STM32091C_EVAL BSP User Manual
|
stm32091c_eval.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32091c_eval.c 00004 * @author MCD Application Team 00005 * @brief This file provides: a set of firmware functions to manage Leds, 00006 * push-button and COM ports 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Includes ------------------------------------------------------------------*/ 00038 #include "stm32091c_eval.h" 00039 00040 /** @addtogroup BSP 00041 * @{ 00042 */ 00043 00044 /** @addtogroup STM32091C_EVAL 00045 * @brief This file provides firmware functions to manage Leds, push-buttons, 00046 * COM ports, SD card on SPI and temperature sensor (LM75) available on 00047 * STM32091C-EVAL evaluation board from STMicroelectronics. 00048 * @{ 00049 */ 00050 00051 /** @addtogroup STM32091C_EVAL_Common 00052 * @{ 00053 */ 00054 00055 /** @addtogroup STM32091C_EVAL_Private_Constants 00056 * @{ 00057 */ 00058 /* LINK LCD */ 00059 #define START_BYTE 0x70 00060 #define SET_INDEX 0x00 00061 #define READ_STATUS 0x01 00062 #define LCD_WRITE_REG 0x02 00063 #define LCD_READ_REG 0x03 00064 00065 /* LINK SD Card */ 00066 #define SD_DUMMY_BYTE 0xFF 00067 #define SD_NO_RESPONSE_EXPECTED 0x80 00068 00069 /** 00070 * @brief STM32091C EVAL BSP Driver version number V2.0.7 00071 */ 00072 #define __STM32091C_EVAL_BSP_VERSION_MAIN (0x02) /*!< [31:24] main version */ 00073 #define __STM32091C_EVAL_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00074 #define __STM32091C_EVAL_BSP_VERSION_SUB2 (0x07) /*!< [15:8] sub2 version */ 00075 #define __STM32091C_EVAL_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00076 #define __STM32091C_EVAL_BSP_VERSION ((__STM32091C_EVAL_BSP_VERSION_MAIN << 24)\ 00077 |(__STM32091C_EVAL_BSP_VERSION_SUB1 << 16)\ 00078 |(__STM32091C_EVAL_BSP_VERSION_SUB2 << 8 )\ 00079 |(__STM32091C_EVAL_BSP_VERSION_RC)) 00080 /** 00081 * @} 00082 */ 00083 00084 /** @addtogroup STM32091C_EVAL_Private_Variables 00085 * @{ 00086 */ 00087 /** 00088 * @brief LED variables 00089 */ 00090 GPIO_TypeDef* LED_PORT[LEDn] = {LED1_GPIO_PORT, 00091 LED2_GPIO_PORT, 00092 LED3_GPIO_PORT, 00093 LED4_GPIO_PORT}; 00094 00095 const uint16_t LED_PIN[LEDn] = {LED1_PIN, 00096 LED2_PIN, 00097 LED3_PIN, 00098 LED4_PIN}; 00099 00100 /** 00101 * @brief BUTTON variables 00102 */ 00103 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {TAMPER_BUTTON_GPIO_PORT}; 00104 const uint16_t BUTTON_PIN[BUTTONn] = {TAMPER_BUTTON_PIN}; 00105 const uint8_t BUTTON_IRQn[BUTTONn] = {TAMPER_BUTTON_EXTI_IRQn}; 00106 00107 /** 00108 * @brief JOYSTICK variables 00109 */ 00110 GPIO_TypeDef* JOY_PORT[JOYn] = {SEL_JOY_GPIO_PORT, 00111 DOWN_JOY_GPIO_PORT, 00112 LEFT_JOY_GPIO_PORT, 00113 RIGHT_JOY_GPIO_PORT, 00114 UP_JOY_GPIO_PORT}; 00115 00116 const uint16_t JOY_PIN[JOYn] = {SEL_JOY_PIN, 00117 DOWN_JOY_PIN, 00118 LEFT_JOY_PIN, 00119 RIGHT_JOY_PIN, 00120 UP_JOY_PIN}; 00121 00122 const uint8_t JOY_IRQn[JOYn] = {SEL_JOY_EXTI_IRQn, 00123 DOWN_JOY_EXTI_IRQn, 00124 LEFT_JOY_EXTI_IRQn, 00125 RIGHT_JOY_EXTI_IRQn, 00126 UP_JOY_EXTI_IRQn}; 00127 00128 /** 00129 * @brief COM variables 00130 */ 00131 #ifdef HAL_UART_MODULE_ENABLED 00132 USART_TypeDef* COM_USART[COMn] = {EVAL_COM1}; 00133 00134 GPIO_TypeDef* COM_TX_PORT[COMn] = {EVAL_COM1_TX_GPIO_PORT}; 00135 00136 GPIO_TypeDef* COM_RX_PORT[COMn] = {EVAL_COM1_RX_GPIO_PORT}; 00137 00138 const uint16_t COM_TX_PIN[COMn] = {EVAL_COM1_TX_PIN}; 00139 00140 const uint16_t COM_RX_PIN[COMn] = {EVAL_COM1_RX_PIN}; 00141 00142 const uint16_t COM_TX_AF[COMn] = {EVAL_COM1_TX_AF}; 00143 00144 const uint16_t COM_RX_AF[COMn] = {EVAL_COM1_RX_AF}; 00145 00146 #endif /*HAL_UART_MODULE_ENABLED*/ 00147 00148 /** 00149 * @brief BUS variables 00150 */ 00151 #if defined(HAL_I2C_MODULE_ENABLED) 00152 uint32_t I2c1Timeout = EVAL_I2C1_TIMEOUT_MAX; /*<! Value of Timeout when I2C1 communication fails */ 00153 uint32_t I2c2Timeout = EVAL_I2C2_TIMEOUT_MAX; /*<! Value of Timeout when I2C2 communication fails */ 00154 I2C_HandleTypeDef heval_I2c1; 00155 I2C_HandleTypeDef heval_I2c2; 00156 #endif /* HAL_I2C_MODULE_ENABLED */ 00157 00158 #if defined(HAL_SPI_MODULE_ENABLED) 00159 uint32_t SpixTimeout = EVAL_SPIx_TIMEOUT_MAX; /*<! Value of Timeout when SPI communication fails */ 00160 static SPI_HandleTypeDef heval_Spi; 00161 #endif /* HAL_SPI_MODULE_ENABLED */ 00162 00163 /** 00164 * @} 00165 */ 00166 00167 /** @defgroup STM32091C_EVAL_BUS Bus Operation functions 00168 * @{ 00169 */ 00170 #if defined(HAL_I2C_MODULE_ENABLED) 00171 /* I2Cx bus function */ 00172 /* Link function for I2C EEPROM, TSENSOR & HDMI_SOURCE peripherals */ 00173 static void I2C1_Init(void); 00174 static void I2C1_Error (void); 00175 static void I2C1_MspInit(I2C_HandleTypeDef *hi2c); 00176 /* Link function for I2C EEPROM & TSENSOR peripherals */ 00177 static HAL_StatusTypeDef I2C1_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00178 static HAL_StatusTypeDef I2C1_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00179 static HAL_StatusTypeDef I2C1_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00180 /* Link function for HDMI_SOURCE peripheral */ 00181 static HAL_StatusTypeDef I2C1_TransmitData(uint8_t *pBuffer, uint16_t Length); 00182 00183 /* Link function for I2C HDMI_SINK peripherals */ 00184 static void I2C2_Init(void); 00185 static void I2C2_Error(void); 00186 static void I2C2_MspInit(I2C_HandleTypeDef *hi2c); 00187 /* Link