LAR Library
1.14
|
Set of pre-defined formatting functions. More...
Functions | |
int | formatFilterISO8583 (const format_t *fmt, const formatField_t *field) |
Use this function as the filter function for a ISO8583 buffer. More... | |
int | formatBodyISO8583 (uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field) |
Read/write the bitmap of a ISO8583 message. More... | |
int | formatBodyPacked (uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field) |
Format a field as a recursive call to formatPack() and formatUnpack(). More... | |
int | formatBodySkip (uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field) |
Skip field->size bytes from message. More... | |
int | formatBodyMemcpy (uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field) |
Copy field->size bytes of field->data to/from buffer . More... | |
int | formatBodyStrcpy (uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field) |
Formatting for variable-sized zero-terminated strings. More... | |
int | formatBodyUint64Dec (uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field) |
Format an uint64_t field as a decimal string. More... | |
int | formatBodyUint32Dec (uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field) |
Format an uint32_t field as a decimal string. More... | |
int | formatBodyUint16Dec (uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field) |
Format an uint16_t field as a decimal string. More... | |
int | formatBodyUint8Dec (uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field) |
Format an uint8_t field as a decimal string. More... | |
int | formatBodyUint64Buf (uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field) |
Format an uint64_t field as a sequence of bytes. More... | |
int | formatBodyBufHex (uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field) |
Format an buffer field as a hexadecimal string. More... | |
int | formatSizeAsc2 (uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field) |
Read or write the value of bodySize as a 2-digit base-10 string. More... | |
int | formatSizeAsc3 (uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field) |
Read or write the value of bodySize as a 3-digit base-10 string. More... | |
int | formatSizeBcd2 (uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field) |
Read of write the value of bodySize as a 2-digit BCD number (1 byte). More... | |
int | formatSizeBcd3 (uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field) |
Read of write the value of bodySize as a 3-digit BCD number (2 bytes). More... | |
int | formatPadSpaces (uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field) |
Write or skip field->size - field->bodySize spaces. More... | |
int | formatPadZeroes (uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field) |
Write or skip field->size - field->bodySize ASCII '0' chars. More... | |
int | formatPadZeroBytes (uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field) |
Write or skip field->size - field->bodySize 0x00 bytes. More... | |
int | formatPadSkip (uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field) |
Skip exactly field->size - field->bodySize bytes. More... | |
Detailed Description
Set of pre-defined formatting functions.
- Todo:
- Review the list of format functions and add new ones.
Function Documentation
int formatBodyBufHex | ( | uint8_t * | buffer, |
int | limit, | ||
formatDirection_t | direction, | ||
format_t * | fmt, | ||
formatField_t * | field | ||
) |
Format an buffer
field as a hexadecimal string.
field->data
must point to an char
array, its values will be read and written as a string in base-16.
- Note
- No padding is done, the return is the number of hexadecimal digits actually used. Use
prefix
orsuffix
for padding.
- Parameters
-
buffer Output buffer. limit Max number of bytes to write to buffer
.direction FORMAT_PACK or FORMAT_UNPACK fmt Formatting context field Information about the field.
- Returns
- Number of bytes actually written to
buffer
, or number of bytes read. - Negative number on error.
int formatBodyISO8583 | ( | uint8_t * | buffer, |
int | limit, | ||
formatDirection_t | direction, | ||
format_t * | fmt, | ||
formatField_t * | field | ||
) |
Read/write the bitmap of a ISO8583 message.
field->size
must include the size of the bitmap, in bytes (8 for 64-bits, 16 for 128-bits).
- Parameters
-
buffer Output buffer. limit Max number of bytes to write to buffer
.direction FORMAT_PACK or FORMAT_UNPACK fmt Formatting context field Information about the ISO8583 bitmap.
- Returns
- Positive number of bytes actually read or written.
- Negative number on error.
int formatBodyMemcpy | ( | uint8_t * | buffer, |
int | limit, | ||
formatDirection_t | direction, | ||
format_t * | fmt, | ||
formatField_t * | field | ||
) |
Copy field->size
bytes of field->data
to/from buffer
.
- Parameters
-
buffer Output buffer. limit Max number of bytes to write to buffer
.direction FORMAT_PACK or FORMAT_UNPACK fmt Formatting context field Information about the field.
- Returns
- Positive number of bytes actually read or written (always
field->size
). - Negative number on error.
int formatBodyPacked | ( | uint8_t * | buffer, |
int | limit, | ||
formatDirection_t | direction, | ||
format_t * | fmt, | ||
formatField_t * | field | ||
) |
Format a field as a recursive call to formatPack() and formatUnpack().
Packs the field by calling formatPack(field->data, buffer, limit)
and unpacks the field by calling formatUnpack(field->data, buffer, field->bodySize)
.
- Parameters
-
buffer Output buffer. limit Max number of bytes to write to buffer
.direction FORMAT_PACK or FORMAT_UNPACK fmt Formatting context field Information about this field, field->data
should point to a format_t instance.
