STM3210C_EVAL BSP User Manual
|
stm3210c_eval_lcd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210c_eval_lcd.c 00004 * @author MCD Application Team 00005 * @version V7.0.0 00006 * @date 14-April-2017 00007 * @brief This file includes the driver for Liquid Crystal Display (LCD) module 00008 * mounted on STM3210C-EVAL evaluation board. 00009 @verbatim 00010 ============================================================================== 00011 ##### How to use this driver ##### 00012 ============================================================================== 00013 [..] 00014 (#) This driver is used to drive indirectly an LCD TFT. 00015 00016 (#) This driver supports the AM-240320L8TNQW00H (LCD_ILI9320) and AM240320D5TOQW01H 00017 (LCD_ILI9325) LCD mounted on MB785 daughter board 00018 00019 (#) The ILI9320 and ILI9325 components driver MUST be included with this driver. 00020 00021 (#) Initialization steps: 00022 (++) Initialize the LCD using the BSP_LCD_Init() function. 00023 00024 (#) Display on LCD 00025 (++) Clear the hole LCD using yhe BSP_LCD_Clear() function or only one specified 00026 string line using the BSP_LCD_ClearStringLine() function. 00027 (++) Display a character on the specified line and column using the BSP_LCD_DisplayChar() 00028 function or a complete string line using the BSP_LCD_DisplayStringAtLine() function. 00029 (++) Display a string line on the specified position (x,y in pixel) and align mode 00030 using the BSP_LCD_DisplayStringAtLine() function. 00031 (++) Draw and fill a basic shapes (dot, line, rectangle, circle, ellipse, .. bitmap, raw picture) 00032 on LCD using a set of functions. 00033 @endverbatim 00034 ****************************************************************************** 00035 * @attention 00036 * 00037 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00038 * 00039 * Redistribution and use in source and binary forms, with or without modification, 00040 * are permitted provided that the following conditions are met: 00041 * 1. Redistributions of source code must retain the above copyright notice, 00042 * this list of conditions and the following disclaimer. 00043 * 2. Redistributions in binary form must reproduce the above copyright notice, 00044 * this list of conditions and the following disclaimer in the documentation 00045 * and/or other materials provided with the distribution. 00046 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00047 * may be used to endorse or promote products derived from this software 00048 * without specific prior written permission. 00049 * 00050 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00051 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00052 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00053 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00054 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00055 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00056 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00057 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00058 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00059 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00060 * 00061 ****************************************************************************** 00062 */ 00063 00064 /* Includes ------------------------------------------------------------------*/ 00065 #include "stm3210c_eval_lcd.h" 00066 #include "../../../Utilities/Fonts/fonts.h" 00067 #include "../../../Utilities/Fonts/font24.c" 00068 #include "../../../Utilities/Fonts/font20.c" 00069 #include "../../../Utilities/Fonts/font16.c" 00070 #include "../../../Utilities/Fonts/font12.c" 00071 #include "../../../Utilities/Fonts/font8.c" 00072 00073 /** @addtogroup BSP 00074 * @{ 00075 */ 00076 00077 /** @addtogroup STM3210C_EVAL 00078 * @{ 00079 */ 00080 00081 /** @defgroup STM3210C_EVAL_LCD STM3210C EVAL LCD 00082 * @{ 00083 */ 00084 00085 00086 /** @defgroup STM3210C_EVAL_LCD_Private_Defines STM3210C EVAL LCD Private Defines 00087 * @{ 00088 */ 00089 #define POLY_X(Z) ((int32_t)((Points + (Z))->X)) 00090 #define POLY_Y(Z) ((int32_t)((Points + (Z))->Y)) 00091 00092 #define MAX_HEIGHT_FONT 17 00093 #define MAX_WIDTH_FONT 24 00094 #define OFFSET_BITMAP 54 00095 /** 00096 * @} 00097 */ 00098 00099 /** @defgroup STM3210C_EVAL_LCD_Private_Macros STM3210C EVAL LCD Private Macros 00100 * @{ 00101 */ 00102 #define ABS(X) ((X) > 0 ? (X) : -(X)) 00103 00104 /** 00105 * @} 00106 */ 00107 00108 /** @defgroup STM3210C_EVAL_LCD_Private_Variables STM3210C EVAL LCD Private Variables 00109 * @{ 00110 */ 00111 LCD_DrawPropTypeDef DrawProp; 00112 00113 static LCD_DrvTypeDef *lcd_drv; 00114 00115 /* Max size of bitmap will based on a font24 (17x24) */ 00116 static uint8_t bitmap[MAX_HEIGHT_FONT*MAX_WIDTH_FONT*2+OFFSET_BITMAP] = {0}; 00117 00118 /** 00119 * @} 00120 */ 00121 00122 /** @defgroup STM3210C_EVAL_LCD_Private_Functions STM3210C EVAL LCD Private Functions 00123 * @{ 00124 */ 00125 static void LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint16_t RGBCode); 00126 static void LCD_DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c); 00127 static void LCD_SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00128 /** 00129 * @} 00130 */ 00131 00132 00133 /** @defgroup STM3210C_EVAL_LCD_Exported_Functions STM3210C EVAL LCD Exported Functions 00134 * @{ 00135 */ 00136 00137 /** 00138 * @brief Initializes the LCD. 00139 * @retval LCD state 00140 */ 00141 uint8_t BSP_LCD_Init(void) 00142 { 00143 uint8_t ret = LCD_ERROR; 00144 00145 /* Default value for draw propriety */ 00146 DrawProp.BackColor = 0xFFFF; 00147 DrawProp.pFont = &Font24; 00148 DrawProp.TextColor = 0x0000; 00149 00150 if(ili9320_drv.ReadID() == ILI9320_ID) 00151 { 00152 lcd_drv = &ili9320_drv; 00153 00154 /* LCD Init */ 00155 lcd_drv->Init(); 00156 00157 /* Initialize the font */ 00158 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00159 00160 ret = LCD_OK; 00161 } 00162 else if(ili9325_drv.ReadID() == ILI9325_ID) 00163 { 00164 lcd_drv = &ili9325_drv; 00165 00166 /* LCD Init */ 00167 lcd_drv->Init(); 00168 00169 /* Initialize the font */ 00170 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00171 00172 ret = LCD_OK; 00173 } 00174 00175 return ret; 00176 } 00177 00178 /** 00179 * @brief Gets the LCD X size. 00180 * @retval Used LCD X size 00181 */ 00182 uint32_t BSP_LCD_GetXSize(void) 00183 { 00184 return(lcd_drv->GetLcdPixelWidth()); 00185 } 00186 00187 /** 00188 * @brief Gets the LCD Y size. 00189 * @retval Used LCD Y size 00190 */ 00191 uint32_t BSP_LCD_GetYSize(void) 00192 { 00193 return(lcd_drv->GetLcdPixelHeight()); 00194 } 00195 00196 /** 00197 * @brief Gets the LCD text color. 00198 * @retval Used text color. 