STM32091C_EVAL BSP User Manual
|
Functions | |
static void | I2C1_Init (void) |
I2C Bus initialization. | |
static void | I2C1_Error (void) |
Manages error callback by re-initializing I2C. | |
static void | I2C1_MspInit (I2C_HandleTypeDef *hi2c) |
I2C MSP Initialization. | |
static HAL_StatusTypeDef | I2C1_WriteBuffer (uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) |
Write a value in a register of the device through BUS. | |
static HAL_StatusTypeDef | I2C1_ReadBuffer (uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) |
Reads multiple data on the BUS. | |
static HAL_StatusTypeDef | I2C1_IsDeviceReady (uint16_t DevAddress, uint32_t Trials) |
Checks if target device is ready for communication. | |
static HAL_StatusTypeDef | I2C1_TransmitData (uint8_t *pBuffer, uint16_t Length) |
Write buffer through I2C. | |
static void | I2C2_Init (void) |
I2C Bus initialization. | |
static void | I2C2_Error (void) |
Discovery I2C2 error treatment function. | |
static void | I2C2_MspInit (I2C_HandleTypeDef *hi2c) |
I2C MSP Initialization. | |
static HAL_StatusTypeDef | I2C2_ReceiveData (uint16_t Addr, uint8_t *pBuffer, uint16_t Length) |
Read a register of the device through I2C. | |
static void | SPIx_Init (void) |
SPIx Bus initialization. | |
static void | SPIx_Write (uint8_t Value) |
SPI Write a byte to device. | |
static void | SPIx_WriteReadData (const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLegnth) |
SPI Write a byte to device. | |
static void | SPIx_FlushFifo (void) |
SPIx_FlushFifo. | |
static uint32_t | SPIx_Read (void) |
SPI Read 4 bytes from device. | |
static void | SPIx_Error (void) |
SPI error treatment function. | |
static void | SPIx_MspInit (SPI_HandleTypeDef *hspi) |
SPI MSP Init. | |
void | SD_IO_CSState (uint8_t state) |
Function Documentation
static void I2C1_Error | ( | void | ) | [static] |
Manages error callback by re-initializing I2C.
- Return values:
-
None
Definition at line 615 of file stm32091c_eval.c.
References heval_I2c1, and I2C1_Init().
Referenced by I2C1_ReadBuffer(), I2C1_TransmitData(), and I2C1_WriteBuffer().
static void I2C1_Init | ( | void | ) | [static] |
I2C Bus initialization.
- Return values:
-
None
Definition at line 509 of file stm32091c_eval.c.
References EVAL_I2C1, heval_I2c1, I2C1_MspInit(), and I2C1_TIMING.
Referenced by EEPROM_IO_Init(), HDMI_CEC_IO_Init(), I2C1_Error(), and TSENSOR_IO_Init().
static HAL_StatusTypeDef I2C1_IsDeviceReady | ( | uint16_t | DevAddress, |
uint32_t | Trials | ||
) | [static] |
Checks if target device is ready for communication.
- Note:
- This function is used with Memory devices
- Parameters:
-
DevAddress Target device address Trials Number of trials
- Return values:
-
HAL status
Definition at line 560 of file stm32091c_eval.c.
References heval_I2c1, and I2c1Timeout.
Referenced by EEPROM_IO_IsDeviceReady(), and TSENSOR_IO_IsDeviceReady().
static void I2C1_MspInit | ( | I2C_HandleTypeDef * | hi2c | ) | [static] |
I2C MSP Initialization.
- Parameters:
-
hi2c I2C handle
- Return values:
-
None
Definition at line 629 of file stm32091c_eval.c.
References EVAL_I2C1_CLK_ENABLE, EVAL_I2C1_FORCE_RESET, EVAL_I2C1_GPIO_CLK_ENABLE, EVAL_I2C1_GPIO_PORT, EVAL_I2C1_RELEASE_RESET, EVAL_I2C1_SCL_PIN, EVAL_I2C1_SCL_SDA_AF, and EVAL_I2C1_SDA_PIN.
