STM32091C_EVAL BSP User Manual
|
Functions | |
uint8_t | BSP_SD_Init (void) |
Initializes the SD/SD communication. | |
uint8_t | BSP_SD_IsDetected (void) |
Detects if SD card is correctly plugged in the memory slot or not. | |
uint8_t | BSP_SD_ReadBlocks (uint32_t *pData, uint32_t ReadAddr, uint16_t BlockSize, uint32_t NumberOfBlocks) |
Reads block(s) from a specified address in the SD card, in polling mode. | |
uint8_t | BSP_SD_WriteBlocks (uint32_t *pData, uint32_t WriteAddr, uint16_t BlockSize, uint32_t NumberOfBlocks) |
Writes block(s) to a specified address in the SD card, in polling mode. | |
uint8_t | BSP_SD_Erase (uint32_t StartAddr, uint32_t EndAddr) |
Erases the specified memory area of the given SD card. | |
uint8_t | BSP_SD_GetStatus (void) |
Returns the SD status. | |
uint8_t | BSP_SD_GetCardInfo (SD_CardInfo *pCardInfo) |
Returns information about specific card. | |
void | SD_IO_Init (void) |
Initializes the SD Card and put it into StandBy State (Ready for data transfer). | |
void | SD_IO_CSState (uint8_t state) |
void | SD_IO_WriteReadData (const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength) |
Write a byte on the SD. | |
uint8_t | SD_IO_WriteByte (uint8_t Data) |
Writes a byte on the SD. |
Function Documentation
uint8_t BSP_SD_Erase | ( | uint32_t | StartAddr, |
uint32_t | EndAddr | ||
) |
Erases the specified memory area of the given SD card.
- Parameters:
-
StartAddr Start byte address EndAddr End byte address
- Return values:
-
SD status
Definition at line 500 of file stm32091c_eval_sd.c.
References BSP_SD_ERROR, BSP_SD_OK, SD_CmdAnswer_typedef::r1, SD_ANSWER_R1_EXPECTED, SD_ANSWER_R1B_EXPECTED, SD_CMD_ERASE, SD_CMD_SD_ERASE_GRP_END, SD_CMD_SD_ERASE_GRP_START, SD_DUMMY_BYTE, SD_IO_CSState(), SD_IO_WriteByte(), SD_R1_NO_ERROR, and SD_SendCmd().
uint8_t BSP_SD_GetCardInfo | ( | SD_CardInfo * | pCardInfo | ) |
Returns information about specific card.
- Parameters:
-
pCardInfo Pointer to a SD_CardInfo structure that contains all SD card information.
- Return values:
-
The SD Response: - MSD_ERROR: Sequence failed
- MSD_OK: Sequence succeed
Definition at line 314 of file stm32091c_eval_sd.c.
References SD_CardInfo::CardBlockSize, SD_CardInfo::CardCapacity, SD_CardInfo::Cid, SD_CardInfo::Csd, struct_v1::DeviceSize, struct_v2::DeviceSize, struct_v1::DeviceSizeMul, SD_CSD::RdBlockLen, SD_GetCIDRegister(), SD_GetCSDRegister(), SD_CSD::csd_version::v1, SD_CSD::csd_version::v2, and SD_CSD::version.
uint8_t BSP_SD_GetStatus | ( | void | ) |
Returns the SD status.
- Return values:
-
The SD status.
Definition at line 535 of file stm32091c_eval_sd.c.
References BSP_SD_ERROR, BSP_SD_OK, SD_CmdAnswer_typedef::r1, SD_CmdAnswer_typedef::r2, SD_ANSWER_R2_EXPECTED, SD_CMD_SEND_STATUS, SD_DUMMY_BYTE, SD_IO_CSState(), SD_IO_WriteByte(), SD_R1_NO_ERROR, SD_R2_NO_ERROR, and SD_SendCmd().
uint8_t BSP_SD_Init | ( | void | ) |
Initializes the SD/SD communication.
- Return values:
-
The SD Response: - MSD_ERROR: Sequence failed
- MSD_OK: Sequence succeed
Definition at line 269 of file stm32091c_eval_sd.c.
References BSP_SD_IsDetected(), MSD_ERROR, SD_GoIdleState(), SD_IO_Init(), SD_NOT_PRESENT, and SD_PRESENT.
uint8_t BSP_SD_IsDetected | ( | void | ) |
Detects if SD card is correctly plugged in the memory slot or not.
- Return values:
-
Returns if SD is detected or not
Definition at line 293 of file stm32091c_eval_sd.c.
References SD_DETECT_GPIO_PORT, SD_DETECT_PIN, SD_NOT_PRESENT, and SD_PRESENT.
