STM3210C_EVAL BSP User Manual
|
stm3210c_eval_lcd.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210c_eval_lcd.h 00004 * @author MCD Application Team 00005 * @version V7.0.0 00006 * @date 14-April-2017 00007 * @brief This file contains the common defines and functions prototypes for 00008 * the stm3210c_eval_lcd.c driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM3210C_EVAL_LCD_H 00041 #define __STM3210C_EVAL_LCD_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 #include "stm3210c_eval.h" 00049 #include "../Components/ili9325/ili9325.h" 00050 #include "../Components/ili9320/ili9320.h" 00051 #include "../../../Utilities/Fonts/fonts.h" 00052 /** @addtogroup BSP 00053 * @{ 00054 */ 00055 00056 /** @addtogroup STM3210C_EVAL 00057 * @{ 00058 */ 00059 00060 /** @addtogroup STM3210C_EVAL_LCD 00061 * @{ 00062 */ 00063 00064 00065 /** @defgroup STM3210C_EVAL_LCD_Exported_Types STM3210C EVAL LCD Exported Types 00066 * @{ 00067 */ 00068 typedef struct 00069 { 00070 uint32_t TextColor; 00071 uint32_t BackColor; 00072 sFONT *pFont; 00073 00074 }LCD_DrawPropTypeDef; 00075 /** 00076 * @} 00077 */ 00078 00079 /** @defgroup STM3210C_EVAL_LCD_Exported_Constants STM3210C EVAL LCD Exported Constants 00080 * @{ 00081 */ 00082 /** 00083 * @brief LCD status structure definition 00084 */ 00085 #define LCD_OK 0x00 00086 #define LCD_ERROR 0x01 00087 #define LCD_TIMEOUT 0x02 00088 00089 typedef struct 00090 { 00091 int16_t X; 00092 int16_t Y; 00093 00094 }Point, * pPoint; 00095 00096 /** 00097 * @brief Line mode structures definition 00098 */ 00099 typedef enum 00100 { 00101 CENTER_MODE = 0x01, /*!< Center mode */ 00102 RIGHT_MODE = 0x02, /*!< Right mode */ 00103 LEFT_MODE = 0x03 /*!< Left mode */ 00104 00105 }Line_ModeTypdef; 00106 00107 /** 00108 * @brief LCD color 00109 */ 00110 #define LCD_COLOR_BLUE 0x001F 00111 #define LCD_COLOR_GREEN 0x07E0 00112 #define LCD_COLOR_RED 0xF800 00113 #define LCD_COLOR_CYAN 0x07FF 00114 #define LCD_COLOR_MAGENTA 0xF81F 00115 #define LCD_COLOR_YELLOW 0xFFE0 00116 #define LCD_COLOR_LIGHTBLUE 0x841F 00117 #define LCD_COLOR_LIGHTGREEN 0x87F0 00118 #define LCD_COLOR_LIGHTRED 0xFC10 00119 #define LCD_COLOR_LIGHTCYAN 0x87FF 00120 #define LCD_COLOR_LIGHTMAGENTA 0xFC1F 00121 #define LCD_COLOR_LIGHTYELLOW 0xFFF0 00122 #define LCD_COLOR_DARKBLUE 0x0010 00123 #define LCD_COLOR_DARKGREEN 0x0400 00124 #define LCD_COLOR_DARKRED 0x8000 00125 #define LCD_COLOR_DARKCYAN 0x0410 00126 #define LCD_COLOR_DARKMAGENTA 0x8010 00127 #define LCD_COLOR_DARKYELLOW 0x8400 00128 #define LCD_COLOR_WHITE 0xFFFF 00129 #define LCD_COLOR_LIGHTGRAY 0xD69A 00130 #define LCD_COLOR_GRAY 0x8410 00131 #define LCD_COLOR_DARKGRAY 0x4208 00132 #define LCD_COLOR_BLACK 0x0000 00133 #define LCD_COLOR_BROWN 0xA145 00134 #define LCD_COLOR_ORANGE 0xFD20 00135 00136 /** 00137 * @brief LCD default font 00138 */ 00139 #define LCD_DEFAULT_FONT Font24 00140 00141 /** 00142 * @} 00143 */ 00144 00145 /** @addtogroup STM3210C_EVAL_LCD_Exported_Functions 00146 * @{ 00147 */ 00148 uint8_t BSP_LCD_Init(void); 00149 uint32_t BSP_LCD_GetXSize(void); 00150 uint32_t BSP_LCD_GetYSize(void); 00151 00152 uint16_t BSP_LCD_GetTextColor(void); 00153 uint16_t BSP_LCD_GetBackColor(void); 00154 void BSP_LCD_SetTextColor(__IO uint16_t Color); 00155 void BSP_LCD_SetBackColor(__IO uint16_t Color); 00156 void BSP_LCD_SetFont(sFONT *fonts); 00157 sFONT *BSP_LCD_GetFont(void); 00158 00159 void BSP_LCD_Clear(uint16_t Color); 00160 void BSP_LCD_ClearStringLine(uint16_t Line); 00161 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *ptr); 00162 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Line_ModeTypdef Mode); 00163 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii); 00164 00165 uint16_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos); 00166 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length); 00167 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length); 00168 void BSP_LCD_DrawLine(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2); 00169 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00170 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius); 00171 void BSP_LCD_DrawPolygon(pPoint Points, uint16_t PointCount); 00172 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius); 00173 void BSP_LCD_DrawBitmap(uint16_t Xpos, uint16_t Ypos, uint8_t *pbmp); 00174 void BSP_LCD_DrawRGBImage(uint16_t Xpos, uint16_t Ypos, uint16_t Xsize, uint16_t Ysize, uint8_t *pbmp); 00175 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00176 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius); 00177 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius); 00178 00179 void BSP_LCD_DisplayOff(void); 00180 void BSP_LCD_DisplayOn(void); 00181 00182 /** 00183 * @} 00184 */ 00185 00186 #ifdef __cplusplus 00187 } 00188 #endif 00189 00190 #endif /* __STM3210C_EVAL_LCD_H */ 00191 /** 00192 * @} 00193 */ 00194 00195 /** 00196 * @} 00197 */ 00198 00199 /** 00200 * @} 00201 */ 00202 00203 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Apr 14 2017 13:00:47 for STM3210C_EVAL BSP User Manual by
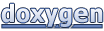