function for HDMI_SINK peripheral */ 00188 static HAL_StatusTypeDef I2C2_ReceiveData(uint16_t Addr, uint8_t * pBuffer, uint16_t Length); 00189 00190 /* Link functions for EEPROM peripheral */ 00191 void EEPROM_IO_Init(void); 00192 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00193 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00194 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00195 00196 /* Link functions for Temperature Sensor peripheral */ 00197 void TSENSOR_IO_Init(void); 00198 void TSENSOR_IO_Write(uint16_t DevAddress, uint8_t* pBuffer, uint8_t WriteAddr, uint16_t Length); 00199 void TSENSOR_IO_Read(uint16_t DevAddress, uint8_t* pBuffer, uint8_t ReadAddr, uint16_t Length); 00200 uint16_t TSENSOR_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00201 00202 /* Link functions for CEC peripheral */ 00203 void HDMI_CEC_IO_Init(void); 00204 HAL_StatusTypeDef HDMI_CEC_IO_WriteData(uint8_t * pBuffer, uint16_t BufferSize); 00205 HAL_StatusTypeDef HDMI_CEC_IO_ReadData(uint16_t DevAddress, uint8_t * pBuffer, uint16_t BufferSize); 00206 #endif /* HAL_I2C_MODULE_ENABLED */ 00207 00208 #if defined(HAL_SPI_MODULE_ENABLED) 00209 /* SPIx bus function */ 00210 static void SPIx_Init(void); 00211 static void SPIx_Write(uint8_t Value); 00212 static void SPIx_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLegnth); 00213 static void SPIx_FlushFifo(void); 00214 static uint32_t SPIx_Read(void); 00215 static void SPIx_Error (void); 00216 static void SPIx_MspInit(SPI_HandleTypeDef *hspi); 00217 00218 /* Link function for LCD peripheral over SPI */ 00219 void LCD_IO_Init(void); 00220 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size); 00221 void LCD_IO_WriteReg(uint8_t Reg); 00222 uint16_t LCD_IO_ReadData(uint16_t RegValue); 00223 void LCD_Delay (uint32_t delay); 00224 00225 /* Link functions for SD Card peripheral */ 00226 void SD_IO_Init(void); 00227 void SD_IO_CSState(uint8_t state); 00228 void SD_IO_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength); 00229 uint8_t SD_IO_WriteByte(uint8_t Data); 00230 #endif /* HAL_SPI_MODULE_ENABLED */ 00231 00232 /** 00233 * @} 00234 */ 00235 00236 /** @addtogroup STM32091C_EVAL_Exported_Functions 00237 * @{ 00238 */ 00239 00240 /** 00241 * @brief This method returns the STM32F091C EVAL BSP Driver revision 00242 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00243 */ 00244 uint32_t BSP_GetVersion(void) 00245 { 00246 return __STM32091C_EVAL_BSP_VERSION; 00247 } 00248 00249 /** 00250 * @brief Configures LED GPIO. 00251 * @param Led Specifies the Led to be configured. 00252 * This parameter can be one of following parameters: 00253 * @arg LED1 00254 * @arg LED2 00255 * @arg LED3 00256 * @arg LED4 00257 * @retval None 00258 */ 00259 void BSP_LED_Init(Led_TypeDef Led) 00260 { 00261 GPIO_InitTypeDef GPIO_InitStruct; 00262 00263 /* Enable the GPIO_LED clock */ 00264 LEDx_GPIO_CLK_ENABLE(Led); 00265 00266 /* Configure the GPIO_LED pin */ 00267 GPIO_InitStruct.Pin = LED_PIN[Led]; 00268 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00269 GPIO_InitStruct.Pull = GPIO_PULLUP; 00270 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00271 00272 HAL_GPIO_Init(LED_PORT[Led], &GPIO_InitStruct); 00273 00274 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00275 } 00276 00277 /** 00278 * @brief Turns selected LED On. 00279 * @param Led Specifies the Led to be set on. 00280 * This parameter can be one of following parameters: 00281 * @arg LED1 00282 * @arg LED2 00283 * @arg LED3 00284 * @arg LED4 00285 * @retval None 00286 */ 00287 void BSP_LED_On(Led_TypeDef Led) 00288 { 00289 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00290 } 00291 00292 /** 00293 * @brief Turns selected LED Off. 00294 * @param Led Specifies the Led to be set off. 00295 * This parameter can be one of following parameters: 00296 * @arg LED1 00297 * @arg LED2 00298 * @arg LED3 00299 * @arg LED4 00300 * @retval None 00301 */ 00302 void BSP_LED_Off(Led_TypeDef Led) 00303 { 00304 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00305 } 00306 00307 /** 00308 * @brief Toggles the selected LED. 00309 * @param Led Specifies the Led to be toggled. 00310 * This parameter can be one of following parameters: 00311 * @arg LED1 00312 * @arg LED2 00313 * @arg LED3 00314 * @arg LED4 00315 * @retval None 00316 */ 00317 void BSP_LED_Toggle(Led_TypeDef Led) 00318 { 00319 HAL_GPIO_TogglePin(LED_PORT[Led], LED_PIN[Led]); 00320 } 00321 00322 /** 00323 * @brief Configures Tamper Button GPIO or EXTI Line. 00324 * @param Button Button to be configured 00325 * This parameter can be one of the following values: 00326 * @arg BUTTON_TAMPER: Tamper Push Button 00327 * @param Mode Button mode 00328 * This parameter can be one of the following values: 00329 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00330 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00331 * with interrupt generation capability 00332 * @retval None 00333 */ 00334 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Mode) 00335 { 00336 GPIO_InitTypeDef GPIO_InitStruct; 00337 00338 /* Enable the Tamper Clock */ 00339 TAMPERx_GPIO_CLK_ENABLE(Button); 00340 00341 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00342 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00343 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00344 00345 if (Mode == BUTTON_MODE_GPIO) 00346 { 00347 /* Configure Button pin as input */ 00348 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00349 00350 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00351 } 00352 00353 if (Mode == BUTTON_MODE_EXTI) 00354 { 00355 /* Configure Button pin as input with External interrupt */ 00356 GPIO_InitStruct.Mode = GPIO_MODE_IT_FALLING; 00357 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00358 00359 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00360 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x03, 0x00); 00361 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00362 } 00363 } 00364 00365 /** 00366 * @brief Returns the selected button state. 