GUIQwt reference
guiqwt.pyplot
The pyplot module provides an interactive plotting interface similar to Matplotlib‘s, i.e. with MATLAB-like syntax.
The guiqwt.pyplot module was designed to be as close as possible to the matplotlib.pyplot module, so that one could easily switch between these two modules by simply changing the import statement. Basically, if guiqwt does support the plotting commands called in your script, replacing import matplotlib.pyplot by import guiqwt.pyplot should suffice, as shown in the following example:
Simple example using matplotlib:
import matplotlib.pyplot as plt import numpy as np x = np.linspace(-10, 10) plt.plot(x, x**2, 'r+') plt.show()Switching from matplotlib to guiqwt is trivial:
import guiqwt.pyplot as plt # only this line has changed! import numpy as np x = np.linspace(-10, 10) plt.plot(x, x**2, 'r+') plt.show()
Examples
>>> import numpy as np
>>> from guiqwt.pyplot import * # ugly but acceptable in an interactive session
>>> ion() # switching to interactive mode
>>> x = np.linspace(-5, 5, 1000)
>>> figure(1)
>>> subplot(2, 1, 1)
>>> plot(x, np.sin(x), "r+")
>>> plot(x, np.cos(x), "g-")
>>> errorbar(x, -1+x**2/20+.2*np.random.rand(len(x)), x/20)
>>> xlabel("Axe x")
>>> ylabel("Axe y")
>>> subplot(2, 1, 2)
>>> img = np.fromfunction(lambda x, y: np.sin((x/200.)*(y/200.)**2), (1000, 1000))
>>> xlabel("pixels")
>>> ylabel("pixels")
>>> zlabel("intensity")
>>> gray()
>>> imshow(img)
>>> figure("plotyy")
>>> plotyy(x, np.sin(x), x, np.cos(x))
>>> ylabel("sinus", "cosinus")
>>> show()
Reference
- guiqwt.pyplot.interactive(state)[source]
Toggle interactive mode
- guiqwt.pyplot.ion()[source]
Turn interactive mode on
- guiqwt.pyplot.ioff()[source]
Turn interactive mode off
- guiqwt.pyplot.figure(N=None)[source]
Create a new figure
- guiqwt.pyplot.gcf()[source]
Get current figure
- guiqwt.pyplot.gca()[source]
Get current axes
- guiqwt.pyplot.show(mainloop=True)[source]
Show all figures and enter Qt event loop This should be the last line of your script
- guiqwt.pyplot.subplot(n, m, k)[source]
Create a subplot command
Example: import numpy as np x = np.linspace(-5, 5, 1000) figure(1) subplot(2, 1, 1) plot(x, np.sin(x), “r+”) subplot(2, 1, 2) plot(x, np.cos(x), “g-”) show()
- guiqwt.pyplot.close(N=None, all=False)[source]
Close figure
- guiqwt.pyplot.title(text)[source]
Set current figure title
- guiqwt.pyplot.xlabel(bottom='', top='')[source]
Set current x-axis label
- guiqwt.pyplot.ylabel(left='', right='')[source]
Set current y-axis label
- guiqwt.pyplot.zlabel(label)[source]
Set current z-axis label
- guiqwt.pyplot.yreverse(reverse)[source]
Set y-axis direction of increasing values
- reverse = False (default)
- y-axis values increase from bottom to top
- reverse = True
- y-axis values increase from top to bottom
- guiqwt.pyplot.grid(act)[source]
Toggle grid visibility
- guiqwt.pyplot.legend(pos='TR')[source]
Add legend to current axes (pos=’TR’, ‘TL’, ‘BR’, ...)
- guiqwt.pyplot.colormap(name)[source]
Set color map to name
- guiqwt.pyplot.savefig(fname, format=None, draft=False)[source]
Save figure
Currently supports PDF and PNG formats only
- guiqwt.pyplot.plot(*args, **kwargs)[source]
Plot curves
Example:
import numpy as np x = np.linspace(-5, 5, 1000) plot(x, np.sin(x), “r+”) plot(x, np.cos(x), “g-”) show()
- guiqwt.pyplot.plotyy(x1, y1, x2, y2)[source]
Plot curves with two different y axes
Example:
import numpy as np x = np.linspace(-5, 5, 1000) plotyy(x, np.sin(x), x, np.cos(x)) ylabel(“sinus”, “cosinus”) show()
- guiqwt.pyplot.semilogx(*args, **kwargs)[source]
Plot curves with logarithmic x-axis scale
Example:
import numpy as np x = np.linspace(-5, 5, 1000) semilogx(x, np.sin(12*x), “g-”) show()
- guiqwt.pyplot.semilogy(*args, **kwargs)[source]
Plot curves with logarithmic y-axis scale
Example:
import numpy as np x = np.linspace(-5, 5, 1000) semilogy(x, np.sin(12*x), “g-”) show()
- guiqwt.pyplot.loglog(*args, **kwargs)[source]
Plot curves with logarithmic x-axis and y-axis scales
Example:
import numpy as np x = np.linspace(-5, 5, 1000) loglog(x, np.sin(12*x), “g-”) show()
- guiqwt.pyplot.errorbar(*args, **kwargs)[source]
Plot curves with error bars
Example:
import numpy as np x = np.linspace(-5, 5, 1000) errorbar(x, -1+x**2/20+.2*np.random.rand(len(x)), x/20) show()
- guiqwt.pyplot.hist(data, bins=None, logscale=None, title=None, color=None)[source]
Plot 1-D histogram
Example:
from numpy.random import normal data = normal(0, 1, (2000, )) hist(data) show()
- guiqwt.pyplot.imshow(data, interpolation=None, mask=None)[source]
Display the image in data to current axes interpolation: ‘nearest’, ‘linear’ (default), ‘antialiasing’
Example:
import numpy as np x = np.linspace(-5, 5, 1000) img = np.fromfunction(lambda x, y:
np.sin((x/200.)*(y/200.)**2), (1000, 1000))gray() imshow(img) show()
- guiqwt.pyplot.pcolor(*args)[source]
Create a pseudocolor plot of a 2-D array
Example:
import numpy as np r = np.linspace(1., 16, 100) th = np.linspace(0., np.pi, 100) R, TH = np.meshgrid(r, th) X = R*np.cos(TH) Y = R*np.sin(TH) Z = 4*TH+R pcolor(X, Y, Z) show()
guiqwt.widgets.fit
- The fit module provides an interactive curve fitting widget/dialog allowing:
- to fit data manually (by moving sliders)
- or automatically (with standard optimization algorithms provided by scipy).
Example
import numpy as np
from guiqwt.widgets.fit import FitParam, guifit
def test():
x = np.linspace(-10, 10, 1000)
y = np.cos(1.5*x)+np.random.rand(x.shape[0])*.2
def fit(x, params):
a, b = params
return np.cos(b*x)+a
a = FitParam("Offset", 1., 0., 2.)
b = FitParam("Frequency", 2., 1., 10., logscale=True)
params = [a, b]
values = guifit(x, y, fit, params, xlabel="Time (s)", ylabel="Power (a.u.)")
print values
print [param.value for param in params]
if __name__ == "__main__":
test()
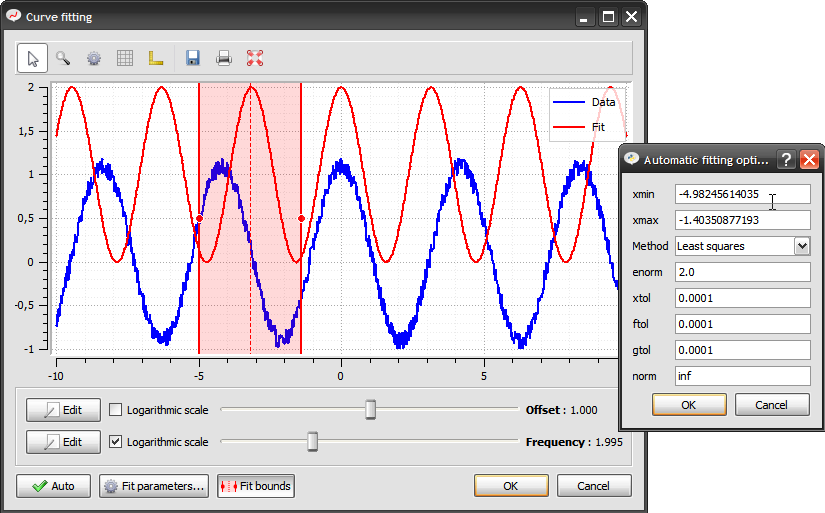
Reference
- guiqwt.widgets.fit.guifit(x, y, fitfunc, fitparams, fitargs=None, fitkwargs=None, wintitle=None, title=None, xlabel=None, ylabel=None, param_cols=1, auto_fit=True, winsize=None, winpos=None)[source]
GUI-based curve fitting tool
- class guiqwt.widgets.fit.FitDialog(wintitle=None, icon='guiqwt.svg', edit=True, toolbar=False, options=None, parent=None, panels=None, param_cols=1, legend_anchor='TR', auto_fit=False)[source]
- class RenderFlags
QWidget.RenderFlags(QWidget.RenderFlags) QWidget.RenderFlags(int) QWidget.RenderFlags()
- FitDialog.accept()
QDialog.accept()
- FitDialog.acceptDrops()
QWidget.acceptDrops() -> bool
- FitDialog.accepted
QDialog.accepted[] [signal]
- FitDialog.actions()
QWidget.actions() -> list-of-QAction
- FitDialog.activateWindow()
QWidget.activateWindow()
- FitDialog.activate_default_tool()
Activate default tool
- FitDialog.addAction()
QWidget.addAction(QAction)
- FitDialog.addActions()
QWidget.addActions(list-of-QAction)
- FitDialog.add_panel(panel)
Register a panel to the plot manager
- Plot manager’s registration sequence is the following:
- add plots
- add panels
- add tools
- FitDialog.add_plot(plot, plot_id=<class 'guiqwt.plot.DefaultPlotID'>)
- Register a plot to the plot manager:
- plot: guiqwt.curve.CurvePlot or guiqwt.image.ImagePlot object
- plot_id (default id is the plot object’s id: id(plot)): unique ID identifying the plot (any Python object), this ID will be asked by the manager to access this plot later.
- Plot manager’s registration sequence is the following:
- add plots
- add panels
- add tools
- FitDialog.add_separator_tool(toolbar_id=None)
Register a separator tool to the plot manager: the separator tool is just a tool which insert a separator in the plot context menu
- FitDialog.add_tool(ToolKlass, *args, **kwargs)
- Register a tool to the manager
- ToolKlass: tool’s class (guiqwt builtin tools are defined in module guiqwt.tools)
- *args: arguments sent to the tool’s class
- **kwargs: keyword arguments sent to the tool’s class
- Plot manager’s registration sequence is the following:
- add plots
- add panels
- add tools
- FitDialog.add_toolbar(toolbar, toolbar_id='default')
- Add toolbar to the plot manager
- toolbar: a QToolBar object toolbar_id: toolbar’s id (default id is string “default”)
- FitDialog.adjustSize()
QWidget.adjustSize()
- FitDialog.autoFillBackground()
QWidget.autoFillBackground() -> bool
- FitDialog.backgroundRole()
QWidget.backgroundRole() -> QPalette.ColorRole
- FitDialog.baseSize()
QWidget.baseSize() -> QSize
- FitDialog.blockSignals()
QObject.blockSignals(bool) -> bool
- FitDialog.childAt()
QWidget.childAt(QPoint) -> QWidget QWidget.childAt(int, int) -> QWidget
- FitDialog.children()
QObject.children() -> list-of-QObject
- FitDialog.childrenRect()
QWidget.childrenRect() -> QRect
- FitDialog.childrenRegion()
QWidget.childrenRegion() -> QRegion
- FitDialog.clearFocus()
QWidget.clearFocus()
- FitDialog.clearMask()
QWidget.clearMask()
- FitDialog.close()
QWidget.close() -> bool
- FitDialog.closeEvent()
QDialog.closeEvent(QCloseEvent)
- FitDialog.colorCount()
QPaintDevice.colorCount() -> int
- FitDialog.configure_panels()
Call all the registred panels ‘configure_panel’ methods to finalize the object construction (this allows to use tools registered to the same plot manager as the panel itself with breaking the registration sequence: “add plots, then panels, then tools”)
- FitDialog.connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- FitDialog.contentsMargins()
QWidget.contentsMargins() -> QMargins
- FitDialog.contentsRect()
QWidget.contentsRect() -> QRect
- FitDialog.contextMenuEvent()
QDialog.contextMenuEvent(QContextMenuEvent)
- FitDialog.contextMenuPolicy()
QWidget.contextMenuPolicy() -> Qt.ContextMenuPolicy
- FitDialog.cursor()
QWidget.cursor() -> QCursor
- FitDialog.customContextMenuRequested
QWidget.customContextMenuRequested[QPoint] [signal]
- FitDialog.deleteLater()
QObject.deleteLater()
- FitDialog.depth()
QPaintDevice.depth() -> int
- FitDialog.destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- FitDialog.devType()
QWidget.devType() -> int
- FitDialog.disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- FitDialog.done()
QDialog.done(int)
- FitDialog.dumpObjectInfo()
QObject.dumpObjectInfo()
- FitDialog.dumpObjectTree()
QObject.dumpObjectTree()
- FitDialog.dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- FitDialog.effectiveWinId()
QWidget.effectiveWinId() -> sip.voidptr
- FitDialog.emit()
QObject.emit(SIGNAL(), ...)
- FitDialog.ensurePolished()
QWidget.ensurePolished()
- FitDialog.eventFilter()
QDialog.eventFilter(QObject, QEvent) -> bool
- FitDialog.exec_()
QDialog.exec_() -> int
- FitDialog.extension()
QDialog.extension() -> QWidget
- FitDialog.find()
QWidget.find(sip.voidptr) -> QWidget
- FitDialog.findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- FitDialog.findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- FitDialog.finished
QDialog.finished[int] [signal]
- FitDialog.focusPolicy()
QWidget.focusPolicy() -> Qt.FocusPolicy
- FitDialog.focusProxy()
QWidget.focusProxy() -> QWidget
- FitDialog.focusWidget()
QWidget.focusWidget() -> QWidget
- FitDialog.font()
QWidget.font() -> QFont
- FitDialog.fontInfo()
QWidget.fontInfo() -> QFontInfo
- FitDialog.fontMetrics()
QWidget.fontMetrics() -> QFontMetrics
- FitDialog.foregroundRole()
QWidget.foregroundRole() -> QPalette.ColorRole
- FitDialog.frameGeometry()
QWidget.frameGeometry() -> QRect
- FitDialog.frameSize()
QWidget.frameSize() -> QSize
- FitDialog.geometry()
QWidget.geometry() -> QRect
- FitDialog.getContentsMargins()
QWidget.getContentsMargins() -> (int, int, int, int)
- FitDialog.get_active_plot()
Return the active plot
The active plot is the plot whose canvas has the focus otherwise it’s the “default” plot
- FitDialog.get_active_tool()
Return active tool
Return widget context menu – built using active tools
- FitDialog.get_contrast_panel()
Convenience function to get the contrast adjustment panel
Return None if the contrast adjustment panel has not been added to this manager
- FitDialog.get_default_plot()
Return default plot
The default plot is the plot on which tools and panels will act.
- FitDialog.get_default_tool()
Get default tool
- FitDialog.get_default_toolbar()
Return default toolbar
- FitDialog.get_fitfunc_arguments()
Return fitargs and fitkwargs
- FitDialog.get_itemlist_panel()
Convenience function to get the item list panel
Return None if the item list panel has not been added to this manager
- FitDialog.get_main()
Return the main (parent) widget
Note that for py:class:guiqwt.plot.CurveWidget or guiqwt.plot.ImageWidget objects, this method will return the widget itself because the plot manager is integrated to it.
- FitDialog.get_panel(panel_id)
Return panel from its ID Panel IDs are listed in module guiqwt.panels
- FitDialog.get_plot(plot_id=<class 'guiqwt.plot.DefaultPlotID'>)
Return plot associated to plot_id (if method is called without specifying the plot_id parameter, return the default plot)
- FitDialog.get_plots()
Return all registered plots
- FitDialog.get_tool(ToolKlass)
Return tool instance from its class
- FitDialog.get_toolbar(toolbar_id='default')
- Return toolbar from its ID
- toolbar_id: toolbar’s id (default id is string “default”)
- FitDialog.get_values()
Convenience method to get fit parameter values
- FitDialog.get_xcs_panel()
Convenience function to get the X-axis cross section panel
Return None if the X-axis cross section panel has not been added to this manager
- FitDialog.get_ycs_panel()
Convenience function to get the Y-axis cross section panel
Return None if the Y-axis cross section panel has not been added to this manager
- FitDialog.grabGesture()
QWidget.grabGesture(Qt.GestureType, Qt.GestureFlags flags=Qt.GestureFlags(0))
- FitDialog.grabKeyboard()
QWidget.grabKeyboard()
- FitDialog.grabMouse()
QWidget.grabMouse() QWidget.grabMouse(QCursor)
- FitDialog.grabShortcut()
QWidget.grabShortcut(QKeySequence, Qt.ShortcutContext context=Qt.WindowShortcut) -> int
- FitDialog.graphicsEffect()
QWidget.graphicsEffect() -> QGraphicsEffect
- FitDialog.graphicsProxyWidget()
QWidget.graphicsProxyWidget() -> QGraphicsProxyWidget
- FitDialog.hasFocus()
QWidget.hasFocus() -> bool
- FitDialog.hasMouseTracking()
QWidget.hasMouseTracking() -> bool
- FitDialog.height()
QWidget.height() -> int
- FitDialog.heightForWidth()
QWidget.heightForWidth(int) -> int
- FitDialog.heightMM()
QPaintDevice.heightMM() -> int
- FitDialog.hide()
QWidget.hide()
- FitDialog.inherits()
QObject.inherits(str) -> bool
- FitDialog.inputContext()
QWidget.inputContext() -> QInputContext
- FitDialog.inputMethodHints()
QWidget.inputMethodHints() -> Qt.InputMethodHints
- FitDialog.inputMethodQuery()
QWidget.inputMethodQuery(Qt.InputMethodQuery) -> QVariant
- FitDialog.insertAction()
QWidget.insertAction(QAction, QAction)
- FitDialog.insertActions()
QWidget.insertActions(QAction, list-of-QAction)
- FitDialog.installEventFilter()
QObject.installEventFilter(QObject)
- FitDialog.isActiveWindow()
QWidget.isActiveWindow() -> bool
- FitDialog.isAncestorOf()
QWidget.isAncestorOf(QWidget) -> bool
- FitDialog.isEnabled()
QWidget.isEnabled() -> bool
- FitDialog.isEnabledTo()
QWidget.isEnabledTo(QWidget) -> bool
- FitDialog.isEnabledToTLW()
QWidget.isEnabledToTLW() -> bool
- FitDialog.isFullScreen()
QWidget.isFullScreen() -> bool
- FitDialog.isHidden()
QWidget.isHidden() -> bool
- FitDialog.isLeftToRight()
QWidget.isLeftToRight() -> bool
- FitDialog.isMaximized()
QWidget.isMaximized() -> bool
- FitDialog.isMinimized()
QWidget.isMinimized() -> bool
- FitDialog.isModal()
QWidget.isModal() -> bool
- FitDialog.isRightToLeft()
QWidget.isRightToLeft() -> bool
- FitDialog.isSizeGripEnabled()
QDialog.isSizeGripEnabled() -> bool
- FitDialog.isTopLevel()
QWidget.isTopLevel() -> bool
- FitDialog.isVisible()
QWidget.isVisible() -> bool
- FitDialog.isVisibleTo()
QWidget.isVisibleTo(QWidget) -> bool
- FitDialog.isWidgetType()
QObject.isWidgetType() -> bool
- FitDialog.isWindow()
QWidget.isWindow() -> bool
- FitDialog.isWindowModified()
QWidget.isWindowModified() -> bool
- FitDialog.keyPressEvent()
QDialog.keyPressEvent(QKeyEvent)
- FitDialog.keyboardGrabber()
QWidget.keyboardGrabber() -> QWidget
- FitDialog.killTimer()
QObject.killTimer(int)
- FitDialog.layout()
QWidget.layout() -> QLayout
- FitDialog.layoutDirection()
QWidget.layoutDirection() -> Qt.LayoutDirection
- FitDialog.locale()
QWidget.locale() -> QLocale
- FitDialog.logicalDpiX()
QPaintDevice.logicalDpiX() -> int
- FitDialog.logicalDpiY()
QPaintDevice.logicalDpiY() -> int
- FitDialog.lower()
QWidget.lower()
- FitDialog.mapFrom()
QWidget.mapFrom(QWidget, QPoint) -> QPoint
- FitDialog.mapFromGlobal()
QWidget.mapFromGlobal(QPoint) -> QPoint
- FitDialog.mapFromParent()
QWidget.mapFromParent(QPoint) -> QPoint
- FitDialog.mapTo()
QWidget.mapTo(QWidget, QPoint) -> QPoint
- FitDialog.mapToGlobal()
QWidget.mapToGlobal(QPoint) -> QPoint
- FitDialog.mapToParent()
QWidget.mapToParent(QPoint) -> QPoint
- FitDialog.mask()
QWidget.mask() -> QRegion
- FitDialog.maximumHeight()
QWidget.maximumHeight() -> int
- FitDialog.maximumSize()
QWidget.maximumSize() -> QSize
- FitDialog.maximumWidth()
QWidget.maximumWidth() -> int
- FitDialog.metaObject()
QObject.metaObject() -> QMetaObject
- FitDialog.minimumHeight()
QWidget.minimumHeight() -> int
- FitDialog.minimumSize()
QWidget.minimumSize() -> QSize
- FitDialog.minimumSizeHint()
QDialog.minimumSizeHint() -> QSize
- FitDialog.minimumWidth()
QWidget.minimumWidth() -> int
- FitDialog.mouseGrabber()
QWidget.mouseGrabber() -> QWidget
- FitDialog.move()
QWidget.move(QPoint) QWidget.move(int, int)
- FitDialog.moveToThread()
QObject.moveToThread(QThread)
- FitDialog.nativeParentWidget()
QWidget.nativeParentWidget() -> QWidget
- FitDialog.nextInFocusChain()
QWidget.nextInFocusChain() -> QWidget
- FitDialog.normalGeometry()
QWidget.normalGeometry() -> QRect
- FitDialog.numColors()
QPaintDevice.numColors() -> int
- FitDialog.objectName()
QObject.objectName() -> QString
- FitDialog.open()
QDialog.open()
- FitDialog.orientation()
QDialog.orientation() -> Qt.Orientation
- FitDialog.overrideWindowFlags()
QWidget.overrideWindowFlags(Qt.WindowFlags)
- FitDialog.overrideWindowState()
QWidget.overrideWindowState(Qt.WindowStates)
- FitDialog.paintEngine()
QWidget.paintEngine() -> QPaintEngine
- FitDialog.paintingActive()
QPaintDevice.paintingActive() -> bool
- FitDialog.palette()
QWidget.palette() -> QPalette
- FitDialog.parent()
QObject.parent() -> QObject
- FitDialog.parentWidget()
QWidget.parentWidget() -> QWidget
- FitDialog.physicalDpiX()
QPaintDevice.physicalDpiX() -> int
- FitDialog.physicalDpiY()
QPaintDevice.physicalDpiY() -> int
- FitDialog.pos()
QWidget.pos() -> QPoint
- FitDialog.previousInFocusChain()
QWidget.previousInFocusChain() -> QWidget
- FitDialog.property()
QObject.property(str) -> QVariant
- FitDialog.pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- FitDialog.raise_()
QWidget.raise_()
- FitDialog.rect()
QWidget.rect() -> QRect
- FitDialog.refresh(slider_value=None)
Refresh Fit Tool dialog box
- FitDialog.register_all_curve_tools()
Register standard, curve-related and other tools
See also
methods
guiqwt.plot.PlotManager.add_tool() guiqwt.plot.PlotManager.register_standard_tools() guiqwt.plot.PlotManager.register_other_tools() guiqwt.plot.PlotManager.register_curve_tools() guiqwt.plot.PlotManager.register_image_tools() guiqwt.plot.PlotManager.register_all_image_tools()
- FitDialog.register_all_image_tools()
Register standard, image-related and other tools
See also
methods
guiqwt.plot.PlotManager.add_tool() guiqwt.plot.PlotManager.register_standard_tools() guiqwt.plot.PlotManager.register_other_tools() guiqwt.plot.PlotManager.register_curve_tools() guiqwt.plot.PlotManager.register_image_tools() guiqwt.plot.PlotManager.register_all_curve_tools()
- FitDialog.register_curve_tools()
Register only curve-related tools
See also
methods
guiqwt.plot.PlotManager.add_tool() guiqwt.plot.PlotManager.register_standard_tools() guiqwt.plot.PlotManager.register_other_tools() guiqwt.plot.PlotManager.register_image_tools()
- FitDialog.register_image_tools()
Register only image-related tools
See also
methods
guiqwt.plot.PlotManager.add_tool() guiqwt.plot.PlotManager.register_standard_tools() guiqwt.plot.PlotManager.register_other_tools() guiqwt.plot.PlotManager.register_curve_tools()
- FitDialog.register_other_tools()
Register other common tools
See also
methods
guiqwt.plot.PlotManager.add_tool() guiqwt.plot.PlotManager.register_standard_tools() guiqwt.plot.PlotManager.register_curve_tools() guiqwt.plot.PlotManager.register_image_tools()
- FitDialog.register_standard_tools()
Registering basic tools for standard plot dialog –> top of the context-menu
- FitDialog.register_tools()
Register the plotting dialog box tools: the base implementation provides standard, curve-related and other tools - i.e. calling this method is exactly the same as calling guiqwt.plot.CurveDialog.register_all_curve_tools()
This method may be overriden to provide a fully customized set of tools
- FitDialog.reject()
QDialog.reject()
- FitDialog.rejected
QDialog.rejected[] [signal]
- FitDialog.releaseKeyboard()
QWidget.releaseKeyboard()
- FitDialog.releaseMouse()
QWidget.releaseMouse()
- FitDialog.releaseShortcut()
QWidget.releaseShortcut(int)
- FitDialog.removeAction()
QWidget.removeAction(QAction)
- FitDialog.removeEventFilter()
QObject.removeEventFilter(QObject)
- FitDialog.render()
QWidget.render(QPaintDevice, QPoint targetOffset=QPoint(), QRegion sourceRegion=QRegion(), QWidget.RenderFlags flags=QWidget.DrawWindowBackground|QWidget.DrawChildren) QWidget.render(QPainter, QPoint targetOffset=QPoint(), QRegion sourceRegion=QRegion(), QWidget.RenderFlags flags=QWidget.DrawWindowBackground|QWidget.DrawChildren)
- FitDialog.repaint()
QWidget.repaint() QWidget.repaint(int, int, int, int) QWidget.repaint(QRect) QWidget.repaint(QRegion)
- FitDialog.resize()
QWidget.resize(QSize) QWidget.resize(int, int)
- FitDialog.resizeEvent()
QDialog.resizeEvent(QResizeEvent)
- FitDialog.restoreGeometry()
QWidget.restoreGeometry(QByteArray) -> bool
- FitDialog.result()
QDialog.result() -> int
- FitDialog.saveGeometry()
QWidget.saveGeometry() -> QByteArray
- FitDialog.scroll()
QWidget.scroll(int, int) QWidget.scroll(int, int, QRect)
- FitDialog.setAcceptDrops()
QWidget.setAcceptDrops(bool)
- FitDialog.setAttribute()
QWidget.setAttribute(Qt.WidgetAttribute, bool on=True)
- FitDialog.setAutoFillBackground()
QWidget.setAutoFillBackground(bool)
- FitDialog.setBackgroundRole()
QWidget.setBackgroundRole(QPalette.ColorRole)
- FitDialog.setBaseSize()
QWidget.setBaseSize(int, int) QWidget.setBaseSize(QSize)
- FitDialog.setContentsMargins()
QWidget.setContentsMargins(int, int, int, int) QWidget.setContentsMargins(QMargins)
- FitDialog.setContextMenuPolicy()
QWidget.setContextMenuPolicy(Qt.ContextMenuPolicy)
- FitDialog.setCursor()
QWidget.setCursor(QCursor)
- FitDialog.setDisabled()
QWidget.setDisabled(bool)
- FitDialog.setEnabled()
QWidget.setEnabled(bool)
- FitDialog.setExtension()
QDialog.setExtension(QWidget)
- FitDialog.setFixedHeight()
QWidget.setFixedHeight(int)
- FitDialog.setFixedSize()
QWidget.setFixedSize(QSize) QWidget.setFixedSize(int, int)
- FitDialog.setFixedWidth()
QWidget.setFixedWidth(int)
- FitDialog.setFocus()
QWidget.setFocus() QWidget.setFocus(Qt.FocusReason)
- FitDialog.setFocusPolicy()
QWidget.setFocusPolicy(Qt.FocusPolicy)
- FitDialog.setFocusProxy()
QWidget.setFocusProxy(QWidget)
- FitDialog.setFont()
QWidget.setFont(QFont)
- FitDialog.setForegroundRole()
QWidget.setForegroundRole(QPalette.ColorRole)
- FitDialog.setGeometry()
QWidget.setGeometry(QRect) QWidget.setGeometry(int, int, int, int)
- FitDialog.setGraphicsEffect()
QWidget.setGraphicsEffect(QGraphicsEffect)
- FitDialog.setHidden()
QWidget.setHidden(bool)
- FitDialog.setInputContext()
QWidget.setInputContext(QInputContext)
- FitDialog.setInputMethodHints()
QWidget.setInputMethodHints(Qt.InputMethodHints)
- FitDialog.setLayout()
QWidget.setLayout(QLayout)
- FitDialog.setLayoutDirection()
QWidget.setLayoutDirection(Qt.LayoutDirection)
- FitDialog.setLocale()
QWidget.setLocale(QLocale)
- FitDialog.setMask()
QWidget.setMask(QBitmap) QWidget.setMask(QRegion)
- FitDialog.setMaximumHeight()
QWidget.setMaximumHeight(int)
- FitDialog.setMaximumSize()
QWidget.setMaximumSize(int, int) QWidget.setMaximumSize(QSize)
- FitDialog.setMaximumWidth()
QWidget.setMaximumWidth(int)
- FitDialog.setMinimumHeight()
QWidget.setMinimumHeight(int)
- FitDialog.setMinimumSize()
QWidget.setMinimumSize(int, int) QWidget.setMinimumSize(QSize)
- FitDialog.setMinimumWidth()
QWidget.setMinimumWidth(int)
- FitDialog.setModal()
QDialog.setModal(bool)
- FitDialog.setMouseTracking()
QWidget.setMouseTracking(bool)
- FitDialog.setObjectName()
QObject.setObjectName(QString)
- FitDialog.setOrientation()
QDialog.setOrientation(Qt.Orientation)
- FitDialog.setPalette()
QWidget.setPalette(QPalette)
- FitDialog.setParent()
QWidget.setParent(QWidget) QWidget.setParent(QWidget, Qt.WindowFlags)
- FitDialog.setProperty()
QObject.setProperty(str, QVariant) -> bool
- FitDialog.setResult()
QDialog.setResult(int)
- FitDialog.setShortcutAutoRepeat()
QWidget.setShortcutAutoRepeat(int, bool enabled=True)
- FitDialog.setShortcutEnabled()
QWidget.setShortcutEnabled(int, bool enabled=True)
- FitDialog.setShown()
QWidget.setShown(bool)
- FitDialog.setSizeGripEnabled()
QDialog.setSizeGripEnabled(bool)
- FitDialog.setSizeIncrement()
QWidget.setSizeIncrement(int, int) QWidget.setSizeIncrement(QSize)
- FitDialog.setSizePolicy()
QWidget.setSizePolicy(QSizePolicy) QWidget.setSizePolicy(QSizePolicy.Policy, QSizePolicy.Policy)
- FitDialog.setStatusTip()
QWidget.setStatusTip(QString)
- FitDialog.setStyle()
QWidget.setStyle(QStyle)
- FitDialog.setStyleSheet()
QWidget.setStyleSheet(QString)
- FitDialog.setTabOrder()
QWidget.setTabOrder(QWidget, QWidget)
- FitDialog.setToolTip()
QWidget.setToolTip(QString)
- FitDialog.setUpdatesEnabled()
QWidget.setUpdatesEnabled(bool)
- FitDialog.setVisible()
QDialog.setVisible(bool)
- FitDialog.setWhatsThis()
QWidget.setWhatsThis(QString)
- FitDialog.setWindowFilePath()
QWidget.setWindowFilePath(QString)
- FitDialog.setWindowFlags()
QWidget.setWindowFlags(Qt.WindowFlags)
- FitDialog.setWindowIcon()
QWidget.setWindowIcon(QIcon)
- FitDialog.setWindowIconText()
QWidget.setWindowIconText(QString)
- FitDialog.setWindowModality()
QWidget.setWindowModality(Qt.WindowModality)
- FitDialog.setWindowModified()
QWidget.setWindowModified(bool)
- FitDialog.setWindowOpacity()
QWidget.setWindowOpacity(float)
- FitDialog.setWindowRole()
QWidget.setWindowRole(QString)
- FitDialog.setWindowState()
QWidget.setWindowState(Qt.WindowStates)
- FitDialog.setWindowTitle()
QWidget.setWindowTitle(QString)
- FitDialog.set_active_tool(tool=None)
Set active tool (if tool argument is None, the active tool will be the default tool)
- FitDialog.set_contrast_range(zmin, zmax)
Convenience function to set the contrast adjustment panel range
This is strictly equivalent to the following:
# Here, *widget* is for example a CurveWidget instance # (the same apply for CurvePlot, ImageWidget, ImagePlot or any # class deriving from PlotManager) widget.get_contrast_panel().set_range(zmin, zmax)
- FitDialog.set_default_plot(plot)
Set default plot
The default plot is the plot on which tools and panels will act.
