 00998 00999 #if defined(HAL_SRAM_MODULE_ENABLED) 01000 /********************************* LINK LCD ***********************************/ 01001 01002 /** 01003 * @brief Initializes LCD low level. 01004 * @retval None 01005 */ 01006 void LCD_IO_Init(void) 01007 { 01008 FSMC_BANK1NORSRAM4_Init(); 01009 } 01010 01011 /** 01012 * @brief Writes data on LCD data register. 01013 * @param RegValue: Data to be written 01014 * @retval None 01015 */ 01016 void LCD_IO_WriteData(uint16_t RegValue) 01017 { 01018 FSMC_BANK1NORSRAM4_WriteData(RegValue); 01019 } 01020 01021 /** 01022 * @brief Writes multiple data on LCD data register. 01023 * @param pData: Data to be written 01024 * @param Size: number of data to write 01025 * @retval None 01026 */ 01027 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size) 01028 { 01029 uint32_t counter = 0; 01030 uint16_t regvalue; 01031 01032 regvalue = *pData | (*(pData+1) << 8); 01033 01034 for (counter = Size; counter != 0; counter--) 01035 { 01036 /* Write 16-bit Reg */ 01037 FSMC_BANK1NORSRAM4_WriteData(regvalue); 01038 counter--; 01039 pData += 2; 01040 regvalue = *pData | (*(pData+1) << 8); 01041 } 01042 } 01043 01044 /** 01045 * @brief Writes register on LCD register. 01046 * @param Reg: Register to be written 01047 * @retval None 01048 */ 01049 void LCD_IO_WriteReg(uint8_t Reg) 01050 { 01051 FSMC_BANK1NORSRAM4_WriteReg(Reg); 01052 } 01053 01054 /** 01055 * @brief Reads data from LCD data register. 01056 * @param Reg: Register to be read 01057 * @retval Read data. 01058 */ 01059 uint16_t LCD_IO_ReadData(uint16_t Reg) 01060 { 01061 /* Read 16-bit Reg */ 01062 return (FSMC_BANK1NORSRAM4_ReadData(Reg)); 01063 } 01064 01065 /** 01066 * @brief Wait for loop in ms. 01067 * @param Delay in ms. 01068 * @retval None 01069 */ 01070 void LCD_Delay (uint32_t Delay) 01071 { 01072 HAL_Delay(Delay); 01073 } 01074 01075 #endif /*HAL_SRAM_MODULE_ENABLED*/ 01076 01077 #ifdef HAL_SPI_MODULE_ENABLED 01078 /******************************** LINK FLASH SPI ********************************/ 01079 /** 01080 * @brief Initializes the FLASH SPI and put it into StandBy State (Ready for 01081 * data transfer). 01082 * @retval None 01083 */ 01084 HAL_StatusTypeDef FLASH_SPI_IO_Init(void) 01085 { 01086 HAL_StatusTypeDef Status = HAL_OK; 01087 01088 GPIO_InitTypeDef gpioinitstruct = {0}; 01089 01090 /* EEPROM_CS_GPIO Periph clock enable */ 01091 FLASH_SPI_CS_GPIO_CLK_ENABLE(); 01092 01093 /* Configure EEPROM_CS_PIN pin: EEPROM SPI CS pin */ 01094 gpioinitstruct.Pin = FLASH_SPI_CS_PIN; 01095 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 01096 gpioinitstruct.Pull = GPIO_PULLUP; 01097 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 01098 HAL_GPIO_Init(FLASH_SPI_CS_GPIO_PORT, &gpioinitstruct); 01099 01100 /* SPI FLASH Config */ 01101 Status = SPIx_Init(); 01102 01103 /* EEPROM chip select high */ 01104 FLASH_SPI_CS_HIGH(); 01105 01106 return Status; 01107 } 01108 01109 /** 01110 * @brief Write a byte on the FLASH SPI. 01111 * @param Data: byte to send. 01112 * @retval None 01113 */ 01114 uint8_t FLASH_SPI_IO_WriteByte(uint8_t Data) 01115 { 01116 /* Send the byte */ 01117 return (SPIx_Write(Data)); 01118 } 01119 01120 /** 01121 * @brief Read a byte from the FLASH SPI. 01122 * @retval uint8_t (The received byte). 01123 */ 01124 uint8_t FLASH_SPI_IO_ReadByte(void) 01125 { 01126 uint8_t data = 0; 01127 01128 /* Get the received data */ 01129 data = SPIx_Read(); 01130 01131 /* Return the shifted data */ 01132 return data; 01133 } 01134 01135 /** 01136 * @brief Read data from FLASH SPI driver 01137 * @param MemAddress: Internal memory address 01138 * @param pBuffer: Pointer to data buffer 01139 * @param BufferSize: Amount of data to be read 01140 * @retval HAL_StatusTypeDef HAL Status 01141 */ 01142 HAL_StatusTypeDef FLASH_SPI_IO_ReadData(uint32_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01143 { 01144 /*!