00199 */ 00200 uint16_t BSP_LCD_GetTextColor(void) 00201 { 00202 return DrawProp.TextColor; 00203 } 00204 00205 /** 00206 * @brief Gets the LCD background color. 00207 * @retval Used background color 00208 */ 00209 uint16_t BSP_LCD_GetBackColor(void) 00210 { 00211 return DrawProp.BackColor; 00212 } 00213 00214 /** 00215 * @brief Sets the LCD text color. 00216 * @param Color: Text color code RGB(5-6-5) 00217 */ 00218 void BSP_LCD_SetTextColor(uint16_t Color) 00219 { 00220 DrawProp.TextColor = Color; 00221 } 00222 00223 /** 00224 * @brief Sets the LCD background color. 00225 * @param Color: Background color code RGB(5-6-5) 00226 */ 00227 void BSP_LCD_SetBackColor(uint16_t Color) 00228 { 00229 DrawProp.BackColor = Color; 00230 } 00231 00232 /** 00233 * @brief Sets the LCD text font. 00234 * @param pFonts: Font to be used 00235 */ 00236 void BSP_LCD_SetFont(sFONT *pFonts) 00237 { 00238 DrawProp.pFont = pFonts; 00239 } 00240 00241 /** 00242 * @brief Gets the LCD text font. 00243 * @retval Used font 00244 */ 00245 sFONT *BSP_LCD_GetFont(void) 00246 { 00247 return DrawProp.pFont; 00248 } 00249 00250 /** 00251 * @brief Clears the hole LCD. 00252 * @param Color: Color of the background 00253 */ 00254 void BSP_LCD_Clear(uint16_t Color) 00255 { 00256 uint32_t counter = 0; 00257 00258 uint32_t color_backup = DrawProp.TextColor; 00259 DrawProp.TextColor = Color; 00260 00261 for(counter = 0; counter < BSP_LCD_GetYSize(); counter++) 00262 { 00263 BSP_LCD_DrawHLine(0, counter, BSP_LCD_GetXSize()); 00264 } 00265 00266 DrawProp.TextColor = color_backup; 00267 BSP_LCD_SetTextColor(DrawProp.TextColor); 00268 } 00269 00270 /** 00271 * @brief Clears the selected line. 00272 * @param Line: Line to be cleared 00273 * This parameter can be one of the following values: 00274 * @arg 0..9: if the Current fonts is Font16x24 00275 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00276 * @arg 0..29: if the Current fonts is Font8x8 00277 */ 00278 void BSP_LCD_ClearStringLine(uint16_t Line) 00279 { 00280 uint32_t colorbackup = DrawProp.TextColor; 00281 DrawProp.TextColor = DrawProp.BackColor;; 00282 00283 /* Draw a rectangle with background color */ 00284 BSP_LCD_FillRect(0, (Line * DrawProp.pFont->Height), BSP_LCD_GetXSize(), DrawProp.pFont->Height); 00285 00286 DrawProp.TextColor = colorbackup; 00287 BSP_LCD_SetTextColor(DrawProp.TextColor); 00288 } 00289 00290 /** 00291 * @brief Displays one character. 00292 * @param Xpos: Start column address 00293 * @param Ypos: Line where to display the character shape. 00294 * @param Ascii: Character ascii code 00295 * This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E 00296 */ 00297 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) 00298 { 00299 LCD_DrawChar(Xpos, Ypos, &DrawProp.pFont->table[(Ascii-' ') *\ 00300 DrawProp.pFont->Height * ((DrawProp.pFont->Width + 7) / 8)]); 00301 } 00302 00303 /** 00304 * @brief Displays characters on the LCD. 00305 * @param Xpos: X position (in pixel) 00306 * @param Ypos: Y position (in pixel) 00307 * @param pText: Pointer to string to display on LCD 00308 * @param Mode: Display mode 00309 * This parameter can be one of the following values: 00310 * @arg CENTER_MODE 00311 * @arg RIGHT_MODE 00312 * @arg LEFT_MODE 00313 */ 00314 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *pText, Line_ModeTypdef Mode) 00315 { 00316 uint16_t refcolumn = 1, counter = 0; 00317 uint32_t