- FitDialog.set_default_tool(tool)
Set default tool
- FitDialog.set_default_toolbar(toolbar)
Set default toolbar
- FitDialog.show()
QWidget.show()
- FitDialog.showEvent()
QDialog.showEvent(QShowEvent)
- FitDialog.showExtension()
QDialog.showExtension(bool)
- FitDialog.showFullScreen()
QWidget.showFullScreen()
- FitDialog.showMaximized()
QWidget.showMaximized()
- FitDialog.showMinimized()
QWidget.showMinimized()
- FitDialog.showNormal()
QWidget.showNormal()
- FitDialog.signalsBlocked()
QObject.signalsBlocked() -> bool
- FitDialog.size()
QWidget.size() -> QSize
- FitDialog.sizeHint()
QDialog.sizeHint() -> QSize
- FitDialog.sizeIncrement()
QWidget.sizeIncrement() -> QSize
- FitDialog.sizePolicy()
QWidget.sizePolicy() -> QSizePolicy
- FitDialog.stackUnder()
QWidget.stackUnder(QWidget)
- FitDialog.startTimer()
QObject.startTimer(int) -> int
- FitDialog.statusTip()
QWidget.statusTip() -> QString
- FitDialog.style()
QWidget.style() -> QStyle
- FitDialog.styleSheet()
QWidget.styleSheet() -> QString
- FitDialog.testAttribute()
QWidget.testAttribute(Qt.WidgetAttribute) -> bool
- FitDialog.thread()
QObject.thread() -> QThread
- FitDialog.toolTip()
QWidget.toolTip() -> QString
- FitDialog.topLevelWidget()
QWidget.topLevelWidget() -> QWidget
- FitDialog.tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- FitDialog.trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- FitDialog.underMouse()
QWidget.underMouse() -> bool
- FitDialog.ungrabGesture()
QWidget.ungrabGesture(Qt.GestureType)
- FitDialog.unsetCursor()
QWidget.unsetCursor()
- FitDialog.unsetLayoutDirection()
QWidget.unsetLayoutDirection()
- FitDialog.unsetLocale()
QWidget.unsetLocale()
- FitDialog.update()
QWidget.update() QWidget.update(QRect) QWidget.update(QRegion) QWidget.update(int, int, int, int)
- FitDialog.updateGeometry()
QWidget.updateGeometry()
- FitDialog.update_cross_sections()
Convenience function to update the cross section panels at once
This is strictly equivalent to the following:
# Here, *widget* is for example a CurveWidget instance # (the same apply for CurvePlot, ImageWidget, ImagePlot or any # class deriving from PlotManager) widget.get_xcs_panel().update_plot() widget.get_ycs_panel().update_plot()
- FitDialog.update_tools_status(plot=None)
Update tools for current plot
- FitDialog.updatesEnabled()
QWidget.updatesEnabled() -> bool
- FitDialog.visibleRegion()
QWidget.visibleRegion() -> QRegion
- FitDialog.whatsThis()
QWidget.whatsThis() -> QString
- FitDialog.width()
QWidget.width() -> int
- FitDialog.widthMM()
QPaintDevice.widthMM() -> int
- FitDialog.winId()
QWidget.winId() -> sip.voidptr
- FitDialog.window()
QWidget.window() -> QWidget
- FitDialog.windowFilePath()
QWidget.windowFilePath() -> QString
- FitDialog.windowFlags()
QWidget.windowFlags() -> Qt.WindowFlags
- FitDialog.windowIcon()
QWidget.windowIcon() -> QIcon
- FitDialog.windowIconText()
QWidget.windowIconText() -> QString
- FitDialog.windowModality()
QWidget.windowModality() -> Qt.WindowModality
- FitDialog.windowOpacity()
QWidget.windowOpacity() -> float
- FitDialog.windowRole()
QWidget.windowRole() -> QString
- FitDialog.windowState()
QWidget.windowState() -> Qt.WindowStates
- FitDialog.windowTitle()
QWidget.windowTitle() -> QString
- FitDialog.windowType()
QWidget.windowType() -> Qt.WindowType
- FitDialog.x()
QWidget.x() -> int
- FitDialog.y()
QWidget.y() -> int
- class guiqwt.widgets.fit.FitParam(name, value, min, max, logscale=False, steps=5000, format='%.3f', size_offset=0, unit='')[source]
- copy()[source]
Return a copy of this fitparam
- class guiqwt.widgets.fit.AutoFitParam(title=None, comment=None, icon='')[source]
- accept(vis)
helper function that passes the visitor to the accept methods of all the items in this dataset
- check()
Check the dataset item values
- edit(parent=None, apply=None, size=None)
- Open a dialog box to edit data set
- parent: parent widget (default is None, meaning no parent)
- apply: apply callback (default is None)
- size: dialog size (QSize object or integer tuple (width, height))
- err_norm
- Construct a string data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- ftol
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- get_comment()
Return data set comment
- get_icon()
Return data set icon
- get_title()
Return data set title
- gtol
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- method
- Construct a data item for a list of choices.
- label [string]: name
- choices [list, tuple or function]: string list or (key, label) list function of two arguments (item, value) returning a list of tuples (key, label, image) where image is an icon path, a QIcon instance or a function of one argument (key) returning a QIcon instance
- default [-]: default label or default key (optional)
- help [string]: text shown in tooltip (optional)
- norm
- Construct a string data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- set_defaults()
Set default values
- text_edit()
Edit data set with text input only
- to_string(debug=False, indent=None, align=False)
Return readable string representation of the data set If debug is True, add more details on data items
- view(parent=None, size=None)
- Open a dialog box to view data set
- parent: parent widget (default is None, meaning no parent)
- size: dialog size (QSize object or integer tuple (width, height))
- xmax
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- xmin
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- xtol
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
guiqwt.plot
- The plot module provides the following features:
- guiqwt.plot.PlotManager: the plot manager is an object to link plots, panels and tools together for designing highly versatile graphical user interfaces
- guiqwt.plot.CurveWidget: a ready-to-use widget for curve displaying with an integrated and preconfigured plot manager providing the item list panel and curve-related tools
- guiqwt.plot.CurveDialog: a ready-to-use dialog box for curve displaying with an integrated and preconfigured plot manager providing the item list panel and curve-related tools
- guiqwt.plot.ImageWidget: a ready-to-use widget for curve and image displaying with an integrated and preconfigured plot manager providing the item list panel, the contrast adjustment panel, the cross section panels (along X and Y axes) and image-related tools (e.g. colormap selection tool)
- guiqwt.plot.ImageDialog: a ready-to-use dialog box for curve and image displaying with an integrated and preconfigured plot manager providing the item list panel, the contrast adjustment panel, the cross section panels (along X and Y axes) and image-related tools (e.g. colormap selection tool)
See also
- Module guiqwt.curve
- Module providing curve-related plot items and plotting widgets
- Module guiqwt.image
- Module providing image-related plot items and plotting widgets
- Module guiqwt.tools
- Module providing the plot tools
- Module guiqwt.panels
- Module providing the plot panels IDs
- Module guiqwt.signals
- Module providing all the end-user Qt SIGNAL objects defined in guiqwt
- Module guiqwt.baseplot
- Module providing the guiqwt plotting widget base class
Class diagrams
Curve-related widgets with integrated plot manager:
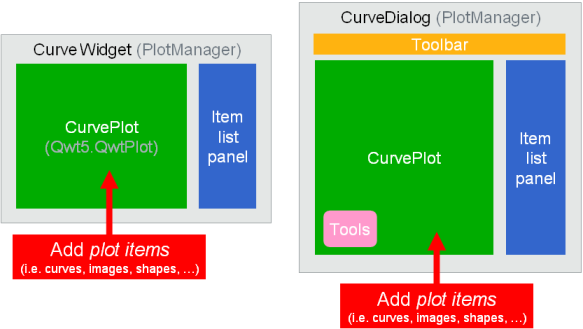
Image-related widgets with integrated plot manager:
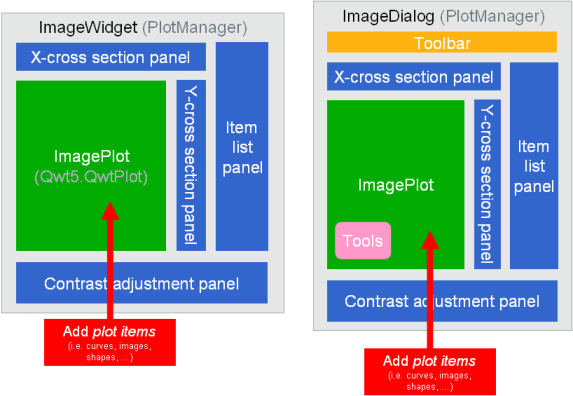
Building your own plot manager:
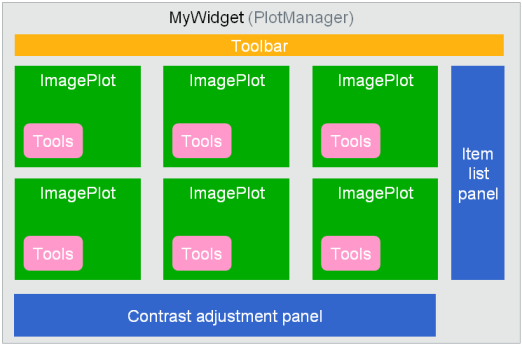
Examples
Simple example without the plot manager:
from guidata.qt.QtGui import QWidget, QVBoxLayout, QHBoxLayout, QPushButton
from guidata.qt.QtCore import SIGNAL
#---Import plot widget base class
from guiqwt.plot import CurveWidget
from guiqwt.builder import make
from guidata.configtools import get_icon
#---
class FilterTestWidget(QWidget):
"""
Filter testing widget
parent: parent widget (QWidget)
x, y: NumPy arrays
func: function object (the signal filter to be tested)
"""
def __init__(self, parent, x, y, func):
QWidget.__init__(self, parent)
self.setMinimumSize(320, 200)
self.x = x
self.y = y
self.func = func
#---guiqwt curve item attribute:
self.curve_item = None
#---
def setup_widget(self, title):
#---Create the plot widget:
curvewidget = CurveWidget(self)
curvewidget.register_all_curve_tools()
self.curve_item = make.curve([], [], color='b')
curvewidget.plot.add_item(self.curve_item)
curvewidget.plot.set_antialiasing(True)
#---
button = QPushButton(u"Test filter: %s" % title)
self.connect(button, SIGNAL('clicked()'), self.process_data)
vlayout = QVBoxLayout()
vlayout.addWidget(curvewidget)
vlayout.addWidget(button)
self.setLayout(vlayout)
self.update_curve()
def process_data(self):
self.y = self.func(self.y)
self.update_curve()
def update_curve(self):
#---Update curve
self.curve_item.set_data(self.x, self.y)
self.curve_item.plot().replot()
#---
class TestWindow(QWidget):
def __init__(self):
QWidget.__init__(self)
self.setWindowTitle("Signal filtering (guiqwt)")
self.setWindowIcon(get_icon('guiqwt.svg'))
hlayout = QHBoxLayout()
self.setLayout(hlayout)
def add_plot(self, x, y, func, title):
widget = FilterTestWidget(self, x, y, func)
widget.setup_widget(title)
self.layout().addWidget(widget)
def test():
"""Testing this simple Qt/guiqwt example"""
from guidata.qt.QtGui import QApplication
import numpy as np
import scipy.signal as sps, scipy.ndimage as spi
app = QApplication([])
win = TestWindow()
x = np.linspace(-10, 10, 500)
y = np.random.rand(len(x))+5*np.sin(2*x**2)/x
win.add_plot(x, y, lambda x: spi.gaussian_filter1d(x, 1.), "Gaussian")
win.add_plot(x, y, sps.wiener, "Wiener")
win.show()
app.exec_()
if __name__ == '__main__':
test()
Simple example with the plot manager: even if this simple example does not justify the use of the plot manager (this is an unnecessary complication here), it shows how to use it. In more complex applications, using the plot manager allows to design highly versatile graphical user interfaces.
from guidata.qt.QtGui import (QWidget, QVBoxLayout, QHBoxLayout, QPushButton,
QMainWindow)
from guidata.qt.QtCore import SIGNAL
#---Import plot widget base class
from guiqwt.curve import CurvePlot
from guiqwt.plot import PlotManager
from guiqwt.builder import make
from guidata.configtools import get_icon
#---
class FilterTestWidget(QWidget):
"""
Filter testing widget
parent: parent widget (QWidget)
x, y: NumPy arrays
func: function object (the signal filter to be tested)
"""
def __init__(self, parent, x, y, func):
QWidget.__init__(self, parent)
self.setMinimumSize(320, 200)
self.x = x
self.y = y
self.func = func
#---guiqwt related attributes:
self.plot = None
self.curve_item = None
#---
def setup_widget(self, title):
#---Create the plot widget:
self.plot = CurvePlot(self)
self.curve_item = make.curve([], [], color='b')
self.plot.add_item(self.curve_item)
self.plot.set_antialiasing(True)
#---
button = QPushButton(u"Test filter: %s" % title)
self.connect(button, SIGNAL('clicked()'), self.process_data)
vlayout = QVBoxLayout()
vlayout.addWidget(self.plot)
vlayout.addWidget(button)
self.setLayout(vlayout)
self.update_curve()
def process_data(self):
self.y = self.func(self.y)
self.update_curve()
def update_curve(self):
#---Update curve
self.curve_item.set_data(self.x, self.y)
self.plot.replot()
#---
class TestWindow(QMainWindow):
def __init__(self):
QMainWindow.__init__(self)
self.setWindowTitle("Signal filtering 2 (guiqwt)")
self.setWindowIcon(get_icon('guiqwt.png'))
hlayout = QHBoxLayout()
central_widget = QWidget(self)
central_widget.setLayout(hlayout)
self.setCentralWidget(central_widget)
#---guiqwt plot manager
self.manager = PlotManager(self)
#---
def add_plot(self, x, y, func, title):
widget = FilterTestWidget(self, x, y, func)
widget.setup_widget(title)
self.centralWidget().layout().addWidget(widget)
#---Register plot to manager
self.manager.add_plot(widget.plot)
#---
def setup_window(self):
#---Add toolbar and register manager tools
toolbar = self.addToolBar("tools")
self.manager.add_toolbar(toolbar, id(toolbar))
self.manager.register_all_curve_tools()
#---
def test():
"""Testing this simple Qt/guiqwt example"""
from guidata.qt.QtGui import QApplication
import numpy as np
import scipy.signal as sps, scipy.ndimage as spi
app = QApplication([])
win = TestWindow()
x = np.linspace(-10, 10, 500)
y = np.random.rand(len(x))+5*np.sin(2*x**2)/x
win.add_plot(x, y, lambda x: spi.gaussian_filter1d(x, 1.), "Gaussian")
win.add_plot(x, y, sps.wiener, "Wiener")
#---Setup window
win.setup_window()
#---
win.show()
app.exec_()
if __name__ == '__main__':
test()
Reference
- class guiqwt.plot.PlotManager(main)[source]
Construct a PlotManager object, a ‘controller’ that organizes relations between plots (i.e. guiqwt.curve.CurvePlot or guiqwt.image.ImagePlot objects), panels, tools (see guiqwt.tools) and toolbars
- activate_default_tool()[source]
Activate default tool
- add_panel(panel)[source]
Register a panel to the plot manager
- Plot manager’s registration sequence is the following:
- add plots
- add panels
- add tools
- add_plot(plot, plot_id=<class 'guiqwt.plot.DefaultPlotID'>)[source]
- Register a plot to the plot manager:
- plot: guiqwt.curve.CurvePlot or guiqwt.image.ImagePlot object
- plot_id (default id is the plot object’s id: id(plot)): unique ID identifying the plot (any Python object), this ID will be asked by the manager to access this plot later.
- Plot manager’s registration sequence is the following:
- add plots
- add panels
- add tools
- add_separator_tool(toolbar_id=None)[source]
Register a separator tool to the plot manager: the separator tool is just a tool which insert a separator in the plot context menu
- add_tool(ToolKlass, *args, **kwargs)[source]
- Register a tool to the manager
- ToolKlass: tool’s class (guiqwt builtin tools are defined in module guiqwt.tools)
- *args: arguments sent to the tool’s class
- **kwargs: keyword arguments sent to the tool’s class
- Plot manager’s registration sequence is the following:
- add plots
- add panels
- add tools
- add_toolbar(toolbar, toolbar_id='default')[source]
- Add toolbar to the plot manager
- toolbar: a QToolBar object toolbar_id: toolbar’s id (default id is string “default”)
- configure_panels()[source]
Call all the registred panels ‘configure_panel’ methods to finalize the object construction (this allows to use tools registered to the same plot manager as the panel itself with breaking the registration sequence: “add plots, then panels, then tools”)
- get_active_plot()[source]
Return the active plot
The active plot is the plot whose canvas has the focus otherwise it’s the “default” plot
- get_active_tool()[source]
Return active tool
Return widget context menu – built using active tools
- get_contrast_panel()[source]
Convenience function to get the contrast adjustment panel
Return None if the contrast adjustment panel has not been added to this manager
- get_default_plot()[source]
Return default plot
The default plot is the plot on which tools and panels will act.
- get_default_tool()[source]
Get default tool
- get_default_toolbar()[source]
Return default toolbar
- get_itemlist_panel()[source]
Convenience function to get the item list panel
Return None if the item list panel has not been added to this manager
- get_main()[source]
Return the main (parent) widget
Note that for py:class:guiqwt.plot.CurveWidget or guiqwt.plot.ImageWidget objects, this method will return the widget itself because the plot manager is integrated to it.
- get_panel(panel_id)[source]
Return panel from its ID Panel IDs are listed in module guiqwt.panels
- get_plot(plot_id=<class 'guiqwt.plot.DefaultPlotID'>)[source]
Return plot associated to plot_id (if method is called without specifying the plot_id parameter, return the default plot)
- get_plots()[source]
Return all registered plots
- get_tool(ToolKlass)[source]
Return tool instance from its class
- get_toolbar(toolbar_id='default')[source]
- Return toolbar from its ID
- toolbar_id: toolbar’s id (default id is string “default”)
- get_xcs_panel()[source]
Convenience function to get the X-axis cross section panel
Return None if the X-axis cross section panel has not been added to this manager
- get_ycs_panel()[source]
Convenience function to get the Y-axis cross section panel
Return None if the Y-axis cross section panel has not been added to this manager
- register_all_curve_tools()[source]
Register standard, curve-related and other tools
See also
methods
guiqwt.plot.PlotManager.add_tool() guiqwt.plot.PlotManager.register_standard_tools() guiqwt.plot.PlotManager.register_other_tools() guiqwt.plot.PlotManager.register_curve_tools() guiqwt.plot.PlotManager.register_image_tools() guiqwt.plot.PlotManager.register_all_image_tools()
- register_all_image_tools()[source]
Register standard, image-related and other tools
See also
methods
guiqwt.plot.PlotManager.add_tool() guiqwt.plot.PlotManager.register_standard_tools() guiqwt.plot.PlotManager.register_other_tools() guiqwt.plot.PlotManager.register_curve_tools() guiqwt.plot.PlotManager.register_image_tools() guiqwt.plot.PlotManager.register_all_curve_tools()
- register_curve_tools()[source]
Register only curve-related tools
See also
methods
guiqwt.plot.PlotManager.add_tool() guiqwt.plot.PlotManager.register_standard_tools() guiqwt.plot.PlotManager.register_other_tools() guiqwt.plot.PlotManager.register_image_tools()
- register_image_tools()[source]
Register only image-related tools
See also
methods
guiqwt.plot.PlotManager.add_tool() guiqwt.plot.PlotManager.register_standard_tools() guiqwt.plot.PlotManager.register_other_tools() guiqwt.plot.PlotManager.register_curve_tools()
- register_other_tools()[source]
Register other common tools
See also
methods
guiqwt.plot.PlotManager.add_tool() guiqwt.plot.PlotManager.register_standard_tools() guiqwt.plot.PlotManager.register_curve_tools() guiqwt.plot.PlotManager.register_image_tools()
- register_standard_tools()[source]
Registering basic tools for standard plot dialog –> top of the context-menu
- set_active_tool(tool=None)[source]
Set active tool (if tool argument is None, the active tool will be the default tool)
- set_contrast_range(zmin, zmax)[source]
Convenience function to set the contrast adjustment panel range
This is strictly equivalent to the following:
# Here, *widget* is for example a CurveWidget instance # (the same apply for CurvePlot, ImageWidget, ImagePlot or any # class deriving from PlotManager) widget.get_contrast_panel().set_range(zmin, zmax)
- set_default_plot(plot)[source]
Set default plot
The default plot is the plot on which tools and panels will act.
- set_default_tool(tool)[source]
Set default tool
- set_default_toolbar(toolbar)[source]
Set default toolbar
- update_cross_sections()[source]
Convenience function to update the cross section panels at once
This is strictly equivalent to the following:
# Here, *widget* is for example a CurveWidget instance # (the same apply for CurvePlot, ImageWidget, ImagePlot or any # class deriving from PlotManager) widget.get_xcs_panel().update_plot() widget.get_ycs_panel().update_plot()
- update_tools_status(plot=None)[source]
Update tools for current plot
- class guiqwt.plot.CurveWidget(parent=None, title=None, xlabel=None, ylabel=None, xunit=None, yunit=None, section='plot', show_itemlist=False, gridparam=None, panels=None)[source]
Construct a CurveWidget object: plotting widget with integrated plot manager
- parent: parent widget
- title: plot title
- xlabel: (bottom axis title, top axis title) or bottom axis title only
- ylabel: (left axis title, right axis title) or left axis title only
- xunit: (bottom axis unit, top axis unit) or bottom axis unit only
- yunit: (left axis unit, right axis unit) or left axis unit only
- panels (optional): additionnal panels (list, tuple)
- class guiqwt.plot.CurveDialog(wintitle='guiqwt plot', icon='guiqwt.svg', edit=False, toolbar=False, options=None, parent=None, panels=None)[source]
Construct a CurveDialog object: plotting dialog box with integrated plot manager
- wintitle: window title
- icon: window icon
- edit: editable state
- toolbar: show/hide toolbar
- options: options sent to the guiqwt.curve.CurvePlot object (dictionary)
- parent: parent widget
- panels (optional): additionnal panels (list, tuple)
Install standard buttons (OK, Cancel) in dialog button box layout (guiqwt.plot.CurveDialog.button_layout)
This method may be overriden to customize the button box
- class guiqwt.plot.ImageWidget(parent=None, title='', xlabel=('', ''), ylabel=('', ''), zlabel=None, xunit=('', ''), yunit=('', ''), zunit=None, yreverse=True, colormap='jet', aspect_ratio=1.0, lock_aspect_ratio=True, show_contrast=False, show_itemlist=False, show_xsection=False, show_ysection=False, xsection_pos='top', ysection_pos='right', gridparam=None, panels=None)[source]
Construct a ImageWidget object: plotting widget with integrated plot manager
- parent: parent widget
- title: plot title (string)
- xlabel, ylabel, zlabel: resp. bottom, left and right axis titles (strings)
- xunit, yunit, zunit: resp. bottom, left and right axis units (strings)
- yreverse: reversing Y-axis (bool)
- aspect_ratio: height to width ratio (float)
- lock_aspect_ratio: locking aspect ratio (bool)
- show_contrast: showing contrast adjustment tool (bool)
- show_xsection: showing x-axis cross section plot (bool)
- show_ysection: showing y-axis cross section plot (bool)
- xsection_pos: x-axis cross section plot position (string: “top”, “bottom”)
- ysection_pos: y-axis cross section plot position (string: “left”, “right”)
- panels (optional): additionnal panels (list, tuple)
- class guiqwt.plot.ImageDialog(wintitle='guiqwt plot', icon='guiqwt.svg', edit=False, toolbar=False, options=None, parent=None, panels=None)[source]
Construct a ImageDialog object: plotting dialog box with integrated plot manager
- wintitle: window title
- icon: window icon
- edit: editable state
- toolbar: show/hide toolbar
- options: options sent to the guiqwt.image.ImagePlot object (dictionary)
- parent: parent widget
- panels (optional): additionnal panels (list, tuple)
guiqwt.builder
The builder module provides a builder singleton class used to simplify the creation of plot items.