Referenced by I2C1_Init().
static HAL_StatusTypeDef I2C1_ReadBuffer | ( | uint16_t | Addr, |
uint8_t | Reg, | ||
uint16_t | RegSize, | ||
uint8_t * | pBuffer, | ||
uint16_t | Length | ||
) | [static] |
Reads multiple data on the BUS.
- Parameters:
-
Addr I2C Address Reg Reg Address RegSize The target register size (can be 8BIT or 16BIT) pBuffer pointer to read data buffer Length length of the data
- Return values:
-
0 if no problems to read multiple data
Definition at line 538 of file stm32091c_eval.c.
References heval_I2c1, I2C1_Error(), and I2c1Timeout.
Referenced by EEPROM_IO_ReadData(), and TSENSOR_IO_Read().
static HAL_StatusTypeDef I2C1_TransmitData | ( | uint8_t * | pBuffer, |
uint16_t | Length | ||
) | [static] |
Write buffer through I2C.
- Parameters:
-
pBuffer The address of the data to be written Length buffer size to be written
- Return values:
-
None
Definition at line 595 of file stm32091c_eval.c.
References heval_I2c1, I2C1_Error(), and I2c1Timeout.
Referenced by HDMI_CEC_IO_WriteData().
static HAL_StatusTypeDef I2C1_WriteBuffer | ( | uint16_t | Addr, |
uint8_t | Reg, | ||
uint16_t | RegSize, | ||
uint8_t * | pBuffer, | ||
uint16_t | Length | ||
) | [static] |
Write a value in a register of the device through BUS.
- Parameters:
-
Addr Device address on BUS Bus. Reg The target register address to write RegSize The target register size (can be 8BIT or 16BIT) pBuffer The target register value to be written Length buffer size to be written
- Return values:
-
None
Definition at line 574 of file stm32091c_eval.c.
References heval_I2c1, I2C1_Error(), and I2c1Timeout.
Referenced by EEPROM_IO_WriteData(), and TSENSOR_IO_Write().
static void I2C2_Error | ( | void | ) | [static] |
Discovery I2C2 error treatment function.
- Return values:
-
None
Definition at line 713 of file stm32091c_eval.c.
References heval_I2c2, and I2C2_Init().
Referenced by I2C2_ReceiveData().
static void I2C2_Init | ( | void | ) | [static] |
I2C Bus initialization.
- Return values:
-
None
Definition at line 667 of file stm32091c_eval.c.
References EVAL_I2C2, heval_I2c2, I2C2_MspInit(), and I2C2_TIMING.
Referenced by HDMI_CEC_IO_Init(), and I2C2_Error().
static void I2C2_MspInit | ( | I2C_HandleTypeDef * | hi2c | ) | [static] |
I2C MSP Initialization.
- Parameters:
-
hi2c I2C handle
- Return values:
-
None
Definition at line 727 of file stm32091c_eval.c.
References EVAL_I2C2_AF, EVAL_I2C2_CLK_ENABLE, EVAL_I2C2_FORCE_RESET, EVAL_I2C2_GPIO_CLK_ENABLE, EVAL_I2C2_GPIO_PORT, EVAL_I2C2_RELEASE_RESET, EVAL_I2C2_SCL_PIN, and EVAL_I2C2_SDA_PIN.
Referenced by I2C2_Init().
static HAL_StatusTypeDef I2C2_ReceiveData | ( | uint16_t | Addr, |
uint8_t * | pBuffer, | ||
uint16_t | Length | ||
) | [static] |
Read a register of the device through I2C.
- Parameters:
-
Addr Device address on I2C Bus. pBuffer The address to store the read data Length buffer size to be read
- Return values:
-
None
Definition at line 694 of file stm32091c_eval.c.
References heval_I2c2, I2C2_Error(), and I2c2Timeout.
Referenced by HDMI_CEC_IO_ReadData().
void SD_IO_CSState | ( | uint8_t | state | ) |
Definition at line 1140 of file stm32091c_eval.c.
