LAR Library
1.14
|
Macros | |
#define | LOG_ALL_CHANNELS 0, 256 |
This is a short-cut to define the parameters of all channels in one single call to logSetChannels(). | |
#define | LOG_ASSERT(cond) |
Assertion using larlib.log for output. More... | |
Typedefs | |
typedef int(* | logWriteFunction_t) (void *context, uint16_t channelLevel, const char *msg, int msgLength) |
Type of the function that does the actual writing on a trace channel. More... | |
typedef int(* | logDumpFunction_t) (logWriteFunction_t write, void *context, uint16_t channelLevel, const void *data, int dataLen) |
Type of function called to format the output on logDump(). More... | |
Enumerations | |
enum | logLevel_t { LOG_DEBUG = 0x0000, LOG_INFO = 0x2000, LOG_WARNING = 0x4000, LOG_ERROR = 0x6000, LOG_CRITICAL = 0x8000, LOG_ALWAYS = 0xFF00 } |
General tracing levels. More... | |
enum | logReservedChannels_t { LOG_CH_BASE = 200, LOG_CH_CONV, LOG_CH_DATE, LOG_CH_FS, LOG_CH_INI, LOG_CH_MEM, LOG_CH_TAB, LOG_CH_STEP, LOG_CH_FORMAT, LOG_CH_TASK } |
List of reserved log channels. More... | |
Functions | |
int | logWriteTeliumTrace (void *context, uint16_t channelLevel, const char *msg, int msgLength) |
A logWriteFunction_t that can be used as parameter to logSetChannels() and writes traces using Telium's trace() function. More... | |
int | logWriteTeliumRemoteDebugger (void *context, uint16_t channelLevel, const char *msg, int msgLength) |
A logWriteFunction_t that can be used as parameter to logSetChannels() and writes traces to the Remote Debugger console on IngeDev. More... | |
int | logDumpFormattedAscii (logWriteFunction_t write, void *context, uint16_t channelLevel, const void *data, int dataLen) |
dumpFormatFunction that formats the received buffer as a two-column hexadecimal and ASCII display and calls the received write function. More... | |
int | logSetChannels (uint8_t firstChannel, int numChannels, uint16_t level, logWriteFunction_t writeFunction, logDumpFunction_t dumpFormatFunction, void *context) |
Configures one or more tracing channels. More... | |
int | logChannelIsEnabled (uint16_t channelLevel) |
Check if a log channel is enabled for a specific priority level. More... | |
int | logPrintf (uint16_t channelLevel, const char *fmt,...) |
sprintf() like formatted traces. More... | |
int | logPrintvf (uint16_t channelLevel, const char *fmt, va_list va) |
A version of logPrintf() that accepts a va_list as parameter. More... | |
int | logDump (uint16_t channelLevel, const void *buffer, int size) |
Write a block of data as a binary dump. More... | |
Detailed Description
Rationale
Tracing is one of the basic helper tools for debugging. Defining a common tracing API that can be used by all LAR libraries and modules makes easier for them to interact. Nevertheless tracing should be optional and highly customizable.
Introduction
The larlib.log
module provides a general logging/tracing system with assertions and other helper routines. It is designed to be easily ported to any platform.
Its mechanics are based on the concept of tracing channels: 256 channels are available, and each one can be separately configured to be disabled, or enabled with a given configuration. An application can use different channel IDs to different modules so to conditionally enable tracing of specific modules.
By default all channels are disabled.
- Note
- Channel numbers 200 and higher are reserved for LAR's internal libraries and modules and should not be used by applications.
Given that tracing is very platform dependent, this library takes the position that all platform-dependency is moved to the configuration step, and after that the actual tracing code should not need to change.
Setup
Each channel has a separated configuration, and each configuration includes, information about how to write, and the tracing level in use.
The tracing level of a given channel is a number that define the priority of the trace. On run-time only traces with a priority value larger than or equal to the configured priority of this channel are actually written.
For example, to have all tracing disabled, except for the channel number 42, which should only print errors or higher priority:
For each channel a write
function should be given, this function is unique for each platform and device, and a format
function may also be given.