Example
Before creating any widget, a QApplication must be instantiated (that is a Qt internal requirement):
>>> import guidata
>>> app = guidata.qapplication()
that is mostly equivalent to the following (the only difference is that the guidata helper function also installs the Qt translation corresponding to the system locale):
>>> from PyQt4.QtGui import QApplication
>>> app = QApplication([])
now that a QApplication object exists, we may create the plotting widget:
>>> from guiqwt.plot import ImageWidget
>>> widget = ImageWidget()
create curves, images, histograms, etc. and attach them to the plot:
>>> from guiqwt.builder import make
>>> curve = make.mcure(x, y, 'r+')
>>> image = make.image(data)
>>> hist = make.histogram(data, 100)
>>> for item in (curve, image, hist):
... widget.plot.add_item()
and then show the widget to screen:
>>> widget.show()
>>> app.exec_()
Reference
- class guiqwt.builder.PlotItemBuilder[source]
This is just a bare class used to regroup a set of factory functions in a single object
- annotated_circle(x0, y0, x1, y1, ratio, title=None, subtitle=None)[source]
Make an annotated circle plot item (guiqwt.annotations.AnnotatedCircle object)
- x0, y0, x1, y1: circle diameter coordinates
- title, subtitle: strings
- annotated_ellipse(x0, y0, x1, y1, ratio, title=None, subtitle=None)[source]
Make an annotated ellipse plot item (guiqwt.annotations.AnnotatedEllipse object)
- x0, y0, x1, y1: ellipse rectangle coordinates
- ratio: ratio between y-axis and x-axis lengths
- title, subtitle: strings
- annotated_rectangle(x0, y0, x1, y1, title=None, subtitle=None)[source]
Make an annotated rectangle plot item (guiqwt.annotations.AnnotatedRectangle object)
- x0, y0, x1, y1: rectangle coordinates
- title, subtitle: strings
- annotated_segment(x0, y0, x1, y1, title=None, subtitle=None)[source]
Make an annotated segment plot item (guiqwt.annotations.AnnotatedSegment object)
- x0, y0, x1, y1: segment coordinates
- title, subtitle: strings
- circle(x0, y0, x1, y1, title=None)[source]
Make a circle shape plot item (guiqwt.shapes.EllipseShape object)
- x0, y0, x1, y1: circle diameter coordinates
- title: label name (optional)
- computation(range, anchor, label, curve, function, title=None)[source]
Make a computation label plot item (guiqwt.label.DataInfoLabel object) (see example: guiqwt.tests.computations)
- computation2d(rect, anchor, label, image, function, title=None)[source]
Make a 2D computation label plot item (guiqwt.label.RangeComputation2d object) (see example: guiqwt.tests.computations)
- computations(range, anchor, specs, title=None)[source]
Make computation labels plot item (guiqwt.label.DataInfoLabel object) (see example: guiqwt.tests.computations)
- computations2d(rect, anchor, specs, title=None)[source]
Make 2D computation labels plot item (guiqwt.label.RangeComputation2d object) (see example: guiqwt.tests.computations)
- static compute_bounds(data, pixel_size, center_on)[source]
Return image bounds from pixel_size (scalar or tuple)
- curve(x, y, title=u'', color=None, linestyle=None, linewidth=None, marker=None, markersize=None, markerfacecolor=None, markeredgecolor=None, shade=None, fitted=None, curvestyle=None, curvetype=None, baseline=None, xaxis='bottom', yaxis='left')[source]
Make a curve plot item from x, y, data (guiqwt.curve.CurveItem object)
- x: 1D NumPy array
- y: 1D NumPy array
- color: curve color name
- linestyle: curve line style (MATLAB-like string or attribute name from the PyQt4.QtCore.Qt.PenStyle enum (i.e. “SolidLine” “DashLine”, “DotLine”, “DashDotLine”, “DashDotDotLine” or “NoPen”)
- linewidth: line width (pixels)
- marker: marker shape (MATLAB-like string or attribute name from the PyQt4.Qwt5.QwtSymbol.Style enum (i.e. “Cross”, “Ellipse”, “Star1”, “XCross”, “Rect”, “Diamond”, “UTriangle”, “DTriangle”, “RTriangle”, “LTriangle”, “Star2” or “NoSymbol”)
- markersize: marker size (pixels)
- markerfacecolor: marker face color name
- markeredgecolor: marker edge color name
- shade: 0 <= float <= 1 (curve shade)
- fitted: boolean (fit curve to data)
- curvestyle: attribute name from the PyQt4.Qwt5.QwtPlotCurve.CurveStyle enum (i.e. “Lines”, “Sticks”, “Steps”, “Dots” or “NoCurve”)
- curvetype: attribute name from the PyQt4.Qwt5.QwtPlotCurve.CurveType enum (i.e. “Yfx” or “Xfy”)
- baseline (float: default=0.0): the baseline is needed for filling the curve with a brush or the Sticks drawing style. The interpretation of the baseline depends on the curve type (horizontal line for “Yfx”, vertical line for “Xfy”)
- xaxis, yaxis: X/Y axes bound to curve
Examples: curve(x, y, marker=’Ellipse’, markerfacecolor=’#ffffff’) which is equivalent to (MATLAB-style support): curve(x, y, marker=’o’, markerfacecolor=’w’)
- ellipse(x0, y0, x1, y1, title=None)[source]
Make an ellipse shape plot item (guiqwt.shapes.EllipseShape object)
- x0, y0, x1, y1: ellipse x-axis coordinates
- title: label name (optional)
- error(x, y, dx, dy, title=u'', color=None, linestyle=None, linewidth=None, errorbarwidth=None, errorbarcap=None, errorbarmode=None, errorbaralpha=None, marker=None, markersize=None, markerfacecolor=None, markeredgecolor=None, shade=None, fitted=None, curvestyle=None, curvetype=None, baseline=None, xaxis='bottom', yaxis='left')[source]
Make an errorbar curve plot item (guiqwt.curve.ErrorBarCurveItem object)
- x: 1D NumPy array
- y: 1D NumPy array
- dx: None, or scalar, or 1D NumPy array
- dy: None, or scalar, or 1D NumPy array
- color: curve color name
- linestyle: curve line style (MATLAB-like string or attribute name from the PyQt4.QtCore.Qt.PenStyle enum (i.e. “SolidLine” “DashLine”, “DotLine”, “DashDotLine”, “DashDotDotLine” or “NoPen”)
- linewidth: line width (pixels)
- marker: marker shape (MATLAB-like string or attribute name from the PyQt4.Qwt5.QwtSymbol.Style enum (i.e. “Cross”, “Ellipse”, “Star1”, “XCross”, “Rect”, “Diamond”, “UTriangle”, “DTriangle”, “RTriangle”, “LTriangle”, “Star2” or “NoSymbol”)
- markersize: marker size (pixels)
- markerfacecolor: marker face color name
- markeredgecolor: marker edge color name
- shade: 0 <= float <= 1 (curve shade)
- fitted: boolean (fit curve to data)
- curvestyle: attribute name from the PyQt4.Qwt5.QwtPlotCurve.CurveStyle enum (i.e. “Lines”, “Sticks”, “Steps”, “Dots” or “NoCurve”)
- curvetype: attribute name from the PyQt4.Qwt5.QwtPlotCurve.CurveType enum (i.e. “Yfx” or “Xfy”)
- baseline (float: default=0.0): the baseline is needed for filling the curve with a brush or the Sticks drawing style. The interpretation of the baseline depends on the curve type (horizontal line for “Yfx”, vertical line for “Xfy”)
- xaxis, yaxis: X/Y axes bound to curve
- Examples::
- error(x, y, None, dy, marker=’Ellipse’, markerfacecolor=’#ffffff’) which is equivalent to (MATLAB-style support): error(x, y, None, dy, marker=’o’, markerfacecolor=’w’)
- grid(background=None, major_enabled=None, minor_enabled=None, major_style=None, minor_style=None)[source]
- Make a grid plot item (guiqwt.curve.GridItem object)
- background = canvas background color
- major_enabled = tuple (major_xenabled, major_yenabled)
- minor_enabled = tuple (minor_xenabled, minor_yenabled)
- major_style = tuple (major_xstyle, major_ystyle)
- minor_style = tuple (minor_xstyle, minor_ystyle)
Style: tuple (style, color, width)
- gridparam(background=None, major_enabled=None, minor_enabled=None, major_style=None, minor_style=None)[source]
- Make guiqwt.styles.GridParam instance
- background = canvas background color
- major_enabled = tuple (major_xenabled, major_yenabled)
- minor_enabled = tuple (minor_xenabled, minor_yenabled)
- major_style = tuple (major_xstyle, major_ystyle)
- minor_style = tuple (minor_xstyle, minor_ystyle)
Style: tuple (style, color, width)
- hcursor(y, label=None, constraint_cb=None, movable=True, readonly=False)[source]
Make an horizontal cursor plot item
Convenient function to make an horizontal marker (guiqwt.shapes.Marker object)
- histogram(data, bins=None, logscale=None, title=u'', color=None, xaxis='bottom', yaxis='left')[source]
Make 1D Histogram plot item (guiqwt.histogram.HistogramItem object)
- data (1D NumPy array)
- bins: number of bins (int)
- logscale: Y-axis scale (bool)
- histogram2D(X, Y, NX=None, NY=None, logscale=None, title=None, transparent=None, Z=None, computation=-1, interpolation=0)[source]
Make a 2D Histogram plot item (guiqwt.image.Histogram2DItem object)
- X: data (1D array)
- Y: data (1D array)
- NX: Number of bins along x-axis (int)
- NY: Number of bins along y-axis (int)
- logscale: Z-axis scale (bool)
- title: item title (string)
- transparent: enable transparency (bool)
- image(data=None, filename=None, title=None, alpha_mask=None, alpha=None, background_color=None, colormap=None, xdata=[None, None], ydata=[None, None], pixel_size=None, center_on=None, interpolation='linear', eliminate_outliers=None, xformat='%.1f', yformat='%.1f', zformat='%.1f')[source]
Make an image plot item from data (guiqwt.image.ImageItem object or guiqwt.image.RGBImageItem object if data has 3 dimensions)
- imagefilter(xmin, xmax, ymin, ymax, imageitem, filter, title=None)[source]
Make a rectangular area image filter plot item (guiqwt.image.ImageFilterItem object)
- xmin, xmax, ymin, ymax: filter area bounds
- imageitem: An imageitem instance
- filter: function (x, y, data) –> data
- info_label(anchor, comps, title=None)[source]
Make an info label plot item (guiqwt.label.DataInfoLabel object)
- label(text, g, c, anchor, title='')[source]
Make a label plot item (guiqwt.label.LabelItem object)
- text: label text (string)
- g: position in plot coordinates (tuple) or relative position (string)
- c: position in canvas coordinates (tuple)
- anchor: anchor position in relative position (string)
- title: label name (optional)
- Examples::
- make.label(“Relative position”, (x[0], y[0]), (10, 10), “BR”) make.label(“Absolute position”, “R”, (0,0), “R”)
- legend(anchor='TR', c=None, restrict_items=None)[source]
Make a legend plot item (guiqwt.label.LegendBoxItem or guiqwt.label.SelectedLegendBoxItem object)
anchor: legend position in relative position (string)
c (optional): position in canvas coordinates (tuple)
- restrict_items (optional):
- None: all items are shown in legend box
- []: no item shown
- [item1, item2]: item1, item2 are shown in legend box
- marker(position=None, label_cb=None, constraint_cb=None, movable=True, readonly=False, markerstyle=None, markerspacing=None, color=None, linestyle=None, linewidth=None, marker=None, markersize=None, markerfacecolor=None, markeredgecolor=None)[source]
Make a marker plot item (guiqwt.shapes.Marker object)
- position: tuple (x, y)
- label_cb: function with two arguments (x, y) returning a string
- constraint_cb: function with two arguments (x, y) returning a tuple (x, y) according to the marker constraint
- movable: if True (default), marker will be movable
- readonly: if False (default), marker can be deleted
- markerstyle: ‘+’, ‘-‘, ‘|’ or None
- markerspacing: spacing between text and marker line
- color: marker color name
- linestyle: marker line style (MATLAB-like string or attribute name from the PyQt4.QtCore.Qt.PenStyle enum (i.e. “SolidLine” “DashLine”, “DotLine”, “DashDotLine”, “DashDotDotLine” or “NoPen”)
- linewidth: line width (pixels)
- marker: marker shape (MATLAB-like string or attribute name from the PyQt4.Qwt5.QwtSymbol.Style enum (i.e. “Cross”, “Ellipse”, “Star1”, “XCross”, “Rect”, “Diamond”, “UTriangle”, “DTriangle”, “RTriangle”, “LTriangle”, “Star2” or “NoSymbol”)
- markersize: marker size (pixels)
- markerfacecolor: marker face color name
- markeredgecolor: marker edge color name
- maskedimage(data=None, mask=None, filename=None, title=None, alpha_mask=False, alpha=1.0, xdata=[None, None], ydata=[None, None], pixel_size=None, center_on=None, background_color=None, colormap=None, show_mask=False, fill_value=None, interpolation='linear', eliminate_outliers=None, xformat='%.1f', yformat='%.1f', zformat='%.1f')[source]
Make a masked image plot item from data (guiqwt.image.MaskedImageItem object)
- mcurve(*args, **kwargs)[source]
Make a curve plot item based on MATLAB-like syntax (may returns a list of curves if data contains more than one signal) (guiqwt.curve.CurveItem object)
Example: mcurve(x, y, ‘r+’)
- merror(*args, **kwargs)[source]
Make an errorbar curve plot item based on MATLAB-like syntax (guiqwt.curve.ErrorBarCurveItem object)
Example: mcurve(x, y, ‘r+’)
- pcolor(*args, **kwargs)[source]
Make a pseudocolor plot item of a 2D array based on MATLAB-like syntax (guiqwt.image.QuadGridItem object)
- Examples:
- pcolor(C) pcolor(X, Y, C)
- pcurve(x, y, param, xaxis='bottom', yaxis='left')[source]
Make a curve plot item based on a guiqwt.styles.CurveParam instance (guiqwt.curve.CurveItem object)
Usage: pcurve(x, y, param)
- perror(x, y, dx, dy, curveparam, errorbarparam, xaxis='bottom', yaxis='left')[source]
Make an errorbar curve plot item based on a guiqwt.styles.ErrorBarParam instance (guiqwt.curve.ErrorBarCurveItem object)
- x: 1D NumPy array
- y: 1D NumPy array
- dx: None, or scalar, or 1D NumPy array
- dy: None, or scalar, or 1D NumPy array
- curveparam: guiqwt.styles.CurveParam object
- errorbarparam: guiqwt.styles.ErrorBarParam object
- xaxis, yaxis: X/Y axes bound to curve
Usage: perror(x, y, dx, dy, curveparam, errorbarparam)
- phistogram(data, curveparam, histparam, xaxis='bottom', yaxis='left')[source]
Make 1D histogram plot item (guiqwt.histogram.HistogramItem object) based on a guiqwt.styles.CurveParam and guiqwt.styles.HistogramParam instances
Usage: phistogram(data, curveparam, histparam)
- quadgrid(X, Y, Z, filename=None, title=None, alpha_mask=None, alpha=None, background_color=None, colormap=None, interpolation='linear')[source]
Make a pseudocolor plot item of a 2D array (guiqwt.image.QuadGridItem object)
- range_info_label(range, anchor, label, function=None, title=None)[source]
Make an info label plot item showing an XRangeSelection object infos
Default function is lambda x, dx: (x, dx).
x = linspace(-10, 10, 10) y = sin(sin(sin(x))) range = make.range(-2, 2) disp = make.range_info_label(range, ‘BL’, u”x = %.1f ± %.1f cm”,
lambda x, dx: (x, dx))(guiqwt.label.DataInfoLabel object) (see example: guiqwt.tests.computations)
- rectangle(x0, y0, x1, y1, title=None)[source]
Make a rectangle shape plot item (guiqwt.shapes.RectangleShape object)
- x0, y0, x1, y1: rectangle coordinates
- title: label name (optional)
- rgbimage(data=None, filename=None, title=None, alpha_mask=False, alpha=1.0, xdata=[None, None], ydata=[None, None], pixel_size=None, center_on=None, interpolation='linear')[source]
Make a RGB image plot item from data (guiqwt.image.RGBImageItem object)
- segment(x0, y0, x1, y1, title=None)[source]
Make a segment shape plot item (guiqwt.shapes.SegmentShape object)
- x0, y0, x1, y1: segment coordinates
- title: label name (optional)
- trimage(data=None, filename=None, title=None, alpha_mask=None, alpha=None, background_color=None, colormap=None, x0=0.0, y0=0.0, angle=0.0, dx=1.0, dy=1.0, interpolation='linear', eliminate_outliers=None, xformat='%.1f', yformat='%.1f', zformat='%.1f')[source]
Make a transformable image plot item (image with an arbitrary affine transform) (guiqwt.image.TrImageItem object)
- data: 2D NumPy array (image pixel data)
- filename: image filename (if data is not specified)
- title: image title (optional)
- x0, y0: position
- angle: angle (radians)
- dx, dy: pixel size along X and Y axes
- interpolation: ‘nearest’, ‘linear’ (default), ‘antialiasing’ (5x5)
- vcursor(x, label=None, constraint_cb=None, movable=True, readonly=False)[source]
Make a vertical cursor plot item
Convenient function to make a vertical marker (guiqwt.shapes.Marker object)
- xcursor(x, y, label=None, constraint_cb=None, movable=True, readonly=False)[source]
Make an cross cursor plot item
Convenient function to make an cross marker (guiqwt.shapes.Marker object)
- xyimage(x, y, data, title=None, alpha_mask=None, alpha=None, background_color=None, colormap=None, interpolation='linear', eliminate_outliers=None, xformat='%.1f', yformat='%.1f', zformat='%.1f')[source]
Make an xyimage plot item (image with non-linear X/Y axes) from data (guiqwt.image.XYImageItem object)
- x: 1D NumPy array
- y: 1D NumPy array
- data: 2D NumPy array (image pixel data)
- title: image title (optional)
- interpolation: ‘nearest’, ‘linear’ (default), ‘antialiasing’ (5x5)
guiqwt.panels
The panels module provides guiqwt.curve.PanelWidget (the panel widget class from which all panels must derived) and identifiers for each kind of panel:
- guiqwt.panels.ID_ITEMLIST: ID of the item list panel
- guiqwt.panels.ID_CONTRAST: ID of the contrast adjustment panel
- guiqwt.panels.ID_XCS: ID of the X-axis cross section panel
- guiqwt.panels.ID_YCS: ID of the Y-axis cross section panel
See also
- Module guiqwt.plot
- Module providing ready-to-use curve and image plotting widgets and dialog boxes
- Module guiqwt.curve
- Module providing curve-related plot items and plotting widgets
- Module guiqwt.image
- Module providing image-related plot items and plotting widgets
- Module guiqwt.tools
- Module providing the plot tools
guiqwt.signals
The signals module contains constants defining the custom Qt SIGNAL objects used by guiqwt: the signals definition are gathered here to avoid mispelling signals at connect and emit sites.
- Signals available:
- guiqwt.signals.SIG_ITEM_MOVED
Emitted by plot when an IBasePlotItem-like object was moved from (x0, y0) to (x1, y1)
Arguments: item object, x0, y0, x1, y1
- guiqwt.signals.SIG_MARKER_CHANGED
Emitted by plot when a guiqwt.shapes.Marker position changes
Arguments: guiqwt.shapes.Marker object
- guiqwt.signals.SIG_AXES_CHANGED
Emitted by plot when a guiqwt.shapes.Axes position (or angle) changes
Arguments: guiqwt.shapes.Axes object
- guiqwt.signals.SIG_ANNOTATION_CHANGED
Emitted by plot when an annotations.AnnotatedShape position changes
Arguments: annotation item
- guiqwt.signals.SIG_RANGE_CHANGED
Emitted by plot when a shapes.XRangeSelection range changes
Arguments: range object, lower_bound, upper_bound
- guiqwt.signals.SIG_ITEMS_CHANGED
Emitted by plot when item list has changed (item removed, added, ...)
Arguments: plot
- guiqwt.signals.SIG_ACTIVE_ITEM_CHANGED
Emitted by plot when selected item has changed
Arguments: plot
- guiqwt.signals.SIG_ITEM_REMOVED
Emitted by plot when an item was deleted from the itemlist or using the delete item tool
Arguments: removed item
- guiqwt.signals.SIG_ITEM_SELECTION_CHANGED
Emitted by plot when an item is selected
Arguments: plot
- guiqwt.signals.SIG_PLOT_LABELS_CHANGED
Emitted (by plot) when plot’s title or any axis label has changed
Arguments: plot
- guiqwt.signals.SIG_AXIS_DIRECTION_CHANGED
Emitted (by plot) when any plot axis direction has changed
Arguments: plot
- guiqwt.signals.SIG_VOI_CHANGED
- Emitted by “contrast” panel’s histogram when the lut range of some items changed (for now, this signal is for guiqwt.histogram module’s internal use only - the ‘public’ counterpart of this signal is SIG_LUT_CHANGED, see below)
- guiqwt.signals.SIG_LUT_CHANGED
Emitted by plot when LUT has been changed by the user
Arguments: plot
- guiqwt.signals.SIG_MASK_CHANGED
Emitted by plot when image mask has changed
Arguments: MaskedImageItem object
- guiqwt.signals.SIG_VISIBILITY_CHANGED
Emitted for example by panels when their visibility has changed
Arguments: state (boolean)
- guiqwt.signals.SIG_VALIDATE_TOOL
Emitted by an interactive tool to notify that the tool has just been “validated”, i.e. <ENTER>, <RETURN> or <SPACE> was pressed
Arguments: filter
guiqwt.baseplot
The baseplot module provides the guiqwt plotting widget base class: guiqwt.baseplot.BasePlot. This is an enhanced version of PyQwt‘s QwtPlot plotting widget which supports the following features:
- add to plot, del from plot, hide/show and save/restore plot items easily
- item selection and multiple selection
- active item
- plot parameters editing
See also
- Module guiqwt.curve
- Module providing curve-related plot items and plotting widgets
- Module guiqwt.image
- Module providing image-related plot items and plotting widgets
- Module guiqwt.plot
- Module providing ready-to-use curve and image plotting widgets and dialog boxes
Reference
- class guiqwt.baseplot.BasePlot(parent=None, section='plot')[source]
An enhanced QwtPlot class that provides methods for handling plotitems and axes better
It distinguishes activatable items from basic QwtPlotItems.
Activatable items must support IBasePlotItem interface and should be added to the plot using add_item methods.
Signals: SIG_ITEMS_CHANGED, SIG_ACTIVE_ITEM_CHANGED
- class RenderFlags
QWidget.RenderFlags(QWidget.RenderFlags) QWidget.RenderFlags(int) QWidget.RenderFlags()
- BasePlot.acceptDrops()
QWidget.acceptDrops() -> bool
- BasePlot.actions()
QWidget.actions() -> list-of-QAction
- BasePlot.activateWindow()
QWidget.activateWindow()
- BasePlot.addAction()
QWidget.addAction(QAction)
- BasePlot.addActions()
QWidget.addActions(list-of-QAction)
- BasePlot.add_item(item, z=None)[source]
Add a plot item instance to this plot widget
- item: QwtPlotItem (PyQt4.Qwt5) object implementing
- the IBasePlotItem interface (guiqwt.interfaces)
- BasePlot.add_item_with_z_offset(item, zoffset)[source]
Add a plot item instance within a specified z range, over zmin
- BasePlot.adjustSize()
QWidget.adjustSize()
- BasePlot.autoFillBackground()
QWidget.autoFillBackground() -> bool
- BasePlot.backgroundRole()
QWidget.backgroundRole() -> QPalette.ColorRole
- BasePlot.baseSize()
QWidget.baseSize() -> QSize
- BasePlot.blockSignals()
QObject.blockSignals(bool) -> bool
- BasePlot.childAt()
QWidget.childAt(QPoint) -> QWidget QWidget.childAt(int, int) -> QWidget
- BasePlot.children()
QObject.children() -> list-of-QObject
- BasePlot.childrenRect()
QWidget.childrenRect() -> QRect
- BasePlot.childrenRegion()
QWidget.childrenRegion() -> QRegion
- BasePlot.clearFocus()
QWidget.clearFocus()
- BasePlot.clearMask()
QWidget.clearMask()
- BasePlot.close()
QWidget.close() -> bool
- BasePlot.colorCount()
QPaintDevice.colorCount() -> int
- BasePlot.connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- BasePlot.contentsMargins()
QWidget.contentsMargins() -> QMargins
- BasePlot.contentsRect()
QWidget.contentsRect() -> QRect
- BasePlot.contextMenuPolicy()
QWidget.contextMenuPolicy() -> Qt.ContextMenuPolicy
- BasePlot.copy_to_clipboard()[source]
Copy widget’s window to clipboard
- BasePlot.cursor()
QWidget.cursor() -> QCursor
- BasePlot.customContextMenuRequested
QWidget.customContextMenuRequested[QPoint] [signal]
- BasePlot.del_all_items()[source]
Remove (detach) all attached items
- BasePlot.del_item(item)[source]
Remove item from widget Convenience function (see ‘del_items’)
- BasePlot.del_items(items)[source]
Remove item from widget
- BasePlot.deleteLater()
QObject.deleteLater()
- BasePlot.depth()
QPaintDevice.depth() -> int
- BasePlot.deserialize(reader)[source]
- Restore items from HDF5 file:
- reader: guidata.hdf5io.HDF5Reader object
See also guiqwt.baseplot.BasePlot.save_items_to_hdf5()
- BasePlot.destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- BasePlot.devType()
QWidget.devType() -> int
- BasePlot.disable_autoscale()[source]
Re-apply the axis scales so as to disable autoscaling without changing the view
- BasePlot.disable_unused_axes()[source]
Disable unused axes
- BasePlot.disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- BasePlot.do_autoscale(replot=True)[source]
Do autoscale on all axes
- BasePlot.dumpObjectInfo()
QObject.dumpObjectInfo()
- BasePlot.dumpObjectTree()
QObject.dumpObjectTree()
- BasePlot.dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- BasePlot.edit_axis_parameters(axis_id)[source]
Edit axis parameters
- BasePlot.edit_plot_parameters(key)[source]
Edit plot parameters
- BasePlot.effectiveWinId()
QWidget.effectiveWinId() -> sip.voidptr
- BasePlot.emit()
QObject.emit(SIGNAL(), ...)
