LAR Library
1.14
|
Data Structures | |
struct | iniElement_t |
Descriptor of elements allowed in an INI file. More... | |
Typedefs | |
typedef char *(* | iniLineReader_t) (void *p, char *line, int maxline) |
Signature of function used by iniParseLines() to read next line from input file. More... | |
Functions | |
int | iniParse (iniElement_t elements[], const char *fileName) |
Process the given INI file and calls handler functions according to the element table given. More... | |
int | iniParseString (iniElement_t elements[], const char *contents) |
Process a previously INI file loaded in memory. More... | |
int | iniParseLines (iniElement_t elements[], iniLineReader_t readLine, void *p) |
Process the lines of an INI file returned from repeated calls to readLine . More... | |
Detailed Description
Rationale
INI files are a common and simple way to specify application parameters in a user-readable and editable manner. Even if the application does not use INI files internally to store configuration data, it can use it to read external user parameters and convert to an internal format.
Introduction
Read/parsing of MS-like INI files.
Only a subset of INI file syntax is accepted:
- Comments start with ';' and must be the first non-space char on a line. Also, comments must be on a line on their own;
- Key names may include spaces in the middle;
- Values may be literals (value) or strings ("value"), literals have spaces before and after trimmed, strings are "as-is";
- Values are optional, they are considered
NULL
if not present; - Keys and Groups are not case-sensitive;
- Groups are marked using
[group name]
and spaces inside the[]
are important.
For example, the pair key name = key value
will be parsed as "key name"
is assigned the value of "key
value"
, whereas key name = " key value "
will have a value of " key value "
(including the spaces, but not the quotes).
Example:
This is a valid INI file that matches the description above:
- Attention
- Remember that the scanning is done from top to bottom, so a very generic entry near the top (i.e. with both
key
andgroup
asNULL
) will be matched before any more specific entries below!
Typedef Documentation
typedef char*(* iniLineReader_t) (void *p, char *line, int maxline) |
Signature of function used by iniParseLines() to read next line from input file.
User-defined functions must follow the signature and behavior defined here.
Each call to a iniLineReader_t should read the next line of input. The p
parameter is the same that is given to iniParseLines() and should be used to identify the source of input.
- Parameters
-
p Opaque pointer passed as parameter to iniParseLines() line Where to store the line read maxline Max number of chars to write to maxline
- Returns
line
on success,NULL
on error.
Function Documentation
int iniParse | ( | iniElement_t | elements[], |
const char * | fileName | ||
) |
Process the given INI file and calls handler functions according to the element table given.
For each key = value
pair found in the input file, the list elements
is scanned sequentially until a match is found, and the associated handler()
function is called. See iniElement_t for information on how matching is done, specially when iniElement_t::group and iniElement_t::key are NULL
.
- Parameters
-
elements List of allowed elements. An entry with handler = NULL
must terminate the list.fileName Name of INI file to parse.
- Returns
- BASE_ERR_OK if file was processed OK.
-
BASE_ERR_DATA_NOT_FOUND if the file
fileName
was not found. - The error code returned by one of the handler functions.
int iniParseLines | ( | iniElement_t | elements[], |
iniLineReader_t | readLine, | ||
void * | p | ||
) |
Process the lines of an INI file returned from repeated calls to readLine
.
This call behaves as iniParse(), but, instead of reading the input from a file, interprets whatever is returned by repeated calls to readLine
.
See the documentation of iniLineReader_t for more information on how readLine
should behave.
- Parameters
-
elements List of allowed elements. An entry with handler = NULL
must terminate the list.readLine Function called to read each line of input. Parsing terminates when readLine
returnsNULL
.p Passed as the first parameter to readLine
. Not accessed in any other way.
- Returns
- BASE_ERR_OK if file was processed OK.
-
BASE_ERR_INVALID_PARAMETER if
elements
orreadLine
areNULL
- The error code returned by one of the handler functions.
int iniParseString | ( | iniElement_t | elements[], |
const char * | contents | ||
) |
Process a previously INI file loaded in memory.
This call behaves as iniParse(), but, instead of reading the input from a file, directly decodes contents
, that should be a zero-terminated string with a valid INI file.
- Parameters
-
elements List of allowed elements. An entry with handler = NULL
must terminate the list.contents Zero-terminated string with the contents of the INI file.
- Returns
- BASE_ERR_OK if file was processed OK.
-
BASE_ERR_INVALID_PARAMETER if
elements
orcontents
areNULL
- The error code returned by one of the handler functions.
Generated on Mon Mar 27 2017 15:42:53 for LAR Library by
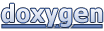