00367 * @param Button Button to be checked. 00368 * This parameter can be one of the following values: 00369 * @arg BUTTON_TAMPER: Tamper Push Button 00370 * @retval The Button GPIO pin value 00371 */ 00372 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00373 { 00374 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00375 } 00376 00377 /** 00378 * @brief Configures joystick GPIO and EXTI modes. 00379 * @param Joy_Mode Button mode. 00380 * This parameter can be one of the following values: 00381 * @arg JOY_MODE_GPIO: Joystick pins will be used as simple IOs 00382 * @arg JOY_MODE_EXTI: Joystick pins will be connected to EXTI line 00383 * with interrupt generation capability 00384 * @retval HAL_OK: if all initializations are OK. Other value if error. 00385 */ 00386 uint8_t BSP_JOY_Init(JOYMode_TypeDef Joy_Mode) 00387 { 00388 JOYState_TypeDef joykey; 00389 GPIO_InitTypeDef GPIO_InitStruct; 00390 00391 /* Initialized the Joystick. */ 00392 for(joykey = JOY_SEL; joykey < (JOY_SEL + JOYn) ; joykey++) 00393 { 00394 /* Enable the JOY clock */ 00395 JOYx_GPIO_CLK_ENABLE(joykey); 00396 00397 GPIO_InitStruct.Pin = JOY_PIN[joykey]; 00398 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00399 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00400 00401 if (Joy_Mode == JOY_MODE_GPIO) 00402 { 00403 /* Configure Joy pin as input */ 00404 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00405 HAL_GPIO_Init(JOY_PORT[joykey], &GPIO_InitStruct); 00406 } 00407 00408 if (Joy_Mode == JOY_MODE_EXTI) 00409 { 00410 /* Configure Joy pin as input with External interrupt */ 00411 GPIO_InitStruct.Mode = GPIO_MODE_IT_RISING; 00412 HAL_GPIO_Init(JOY_PORT[joykey], &GPIO_InitStruct); 00413 00414 /* Enable and set Joy EXTI Interrupt to the lowest priority */ 00415 HAL_NVIC_SetPriority((IRQn_Type)(JOY_IRQn[joykey]), 0x03, 0x00); 00416 HAL_NVIC_EnableIRQ((IRQn_Type)(JOY_IRQn[joykey])); 00417 } 00418 } 00419 00420 return HAL_OK; 00421 } 00422 00423 /** 00424 * @brief Returns the current joystick status. 00425 * @retval Code of the joystick key pressed 00426 * This code can be one of the following values: 00427 * @arg JOY_NONE 00428 * @arg JOY_SEL 00429 * @arg JOY_DOWN 00430 * @arg JOY_LEFT 00431 * @arg JOY_RIGHT 00432 * @arg JOY_UP 00433 */ 00434 JOYState_TypeDef BSP_JOY_GetState(void) 00435 { 00436 JOYState_TypeDef joykey; 00437 00438 for (joykey = JOY_SEL; joykey < (JOY_SEL + JOYn) ; joykey++) 00439 { 00440 if (HAL_GPIO_ReadPin(JOY_PORT[joykey], JOY_PIN[joykey]) == GPIO_PIN_SET) 00441 { 00442 /* Return Code Joystick key pressed */ 00443 return joykey; 00444 } 00445 } 00446 00447 /* No Joystick key pressed */ 00448 return JOY_NONE; 00449 } 00450 00451 #if defined (HAL_UART_MODULE_ENABLED) 00452 /** 00453 * @brief Configures COM port. 00454 * @param COM Specifies the COM port to be configured. 00455 * This parameter can be one of following parameters: 00456 * @arg COM1 00457 * @param huart pointer to a UART_HandleTypeDef structure that 00458 * contains the configuration information for the specified UART peripheral. 00459 * @retval None 00460 */ 00461 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef* huart) 00462 { 00463 GPIO_InitTypeDef GPIO_InitStruct; 00464 00465 /* Enable GPIO clock */ 00466 COMx_TX_GPIO_CLK_ENABLE(COM); 00467 COMx_RX_GPIO_CLK_ENABLE(COM); 00468 00469 /* Enable USART clock */ 00470 COMx_CLK_ENABLE(COM); 00471 00472 /* Configure USART Tx as alternate function push-pull */ 00473 GPIO_InitStruct.Pin = COM_TX_PIN[COM]; 00474 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00475 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00476 GPIO_InitStruct.Pull = GPIO_PULLUP; 00477 GPIO_InitStruct.Alternate = COM_TX_AF[COM]; 00478 HAL_GPIO_Init(COM_TX_PORT[COM], &GPIO_InitStruct); 00479 00480 /* Configure USART Rx as alternate function push-pull */ 00481 GPIO_InitStruct.Pin = COM_RX_PIN[COM]; 00482 GPIO_InitStruct.Alternate = COM_RX_AF[COM]; 00483 HAL_GPIO_Init(COM_RX_PORT[COM], &GPIO_InitStruct); 00484 00485 /* USART configuration */ 00486 huart->Instance = COM_USART[COM]; 00487 HAL_UART_Init(huart); 00488 } 00489 #endif /* HAL_UART_MODULE_ENABLED*/ 00490 00491 /** 00492 * @} 00493 */ 00494 00495 /** @addtogroup STM32091C_EVAL_BUS 00496 * @{ 00497 */ 00498 00499 /******************************************************************************* 00500 BUS OPERATIONS 00501 *******************************************************************************/ 00502 #if defined(HAL_I2C_MODULE_ENABLED) 00503 /******************************* I2C Routines *********************************/ 00504 00505 /** 00506 * @brief I2C Bus initialization 00507 * @retval None 00508 */ 00509 static void I2C1_Init(void) 00510 { 00511 if(HAL_I2C_GetState(&heval_I2c1) == HAL_I2C_STATE_RESET) 00512 { 00513 heval_I2c1.Instance = EVAL_I2C1; 00514 heval_I2c1.Init.Timing = I2C1_TIMING; 00515 heval_I2c1.Init.OwnAddress1 = 0; 00516 heval_I2c1.