- BasePlot.enable_used_axes()[source]
Enable only used axes For now, this is needed only by the pyplot interface
- BasePlot.ensurePolished()
QWidget.ensurePolished()
- BasePlot.eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- BasePlot.find()
QWidget.find(sip.voidptr) -> QWidget
- BasePlot.findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- BasePlot.findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- BasePlot.focusPolicy()
QWidget.focusPolicy() -> Qt.FocusPolicy
- BasePlot.focusProxy()
QWidget.focusProxy() -> QWidget
- BasePlot.focusWidget()
QWidget.focusWidget() -> QWidget
- BasePlot.font()
QWidget.font() -> QFont
- BasePlot.fontInfo()
QWidget.fontInfo() -> QFontInfo
- BasePlot.fontMetrics()
QWidget.fontMetrics() -> QFontMetrics
- BasePlot.foregroundRole()
QWidget.foregroundRole() -> QPalette.ColorRole
- BasePlot.frameGeometry()
QWidget.frameGeometry() -> QRect
- BasePlot.frameRect()
QFrame.frameRect() -> QRect
- BasePlot.frameShadow()
QFrame.frameShadow() -> QFrame.Shadow
- BasePlot.frameShape()
QFrame.frameShape() -> QFrame.Shape
- BasePlot.frameSize()
QWidget.frameSize() -> QSize
- BasePlot.frameStyle()
QFrame.frameStyle() -> int
- BasePlot.frameWidth()
QFrame.frameWidth() -> int
- BasePlot.geometry()
QWidget.geometry() -> QRect
- BasePlot.getContentsMargins()
QWidget.getContentsMargins() -> (int, int, int, int)
- BasePlot.get_active_axes()[source]
Return active axes
- BasePlot.get_active_item(force=False)[source]
Return active item Force item activation if there is no active item
- BasePlot.get_axesparam_class(item)[source]
Return AxesParam dataset class associated to item’s type
- BasePlot.get_axis_color(axis_id)[source]
Get axis color (color name, i.e. string)
- BasePlot.get_axis_font(axis_id)[source]
Get axis font
- BasePlot.get_axis_id(axis_name)[source]
Return axis ID from axis name If axis ID is passed directly, check the ID
- BasePlot.get_axis_limits(axis_id)[source]
Return axis limits (minimum and maximum values)
- BasePlot.get_axis_scale(axis_id)[source]
Return the name (‘lin’ or ‘log’) of the scale used by axis
- BasePlot.get_axis_title(axis_id)[source]
Get axis title
- BasePlot.get_axis_unit(axis_id)[source]
Get axis unit
Return widget context menu
- BasePlot.get_items(z_sorted=False, item_type=None)[source]
Return widget’s item list (items are based on IBasePlotItem’s interface)
- BasePlot.get_last_active_item(item_type)[source]
Return last active item corresponding to passed item_type
- BasePlot.get_max_z()[source]
Return maximum z-order for all items registered in plot If there is no item, return 0
- BasePlot.get_nearest_object(pos, close_dist=0)[source]
Return nearest item from position ‘pos’ If close_dist > 0: return the first found item (higher z) which
distance to ‘pos’ is less than close_distelse: return the closest item
- BasePlot.get_nearest_object_in_z(pos)[source]
Return nearest item for which position ‘pos’ is inside of it (iterate over items with respect to their ‘z’ coordinate)
- BasePlot.get_plot_parameters(key, itemparams)[source]
Return a list of DataSets for a given parameter key the datasets will be edited and passed back to set_plot_parameters
this is a generic interface to help building context menus using the BasePlotMenuTool
- BasePlot.get_private_items(z_sorted=False, item_type=None)[source]
Return widget’s private item list (items are based on IBasePlotItem’s interface)
- BasePlot.get_public_items(z_sorted=False, item_type=None)[source]
Return widget’s public item list (items are based on IBasePlotItem’s interface)
- BasePlot.get_scales()[source]
Return active curve scales
- BasePlot.get_selected_items(z_sorted=False, item_type=None)[source]
Return selected items
- BasePlot.get_title()[source]
Get plot title
- BasePlot.grabGesture()
QWidget.grabGesture(Qt.GestureType, Qt.GestureFlags flags=Qt.GestureFlags(0))
- BasePlot.grabKeyboard()
QWidget.grabKeyboard()
- BasePlot.grabMouse()
QWidget.grabMouse() QWidget.grabMouse(QCursor)
- BasePlot.grabShortcut()
QWidget.grabShortcut(QKeySequence, Qt.ShortcutContext context=Qt.WindowShortcut) -> int
- BasePlot.graphicsEffect()
QWidget.graphicsEffect() -> QGraphicsEffect
- BasePlot.graphicsProxyWidget()
QWidget.graphicsProxyWidget() -> QGraphicsProxyWidget
- BasePlot.hasFocus()
QWidget.hasFocus() -> bool
- BasePlot.hasMouseTracking()
QWidget.hasMouseTracking() -> bool
- BasePlot.height()
QWidget.height() -> int
- BasePlot.heightForWidth()
QWidget.heightForWidth(int) -> int
- BasePlot.heightMM()
QPaintDevice.heightMM() -> int
- BasePlot.hide()
QWidget.hide()
- BasePlot.hide_items(items=None, item_type=None)[source]
Hide items (if items is None, hide all items)
- BasePlot.inherits()
QObject.inherits(str) -> bool
- BasePlot.inputContext()
QWidget.inputContext() -> QInputContext
- BasePlot.inputMethodHints()
QWidget.inputMethodHints() -> Qt.InputMethodHints
- BasePlot.inputMethodQuery()
QWidget.inputMethodQuery(Qt.InputMethodQuery) -> QVariant
- BasePlot.insertAction()
QWidget.insertAction(QAction, QAction)
- BasePlot.insertActions()
QWidget.insertActions(QAction, list-of-QAction)
- BasePlot.installEventFilter()
QObject.installEventFilter(QObject)
- BasePlot.invalidate()[source]
Invalidate paint cache and schedule redraw use instead of replot when only the content of the canvas needs redrawing (axes, shouldn’t change)
- BasePlot.isActiveWindow()
QWidget.isActiveWindow() -> bool
- BasePlot.isAncestorOf()
QWidget.isAncestorOf(QWidget) -> bool
- BasePlot.isEnabled()
QWidget.isEnabled() -> bool
- BasePlot.isEnabledTo()
QWidget.isEnabledTo(QWidget) -> bool
- BasePlot.isEnabledToTLW()
QWidget.isEnabledToTLW() -> bool
- BasePlot.isFullScreen()
QWidget.isFullScreen() -> bool
- BasePlot.isHidden()
QWidget.isHidden() -> bool
- BasePlot.isLeftToRight()
QWidget.isLeftToRight() -> bool
- BasePlot.isMaximized()
QWidget.isMaximized() -> bool
- BasePlot.isMinimized()
QWidget.isMinimized() -> bool
- BasePlot.isModal()
QWidget.isModal() -> bool
- BasePlot.isRightToLeft()
QWidget.isRightToLeft() -> bool
- BasePlot.isTopLevel()
QWidget.isTopLevel() -> bool
- BasePlot.isVisible()
QWidget.isVisible() -> bool
- BasePlot.isVisibleTo()
QWidget.isVisibleTo(QWidget) -> bool
- BasePlot.isWidgetType()
QObject.isWidgetType() -> bool
- BasePlot.isWindow()
QWidget.isWindow() -> bool
- BasePlot.isWindowModified()
QWidget.isWindowModified() -> bool
- BasePlot.keyboardGrabber()
QWidget.keyboardGrabber() -> QWidget
- BasePlot.killTimer()
QObject.killTimer(int)
- BasePlot.layout()
QWidget.layout() -> QLayout
- BasePlot.layoutDirection()
QWidget.layoutDirection() -> Qt.LayoutDirection
- BasePlot.lineWidth()
QFrame.lineWidth() -> int
- BasePlot.locale()
QWidget.locale() -> QLocale
- BasePlot.logicalDpiX()
QPaintDevice.logicalDpiX() -> int
- BasePlot.logicalDpiY()
QPaintDevice.logicalDpiY() -> int
- BasePlot.lower()
QWidget.lower()
- BasePlot.mapFrom()
QWidget.mapFrom(QWidget, QPoint) -> QPoint
- BasePlot.mapFromGlobal()
QWidget.mapFromGlobal(QPoint) -> QPoint
- BasePlot.mapFromParent()
QWidget.mapFromParent(QPoint) -> QPoint
- BasePlot.mapTo()
QWidget.mapTo(QWidget, QPoint) -> QPoint
- BasePlot.mapToGlobal()
QWidget.mapToGlobal(QPoint) -> QPoint
- BasePlot.mapToParent()
QWidget.mapToParent(QPoint) -> QPoint
- BasePlot.mask()
QWidget.mask() -> QRegion
- BasePlot.maximumHeight()
QWidget.maximumHeight() -> int
- BasePlot.maximumSize()
QWidget.maximumSize() -> QSize
- BasePlot.maximumWidth()
QWidget.maximumWidth() -> int
- BasePlot.metaObject()
QObject.metaObject() -> QMetaObject
- BasePlot.midLineWidth()
QFrame.midLineWidth() -> int
- BasePlot.minimumHeight()
QWidget.minimumHeight() -> int
- BasePlot.minimumSize()
QWidget.minimumSize() -> QSize
- BasePlot.minimumWidth()
QWidget.minimumWidth() -> int
- BasePlot.mouseDoubleClickEvent(event)[source]
Reimplement QWidget method
- BasePlot.mouseGrabber()
QWidget.mouseGrabber() -> QWidget
- BasePlot.move()
QWidget.move(QPoint) QWidget.move(int, int)
- BasePlot.moveToThread()
QObject.moveToThread(QThread)
- BasePlot.move_down(item_list)[source]
Move item(s) down, i.e. to the background (swap item with the previous item in z-order)
item: plot item or list of plot items
Return True if items have been moved effectively
- BasePlot.move_up(item_list)[source]
Move item(s) up, i.e. to the foreground (swap item with the next item in z-order)
item: plot item or list of plot items
Return True if items have been moved effectively
- BasePlot.nativeParentWidget()
QWidget.nativeParentWidget() -> QWidget
- BasePlot.nextInFocusChain()
QWidget.nextInFocusChain() -> QWidget
- BasePlot.normalGeometry()
QWidget.normalGeometry() -> QRect
- BasePlot.numColors()
QPaintDevice.numColors() -> int
- BasePlot.objectName()
QObject.objectName() -> QString
- BasePlot.overrideWindowFlags()
QWidget.overrideWindowFlags(Qt.WindowFlags)
- BasePlot.overrideWindowState()
QWidget.overrideWindowState(Qt.WindowStates)
- BasePlot.paintEngine()
QWidget.paintEngine() -> QPaintEngine
- BasePlot.paintingActive()
QPaintDevice.paintingActive() -> bool
- BasePlot.palette()
QWidget.palette() -> QPalette
- BasePlot.parent()
QObject.parent() -> QObject
- BasePlot.parentWidget()
QWidget.parentWidget() -> QWidget
- BasePlot.physicalDpiX()
QPaintDevice.physicalDpiX() -> int
- BasePlot.physicalDpiY()
QPaintDevice.physicalDpiY() -> int
- BasePlot.pos()
QWidget.pos() -> QPoint
- BasePlot.previousInFocusChain()
QWidget.previousInFocusChain() -> QWidget
- BasePlot.property()
QObject.property(str) -> QVariant
- BasePlot.pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- BasePlot.raise_()
QWidget.raise_()
- BasePlot.read_axes_styles(section, options)[source]
Read axes styles from section and options (one option for each axis in the order left, right, bottom, top)
Skip axis if option is None
- BasePlot.rect()
QWidget.rect() -> QRect
- BasePlot.releaseKeyboard()
QWidget.releaseKeyboard()
- BasePlot.releaseMouse()
QWidget.releaseMouse()
- BasePlot.releaseShortcut()
QWidget.releaseShortcut(int)
- BasePlot.removeAction()
QWidget.removeAction(QAction)
- BasePlot.removeEventFilter()
QObject.removeEventFilter(QObject)
- BasePlot.render()
QWidget.render(QPaintDevice, QPoint targetOffset=QPoint(), QRegion sourceRegion=QRegion(), QWidget.RenderFlags flags=QWidget.DrawWindowBackground|QWidget.DrawChildren) QWidget.render(QPainter, QPoint targetOffset=QPoint(), QRegion sourceRegion=QRegion(), QWidget.RenderFlags flags=QWidget.DrawWindowBackground|QWidget.DrawChildren)
- BasePlot.repaint()
QWidget.repaint() QWidget.repaint(int, int, int, int) QWidget.repaint(QRect) QWidget.repaint(QRegion)
- BasePlot.resize()
QWidget.resize(QSize) QWidget.resize(int, int)
- BasePlot.restoreGeometry()
QWidget.restoreGeometry(QByteArray) -> bool
- BasePlot.restore_items(iofile)[source]
- Restore items from file using the pickle protocol
- iofile: file object or filename
- BasePlot.saveGeometry()
QWidget.saveGeometry() -> QByteArray
- BasePlot.save_items(iofile, selected=False)[source]
- Save (serializable) items to file using the pickle protocol
- iofile: file object or filename
- selected=False: if True, will save only selected items
- BasePlot.scroll()
QWidget.scroll(int, int) QWidget.scroll(int, int, QRect)
- BasePlot.select_all()[source]
Select all selectable items
- BasePlot.select_item(item)[source]
Select item
- BasePlot.select_some_items(items)[source]
Select items
- BasePlot.serialize(writer, selected=False)[source]
- Save (serializable) items to HDF5 file:
- writer: guidata.hdf5io.HDF5Writer object
- selected=False: if True, will save only selected items
See also guiqwt.baseplot.BasePlot.restore_items_from_hdf5()
- BasePlot.setAcceptDrops()
QWidget.setAcceptDrops(bool)
- BasePlot.setAttribute()
QWidget.setAttribute(Qt.WidgetAttribute, bool on=True)
- BasePlot.setAutoFillBackground()
QWidget.setAutoFillBackground(bool)
- BasePlot.setBackgroundRole()
QWidget.setBackgroundRole(QPalette.ColorRole)
- BasePlot.setBaseSize()
QWidget.setBaseSize(int, int) QWidget.setBaseSize(QSize)
- BasePlot.setContentsMargins()
QWidget.setContentsMargins(int, int, int, int) QWidget.setContentsMargins(QMargins)
- BasePlot.setContextMenuPolicy()
QWidget.setContextMenuPolicy(Qt.ContextMenuPolicy)
- BasePlot.setCursor()
QWidget.setCursor(QCursor)
- BasePlot.setDisabled()
QWidget.setDisabled(bool)
- BasePlot.setEnabled()
QWidget.setEnabled(bool)
- BasePlot.setFixedHeight()
QWidget.setFixedHeight(int)
- BasePlot.setFixedSize()
QWidget.setFixedSize(QSize) QWidget.setFixedSize(int, int)
- BasePlot.setFixedWidth()
QWidget.setFixedWidth(int)
- BasePlot.setFocus()
QWidget.setFocus() QWidget.setFocus(Qt.FocusReason)
- BasePlot.setFocusPolicy()
QWidget.setFocusPolicy(Qt.FocusPolicy)
- BasePlot.setFocusProxy()
QWidget.setFocusProxy(QWidget)
- BasePlot.setFont()
QWidget.setFont(QFont)
- BasePlot.setForegroundRole()
QWidget.setForegroundRole(QPalette.ColorRole)
- BasePlot.setFrameRect()
QFrame.setFrameRect(QRect)
- BasePlot.setFrameShadow()
QFrame.setFrameShadow(QFrame.Shadow)
- BasePlot.setFrameShape()
QFrame.setFrameShape(QFrame.Shape)
- BasePlot.setFrameStyle()
QFrame.setFrameStyle(int)
- BasePlot.setGeometry()
QWidget.setGeometry(QRect) QWidget.setGeometry(int, int, int, int)
- BasePlot.setGraphicsEffect()
QWidget.setGraphicsEffect(QGraphicsEffect)
- BasePlot.setHidden()
QWidget.setHidden(bool)
- BasePlot.setInputContext()
QWidget.setInputContext(QInputContext)
- BasePlot.setInputMethodHints()
QWidget.setInputMethodHints(Qt.InputMethodHints)
- BasePlot.setLayout()
QWidget.setLayout(QLayout)
- BasePlot.setLayoutDirection()
QWidget.setLayoutDirection(Qt.LayoutDirection)
- BasePlot.setLineWidth()
QFrame.setLineWidth(int)
- BasePlot.setLocale()
QWidget.setLocale(QLocale)
- BasePlot.setMask()
QWidget.setMask(QBitmap) QWidget.setMask(QRegion)
- BasePlot.setMaximumHeight()
QWidget.setMaximumHeight(int)
- BasePlot.setMaximumSize()
QWidget.setMaximumSize(int, int) QWidget.setMaximumSize(QSize)
- BasePlot.setMaximumWidth()
QWidget.setMaximumWidth(int)
- BasePlot.setMidLineWidth()
QFrame.setMidLineWidth(int)
- BasePlot.setMinimumHeight()
QWidget.setMinimumHeight(int)
- BasePlot.setMinimumSize()
QWidget.setMinimumSize(int, int) QWidget.setMinimumSize(QSize)
- BasePlot.setMinimumWidth()
QWidget.setMinimumWidth(int)
- BasePlot.setMouseTracking()
QWidget.setMouseTracking(bool)
- BasePlot.setObjectName()
QObject.setObjectName(QString)
- BasePlot.setPalette()
QWidget.setPalette(QPalette)
- BasePlot.setParent()
QWidget.setParent(QWidget) QWidget.setParent(QWidget, Qt.WindowFlags)
- BasePlot.setProperty()
QObject.setProperty(str, QVariant) -> bool
- BasePlot.setShortcutAutoRepeat()
QWidget.setShortcutAutoRepeat(int, bool enabled=True)
- BasePlot.setShortcutEnabled()
QWidget.setShortcutEnabled(int, bool enabled=True)
- BasePlot.setShown()
QWidget.setShown(bool)
- BasePlot.setSizeIncrement()
QWidget.setSizeIncrement(int, int) QWidget.setSizeIncrement(QSize)
- BasePlot.setSizePolicy()
QWidget.setSizePolicy(QSizePolicy) QWidget.setSizePolicy(QSizePolicy.Policy, QSizePolicy.Policy)
- BasePlot.setStatusTip()
QWidget.setStatusTip(QString)
- BasePlot.setStyle()
QWidget.setStyle(QStyle)
- BasePlot.setStyleSheet()
QWidget.setStyleSheet(QString)
- BasePlot.setTabOrder()
QWidget.setTabOrder(QWidget, QWidget)
- BasePlot.setToolTip()
QWidget.setToolTip(QString)
- BasePlot.setUpdatesEnabled()
QWidget.setUpdatesEnabled(bool)
- BasePlot.setVisible()
QWidget.setVisible(bool)
- BasePlot.setWhatsThis()
QWidget.setWhatsThis(QString)
- BasePlot.setWindowFilePath()
QWidget.setWindowFilePath(QString)
- BasePlot.setWindowFlags()
QWidget.setWindowFlags(Qt.WindowFlags)
- BasePlot.setWindowIcon()
QWidget.setWindowIcon(QIcon)
- BasePlot.setWindowIconText()
QWidget.setWindowIconText(QString)
- BasePlot.setWindowModality()
QWidget.setWindowModality(Qt.WindowModality)
- BasePlot.setWindowModified()
QWidget.setWindowModified(bool)
- BasePlot.setWindowOpacity()
QWidget.setWindowOpacity(float)
- BasePlot.setWindowRole()
QWidget.setWindowRole(QString)
- BasePlot.setWindowState()
QWidget.setWindowState(Qt.WindowStates)
- BasePlot.setWindowTitle()
QWidget.setWindowTitle(QString)
- BasePlot.set_active_item(item)[source]
Set active item, and unselect the old active item
- BasePlot.set_axis_color(axis_id, color)[source]
Set axis color color: color name (string) or QColor instance
- BasePlot.set_axis_font(axis_id, font)[source]
Set axis font
- BasePlot.set_axis_limits(axis_id, vmin, vmax, stepsize=0)[source]
Set axis limits (minimum and maximum values) and optional step size
- BasePlot.set_axis_scale(axis_id, scale, autoscale=True)[source]
Set axis scale Example: self.set_axis_scale(curve.yAxis(), ‘lin’)
- BasePlot.set_axis_ticks(axis_id, nmajor=None, nminor=None)[source]
Set axis maximum number of major ticks and maximum of minor ticks
- BasePlot.set_axis_title(axis_id, text)[source]
Set axis title
- BasePlot.set_axis_unit(axis_id, text)[source]
Set axis unit
- BasePlot.set_item_parameters(itemparams)[source]
Set item (plot, here) parameters
- BasePlot.set_item_visible(item, state, notify=True, replot=True)[source]
Show/hide item and emit a SIG_ITEMS_CHANGED signal
- BasePlot.set_items(*args)[source]
Utility function used to quickly setup a plot with a set of items
- BasePlot.set_items_readonly(state)[source]
Set all items readonly state to state Default item’s readonly state: False (items may be deleted)
- BasePlot.set_manager(manager, plot_id)[source]
Set the associated guiqwt.plot.PlotManager instance
- BasePlot.set_scales(xscale, yscale)[source]
Set active curve scales Example: self.set_scales(‘lin’, ‘lin’)
- BasePlot.set_title(title)[source]
Set plot title
- BasePlot.show()
QWidget.show()
- BasePlot.showEvent(event)[source]
Reimplement Qwt method
- BasePlot.showFullScreen()
QWidget.showFullScreen()
- BasePlot.showMaximized()
QWidget.showMaximized()
- BasePlot.showMinimized()
QWidget.showMinimized()
- BasePlot.showNormal()
QWidget.showNormal()
- BasePlot.show_items(items=None, item_type=None)[source]
Show items (if items is None, show all items)
- BasePlot.signalsBlocked()
QObject.signalsBlocked() -> bool
- BasePlot.size()
QWidget.size() -> QSize
- BasePlot.sizeHint()[source]
Preferred size
- BasePlot.sizeIncrement()
QWidget.sizeIncrement() -> QSize
- BasePlot.sizePolicy()
QWidget.sizePolicy() -> QSizePolicy
- BasePlot.stackUnder()
QWidget.stackUnder(QWidget)
- BasePlot.startTimer()
QObject.startTimer(int) -> int
- BasePlot.statusTip()
QWidget.statusTip() -> QString
- BasePlot.style()
QWidget.style() -> QStyle
- BasePlot.styleSheet()
QWidget.styleSheet() -> QString
- BasePlot.testAttribute()
QWidget.testAttribute(Qt.WidgetAttribute) -> bool
- BasePlot.thread()
QObject.thread() -> QThread
- BasePlot.toolTip()
QWidget.toolTip() -> QString
- BasePlot.topLevelWidget()
QWidget.topLevelWidget() -> QWidget
- BasePlot.tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- BasePlot.trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- BasePlot.underMouse()
QWidget.underMouse() -> bool
- BasePlot.ungrabGesture()
QWidget.ungrabGesture(Qt.GestureType)
- BasePlot.unselect_all()[source]
Unselect all selected items
- BasePlot.unselect_item(item)[source]
Unselect item
- BasePlot.unsetCursor()
QWidget.unsetCursor()
- BasePlot.unsetLayoutDirection()
QWidget.unsetLayoutDirection()
- BasePlot.unsetLocale()
QWidget.unsetLocale()
- BasePlot.update()
QWidget.update() QWidget.update(QRect) QWidget.update(QRegion) QWidget.update(int, int, int, int)
- BasePlot.updateGeometry()
QWidget.updateGeometry()
- BasePlot.update_all_axes_styles()[source]
Update all axes styles
- BasePlot.update_axis_style(axis_id)[source]
Update axis style
- BasePlot.updatesEnabled()
QWidget.updatesEnabled() -> bool
- BasePlot.visibleRegion()
QWidget.visibleRegion() -> QRegion
- BasePlot.whatsThis()
QWidget.whatsThis() -> QString
- BasePlot.width()
QWidget.width() -> int
- BasePlot.widthMM()
QPaintDevice.widthMM() -> int
- BasePlot.winId()
QWidget.winId() -> sip.voidptr
- BasePlot.window()
QWidget.window() -> QWidget
- BasePlot.windowFilePath()
QWidget.windowFilePath() -> QString
- BasePlot.windowFlags()
QWidget.windowFlags() -> Qt.WindowFlags
- BasePlot.windowIcon()
QWidget.windowIcon() -> QIcon
- BasePlot.windowIconText()
QWidget.windowIconText() -> QString
- BasePlot.windowModality()
QWidget.windowModality() -> Qt.WindowModality
- BasePlot.windowOpacity()
QWidget.windowOpacity() -> float
- BasePlot.windowRole()
QWidget.windowRole() -> QString
- BasePlot.windowState()
QWidget.windowState() -> Qt.WindowStates
- BasePlot.windowTitle()
QWidget.windowTitle() -> QString
- BasePlot.windowType()
QWidget.windowType() -> Qt.WindowType
- BasePlot.x()
QWidget.x() -> int
- BasePlot.y()
QWidget.y() -> int
guiqwt.curve
- The curve module provides curve-related objects:
- guiqwt.curve.CurvePlot: a 2d curve plotting widget
- guiqwt.curve.CurveItem: a curve plot item
- guiqwt.curve.ErrorBarCurveItem: a curve plot item with error bars
- guiqwt.curve.GridItem
- guiqwt.curve.ItemListWidget: base widget implementing the plot item list panel
- guiqwt.curve.PlotItemList: the plot item list panel
CurveItem and GridItem objects are plot items (derived from QwtPlotItem) that may be displayed on a 2D plotting widget like guiqwt.curve.CurvePlot or guiqwt.image.ImagePlot.
See also
- Module guiqwt.image
- Module providing image-related plot items and plotting widgets
- Module guiqwt.plot
- Module providing ready-to-use curve and image plotting widgets and dialog boxes
Examples
- Create a basic curve plotting widget:
- before creating any widget, a QApplication must be instantiated (that is a Qt internal requirement):
>>> import guidata
>>> app = guidata.qapplication()
- that is mostly equivalent to the following (the only difference is that the guidata helper function also installs the Qt translation corresponding to the system locale):
>>> from PyQt4.QtGui import QApplication
>>> app = QApplication([])
- now that a QApplication object exists, we may create the plotting widget:
>>> from guiqwt.curve import CurvePlot
>>> plot = CurvePlot(title="Example", xlabel="X", ylabel="Y")
- Create a curve item:
- from the associated plot item class (e.g. ErrorBarCurveItem to create a curve with error bars): the item properties are then assigned by creating the appropriate style parameters object (e.g. guiqwt.styles.ErrorBarParam)
>>> from guiqwt.curve import CurveItem
>>> from guiqwt.styles import CurveParam
>>> param = CurveParam()
>>> param.label = 'My curve'
>>> curve = CurveItem(param)
>>> curve.set_data(x, y)
- or using the plot item builder (see guiqwt.builder.make()):
>>> from guiqwt.builder import make
>>> curve = make.curve(x, y, title='My curve')
Attach the curve to the plotting widget:
>>> plot.add_item(curve)
Display the plotting widget:
>>> plot.show()
>>> app.exec_()
Reference
- class guiqwt.curve.CurvePlot(parent=None, title=None, xlabel=None, ylabel=None, xunit=None, yunit=None, gridparam=None, section='plot', axes_synchronised=False)[source]
Construct a 2D curve plotting widget (this class inherits guiqwt.baseplot.BasePlot)
parent: parent widget
title: plot title
xlabel: (bottom axis title, top axis title) or bottom axis title only
ylabel: (left axis title, right axis title) or left axis title only
xunit: (bottom axis unit, top axis unit) or bottom axis unit only
yunit: (left axis unit, right axis unit) or left axis unit only
gridparam: GridParam instance
- axes_synchronised: keep all x and y axes synchronised when zomming or
panning
- DEFAULT_ITEM_TYPE
alias of ICurveItemType
- class RenderFlags
QWidget.RenderFlags(QWidget.RenderFlags) QWidget.RenderFlags(int) QWidget.RenderFlags()
- CurvePlot.acceptDrops()
QWidget.acceptDrops() -> bool
- CurvePlot.actions()
QWidget.actions() -> list-of-QAction
- CurvePlot.activateWindow()
QWidget.activateWindow()
- CurvePlot.addAction()
QWidget.addAction(QAction)
- CurvePlot.addActions()
QWidget.addActions(list-of-QAction)
- CurvePlot.add_item(item, z=None)[source]
- Add a plot item instance to this plot widget
- item: QwtPlotItem (PyQt4.Qwt5) object implementing the IBasePlotItem interface (guiqwt.interfaces)
- z: item’s z order (None -> z = max(self.get_items())+1)
- CurvePlot.add_item_with_z_offset(item, zoffset)
Add a plot item instance within a specified z range, over zmin
- CurvePlot.adjustSize()
QWidget.adjustSize()
- CurvePlot.autoFillBackground()
QWidget.autoFillBackground() -> bool
- CurvePlot.backgroundRole()
QWidget.backgroundRole() -> QPalette.ColorRole
- CurvePlot.baseSize()
QWidget.baseSize() -> QSize
- CurvePlot.blockSignals()
QObject.blockSignals(bool) -> bool
- CurvePlot.childAt()
QWidget.childAt(QPoint) -> QWidget QWidget.childAt(int, int) -> QWidget
- CurvePlot.children()
QObject.children() -> list-of-QObject
- CurvePlot.childrenRect()
QWidget.childrenRect() -> QRect
- CurvePlot.childrenRegion()
QWidget.childrenRegion() -> QRegion
- CurvePlot.clearFocus()
QWidget.clearFocus()
- CurvePlot.clearMask()
QWidget.clearMask()
- CurvePlot.close()
QWidget.close() -> bool
- CurvePlot.colorCount()
QPaintDevice.colorCount() -> int
- CurvePlot.connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- CurvePlot.contentsMargins()
QWidget.contentsMargins() -> QMargins
- CurvePlot.contentsRect()
QWidget.contentsRect() -> QRect
- CurvePlot.contextMenuPolicy()
QWidget.contextMenuPolicy() -> Qt.ContextMenuPolicy
- CurvePlot.copy_to_clipboard()
Copy widget’s window to clipboard
- CurvePlot.cursor()
QWidget.cursor() -> QCursor
- CurvePlot.customContextMenuRequested
QWidget.customContextMenuRequested[QPoint] [signal]
- CurvePlot.del_all_items(except_grid=True)[source]
Del all items, eventually (default) except grid
- CurvePlot.del_item(item)
Remove item from widget Convenience function (see ‘del_items’)
- CurvePlot.del_items(items)
Remove item from widget
- CurvePlot.deleteLater()
QObject.deleteLater()
- CurvePlot.depth()
QPaintDevice.depth() -> int
- CurvePlot.deserialize(reader)
- Restore items from HDF5 file:
- reader: guidata.hdf5io.HDF5Reader object
See also guiqwt.baseplot.BasePlot.save_items_to_hdf5()
- CurvePlot.destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- CurvePlot.devType()
QWidget.devType() -> int
- CurvePlot.disable_autoscale()
Re-apply the axis scales so as to disable autoscaling without changing the view
- CurvePlot.disable_unused_axes()
Disable unused axes
- CurvePlot.disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- CurvePlot.do_autoscale(replot=True)[source]
Do autoscale on all axes
- CurvePlot.do_pan_view(dx, dy)[source]
Translate the active axes by dx, dy dx, dy are tuples composed of (initial pos, dest pos)
- CurvePlot.do_zoom_view(dx, dy, lock_aspect_ratio=False)[source]
Change the scale of the active axes (zoom/dezoom) according to dx, dy dx, dy are tuples composed of (initial pos, dest pos) We try to keep initial pos fixed on the canvas as the scale changes
- CurvePlot.dumpObjectInfo()
QObject.dumpObjectInfo()
- CurvePlot.dumpObjectTree()
QObject.dumpObjectTree()
- CurvePlot.dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- CurvePlot.edit_axis_parameters(axis_id)
Edit axis parameters
- CurvePlot.edit_plot_parameters(key)
Edit plot parameters
- CurvePlot.effectiveWinId()
QWidget.effectiveWinId() -> sip.voidptr
- CurvePlot.emit()
QObject.emit(SIGNAL(), ...)
- CurvePlot.enable_used_axes()
Enable only used axes For now, this is needed only by the pyplot interface
- CurvePlot.ensurePolished()
QWidget.ensurePolished()
- CurvePlot.eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- CurvePlot.find()
QWidget.find(sip.voidptr) -> QWidget
- CurvePlot.findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- CurvePlot.findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- CurvePlot.focusPolicy()
QWidget.focusPolicy() -> Qt.FocusPolicy
- CurvePlot.focusProxy()
QWidget.focusProxy() -> QWidget
- CurvePlot.focusWidget()
QWidget.focusWidget() -> QWidget
- CurvePlot.font()
QWidget.font() -> QFont
- CurvePlot.fontInfo()
QWidget.fontInfo() -> QFontInfo
- CurvePlot.fontMetrics()
QWidget.fontMetrics() -> QFontMetrics
- CurvePlot.foregroundRole()
QWidget.foregroundRole() -> QPalette.ColorRole
- CurvePlot.frameGeometry()
QWidget.frameGeometry() -> QRect
- CurvePlot.frameRect()
QFrame.frameRect() -> QRect
- CurvePlot.frameShadow()
QFrame.frameShadow() -> QFrame.Shadow
- CurvePlot.frameShape()
QFrame.frameShape() -> QFrame.Shape
- CurvePlot.frameSize()
QWidget.frameSize() -> QSize
- CurvePlot.frameStyle()
QFrame.frameStyle() -> int
- CurvePlot.frameWidth()
QFrame.frameWidth() -> int
- CurvePlot.geometry()
QWidget.geometry() -> QRect
- CurvePlot.getContentsMargins()
QWidget.getContentsMargins() -> (int, int, int, int)
- CurvePlot.get_active_axes()
Return active axes
- CurvePlot.get_active_item(force=False)
Return active item Force item activation if there is no active item
- CurvePlot.get_axesparam_class(item)
Return AxesParam dataset class associated to item’s type
- CurvePlot.get_axis_color(axis_id)
Get axis color (color name, i.e. string)
- CurvePlot.get_axis_direction(axis_id)[source]
- Return axis direction of increasing values
- axis_id: axis id (BasePlot.Y_LEFT, BasePlot.X_BOTTOM, ...) or string: ‘bottom’, ‘left’, ‘top’ or ‘right’
- CurvePlot.get_axis_font(axis_id)
Get axis font
- CurvePlot.get_axis_id(axis_name)
Return axis ID from axis name If axis ID is passed directly, check the ID
- CurvePlot.get_axis_limits(axis_id)
Return axis limits (minimum and maximum values)
- CurvePlot.get_axis_scale(axis_id)
Return the name (‘lin’ or ‘log’) of the scale used by axis
- CurvePlot.get_axis_title(axis_id)
Get axis title
- CurvePlot.get_axis_unit(axis_id)
Get axis unit
Return widget context menu
- CurvePlot.get_default_item()[source]
Return default item, depending on plot’s default item type (e.g. for a curve plot, this is a curve item type).