< Select the FLASH: Chip Select low */ 01145 FLASH_SPI_CS_LOW(); 01146 01147 /*!< Send "Read from Memory " instruction */ 01148 SPIx_Write(FLASH_SPI_CMD_READ); 01149 01150 /*!< Send ReadAddr high nibble address byte to read from */ 01151 SPIx_Write((MemAddress & 0xFF0000) >> 16); 01152 /*!< Send ReadAddr medium nibble address byte to read from */ 01153 SPIx_Write((MemAddress& 0xFF00) >> 8); 01154 /*!< Send ReadAddr low nibble address byte to read from */ 01155 SPIx_Write(MemAddress & 0xFF); 01156 01157 while (BufferSize--) /*!< while there is data to be read */ 01158 { 01159 /*!< Read a byte from the FLASH */ 01160 *pBuffer = SPIx_Write(FLASH_SPI_DUMMY_BYTE); 01161 /*!< Point to the next location where the byte read will be saved */ 01162 pBuffer++; 01163 } 01164 01165 /*!< Deselect the FLASH: Chip Select high */ 01166 FLASH_SPI_CS_HIGH(); 01167 01168 return HAL_OK; 01169 } 01170 01171 /** 01172 * @brief Select the FLASH SPI and send "Write Enable" instruction 01173 * @retval None 01174 */ 01175 void FLASH_SPI_IO_WriteEnable(void) 01176 { 01177 /*!< Select the FLASH: Chip Select low */ 01178 FLASH_SPI_CS_LOW(); 01179 01180 /*!< Send "Write Enable" instruction */ 01181 SPIx_Write(FLASH_SPI_CMD_WREN); 01182 01183 /*!< Select the FLASH: Chip Select low */ 01184 FLASH_SPI_CS_HIGH(); 01185 01186 /*!< Select the FLASH: Chip Select low */ 01187 FLASH_SPI_CS_LOW(); 01188 } 01189 01190 /** 01191 * @brief Wait response from the FLASH SPI and Deselect the device 01192 * @retval HAL_StatusTypeDef HAL Status 01193 */ 01194 HAL_StatusTypeDef FLASH_SPI_IO_WaitForWriteEnd(void) 01195 { 01196 /*!< Select the FLASH: Chip Select low */ 01197 FLASH_SPI_CS_HIGH(); 01198 01199 /*!< Select the FLASH: Chip Select low */ 01200 FLASH_SPI_CS_LOW(); 01201 01202 uint8_t flashstatus = 0; 01203 01204 /*!< Send "Read Status Register" instruction */ 01205 SPIx_Write(FLASH_SPI_CMD_RDSR); 01206 01207 /*!< Loop as long as the memory is busy with a write cycle */ 01208 do 01209 { 01210 /*!< Send a dummy byte to generate the clock needed by the FLASH 01211 and put the value of the status register in FLASH_Status variable */ 01212 flashstatus = SPIx_Write(FLASH_SPI_DUMMY_BYTE); 01213 01214 } 01215 while ((flashstatus & FLASH_SPI_WIP_FLAG) == SET); /* Write in progress */ 01216 01217 /*!< Deselect the FLASH: Chip Select high */ 01218 FLASH_SPI_CS_HIGH(); 01219 01220 return HAL_OK; 01221 } 01222 01223 /** 01224 * @brief Reads FLASH SPI identification. 01225 * @retval FLASH identification 01226 */ 01227 uint32_t FLASH_SPI_IO_ReadID(void) 01228 { 01229 uint32_t Temp = 0, Temp0 = 0, Temp1 = 0, Temp2 = 0; 01230 01231 /*!< Select the FLASH: Chip Select low */ 01232 FLASH_SPI_CS_LOW(); 01233 01234 /*!< Send "RDID " instruction */ 01235 SPIx_Write(0x9F); 01236 01237 /*!< Read a byte from the FLASH */ 01238 Temp0 = SPIx_Write(FLASH_SPI_DUMMY_BYTE); 01239 01240 /*!< Read a byte from the FLASH */ 01241 Temp1 = SPIx_Write(FLASH_SPI_DUMMY_BYTE); 01242 01243 /*!< Read a byte from the FLASH */ 01244 Temp2 = SPIx_Write(FLASH_SPI_DUMMY_BYTE); 01245 01246 /*!< Deselect the FLASH: Chip Select high */ 01247 FLASH_SPI_CS_HIGH(); 01248 01249 Temp = (Temp0 << 16) | (Temp1 << 8) | Temp2; 01250 01251 return Temp; 01252 } 01253 #endif /* HAL_SPI_MODULE_ENABLED */ 01254 01255 #ifdef HAL_I2C_MODULE_ENABLED 01256 /********************************* LINK I2C TEMPERATURE SENSOR *****************************/ 01257 /** 01258 * @brief Initializes peripherals used by the I2C Temperature Sensor driver. 