size = 0, xsize = 0; 00318 uint8_t *ptr = pText; 00319 00320 /* Get the text size */ 00321 while (*ptr++) size ++ ; 00322 00323 /* Characters number per line */ 00324 xsize = (BSP_LCD_GetXSize()/DrawProp.pFont->Width); 00325 00326 switch (Mode) 00327 { 00328 case CENTER_MODE: 00329 { 00330 refcolumn = Xpos + ((xsize - size)* DrawProp.pFont->Width) / 2; 00331 break; 00332 } 00333 case LEFT_MODE: 00334 { 00335 refcolumn = Xpos; 00336 break; 00337 } 00338 case RIGHT_MODE: 00339 { 00340 refcolumn = - Xpos + ((xsize - size)*DrawProp.pFont->Width); 00341 break; 00342 } 00343 default: 00344 { 00345 refcolumn = Xpos; 00346 break; 00347 } 00348 } 00349 00350 /* Send the string character by character on lCD */ 00351 while ((*pText != 0) & (((BSP_LCD_GetXSize() - (counter*DrawProp.pFont->Width)) & 0xFFFF) >= DrawProp.pFont->Width)) 00352 { 00353 /* Display one character on LCD */ 00354 BSP_LCD_DisplayChar(refcolumn, Ypos, *pText); 00355 /* Decrement the column position by 16 */ 00356 refcolumn += DrawProp.pFont->Width; 00357 /* Point on the next character */ 00358 pText++; 00359 counter++; 00360 } 00361 } 00362 00363 /** 00364 * @brief Displays a character on the LCD. 00365 * @param Line: Line where to display the character shape 00366 * This parameter can be one of the following values: 00367 * @arg 0..9: if the Current fonts is Font16x24 00368 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00369 * @arg 0..29: if the Current fonts is Font8x8 00370 * @param pText: Pointer to string to display on LCD 00371 */ 00372 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *pText) 00373 { 00374 BSP_LCD_DisplayStringAt(0, LINE(Line),pText, LEFT_MODE); 00375 } 00376 00377 /** 00378 * @brief Reads an LCD pixel. 00379 * @param Xpos: X position 00380 * @param Ypos: Y position 00381 * @retval RGB pixel color 00382 */ 00383 uint16_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos) 00384 { 00385 uint16_t ret = 0; 00386 00387 if(lcd_drv->ReadPixel != NULL) 00388 { 00389 ret = lcd_drv->ReadPixel(Xpos, Ypos); 00390 } 00391 00392 return ret; 00393 } 00394 00395 /** 00396 * @brief Draws an horizontal line. 00397 * @param Xpos: X position 00398 * @param Ypos: Y position 00399 * @param Length: Line length 00400 */ 00401 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00402 { 00403 uint32_t index = 0; 00404 00405 if(lcd_drv->DrawHLine != NULL) 00406 { 00407 lcd_drv->DrawHLine(DrawProp.TextColor, Xpos, Ypos, Length); 00408 } 00409 else 00410 { 00411 for(index = 0; index < Length; index++) 00412 { 00413 LCD_DrawPixel((Xpos + index), Ypos, DrawProp.TextColor); 00414 } 00415 } 00416 } 00417 00418 /** 00419 * @brief Draws a vertical line. 00420 * @param Xpos: X position 00421 * @param Ypos: Y position 00422 * @param Length: Line length 00423 */ 00424 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00425 { 00426 uint32_t index = 0; 00427 00428 if(lcd_drv->DrawVLine != NULL) 00429 { 00430 lcd_drv->DrawVLine(DrawProp.TextColor, Xpos, Ypos, Length); 00431 } 00432 else 00433 { 00434 for(index = 0; index < Length; index++) 00435 { 00436 LCD_DrawPixel(Xpos, Ypos + index, DrawProp.TextColor); 00437 } 00438 } 00439 } 00440 00441 /** 00442 * @brief Draws an uni-line (between two points). 