Main Page | Class Hierarchy | Alphabetical List | Class List | Directories | File List | Class Members | File Members
CList.h
Go to the documentation of this file.00001 #ifndef __CList_h__ 00002 #define __CList_h__ 00003 00005 // MarSTD version 2004 - (c)2004 - Marcel Smit // 00006 // // 00007 // [email protected] // 00008 // [email protected] // 00009 // // 00010 // This code may not be used in a commercial product without my // 00011 // permission. If you redistribute it, this message must remain // 00012 // intact. If you use this code, some acknowledgement would be // 00013 // appreciated. ;-) // 00015 00017 00018 00019 00020 #define DLLIST_UNLINK_SELF() \ 00021 { \ 00022 if (prev) \ 00023 prev->next = next; \ 00024 if (next) \ 00025 next->prev = prev; \ 00026 prev = 0; \ 00027 next = 0; \ 00028 return this; \ 00029 } 00030 00031 #define DLLIST_LINK_HEAD(_head, _tail, _item, _count) \ 00032 { \ 00033 _item->prev = 0; \ 00034 _item->next = _head; \ 00035 if (_head) \ 00036 _head->prev = _item; \ 00037 _head = _item; \ 00038 if (!_tail) \ 00039 _tail = _head; \ 00040 _count++; \ 00041 } 00042 00043 #define DLLIST_LINK_TAIL(_head, _tail, _item, _count) \ 00044 { \ 00045 _item->prev = _tail; \ 00046 _item->next = 0; \ 00047 if (_tail) \ 00048 _tail->next = _item; \ 00049 _tail = _item; \ 00050 if (!_head) \ 00051 _head = _tail; \ 00052 _count++; \ 00053 } 00054 00055 #define DLLIST_UNLINK(_head, _tail, _item, _count) \ 00056 { \ 00057 if (_item == _head) \ 00058 _head = _item->next; \ 00059 if (_item == _tail) \ 00060 _tail = _item->prev; \ 00061 _item->unlink(); \ 00062 _count--; \ 00063 } 00064 00065 #define DLLIST_REMOVE(_item) \ 00066 { \ 00067 delete unlink(_item); \ 00068 } 00069 00070 #define DLLIST_CLEAR(_head) \ 00071 { \ 00072 while (_head) \ 00073 remove(_head); \ 00074 } 00075 00076 #endif
Generated on Tue Feb 8 21:59:02 2005 for MarSTDv2004 by
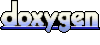