LAR Library
1.14
|
Enumerations | |
enum | convErrors_t { CONV_ERR_INVALID_BASE = -101 } |
Error codes specific to this module. More... | |
Functions | |
int | convTxtToInt (const char *txt, int cnt, int base, uint64_t *n) |
Convert Text to Integer. More... | |
int | convTxtToInt8 (const char *txt, int cnt, int base, uint8_t *n) |
Convert Text to an uint8_t. More... | |
int | convTxtToInt16 (const char *txt, int cnt, int base, uint16_t *n) |
Convert Text to an uint16_t. More... | |
int | convTxtToInt32 (const char *txt, int cnt, int base, uint32_t *n) |
Convert Text to an uint32_t. More... | |
int | convTxtToInt64 (const char *txt, int cnt, int base, uint64_t *n) |
This function is a synonym to convTxtToInt(). More... | |
int | convIntToTxt (uint64_t n, char *txt, int maxcnt, int base) |
Convert Integer to Text. More... | |
int | convIntToTxtPad (uint64_t n, char *txt, int cnt, int base) |
Convert Integer to Text padding left with zeroes to fill cnt chars. More... | |
int | convBufToHex (const uint8_t *buf, int nbuf, char *hex, int maxcnt) |
Convert buffer to hexadecimal string. More... | |
int | convHexToBuf (const char *hex, int cnt, uint8_t *buf, int maxbuf) |
Convert hexadecimal string to buffer. More... | |
int | convIntToBcd (uint64_t n, uint8_t *bcd, int maxcnt, int padRight) |
Convert Integer to BCD. More... | |
int | convIntToBcdPad (uint64_t n, uint8_t *bcd, int nibbles) |
Convert Integer to BCD with left padding. More... | |
int | convBcdToInt (const uint8_t *bcd, int cnt, uint64_t *n) |
Convert BCD to Integer. More... | |
int | convTxtToBcd (const char *txt, int maxtxt, uint8_t *bcd, int maxbcd) |
Convert a string of decimal digits (or ISO PAN) to BCD. More... | |
int | convBcdToTxt (const uint8_t *bcd, int maxbcd, char *txt, int maxtxt) |
Convert a sequence of digits in BCD format to a decimal string. More... | |
int | convIntToBuf (uint64_t n, uint8_t *buf, int maxbuf) |
Convert an integer to a sequence of bytes in big-endian ordering. More... | |
int | convIntToBufPad (uint64_t n, uint8_t *buf, int maxbuf) |
Convert an integer to a sequence of bytes in big-endian ordering and with padding. More... | |
uint64_t | convBufToInt (const uint8_t *buf, int nbuf) |
Convert a sequence of bytes in big-endian ordering to an integer. More... | |
int | convBase64ToBuf (const char *b64, int cnt, uint8_t *buf, int maxbuf) |
Decode a base-64 encoded string into a sequence of bytes. More... | |
int | convBufToBase64 (const uint8_t *buf, int nbuf, char *b64, int maxb64) |
Encode a sequence of bytes into a Base-64 string. More... | |
Detailed Description
Rationale
There are many existing conversion libraries, but all of them have their own definition of which format is what, how to name them, and API look-and-feel. This module is a unification of all existing libraries with clearer names and error handling.
Introduction
This package implements routines to convert between a few widely used formats for generic data (specially numerical data):
- Text (Txt): a string representation of a number. It only makes sense with a base. For example, the string "1001" is either 4097 in hexadecimal, 9 in binary or 1001 in decimal. Only bases in the range [2, 36] are supported.
- Integer (Int): internal numerical representation of integers for the current machine. The actual representation as bits in memory is not defined (or, more precisely, is platform dependent). In general only non-negative integers are accepted.
- Binary-Coded Decimals (Bcd): each digit of a decimal number is represented by a 4-bit value, for example the decimal number 1234 is encoded as the number 0x1234. If the number of digits is odd, the number may either start with 0x0 (as in 0x012345) or use 0xF as a terminator (0x12345F).