01259 * @retval None 01260 */ 01261 void TSENSOR_IO_Init(void) 01262 { 01263 I2Cx_Init(); 01264 } 01265 01266 /** 01267 * @brief Writes one byte to the TSENSOR. 01268 * @param DevAddress: Target device address 01269 * @param pBuffer: Pointer to data buffer 01270 * @param WriteAddr: TSENSOR's internal address to write to. 01271 * @param Length: Number of data to write 01272 * @retval None 01273 */ 01274 void TSENSOR_IO_Write(uint16_t DevAddress, uint8_t* pBuffer, uint8_t WriteAddr, uint16_t Length) 01275 { 01276 I2Cx_WriteBuffer(DevAddress, WriteAddr, I2C_MEMADD_SIZE_8BIT, pBuffer, Length); 01277 } 01278 01279 /** 01280 * @brief Reads one byte from the TSENSOR. 01281 * @param DevAddress: Target device address 01282 * @param pBuffer : pointer to the buffer that receives the data read from the TSENSOR. 01283 * @param ReadAddr : TSENSOR's internal address to read from. 01284 * @param Length: Number of data to read 01285 * @retval None 01286 */ 01287 void TSENSOR_IO_Read(uint16_t DevAddress, uint8_t* pBuffer, uint8_t ReadAddr, uint16_t Length) 01288 { 01289 I2Cx_ReadBuffer(DevAddress, ReadAddr, I2C_MEMADD_SIZE_8BIT, pBuffer, Length); 01290 } 01291 01292 /** 01293 * @brief Checks if Temperature Sensor is ready for communication. 01294 * @param DevAddress: Target device address 01295 * @param Trials: Number of trials 01296 * @retval HAL status 01297 */ 01298 uint16_t TSENSOR_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01299 { 01300 return (I2Cx_IsDeviceReady(DevAddress, Trials)); 01301 } 01302 01303 /********************************* LINK AUDIO ***********************************/ 01304 01305 /** 01306 * @brief Initializes Audio low level. 01307 * @retval None 01308 */ 01309 void AUDIO_IO_Init (void) 01310 { 01311 GPIO_InitTypeDef gpioinitstruct = {0}; 01312 01313 /* Enable Reset GPIO Clock */ 01314 AUDIO_RESET_GPIO_CLK_ENABLE(); 01315 01316 /* Audio reset pin configuration -------------------------------------------------*/ 01317 gpioinitstruct.Pin = AUDIO_RESET_PIN; 01318 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 01319 gpioinitstruct.Speed = GPIO_SPEED_MEDIUM; 01320 gpioinitstruct.Pull = GPIO_NOPULL; 01321 HAL_GPIO_Init(AUDIO_RESET_GPIO, &gpioinitstruct); 01322 01323 I2Cx_Init(); 01324 01325 /* Power Down the codec */ 01326 HAL_GPIO_WritePin(AUDIO_RESET_GPIO, AUDIO_RESET_PIN, GPIO_PIN_RESET); 01327 01328 /* wait for a delay to insure registers erasing */ 01329 HAL_Delay(5); 01330 01331 /* Power on the codec */ 01332 HAL_GPIO_WritePin(AUDIO_RESET_GPIO, AUDIO_RESET_PIN, GPIO_PIN_SET); 01333 01334 /* wait for a delay to insure registers erasing */ 01335 HAL_Delay(5); 01336 } 01337 01338 /** 01339 * @brief Writes a single data. 01340 * @param Addr: I2C address 01341 * @param Reg: Reg address 01342 * @param Value: Data to be written 01343 * @retval None 01344 */ 01345 void AUDIO_IO_Write (uint8_t Addr, uint8_t Reg, uint8_t Value) 01346 { 01347 I2Cx_WriteData(Addr, Reg, Value); 01348 } 01349 01350 /** 01351 * @brief Reads a single data. 01352 * @param Addr: I2C address 01353 * @param Reg: Reg address 01354 * @retval Data to be read 01355 */ 01356 uint8_t AUDIO_IO_Read (uint8_t Addr, uint8_t Reg) 01357 { 01358 return I2Cx_ReadData(Addr, Reg); 01359 } 01360 01361 #endif /* HAL_I2C_MODULE_ENABLED */ 01362 01363 /** 01364 * @} 01365 */ 01366 01367 /** 01368 * @} 01369 */ 01370 01371 /** 01372 * @} 01373 */ 01374 01375 /** 01376 * @} 01377 */ 01378 01379 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 01380
Generated on Thu Dec 11 2014 16:16:37 for _BSP_User_Manual by
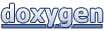