Return nothing if there is more than one item matching the default item type.
- CurvePlot.get_items(z_sorted=False, item_type=None)
Return widget’s item list (items are based on IBasePlotItem’s interface)
- CurvePlot.get_last_active_item(item_type)
Return last active item corresponding to passed item_type
- CurvePlot.get_max_z()
Return maximum z-order for all items registered in plot If there is no item, return 0
- CurvePlot.get_nearest_object(pos, close_dist=0)
Return nearest item from position ‘pos’ If close_dist > 0: return the first found item (higher z) which
distance to ‘pos’ is less than close_distelse: return the closest item
- CurvePlot.get_nearest_object_in_z(pos)
Return nearest item for which position ‘pos’ is inside of it (iterate over items with respect to their ‘z’ coordinate)
- CurvePlot.get_plot_limits(xaxis='bottom', yaxis='left')[source]
Return plot scale limits
- CurvePlot.get_private_items(z_sorted=False, item_type=None)
Return widget’s private item list (items are based on IBasePlotItem’s interface)
- CurvePlot.get_public_items(z_sorted=False, item_type=None)
Return widget’s public item list (items are based on IBasePlotItem’s interface)
- CurvePlot.get_scales()
Return active curve scales
- CurvePlot.get_selected_items(z_sorted=False, item_type=None)
Return selected items
- CurvePlot.get_title()
Get plot title
- CurvePlot.grabGesture()
QWidget.grabGesture(Qt.GestureType, Qt.GestureFlags flags=Qt.GestureFlags(0))
- CurvePlot.grabKeyboard()
QWidget.grabKeyboard()
- CurvePlot.grabMouse()
QWidget.grabMouse() QWidget.grabMouse(QCursor)
- CurvePlot.grabShortcut()
QWidget.grabShortcut(QKeySequence, Qt.ShortcutContext context=Qt.WindowShortcut) -> int
- CurvePlot.graphicsEffect()
QWidget.graphicsEffect() -> QGraphicsEffect
- CurvePlot.graphicsProxyWidget()
QWidget.graphicsProxyWidget() -> QGraphicsProxyWidget
- CurvePlot.hasFocus()
QWidget.hasFocus() -> bool
- CurvePlot.hasMouseTracking()
QWidget.hasMouseTracking() -> bool
- CurvePlot.height()
QWidget.height() -> int
- CurvePlot.heightForWidth()
QWidget.heightForWidth(int) -> int
- CurvePlot.heightMM()
QPaintDevice.heightMM() -> int
- CurvePlot.hide()
QWidget.hide()
- CurvePlot.hide_items(items=None, item_type=None)
Hide items (if items is None, hide all items)
- CurvePlot.inherits()
QObject.inherits(str) -> bool
- CurvePlot.inputContext()
QWidget.inputContext() -> QInputContext
- CurvePlot.inputMethodHints()
QWidget.inputMethodHints() -> Qt.InputMethodHints
- CurvePlot.inputMethodQuery()
QWidget.inputMethodQuery(Qt.InputMethodQuery) -> QVariant
- CurvePlot.insertAction()
QWidget.insertAction(QAction, QAction)
- CurvePlot.insertActions()
QWidget.insertActions(QAction, list-of-QAction)
- CurvePlot.installEventFilter()
QObject.installEventFilter(QObject)
- CurvePlot.invalidate()
Invalidate paint cache and schedule redraw use instead of replot when only the content of the canvas needs redrawing (axes, shouldn’t change)
- CurvePlot.isActiveWindow()
QWidget.isActiveWindow() -> bool
- CurvePlot.isAncestorOf()
QWidget.isAncestorOf(QWidget) -> bool
- CurvePlot.isEnabled()
QWidget.isEnabled() -> bool
- CurvePlot.isEnabledTo()
QWidget.isEnabledTo(QWidget) -> bool
- CurvePlot.isEnabledToTLW()
QWidget.isEnabledToTLW() -> bool
- CurvePlot.isFullScreen()
QWidget.isFullScreen() -> bool
- CurvePlot.isHidden()
QWidget.isHidden() -> bool
- CurvePlot.isLeftToRight()
QWidget.isLeftToRight() -> bool
- CurvePlot.isMaximized()
QWidget.isMaximized() -> bool
- CurvePlot.isMinimized()
QWidget.isMinimized() -> bool
- CurvePlot.isModal()
QWidget.isModal() -> bool
- CurvePlot.isRightToLeft()
QWidget.isRightToLeft() -> bool
- CurvePlot.isTopLevel()
QWidget.isTopLevel() -> bool
- CurvePlot.isVisible()
QWidget.isVisible() -> bool
- CurvePlot.isVisibleTo()
QWidget.isVisibleTo(QWidget) -> bool
- CurvePlot.isWidgetType()
QObject.isWidgetType() -> bool
- CurvePlot.isWindow()
QWidget.isWindow() -> bool
- CurvePlot.isWindowModified()
QWidget.isWindowModified() -> bool
- CurvePlot.keyboardGrabber()
QWidget.keyboardGrabber() -> QWidget
- CurvePlot.killTimer()
QObject.killTimer(int)
- CurvePlot.layout()
QWidget.layout() -> QLayout
- CurvePlot.layoutDirection()
QWidget.layoutDirection() -> Qt.LayoutDirection
- CurvePlot.lineWidth()
QFrame.lineWidth() -> int
- CurvePlot.locale()
QWidget.locale() -> QLocale
- CurvePlot.logicalDpiX()
QPaintDevice.logicalDpiX() -> int
- CurvePlot.logicalDpiY()
QPaintDevice.logicalDpiY() -> int
- CurvePlot.lower()
QWidget.lower()
- CurvePlot.mapFrom()
QWidget.mapFrom(QWidget, QPoint) -> QPoint
- CurvePlot.mapFromGlobal()
QWidget.mapFromGlobal(QPoint) -> QPoint
- CurvePlot.mapFromParent()
QWidget.mapFromParent(QPoint) -> QPoint
- CurvePlot.mapTo()
QWidget.mapTo(QWidget, QPoint) -> QPoint
- CurvePlot.mapToGlobal()
QWidget.mapToGlobal(QPoint) -> QPoint
- CurvePlot.mapToParent()
QWidget.mapToParent(QPoint) -> QPoint
- CurvePlot.mask()
QWidget.mask() -> QRegion
- CurvePlot.maximumHeight()
QWidget.maximumHeight() -> int
- CurvePlot.maximumSize()
QWidget.maximumSize() -> QSize
- CurvePlot.maximumWidth()
QWidget.maximumWidth() -> int
- CurvePlot.metaObject()
QObject.metaObject() -> QMetaObject
- CurvePlot.midLineWidth()
QFrame.midLineWidth() -> int
- CurvePlot.minimumHeight()
QWidget.minimumHeight() -> int
- CurvePlot.minimumSize()
QWidget.minimumSize() -> QSize
- CurvePlot.minimumWidth()
QWidget.minimumWidth() -> int
- CurvePlot.mouseDoubleClickEvent(event)
Reimplement QWidget method
- CurvePlot.mouseGrabber()
QWidget.mouseGrabber() -> QWidget
- CurvePlot.move()
QWidget.move(QPoint) QWidget.move(int, int)
- CurvePlot.moveToThread()
QObject.moveToThread(QThread)
- CurvePlot.move_down(item_list)
Move item(s) down, i.e. to the background (swap item with the previous item in z-order)
item: plot item or list of plot items
Return True if items have been moved effectively
- CurvePlot.move_up(item_list)
Move item(s) up, i.e. to the foreground (swap item with the next item in z-order)
item: plot item or list of plot items
Return True if items have been moved effectively
- CurvePlot.nativeParentWidget()
QWidget.nativeParentWidget() -> QWidget
- CurvePlot.nextInFocusChain()
QWidget.nextInFocusChain() -> QWidget
- CurvePlot.normalGeometry()
QWidget.normalGeometry() -> QRect
- CurvePlot.numColors()
QPaintDevice.numColors() -> int
- CurvePlot.objectName()
QObject.objectName() -> QString
- CurvePlot.overrideWindowFlags()
QWidget.overrideWindowFlags(Qt.WindowFlags)
- CurvePlot.overrideWindowState()
QWidget.overrideWindowState(Qt.WindowStates)
- CurvePlot.paintEngine()
QWidget.paintEngine() -> QPaintEngine
- CurvePlot.paintingActive()
QPaintDevice.paintingActive() -> bool
- CurvePlot.palette()
QWidget.palette() -> QPalette
- CurvePlot.parent()
QObject.parent() -> QObject
- CurvePlot.parentWidget()
QWidget.parentWidget() -> QWidget
- CurvePlot.physicalDpiX()
QPaintDevice.physicalDpiX() -> int
- CurvePlot.physicalDpiY()
QPaintDevice.physicalDpiY() -> int
- CurvePlot.pos()
QWidget.pos() -> QPoint
- CurvePlot.previousInFocusChain()
QWidget.previousInFocusChain() -> QWidget
- CurvePlot.property()
QObject.property(str) -> QVariant
- CurvePlot.pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- CurvePlot.raise_()
QWidget.raise_()
- CurvePlot.read_axes_styles(section, options)
Read axes styles from section and options (one option for each axis in the order left, right, bottom, top)
Skip axis if option is None
- CurvePlot.rect()
QWidget.rect() -> QRect
- CurvePlot.releaseKeyboard()
QWidget.releaseKeyboard()
- CurvePlot.releaseMouse()
QWidget.releaseMouse()
- CurvePlot.releaseShortcut()
QWidget.releaseShortcut(int)
- CurvePlot.removeAction()
QWidget.removeAction(QAction)
- CurvePlot.removeEventFilter()
QObject.removeEventFilter(QObject)
- CurvePlot.render()
QWidget.render(QPaintDevice, QPoint targetOffset=QPoint(), QRegion sourceRegion=QRegion(), QWidget.RenderFlags flags=QWidget.DrawWindowBackground|QWidget.DrawChildren) QWidget.render(QPainter, QPoint targetOffset=QPoint(), QRegion sourceRegion=QRegion(), QWidget.RenderFlags flags=QWidget.DrawWindowBackground|QWidget.DrawChildren)
- CurvePlot.repaint()
QWidget.repaint() QWidget.repaint(int, int, int, int) QWidget.repaint(QRect) QWidget.repaint(QRegion)
- CurvePlot.resize()
QWidget.resize(QSize) QWidget.resize(int, int)
- CurvePlot.restoreGeometry()
QWidget.restoreGeometry(QByteArray) -> bool
- CurvePlot.restore_items(iofile)
- Restore items from file using the pickle protocol
- iofile: file object or filename
- CurvePlot.saveGeometry()
QWidget.saveGeometry() -> QByteArray
- CurvePlot.save_items(iofile, selected=False)
- Save (serializable) items to file using the pickle protocol
- iofile: file object or filename
- selected=False: if True, will save only selected items
- CurvePlot.scroll()
QWidget.scroll(int, int) QWidget.scroll(int, int, QRect)
- CurvePlot.select_all()
Select all selectable items
- CurvePlot.select_item(item)
Select item
- CurvePlot.select_some_items(items)
Select items
- CurvePlot.serialize(writer, selected=False)
- Save (serializable) items to HDF5 file:
- writer: guidata.hdf5io.HDF5Writer object
- selected=False: if True, will save only selected items
See also guiqwt.baseplot.BasePlot.restore_items_from_hdf5()
- CurvePlot.setAcceptDrops()
QWidget.setAcceptDrops(bool)
- CurvePlot.setAttribute()
QWidget.setAttribute(Qt.WidgetAttribute, bool on=True)
- CurvePlot.setAutoFillBackground()
QWidget.setAutoFillBackground(bool)
- CurvePlot.setBackgroundRole()
QWidget.setBackgroundRole(QPalette.ColorRole)
- CurvePlot.setBaseSize()
QWidget.setBaseSize(int, int) QWidget.setBaseSize(QSize)
- CurvePlot.setContentsMargins()
QWidget.setContentsMargins(int, int, int, int) QWidget.setContentsMargins(QMargins)
- CurvePlot.setContextMenuPolicy()
QWidget.setContextMenuPolicy(Qt.ContextMenuPolicy)
- CurvePlot.setCursor()
QWidget.setCursor(QCursor)
- CurvePlot.setDisabled()
QWidget.setDisabled(bool)
- CurvePlot.setEnabled()
QWidget.setEnabled(bool)
- CurvePlot.setFixedHeight()
QWidget.setFixedHeight(int)
- CurvePlot.setFixedSize()
QWidget.setFixedSize(QSize) QWidget.setFixedSize(int, int)
- CurvePlot.setFixedWidth()
QWidget.setFixedWidth(int)
- CurvePlot.setFocus()
QWidget.setFocus() QWidget.setFocus(Qt.FocusReason)
- CurvePlot.setFocusPolicy()
QWidget.setFocusPolicy(Qt.FocusPolicy)
- CurvePlot.setFocusProxy()
QWidget.setFocusProxy(QWidget)
- CurvePlot.setFont()
QWidget.setFont(QFont)
- CurvePlot.setForegroundRole()
QWidget.setForegroundRole(QPalette.ColorRole)
- CurvePlot.setFrameRect()
QFrame.setFrameRect(QRect)
- CurvePlot.setFrameShadow()
QFrame.setFrameShadow(QFrame.Shadow)
- CurvePlot.setFrameShape()
QFrame.setFrameShape(QFrame.Shape)
- CurvePlot.setFrameStyle()
QFrame.setFrameStyle(int)
- CurvePlot.setGeometry()
QWidget.setGeometry(QRect) QWidget.setGeometry(int, int, int, int)
- CurvePlot.setGraphicsEffect()
QWidget.setGraphicsEffect(QGraphicsEffect)
- CurvePlot.setHidden()
QWidget.setHidden(bool)
- CurvePlot.setInputContext()
QWidget.setInputContext(QInputContext)
- CurvePlot.setInputMethodHints()
QWidget.setInputMethodHints(Qt.InputMethodHints)
- CurvePlot.setLayout()
QWidget.setLayout(QLayout)
- CurvePlot.setLayoutDirection()
QWidget.setLayoutDirection(Qt.LayoutDirection)
- CurvePlot.setLineWidth()
QFrame.setLineWidth(int)
- CurvePlot.setLocale()
QWidget.setLocale(QLocale)
- CurvePlot.setMask()
QWidget.setMask(QBitmap) QWidget.setMask(QRegion)
- CurvePlot.setMaximumHeight()
QWidget.setMaximumHeight(int)
- CurvePlot.setMaximumSize()
QWidget.setMaximumSize(int, int) QWidget.setMaximumSize(QSize)
- CurvePlot.setMaximumWidth()
QWidget.setMaximumWidth(int)
- CurvePlot.setMidLineWidth()
QFrame.setMidLineWidth(int)
- CurvePlot.setMinimumHeight()
QWidget.setMinimumHeight(int)
- CurvePlot.setMinimumSize()
QWidget.setMinimumSize(int, int) QWidget.setMinimumSize(QSize)
- CurvePlot.setMinimumWidth()
QWidget.setMinimumWidth(int)
- CurvePlot.setMouseTracking()
QWidget.setMouseTracking(bool)
- CurvePlot.setObjectName()
QObject.setObjectName(QString)
- CurvePlot.setPalette()
QWidget.setPalette(QPalette)
- CurvePlot.setParent()
QWidget.setParent(QWidget) QWidget.setParent(QWidget, Qt.WindowFlags)
- CurvePlot.setProperty()
QObject.setProperty(str, QVariant) -> bool
- CurvePlot.setShortcutAutoRepeat()
QWidget.setShortcutAutoRepeat(int, bool enabled=True)
- CurvePlot.setShortcutEnabled()
QWidget.setShortcutEnabled(int, bool enabled=True)
- CurvePlot.setShown()
QWidget.setShown(bool)
- CurvePlot.setSizeIncrement()
QWidget.setSizeIncrement(int, int) QWidget.setSizeIncrement(QSize)
- CurvePlot.setSizePolicy()
QWidget.setSizePolicy(QSizePolicy) QWidget.setSizePolicy(QSizePolicy.Policy, QSizePolicy.Policy)
- CurvePlot.setStatusTip()
QWidget.setStatusTip(QString)
- CurvePlot.setStyle()
QWidget.setStyle(QStyle)
- CurvePlot.setStyleSheet()
QWidget.setStyleSheet(QString)
- CurvePlot.setTabOrder()
QWidget.setTabOrder(QWidget, QWidget)
- CurvePlot.setToolTip()
QWidget.setToolTip(QString)
- CurvePlot.setUpdatesEnabled()
QWidget.setUpdatesEnabled(bool)
- CurvePlot.setVisible()
QWidget.setVisible(bool)
- CurvePlot.setWhatsThis()
QWidget.setWhatsThis(QString)
- CurvePlot.setWindowFilePath()
QWidget.setWindowFilePath(QString)
- CurvePlot.setWindowFlags()
QWidget.setWindowFlags(Qt.WindowFlags)
- CurvePlot.setWindowIcon()
QWidget.setWindowIcon(QIcon)
- CurvePlot.setWindowIconText()
QWidget.setWindowIconText(QString)
- CurvePlot.setWindowModality()
QWidget.setWindowModality(Qt.WindowModality)
- CurvePlot.setWindowModified()
QWidget.setWindowModified(bool)
- CurvePlot.setWindowOpacity()
QWidget.setWindowOpacity(float)
- CurvePlot.setWindowRole()
QWidget.setWindowRole(QString)
- CurvePlot.setWindowState()
QWidget.setWindowState(Qt.WindowStates)
- CurvePlot.setWindowTitle()
QWidget.setWindowTitle(QString)
- CurvePlot.set_active_item(item)[source]
Override base set_active_item to change the grid’s axes according to the selected item
- CurvePlot.set_antialiasing(checked)[source]
Toggle curve antialiasing
- CurvePlot.set_axis_color(axis_id, color)
Set axis color color: color name (string) or QColor instance
- CurvePlot.set_axis_direction(axis_id, reverse=False)[source]
- Set axis direction of increasing values
axis_id: axis id (BasePlot.Y_LEFT, BasePlot.X_BOTTOM, ...) or string: ‘bottom’, ‘left’, ‘top’ or ‘right’
- reverse: False (default)
- x-axis values increase from left to right
- y-axis values increase from bottom to top
- reverse: True
- x-axis values increase from right to left
- y-axis values increase from top to bottom
- CurvePlot.set_axis_font(axis_id, font)
Set axis font
- CurvePlot.set_axis_limits(axis_id, vmin, vmax, stepsize=0)[source]
Set axis limits (minimum and maximum values)
- CurvePlot.set_axis_scale(axis_id, scale, autoscale=True)
Set axis scale Example: self.set_axis_scale(curve.yAxis(), ‘lin’)
- CurvePlot.set_axis_ticks(axis_id, nmajor=None, nminor=None)
Set axis maximum number of major ticks and maximum of minor ticks
- CurvePlot.set_axis_title(axis_id, text)
Set axis title
- CurvePlot.set_axis_unit(axis_id, text)
Set axis unit
- CurvePlot.set_item_visible(item, state, notify=True, replot=True)
Show/hide item and emit a SIG_ITEMS_CHANGED signal
- CurvePlot.set_items(*args)
Utility function used to quickly setup a plot with a set of items
- CurvePlot.set_items_readonly(state)
Set all items readonly state to state Default item’s readonly state: False (items may be deleted)
- CurvePlot.set_manager(manager, plot_id)
Set the associated guiqwt.plot.PlotManager instance
- CurvePlot.set_plot_limits(x0, x1, y0, y1, xaxis='bottom', yaxis='left')[source]
Set plot scale limits
- CurvePlot.set_pointer(pointer_type)[source]
Set pointer. Valid values of pointer_type:
- None: disable pointer
- “canvas”: enable canvas pointer
- “curve”: enable on-curve pointer
- CurvePlot.set_scales(xscale, yscale)
Set active curve scales Example: self.set_scales(‘lin’, ‘lin’)
- CurvePlot.set_title(title)
Set plot title
- CurvePlot.set_titles(title=None, xlabel=None, ylabel=None, xunit=None, yunit=None)[source]
- Set plot and axes titles at once
- title: plot title
- xlabel: (bottom axis title, top axis title) or bottom axis title only
- ylabel: (left axis title, right axis title) or left axis title only
- xunit: (bottom axis unit, top axis unit) or bottom axis unit only
- yunit: (left axis unit, right axis unit) or left axis unit only
- CurvePlot.show()
QWidget.show()
- CurvePlot.showEvent(event)
Reimplement Qwt method
- CurvePlot.showFullScreen()
QWidget.showFullScreen()
- CurvePlot.showMaximized()
QWidget.showMaximized()
- CurvePlot.showMinimized()
QWidget.showMinimized()
- CurvePlot.showNormal()
QWidget.showNormal()
- CurvePlot.show_items(items=None, item_type=None)
Show items (if items is None, show all items)
- CurvePlot.signalsBlocked()
QObject.signalsBlocked() -> bool
- CurvePlot.size()
QWidget.size() -> QSize
- CurvePlot.sizeHint()
Preferred size
- CurvePlot.sizeIncrement()
QWidget.sizeIncrement() -> QSize
- CurvePlot.sizePolicy()
QWidget.sizePolicy() -> QSizePolicy
- CurvePlot.stackUnder()
QWidget.stackUnder(QWidget)
- CurvePlot.startTimer()
QObject.startTimer(int) -> int
- CurvePlot.statusTip()
QWidget.statusTip() -> QString
- CurvePlot.style()
QWidget.style() -> QStyle
- CurvePlot.styleSheet()
QWidget.styleSheet() -> QString
- CurvePlot.testAttribute()
QWidget.testAttribute(Qt.WidgetAttribute) -> bool
- CurvePlot.thread()
QObject.thread() -> QThread
- CurvePlot.toolTip()
QWidget.toolTip() -> QString
- CurvePlot.topLevelWidget()
QWidget.topLevelWidget() -> QWidget
- CurvePlot.tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- CurvePlot.trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- CurvePlot.underMouse()
QWidget.underMouse() -> bool
- CurvePlot.ungrabGesture()
QWidget.ungrabGesture(Qt.GestureType)
- CurvePlot.unselect_all()
Unselect all selected items
- CurvePlot.unselect_item(item)
Unselect item
- CurvePlot.unsetCursor()
QWidget.unsetCursor()
- CurvePlot.unsetLayoutDirection()
QWidget.unsetLayoutDirection()
- CurvePlot.unsetLocale()
QWidget.unsetLocale()
- CurvePlot.update()
QWidget.update() QWidget.update(QRect) QWidget.update(QRegion) QWidget.update(int, int, int, int)
- CurvePlot.updateGeometry()
QWidget.updateGeometry()
- CurvePlot.update_all_axes_styles()
Update all axes styles
- CurvePlot.update_axis_style(axis_id)
Update axis style
- CurvePlot.updatesEnabled()
QWidget.updatesEnabled() -> bool
- CurvePlot.visibleRegion()
QWidget.visibleRegion() -> QRegion
- CurvePlot.whatsThis()
QWidget.whatsThis() -> QString
- CurvePlot.width()
QWidget.width() -> int
- CurvePlot.widthMM()
QPaintDevice.widthMM() -> int
- CurvePlot.winId()
QWidget.winId() -> sip.voidptr
- CurvePlot.window()
QWidget.window() -> QWidget
- CurvePlot.windowFilePath()
QWidget.windowFilePath() -> QString
- CurvePlot.windowFlags()
QWidget.windowFlags() -> Qt.WindowFlags
- CurvePlot.windowIcon()
QWidget.windowIcon() -> QIcon
- CurvePlot.windowIconText()
QWidget.windowIconText() -> QString
- CurvePlot.windowModality()
QWidget.windowModality() -> Qt.WindowModality
- CurvePlot.windowOpacity()
QWidget.windowOpacity() -> float
- CurvePlot.windowRole()
QWidget.windowRole() -> QString
- CurvePlot.windowState()
QWidget.windowState() -> Qt.WindowStates
- CurvePlot.windowTitle()
QWidget.windowTitle() -> QString
- CurvePlot.windowType()
QWidget.windowType() -> Qt.WindowType
- CurvePlot.x()
QWidget.x() -> int
- CurvePlot.y()
QWidget.y() -> int
- class guiqwt.curve.CurveItem(curveparam=None)[source]
Construct a curve plot item with the parameters curveparam (see guiqwt.styles.CurveParam)
- boundingRect()[source]
Return the bounding rectangle of the data
- deserialize(reader)[source]
Deserialize object from HDF5 reader
- get_closest_coordinates(x, y)[source]
Renvoie les coordonnées (x’,y’) du point le plus proche de (x,y) Méthode surchargée pour ErrorBarSignalCurve pour renvoyer les coordonnées des pointes des barres d’erreur
- get_data()[source]
Return curve data x, y (NumPy arrays)
- hit_test(pos)[source]
Calcul de la distance d’un point à une courbe renvoie (dist, handle, inside)
- is_empty()[source]
Return True if item data is empty
- is_private()[source]
Return True if object is private
- is_readonly()[source]
Return object readonly state
- move_local_shape(old_pos, new_pos)[source]
Translate the shape such that old_pos becomes new_pos in canvas coordinates
- move_with_selection(delta_x, delta_y)[source]
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()[source]
Select item
- serialize(writer)[source]
Serialize object to HDF5 writer
- set_data(x, y)[source]
- Set curve data:
- x: NumPy array
- y: NumPy array
- set_movable(state)[source]
Set item movable state
- set_private(state)[source]
Set object as private
- set_readonly(state)[source]
Set object readonly state
- set_resizable(state)[source]
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)[source]
Set item rotatable state
- set_selectable(state)[source]
Set item selectable state
- unselect()[source]
Unselect item
- class guiqwt.curve.ErrorBarCurveItem(curveparam=None, errorbarparam=None)[source]
Construct an error-bar curve plot item with the parameters errorbarparam (see guiqwt.styles.ErrorBarParam)
- boundingRect()[source]
Return the bounding rectangle of the data, error bars included
- deserialize(reader)[source]
Deserialize object from HDF5 reader
- get_data()[source]
- Return error-bar curve data: x, y, dx, dy
- x: NumPy array
- y: NumPy array
- dx: float or NumPy array (non-constant error bars)
- dy: float or NumPy array (non-constant error bars)
- hit_test(pos)
Calcul de la distance d’un point à une courbe renvoie (dist, handle, inside)
- is_empty()
Return True if item data is empty
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- move_local_shape(old_pos, new_pos)
Translate the shape such that old_pos becomes new_pos in canvas coordinates
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- serialize(writer)[source]
Serialize object to HDF5 writer
- set_data(x, y, dx=None, dy=None)[source]
- Set error-bar curve data:
- x: NumPy array
- y: NumPy array
- dx: float or NumPy array (non-constant error bars)
- dy: float or NumPy array (non-constant error bars)
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- unselect()[source]
Unselect item
guiqwt.image
The image module provides image-related objects and functions:
- guiqwt.image.ImagePlot: a 2D curve and image plotting widget, derived from guiqwt.curve.CurvePlot
- guiqwt.image.ImageItem: simple images
- guiqwt.image.TrImageItem: images supporting arbitrary affine transform
- guiqwt.image.XYImageItem: images with non-linear X/Y axes
- guiqwt.image.Histogram2DItem: 2D histogram
- guiqwt.image.ImageFilterItem: rectangular filtering area that may be resized and moved onto the processed image
- guiqwt.image.assemble_imageitems()
- guiqwt.image.get_plot_source_rect()
- guiqwt.image.get_image_from_plot()
ImageItem, TrImageItem, XYImageItem, Histogram2DItem and ImageFilterItem objects are plot items (derived from QwtPlotItem) that may be displayed on a guiqwt.image.ImagePlot plotting widget.