- Buffer (Buf): a binary representation of numbers in binary format. The decimal number 3405692655 is
"CAFEBEEF"
in base 16, and the buffer{ 0xCA, 0xFE, 0xBE, 0xEF }
. - Hexadecimal (Hex): a sub-class of Text (using base 16). It is differentiated since some functions only provide conversion to or from Hex.
- Base-64 (Base64): the common Base-64 encoding of binary data.
All routines have names with the format convXxxToYyy()
where Xxx
and Yyy
are either Bcd
, Txt
, Int
, Buf
or Hex
, and Xxx
defines the source format and Yyy
the destination format.
- Note
- For enhanced domain, all functions support Integer values represented by an
uint64_t
. This may cause a slight performance penalty in lieu of a much larger Integer range. This also means that most functions are limited to numbers up to 264-1.
Not all possible conversion routines are implemented, some conversions are used much more often than others, and this module tries to select the most used ones. Also it should provide a "path" from one format to another, so it may be possible to define a function that convert from any given format to another by calling pre-existing functions (i.e. the conversion graph should be fully connected).
Except otherwise noted, all functions are O(n) on the number of digits of the input number in whatever base and format it is to be processed.
Enumeration Type Documentation
enum convErrors_t |
Function Documentation
int convBase64ToBuf | ( | const char * | b64, |
int | cnt, | ||
uint8_t * | buf, | ||
int | maxbuf | ||
) |
Decode a base-64 encoded string into a sequence of bytes.
Use the Base-64 index table "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/".
Example:
- Parameters
-
b64 Input base-64 text, a sequence of chars from the Base-64 index table above cnt Number of chars to decode, or (-1) to use strlen()
[out] buf Where to store the decoded bytes maxbuf Max number of bytes to write to maxbuf
- Returns
- The number of bytes that
b64
decode to. If the returned value is larger thanmaxbuf
then an overflow was detected, andbuf
was not written to. -
BASE_ERR_INVALID_PARAMETER if
b64
orbuf
areNULL
Convert BCD to Integer.
- Parameters
-
bcd The BCD number to convert. cnt The max number of bytes to convert. Conversion also stops when a non-decimal nibble is found (usually 0xF
).[out] n Where to store the converted number.
- Returns
- The number of decimal digits of the number stored in
n
. -
BASE_ERR_INVALID_PARAMETER if
bcd
orn
are invalid.
int convBcdToTxt | ( | const uint8_t * | bcd, |
int | maxbcd, | ||
char * | txt, | ||
int | maxtxt | ||
) |
Convert a sequence of digits in BCD format to a decimal string.
This function was designed to be primarily used with PAN number conversion for ISO messages. It assumes that, if the sequence has an odd number of digits, it will be terminated with 0xF
.
If bcd
includes the code 0xD
, it will be changed to the char '=' on txt
. Any other digit code in bcd
terminates execution.
- Note
- The output string
txt
is NOT zero-terminated!
- Parameters
-
bcd Data in BCD format. maxbcd Max number of bytes to decode from bcd
.[out] txt Output string, will not be zero-terminated! maxtxt Max number of chars to write to txt
- Returns
- The number of valid digits in
bcd
. If this is larger thanmaxtxt
then no conversion was done. -
BASE_ERR_INVALID_PARAMETER if
bcd
isNULL
ortxt
isNULL
andmaxtxt > 0
.
int convBufToBase64 | ( | const uint8_t * | buf, |
int | nbuf, | ||
char * | b64, | ||
int | maxb64 | ||
) |
Encode a sequence of bytes into a Base-64 string.
Use the Base-64 index table "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/".
- Parameters
-
buf Input sequence of bytes nbuf Number of bytes to buf
to encode[out] b64 Where to store the encoded Base-64 chars maxb64 Max number of chars to write to b64
, including terminating zero!
- Returns
- The number of bytes necessary to fully encode
buf
. If the returned value is larger thanmaxb64
then an overflow was detected, andb64
was not written to. -
BASE_ERR_INVALID_PARAMETER if
buf
orb64
areNULL
int convBufToHex | ( | const uint8_t * | buf, |
int | nbuf, | ||
char * | hex, | ||
int | maxcnt | ||
) |
Convert buffer to hexadecimal string.