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00517 heval_I2c1.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00518 heval_I2c1.Init.OwnAddress2 = 0; 00519 heval_I2c1.Init.OwnAddress2Masks = I2C_OA2_NOMASK; 00520 heval_I2c1.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00521 heval_I2c1.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00522 00523 /* Init the I2C */ 00524 I2C1_MspInit(&heval_I2c1); 00525 HAL_I2C_Init(&heval_I2c1); 00526 } 00527 } 00528 00529 /** 00530 * @brief Reads multiple data on the BUS. 00531 * @param Addr I2C Address 00532 * @param Reg Reg Address 00533 * @param RegSize The target register size (can be 8BIT or 16BIT) 00534 * @param pBuffer pointer to read data buffer 00535 * @param Length length of the data 00536 * @retval 0 if no problems to read multiple data 00537 */ 00538 static HAL_StatusTypeDef I2C1_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00539 { 00540 HAL_StatusTypeDef status = HAL_OK; 00541 00542 status = HAL_I2C_Mem_Read(&heval_I2c1, Addr, Reg, RegSize, pBuffer, Length, I2c1Timeout); 00543 00544 /* Check the communication status */ 00545 if(status != HAL_OK) 00546 { 00547 /* Re-Initiaize the BUS */ 00548 I2C1_Error(); 00549 } 00550 return status; 00551 } 00552 00553 /** 00554 * @brief Checks if target device is ready for communication. 00555 * @note This function is used with Memory devices 00556 * @param DevAddress Target device address 00557 * @param Trials Number of trials 00558 * @retval HAL status 00559 */ 00560 static HAL_StatusTypeDef I2C1_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00561 { 00562 return (HAL_I2C_IsDeviceReady(&heval_I2c1, DevAddress, Trials, I2c1Timeout)); 00563 } 00564 00565 /** 00566 * @brief Write a value in a register of the device through BUS. 00567 * @param Addr Device address on BUS Bus. 00568 * @param Reg The target register address to write 00569 * @param RegSize The target register size (can be 8BIT or 16BIT) 00570 * @param pBuffer The target register value to be written 00571 * @param Length buffer size to be written 00572 * @retval None 00573 */ 00574 static HAL_StatusTypeDef I2C1_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00575 { 00576 HAL_StatusTypeDef status = HAL_OK; 00577 00578 status = HAL_I2C_Mem_Write(&heval_I2c1, Addr, Reg, RegSize, pBuffer, Length, I2c1Timeout); 00579 00580 /* Check the communication status */ 00581 if(status != HAL_OK) 00582 { 00583 /* Re-Initiaize the BUS */ 00584 I2C1_Error(); 00585 } 00586 return status; 00587 } 00588 00589 /** 00590 * @brief Write buffer through I2C. 00591 * @param pBuffer The address of the data to be written 00592 * @param Length buffer size to be written 00593 * @retval None 00594 */ 00595 static HAL_StatusTypeDef I2C1_TransmitData(uint8_t *pBuffer, uint16_t Length) 00596 { 00597 HAL_StatusTypeDef status = HAL_OK; 00598 00599 status = HAL_I2C_Slave_Transmit(&heval_I2c1, pBuffer, Length, I2c1Timeout); 00600 00601 /* Check the communication status */ 00602 if(status != HAL_OK) 00603 { 00604 /* Execute user timeout callback */ 00605 I2C1_Error(); 00606 return HAL_ERROR; 00607 } 00608 return HAL_OK; 00609 } 00610 00611 /** 00612 * @brief Manages error callback by re-initializing I2C. 00613 * @retval None 00614 */ 00615 static void I2C1_Error(void) 00616 { 00617 /* De-initialize the I2C communication BUS */ 00618 HAL_I2C_DeInit(&heval_I2c1); 00619 00620 /* Re-Initiaize the I2C communication BUS */ 00621 I2C1_Init(); 00622 } 00623 00624 /** 00625 * @brief I2C MSP Initialization 00626 * @param hi2c I2C handle 00627 * @retval None 00628 */ 00629 static void I2C1_MspInit(I2C_HandleTypeDef *hi2c) 00630 { 00631 GPIO_InitTypeDef GPIO_InitStruct; 00632 RCC_PeriphCLKInitTypeDef RCC_PeriphCLKInitStruct; 00633 00634 /*##-1- Set source clock to SYSCLK for I2C1 ################################################*/ 00635 RCC_PeriphCLKInitStruct.PeriphClockSelection = RCC_PERIPHCLK_I2C1; 00636 RCC_PeriphCLKInitStruct.I2c1ClockSelection = RCC_I2C1CLKSOURCE_SYSCLK; 00637 HAL_RCCEx_PeriphCLKConfig(&RCC_PeriphCLKInitStruct); 00638 00639 /*##-2- Configure the GPIOs ################################################*/ 00640 00641 /* Enable GPIO clock */ 00642 EVAL_I2C1_GPIO_CLK_ENABLE(); 00643 00644 /* Configure I2C SCL & SDA as alternate function */ 00645 GPIO_InitStruct.Pin = (EVAL_I2C1_SCL_PIN| EVAL_I2C1_SDA_PIN); 00646 GPIO_InitStruct.Mode = GPIO_MODE_AF_OD; 00647 GPIO_InitStruct.Pull = GPIO_NOPULL; 00648 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00649 GPIO_InitStruct.Alternate = EVAL_I2C1_SCL_SDA_AF; 00650 HAL_GPIO_Init(EVAL_I2C1_GPIO_PORT, &GPIO_InitStruct); 00651 00652 /*##-3- Configure the Eval I2C peripheral #######################################*/ 00653 /* Enable I2C clock */ 00654 EVAL_I2C1_CLK_ENABLE(); 00655 00656 /* Force the I2C peripheral clock reset */ 00657 EVAL_I2C1_FORCE_RESET(); 00658 00659 /* Release the I2C peripheral clock reset */ 00660 EVAL_I2C1_RELEASE_RESET(); 00661 } 00662 00663 /** 00664 * @brief I2C Bus initialization 00665 * @retval None 00666 */ 00667 static void I2C2_Init(void) 00668 { 00669 if(HAL_I2C_GetState(&heval_I2c2) == HAL_I2C_STATE_RESET) 00670 { 00671 heval_I2c2.Instance = EVAL_I2C2; 00672 heval_I2c2.Init.Timing = I2C2_TIMING; 00673 heval_I2c2.Init.OwnAddress1 = 0; 00674 heval_I2c2.