See also
- Module guiqwt.curve
- Module providing curve-related plot items and plotting widgets
- Module guiqwt.plot
- Module providing ready-to-use curve and image plotting widgets and dialog boxes
Examples
- Create a basic image plotting widget:
- before creating any widget, a QApplication must be instantiated (that is a Qt internal requirement):
>>> import guidata
>>> app = guidata.qapplication()
- that is mostly equivalent to the following (the only difference is that the guidata helper function also installs the Qt translation corresponding to the system locale):
>>> from PyQt4.QtGui import QApplication
>>> app = QApplication([])
- now that a QApplication object exists, we may create the plotting widget:
>>> from guiqwt.image import ImagePlot
>>> plot = ImagePlot(title="Example")
Generate random data for testing purpose:
>>> import numpy as np
>>> data = np.random.rand(100, 100)
- Create a simple image item:
- from the associated plot item class (e.g. XYImageItem to create an image with non-linear X/Y axes): the item properties are then assigned by creating the appropriate style parameters object (e.g. :py:class:`guiqwt.styles.ImageParam)
>>> from guiqwt.curve import ImageItem
>>> from guiqwt.styles import ImageParam
>>> param = ImageParam()
>>> param.label = 'My image'
>>> image = ImageItem(param)
>>> image.set_data(data)
- or using the plot item builder (see guiqwt.builder.make()):
>>> from guiqwt.builder import make
>>> image = make.image(data, title='My image')
Attach the image to the plotting widget:
>>> plot.add_item(image)
Display the plotting widget:
>>> plot.show()
>>> app.exec_()
Reference
- class guiqwt.image.ImagePlot(parent=None, title=None, xlabel=None, ylabel=None, zlabel=None, xunit=None, yunit=None, zunit=None, yreverse=True, aspect_ratio=1.0, lock_aspect_ratio=True, gridparam=None, section='plot')[source]
Construct a 2D curve and image plotting widget (this class inherits guiqwt.curve.CurvePlot)
- parent: parent widget
- title: plot title (string)
- xlabel, ylabel, zlabel: resp. bottom, left and right axis titles (strings)
- xunit, yunit, zunit: resp. bottom, left and right axis units (strings)
- yreverse: reversing y-axis direction of increasing values (bool)
- aspect_ratio: height to width ratio (float)
- lock_aspect_ratio: locking aspect ratio (bool)
- DEFAULT_ITEM_TYPE
alias of IImageItemType
- class RenderFlags
QWidget.RenderFlags(QWidget.RenderFlags) QWidget.RenderFlags(int) QWidget.RenderFlags()
- ImagePlot.acceptDrops()
QWidget.acceptDrops() -> bool
- ImagePlot.actions()
QWidget.actions() -> list-of-QAction
- ImagePlot.activateWindow()
QWidget.activateWindow()
- ImagePlot.addAction()
QWidget.addAction(QAction)
- ImagePlot.addActions()
QWidget.addActions(list-of-QAction)
- ImagePlot.add_item(item, z=None, autoscale=True)[source]
Add a plot item instance to this plot widget
- item: QwtPlotItem (PyQt4.Qwt5) object implementing
- the IBasePlotItem interface (guiqwt.interfaces)
z: item’s z order (None -> z = max(self.get_items())+1) autoscale: True -> rescale plot to fit image bounds
- ImagePlot.add_item_with_z_offset(item, zoffset)
Add a plot item instance within a specified z range, over zmin
- ImagePlot.adjustSize()
QWidget.adjustSize()
- ImagePlot.autoFillBackground()
QWidget.autoFillBackground() -> bool
- ImagePlot.backgroundRole()
QWidget.backgroundRole() -> QPalette.ColorRole
- ImagePlot.baseSize()
QWidget.baseSize() -> QSize
- ImagePlot.blockSignals()
QObject.blockSignals(bool) -> bool
- ImagePlot.childAt()
QWidget.childAt(QPoint) -> QWidget QWidget.childAt(int, int) -> QWidget
- ImagePlot.children()
QObject.children() -> list-of-QObject
- ImagePlot.childrenRect()
QWidget.childrenRect() -> QRect
- ImagePlot.childrenRegion()
QWidget.childrenRegion() -> QRegion
- ImagePlot.clearFocus()
QWidget.clearFocus()
- ImagePlot.clearMask()
QWidget.clearMask()
- ImagePlot.close()
QWidget.close() -> bool
- ImagePlot.colorCount()
QPaintDevice.colorCount() -> int
- ImagePlot.connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- ImagePlot.contentsMargins()
QWidget.contentsMargins() -> QMargins
- ImagePlot.contentsRect()
QWidget.contentsRect() -> QRect
- ImagePlot.contextMenuPolicy()
QWidget.contextMenuPolicy() -> Qt.ContextMenuPolicy
- ImagePlot.copy_to_clipboard()
Copy widget’s window to clipboard
- ImagePlot.cursor()
QWidget.cursor() -> QCursor
- ImagePlot.customContextMenuRequested
QWidget.customContextMenuRequested[QPoint] [signal]
- ImagePlot.del_all_items(except_grid=True)
Del all items, eventually (default) except grid
- ImagePlot.del_item(item)
Remove item from widget Convenience function (see ‘del_items’)
- ImagePlot.del_items(items)
Remove item from widget
- ImagePlot.deleteLater()
QObject.deleteLater()
- ImagePlot.depth()
QPaintDevice.depth() -> int
- ImagePlot.deserialize(reader)
- Restore items from HDF5 file:
- reader: guidata.hdf5io.HDF5Reader object
See also guiqwt.baseplot.BasePlot.save_items_to_hdf5()
- ImagePlot.destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- ImagePlot.devType()
QWidget.devType() -> int
- ImagePlot.disable_autoscale()
Re-apply the axis scales so as to disable autoscaling without changing the view
- ImagePlot.disable_unused_axes()
Disable unused axes
- ImagePlot.disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- ImagePlot.do_autoscale(replot=True)[source]
Do autoscale on all axes
- ImagePlot.do_pan_view(dx, dy)
Translate the active axes by dx, dy dx, dy are tuples composed of (initial pos, dest pos)
- ImagePlot.do_zoom_view(dx, dy)[source]
Reimplement CurvePlot method
- ImagePlot.dumpObjectInfo()
QObject.dumpObjectInfo()
- ImagePlot.dumpObjectTree()
QObject.dumpObjectTree()
- ImagePlot.dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- ImagePlot.edit_axis_parameters(axis_id)[source]
Edit axis parameters
- ImagePlot.edit_plot_parameters(key)
Edit plot parameters
- ImagePlot.effectiveWinId()
QWidget.effectiveWinId() -> sip.voidptr
- ImagePlot.emit()
QObject.emit(SIGNAL(), ...)
- ImagePlot.enable_used_axes()
Enable only used axes For now, this is needed only by the pyplot interface
- ImagePlot.ensurePolished()
QWidget.ensurePolished()
- ImagePlot.eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- ImagePlot.find()
QWidget.find(sip.voidptr) -> QWidget
- ImagePlot.findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- ImagePlot.findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- ImagePlot.focusPolicy()
QWidget.focusPolicy() -> Qt.FocusPolicy
- ImagePlot.focusProxy()
QWidget.focusProxy() -> QWidget
- ImagePlot.focusWidget()
QWidget.focusWidget() -> QWidget
- ImagePlot.font()
QWidget.font() -> QFont
- ImagePlot.fontInfo()
QWidget.fontInfo() -> QFontInfo
- ImagePlot.fontMetrics()
QWidget.fontMetrics() -> QFontMetrics
- ImagePlot.foregroundRole()
QWidget.foregroundRole() -> QPalette.ColorRole
- ImagePlot.frameGeometry()
QWidget.frameGeometry() -> QRect
- ImagePlot.frameRect()
QFrame.frameRect() -> QRect
- ImagePlot.frameShadow()
QFrame.frameShadow() -> QFrame.Shadow
- ImagePlot.frameShape()
QFrame.frameShape() -> QFrame.Shape
- ImagePlot.frameSize()
QWidget.frameSize() -> QSize
- ImagePlot.frameStyle()
QFrame.frameStyle() -> int
- ImagePlot.frameWidth()
QFrame.frameWidth() -> int
- ImagePlot.geometry()
QWidget.geometry() -> QRect
- ImagePlot.getContentsMargins()
QWidget.getContentsMargins() -> (int, int, int, int)
- ImagePlot.get_active_axes()
Return active axes
- ImagePlot.get_active_item(force=False)
Return active item Force item activation if there is no active item
- ImagePlot.get_aspect_ratio()[source]
Return aspect ratio
- ImagePlot.get_axesparam_class(item)[source]
Return AxesParam dataset class associated to item’s type
- ImagePlot.get_axis_color(axis_id)
Get axis color (color name, i.e. string)
- ImagePlot.get_axis_direction(axis_id)
- Return axis direction of increasing values
- axis_id: axis id (BasePlot.Y_LEFT, BasePlot.X_BOTTOM, ...) or string: ‘bottom’, ‘left’, ‘top’ or ‘right’
- ImagePlot.get_axis_font(axis_id)
Get axis font
- ImagePlot.get_axis_id(axis_name)
Return axis ID from axis name If axis ID is passed directly, check the ID
- ImagePlot.get_axis_limits(axis_id)
Return axis limits (minimum and maximum values)
- ImagePlot.get_axis_scale(axis_id)
Return the name (‘lin’ or ‘log’) of the scale used by axis
- ImagePlot.get_axis_title(axis_id)
Get axis title
- ImagePlot.get_axis_unit(axis_id)
Get axis unit
Return widget context menu
- ImagePlot.get_current_aspect_ratio()[source]
Return current aspect ratio
- ImagePlot.get_default_item()
Return default item, depending on plot’s default item type (e.g. for a curve plot, this is a curve item type).
Return nothing if there is more than one item matching the default item type.
- ImagePlot.get_items(z_sorted=False, item_type=None)
Return widget’s item list (items are based on IBasePlotItem’s interface)
- ImagePlot.get_last_active_item(item_type)
Return last active item corresponding to passed item_type
- ImagePlot.get_max_z()
Return maximum z-order for all items registered in plot If there is no item, return 0
- ImagePlot.get_nearest_object(pos, close_dist=0)
Return nearest item from position ‘pos’ If close_dist > 0: return the first found item (higher z) which
distance to ‘pos’ is less than close_distelse: return the closest item
- ImagePlot.get_nearest_object_in_z(pos)
Return nearest item for which position ‘pos’ is inside of it (iterate over items with respect to their ‘z’ coordinate)
- ImagePlot.get_plot_limits(xaxis='bottom', yaxis='left')
Return plot scale limits
- ImagePlot.get_private_items(z_sorted=False, item_type=None)
Return widget’s private item list (items are based on IBasePlotItem’s interface)
- ImagePlot.get_public_items(z_sorted=False, item_type=None)
Return widget’s public item list (items are based on IBasePlotItem’s interface)
- ImagePlot.get_scales()
Return active curve scales
- ImagePlot.get_selected_items(z_sorted=False, item_type=None)
Return selected items
- ImagePlot.get_title()
Get plot title
- ImagePlot.grabGesture()
QWidget.grabGesture(Qt.GestureType, Qt.GestureFlags flags=Qt.GestureFlags(0))
- ImagePlot.grabKeyboard()
QWidget.grabKeyboard()
- ImagePlot.grabMouse()
QWidget.grabMouse() QWidget.grabMouse(QCursor)
- ImagePlot.grabShortcut()
QWidget.grabShortcut(QKeySequence, Qt.ShortcutContext context=Qt.WindowShortcut) -> int
- ImagePlot.graphicsEffect()
QWidget.graphicsEffect() -> QGraphicsEffect
- ImagePlot.graphicsProxyWidget()
QWidget.graphicsProxyWidget() -> QGraphicsProxyWidget
- ImagePlot.hasFocus()
QWidget.hasFocus() -> bool
- ImagePlot.hasMouseTracking()
QWidget.hasMouseTracking() -> bool
- ImagePlot.height()
QWidget.height() -> int
- ImagePlot.heightForWidth()
QWidget.heightForWidth(int) -> int
- ImagePlot.heightMM()
QPaintDevice.heightMM() -> int
- ImagePlot.hide()
QWidget.hide()
- ImagePlot.hide_items(items=None, item_type=None)
Hide items (if items is None, hide all items)
- ImagePlot.inherits()
QObject.inherits(str) -> bool
- ImagePlot.inputContext()
QWidget.inputContext() -> QInputContext
- ImagePlot.inputMethodHints()
QWidget.inputMethodHints() -> Qt.InputMethodHints
- ImagePlot.inputMethodQuery()
QWidget.inputMethodQuery(Qt.InputMethodQuery) -> QVariant
- ImagePlot.insertAction()
QWidget.insertAction(QAction, QAction)
- ImagePlot.insertActions()
QWidget.insertActions(QAction, list-of-QAction)
- ImagePlot.installEventFilter()
QObject.installEventFilter(QObject)
- ImagePlot.invalidate()
Invalidate paint cache and schedule redraw use instead of replot when only the content of the canvas needs redrawing (axes, shouldn’t change)
- ImagePlot.isActiveWindow()
QWidget.isActiveWindow() -> bool
- ImagePlot.isAncestorOf()
QWidget.isAncestorOf(QWidget) -> bool
- ImagePlot.isEnabled()
QWidget.isEnabled() -> bool
- ImagePlot.isEnabledTo()
QWidget.isEnabledTo(QWidget) -> bool
- ImagePlot.isEnabledToTLW()
QWidget.isEnabledToTLW() -> bool
- ImagePlot.isFullScreen()
QWidget.isFullScreen() -> bool
- ImagePlot.isHidden()
QWidget.isHidden() -> bool
- ImagePlot.isLeftToRight()
QWidget.isLeftToRight() -> bool
- ImagePlot.isMaximized()
QWidget.isMaximized() -> bool
- ImagePlot.isMinimized()
QWidget.isMinimized() -> bool
- ImagePlot.isModal()
QWidget.isModal() -> bool
- ImagePlot.isRightToLeft()
QWidget.isRightToLeft() -> bool
- ImagePlot.isTopLevel()
QWidget.isTopLevel() -> bool
- ImagePlot.isVisible()
QWidget.isVisible() -> bool
- ImagePlot.isVisibleTo()
QWidget.isVisibleTo(QWidget) -> bool
- ImagePlot.isWidgetType()
QObject.isWidgetType() -> bool
- ImagePlot.isWindow()
QWidget.isWindow() -> bool
- ImagePlot.isWindowModified()
QWidget.isWindowModified() -> bool
- ImagePlot.keyboardGrabber()
QWidget.keyboardGrabber() -> QWidget
- ImagePlot.killTimer()
QObject.killTimer(int)
- ImagePlot.layout()
QWidget.layout() -> QLayout
- ImagePlot.layoutDirection()
QWidget.layoutDirection() -> Qt.LayoutDirection
- ImagePlot.lineWidth()
QFrame.lineWidth() -> int
- ImagePlot.locale()
QWidget.locale() -> QLocale
- ImagePlot.logicalDpiX()
QPaintDevice.logicalDpiX() -> int
- ImagePlot.logicalDpiY()
QPaintDevice.logicalDpiY() -> int
- ImagePlot.lower()
QWidget.lower()
- ImagePlot.mapFrom()
QWidget.mapFrom(QWidget, QPoint) -> QPoint
- ImagePlot.mapFromGlobal()
QWidget.mapFromGlobal(QPoint) -> QPoint
- ImagePlot.mapFromParent()
QWidget.mapFromParent(QPoint) -> QPoint
- ImagePlot.mapTo()
QWidget.mapTo(QWidget, QPoint) -> QPoint
- ImagePlot.mapToGlobal()
QWidget.mapToGlobal(QPoint) -> QPoint
- ImagePlot.mapToParent()
QWidget.mapToParent(QPoint) -> QPoint
- ImagePlot.mask()
QWidget.mask() -> QRegion
- ImagePlot.maximumHeight()
QWidget.maximumHeight() -> int
- ImagePlot.maximumSize()
QWidget.maximumSize() -> QSize
- ImagePlot.maximumWidth()
QWidget.maximumWidth() -> int
- ImagePlot.metaObject()
QObject.metaObject() -> QMetaObject
- ImagePlot.midLineWidth()
QFrame.midLineWidth() -> int
- ImagePlot.minimumHeight()
QWidget.minimumHeight() -> int
- ImagePlot.minimumSize()
QWidget.minimumSize() -> QSize
- ImagePlot.minimumWidth()
QWidget.minimumWidth() -> int
- ImagePlot.mouseDoubleClickEvent(event)
Reimplement QWidget method
- ImagePlot.mouseGrabber()
QWidget.mouseGrabber() -> QWidget
- ImagePlot.move()
QWidget.move(QPoint) QWidget.move(int, int)
- ImagePlot.moveToThread()
QObject.moveToThread(QThread)
- ImagePlot.move_down(item_list)
Move item(s) down, i.e. to the background (swap item with the previous item in z-order)
item: plot item or list of plot items
Return True if items have been moved effectively
- ImagePlot.move_up(item_list)
Move item(s) up, i.e. to the foreground (swap item with the next item in z-order)
item: plot item or list of plot items
Return True if items have been moved effectively
- ImagePlot.nativeParentWidget()
QWidget.nativeParentWidget() -> QWidget
- ImagePlot.nextInFocusChain()
QWidget.nextInFocusChain() -> QWidget
- ImagePlot.normalGeometry()
QWidget.normalGeometry() -> QRect
- ImagePlot.notify_colormap_changed()[source]
Levels histogram range has changed
- ImagePlot.numColors()
QPaintDevice.numColors() -> int
- ImagePlot.objectName()
QObject.objectName() -> QString
- ImagePlot.overrideWindowFlags()
QWidget.overrideWindowFlags(Qt.WindowFlags)
- ImagePlot.overrideWindowState()
QWidget.overrideWindowState(Qt.WindowStates)
- ImagePlot.paintEngine()
QWidget.paintEngine() -> QPaintEngine
- ImagePlot.paintingActive()
QPaintDevice.paintingActive() -> bool
- ImagePlot.palette()
QWidget.palette() -> QPalette
- ImagePlot.parent()
QObject.parent() -> QObject
- ImagePlot.parentWidget()
QWidget.parentWidget() -> QWidget
- ImagePlot.physicalDpiX()
QPaintDevice.physicalDpiX() -> int
- ImagePlot.physicalDpiY()
QPaintDevice.physicalDpiY() -> int
- ImagePlot.pos()
QWidget.pos() -> QPoint
- ImagePlot.previousInFocusChain()
QWidget.previousInFocusChain() -> QWidget
- ImagePlot.property()
QObject.property(str) -> QVariant
- ImagePlot.pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- ImagePlot.raise_()
QWidget.raise_()
- ImagePlot.read_axes_styles(section, options)
Read axes styles from section and options (one option for each axis in the order left, right, bottom, top)
Skip axis if option is None
- ImagePlot.rect()
QWidget.rect() -> QRect
- ImagePlot.releaseKeyboard()
QWidget.releaseKeyboard()
- ImagePlot.releaseMouse()
QWidget.releaseMouse()
- ImagePlot.releaseShortcut()
QWidget.releaseShortcut(int)
- ImagePlot.removeAction()
QWidget.removeAction(QAction)
- ImagePlot.removeEventFilter()
QObject.removeEventFilter(QObject)
- ImagePlot.render()
QWidget.render(QPaintDevice, QPoint targetOffset=QPoint(), QRegion sourceRegion=QRegion(), QWidget.RenderFlags flags=QWidget.DrawWindowBackground|QWidget.DrawChildren) QWidget.render(QPainter, QPoint targetOffset=QPoint(), QRegion sourceRegion=QRegion(), QWidget.RenderFlags flags=QWidget.DrawWindowBackground|QWidget.DrawChildren)
- ImagePlot.repaint()
QWidget.repaint() QWidget.repaint(int, int, int, int) QWidget.repaint(QRect) QWidget.repaint(QRegion)
- ImagePlot.resize()
QWidget.resize(QSize) QWidget.resize(int, int)
- ImagePlot.resizeEvent(event)[source]
Reimplement Qt method to resize widget
- ImagePlot.restoreGeometry()
QWidget.restoreGeometry(QByteArray) -> bool
- ImagePlot.restore_items(iofile)
- Restore items from file using the pickle protocol
- iofile: file object or filename
- ImagePlot.saveGeometry()
QWidget.saveGeometry() -> QByteArray
- ImagePlot.save_items(iofile, selected=False)
- Save (serializable) items to file using the pickle protocol
- iofile: file object or filename
- selected=False: if True, will save only selected items
- ImagePlot.scroll()
QWidget.scroll(int, int) QWidget.scroll(int, int, QRect)
- ImagePlot.select_all()
Select all selectable items
- ImagePlot.select_item(item)
Select item
- ImagePlot.select_some_items(items)
Select items
- ImagePlot.serialize(writer, selected=False)
- Save (serializable) items to HDF5 file:
- writer: guidata.hdf5io.HDF5Writer object
- selected=False: if True, will save only selected items
See also guiqwt.baseplot.BasePlot.restore_items_from_hdf5()
- ImagePlot.setAcceptDrops()
QWidget.setAcceptDrops(bool)
- ImagePlot.setAttribute()
QWidget.setAttribute(Qt.WidgetAttribute, bool on=True)
- ImagePlot.setAutoFillBackground()
QWidget.setAutoFillBackground(bool)
- ImagePlot.setBackgroundRole()
QWidget.setBackgroundRole(QPalette.ColorRole)
- ImagePlot.setBaseSize()
QWidget.setBaseSize(int, int) QWidget.setBaseSize(QSize)
- ImagePlot.setContentsMargins()
QWidget.setContentsMargins(int, int, int, int) QWidget.setContentsMargins(QMargins)
- ImagePlot.setContextMenuPolicy()
QWidget.setContextMenuPolicy(Qt.ContextMenuPolicy)
- ImagePlot.setCursor()
QWidget.setCursor(QCursor)
- ImagePlot.setDisabled()
QWidget.setDisabled(bool)
- ImagePlot.setEnabled()
QWidget.setEnabled(bool)
- ImagePlot.setFixedHeight()
QWidget.setFixedHeight(int)
- ImagePlot.setFixedSize()
QWidget.setFixedSize(QSize) QWidget.setFixedSize(int, int)
- ImagePlot.setFixedWidth()
QWidget.setFixedWidth(int)
- ImagePlot.setFocus()
QWidget.setFocus() QWidget.setFocus(Qt.FocusReason)
- ImagePlot.setFocusPolicy()
QWidget.setFocusPolicy(Qt.FocusPolicy)
- ImagePlot.setFocusProxy()
QWidget.setFocusProxy(QWidget)
- ImagePlot.setFont()
QWidget.setFont(QFont)
- ImagePlot.setForegroundRole()
QWidget.setForegroundRole(QPalette.ColorRole)
- ImagePlot.setFrameRect()
QFrame.setFrameRect(QRect)
- ImagePlot.setFrameShadow()
QFrame.setFrameShadow(QFrame.Shadow)
- ImagePlot.setFrameShape()
QFrame.setFrameShape(QFrame.Shape)
- ImagePlot.setFrameStyle()
QFrame.setFrameStyle(int)
- ImagePlot.setGeometry()
QWidget.setGeometry(QRect) QWidget.setGeometry(int, int, int, int)
- ImagePlot.setGraphicsEffect()
QWidget.setGraphicsEffect(QGraphicsEffect)
- ImagePlot.setHidden()
QWidget.setHidden(bool)
- ImagePlot.setInputContext()
QWidget.setInputContext(QInputContext)
- ImagePlot.setInputMethodHints()
QWidget.setInputMethodHints(Qt.InputMethodHints)
- ImagePlot.setLayout()
QWidget.setLayout(QLayout)
- ImagePlot.setLayoutDirection()
QWidget.setLayoutDirection(Qt.LayoutDirection)
- ImagePlot.setLineWidth()
QFrame.setLineWidth(int)
- ImagePlot.setLocale()
QWidget.setLocale(QLocale)
- ImagePlot.setMask()
QWidget.setMask(QBitmap) QWidget.setMask(QRegion)
- ImagePlot.setMaximumHeight()
QWidget.setMaximumHeight(int)
- ImagePlot.setMaximumSize()
QWidget.setMaximumSize(int, int) QWidget.setMaximumSize(QSize)
- ImagePlot.setMaximumWidth()
QWidget.setMaximumWidth(int)
- ImagePlot.setMidLineWidth()
QFrame.setMidLineWidth(int)
- ImagePlot.setMinimumHeight()
QWidget.setMinimumHeight(int)
- ImagePlot.setMinimumSize()
QWidget.setMinimumSize(int, int) QWidget.setMinimumSize(QSize)
- ImagePlot.setMinimumWidth()
QWidget.setMinimumWidth(int)
- ImagePlot.setMouseTracking()
QWidget.setMouseTracking(bool)
- ImagePlot.setObjectName()
QObject.setObjectName(QString)
- ImagePlot.setPalette()
QWidget.setPalette(QPalette)
- ImagePlot.setParent()
QWidget.setParent(QWidget) QWidget.setParent(QWidget, Qt.WindowFlags)
- ImagePlot.setProperty()
QObject.setProperty(str, QVariant) -> bool
- ImagePlot.setShortcutAutoRepeat()
QWidget.setShortcutAutoRepeat(int, bool enabled=True)
- ImagePlot.setShortcutEnabled()
QWidget.setShortcutEnabled(int, bool enabled=True)
- ImagePlot.setShown()
QWidget.setShown(bool)
- ImagePlot.setSizeIncrement()
QWidget.setSizeIncrement(int, int) QWidget.setSizeIncrement(QSize)
- ImagePlot.setSizePolicy()
QWidget.setSizePolicy(QSizePolicy) QWidget.setSizePolicy(QSizePolicy.Policy, QSizePolicy.Policy)
- ImagePlot.setStatusTip()
QWidget.setStatusTip(QString)
- ImagePlot.setStyle()
QWidget.setStyle(QStyle)
- ImagePlot.setStyleSheet()
QWidget.setStyleSheet(QString)
- ImagePlot.setTabOrder()
QWidget.setTabOrder(QWidget, QWidget)
- ImagePlot.setToolTip()
QWidget.setToolTip(QString)
- ImagePlot.setUpdatesEnabled()
QWidget.setUpdatesEnabled(bool)
- ImagePlot.setVisible()
QWidget.setVisible(bool)
- ImagePlot.setWhatsThis()
QWidget.setWhatsThis(QString)
- ImagePlot.setWindowFilePath()
QWidget.setWindowFilePath(QString)
- ImagePlot.setWindowFlags()
QWidget.setWindowFlags(Qt.WindowFlags)
- ImagePlot.setWindowIcon()
QWidget.setWindowIcon(QIcon)
- ImagePlot.setWindowIconText()
QWidget.setWindowIconText(QString)
- ImagePlot.setWindowModality()
QWidget.setWindowModality(Qt.WindowModality)
- ImagePlot.setWindowModified()
QWidget.setWindowModified(bool)
- ImagePlot.setWindowOpacity()
QWidget.setWindowOpacity(float)
- ImagePlot.setWindowRole()
QWidget.setWindowRole(QString)
- ImagePlot.setWindowState()
QWidget.setWindowState(Qt.WindowStates)
- ImagePlot.setWindowTitle()
QWidget.setWindowTitle(QString)
- ImagePlot.set_active_item(item)
Override base set_active_item to change the grid’s axes according to the selected item
- ImagePlot.set_antialiasing(checked)
Toggle curve antialiasing
- ImagePlot.set_aspect_ratio(ratio=None, lock=None)[source]
Set aspect ratio
- ImagePlot.set_axis_color(axis_id, color)
Set axis color color: color name (string) or QColor instance
- ImagePlot.set_axis_direction(axis_id, reverse=False)
- Set axis direction of increasing values
axis_id: axis id (BasePlot.Y_LEFT, BasePlot.X_BOTTOM, ...) or string: ‘bottom’, ‘left’, ‘top’ or ‘right’
- reverse: False (default)
- x-axis values increase from left to right
- y-axis values increase from bottom to top
- reverse: True
- x-axis values increase from right to left
- y-axis values increase from top to bottom
- ImagePlot.set_axis_font(axis_id, font)
Set axis font
- ImagePlot.set_axis_limits(axis_id, vmin, vmax, stepsize=0)
Set axis limits (minimum and maximum values)
- ImagePlot.set_axis_scale(axis_id, scale, autoscale=True)
Set axis scale Example: self.set_axis_scale(curve.yAxis(), ‘lin’)
- ImagePlot.set_axis_ticks(axis_id, nmajor=None, nminor=None)
Set axis maximum number of major ticks and maximum of minor ticks
- ImagePlot.set_axis_title(axis_id, text)
Set axis title
- ImagePlot.set_axis_unit(axis_id, text)
Set axis unit
- ImagePlot.set_item_visible(item, state, notify=True, replot=True)
Show/hide item and emit a SIG_ITEMS_CHANGED signal
- ImagePlot.set_items(*args)
Utility function used to quickly setup a plot with a set of items
- ImagePlot.set_items_readonly(state)
Set all items readonly state to state Default item’s readonly state: False (items may be deleted)
- ImagePlot.set_manager(manager, plot_id)
Set the associated guiqwt.plot.PlotManager instance
- ImagePlot.set_plot_limits(x0, x1, y0, y1, xaxis='bottom', yaxis='left')
Set plot scale limits
- ImagePlot.set_pointer(pointer_type)
Set pointer. Valid values of pointer_type:
- None: disable pointer
- “canvas”: enable canvas pointer
- “curve”: enable on-curve pointer
- ImagePlot.set_scales(xscale, yscale)
Set active curve scales Example: self.set_scales(‘lin’, ‘lin’)
- ImagePlot.set_title(title)
Set plot title
- ImagePlot.set_titles(title=None, xlabel=None, ylabel=None, xunit=None, yunit=None)
- Set plot and axes titles at once
- title: plot title
- xlabel: (bottom axis title, top axis title) or bottom axis title only
- ylabel: (left axis title, right axis title) or left axis title only
- xunit: (bottom axis unit, top axis unit) or bottom axis unit only
- yunit: (left axis unit, right axis unit) or left axis unit only
- ImagePlot.show()
QWidget.show()
- ImagePlot.showEvent(event)[source]
Override BasePlot method
- ImagePlot.showFullScreen()
QWidget.showFullScreen()
- ImagePlot.showMaximized()
QWidget.showMaximized()
- ImagePlot.showMinimized()
QWidget.showMinimized()
- ImagePlot.showNormal()
QWidget.showNormal()
- ImagePlot.show_items(items=None, item_type=None)
Show items (if items is None, show all items)
- ImagePlot.signalsBlocked()
QObject.signalsBlocked() -> bool
- ImagePlot.size()
QWidget.size() -> QSize
- ImagePlot.sizeHint()
Preferred size
- ImagePlot.sizeIncrement()
QWidget.sizeIncrement() -> QSize
- ImagePlot.sizePolicy()
QWidget.sizePolicy() -> QSizePolicy
- ImagePlot.stackUnder()
QWidget.stackUnder(QWidget)
- ImagePlot.startTimer()
QObject.startTimer(int) -> int
- ImagePlot.statusTip()
QWidget.statusTip() -> QString
- ImagePlot.style()
QWidget.style() -> QStyle
- ImagePlot.styleSheet()
QWidget.styleSheet() -> QString
- ImagePlot.testAttribute()
QWidget.testAttribute(Qt.WidgetAttribute) -> bool
- ImagePlot.thread()
QObject.thread() -> QThread
- ImagePlot.toolTip()
QWidget.toolTip() -> QString
- ImagePlot.topLevelWidget()
QWidget.topLevelWidget() -> QWidget
- ImagePlot.tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- ImagePlot.trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- ImagePlot.underMouse()
QWidget.underMouse() -> bool
- ImagePlot.ungrabGesture()
QWidget.ungrabGesture(Qt.GestureType)
- ImagePlot.unselect_all()
Unselect all selected items
- ImagePlot.unselect_item(item)
Unselect item
- ImagePlot.unsetCursor()
QWidget.unsetCursor()
- ImagePlot.unsetLayoutDirection()
QWidget.unsetLayoutDirection()
- ImagePlot.unsetLocale()
QWidget.unsetLocale()
- ImagePlot.update()
QWidget.update() QWidget.update(QRect) QWidget.update(QRegion) QWidget.update(int, int, int, int)
- ImagePlot.updateGeometry()
QWidget.updateGeometry()
- ImagePlot.update_all_axes_styles()
Update all axes styles
- ImagePlot.update_axis_style(axis_id)
Update axis style
- ImagePlot.update_lut_range(_min, _max)[source]
update the LUT scale
- ImagePlot.updatesEnabled()
QWidget.updatesEnabled() -> bool
- ImagePlot.visibleRegion()
QWidget.visibleRegion() -> QRegion
- ImagePlot.whatsThis()
QWidget.whatsThis() -> QString
- ImagePlot.width()
QWidget.width() -> int
- ImagePlot.widthMM()
QPaintDevice.widthMM() -> int
- ImagePlot.winId()
QWidget.winId() -> sip.voidptr
- ImagePlot.window()
QWidget.window() -> QWidget
- ImagePlot.windowFilePath()
QWidget.windowFilePath() -> QString
- ImagePlot.windowFlags()
QWidget.windowFlags() -> Qt.WindowFlags
- ImagePlot.windowIcon()
QWidget.windowIcon() -> QIcon
- ImagePlot.windowIconText()
QWidget.windowIconText() -> QString
- ImagePlot.windowModality()
QWidget.windowModality() -> Qt.WindowModality
- ImagePlot.windowOpacity()
QWidget.windowOpacity() -> float
- ImagePlot.windowRole()
QWidget.windowRole() -> QString
- ImagePlot.windowState()
QWidget.windowState() -> Qt.WindowStates
- ImagePlot.windowTitle()
QWidget.windowTitle() -> QString
- ImagePlot.windowType()
QWidget.windowType() -> Qt.WindowType
- ImagePlot.x()
QWidget.x() -> int
- ImagePlot.y()
QWidget.y() -> int
- class guiqwt.image.BaseImageItem(data=None, param=None)[source]
- align_rectangular_shape(shape)[source]
Align rectangular shape to image pixels
- draw_border(painter, xMap, yMap, canvasRect)[source]
Draw image border rectangle
- draw_image(painter, canvasRect, src_rect, dst_rect, xMap, yMap)[source]
Draw image with painter on canvasRect <!> src_rect and dst_rect are coord tuples (xleft, ytop, xright, ybottom)
- export_roi(src_rect, dst_rect, dst_image, apply_lut=False, apply_interpolation=False, original_resolution=False)[source]