00443 * @param x1: Point 1 X position 00444 * @param y1: Point 1 Y position 00445 * @param x2: Point 2 X position 00446 * @param y2: Point 2 Y position 00447 */ 00448 void BSP_LCD_DrawLine(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2) 00449 { 00450 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00451 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 00452 curpixel = 0; 00453 00454 deltax = ABS(x2 - x1); /* The difference between the x's */ 00455 deltay = ABS(y2 - y1); /* The difference between the y's */ 00456 x = x1; /* Start x off at the first pixel */ 00457 y = y1; /* Start y off at the first pixel */ 00458 00459 if (x2 >= x1) /* The x-values are increasing */ 00460 { 00461 xinc1 = 1; 00462 xinc2 = 1; 00463 } 00464 else /* The x-values are decreasing */ 00465 { 00466 xinc1 = -1; 00467 xinc2 = -1; 00468 } 00469 00470 if (y2 >= y1) /* The y-values are increasing */ 00471 { 00472 yinc1 = 1; 00473 yinc2 = 1; 00474 } 00475 else /* The y-values are decreasing */ 00476 { 00477 yinc1 = -1; 00478 yinc2 = -1; 00479 } 00480 00481 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00482 { 00483 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00484 yinc2 = 0; /* Don't change the y for every iteration */ 00485 den = deltax; 00486 num = deltax / 2; 00487 numadd = deltay; 00488 numpixels = deltax; /* There are more x-values than y-values */ 00489 } 00490 else /* There is at least one y-value for every x-value */ 00491 { 00492 xinc2 = 0; /* Don't change the x for every iteration */ 00493 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00494 den = deltay; 00495 num = deltay / 2; 00496 numadd = deltax; 00497 numpixels = deltay; /* There are more y-values than x-values */ 00498 } 00499 00500 for (curpixel = 0; curpixel <= numpixels; curpixel++) 00501 { 00502 LCD_DrawPixel(x, y, DrawProp.TextColor); /* Draw the current pixel */ 00503 num += numadd; /* Increase the numerator by the top of the fraction */ 00504 if (num >= den) /* Check if numerator >= denominator */ 00505 { 00506 num -= den; /* Calculate the new numerator value */ 00507 x += xinc1; /* Change the x as appropriate */ 00508 y += yinc1; /* Change the y as appropriate */ 00509 } 00510 x += xinc2; /* Change the x as appropriate */ 00511 y += yinc2; /* Change the y as appropriate */ 00512 } 00513 } 00514 00515 /** 00516 * @brief Draws a rectangle. 00517 * @param Xpos: X position 00518 * @param Ypos: Y position 00519 * @param Width: Rectangle width 00520 * @param Height: Rectangle height 00521 */ 00522 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00523 { 00524 /* Draw horizontal lines */ 00525 BSP_LCD_DrawHLine(Xpos, Ypos, Width); 00526 BSP_LCD_DrawHLine(Xpos, (Ypos+ Height), Width); 00527 00528 /* Draw vertical lines */ 00529 BSP_LCD_DrawVLine(Xpos, Ypos, Height); 00530 BSP_LCD_DrawVLine((Xpos + Width), Ypos, Height); 00531 } 00532 00533 /** 00534 * @brief Draws a circle. 00535 * @param Xpos: X position 00536 * @param Ypos: Y position 00537 * @param Radius: Circle radius 00538 */ 00539 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00540 { 00541 int32_t D; /* Decision Variable */ 00542 uint32_t CurX; /* Current X Value */ 00543 uint32_t CurY; /* Current Y Value */ 00544 00545 D = 3 - (Radius << 1); 00546 CurX = 0; 00547 CurY = Radius; 00548 00549 while (CurX <= CurY) 00550 { 00551 LCD_DrawPixel((Xpos + CurX), (Ypos - CurY), DrawProp.TextColor); 00552 00553 LCD_DrawPixel((Xpos - CurX), (Ypos - CurY), DrawProp.TextColor); 00554 00555 LCD_DrawPixel((Xpos + CurY), (Ypos - CurX), DrawProp.TextColor); 00556 00557 LCD_DrawPixel((Xpos - CurY), (Ypos - CurX), DrawProp.TextColor); 00558 00559 LCD_DrawPixel((Xpos + CurX), (Ypos + CurY), DrawProp.TextColor); 00560 00561 LCD_DrawPixel((Xpos - CurX), (Ypos + CurY), DrawProp.TextColor); 00562 00563 LCD_DrawPixel((Xpos + CurY), (Ypos + CurX), DrawProp.TextColor); 00564 00565 LCD_DrawPixel((Xpos - CurY), (Ypos + CurX), DrawProp.TextColor); 00566 00567 /* Initialize the font */ 00568 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00569 00570 if (D < 0) 00571 { 00572 D += (CurX << 2) + 6; 00573 } 00574 else 00575 { 00576 D += ((CurX - CurY) << 2) + 10; 00577 CurY--; 00578 } 00579 CurX++; 00580 } 00581 } 00582 00583 /** 00584 * @brief Draws an poly-line (between many points). 00585 * @param Points: Pointer to the points array 00586 * @param PointCount: Number of points 00587 */ 00588 void BSP_LCD_DrawPolygon(pPoint Points, uint16_t PointCount) 00589 { 00590 int16_t X = 0, Y = 0; 00591 00592 if(PointCount < 2) 00593 { 00594 return; 00595 } 00596 00597 BSP_LCD_DrawLine(Points->X, Points->Y, (Points+PointCount-1)->X, (Points+PointCount-1)->Y); 00598 00599 while(--PointCount) 00600 { 00601 X = Points->X; 00602 Y = Points->Y; 00603 Points++; 00604 BSP_LCD_DrawLine(X, Y, Points->X, Points->Y); 00605 } 00606 00607 } 00608 00609 /** 00610 * @brief Draws an ellipse on LCD. 00611 * @param Xpos: X position 00612 * @param Ypos: Y position 00613 * @param XRadius: Ellipse X radius 00614 * @param YRadius: Ellipse Y radius 00615 */ 00616 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00617 { 00618 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 00619 float K = 0, rad1 = 0, rad2 = 0; 00620 00621 rad1 = XRadius; 00622 rad2 = YRadius; 00623 00624 K = (float)(rad2/rad1); 00625 00626 do { 00627 LCD_DrawPixel((Xpos-(uint16_t)(x/K)), (Ypos+y), DrawProp.TextColor); 00628 LCD_DrawPixel((Xpos+(uint16_t)(x/K)), (Ypos+y), DrawProp.TextColor); 00629 LCD_DrawPixel((Xpos+(uint16_t)(x/K)), (Ypos-y), DrawProp.TextColor); 00630 LCD_DrawPixel((Xpos-(uint16_t)(x/K)), (Ypos-y), DrawProp.TextColor); 00631 00632 e2 = err; 00633 if (e2 <= x) { 00634 err += ++x*2+1; 00635 if (-y == x && e2 <= y) e2 = 0; 00636 } 00637 if (e2 > y) err += ++y*2+1; 00638 } 00639 while (y <= 0); 00640 } 00641 00642 /** 00643 * @brief Draws a bitmap picture (16 bpp). 00644 * @param Xpos: Bmp X position in the LCD 00645 * @param Ypos: Bmp Y position in the LCD 00646 * @param pbmp: Pointer to Bmp picture address. 00647 */ 00648 void BSP_LCD_DrawBitmap(uint16_t Xpos, uint16_t Ypos, uint8_t *pbmp) 00649 { 00650 uint32_t height = 0; 00651 uint32_t width = 0; 00652 00653 00654 /* Read bitmap width */ 00655 width = *(uint16_t *) (pbmp + 18); 00656 width |= (*(uint16_t *) (pbmp + 20)) << 16; 00657 00658 /* Read bitmap height */ 00659 height = *(uint16_t *) (pbmp + 22); 00660 height |= (*(uint16_t *) (pbmp + 24)) << 16; 00661 00662 LCD_SetDisplayWindow(Xpos, Ypos, width, height); 00663 00664 if(lcd_drv->DrawBitmap != NULL) 00665 { 00666 lcd_drv->DrawBitmap(Xpos, Ypos, pbmp); 00667 } 00668 LCD_SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00669 } 00670 00671 /** 00672 * @brief Draws RGB Image (16 bpp). 00673 * @param Xpos: X position in the LCD 00674 * @param Ypos: Y position in the LCD 00675 * @param Xsize: X size in the LCD 00676 * @param Ysize: Y size in the LCD 00677 * @param pdata: Pointer to the RGB Image address. 