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00675 heval_I2c2.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00676 heval_I2c2.Init.OwnAddress2 = 0; 00677 heval_I2c2.Init.OwnAddress2Masks = I2C_OA2_NOMASK; 00678 heval_I2c2.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00679 heval_I2c2.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00680 00681 /* Init the I2C */ 00682 I2C2_MspInit(&heval_I2c2); 00683 HAL_I2C_Init(&heval_I2c2); 00684 } 00685 } 00686 00687 /** 00688 * @brief Read a register of the device through I2C. 00689 * @param Addr Device address on I2C Bus. 00690 * @param pBuffer The address to store the read data 00691 * @param Length buffer size to be read 00692 * @retval None 00693 */ 00694 static HAL_StatusTypeDef I2C2_ReceiveData(uint16_t Addr, uint8_t * pBuffer, uint16_t Length) 00695 { 00696 HAL_StatusTypeDef status = HAL_OK; 00697 00698 status = HAL_I2C_Master_Receive(&heval_I2c2, Addr, pBuffer, Length, I2c2Timeout); 00699 00700 /* Check the communication status */ 00701 if(status != HAL_OK) 00702 { 00703 /* Execute user timeout callback */ 00704 I2C2_Error(); 00705 } 00706 return status; 00707 } 00708 00709 /** 00710 * @brief Discovery I2C2 error treatment function 00711 * @retval None 00712 */ 00713 static void I2C2_Error(void) 00714 { 00715 /* De-initialize the I2C communication BUS */ 00716 HAL_I2C_DeInit(&heval_I2c2); 00717 00718 /* Re-Initiaize the I2C communication BUS */ 00719 I2C2_Init(); 00720 } 00721 00722 /** 00723 * @brief I2C MSP Initialization 00724 * @param hi2c I2C handle 00725 * @retval None 00726 */ 00727 static void I2C2_MspInit(I2C_HandleTypeDef *hi2c) 00728 { 00729 GPIO_InitTypeDef GPIO_InitStruct; 00730 /* Enable GPIO clock */ 00731 EVAL_I2C2_GPIO_CLK_ENABLE(); 00732 00733 /* Configure I2C SCL and SDA as alternate function */ 00734 GPIO_InitStruct.Pin = (EVAL_I2C2_SCL_PIN | EVAL_I2C2_SDA_PIN); 00735 GPIO_InitStruct.Mode = GPIO_MODE_AF_OD; 00736 GPIO_InitStruct.Pull = GPIO_NOPULL; 00737 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00738 GPIO_InitStruct.Alternate = EVAL_I2C2_AF; 00739 HAL_GPIO_Init(EVAL_I2C2_GPIO_PORT, &GPIO_InitStruct); 00740 00741 /* Enable I2C clock */ 00742 EVAL_I2C2_CLK_ENABLE(); 00743 00744 /* Force the I2C peripheral clock reset */ 00745 EVAL_I2C2_FORCE_RESET(); 00746 00747 /* Release the I2C peripheral clock reset */ 00748 EVAL_I2C2_RELEASE_RESET(); 00749 } 00750 #endif /*HAL_I2C_MODULE_ENABLED*/ 00751 00752 #if defined(HAL_SPI_MODULE_ENABLED) 00753 /******************************* SPI Routines *********************************/ 00754 00755 /** 00756 * @brief SPIx Bus initialization 00757 * @retval None 00758 */ 00759 static void SPIx_Init(void) 00760 { 00761 if(HAL_SPI_GetState(&heval_Spi) == HAL_SPI_STATE_RESET) 00762 { 00763 /* SPI Config */ 00764 heval_Spi.Instance = EVAL_SPIx; 00765 /* SPI baudrate is set to 12 MHz (PCLK1/SPI_BaudRatePrescaler = 48/4 = 12 MHz) 00766 to verify these constraints: 00767 HX8347D LCD SPI interface max baudrate is 50MHz for write and 6.66MHz for read 00768 PCLK1 frequency is set to 48 MHz 00769 - SD card SPI interface max baudrate is 25MHz for write/read 00770 */ 00771 heval_Spi.Init.BaudRatePrescaler = SPI_BAUDRATEPRESCALER_4; 00772 heval_Spi.Init.Direction = SPI_DIRECTION_2LINES; 00773 heval_Spi.Init.CLKPhase = SPI_PHASE_2EDGE; 00774 heval_Spi.Init.CLKPolarity = SPI_POLARITY_HIGH; 00775 heval_Spi.Init.CRCCalculation = SPI_CRCCALCULATION_DISABLE; 00776 heval_Spi.Init.CRCPolynomial = 7; 00777 heval_Spi.Init.DataSize = SPI_DATASIZE_8BIT; 00778 heval_Spi.Init.FirstBit = SPI_FIRSTBIT_MSB; 00779 heval_Spi.Init.NSS = SPI_NSS_SOFT; 00780 heval_Spi.Init.TIMode = SPI_TIMODE_DISABLE; 00781 heval_Spi.Init.Mode = SPI_MODE_MASTER; 00782 00783 SPIx_MspInit(&heval_Spi); 00784 HAL_SPI_Init(&heval_Spi); 00785 } 00786 } 00787 00788 /** 00789 * @brief SPI Read 4 bytes from device 00790 * @retval Read data 00791 */ 00792 static uint32_t SPIx_Read(void) 00793 { 00794 HAL_StatusTypeDef status = HAL_OK; 00795 uint32_t readvalue = 0x0; 00796 uint32_t writevalue = 0xFFFFFFFF; 00797 00798 status = HAL_SPI_TransmitReceive(&heval_Spi, (uint8_t*) &writevalue, (uint8_t*) &readvalue, 1, SpixTimeout); 00799 00800 /* Check the communication status */ 00801 if(status != HAL_OK) 00802 { 00803 /* Execute user timeout callback */ 00804 SPIx_Error(); 00805 } 00806 00807 return readvalue; 00808 } 00809 00810 /** 00811 * @brief SPI Write a byte to device 00812 * @param DataIn value to be written 00813 * @param DataOut read value to be written 00814 * @param DataLegnth data length 00815 */ 00816 static void SPIx_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLegnth) 00817 { 00818 HAL_StatusTypeDef status = HAL_OK; 00819 00820 status = HAL_SPI_TransmitReceive(&heval_Spi, (uint8_t*) DataIn, DataOut, DataLegnth, SpixTimeout); 00821 00822 /* Check the communication status */ 00823 if(status != HAL_OK) 00824 { 00825 /* Execute user timeout callback */ 00826 SPIx_Error(); 00827 } 00828 } 00829 00830 /** 00831 * @brief SPI Write a byte to device 00832 * @param Value value to be written 00833 * @retval None 00834 */ 00835 static void SPIx_Write(uint8_t Value) 00836 { 00837 HAL_StatusTypeDef status = HAL_OK; 00838 uint8_t data; 00839 00840 status = HAL_SPI_TransmitReceive(&heval_Spi, (uint8_t*) &Value, &data, 1, SpixTimeout); 00841 00842 /* Check the communication status */ 00843 if(status != HAL_OK) 00844 { 00845 /* Execute user timeout callback */ 00846 SPIx_Error(); 00847 } 00848 } 00849 00850 /** 00851 * @brief SPIx_FlushFifo 00852 * @retval None 00853 */ 00854 static void SPIx_FlushFifo(void) 00855 { 00856 HAL_SPIEx_FlushRxFifo(&heval_Spi); 00857 } 00858 00859 /** 00860 * @brief SPI error treatment function 00861 * @retval None 00862 */ 00863 static void SPIx_Error (void) 00864 { 00865 /* De-initialize the SPI communication BUS */ 00866 HAL_SPI_DeInit(&heval_Spi); 00867 00868 /* Re- Initiaize the SPI communication BUS */ 00869 SPIx_Init(); 00870 } 00871 00872 /** 00873 * @brief SPI MSP Init 00874 * @param hspi SPI handle 00875 * @retval None 00876 */ 00877 static void SPIx_MspInit(SPI_HandleTypeDef *hspi) 00878 { 00879 GPIO_InitTypeDef GPIO_InitStruct; 00880 00881 /* Enable SPI clock */ 00882 EVAL_SPIx_CLK_ENABLE(); 00883 00884 /* enable EVAL_SPI gpio clocks */ 00885 EVAL_SPIx_SCK_GPIO_CLK_ENABLE(); 00886 EVAL_SPIx_MISO_GPIO_CLK_ENABLE(); 00887 EVAL_SPIx_MOSI_GPIO_CLK_ENABLE(); 00888 EVAL_SPIx_MOSI_DIR_GPIO_CLK_ENABLE(); 00889 00890 /* configure SPI SCK */ 00891 GPIO_InitStruct.Pin = EVAL_SPIx_SCK_PIN; 00892 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00893 GPIO_InitStruct.Pull = GPIO_NOPULL; 00894 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00895 GPIO_InitStruct.Alternate = EVAL_SPIx_SCK_AF; 00896 HAL_GPIO_Init(EVAL_SPIx_SCK_GPIO_PORT, &GPIO_InitStruct); 00897 00898 /* configure SPI MOSI */ 00899 GPIO_InitStruct.Pin = EVAL_SPIx_MOSI_PIN; 00900 GPIO_InitStruct.Alternate = EVAL_SPIx_MOSI_AF; 00901 HAL_GPIO_Init(EVAL_SPIx_MOSI_GPIO_PORT, &GPIO_InitStruct); 00902 00903 /* configure SPI MISO */ 00904 GPIO_InitStruct.Pin = EVAL_SPIx_MISO_PIN; 00905 GPIO_InitStruct.Alternate = EVAL_SPIx_MISO_AF; 00906 HAL_GPIO_Init(EVAL_SPIx_MISO_GPIO_PORT, &GPIO_InitStruct); 00907 00908 /* Set PB.2 as Out PP, as direction pin for MOSI */ 00909 GPIO_InitStruct.Pin = EVAL_SPIx_MOSI_DIR_PIN; 00910 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00911 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00912 GPIO_InitStruct.Pull = GPIO_NOPULL; 00913 HAL_GPIO_Init(EVAL_SPIx_MOSI_DIR_GPIO_PORT, &GPIO_InitStruct); 00914 00915 /* MOSI DIRECTION as output */ 00916 HAL_GPIO_WritePin(EVAL_SPIx_MOSI_DIR_GPIO_PORT, EVAL_SPIx_MOSI_DIR_PIN, GPIO_PIN_SET); 00917 00918 /* Force the SPI peripheral clock reset */ 00919 EVAL_SPIx_FORCE_RESET(); 00920 00921 /* Release the SPI peripheral clock reset */ 00922 EVAL_SPIx_RELEASE_RESET(); 00923 } 00924 00925 #endif /*HAL_SPI_MODULE_ENABLED*/ 00926 00927 /** 00928 * @} 00929 */ 00930 00931 /** @defgroup STM32091C_EVAL_LINK_OPERATIONS Link Operation functions 00932 * @{ 00933 */ 00934 00935 /****************************************************************************** 00936 LINK OPERATIONS 00937 *******************************************************************************/ 00938 00939 #if defined(HAL_SPI_MODULE_ENABLED) 00940 /********************************* LINK LCD ***********************************/ 00941 00942 /** 00943 * @brief Configures the LCD_SPI interface. 00944 * @retval None 00945 */ 00946 void LCD_IO_Init(void) 00947 { 00948 GPIO_InitTypeDef GPIO_InitStruct; 00949 00950 /* Configure the LCD Control pins ------------------------------------------*/ 00951 LCD_NCS_GPIO_CLK_ENABLE(); 00952 00953 /* Configure NCS in Output Push-Pull mode */ 00954 GPIO_InitStruct.Pin = LCD_NCS_PIN; 00955 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_OD; 00956 GPIO_InitStruct.Pull = GPIO_NOPULL; 00957 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW; 00958 HAL_GPIO_Init(LCD_NCS_GPIO_PORT, &GPIO_InitStruct); 00959 00960 /* Set or Reset the control line */ 00961 LCD_CS_LOW(); 00962 LCD_CS_HIGH(); 00963 00964 SPIx_Init(); 00965 } 00966 00967 /** 00968 * @brief Write register value. 00969 * @param pData Pointer on the register value 00970 * @param Size Size of byte to transmit to the register 00971 * @retval None 00972 */ 00973 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size) 00974 { 00975 uint32_t counter = 0; 00976 00977 /* Reset LCD control line(/CS) and Send data */ 00978 LCD_CS_LOW(); 00979 00980 /* Send Start Byte */ 00981 SPIx_Write(START_BYTE | LCD_WRITE_REG); 00982 00983 if (Size == 1) 00984 { 00985 /* Only 1 byte to be sent to LCD - general interface can be used */ 00986 /* Send Data */ 00987 SPIx_Write(*pData); 00988 } 00989 else 00990 { 00991 for (counter = Size; counter != 0; counter--) 00992 { 00993 while(((heval_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 00994 { 00995 } 00996 /* Need to invert bytes for LCD*/ 00997 *((__IO uint8_t*)&heval_Spi.Instance->DR) = *(pData+1); 00998 00999 while(((heval_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 01000 { 01001 } 01002 *((__IO uint8_t*)&heval_Spi.Instance->DR) = *pData; 01003 counter--; 01004 pData += 2; 01005 } 01006 01007 /* Wait until the bus is ready before releasing Chip select */ 01008 while(((heval_Spi.Instance->SR) & SPI_FLAG_BSY) != RESET) 01009 { 01010 } 01011 } 01012 01013 /* Empty the Rx fifo */ 01014 SPIx_FlushFifo(); 01015 01016 /* Reset LCD control line(/CS) and Send data */ 01017 LCD_CS_HIGH(); 01018 } 01019 01020 /** 01021 * @brief Writes address on LCD register. 