LAR Library
1.14
|
Data Structures | |
struct | dateDate_t |
A gregorian date. More... | |
struct | dateTime_t |
A gregorian date with time. More... | |
Enumerations | |
enum | dateMonths_t { DATE_JAN = 1, DATE_FEB, DATE_MAR, DATE_APR, DATE_MAY, DATE_JUN, DATE_JUL, DATE_AUG, DATE_SEP, DATE_OCT, DATE_NOV, DATE_DEC } |
Enumeration of months. More... | |
enum | dateWeekdays_t { DATE_SUN = 0, DATE_MON, DATE_TUE, DATE_WED, DATE_THU, DATE_FRI, DATE_SAT } |
Enumeration of days of a week. More... | |
Functions | |
int | dateGet (dateDate_t *d) |
Return the current system date. More... | |
int | dateTimeGet (dateTime_t *dt) |
Return the current system date and time. More... | |
uint64_t | dateTimeGetMs (void) |
Return an increasing count of milliseconds. More... | |
int | dateSet (const dateDate_t *d) |
Update the system date. More... | |
int | dateTimeSet (const dateTime_t *dt) |
Update the system date and time. More... | |
int | dateToJulianDay (const dateDate_t *d) |
Return the Julian Day Number of d . More... | |
int | dateFromJulianDay (int jdn, dateDate_t *d) |
Convert the Julian Day Number to the Gregorian calendar. More... | |
int | dateWeekday (const dateDate_t *d) |
Return the weekday of a given date. More... | |
int | dateIsLeap (uint16_t year) |
Return non-zero if given year number is a leap year. More... | |
int | dateIsValid (const dateDate_t *d) |
Checks if a date is valid. More... | |
int | dateTimeIsValid (const dateTime_t *dt) |
Checks if both time and date are valid. More... | |
int | dateDiff (const dateDate_t *a, const dateDate_t *b) |
Return the difference, in days, between two dates. More... | |
int | dateAddDays (dateDate_t *d, int ndays) |
Add a number of days to a given date. More... | |
int | dateCompare (const dateDate_t *a, const dateDate_t *b) |
Compare two dates. More... | |
int | dateTimeAddSeconds (dateTime_t *dt, int nsecs) |
Add seconds to a given date and time. More... | |
int | dateTimeCompare (const dateTime_t *a, const dateTime_t *b) |
Compare two date times. More... | |
int | dateTimeDiff (const dateTime_t *a, const dateTime_t *b) |
Return the difference, in seconds, between two date times. More... | |
Detailed Description
Rationale
Date handling is widely known to be hard. Our day-to-day uses are basically reading and setting the system date, and doing simple date math to skip over weekends and computing closing and opening times.
Introduction
This module provides basic functionality to work with dates and time. Time-zones or daylight saving time are ignored, all operations are assumed to take place in a common neutral time zone.
Enumeration Type Documentation
enum dateMonths_t |
Enumeration of months.
Notice that DATE_JAN is 1
, there is no month zero.
enum dateWeekdays_t |
Function Documentation
int dateAddDays | ( | dateDate_t * | d, |
int | ndays | ||
) |
Add a number of days to a given date.
- Parameters
-
[in,out] d Date to be modified in place. ndays Number of days to add, positive or negative.
- Returns
- BASE_ERR_OK on success.
-
BASE_ERR_INVALID_PARAMETER if
d == NULL
or!dateIsValid(d)
.
int dateCompare | ( | const dateDate_t * | a, |
const dateDate_t * | b | ||
) |
Compare two dates.
- Precondition
dateIsValid(a) && dateIsValid(b)
- See also
- dateDiff()
- Parameters
-
a Left-hand of comparison. b Right-hand of comparison.
- Returns
- > 0 if
a > b
. -
== 0 if
a == b
or eithera
orb
are invalid. -
< 0 if
a < b
.
int dateDiff | ( | const dateDate_t * | a, |
const dateDate_t * | b | ||
) |
Return the difference, in days, between two dates.
Given the defined possible return values for this function, it is recommended to check if both a
and b
are valid before calling:
- Precondition
dateIsValid(a) && dateIsValid(b)
- Parameters
-
a Minuend. b Subtrahend.
- Returns
- The difference
a - b
in days. This maybe a positive or negative number. -
Zero if either
a
orb
are invalid. Note that there is no way to differentiate this case froma == b
, the caller must guarantee that the preconditions apply.
int dateFromJulianDay | ( | int | jdn, |
dateDate_t * | d | ||
) |
Convert the Julian Day Number to the Gregorian calendar.
- Parameters
-
jdn The Julian Day Number to convert. [out] d Gregorian date.
- Returns
- BASE_ERR_OK on success.
-
BASE_ERR_INVALID_PARAMETER if
d == NULL
orjdn < 0
.
int dateGet | ( | dateDate_t * | d | ) |
Return the current system date.
- Parameters
-
[out] d Current system date.