- Parameters
-
buf Buffer to convert. nbuf Number of bytes to convert from buffer. [out] hex Where to store the converted data. maxcnt Max number of digits to write to hex
(including the terminating zero!)
- Note
maxcnt
should be at least twicenbuf
as each bytes converts to two hexadecimal digits.
- Returns
- The number of digits written to
hex
. -
BASE_ERR_INVALID_PARAMETER if
buf
orhex
are invalid.
Convert a sequence of bytes in big-endian ordering to an integer.
The opposite of convIntToBuf().
- Parameters
-
buf Sequence of bytes to be converted nbuf How many bytes of buf
to convert
- Returns
- The converted value of
buf[0 .. nbuf - 1]
- Zero in any error
int convHexToBuf | ( | const char * | hex, |
int | cnt, | ||
uint8_t * | buf, | ||
int | maxbuf | ||
) |
Convert hexadecimal string to buffer.
- Parameters
-
hex Hexadecimal string to convert. cnt Max number of digits to convert from hex
. Usecnt == -1
to usestrlen(hex)
instead.[out] buf Output buffer with converted bytes. maxbuf Max number of bytes to write to buf
.
- Note
maxbuf
should be at least halfmin(strlen(hex), cnt)
, as each pair of hexadecimal digits convert to one byte.-
If the number of hexadecimal digits is odd, the last byte will be padded with 0 to the right. For example
"ABCDE"
will be encoded as{ 0xAB, 0xCD, 0xE0 }
.
If a non-hexadecimal digit is found in hex
, conversion will stop and the number of complete bytes written to buf
is returned. If the number of converted digits is odd, the last (partial) byte is not counted in the return, but will be partially filed as if the number of input digits were odd. (E.g. "ABCDEX"
returns 2 and writes {0xAB, 0xCD, 0xE0}
to buf
).
- Returns
- The number of bytes written to
buf
. -
BASE_ERR_INVALID_PARAMETER if
hex
orbuf
are invalid.
Convert Integer to BCD.
- Parameters
-
n The integer to convert. [out] bcd Where to store the BCD-coded n
. Note thatbcd
will not be zero-terminated!maxcnt Max number of bytes to write to bcd
.padRight If non-zero, the resulting BCD is padded left with a terminating 0xF
. If zero odd-sized BCD numbers start with a0x0
as padding. Note that this flag only applies to BCD numbers where the resulting number of digits is odd (thus requiring an "extra" nibble).
- Returns
- The number of bytes necessary to fully convert
n
. If> maxcnt
, then the conversion was not done, andbcd
was not modified. -
BASE_ERR_INVALID_PARAMETER if
bcd
is invalid.
Convert Integer to BCD with left padding.
This function is similar to convIntToBcd(), but instead of using the least necessary number of bytes to express the BCD encoded number, it fills all the nibbles requested in the nibbles
parameter.
If the value of nibbles
is odd, the last half of the last byte would be unused by the encoding function, and is filled with 0xF
instead.
If the value of nibbles
is even, all the bytes are wholly used, and there is no need to fill with 0xF
the last byte.
In both cases, the bcd
output buffer is prefixed with enough 0x00
bytes so that the encoding of n
uses (nibbles + 1) / 2
bytes.
- Parameters
-
n The integer to convert. [out] bcd Where to store the BCD-coded n
. Note thatbcd
will not be zero terminated. If is assumed thatbcd
has enough space for(nibble + 1) / 2
bytes.nibbles The number of nibbles to pad the converted BCD number to. See the notes above for more information.
- Returns
- If
nibbles
is too small, return the number of bytes that would be required to fully encoden
-
On success return the number of bytes actually written to
bcd
(that should always be equal to(nibbles + 1) / 2
-
BASE_ERR_INVALID_PARAMETER if
bcd
ornibbles
is invalid.
Convert an integer to a sequence of bytes in big-endian ordering.
