LAR Library
1.14
|
format.h
Go to the documentation of this file.
397 int (*prefix)(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field);
402 int (*body)(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field);
407 int (*suffix)(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field);
490 int formatBodyISO8583(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field);
508 int formatBodyPacked(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field);
522 int formatBodySkip (uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field);
536 int formatBodyMemcpy(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field);
550 int formatBodyStrcpy(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field);
571 int formatBodyUint64Dec(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field);
592 int formatBodyUint32Dec(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field);
613 int formatBodyUint16Dec(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field);
634 int formatBodyUint8Dec(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field);
651 int formatBodyUint64Buf(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field);
671 int formatBodyBufHex(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field);
685 int formatSizeAsc2(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field);
699 int formatSizeAsc3(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field);
713 int formatSizeBcd2(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field);
727 int formatSizeBcd3(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field);
741 int formatPadSpaces(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field);
755 int formatPadZeroes(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field);
769 int formatPadZeroBytes(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field);
784 int formatPadSkip(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field);
int formatBodyUint64Dec(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field)
Format an uint64_t field as a decimal string.
int formatPadSkip(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field)
Skip exactly field->size - field->bodySize bytes.
formatDirection_t
Used as parameter to the formatting functions to inform if they should handle packing or unpacking...
Definition: format.h:335
int formatSizeAsc3(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field)
Read or write the value of bodySize as a 3-digit base-10 string.
int formatBodyUint32Dec(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field)
Format an uint32_t field as a decimal string.
int formatSizeAsc2(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field)
Read or write the value of bodySize as a 2-digit base-10 string.
int formatBodyUint8Dec(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field)
Format an uint8_t field as a decimal string.
formatField_t * errorField
Pointer to formatField_t where error was detected.
Definition: format.h:380
int formatBodyUint16Dec(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field)
Format an uint16_t field as a decimal string.
int formatPack(format_t *fmt, uint8_t *output, int maxOutput)
Write to output the packed values as described in fmt.
void * fieldMap
Should point to an array with the information that is read by filter to decide if a field is or not a...
Definition: format.h:370
int formatBodySkip(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field)
Skip field->size bytes from message.
int formatSizeBcd3(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field)
Read of write the value of bodySize as a 3-digit BCD number (2 bytes).
int index
Index of this field (usually on fieldMap in the parent format_t).
Definition: format.h:392
int formatBodyISO8583(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field)
Read/write the bitmap of a ISO8583 message.
int formatBodyPacked(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field)
Format a field as a recursive call to formatPack() and formatUnpack().
int formatPadZeroBytes(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field)
Write or skip field->size - field->bodySize 0x00 bytes.
int formatBodyStrcpy(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field)
Formatting for variable-sized zero-terminated strings.
int(* body)(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field)
Pack/unpack actual field contents.
Definition: format.h:402
formatField_t * fields
Pointer to array of fields to be processed.
Definition: format.h:347
int formatUnpack(format_t *fmt, uint8_t *input, int inputSize)
Read from input and unpack the fields to fmt.
int formatBodyMemcpy(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field)
Copy field->size bytes of field->data to/from buffer.
int formatPadZeroes(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field)
Write or skip field->size - field->bodySize ASCII '0' chars.
Larlib basic definitions.
int formatBodyUint64Buf(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field)
Format an uint64_t field as a sequence of bytes.
int(* prefix)(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field)
Pack/unpack prefix of field.
Definition: format.h:397
int(* suffix)(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field)
Pack/unpack suffix of field.
Definition: format.h:407
int formatPadSpaces(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field)
Write or skip field->size - field->bodySize spaces.
int formatBodyBufHex(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field)
Format an buffer field as a hexadecimal string.
int bodySize
Actual size of the field, as returned by body.
Definition: format.h:423
int(* filter)(const format_t *fmt, const formatField_t *field)
This function is called before each field is processed, receiving a pointer to the field being proces...
Definition: format.h:364
int formatFilterISO8583(const format_t *fmt, const formatField_t *field)
Use this function as the filter function for a ISO8583 buffer.
int formatSizeBcd2(uint8_t *buffer, int limit, formatDirection_t direction, format_t *fmt, formatField_t *field)
Read of write the value of bodySize as a 2-digit BCD number (1 byte).
Generated on Mon Mar 27 2017 15:42:52 for LAR Library by
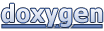