- Returns
- BASE_ERR_OK on success.
-
BASE_ERR_INVALID_PARAMETER if
d == NULL
.
int dateIsLeap | ( | uint16_t | year | ) |
Return non-zero if given year number is a leap year.
- Parameters
-
year Year to check, with century (for example 1998 or 2011).
- Returns
- Zero if
year
is not a leap year. -
Non-zero if
year
is a leap year.
int dateIsValid | ( | const dateDate_t * | d | ) |
Checks if a date is valid.
Takes into account leap years.
- Parameters
-
d Date to verify.
- Returns
- Zero if
d
is invalid, this includesd == NULL
. -
Non-zero if
d
is valid.
int dateSet | ( | const dateDate_t * | d | ) |
Update the system date.
- Parameters
-
d Date to set.
- Returns
- BASE_ERR_OK on success.
-
BASE_ERR_INVALID_PARAMETER if
d == NULL
or!dateIsValid(d)
. - BASE_ERR_ACCESS if the application does not have permission to change the system date.
int dateTimeAddSeconds | ( | dateTime_t * | dt, |
int | nsecs | ||
) |
Add seconds to a given date and time.
- Parameters
-
[in,out] dt Date time to be modified in place. nsecs Number of seconds to add, positive or negative. The date component is also updated if necessary.
- Returns
- BASE_ERR_OK on success.
-
BASE_ERR_INVALID_PARAMETER if
dt == NULL
or!timeIsValid(dt)
.
int dateTimeCompare | ( | const dateTime_t * | a, |
const dateTime_t * | b | ||
) |
Compare two date times.
- Precondition
timeIsValid(a) && timeIsValid(b)
- Parameters
-
a Left-hand of comparison. b Right-hand of comparison.
- Returns
- > 0 if
a > b
. -
== 0 if
a == b
or eithera
orb
are invalid. -
< 0 if
a < b
.
int dateTimeDiff | ( | const dateTime_t * | a, |
const dateTime_t * | b | ||
) |
Return the difference, in seconds, between two date times.
Given the defined possible return values for this function, it is recommended to check if both a
and b
are valid before calling:
- Precondition
timeIsValid(a) && timeIsValid(b)
- Parameters
-
a Minuend. b Subtrahend.
- Note
- Given the limited return range, this function may not work properly if
a
andb
are too far way (about 68 years in 32-bit machines).
- Returns
- The difference
a - b
in seconds. This maybe a positive or negative number. -
Zero if either
a
orb
are invalid. Note that there is no way to differentiate this case froma == b
, the caller must guarantee that the preconditions apply.
int dateTimeGet | ( | dateTime_t * | dt | ) |
Return the current system date and time.
- Parameters
-
[out] dt Current system date and time.
- Returns
- BASE_ERR_OK on success.
-
BASE_ERR_INVALID_PARAMETER if
dt == NULL
.
uint64_t dateTimeGetMs | ( | void | ) |
Return an increasing count of milliseconds.
Given the architectural differences, this call does not guarantee from when the count starts. It may start on the device boot or may be the number of milliseconds since a certain past date.
Also, there is no guarantee that the resolution is effectively "thousands of seconds", it may be "hundreds of seconds", for example.
The only guarantee is that in two sequential calls, the second one will never return a value smaller than the first, and that the returned value increases in a constant frequency.
Given those limitations, this call is intended to be used as a chronometer, not to actually mark current time.
- Returns
- Number of milliseconds since a certain date.
int dateTimeIsValid | ( | const dateTime_t * | dt | ) |
Checks if both time and date are valid.
- Parameters
-
dt Date/time to verify.
- Returns
- Zero if
d
is invalid, this includesd == NULL
. -
Non-zero if
d
is valid.
int dateTimeSet | ( | const dateTime_t * | dt | ) |
Update the system date and time.
- Parameters
-
dt Date and time to set.
- Returns
- BASE_ERR_OK on success.
-
BASE_ERR_INVALID_PARAMETER if
dt == NULL
or!dateIsValid(dt)
. - BASE_ERR_ACCESS if the application does not have permission to change the system date.
int dateToJulianDay | ( | const dateDate_t * | d | ) |
Return the Julian Day Number of d
.
- Precondition
dateIsValid(d)
- Parameters
-
d Date to convert.
- Returns
- The non-negative number of days since January 1, 4713 BC, aka Julian Day Number.
-
BASE_ERR_INVALID_PARAMETER if
d == NULL
or!dateIsValid(d)
.
int dateWeekday | ( | const dateDate_t * | d | ) |
Return the weekday of a given date.
- Precondition
dateIsValid(d)
- Parameters
-
d Date to be processed.
- Returns
- Weekday of the date in
d
. See dateWeekdays_t. -
BASE_ERR_INVALID_PARAMETER if
d == NULL
or!dateIsValid(d)
.
Generated on Mon Mar 27 2017 15:42:52 for LAR Library by
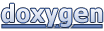