In platforms and devices where the write
function expects binary data (Telium's trace()
for instance) the format
function may not be given, and all binary data written using logDump() will be directly sent to the write
function.
In platforms where the write
function only accepts ASCII chars (the printer for example) then the format
function must be given and it should convert the binary data received by logDump() to a printable (ASCII-only) format. The provided function logDumpFormattedAscii() is an example of this formatting function, and will convert the binary data to a two-column hexadecimal/ascii format.
Telium
The Telium platform has a built-in system for tracing, using the trace()
function. Also, each trace may have an associated code (called its SAP code).
To use the built-in trace()
function, use logWriteTeliumTrace() as writeFunction
and NULL
as dumpFormatFunction
parameters to logSetChannels().
Tracing
After the configuration is done using logSetChannels(), one should use logPrintf(), logPrintvf() or logDump() to output traces:
LOG_ASSERT() uses channel LOG_CH_BASE to display the assertion failure message and resets the device. If channel LOG_CH_BASE is not enabled the message is not displayed, but the device will be reseted anyway. See logReservedChannels_t.
Macro Definition Documentation
#define LOG_ASSERT | ( | cond | ) |
Assertion using larlib.log
for output.
If cond
evaluates to zero, write an error message on channel LOG_CH_BASE and either halt execution or reset the device.
Typedef Documentation
typedef int(* logDumpFunction_t) (logWriteFunction_t write, void *context, uint16_t channelLevel, const void *data, int dataLen) |
Type of function called to format the output on logDump().
It should call the received write
function to actually output the formatted data.
- Parameters
-
write The function to call to actually generate the trace context The same context
variable that is passed as parameter to logSetChannels()channelLevel Channel and level that triggered this trace data Pointer to binary data to write. dataLen Length of data
, in bytes.
- Returns
- Zero on success, non-zero on error.
typedef int(* logWriteFunction_t) (void *context, uint16_t channelLevel, const char *msg, int msgLength) |
Type of the function that does the actual writing on a trace channel.
- Parameters
-
context The same context
variable that is passed as parameter to logSetChannels()channelLevel Channel and level that triggered this trace msg Data to write. msgLength Length, in bytes, of msg
.
- Returns
- Zero on success, non-zero on error.
Enumeration Type Documentation
enum logLevel_t |
Function Documentation
int logChannelIsEnabled | ( | uint16_t | channelLevel | ) |
Check if a log channel is enabled for a specific priority level.
Given a channelLevel
, formatted exactly as would for logPrintf() or logDump(), check if a write to this configuration would generate a trace.
- Parameters
-
channelLevel Defines both the channel and the level of this trace. The lower 8-bits define the channel to use, and the upper 8-bits define the level.
- Returns
- Non-zero if a logPrintf() or logDump() to
channelLevel
would generate a call to the configuredwriteFunction
. - Zero otherwise.
int logDump | ( | uint16_t | channelLevel, |
const void * | buffer, | ||
int | size | ||
) |
Write a block of data as a binary dump.
The output format should include both the readable part of the data pointed by buffer
and the hexadecimal of its bytes.
- Parameters
-
channelLevel Channel and level of this trace (see logPrintf()) buffer Data block to dump. size Number of bytes of buffer
to print.
- Returns
- BASE_ERR_OK
- See also
- logSetChannels
int logDumpFormattedAscii | ( | logWriteFunction_t | write, |
void * | context, | ||
uint16_t | channelLevel, | ||
const void * | data, | ||
int | dataLen | ||
) |
dumpFormatFunction
that formats the received buffer as a two-column hexadecimal and ASCII display and calls the received write
function.
This function can be used as the dumpFormatFunction
parameter of logSetChannels() for channels where write
is not ready to directly receive binary (non-ASCII) data.
- Parameters
-
write Function that will be used to actually write the formatted lines. context Ignored and passed as received to write
.channelLevel Channel and level that triggered this trace data Binary data to format. dataLen Length of data
in bytes.
- Returns
- The return of
write
.
int logPrintf | ( | uint16_t | channelLevel, |
const char * | fmt, | ||
... | |||
) |
sprintf()
like formatted traces.