- Returns
- Positive number of bytes actually read or written.
- Negative number on error.
int formatBodySkip | ( | uint8_t * | buffer, |
int | limit, | ||
formatDirection_t | direction, | ||
format_t * | fmt, | ||
formatField_t * | field | ||
) |
Skip field->size
bytes from message.
- Parameters
-
buffer Output buffer. limit Max number of bytes to write to buffer
.direction FORMAT_PACK or FORMAT_UNPACK fmt Formatting context field Information about the field.
- Returns
- Positive number of bytes actually skipped (always
field->size
). - Negative number on error.
int formatBodyStrcpy | ( | uint8_t * | buffer, |
int | limit, | ||
formatDirection_t | direction, | ||
format_t * | fmt, | ||
formatField_t * | field | ||
) |
Formatting for variable-sized zero-terminated strings.
- Parameters
-
buffer Output buffer. limit Max number of bytes to write to buffer
.direction FORMAT_PACK or FORMAT_UNPACK fmt Formatting context field Information about the field.
- Returns
- Number of bytes actually written to
buffer
, or number of bytes read (alwaysbodySize
). - Negative number on error.
int formatBodyUint16Dec | ( | uint8_t * | buffer, |
int | limit, | ||
formatDirection_t | direction, | ||
format_t * | fmt, | ||
formatField_t * | field | ||
) |
Format an uint16_t
field as a decimal string.
field->data
must point to an unsigned short
, its value will be read and written as a string in base-10.
- Note
- No padding with zeroes is done, the return is the number of decimal digits actually used. Use
prefix
orsuffix
for padding. Also note that negative numbers are not supported!
- Parameters
-
buffer Output buffer. limit Max number of bytes to write to buffer
.direction FORMAT_PACK or FORMAT_UNPACK fmt Formatting context field Information about the field.
- Returns
- Number of bytes actually written to
buffer
, or number of bytes read. - Negative number on error.
int formatBodyUint32Dec | ( | uint8_t * | buffer, |
int | limit, | ||
formatDirection_t | direction, | ||
format_t * | fmt, | ||
formatField_t * | field | ||
) |
Format an uint32_t
field as a decimal string.
field->data
must point to an unsigned int
, its value will be read and written as a string in base-10.
- Note
- No padding with zeroes is done, the return is the number of decimal digits actually used. Use
prefix
orsuffix
for padding. Also note that negative numbers are not supported!
- Parameters
-
buffer Output buffer. limit Max number of bytes to write to buffer
.direction FORMAT_PACK or FORMAT_UNPACK fmt Formatting context field Information about the field.
- Returns
- Number of bytes actually written to
buffer
, or number of bytes read. - Negative number on error.
int formatBodyUint64Buf | ( | uint8_t * | buffer, |
int | limit, | ||
formatDirection_t | direction, | ||
format_t * | fmt, | ||
formatField_t * | field | ||
) |
Format an uint64_t
field as a sequence of bytes.
field->data
must point to an uint64_t
, its value will be read and written as a sequence of bytes in big-endian order.
- Parameters
-
buffer Output buffer. limit Max number of bytes to write to buffer
.direction FORMAT_PACK or FORMAT_UNPACK fmt Formatting context field Information about the field.
- Returns
- Number of bytes actually written to
buffer
, or number of bytes read. - Negative number on error
int formatBodyUint64Dec | ( | uint8_t * | buffer, |
int | limit, | ||
formatDirection_t | direction, | ||
format_t * | fmt, | ||
formatField_t * | field | ||
) |
Format an uint64_t
field as a decimal string.
field->data
must point to an uint64_t
, its value will be read and written as a string in base-10.
- Note
- No padding with zeroes is done, the return is the number of decimal digits actually used. Use
prefix
orsuffix
for padding. Also note that negative numbers are not supported!
- Parameters
-
buffer Output buffer. limit Max number of bytes to write to buffer
.direction FORMAT_PACK or FORMAT_UNPACK fmt Formatting context field Information about the field.
- Returns
- Number of bytes actually written to
buffer
, or number of bytes read. - Negative number on error.
int formatBodyUint8Dec | ( | uint8_t * | buffer, |
int | limit, | ||
formatDirection_t | direction, | ||
format_t * | fmt, | ||
formatField_t * | field | ||
) |
Format an uint8_t
field as a decimal string.
field->data
must point to an unsigned char
, its value will be read and written as a string in base-10.
- Note
- No padding with zeroes is done, the return is the number of decimal digits actually used. Use
prefix
orsuffix
for padding. Also note that negative numbers are not supported!
- Parameters
-
buffer Output buffer. limit Max number of bytes to write to buffer
.direction FORMAT_PACK or FORMAT_UNPACK fmt Formatting context field Information about the field.
- Returns
- Number of bytes actually written to
buffer
, or number of bytes read. - Negative number on error.
int formatFilterISO8583 | ( | const format_t * | fmt, |
const formatField_t * | field | ||
) |
Use this function as the filter
function for a ISO8583 buffer.
