Main Page | Class Hierarchy | Alphabetical List | Class List | Directories | File List | Class Members | File Members
CGeomCompiler.h
00001 #ifndef __CGeomCompiler_h__ 00002 #define __CGeomCompiler_h__ 00003 00005 // MarSTD version 2004 - (c)2004 - Marcel Smit // 00006 // // 00007 // [email protected] // 00008 // [email protected] // 00009 // // 00010 // This code may not be used in a commercial product without my // 00011 // permission. If you redistribute it, this message must remain // 00012 // intact. If you use this code, some acknowledgement would be // 00013 // appreciated. ;-) // 00015 00017 00018 00019 00020 // FIXME: Fix / implement texcoord stuff. 00021 00022 #include "CPlane.h" 00023 #include "CPoly.h" 00024 00025 //--------------------------------------------------------------------------- 00026 00027 #define GEOM_COMPILER_VERTEX_EPS 0.001 00028 #define GEOM_COMPILER_TEXCOORD_EPS 0.001 00029 00030 00031 00035 class CCompiledVertex 00036 { 00037 00038 public: 00039 00040 CVector p; 00041 00042 std::vector<int> edge; 00043 std::vector<int> polygon; 00044 00045 public: 00046 00047 CPlane plane; 00048 00049 }; 00050 00052 00056 class CCompiledTexcoord 00057 { 00058 00059 public: 00060 00061 float t[2]; 00062 00063 std::vector<int> edge; 00064 std::vector<int> polygon; 00065 00066 }; 00067 00069 00073 class CCompiledEdge 00074 { 00075 00076 public: 00077 00078 std::vector<int> vertex; 00079 std::vector<int> texCoord; 00080 00081 }; 00082 00084 00088 class CCompiledPolygon 00089 { 00090 00091 public: 00092 00093 std::vector<int> vertex; 00094 std::vector<int> texcoord; 00095 std::vector<int> edge; 00096 00097 public: 00098 00099 CPlane plane; 00100 CVector center; 00101 00102 public: 00103 00104 void* data; 00105 00106 }; 00107 00109 00115 class CCompiledMesh 00116 { 00117 00118 public: 00119 00120 CCompiledMesh() 00121 { 00122 base = this; 00123 } 00124 00125 public: 00126 00127 std::vector<CCompiledVertex> vertex; 00128 std::vector<CCompiledTexcoord> texcoord; 00129 std::vector<CCompiledEdge> edge; 00130 std::vector<CCompiledPolygon> polygon; 00131 00132 public: 00133 00134 CCompiledMesh* base; 00135 std::vector<int> polygonIndex; 00136 00137 public: 00138 00139 void clear(); 00140 void init(); 00141 00142 }; 00143 00145 00172 class CGeomCompiler 00173 { 00174 00175 private: 00176 00177 CGeomCompiler(); 00178 00179 public: 00180 00181 ~CGeomCompiler(); 00182 00183 public: 00184 00188 static CGeomCompiler& I() 00189 { 00190 static CGeomCompiler geomCompiler; 00191 return geomCompiler; 00192 } 00193 00194 public: 00195 00200 void compile(CMesh& mesh, CCompiledMesh& out); 00201 void compile(CMesh& mesh, CCompiledMesh& base, CCompiledMesh& index); 00202 00203 private: 00204 00205 int findVertex(CCompiledMesh& mesh, CCompiledVertex& vertex); 00206 int findTexcoord(CCompiledMesh& mesh, CCompiledTexcoord& texCoord); 00207 int findEdge(CCompiledMesh& mesh, CCompiledEdge& edge); 00208 00209 public: 00210 00211 void removeDuplicateIndices(CCompiledMesh& mesh); 00212 00213 }; 00214 00215 #endif
Generated on Tue Feb 8 21:59:02 2005 for MarSTDv2004 by
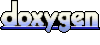