Export Region Of Interest to array
- get_average_xsection(x0, y0, x1, y1, apply_lut=False)[source]
Return average cross section along x-axis
- get_average_ysection(x0, y0, x1, y1, apply_lut=False)[source]
Return average cross section along y-axis
- get_closest_coordinates(x, y)[source]
Return closest image pixel coordinates
- get_closest_index_rect(x0, y0, x1, y1)[source]
Return closest image rectangular pixel area index bounds Avoid returning empty rectangular area (return 1x1 pixel area instead) Handle reversed/not-reversed Y-axis orientation
- get_closest_indexes(x, y, corner=None)[source]
Return closest image pixel indexes corner: None (not a corner), ‘TL’ (top-left corner), ‘BR’ (bottom-right corner)
- get_closest_pixel_indexes(x, y)[source]
Return closest pixel indexes Instead of returning indexes of an image pixel like the method ‘get_closest_indexes’, this method returns the indexes of the closest pixel which is not necessarily on the image itself (i.e. indexes may be outside image index bounds: negative or superior than the image dimension)
Note: this is not the same as retrieving the canvas pixel coordinates (which depends on the zoom level)
- get_data(x0, y0, x1=None, y1=None)[source]
Return image data Arguments:
x0, y0 [, x1, y1]Return image level at coordinates (x0,y0) If x1,y1 are specified:
return image levels (np.ndarray) in rectangular area (x0,y0,x1,y1)
- get_default_param()[source]
Return instance of the default imageparam DataSet
- get_filter(filterobj, filterparam)[source]
Provides a filter object over this image’s content
- get_histogram(nbins)[source]
interface de IHistDataSource
- get_interpolation()[source]
Get interpolation mode
- get_lut_range()[source]
Return the LUT transform range tuple: (min, max)
- get_lut_range_full()[source]
Return full dynamic range
- get_lut_range_max()[source]
Get maximum range for this dataset
- get_pixel_coordinates(xplot, yplot)[source]
Return (image) pixel coordinates Transform the plot coordinates (arbitrary plot Z-axis unit) into the image coordinates (pixel unit)
Rounding is necessary to obtain array indexes from these coordinates
- get_plot_coordinates(xpixel, ypixel)[source]
Return plot coordinates Transform the image coordinates (pixel unit) into the plot coordinates (arbitrary plot Z-axis unit)
- get_stats(x0, y0, x1, y1)[source]
Return formatted string with stats on image rectangular area (output should be compatible with AnnotatedShape.get_infos)
- get_xsection(y0, apply_lut=False)[source]
Return cross section along x-axis at y=y0
- get_ysection(x0, apply_lut=False)[source]
Return cross section along y-axis at x=x0
- is_empty()[source]
Return True if item data is empty
- is_private()[source]
Return True if object is private
- is_readonly()[source]
Return object readonly state
- move_local_point_to(handle, pos, ctrl=None)[source]
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_local_shape(old_pos, new_pos)[source]
Translate the shape such that old_pos becomes new_pos in canvas coordinates
- move_with_selection(delta_x, delta_y)[source]
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()[source]
Select item
- set_interpolation(interp_mode, size=None)[source]
Set image interpolation mode
interp_mode: INTERP_NEAREST, INTERP_LINEAR, INTERP_AA size (integer): (for anti-aliasing only) AA matrix size
- set_lut_range(lut_range)[source]
Set LUT transform range lut_range is a tuple: (min, max)
- set_movable(state)[source]
Set item movable state
- set_private(state)[source]
Set object as private
- set_readonly(state)[source]
Set object readonly state
- set_resizable(state)[source]
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)[source]
Set item rotatable state
- set_selectable(state)[source]
Set item selectable state
- unselect()[source]
Unselect item
- update_border()[source]
Update image border rectangle to fit image shape
- class guiqwt.image.RawImageItem(data=None, param=None)[source]
- Construct a simple image item
- data: 2D NumPy array
- param (optional): image parameters (guiqwt.styles.RawImageParam instance)
- align_rectangular_shape(shape)
Align rectangular shape to image pixels
- deserialize(reader)[source]
Deserialize object from HDF5 reader
- draw_border(painter, xMap, yMap, canvasRect)
Draw image border rectangle
- draw_image(painter, canvasRect, src_rect, dst_rect, xMap, yMap)
Draw image with painter on canvasRect <!> src_rect and dst_rect are coord tuples (xleft, ytop, xright, ybottom)
- export_roi(src_rect, dst_rect, dst_image, apply_lut=False, apply_interpolation=False, original_resolution=False)
Export Region Of Interest to array
- get_average_xsection(x0, y0, x1, y1, apply_lut=False)
Return average cross section along x-axis
- get_average_ysection(x0, y0, x1, y1, apply_lut=False)
Return average cross section along y-axis
- get_closest_coordinates(x, y)
Return closest image pixel coordinates
- get_closest_index_rect(x0, y0, x1, y1)
Return closest image rectangular pixel area index bounds Avoid returning empty rectangular area (return 1x1 pixel area instead) Handle reversed/not-reversed Y-axis orientation
- get_closest_indexes(x, y, corner=None)
Return closest image pixel indexes corner: None (not a corner), ‘TL’ (top-left corner), ‘BR’ (bottom-right corner)
- get_closest_pixel_indexes(x, y)
Return closest pixel indexes Instead of returning indexes of an image pixel like the method ‘get_closest_indexes’, this method returns the indexes of the closest pixel which is not necessarily on the image itself (i.e. indexes may be outside image index bounds: negative or superior than the image dimension)
Note: this is not the same as retrieving the canvas pixel coordinates (which depends on the zoom level)
- get_data(x0, y0, x1=None, y1=None)
Return image data Arguments:
x0, y0 [, x1, y1]Return image level at coordinates (x0,y0) If x1,y1 are specified:
return image levels (np.ndarray) in rectangular area (x0,y0,x1,y1)
- get_default_param()[source]
Return instance of the default imageparam DataSet
- get_filter(filterobj, filterparam)
Provides a filter object over this image’s content
- get_histogram(nbins)
interface de IHistDataSource
- get_interpolation()
Get interpolation mode
- get_lut_range()
Return the LUT transform range tuple: (min, max)
- get_lut_range_full()
Return full dynamic range
- get_lut_range_max()
Get maximum range for this dataset
- get_pixel_coordinates(xplot, yplot)
Return (image) pixel coordinates Transform the plot coordinates (arbitrary plot Z-axis unit) into the image coordinates (pixel unit)
Rounding is necessary to obtain array indexes from these coordinates
- get_plot_coordinates(xpixel, ypixel)
Return plot coordinates Transform the image coordinates (pixel unit) into the plot coordinates (arbitrary plot Z-axis unit)
- get_stats(x0, y0, x1, y1)
Return formatted string with stats on image rectangular area (output should be compatible with AnnotatedShape.get_infos)
- get_xsection(y0, apply_lut=False)
Return cross section along x-axis at y=y0
- get_ysection(x0, apply_lut=False)
Return cross section along y-axis at x=x0
- is_empty()
Return True if item data is empty
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- load_data(lut_range=None)[source]
Load data from filename and eventually apply specified lut_range filename has been set using method ‘set_filename’
- move_local_point_to(handle, pos, ctrl=None)
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_local_shape(old_pos, new_pos)
Translate the shape such that old_pos becomes new_pos in canvas coordinates
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- serialize(writer)[source]
Serialize object to HDF5 writer
- set_data(data, lut_range=None)[source]
- Set Image item data
- data: 2D NumPy array
- lut_range: LUT range – tuple (levelmin, levelmax)
- set_interpolation(interp_mode, size=None)
Set image interpolation mode
interp_mode: INTERP_NEAREST, INTERP_LINEAR, INTERP_AA size (integer): (for anti-aliasing only) AA matrix size
- set_lut_range(lut_range)
Set LUT transform range lut_range is a tuple: (min, max)
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- unselect()
Unselect item
- update_border()
Update image border rectangle to fit image shape
- class guiqwt.image.ImageItem(data=None, param=None)[source]
- Construct a simple image item
- data: 2D NumPy array
- param (optional): image parameters (guiqwt.styles.ImageParam instance)
- align_rectangular_shape(shape)
Align rectangular shape to image pixels
- deserialize(reader)[source]
Deserialize object from HDF5 reader
- draw_border(painter, xMap, yMap, canvasRect)
Draw image border rectangle
- export_roi(src_rect, dst_rect, dst_image, apply_lut=False, apply_interpolation=False, original_resolution=False)[source]
Export Region Of Interest to array
- get_average_xsection(x0, y0, x1, y1, apply_lut=False)
Return average cross section along x-axis
- get_average_ysection(x0, y0, x1, y1, apply_lut=False)
Return average cross section along y-axis
- get_closest_coordinates(x, y)[source]
Return closest image pixel coordinates
- get_closest_index_rect(x0, y0, x1, y1)
Return closest image rectangular pixel area index bounds Avoid returning empty rectangular area (return 1x1 pixel area instead) Handle reversed/not-reversed Y-axis orientation
- get_closest_indexes(x, y, corner=None)
Return closest image pixel indexes corner: None (not a corner), ‘TL’ (top-left corner), ‘BR’ (bottom-right corner)
- get_closest_pixel_indexes(x, y)
Return closest pixel indexes Instead of returning indexes of an image pixel like the method ‘get_closest_indexes’, this method returns the indexes of the closest pixel which is not necessarily on the image itself (i.e. indexes may be outside image index bounds: negative or superior than the image dimension)
Note: this is not the same as retrieving the canvas pixel coordinates (which depends on the zoom level)
- get_data(x0, y0, x1=None, y1=None)
Return image data Arguments:
x0, y0 [, x1, y1]Return image level at coordinates (x0,y0) If x1,y1 are specified:
return image levels (np.ndarray) in rectangular area (x0,y0,x1,y1)
- get_default_param()[source]
Return instance of the default imageparam DataSet
- get_filter(filterobj, filterparam)
Provides a filter object over this image’s content
- get_histogram(nbins)
interface de IHistDataSource
- get_interpolation()
Get interpolation mode
- get_lut_range()
Return the LUT transform range tuple: (min, max)
- get_lut_range_full()
Return full dynamic range
- get_lut_range_max()
Get maximum range for this dataset
- get_pixel_coordinates(xplot, yplot)[source]
Return (image) pixel coordinates (from plot coordinates)
- get_plot_coordinates(xpixel, ypixel)[source]
Return plot coordinates (from image pixel coordinates)
- get_stats(x0, y0, x1, y1)
Return formatted string with stats on image rectangular area (output should be compatible with AnnotatedShape.get_infos)
- get_xdata()[source]
Return (xmin, xmax)
- get_xsection(y0, apply_lut=False)
Return cross section along x-axis at y=y0
- get_ydata()[source]
Return (ymin, ymax)
- get_ysection(x0, apply_lut=False)
Return cross section along y-axis at x=x0
- is_empty()
Return True if item data is empty
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- load_data(lut_range=None)
Load data from filename and eventually apply specified lut_range filename has been set using method ‘set_filename’
- move_local_point_to(handle, pos, ctrl=None)
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_local_shape(old_pos, new_pos)
Translate the shape such that old_pos becomes new_pos in canvas coordinates
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- serialize(writer)[source]
Serialize object to HDF5 writer
- set_data(data, lut_range=None)
- Set Image item data
- data: 2D NumPy array
- lut_range: LUT range – tuple (levelmin, levelmax)
- set_interpolation(interp_mode, size=None)
Set image interpolation mode
interp_mode: INTERP_NEAREST, INTERP_LINEAR, INTERP_AA size (integer): (for anti-aliasing only) AA matrix size
- set_lut_range(lut_range)
Set LUT transform range lut_range is a tuple: (min, max)
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- unselect()
Unselect item
- update_border()
Update image border rectangle to fit image shape
- class guiqwt.image.TrImageItem(data=None, param=None)[source]
- Construct a transformable image item
- data: 2D NumPy array
- param (optional): image parameters (guiqwt.styles.TrImageParam instance)
- align_rectangular_shape(shape)
Align rectangular shape to image pixels
- deserialize(reader)
Deserialize object from HDF5 reader
- export_roi(src_rect, dst_rect, dst_image, apply_lut=False, apply_interpolation=False, original_resolution=False)[source]
Export Region Of Interest to array
- get_average_xsection(x0, y0, x1, y1, apply_lut=False)
Return average cross section along x-axis
- get_average_ysection(x0, y0, x1, y1, apply_lut=False)
Return average cross section along y-axis
- get_closest_coordinates(x, y)[source]
Return closest image pixel coordinates
- get_closest_index_rect(x0, y0, x1, y1)
Return closest image rectangular pixel area index bounds Avoid returning empty rectangular area (return 1x1 pixel area instead) Handle reversed/not-reversed Y-axis orientation
- get_closest_indexes(x, y, corner=None)
Return closest image pixel indexes corner: None (not a corner), ‘TL’ (top-left corner), ‘BR’ (bottom-right corner)
- get_closest_pixel_indexes(x, y)
Return closest pixel indexes Instead of returning indexes of an image pixel like the method ‘get_closest_indexes’, this method returns the indexes of the closest pixel which is not necessarily on the image itself (i.e. indexes may be outside image index bounds: negative or superior than the image dimension)
Note: this is not the same as retrieving the canvas pixel coordinates (which depends on the zoom level)
- get_crop_coordinates()[source]
Return crop rectangle coordinates
- get_data(x0, y0, x1=None, y1=None)
Return image data Arguments:
x0, y0 [, x1, y1]Return image level at coordinates (x0,y0) If x1,y1 are specified:
return image levels (np.ndarray) in rectangular area (x0,y0,x1,y1)
- get_default_param()[source]
Return instance of the default imageparam DataSet
- get_filter(filterobj, filterparam)[source]
Provides a filter object over this image’s content
- get_histogram(nbins)
interface de IHistDataSource
- get_interpolation()
Get interpolation mode
- get_lut_range()
Return the LUT transform range tuple: (min, max)
- get_lut_range_full()
Return full dynamic range
- get_lut_range_max()
Get maximum range for this dataset
- get_pixel_coordinates(xplot, yplot)[source]
Return (image) pixel coordinates (from plot coordinates)
- get_plot_coordinates(xpixel, ypixel)[source]
Return plot coordinates (from image pixel coordinates)
- get_stats(x0, y0, x1, y1)
Return formatted string with stats on image rectangular area (output should be compatible with AnnotatedShape.get_infos)
- get_xsection(y0, apply_lut=False)
Return cross section along x-axis at y=y0
- get_ysection(x0, apply_lut=False)
Return cross section along y-axis at x=x0
- is_empty()
Return True if item data is empty
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- load_data(lut_range=None)
Load data from filename and eventually apply specified lut_range filename has been set using method ‘set_filename’
- move_local_point_to(handle, pos, ctrl=None)[source]
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_local_shape(old_pos, new_pos)[source]
Translate the shape such that old_pos becomes new_pos in canvas coordinates
- move_with_selection(delta_x, delta_y)[source]
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- serialize(writer)
Serialize object to HDF5 writer
- set_interpolation(interp_mode, size=None)
Set image interpolation mode
interp_mode: INTERP_NEAREST, INTERP_LINEAR, INTERP_AA size (integer): (for anti-aliasing only) AA matrix size
- set_lut_range(lut_range)
Set LUT transform range lut_range is a tuple: (min, max)
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- unselect()
Unselect item
- class guiqwt.image.XYImageItem(x=None, y=None, data=None, param=None)[source]
- Construct an image item with non-linear X/Y axes
- x: 1D NumPy array, must be increasing
- y: 1D NumPy array, must be increasing
- data: 2D NumPy array
- param (optional): image parameters (guiqwt.styles.XYImageParam instance)
- align_rectangular_shape(shape)
Align rectangular shape to image pixels
- deserialize(reader)[source]
Deserialize object from HDF5 reader
- draw_border(painter, xMap, yMap, canvasRect)
Draw image border rectangle
- export_roi(src_rect, dst_rect, dst_image, apply_lut=False, apply_interpolation=False, original_resolution=False)
Export Region Of Interest to array
- get_average_xsection(x0, y0, x1, y1, apply_lut=False)
Return average cross section along x-axis
- get_average_ysection(x0, y0, x1, y1, apply_lut=False)
Return average cross section along y-axis
- get_closest_coordinates(x, y)[source]
Return closest image pixel coordinates
- get_closest_index_rect(x0, y0, x1, y1)
Return closest image rectangular pixel area index bounds Avoid returning empty rectangular area (return 1x1 pixel area instead) Handle reversed/not-reversed Y-axis orientation
- get_closest_indexes(x, y, corner=None)
Return closest image pixel indexes corner: None (not a corner), ‘TL’ (top-left corner), ‘BR’ (bottom-right corner)
- get_closest_pixel_indexes(x, y)
Return closest pixel indexes Instead of returning indexes of an image pixel like the method ‘get_closest_indexes’, this method returns the indexes of the closest pixel which is not necessarily on the image itself (i.e. indexes may be outside image index bounds: negative or superior than the image dimension)
Note: this is not the same as retrieving the canvas pixel coordinates (which depends on the zoom level)
- get_data(x0, y0, x1=None, y1=None)
Return image data Arguments:
x0, y0 [, x1, y1]Return image level at coordinates (x0,y0) If x1,y1 are specified:
return image levels (np.ndarray) in rectangular area (x0,y0,x1,y1)
- get_default_param()[source]
Return instance of the default imageparam DataSet
- get_filter(filterobj, filterparam)[source]
Provides a filter object over this image’s content
- get_histogram(nbins)
interface de IHistDataSource
- get_interpolation()
Get interpolation mode
- get_lut_range()
Return the LUT transform range tuple: (min, max)
- get_lut_range_full()
Return full dynamic range
- get_lut_range_max()
Get maximum range for this dataset
- get_pixel_coordinates(xplot, yplot)[source]
Return (image) pixel coordinates (from plot coordinates)
- get_plot_coordinates(xpixel, ypixel)[source]
Return plot coordinates (from image pixel coordinates)
- get_stats(x0, y0, x1, y1)
Return formatted string with stats on image rectangular area (output should be compatible with AnnotatedShape.get_infos)
- get_xsection(y0, apply_lut=False)
Return cross section along x-axis at y=y0
- get_ysection(x0, apply_lut=False)
Return cross section along y-axis at x=x0
- is_empty()
Return True if item data is empty
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- load_data(lut_range=None)
Load data from filename and eventually apply specified lut_range filename has been set using method ‘set_filename’
- move_local_point_to(handle, pos, ctrl=None)
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_local_shape(old_pos, new_pos)
Translate the shape such that old_pos becomes new_pos in canvas coordinates
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- serialize(writer)[source]
Serialize object to HDF5 writer
- set_data(data, lut_range=None)
- Set Image item data
- data: 2D NumPy array
- lut_range: LUT range – tuple (levelmin, levelmax)
- set_interpolation(interp_mode, size=None)
Set image interpolation mode
interp_mode: INTERP_NEAREST, INTERP_LINEAR, INTERP_AA size (integer): (for anti-aliasing only) AA matrix size
- set_lut_range(lut_range)
Set LUT transform range lut_range is a tuple: (min, max)
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- unselect()
Unselect item
- update_border()
Update image border rectangle to fit image shape
- class guiqwt.image.RGBImageItem(data=None, param=None)[source]
- Construct a RGB/RGBA image item
- data: NumPy array of uint8 (shape: NxMx[34] – 3: RGB, 4: RGBA)
(last dimension: 0:Red, 1:Green, 2:Blue[, 3:Alpha]) * param (optional): image parameters
(guiqwt.styles.RGBImageParam instance)
- align_rectangular_shape(shape)
Align rectangular shape to image pixels
- deserialize(reader)
Deserialize object from HDF5 reader
- draw_border(painter, xMap, yMap, canvasRect)
Draw image border rectangle
- export_roi(src_rect, dst_rect, dst_image, apply_lut=False, apply_interpolation=False, original_resolution=False)
Export Region Of Interest to array
- get_average_xsection(x0, y0, x1, y1, apply_lut=False)
Return average cross section along x-axis
- get_average_ysection(x0, y0, x1, y1, apply_lut=False)
Return average cross section along y-axis
- get_closest_coordinates(x, y)
Return closest image pixel coordinates
- get_closest_index_rect(x0, y0, x1, y1)
Return closest image rectangular pixel area index bounds Avoid returning empty rectangular area (return 1x1 pixel area instead) Handle reversed/not-reversed Y-axis orientation
- get_closest_indexes(x, y, corner=None)
Return closest image pixel indexes corner: None (not a corner), ‘TL’ (top-left corner), ‘BR’ (bottom-right corner)
- get_closest_pixel_indexes(x, y)
Return closest pixel indexes Instead of returning indexes of an image pixel like the method ‘get_closest_indexes’, this method returns the indexes of the closest pixel which is not necessarily on the image itself (i.e. indexes may be outside image index bounds: negative or superior than the image dimension)
Note: this is not the same as retrieving the canvas pixel coordinates (which depends on the zoom level)
- get_data(x0, y0, x1=None, y1=None)
Return image data Arguments:
x0, y0 [, x1, y1]Return image level at coordinates (x0,y0) If x1,y1 are specified:
return image levels (np.ndarray) in rectangular area (x0,y0,x1,y1)
- get_default_param()[source]
Return instance of the default imageparam DataSet
- get_filter(filterobj, filterparam)
Provides a filter object over this image’s content
- get_histogram(nbins)
interface de IHistDataSource
- get_interpolation()
Get interpolation mode
- get_lut_range()
Return the LUT transform range tuple: (min, max)
- get_lut_range_full()
Return full dynamic range
- get_lut_range_max()
Get maximum range for this dataset
- get_pixel_coordinates(xplot, yplot)
Return (image) pixel coordinates (from plot coordinates)
- get_plot_coordinates(xpixel, ypixel)
Return plot coordinates (from image pixel coordinates)
- get_stats(x0, y0, x1, y1)
Return formatted string with stats on image rectangular area (output should be compatible with AnnotatedShape.get_infos)
- get_xdata()
Return (xmin, xmax)
- get_xsection(y0, apply_lut=False)
Return cross section along x-axis at y=y0
- get_ydata()
Return (ymin, ymax)
- get_ysection(x0, apply_lut=False)
Return cross section along y-axis at x=x0
- is_empty()
Return True if item data is empty
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- load_data()[source]
Load data from filename filename has been set using method ‘set_filename’
- move_local_point_to(handle, pos, ctrl=None)
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_local_shape(old_pos, new_pos)
Translate the shape such that old_pos becomes new_pos in canvas coordinates
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- serialize(writer)
Serialize object to HDF5 writer
- set_interpolation(interp_mode, size=None)
Set image interpolation mode
interp_mode: INTERP_NEAREST, INTERP_LINEAR, INTERP_AA size (integer): (for anti-aliasing only) AA matrix size
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- unselect()
Unselect item
- update_border()
Update image border rectangle to fit image shape
- class guiqwt.image.MaskedImageItem(data=None, mask=None, param=None)[source]
- Construct a masked image item
- data: 2D NumPy array
- mask (optional): 2D NumPy array
- param (optional): image parameters (guiqwt.styles.MaskedImageParam instance)
- align_rectangular_shape(shape)
Align rectangular shape to image pixels
- apply_masked_areas()[source]
Apply masked areas
- deserialize(reader)[source]
Deserialize object from HDF5 reader
- draw_border(painter, xMap, yMap, canvasRect)
Draw image border rectangle
- export_roi(src_rect, dst_rect, dst_image, apply_lut=False, apply_interpolation=False, original_resolution=False)
Export Region Of Interest to array
- get_average_xsection(x0, y0, x1, y1, apply_lut=False)
Return average cross section along x-axis
- get_average_ysection(x0, y0, x1, y1, apply_lut=False)
Return average cross section along y-axis
- get_closest_coordinates(x, y)
Return closest image pixel coordinates
- get_closest_index_rect(x0, y0, x1, y1)
Return closest image rectangular pixel area index bounds Avoid returning empty rectangular area (return 1x1 pixel area instead) Handle reversed/not-reversed Y-axis orientation
- get_closest_indexes(x, y, corner=None)
Return closest image pixel indexes corner: None (not a corner), ‘TL’ (top-left corner), ‘BR’ (bottom-right corner)
- get_closest_pixel_indexes(x, y)
Return closest pixel indexes Instead of returning indexes of an image pixel like the method ‘get_closest_indexes’, this method returns the indexes of the closest pixel which is not necessarily on the image itself (i.e. indexes may be outside image index bounds: negative or superior than the image dimension)
Note: this is not the same as retrieving the canvas pixel coordinates (which depends on the zoom level)
- get_data(x0, y0, x1=None, y1=None)
Return image data Arguments:
x0, y0 [, x1, y1]Return image level at coordinates (x0,y0) If x1,y1 are specified:
return image levels (np.ndarray) in rectangular area (x0,y0,x1,y1)
- get_default_param()[source]
Return instance of the default imageparam DataSet
- get_filter(filterobj, filterparam)
Provides a filter object over this image’s content
- get_histogram(nbins)
interface de IHistDataSource
- get_interpolation()
Get interpolation mode
- get_lut_range()
Return the LUT transform range tuple: (min, max)
- get_lut_range_full()
Return full dynamic range
- get_lut_range_max()
Get maximum range for this dataset
- get_mask()[source]
Return image mask
- get_pixel_coordinates(xplot, yplot)
Return (image) pixel coordinates (from plot coordinates)
- get_plot_coordinates(xpixel, ypixel)
Return plot coordinates (from image pixel coordinates)
- get_stats(x0, y0, x1, y1)
Return formatted string with stats on image rectangular area (output should be compatible with AnnotatedShape.get_infos)
- get_xdata()
Return (xmin, xmax)
- get_xsection(y0, apply_lut=False)
Return cross section along x-axis at y=y0
- get_ydata()
Return (ymin, ymax)
- get_ysection(x0, apply_lut=False)
Return cross section along y-axis at x=x0
- is_empty()
Return True if item data is empty
- is_mask_visible()[source]
Return mask visibility
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- load_data(lut_range=None)
Load data from filename and eventually apply specified lut_range filename has been set using method ‘set_filename’
- mask_all()[source]
Mask all pixels
- mask_circular_area(x0, y0, x1, y1, inside=True, trace=True, do_signal=True)[source]
Mask circular area, inside the rectangle (x0, y0, x1, y1), i.e. circle with a radius of .5*(x1-x0) If inside is True (default), mask the inside of the area Otherwise, mask the outside
- mask_rectangular_area(x0, y0, x1, y1, inside=True, trace=True, do_signal=True)[source]
Mask rectangular area If inside is True (default), mask the inside of the area Otherwise, mask the outside
- move_local_point_to(handle, pos, ctrl=None)
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_local_shape(old_pos, new_pos)
Translate the shape such that old_pos becomes new_pos in canvas coordinates
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- serialize(writer)[source]
Serialize object to HDF5 writer
- set_data(data, lut_range=None)[source]
- Set Image item data
- data: 2D NumPy array
- lut_range: LUT range – tuple (levelmin, levelmax)
- set_interpolation(interp_mode, size=None)
Set image interpolation mode
interp_mode: INTERP_NEAREST, INTERP_LINEAR, INTERP_AA size (integer): (for anti-aliasing only) AA matrix size
- set_lut_range(lut_range)
Set LUT transform range lut_range is a tuple: (min, max)
- set_mask(mask)[source]
Set image mask
- set_mask_filename(fname)[source]
Set mask filename There are two ways for pickling mask data of MaskedImageItem objects:
- using the mask filename (as for data itself)
- using the mask areas (MaskedAreas instance, see set_mask_areas)
When saving objects, the first method is tried and then, if no filename has been defined for mask data, the second method is used.