01022 * @param Reg Register to be written 01023 * @retval None 01024 */ 01025 void LCD_IO_WriteReg(uint8_t Reg) 01026 { 01027 /* Reset LCD control line(/CS) and Send command */ 01028 LCD_CS_LOW(); 01029 01030 /* Send Start Byte */ 01031 SPIx_Write(START_BYTE | SET_INDEX); 01032 01033 /* Write 16-bit Reg Index (High Byte is 0) */ 01034 SPIx_Write(0x00); 01035 SPIx_Write(Reg); 01036 01037 /* Deselect : Chip Select high */ 01038 LCD_CS_HIGH(); 01039 } 01040 01041 /** 01042 * @brief Read data from LCD data register. 01043 * @param Reg Regsiter to be read 01044 * @retval readvalue 01045 */ 01046 uint16_t LCD_IO_ReadData(uint16_t Reg) 01047 { 01048 uint32_t readvalue = 0; 01049 01050 /* Send Reg value to Read */ 01051 LCD_IO_WriteReg(Reg); 01052 01053 /* Reset LCD control line(/CS) and Send command */ 01054 LCD_CS_LOW(); 01055 01056 /* Send Start Byte */ 01057 SPIx_Write(START_BYTE | LCD_READ_REG); 01058 01059 /* Read Upper Byte */ 01060 SPIx_Write(0xFF); 01061 readvalue = SPIx_Read(); 01062 readvalue = readvalue << 8; 01063 readvalue |= SPIx_Read(); 01064 01065 HAL_Delay(10); 01066 01067 /* Deselect : Chip Select high */ 01068 LCD_CS_HIGH(); 01069 return readvalue; 01070 } 01071 01072 /** 01073 * @brief Wait for loop in ms. 01074 * @param Delay in ms. 01075 * @retval None 01076 */ 01077 void LCD_Delay (uint32_t Delay) 01078 { 01079 HAL_Delay (Delay); 01080 } 01081 01082 /******************************** LINK SD Card ********************************/ 01083 01084 /** 01085 * @brief Initializes the SD Card and put it into StandBy State (Ready for 01086 * data transfer). 01087 * @retval None 01088 */ 01089 void SD_IO_Init(void) 01090 { 01091 GPIO_InitTypeDef GPIO_InitStruct; 01092 uint8_t counter; 01093 01094 /* SD_CS_GPIO and SD_DETECT_GPIO Periph clock enable */ 01095 SD_CS_GPIO_CLK_ENABLE(); 01096 SD_DETECT_GPIO_CLK_ENABLE(); 01097 01098 /* Configure SD_CS_PIN pin: SD Card CS pin */ 01099 GPIO_InitStruct.Pin = SD_CS_PIN; 01100 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_OD; 01101 GPIO_InitStruct.Pull = GPIO_PULLUP; 01102 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_MEDIUM; 01103 HAL_GPIO_Init(SD_CS_GPIO_PORT, &GPIO_InitStruct); 01104 01105 /* Configure SD_DETECT_PIN pin: SD Card detect pin */ 01106 GPIO_InitStruct.Pin = SD_DETECT_PIN; 01107 GPIO_InitStruct.Mode = GPIO_MODE_IT_RISING_FALLING; 01108 GPIO_InitStruct.Pull = GPIO_PULLUP; 01109 HAL_GPIO_Init(SD_DETECT_GPIO_PORT, &GPIO_InitStruct); 01110 01111 /* Configure LCD_CS_PIN pin: LCD Card CS pin */ 01112 GPIO_InitStruct.Pin = LCD_NCS_PIN; 01113 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 01114 GPIO_InitStruct.Pull = GPIO_NOPULL; 01115 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_MEDIUM; 01116 HAL_GPIO_Init(LCD_NCS_GPIO_PORT, &GPIO_InitStruct); 01117 LCD_CS_HIGH(); 01118 01119 /* Enable and set SD EXTI Interrupt to the lowest priority */ 01120 HAL_NVIC_SetPriority(SD_DETECT_EXTI_IRQn, 0x03, 0); 01121 HAL_NVIC_EnableIRQ(SD_DETECT_EXTI_IRQn); 01122 01123 /*------------Put SD in SPI mode--------------*/ 01124 /* SD SPI Config */ 01125 SPIx_Init(); 01126 01127 /* SD chip select high */ 01128 SD_CS_HIGH(); 01129 01130 /* Send dummy byte 0xFF, 10 times with CS high */ 01131 /* Rise CS and MOSI for 80 clocks cycles */ 01132 for (counter = 0; counter <= 9; counter++) 01133 { 01134 /* Send dummy byte 0xFF */ 01135 SD_IO_WriteByte(SD_DUMMY_BYTE); 01136 } 01137 } 01138 01139 01140 void SD_IO_CSState(uint8_t val) 01141 { 01142 if(val == 1) 01143 { 01144 SD_CS_HIGH(); 01145 } 01146 else 01147 { 01148 SD_CS_LOW(); 01149 } 01150 } 01151 01152 /** 01153 * @brief Write a byte on the SD. 01154 * @param DataIn byte to send. 01155 * @param DataOut read byte. 01156 * @param DataLength data length. 01157 * @retval None 01158 */ 01159 void SD_IO_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength) 01160 { 01161 // /* SD chip select low */ 01162 // SD_CS_LOW(); 01163 /* Send the byte */ 01164 SPIx_WriteReadData(DataIn, DataOut, DataLength); 01165 } 01166 01167 /** 01168 * @brief Writes a byte on the SD. 01169 * @param Data byte to send. 01170 * @retval None 01171 */ 01172 uint8_t SD_IO_WriteByte(uint8_t Data) 01173 { 01174 uint8_t tmp; 01175 // /* SD chip select low */ 01176 // SD_CS_LOW(); 01177 // 01178 /* Send the byte */ 01179 SPIx_WriteReadData(&Data,&tmp,1); 01180 return tmp; 01181 } 01182 01183 #endif /* HAL_SPI_MODULE_ENABLED */ 01184 01185 #if defined(HAL_I2C_MODULE_ENABLED) 01186 /********************************* LINK I2C EEPROM *****************************/ 01187 /** 01188 * @brief Initializes peripherals used by the I2C EEPROM driver. 01189 * @retval None 01190 */ 01191 void EEPROM_IO_Init(void) 01192 { 01193 I2C1_Init(); 01194 } 01195 01196 /** 01197 * @brief Write data to I2C EEPROM driver 01198 * @param DevAddress Target device address 01199 * @param MemAddress Internal memory address 01200 * @param pBuffer Pointer to data buffer 01201 * @param BufferSize Amount of data to be sent 01202 * @retval HAL status 01203 */ 01204 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01205 { 01206 return (I2C1_WriteBuffer(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01207 } 01208 01209 /** 01210 * @brief Read data from I2C EEPROM driver 01211 * @param DevAddress Target device address 01212 * @param MemAddress Internal memory address 01213 * @param pBuffer Pointer to data buffer 01214 * @param BufferSize Amount of data to be read 01215 * @retval HAL status 01216 */ 01217 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01218 { 01219 return (I2C1_ReadBuffer(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01220 } 01221 01222 /** 01223 * @brief Checks if target device is ready for communication. 