Main Page | Class Hierarchy | Alphabetical List | Class List | Directories | File List | Class Members | File Members
CPlane.h
Go to the documentation of this file.00001 #ifndef __cPLANE_h__ 00002 #define __cPLANE_h__ 00003 00005 // MarSTD version 2004 - (c)2004 - Marcel Smit // 00006 // // 00007 // [email protected] // 00008 // [email protected] // 00009 // // 00010 // This code may not be used in a commercial product without my // 00011 // permission. If you redistribute it, this message must remain // 00012 // intact. If you use this code, some acknowledgement would be // 00013 // appreciated. ;-) // 00015 00017 00018 00019 00020 #include <vector> 00021 #include "CVector.h" 00022 #include "CList.h" 00023 00024 //--------------------------------------------------------------------------- 00025 00029 enum CPlaneClassification 00030 { 00031 pOn, 00032 pFront, 00033 pBack, 00034 pSpan 00035 }; 00036 00038 00041 class CPlane 00042 { 00043 00044 public: 00045 00046 CPlane() 00047 { 00048 prev = 0; 00049 next = 0; 00050 } 00051 ~CPlane() 00052 { 00053 unlink(); 00054 } 00055 00056 public: 00057 00058 CVector normal; 00059 float distance; 00060 00061 public: 00062 00066 void normalize() 00067 { 00068 float size = normal.size(); 00069 if (size == 0.0) 00070 return; 00071 normal /= size; 00072 distance /= size; 00073 } 00080 void setup(CVector vector1, CVector vector2, CVector vector3) 00081 { 00082 CVector delta1 = vector2 - vector1; 00083 CVector delta2 = vector3 - vector2; 00084 00085 normal = delta1 % delta2; 00086 00087 distance = normal * vector1; 00088 } 00089 00090 public: 00091 00095 void operator=(CPlane plane) 00096 { 00097 normal = plane.normal; 00098 distance = plane.distance; 00099 } 00104 float operator*(CVector vector) 00105 { 00106 return normal * vector - distance; 00107 } 00111 CPlane operator-() 00112 { 00113 CPlane tmp; 00114 tmp.normal = -normal; 00115 tmp.distance = -distance; 00116 return tmp; 00117 } 00118 00119 public: 00120 00121 CPlane* prev; 00122 CPlane* next; 00123 00124 public: 00125 00126 CPlane* unlink() 00127 { 00128 DLLIST_UNLINK_SELF(); 00129 return this; 00130 } 00131 00132 }; 00133 00134 //--------------------------------------------------------------------------- 00135 00139 typedef std::vector<CPlane> CPlaneArray; 00140 00144 typedef CPlaneArray::iterator CPlaneArrayItr; 00145 00146 //--------------------------------------------------------------------------- 00147 00148 #endif
Generated on Tue Feb 8 21:59:02 2005 for MarSTDv2004 by
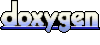