LAR Library
1.14
|
task.h
Go to the documentation of this file.
taskQueue_t * taskQueueCreate(int maxItems)
Create a new queue with up to maxItems allowed.
int taskEventWaitAny(taskEvent_t *events[], int nevents, uint32_t timeout)
Wait for at least one among a list of events to be signaled.
taskThread_t * taskThreadCreate(taskThreadFunction_t fn, void *param, uint32_t stackSize)
Create a new execution thread with entry point fn.
taskSemaphore_t * taskSemaphoreCreate(int limit)
Create a semaphore with a maximum entry count of limit.
int taskQueuePop(taskQueue_t *q, void **item, uint32_t timeout)
Extract the element from the front of the queue.
void(* taskThreadFunction_t)(void *param)
Type of a function pointer to be used as entry point of a new thread.
Definition: task.h:181
void taskEventDestroy(taskEvent_t *e)
Release the resources associated with an event handler.
void taskQueueDestroy(taskQueue_t *q)
Destroy a queue and discards all pending elements.
taskThread_t * taskThreadCurrent(void)
Return the handle of the current running thread.
int taskEventWait(taskEvent_t *e, uint32_t timeout)
Wait for e to change to signaled state.
int taskQueuePost(taskQueue_t *q, void *item)
Insert an element on the back of the queue q.
int taskEventCheck(taskEvent_t *e)
Check if an event if signaled, without waiting or clearing the signal.
Larlib basic definitions.
taskEvent_t * taskEventCreateSystem(uint32_t bitmask)
Creates an event handler to wait for system peripheral events.
int taskSemaphoreAcquire(taskSemaphore_t *s, uint32_t timeout)
Acquire (also called wait or down) the semaphore.
int taskSemaphoreRelease(taskSemaphore_t *s)
Release (also called signal or up) a previously acquired semaphore.
Generated on Mon Mar 27 2017 15:42:52 for LAR Library by
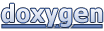