References SD_CS_HIGH, and SD_CS_LOW.
Referenced by BSP_SD_Erase(), BSP_SD_GetStatus(), BSP_SD_ReadBlocks(), BSP_SD_WriteBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), SD_GetDataResponse(), SD_GoIdleState(), and SD_SendCmd().
static void SPIx_Error | ( | void | ) | [static] |
SPI error treatment function.
- Return values:
-
None
Definition at line 863 of file stm32091c_eval.c.
References heval_Spi, and SPIx_Init().
Referenced by SPIx_Read(), SPIx_Write(), and SPIx_WriteReadData().
static void SPIx_FlushFifo | ( | void | ) | [static] |
SPIx_FlushFifo.
- Return values:
-
None
Definition at line 854 of file stm32091c_eval.c.
References heval_Spi.
Referenced by LCD_IO_WriteMultipleData().
static void SPIx_Init | ( | void | ) | [static] |
SPIx Bus initialization.
- Return values:
-
None
Definition at line 759 of file stm32091c_eval.c.
References EVAL_SPIx, heval_Spi, and SPIx_MspInit().
Referenced by LCD_IO_Init(), SD_IO_Init(), and SPIx_Error().
static void SPIx_MspInit | ( | SPI_HandleTypeDef * | hspi | ) | [static] |
SPI MSP Init.
- Parameters:
-
hspi SPI handle
- Return values:
-
None
Definition at line 877 of file stm32091c_eval.c.
References EVAL_SPIx_CLK_ENABLE, EVAL_SPIx_FORCE_RESET, EVAL_SPIx_MISO_AF, EVAL_SPIx_MISO_GPIO_CLK_ENABLE, EVAL_SPIx_MISO_GPIO_PORT, EVAL_SPIx_MISO_PIN, EVAL_SPIx_MOSI_AF, EVAL_SPIx_MOSI_DIR_GPIO_CLK_ENABLE, EVAL_SPIx_MOSI_DIR_GPIO_PORT, EVAL_SPIx_MOSI_DIR_PIN, EVAL_SPIx_MOSI_GPIO_CLK_ENABLE, EVAL_SPIx_MOSI_GPIO_PORT, EVAL_SPIx_MOSI_PIN, EVAL_SPIx_RELEASE_RESET, EVAL_SPIx_SCK_AF, EVAL_SPIx_SCK_GPIO_CLK_ENABLE, EVAL_SPIx_SCK_GPIO_PORT, and EVAL_SPIx_SCK_PIN.
Referenced by SPIx_Init().
static uint32_t SPIx_Read | ( | void | ) | [static] |
SPI Read 4 bytes from device.
- Return values:
-
Read data
Definition at line 792 of file stm32091c_eval.c.
References heval_Spi, SPIx_Error(), and SpixTimeout.
Referenced by LCD_IO_ReadData().
static void SPIx_Write | ( | uint8_t | Value | ) | [static] |
SPI Write a byte to device.
- Parameters:
-
Value value to be written
- Return values:
-
None
Definition at line 835 of file stm32091c_eval.c.
References heval_Spi, SPIx_Error(), and SpixTimeout.
Referenced by LCD_IO_ReadData(), LCD_IO_WriteMultipleData(), and LCD_IO_WriteReg().
static void SPIx_WriteReadData | ( | const uint8_t * | DataIn, |
uint8_t * | DataOut, | ||
uint16_t | DataLegnth | ||
) | [static] |
SPI Write a byte to device.
- Parameters:
-
DataIn value to be written DataOut read value to be written DataLegnth data length
Definition at line 816 of file stm32091c_eval.c.
References heval_Spi, SPIx_Error(), and SpixTimeout.
Referenced by SD_IO_WriteByte(), and SD_IO_WriteReadData().
Generated on Wed Jul 5 2017 09:21:50 for STM32091C_EVAL BSP User Manual by
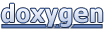