This call formats and sends the message defined by fmt
using the parameters associated with the channel and priorities defined in channelLevel
.
The values in logLevel_t are defined such that a simple OR'ing of the values with the channel number work.
For example, to write to channel 42
with the default priority:
And to write to the same channel using the LOG_CRITICAL priority:
- Parameters
-
channelLevel Defines both the channel and the level of this trace. The lower 8-bits define the channel to use, and the upper 8-bits define the level. fmt String format (as sprintf()
).
- Returns
- BASE_ERR_OK even if
channel
is disabled or message not written by priority -
An error code returned by the
writeFunction
defined for this channel
- See also
- logSetChannels
int logPrintvf | ( | uint16_t | channelLevel, |
const char * | fmt, | ||
va_list | va | ||
) |
A version of logPrintf() that accepts a va_list
as parameter.
This is quite useful for creating other abstractions on top of this lib.
- Parameters
-
channelLevel Channel and level of tracing for this trace (see logPrintf()). fmt String format (as sprintf()
).va va_list
with the list of parameters to be formatted.
- Returns
- BASE_ERR_OK
- See also
- logSetChannels
int logSetChannels | ( | uint8_t | firstChannel, |
int | numChannels, | ||
uint16_t | level, | ||
logWriteFunction_t | writeFunction, | ||
logDumpFunction_t | dumpFormatFunction, | ||
void * | context | ||
) |
Configures one or more tracing channels.
Configures the first numChannels
starting at firstChannel
to use the tracing priority level
and write output using writeFunction
and dumpFormatFunction
.
For example, to configure the channels in the range [5,10]
:
To configure all channels, use the macro LOG_ALL_CHANNELS:
- Parameters
-
firstChannel Index of the first channel to configure. numChannels How many channels starting at firstChannel
to configure. If zero no channels are configured!level Tracing level enabled for the selected channels. Only the most significant 8-bits are used, see the values in logLevel_t. writeFunction A function that is called with the string to write. It is called only with a zero-terminated string of chars in the ASCII range. Using NULL
disables this channel.dumpFormatFunction A function that is called to pre-process the binary buffer received by logDump(). If NULL
then logDump() will directly callwriteFunction
with the received data buffer. If notNULL
then this function should format the input buffer and call the receivedwrite
function with the formatted output. See logDumpFormattedAscii().context This value is stored and passed unchanged to writeFunction
, and can be used to configure its behavior. See the documentation of each logWrite function for more information.
- Returns
- BASE_ERR_OK
-
BASE_ERR_INVALID_PARAMETER if the range defined by
firstChannel
andnumChannels
is invalid
int logWriteTeliumRemoteDebugger | ( | void * | context, |
uint16_t | channelLevel, | ||
const char * | msg, | ||
int | msgLength | ||
) |
A logWriteFunction_t that can be used as parameter to logSetChannels() and writes traces to the Remote Debugger console on IngeDev.
- Attention
- This function is Telium-specific and may not be defined on other platforms.
- Parameters
-
context Not used channelLevel Channel and level that triggered this trace msg Zero-terminated string to write. msgLength Length of msg
in bytes.
- Returns
- Always return BASE_ERR_OK.
- See also
- logSetChannels
int logWriteTeliumTrace | ( | void * | context, |
uint16_t | channelLevel, | ||
const char * | msg, | ||
int | msgLength | ||
) |
A logWriteFunction_t that can be used as parameter to logSetChannels() and writes traces using Telium's trace()
function.
Traces are written using the SAP code defined by the integer value of context
. For example, to use SAP code 0xB000, use:
To configure the channel 42 to use this tracing function with SAP code 0x1234, use:
- Attention
- This function is Telium-specific and may not be defined on other platforms.
- Parameters
-
context The value of this pointer indicates which SAP code to use. channelLevel Channel and level that triggered this trace msg Zero-terminated string to write. msgLength Length of msg
in bytes.
- Returns
- Always return BASE_ERR_OK.
- See also
- logSetChannels
Generated on Mon Mar 27 2017 15:42:53 for LAR Library by
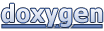