01224 * @note This function is used with Memory devices 01225 * @param DevAddress Target device address 01226 * @param Trials Number of trials 01227 * @retval HAL status 01228 */ 01229 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01230 { 01231 return (I2C1_IsDeviceReady(DevAddress, Trials)); 01232 } 01233 01234 /********************************* LINK I2C TEMPERATURE SENSOR *****************************/ 01235 /** 01236 * @brief Initializes peripherals used by the I2C Temperature Sensor driver. 01237 * @retval None 01238 */ 01239 void TSENSOR_IO_Init(void) 01240 { 01241 I2C1_Init(); 01242 } 01243 01244 /** 01245 * @brief Writes one byte to the TSENSOR. 01246 * @param DevAddress Target device address 01247 * @param pBuffer Pointer to data buffer 01248 * @param WriteAddr TSENSOR's internal address to write to. 01249 * @param Length Number of data to write 01250 * @retval None 01251 */ 01252 void TSENSOR_IO_Write(uint16_t DevAddress, uint8_t* pBuffer, uint8_t WriteAddr, uint16_t Length) 01253 { 01254 I2C1_WriteBuffer(DevAddress, WriteAddr, I2C_MEMADD_SIZE_8BIT, pBuffer, Length); 01255 } 01256 01257 /** 01258 * @brief Reads one byte from the TSENSOR. 01259 * @param DevAddress Target device address 01260 * @param pBuffer pointer to the buffer that receives the data read from the TSENSOR. 01261 * @param ReadAddr TSENSOR's internal address to read from. 01262 * @param Length Number of data to read 01263 * @retval None 01264 */ 01265 void TSENSOR_IO_Read(uint16_t DevAddress, uint8_t* pBuffer, uint8_t ReadAddr, uint16_t Length) 01266 { 01267 I2C1_ReadBuffer(DevAddress, ReadAddr, I2C_MEMADD_SIZE_8BIT, pBuffer, Length); 01268 } 01269 01270 /** 01271 * @brief Checks if Temperature Sensor is ready for communication. 01272 * @param DevAddress Target device address 01273 * @param Trials Number of trials 01274 * @retval HAL status 01275 */ 01276 uint16_t TSENSOR_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01277 { 01278 return (I2C1_IsDeviceReady(DevAddress, Trials)); 01279 } 01280 01281 /****************************** LINK HDMI CEC *********************************/ 01282 /** 01283 * @brief Initializes CEC low level. 01284 * @retval None 01285 */ 01286 void HDMI_CEC_IO_Init (void) 01287 { 01288 GPIO_InitTypeDef GPIO_InitStruct; 01289 01290 /* Enable CEC clock */ 01291 __HAL_RCC_CEC_CLK_ENABLE(); 01292 01293 /* Enable CEC LINE GPIO clock */ 01294 HDMI_CEC_LINE_CLK_ENABLE(); 01295 01296 /* Configure CEC LINE GPIO as alternate function open drain */ 01297 GPIO_InitStruct.Pin = HDMI_CEC_LINE_PIN; 01298 GPIO_InitStruct.Mode = GPIO_MODE_AF_OD; 01299 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 01300 GPIO_InitStruct.Pull = GPIO_NOPULL; 01301 GPIO_InitStruct.Alternate = HDMI_CEC_LINE_AF; 01302 HAL_GPIO_Init(HDMI_CEC_LINE_GPIO_PORT, &GPIO_InitStruct); 01303 01304 /* CEC IRQ Channel configuration */ 01305 HAL_NVIC_SetPriority((IRQn_Type)HDMI_CEC_IRQn, 0x3, 0x0); 01306 HAL_NVIC_EnableIRQ((IRQn_Type)HDMI_CEC_IRQn); 01307 01308 /* Enable CEC HPD SINK GPIO clock */ 01309 HDMI_CEC_HPD_SINK_CLK_ENABLE(); 01310 01311 /* Configure CEC HPD SINK GPIO as output push pull */ 01312 GPIO_InitStruct.Pin = HDMI_CEC_HPD_SINK_PIN; 01313 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 01314 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW; 01315 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 01316 HAL_GPIO_Init(HDMI_CEC_HPD_SINK_GPIO_PORT, &GPIO_InitStruct); 01317 01318 I2C1_Init(); 01319 01320 /* Enable CEC HPD SOURCE GPIO clock */ 01321 HDMI_CEC_HPD_SOURCE_CLK_ENABLE(); 01322 01323 /* Configure CEC HPD SOURCE GPIO as output push pull */ 01324 GPIO_InitStruct.Pin = HDMI_CEC_HPD_SOURCE_PIN; 01325 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 01326 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW; 01327 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 01328 HAL_GPIO_Init(HDMI_CEC_HPD_SOURCE_GPIO_PORT, &GPIO_InitStruct); 01329 01330 I2C2_Init(); 01331 } 01332 01333 /** 01334 * @brief Write data to I2C HDMI CEC driver 01335 * @param pBuffer Pointer to data buffer 01336 * @param BufferSize Amount of data to be sent 01337 * @retval HAL status 01338 */ 01339 HAL_StatusTypeDef HDMI_CEC_IO_WriteData(uint8_t * pBuffer, uint16_t BufferSize) 01340 { 01341 return (I2C1_TransmitData(pBuffer, BufferSize)); 01342 } 01343 01344 /** 01345 * @brief Read data to I2C HDMI CEC driver 01346 * @param DevAddress Target device address 01347 * @param pBuffer Pointer to data buffer 01348 * @param BufferSize Amount of data to be sent 01349 * @retval HAL status 01350 */ 01351 HAL_StatusTypeDef HDMI_CEC_IO_ReadData(uint16_t DevAddress, uint8_t * pBuffer, uint16_t BufferSize) 01352 { 01353 return (I2C2_ReceiveData(DevAddress, pBuffer, BufferSize)); 01354 } 01355 01356 #endif /* HAL_I2C_MODULE_ENABLED */ 01357 01358 /** 01359 * @} 01360 */ 01361 01362 /** 01363 * @} 01364 */ 01365 01366 /** 01367 * @} 01368 */ 01369 01370 /** 01371 * @} 01372 */ 01373 01374 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jul 5 2017 09:21:50 for STM32091C_EVAL BSP User Manual by
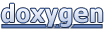