00678 */ 00679 void BSP_LCD_DrawRGBImage(uint16_t Xpos, uint16_t Ypos, uint16_t Xsize, uint16_t Ysize, uint8_t *pdata) 00680 { 00681 00682 LCD_SetDisplayWindow(Xpos, Ypos, Xsize, Ysize); 00683 00684 if(lcd_drv->DrawRGBImage != NULL) 00685 { 00686 lcd_drv->DrawRGBImage(Xpos, Ypos, Xsize, Ysize, pdata); 00687 } 00688 LCD_SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00689 } 00690 00691 /** 00692 * @brief Draws a full rectangle. 00693 * @param Xpos: X position 00694 * @param Ypos: Y position 00695 * @param Width: Rectangle width 00696 * @param Height: Rectangle height 00697 */ 00698 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00699 { 00700 BSP_LCD_SetTextColor(DrawProp.TextColor); 00701 do 00702 { 00703 BSP_LCD_DrawHLine(Xpos, Ypos++, Width); 00704 } 00705 while(Height--); 00706 } 00707 00708 /** 00709 * @brief Draws a full circle. 00710 * @param Xpos: X position 00711 * @param Ypos: Y position 00712 * @param Radius: Circle radius 00713 */ 00714 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00715 { 00716 int32_t D; /* Decision Variable */ 00717 uint32_t CurX; /* Current X Value */ 00718 uint32_t CurY; /* Current Y Value */ 00719 00720 D = 3 - (Radius << 1); 00721 00722 CurX = 0; 00723 CurY = Radius; 00724 00725 BSP_LCD_SetTextColor(DrawProp.TextColor); 00726 00727 while (CurX <= CurY) 00728 { 00729 if(CurY > 0) 00730 { 00731 BSP_LCD_DrawHLine(Xpos - CurY, Ypos + CurX, 2*CurY); 00732 BSP_LCD_DrawHLine(Xpos - CurY, Ypos - CurX, 2*CurY); 00733 } 00734 00735 if(CurX > 0) 00736 { 00737 BSP_LCD_DrawHLine(Xpos - CurX, Ypos - CurY, 2*CurX); 00738 BSP_LCD_DrawHLine(Xpos - CurX, Ypos + CurY, 2*CurX); 00739 } 00740 if (D < 0) 00741 { 00742 D += (CurX << 2) + 6; 00743 } 00744 else 00745 { 00746 D += ((CurX - CurY) << 2) + 10; 00747 CurY--; 00748 } 00749 CurX++; 00750 } 00751 00752 BSP_LCD_SetTextColor(DrawProp.TextColor); 00753 BSP_LCD_DrawCircle(Xpos, Ypos, Radius); 00754 } 00755 00756 /** 00757 * @brief Draws a full ellipse. 00758 * @param Xpos: X position 00759 * @param Ypos: Y position 00760 * @param XRadius: Ellipse X radius 00761 * @param YRadius: Ellipse Y radius 00762 */ 00763 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00764 { 00765 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 00766 float K = 0, rad1 = 0, rad2 = 0; 00767 00768 rad1 = XRadius; 00769 rad2 = YRadius; 00770 00771 K = (float)(rad2/rad1); 00772 00773 do 00774 { 00775 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/K)), (Ypos+y), (2*(uint16_t)(x/K) + 1)); 00776 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/K)), (Ypos-y), (2*(uint16_t)(x/K) + 1)); 00777 00778 e2 = err; 00779 if (e2 <= x) 00780 { 00781 err += ++x*2+1; 00782 if (-y == x && e2 <= y) e2 = 0; 00783 } 00784 if (e2 > y) err += ++y*2+1; 00785 } 00786 while (y <= 0); 00787 } 00788 00789 /** 00790 * @brief Enables the display. 00791 */ 00792 void BSP_LCD_DisplayOn(void) 00793 { 00794 lcd_drv->DisplayOn(); 00795 } 00796 00797 /** 00798 * @brief Disables the display. 