Main Page | Class Hierarchy | Alphabetical List | Class List | Directories | File List | Class Members | File Members
CBsp.h
Go to the documentation of this file.00001 #ifndef __cBSP_h__ 00002 #define __cBSP_h__ 00003 00005 // MarSTD version 2004 - (c)2004 - Marcel Smit // 00006 // // 00007 // [email protected] // 00008 // [email protected] // 00009 // // 00010 // This code may not be used in a commercial product without my // 00011 // permission. If you redistribute it, this message must remain // 00012 // intact. If you use this code, some acknowledgement would be // 00013 // appreciated. ;-) // 00015 00017 00018 00019 00020 #include "CDebug.h" 00021 #include "CVector.h" 00022 #include "CPlane.h" 00023 #include "CPoly.h" 00024 #include "CSphere.h" 00025 00026 //#define BSP_ALTERNATE_CANCEL ///< If uncommented, an alternate 'bail out method' will be used. This bail out method will not create a complete BSP, in that it will gaurantee full front-to-back sorting. Polygons that are not coplanar might end up in the same leaf node. 00027 #define BSP_USE_SPHERES 00028 #define BSP_DEFAULT_EPS 0.001 00029 00030 00033 typedef struct 00034 { 00035 00036 CPlane plane; 00037 int planeFlipped; 00038 00039 int fc; 00040 int bc; 00041 int dc; 00042 int sc; 00043 00044 } CBspSplitInfo; 00045 00049 typedef struct 00050 { 00051 00052 void* data; 00053 CSphere sphere; 00054 00055 } CBspPolyData; 00056 00058 00092 class CBsp : public CMesh 00093 { 00094 00095 public: 00096 00097 CBsp(); 00098 ~CBsp(); 00099 00100 public: 00101 00102 CPlane plane; 00103 int planeFlipped; 00104 CBsp* child[2]; 00105 CBsp* parent; 00106 float eps; 00107 00108 int initialized; 00109 CBspPolyData* polyData; 00110 CVector mins, maxs; 00111 CSphere sphere; 00112 float volume; 00113 int noSplittingPlane; 00114 00115 public: 00116 00120 int coplanar(); 00126 void move(CPoly* poly, CBsp* bsp); 00132 int onFront(CPoly* poly, int bias = 0); 00138 int onBack(CPoly* poly, int bias = 0); 00146 void getSplitInfo(CPlane& p, CBspSplitInfo& i, int flip); 00152 int test(CBspSplitInfo* i1, CBspSplitInfo* i2); 00158 int test1(CBspSplitInfo* i1, CBspSplitInfo* i2); 00164 int test2(CBspSplitInfo* i1, CBspSplitInfo* i2); 00168 CPlane getSplitPlane(); 00173 void split(CPoly* poly); 00177 void split(); // Splits / Moves all polys. 00182 CSphere getBoundingSphere(CPoly* poly); 00183 void initBoundingSpheres(); 00184 void freeBoundingSpheres(); 00185 void restorePolyData(); 00186 00189 void initBoundingBox(); 00190 00191 public: 00192 00196 void getHitLeafs(CVector point, std::vector<CBsp*>* bspArray); 00200 void getHitLeafs(CSphere sohere, std::vector<CBsp*>* bspArray); 00201 00202 00203 }; 00204 00205 #endif
Generated on Tue Feb 8 21:59:02 2005 for MarSTDv2004 by
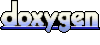