Example:
- Parameters
-
n The integer to convert [out] buf Where to store the bytes of n
maxbuf Max number of bytes that may be written to buf
- Returns
- The number of bytes necessary to fully encode
n
. If> maxbuf
then conversion was not performed and nothing is written tobuf
-
BASE_ERR_INVALID_PARAMETER if
buf
is NULL ormaxbuf < 0
Convert an integer to a sequence of bytes in big-endian ordering and with padding.
This call is equivalent to convIntToBuf() but the output buffer buf
will be zero-padded to the left so that always maxbuf
bytes are filled.
Example:
- Parameters
-
n The integer to convert [out] buf Where to store the bytes of n
maxbuf Number of bytes that will be written to buf
- Returns
- The number of bytes written to
buf
-
In case of overflow, return how many bytes would be necessary to fully encode
n
-
BASE_ERR_INVALID_PARAMETER if
buf
is NULL ormaxbuf < 0
int convIntToTxt | ( | uint64_t | n, |
char * | txt, | ||
int | maxcnt, | ||
int | base | ||
) |
Convert Integer to Text.
If maxcnt
is not enough to store all converted digits plus the terminating zero, conversion will not be done, but the number of digits required for conversion will be returned. Calling this function with maxcnt == 0
and txt == NULL
is a valid option to calculate the number of digits necessary to represent a number on a certain base.
- Parameters
-
n Number to be converted. [out] txt Where the converted digits will be stored. maxcnt Maximum number of digits that may be written to txt (including the terminating zero!) base Base to use for txt
.
- Returns
- The number of digits that would be necessary to encode
n
totally (including the terminating zero). If the return value is>= maxcnt
the conversion did not happen andtxt
was never written to. -
CONV_ERR_INVALID_BASE if
base
is out of range. -
BASE_ERR_INVALID_PARAMETER if
txt
is invalid.
int convIntToTxtPad | ( | uint64_t | n, |
char * | txt, | ||
int | cnt, | ||
int | base | ||
) |
Convert Integer to Text padding left with zeroes to fill cnt
chars.
For example, converting the number 13 with cnt = 4
and base = 10
results in the string txt = "0013"
. Note that zero is used whichever base
is used.
- Note
- The output string
txt
is not zero-terminated!
- Parameters
-
n Number to be converted. [out] txt Where the converted digits will be stored. cnt Number of digits that should be written to txt
.base Base to use for txt
.
- Returns
- The number of significant digits of
n
inbase
(that is, discounting the zeroes to the left inserted for padding). If the return is larger thancnt
thentxt
is not large enough and no conversion was done. -
CONV_ERR_INVALID_BASE if
base
is out of range. -
BASE_ERR_INVALID_PARAMETER if
txt
is invalid.
int convTxtToBcd | ( | const char * | txt, |
int | maxtxt, | ||
uint8_t * | bcd, | ||
int | maxbcd | ||
) |
Convert a string of decimal digits (or ISO PAN) to BCD.
This function was designed to be primarily used with PAN number conversion for ISO messages. If the string is odd-sized, it is padded left with a terminating 0xF
.
If the sequence includes the char '=', it is changed to 0xD
in the BCD output. Conversion ends at any char that is not a decimal digit or '='.
- Parameters
-
txt The source string. May be zero-terminated or not, see maxcnt
.maxtxt Max numbers of chars to convert from txt
. If (-1) usestrlen(txt)
.[out] bcd Target buffer for the BCD-coded value. maxbcd Max number of bytes that can be written to bcd
.
- Returns
- The number of bytes necessary to fully convert
txt
. If the return value is larger thanmaxbcd
, then conversion was not done. -
BASE_ERR_INVALID_PARAMETER if
txt
isNULL
or ifbcd
isNULL
andmaxbcd > 0
int convTxtToInt | ( | const char * | txt, |
int | cnt, | ||
int | base, | ||
uint64_t * | n | ||
) |
Convert Text to Integer.
Up to to cnt
digits of txt
are converted to integer, using base
. The result is stored in n
.