Referenced by BSP_SD_Init().
uint8_t BSP_SD_ReadBlocks | ( | uint32_t * | pData, |
uint32_t | ReadAddr, | ||
uint16_t | BlockSize, | ||
uint32_t | NumberOfBlocks | ||
) |
Reads block(s) from a specified address in the SD card, in polling mode.
- Parameters:
-
pData Pointer to the buffer that will contain the data to transmit ReadAddr Address from where data is to be read BlockSize SD card data block size, that should be 512 NumberOfBlocks Number of SD blocks to read
- Return values:
-
SD status
Definition at line 344 of file stm32091c_eval_sd.c.
References BSP_SD_ERROR, BSP_SD_OK, SD_CmdAnswer_typedef::r1, SD_ANSWER_R1_EXPECTED, SD_CMD_READ_SINGLE_BLOCK, SD_CMD_SET_BLOCKLEN, SD_DUMMY_BYTE, SD_IO_CSState(), SD_IO_WriteByte(), SD_IO_WriteReadData(), SD_R1_NO_ERROR, SD_SendCmd(), SD_TOKEN_START_DATA_SINGLE_BLOCK_READ, and SD_WaitData().
uint8_t BSP_SD_WriteBlocks | ( | uint32_t * | pData, |
uint32_t | WriteAddr, | ||
uint16_t | BlockSize, | ||
uint32_t | NumberOfBlocks | ||
) |
Writes block(s) to a specified address in the SD card, in polling mode.
- Parameters:
-
pData Pointer to the buffer that will contain the data to transmit WriteAddr Address from where data is to be written BlockSize SD card data block size, that should be 512 NumberOfBlocks Number of SD blocks to write
- Return values:
-
SD status
Definition at line 421 of file stm32091c_eval_sd.c.
References BSP_SD_ERROR, BSP_SD_OK, SD_CmdAnswer_typedef::r1, SD_ANSWER_R1_EXPECTED, SD_CMD_SET_BLOCKLEN, SD_CMD_WRITE_SINGLE_BLOCK, SD_DATA_OK, SD_DUMMY_BYTE, SD_GetDataResponse(), SD_IO_CSState(), SD_IO_WriteByte(), SD_IO_WriteReadData(), SD_R1_NO_ERROR, SD_SendCmd(), and SD_TOKEN_START_DATA_SINGLE_BLOCK_WRITE.
void SD_IO_CSState | ( | uint8_t | state | ) |
Definition at line 1140 of file stm32091c_eval.c.
References SD_CS_HIGH, and SD_CS_LOW.
Referenced by BSP_SD_Erase(), BSP_SD_GetStatus(), BSP_SD_ReadBlocks(), BSP_SD_WriteBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), SD_GetDataResponse(), SD_GoIdleState(), and SD_SendCmd().
void SD_IO_Init | ( | void | ) |
Initializes the SD Card and put it into StandBy State (Ready for data transfer).
- Return values:
-
None
Definition at line 1089 of file stm32091c_eval.c.
References LCD_CS_HIGH, LCD_NCS_GPIO_PORT, LCD_NCS_PIN, SD_CS_GPIO_CLK_ENABLE, SD_CS_GPIO_PORT, SD_CS_HIGH, SD_CS_PIN, SD_DETECT_EXTI_IRQn, SD_DETECT_GPIO_CLK_ENABLE, SD_DETECT_GPIO_PORT, SD_DETECT_PIN, SD_DUMMY_BYTE, SD_IO_WriteByte(), and SPIx_Init().
Referenced by BSP_SD_Init().
uint8_t SD_IO_WriteByte | ( | uint8_t | Data | ) |
Writes a byte on the SD.
- Parameters:
-
Data byte to send.
- Return values:
-
None
Definition at line 1172 of file stm32091c_eval.c.
References SPIx_WriteReadData().
Referenced by BSP_SD_Erase(), BSP_SD_GetStatus(), BSP_SD_ReadBlocks(), BSP_SD_WriteBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), SD_GetDataResponse(), SD_GoIdleState(), SD_IO_Init(), SD_ReadData(), SD_SendCmd(), and SD_WaitData().
void SD_IO_WriteReadData | ( | const uint8_t * | DataIn, |
uint8_t * | DataOut, | ||
uint16_t | DataLength | ||
) |
Write a byte on the SD.
- Parameters:
-
DataIn byte to send. DataOut read byte. DataLength data length.
- Return values:
-
None
Definition at line 1159 of file stm32091c_eval.c.
References SPIx_WriteReadData().
Referenced by BSP_SD_ReadBlocks(), BSP_SD_WriteBlocks(), and SD_SendCmd().
Generated on Wed Jul 5 2017 09:21:50 for STM32091C_EVAL BSP User Manual by
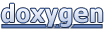