00799 */ 00800 void BSP_LCD_DisplayOff(void) 00801 { 00802 lcd_drv->DisplayOff(); 00803 } 00804 00805 /** 00806 * @} 00807 */ 00808 00809 /** @addtogroup STM3210C_EVAL_LCD_Private_Functions 00810 * @{ 00811 */ 00812 00813 /****************************************************************************** 00814 Static Function 00815 *******************************************************************************/ 00816 /** 00817 * @brief Draws a pixel on LCD. 00818 * @param Xpos: X position 00819 * @param Ypos: Y position 00820 * @param RGBCode: Pixel color in RGB mode (5-6-5) 00821 */ 00822 static void LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint16_t RGBCode) 00823 { 00824 if(lcd_drv->WritePixel != NULL) 00825 { 00826 lcd_drv->WritePixel(Xpos, Ypos, RGBCode); 00827 } 00828 } 00829 00830 /** 00831 * @brief Draws a character on LCD. 00832 * @param Xpos: Line where to display the character shape 00833 * @param Ypos: Start column address 00834 * @param pChar: Pointer to the character data 00835 */ 00836 static void LCD_DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *pChar) 00837 { 00838 uint32_t counterh = 0, counterw = 0, index = 0; 00839 uint16_t height = 0, width = 0; 00840 uint8_t offset = 0; 00841 uint8_t *pchar = NULL; 00842 uint32_t line = 0; 00843 00844 00845 height = DrawProp.pFont->Height; 00846 width = DrawProp.pFont->Width; 00847 00848 /* Fill bitmap header*/ 00849 *(uint16_t *) (bitmap + 2) = (uint16_t)(height*width*2+OFFSET_BITMAP); 00850 *(uint16_t *) (bitmap + 4) = (uint16_t)((height*width*2+OFFSET_BITMAP)>>16); 00851 *(uint16_t *) (bitmap + 10) = OFFSET_BITMAP; 00852 *(uint16_t *) (bitmap + 18) = (uint16_t)(width); 00853 *(uint16_t *) (bitmap + 20) = (uint16_t)((width)>>16); 00854 *(uint16_t *) (bitmap + 22) = (uint16_t)(height); 00855 *(uint16_t *) (bitmap + 24) = (uint16_t)((height)>>16); 00856 00857 offset = 8 *((width + 7)/8) - width ; 00858 00859 for(counterh = 0; counterh < height; counterh++) 00860 { 00861 pchar = ((uint8_t *)pChar + (width + 7)/8 * counterh); 00862 00863 if(((width + 7)/8) == 3) 00864 { 00865 line = (pchar[0]<< 16) | (pchar[1]<< 8) | pchar[2]; 00866 } 00867 00868 if(((width + 7)/8) == 2) 00869 { 00870 line = (pchar[0]<< 8) | pchar[1]; 00871 } 00872 00873 if(((width + 7)/8) == 1) 00874 { 00875 line = pchar[0]; 00876 } 00877 00878 for (counterw = 0; counterw < width; counterw++) 00879 { 00880 /* Image in the bitmap is written from the bottom to the top */ 00881 /* Need to invert image in the bitmap */ 00882 index = (((height-counterh-1)*width)+(counterw))*2+OFFSET_BITMAP; 00883 if(line & (1 << (width- counterw + offset- 1))) 00884 { 00885 bitmap[index] = (uint8_t)DrawProp.TextColor; 00886 bitmap[index+1] = (uint8_t)(DrawProp.TextColor >> 8); 00887 } 00888 else 00889 { 00890 bitmap[index] = (uint8_t)DrawProp.BackColor; 00891 bitmap[index+1] = (uint8_t)(DrawProp.BackColor >> 8); 00892 } 00893 } 00894 } 00895 00896 BSP_LCD_DrawBitmap(Xpos, Ypos, bitmap); 00897 } 00898 00899 /** 00900 * @brief Sets display window. 00901 * @param Xpos: LCD X position 00902 * @param Ypos: LCD Y position 00903 * @param Width: LCD window width 00904 * @param Height: LCD window height 00905 */ 00906 static void LCD_SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00907 { 00908 if(lcd_drv->SetDisplayWindow != NULL) 00909 { 00910 lcd_drv->SetDisplayWindow(Xpos, Ypos, Width, Height); 00911 } 00912 } 00913 00914 /** 00915 * @} 00916 */ 00917 00918 /** 00919 * @} 00920 */ 00921 00922 /** 00923 * @} 00924 */ 00925 00926 /** 00927 * @} 00928 */ 00929 00930 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00931
Generated on Fri Apr 14 2017 13:00:47 for STM3210C_EVAL BSP User Manual by
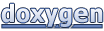