This function uses field->index
as the index of a bit in fmt->fieldMap
to check if this field should be read or written.
Always return non-zero (allowed) if fieldMap
is NULL
or field->index
is negative.
- Parameters
-
fmt Formatting instance. field Pointer to field data.
- Returns
- Non-zero if
field
should be read or written. - Zero if not.
int formatPadSkip | ( | uint8_t * | buffer, |
int | limit, | ||
formatDirection_t | direction, | ||
format_t * | fmt, | ||
formatField_t * | field | ||
) |
Skip exactly field->size - field->bodySize
bytes.
- Attention
- Nothing is written to the output buffer during FORMAT_PACK!
- Parameters
-
buffer Output buffer. limit Max number of bytes to write to buffer
.direction FORMAT_PACK or FORMAT_UNPACK fmt Formatting context field Information about the field.
- Returns
field->size - field->bodySize
int formatPadSpaces | ( | uint8_t * | buffer, |
int | limit, | ||
formatDirection_t | direction, | ||
format_t * | fmt, | ||
formatField_t * | field | ||
) |
Write or skip field->size - field->bodySize
spaces.
- Parameters
-
buffer Output buffer. limit Max number of bytes to write to buffer
.direction FORMAT_PACK or FORMAT_UNPACK fmt Formatting context field Information about the field.
- Returns
field->size - field->bodySize
- Negative number on error.
int formatPadZeroBytes | ( | uint8_t * | buffer, |
int | limit, | ||
formatDirection_t | direction, | ||
format_t * | fmt, | ||
formatField_t * | field | ||
) |
Write or skip field->size - field->bodySize
0x00 bytes.
- Parameters
-
buffer Output buffer. limit Max number of bytes to write to buffer
.direction FORMAT_PACK or FORMAT_UNPACK fmt Formatting context field Information about the field.
- Returns
field->size - field->bodySize
- Negative number on error.
int formatPadZeroes | ( | uint8_t * | buffer, |
int | limit, | ||
formatDirection_t | direction, | ||
format_t * | fmt, | ||
formatField_t * | field | ||
) |
Write or skip field->size - field->bodySize
ASCII '0' chars.
- Parameters
-
buffer Output buffer. limit Max number of bytes to write to buffer
.direction FORMAT_PACK or FORMAT_UNPACK fmt Formatting context field Information about the field.
- Returns
field->size - field->bodySize
- Negative number on error.
int formatSizeAsc2 | ( | uint8_t * | buffer, |
int | limit, | ||
formatDirection_t | direction, | ||
format_t * | fmt, | ||
formatField_t * | field | ||
) |
Read or write the value of bodySize
as a 2-digit base-10 string.
- Parameters
-
buffer Output buffer. limit Max number of bytes to write to buffer
.direction FORMAT_PACK or FORMAT_UNPACK fmt Formatting context field Information about the field. Only bodySize
is used.
- Returns
- Number of bytes actually written to
buffer
, or number of bytes read. - Negative number on error.
int formatSizeAsc3 | ( | uint8_t * | buffer, |
int | limit, | ||
formatDirection_t | direction, | ||
format_t * | fmt, | ||
formatField_t * | field | ||
) |
Read or write the value of bodySize
as a 3-digit base-10 string.
- Parameters
-
buffer Output buffer. limit Max number of bytes to write to buffer
.direction FORMAT_PACK or FORMAT_UNPACK fmt Formatting context field Information about the field. Only bodySize
is used.
- Returns
- Number of bytes actually written to
buffer
, or number of bytes read. - Negative number on error.
int formatSizeBcd2 | ( | uint8_t * | buffer, |
int | limit, | ||
formatDirection_t | direction, | ||
format_t * | fmt, | ||
formatField_t * | field | ||
) |
Read of write the value of bodySize
as a 2-digit BCD number (1 byte).
- Parameters
-
buffer Output buffer. limit Max number of bytes to write to buffer
.direction FORMAT_PACK or FORMAT_UNPACK fmt Formatting context field Information about the field. Only bodySize
is used.
- Returns
- Number of bytes actually written to
buffer
, or number of bytes read. - Negative number on error.
int formatSizeBcd3 | ( | uint8_t * | buffer, |
int | limit, | ||
formatDirection_t | direction, | ||
format_t * | fmt, | ||
formatField_t * | field | ||
) |
Read of write the value of bodySize
as a 3-digit BCD number (2 bytes).
- Parameters
-
buffer Output buffer. limit Max number of bytes to write to buffer
.direction FORMAT_PACK or FORMAT_UNPACK fmt Formatting context field Information about the field. Only bodySize
is used.
- Returns
- Number of bytes actually written to
buffer
, or number of bytes read. - Negative number on error.
Generated on Mon Mar 27 2017 15:42:53 for LAR Library by
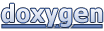