- Parameters
-
txt String to convert. cnt Up to cnt
digits will be read fromtxt
. Conversion also stops at any digit out of range. Usecnt == -1
to usestrlen(txt)
instead.base Base of the number represented in txt
.[out] n Where the converted value should be stored.
- Returns
- The number of digits converted from
txt
. -
CONV_ERR_INVALID_BASE if
base
is out of range. -
BASE_ERR_INVALID_PARAMETER if
txt
,cnt
, orn
are invalid. - BASE_ERR_OVERFLOW if the resulting integer does not fit on a uint64_t
int convTxtToInt16 | ( | const char * | txt, |
int | cnt, | ||
int | base, | ||
uint16_t * | n | ||
) |
Convert Text to an uint16_t.
Behaves exactly as convTxtToInt(), but stores the result on a 16-bit unsigned integer instead.
- Parameters
-
txt String to convert. cnt Up to cnt
digits will be read fromtxt
. Conversion also stops at any digit out of range. Usecnt == -1
to usestrlen(txt)
instead.base Base of the number represented in txt
.[out] n Where the converted value should be stored.
- Returns
- The number of digits converted from
txt
. -
CONV_ERR_INVALID_BASE if
base
is out of range. -
BASE_ERR_INVALID_PARAMETER if
txt
,cnt
, orn
are invalid. - BASE_ERR_OVERFLOW if the resulting integer does not fit on a uint16_t
int convTxtToInt32 | ( | const char * | txt, |
int | cnt, | ||
int | base, | ||
uint32_t * | n | ||
) |
Convert Text to an uint32_t.
Behaves exactly as convTxtToInt(), but stores the result on a 32-bit unsigned integer instead.
- Parameters
-
txt String to convert. cnt Up to cnt
digits will be read fromtxt
. Conversion also stops at any digit out of range. Usecnt == -1
to usestrlen(txt)
instead.base Base of the number represented in txt
.[out] n Where the converted value should be stored.
- Returns
- The number of digits converted from
txt
. -
CONV_ERR_INVALID_BASE if
base
is out of range. -
BASE_ERR_INVALID_PARAMETER if
txt
,cnt
, orn
are invalid. - BASE_ERR_OVERFLOW if the resulting integer does not fit on a uint32_t
int convTxtToInt64 | ( | const char * | txt, |
int | cnt, | ||
int | base, | ||
uint64_t * | n | ||
) |
This function is a synonym to convTxtToInt().
It is added only for completeness, you may use it to make clear the intent of using a uint64_t as the conversion target.
- Parameters
-
txt String to convert. cnt Up to cnt
digits will be read fromtxt
. Conversion also stops at any digit out of range. Usecnt == -1
to usestrlen(txt)
instead.base Base of the number represented in txt
.[out] n Where the converted value should be stored.
- Returns
- The number of digits converted from
txt
. -
CONV_ERR_INVALID_BASE if
base
is out of range. -
BASE_ERR_INVALID_PARAMETER if
txt
,cnt
, orn
are invalid. - BASE_ERR_OVERFLOW if the resulting integer does not fit on a uint64_t
int convTxtToInt8 | ( | const char * | txt, |
int | cnt, | ||
int | base, | ||
uint8_t * | n | ||
) |
Convert Text to an uint8_t.
Behaves exactly as convTxtToInt(), but stores the result on an 8-bit unsigned integer instead.
- Parameters
-
txt String to convert. cnt Up to cnt
digits will be read fromtxt
. Conversion also stops at any digit out of range. Usecnt == -1
to usestrlen(txt)
instead.base Base of the number represented in txt
.[out] n Where the converted value should be stored.
- Returns
- The number of digits converted from
txt
. -
CONV_ERR_INVALID_BASE if
base
is out of range. -
BASE_ERR_INVALID_PARAMETER if
txt
,cnt
, orn
are invalid. - BASE_ERR_OVERFLOW if the resulting integer does not fit on a uint8_t
Generated on Mon Mar 27 2017 15:42:52 for LAR Library by
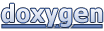