- set_mask_visible(state)[source]
Set mask visibility
- set_masked_areas(areas)[source]
Set masked areas (see set_mask_filename)
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- unmask_all()[source]
Unmask all pixels
- unselect()
Unselect item
- update_border()
Update image border rectangle to fit image shape
- class guiqwt.image.ImageFilterItem(image, filter, param)[source]
- Construct a rectangular area image filter item
- image: guiqwt.image.RawImageItem instance
- filter: function (x, y, data) –> data
- param: image filter parameters (guiqwt.styles.ImageFilterParam instance)
- align_rectangular_shape(shape)
Align rectangular shape to image pixels
- draw_border(painter, xMap, yMap, canvasRect)
Draw image border rectangle
- draw_image(painter, canvasRect, src_rect, dst_rect, xMap, yMap)
Draw image with painter on canvasRect <!> src_rect and dst_rect are coord tuples (xleft, ytop, xright, ybottom)
- export_roi(src_rect, dst_rect, dst_image, apply_lut=False, apply_interpolation=False, original_resolution=False)
Export Region Of Interest to array
- get_average_xsection(x0, y0, x1, y1, apply_lut=False)
Return average cross section along x-axis
- get_average_ysection(x0, y0, x1, y1, apply_lut=False)
Return average cross section along y-axis
- get_closest_coordinates(x, y)
Return closest image pixel coordinates
- get_closest_index_rect(x0, y0, x1, y1)
Return closest image rectangular pixel area index bounds Avoid returning empty rectangular area (return 1x1 pixel area instead) Handle reversed/not-reversed Y-axis orientation
- get_closest_indexes(x, y, corner=None)
Return closest image pixel indexes corner: None (not a corner), ‘TL’ (top-left corner), ‘BR’ (bottom-right corner)
- get_closest_pixel_indexes(x, y)
Return closest pixel indexes Instead of returning indexes of an image pixel like the method ‘get_closest_indexes’, this method returns the indexes of the closest pixel which is not necessarily on the image itself (i.e. indexes may be outside image index bounds: negative or superior than the image dimension)
Note: this is not the same as retrieving the canvas pixel coordinates (which depends on the zoom level)
- get_data(x0, y0, x1=None, y1=None)
Return image data Arguments:
x0, y0 [, x1, y1]Return image level at coordinates (x0,y0) If x1,y1 are specified:
return image levels (np.ndarray) in rectangular area (x0,y0,x1,y1)
- get_default_param()
Return instance of the default imageparam DataSet
- get_filter(filterobj, filterparam)
Provides a filter object over this image’s content
- get_histogram(nbins)
interface de IHistDataSource
- get_interpolation()
Get interpolation mode
- get_lut_range_full()
Return full dynamic range
- get_lut_range_max()
Get maximum range for this dataset
- get_pixel_coordinates(xplot, yplot)
Return (image) pixel coordinates Transform the plot coordinates (arbitrary plot Z-axis unit) into the image coordinates (pixel unit)
Rounding is necessary to obtain array indexes from these coordinates
- get_plot_coordinates(xpixel, ypixel)
Return plot coordinates Transform the image coordinates (pixel unit) into the plot coordinates (arbitrary plot Z-axis unit)
- get_stats(x0, y0, x1, y1)
Return formatted string with stats on image rectangular area (output should be compatible with AnnotatedShape.get_infos)
- get_xsection(y0, apply_lut=False)
Return cross section along x-axis at y=y0
- get_ysection(x0, apply_lut=False)
Return cross section along y-axis at x=x0
- is_empty()
Return True if item data is empty
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- move_local_point_to(handle, pos, ctrl=None)[source]
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_local_shape(old_pos, new_pos)[source]
Translate the shape such that old_pos becomes new_pos in canvas coordinates
- move_with_selection(delta_x, delta_y)[source]
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- set_filter(filter)[source]
- Set the filter function
- filter: function (x, y, data) –> data
- set_image(image)[source]
- Set the image item on which the filter will be applied
- image: guiqwt.image.RawImageItem instance
- set_interpolation(interp_mode, size=None)
Set image interpolation mode
interp_mode: INTERP_NEAREST, INTERP_LINEAR, INTERP_AA size (integer): (for anti-aliasing only) AA matrix size
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- unselect()
Unselect item
- update_border()
Update image border rectangle to fit image shape
- class guiqwt.image.XYImageFilterItem(image, filter, param)[source]
- Construct a rectangular area image filter item
- image: guiqwt.image.XYImageItem instance
- filter: function (x, y, data) –> data
- param: image filter parameters (guiqwt.styles.ImageFilterParam instance)
- align_rectangular_shape(shape)
Align rectangular shape to image pixels
- draw_border(painter, xMap, yMap, canvasRect)
Draw image border rectangle
- export_roi(src_rect, dst_rect, dst_image, apply_lut=False, apply_interpolation=False, original_resolution=False)
Export Region Of Interest to array
- get_average_xsection(x0, y0, x1, y1, apply_lut=False)
Return average cross section along x-axis
- get_average_ysection(x0, y0, x1, y1, apply_lut=False)
Return average cross section along y-axis
- get_closest_coordinates(x, y)
Return closest image pixel coordinates
- get_closest_index_rect(x0, y0, x1, y1)
Return closest image rectangular pixel area index bounds Avoid returning empty rectangular area (return 1x1 pixel area instead) Handle reversed/not-reversed Y-axis orientation
- get_closest_indexes(x, y, corner=None)
Return closest image pixel indexes corner: None (not a corner), ‘TL’ (top-left corner), ‘BR’ (bottom-right corner)
- get_closest_pixel_indexes(x, y)
Return closest pixel indexes Instead of returning indexes of an image pixel like the method ‘get_closest_indexes’, this method returns the indexes of the closest pixel which is not necessarily on the image itself (i.e. indexes may be outside image index bounds: negative or superior than the image dimension)
Note: this is not the same as retrieving the canvas pixel coordinates (which depends on the zoom level)
- get_data(x0, y0, x1=None, y1=None)
Return image data Arguments:
x0, y0 [, x1, y1]Return image level at coordinates (x0,y0) If x1,y1 are specified:
return image levels (np.ndarray) in rectangular area (x0,y0,x1,y1)
- get_default_param()
Return instance of the default imageparam DataSet
- get_filter(filterobj, filterparam)
Provides a filter object over this image’s content
- get_histogram(nbins)
interface de IHistDataSource
- get_interpolation()
Get interpolation mode
- get_lut_range_full()
Return full dynamic range
- get_lut_range_max()
Get maximum range for this dataset
- get_pixel_coordinates(xplot, yplot)
Return (image) pixel coordinates Transform the plot coordinates (arbitrary plot Z-axis unit) into the image coordinates (pixel unit)
Rounding is necessary to obtain array indexes from these coordinates
- get_plot_coordinates(xpixel, ypixel)
Return plot coordinates Transform the image coordinates (pixel unit) into the plot coordinates (arbitrary plot Z-axis unit)
- get_stats(x0, y0, x1, y1)
Return formatted string with stats on image rectangular area (output should be compatible with AnnotatedShape.get_infos)
- get_xsection(y0, apply_lut=False)
Return cross section along x-axis at y=y0
- get_ysection(x0, apply_lut=False)
Return cross section along y-axis at x=x0
- is_empty()
Return True if item data is empty
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- move_local_point_to(handle, pos, ctrl=None)
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_local_shape(old_pos, new_pos)
Translate the shape such that old_pos becomes new_pos in canvas coordinates
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- set_filter(filter)
- Set the filter function
- filter: function (x, y, data) –> data
- set_image(image)[source]
- Set the image item on which the filter will be applied
- image: guiqwt.image.XYImageItem instance
- set_interpolation(interp_mode, size=None)
Set image interpolation mode
interp_mode: INTERP_NEAREST, INTERP_LINEAR, INTERP_AA size (integer): (for anti-aliasing only) AA matrix size
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- unselect()
Unselect item
- update_border()
Update image border rectangle to fit image shape
- class guiqwt.image.Histogram2DItem(X, Y, param=None, Z=None)[source]
- Construct a 2D histogram item
- X: data (1-D array)
- Y: data (1-D array)
- param (optional): style parameters (guiqwt.styles.Histogram2DParam instance)
- align_rectangular_shape(shape)
Align rectangular shape to image pixels
- draw_border(painter, xMap, yMap, canvasRect)
Draw image border rectangle
- export_roi(src_rect, dst_rect, dst_image, apply_lut=False, apply_interpolation=False, original_resolution=False)
Export Region Of Interest to array
- get_average_xsection(x0, y0, x1, y1, apply_lut=False)
Return average cross section along x-axis
- get_average_ysection(x0, y0, x1, y1, apply_lut=False)
Return average cross section along y-axis
- get_closest_coordinates(x, y)
Return closest image pixel coordinates
- get_closest_index_rect(x0, y0, x1, y1)
Return closest image rectangular pixel area index bounds Avoid returning empty rectangular area (return 1x1 pixel area instead) Handle reversed/not-reversed Y-axis orientation
- get_closest_indexes(x, y, corner=None)
Return closest image pixel indexes corner: None (not a corner), ‘TL’ (top-left corner), ‘BR’ (bottom-right corner)
- get_closest_pixel_indexes(x, y)
Return closest pixel indexes Instead of returning indexes of an image pixel like the method ‘get_closest_indexes’, this method returns the indexes of the closest pixel which is not necessarily on the image itself (i.e. indexes may be outside image index bounds: negative or superior than the image dimension)
Note: this is not the same as retrieving the canvas pixel coordinates (which depends on the zoom level)
- get_data(x0, y0, x1=None, y1=None)
Return image data Arguments:
x0, y0 [, x1, y1]Return image level at coordinates (x0,y0) If x1,y1 are specified:
return image levels (np.ndarray) in rectangular area (x0,y0,x1,y1)
- get_default_param()
Return instance of the default imageparam DataSet
- get_filter(filterobj, filterparam)
Provides a filter object over this image’s content
- get_histogram(nbins)[source]
interface de IHistDataSource
- get_interpolation()
Get interpolation mode
- get_lut_range()
Return the LUT transform range tuple: (min, max)
- get_lut_range_full()
Return full dynamic range
- get_lut_range_max()
Get maximum range for this dataset
- get_pixel_coordinates(xplot, yplot)
Return (image) pixel coordinates Transform the plot coordinates (arbitrary plot Z-axis unit) into the image coordinates (pixel unit)
Rounding is necessary to obtain array indexes from these coordinates
- get_plot_coordinates(xpixel, ypixel)
Return plot coordinates Transform the image coordinates (pixel unit) into the plot coordinates (arbitrary plot Z-axis unit)
- get_stats(x0, y0, x1, y1)
Return formatted string with stats on image rectangular area (output should be compatible with AnnotatedShape.get_infos)
- get_xsection(y0, apply_lut=False)
Return cross section along x-axis at y=y0
- get_ysection(x0, apply_lut=False)
Return cross section along y-axis at x=x0
- is_empty()
Return True if item data is empty
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- move_local_point_to(handle, pos, ctrl=None)
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_local_shape(old_pos, new_pos)
Translate the shape such that old_pos becomes new_pos in canvas coordinates
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- set_bins(NX, NY)[source]
Set histogram bins
- set_data(X, Y, Z=None)[source]
Set histogram data
- set_interpolation(interp_mode, size=None)
Set image interpolation mode
interp_mode: INTERP_NEAREST, INTERP_LINEAR, INTERP_AA size (integer): (for anti-aliasing only) AA matrix size
- set_lut_range(lut_range)
Set LUT transform range lut_range is a tuple: (min, max)
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- unselect()
Unselect item
- update_border()
Update image border rectangle to fit image shape
- guiqwt.image.assemble_imageitems(items, src_qrect, destw, desth, align=None, add_images=False, apply_lut=False, apply_interpolation=False, original_resolution=False)[source]
Assemble together image items in qrect (QRectF object) and return resulting pixel data <!> Does not support XYImageItem objects
- guiqwt.image.get_plot_qrect(plot, p0, p1)[source]
Return QRectF rectangle object in plot coordinates from top-left and bottom-right QPoint objects in canvas coordinates
- guiqwt.image.get_image_from_plot(plot, p0, p1, destw=None, desth=None, add_images=False, apply_lut=False, apply_interpolation=False, original_resolution=False)[source]
Return pixel data of a rectangular plot area (image items only) p0, p1: resp. top-left and bottom-right points (QPoint objects) apply_lut: apply contrast settings add_images: add superimposed images (instead of replace by the foreground)
Support only the image items implementing the IExportROIImageItemType interface, i.e. this does not support XYImageItem objects
guiqwt.histogram
- The histogram module provides histogram related objects:
- guiqwt.histogram.HistogramItem: an histogram plot item
- guiqwt.histogram.ContrastAdjustment: the contrast adjustment panel
- guiqwt.histogram.LevelsHistogram: a curve plotting widget used by the contrast adjustment panel to compute, manipulate and display the image levels histogram
HistogramItem objects are plot items (derived from QwtPlotItem) that may be displayed on a 2D plotting widget like guiqwt.curve.CurvePlot or guiqwt.image.ImagePlot.
Example
Simple histogram plotting example:
# -*- coding: utf-8 -*-
#
# Copyright © 2009-2010 CEA
# Pierre Raybaut
# Licensed under the terms of the CECILL License
# (see guiqwt/__init__.py for details)
"""Histogram test"""
SHOW = True # Show test in GUI-based test launcher
from guiqwt.plot import CurveDialog
from guiqwt.builder import make
def test():
"""Test"""
from numpy.random import normal
data = normal(0, 1, (2000, ))
win = CurveDialog(edit=False, toolbar=True, wintitle="Histogram test")
plot = win.get_plot()
plot.add_item(make.histogram(data))
win.show()
win.exec_()
if __name__ == "__main__":
# Create QApplication
import guidata
_app = guidata.qapplication()
test()
Reference
- class guiqwt.histogram.HistogramItem(curveparam=None, histparam=None)[source]
A Qwt item representing histogram data
- boundingRect()
Return the bounding rectangle of the data
- deserialize(reader)
Deserialize object from HDF5 reader
- get_closest_coordinates(x, y)
Renvoie les coordonnées (x’,y’) du point le plus proche de (x,y) Méthode surchargée pour ErrorBarSignalCurve pour renvoyer les coordonnées des pointes des barres d’erreur
- get_data()
Return curve data x, y (NumPy arrays)
- get_hist_source()[source]
Return histogram source (source: object with method ‘get_histogram’,
e.g. objects derived from guiqwt.image.ImageItem)
- get_logscale()[source]
Returns the status of the scale
- hit_test(pos)
Calcul de la distance d’un point à une courbe renvoie (dist, handle, inside)
- is_empty()
Return True if item data is empty
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- move_local_shape(old_pos, new_pos)
Translate the shape such that old_pos becomes new_pos in canvas coordinates
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- serialize(writer)
Serialize object to HDF5 writer
- set_data(x, y)
- Set curve data:
- x: NumPy array
- y: NumPy array
- set_hist_data(data)[source]
Set histogram data
- set_hist_source(src)[source]
Set histogram source (source: object with method ‘get_histogram’,
e.g. objects derived from guiqwt.image.ImageItem)
- set_logscale(state)[source]
Sets whether we use a logarithm or linear scale for the histogram counts
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- unselect()
Unselect item
- class guiqwt.histogram.ContrastAdjustment(parent=None)[source]
Contrast adjustment tool
- class RenderFlags
QWidget.RenderFlags(QWidget.RenderFlags) QWidget.RenderFlags(int) QWidget.RenderFlags()
- ContrastAdjustment.acceptDrops()
QWidget.acceptDrops() -> bool
- ContrastAdjustment.actionEvent()
QWidget.actionEvent(QActionEvent)
- ContrastAdjustment.actions()
QWidget.actions() -> list-of-QAction
- ContrastAdjustment.activateWindow()
QWidget.activateWindow()
- ContrastAdjustment.addAction()
QWidget.addAction(QAction)
- ContrastAdjustment.addActions()
QWidget.addActions(list-of-QAction)
- ContrastAdjustment.adjustSize()
QWidget.adjustSize()
- ContrastAdjustment.autoFillBackground()
QWidget.autoFillBackground() -> bool
- ContrastAdjustment.backgroundRole()
QWidget.backgroundRole() -> QPalette.ColorRole
- ContrastAdjustment.baseSize()
QWidget.baseSize() -> QSize
- ContrastAdjustment.blockSignals()
QObject.blockSignals(bool) -> bool
- ContrastAdjustment.changeEvent()
QWidget.changeEvent(QEvent)
- ContrastAdjustment.childAt()
QWidget.childAt(QPoint) -> QWidget QWidget.childAt(int, int) -> QWidget
- ContrastAdjustment.children()
QObject.children() -> list-of-QObject
- ContrastAdjustment.childrenRect()
QWidget.childrenRect() -> QRect
- ContrastAdjustment.childrenRegion()
QWidget.childrenRegion() -> QRegion
- ContrastAdjustment.clearFocus()
QWidget.clearFocus()
- ContrastAdjustment.clearMask()
QWidget.clearMask()
- ContrastAdjustment.close()
QWidget.close() -> bool
- ContrastAdjustment.colorCount()
QPaintDevice.colorCount() -> int
- ContrastAdjustment.configure_panel()[source]
Configure panel
- ContrastAdjustment.connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- ContrastAdjustment.contentsMargins()
QWidget.contentsMargins() -> QMargins
- ContrastAdjustment.contentsRect()
QWidget.contentsRect() -> QRect
- ContrastAdjustment.contextMenuEvent()
QWidget.contextMenuEvent(QContextMenuEvent)
- ContrastAdjustment.contextMenuPolicy()
QWidget.contextMenuPolicy() -> Qt.ContextMenuPolicy
- ContrastAdjustment.create()
QWidget.create(sip.voidptr window=None, bool initializeWindow=True, bool destroyOldWindow=True)
- ContrastAdjustment.create_dockwidget(title)
Add to parent QMainWindow as a dock widget
- ContrastAdjustment.cursor()
QWidget.cursor() -> QCursor
- ContrastAdjustment.customContextMenuRequested
QWidget.customContextMenuRequested[QPoint] [signal]
- ContrastAdjustment.deleteLater()
QObject.deleteLater()
- ContrastAdjustment.depth()
QPaintDevice.depth() -> int
- ContrastAdjustment.destroy()
QWidget.destroy(bool destroyWindow=True, bool destroySubWindows=True)
- ContrastAdjustment.destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- ContrastAdjustment.devType()
QWidget.devType() -> int
- ContrastAdjustment.disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- ContrastAdjustment.dragEnterEvent()
QWidget.dragEnterEvent(QDragEnterEvent)
- ContrastAdjustment.dragLeaveEvent()
QWidget.dragLeaveEvent(QDragLeaveEvent)
- ContrastAdjustment.dragMoveEvent()
QWidget.dragMoveEvent(QDragMoveEvent)
- ContrastAdjustment.dropEvent()
QWidget.dropEvent(QDropEvent)
- ContrastAdjustment.dumpObjectInfo()
QObject.dumpObjectInfo()
- ContrastAdjustment.dumpObjectTree()
QObject.dumpObjectTree()
- ContrastAdjustment.dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- ContrastAdjustment.effectiveWinId()
QWidget.effectiveWinId() -> sip.voidptr
- ContrastAdjustment.emit()
QObject.emit(SIGNAL(), ...)
- ContrastAdjustment.enabledChange()
QWidget.enabledChange(bool)
- ContrastAdjustment.ensurePolished()
QWidget.ensurePolished()
- ContrastAdjustment.enterEvent()
QWidget.enterEvent(QEvent)
- ContrastAdjustment.event()
QWidget.event(QEvent) -> bool
- ContrastAdjustment.eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- ContrastAdjustment.find()
QWidget.find(sip.voidptr) -> QWidget
- ContrastAdjustment.findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- ContrastAdjustment.findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- ContrastAdjustment.focusInEvent()
QWidget.focusInEvent(QFocusEvent)
- ContrastAdjustment.focusNextChild()
QWidget.focusNextChild() -> bool
- ContrastAdjustment.focusNextPrevChild()
QWidget.focusNextPrevChild(bool) -> bool
- ContrastAdjustment.focusOutEvent()
QWidget.focusOutEvent(QFocusEvent)
- ContrastAdjustment.focusPolicy()
QWidget.focusPolicy() -> Qt.FocusPolicy
- ContrastAdjustment.focusPreviousChild()
QWidget.focusPreviousChild() -> bool
- ContrastAdjustment.focusProxy()
QWidget.focusProxy() -> QWidget
- ContrastAdjustment.focusWidget()
QWidget.focusWidget() -> QWidget
- ContrastAdjustment.font()
QWidget.font() -> QFont
- ContrastAdjustment.fontChange()
QWidget.fontChange(QFont)
- ContrastAdjustment.fontInfo()
QWidget.fontInfo() -> QFontInfo
- ContrastAdjustment.fontMetrics()
QWidget.fontMetrics() -> QFontMetrics
- ContrastAdjustment.foregroundRole()
QWidget.foregroundRole() -> QPalette.ColorRole
- ContrastAdjustment.frameGeometry()
QWidget.frameGeometry() -> QRect
- ContrastAdjustment.frameSize()
QWidget.frameSize() -> QSize
- ContrastAdjustment.geometry()
QWidget.geometry() -> QRect
- ContrastAdjustment.getContentsMargins()
QWidget.getContentsMargins() -> (int, int, int, int)
- ContrastAdjustment.grabGesture()
QWidget.grabGesture(Qt.GestureType, Qt.GestureFlags flags=Qt.GestureFlags(0))
- ContrastAdjustment.grabKeyboard()
QWidget.grabKeyboard()
- ContrastAdjustment.grabMouse()
QWidget.grabMouse() QWidget.grabMouse(QCursor)
- ContrastAdjustment.grabShortcut()
QWidget.grabShortcut(QKeySequence, Qt.ShortcutContext context=Qt.WindowShortcut) -> int
- ContrastAdjustment.graphicsEffect()
QWidget.graphicsEffect() -> QGraphicsEffect
- ContrastAdjustment.graphicsProxyWidget()
QWidget.graphicsProxyWidget() -> QGraphicsProxyWidget
- ContrastAdjustment.hasFocus()
QWidget.hasFocus() -> bool
- ContrastAdjustment.hasMouseTracking()
QWidget.hasMouseTracking() -> bool
- ContrastAdjustment.height()
QWidget.height() -> int
- ContrastAdjustment.heightForWidth()
QWidget.heightForWidth(int) -> int
- ContrastAdjustment.heightMM()
QPaintDevice.heightMM() -> int
- ContrastAdjustment.hide()
QWidget.hide()
- ContrastAdjustment.inherits()
QObject.inherits(str) -> bool
- ContrastAdjustment.inputContext()
QWidget.inputContext() -> QInputContext
- ContrastAdjustment.inputMethodEvent()
QWidget.inputMethodEvent(QInputMethodEvent)
- ContrastAdjustment.inputMethodHints()
QWidget.inputMethodHints() -> Qt.InputMethodHints
- ContrastAdjustment.inputMethodQuery()
QWidget.inputMethodQuery(Qt.InputMethodQuery) -> QVariant
- ContrastAdjustment.insertAction()
QWidget.insertAction(QAction, QAction)
- ContrastAdjustment.insertActions()
QWidget.insertActions(QAction, list-of-QAction)
- ContrastAdjustment.installEventFilter()
QObject.installEventFilter(QObject)
- ContrastAdjustment.isActiveWindow()
QWidget.isActiveWindow() -> bool
- ContrastAdjustment.isAncestorOf()
QWidget.isAncestorOf(QWidget) -> bool
- ContrastAdjustment.isEnabled()
QWidget.isEnabled() -> bool
- ContrastAdjustment.isEnabledTo()
QWidget.isEnabledTo(QWidget) -> bool
- ContrastAdjustment.isEnabledToTLW()
QWidget.isEnabledToTLW() -> bool
- ContrastAdjustment.isFullScreen()
QWidget.isFullScreen() -> bool
- ContrastAdjustment.isHidden()
QWidget.isHidden() -> bool
- ContrastAdjustment.isLeftToRight()
QWidget.isLeftToRight() -> bool
- ContrastAdjustment.isMaximized()
QWidget.isMaximized() -> bool
- ContrastAdjustment.isMinimized()
QWidget.isMinimized() -> bool
- ContrastAdjustment.isModal()
QWidget.isModal() -> bool
- ContrastAdjustment.isRightToLeft()
QWidget.isRightToLeft() -> bool
- ContrastAdjustment.isTopLevel()
QWidget.isTopLevel() -> bool
- ContrastAdjustment.isVisible()
QWidget.isVisible() -> bool
- ContrastAdjustment.isVisibleTo()
QWidget.isVisibleTo(QWidget) -> bool
- ContrastAdjustment.isWidgetType()
QObject.isWidgetType() -> bool
- ContrastAdjustment.isWindow()
QWidget.isWindow() -> bool
- ContrastAdjustment.isWindowModified()
QWidget.isWindowModified() -> bool
- ContrastAdjustment.keyPressEvent()
QWidget.keyPressEvent(QKeyEvent)
- ContrastAdjustment.keyReleaseEvent()
QWidget.keyReleaseEvent(QKeyEvent)
- ContrastAdjustment.keyboardGrabber()
QWidget.keyboardGrabber() -> QWidget
- ContrastAdjustment.killTimer()
QObject.killTimer(int)
- ContrastAdjustment.languageChange()
QWidget.languageChange()
- ContrastAdjustment.layout()
QWidget.layout() -> QLayout
- ContrastAdjustment.layoutDirection()
QWidget.layoutDirection() -> Qt.LayoutDirection
- ContrastAdjustment.leaveEvent()
QWidget.leaveEvent(QEvent)
- ContrastAdjustment.locale()
QWidget.locale() -> QLocale
- ContrastAdjustment.logicalDpiX()
QPaintDevice.logicalDpiX() -> int
- ContrastAdjustment.logicalDpiY()
QPaintDevice.logicalDpiY() -> int
- ContrastAdjustment.lower()
QWidget.lower()
- ContrastAdjustment.mapFrom()
QWidget.mapFrom(QWidget, QPoint) -> QPoint
- ContrastAdjustment.mapFromGlobal()
QWidget.mapFromGlobal(QPoint) -> QPoint
- ContrastAdjustment.mapFromParent()
QWidget.mapFromParent(QPoint) -> QPoint
- ContrastAdjustment.mapTo()
QWidget.mapTo(QWidget, QPoint) -> QPoint
- ContrastAdjustment.mapToGlobal()
QWidget.mapToGlobal(QPoint) -> QPoint
- ContrastAdjustment.mapToParent()
QWidget.mapToParent(QPoint) -> QPoint
- ContrastAdjustment.mask()
QWidget.mask() -> QRegion
- ContrastAdjustment.maximumHeight()
QWidget.maximumHeight() -> int
- ContrastAdjustment.maximumSize()
QWidget.maximumSize() -> QSize
- ContrastAdjustment.maximumWidth()
QWidget.maximumWidth() -> int
- ContrastAdjustment.metaObject()
QObject.metaObject() -> QMetaObject
- ContrastAdjustment.metric()
QWidget.metric(QPaintDevice.PaintDeviceMetric) -> int
- ContrastAdjustment.minimumHeight()
QWidget.minimumHeight() -> int
- ContrastAdjustment.minimumSize()
QWidget.minimumSize() -> QSize
- ContrastAdjustment.minimumSizeHint()
QWidget.minimumSizeHint() -> QSize
- ContrastAdjustment.minimumWidth()
QWidget.minimumWidth() -> int
- ContrastAdjustment.mouseDoubleClickEvent()
QWidget.mouseDoubleClickEvent(QMouseEvent)
- ContrastAdjustment.mouseGrabber()
QWidget.mouseGrabber() -> QWidget
- ContrastAdjustment.mouseMoveEvent()
QWidget.mouseMoveEvent(QMouseEvent)
- ContrastAdjustment.mousePressEvent()
QWidget.mousePressEvent(QMouseEvent)
- ContrastAdjustment.mouseReleaseEvent()
QWidget.mouseReleaseEvent(QMouseEvent)
- ContrastAdjustment.move()
QWidget.move(QPoint) QWidget.move(int, int)
- ContrastAdjustment.moveEvent()
QWidget.moveEvent(QMoveEvent)
- ContrastAdjustment.moveToThread()
QObject.moveToThread(QThread)
- ContrastAdjustment.nativeParentWidget()
QWidget.nativeParentWidget() -> QWidget
- ContrastAdjustment.nextInFocusChain()
QWidget.nextInFocusChain() -> QWidget
- ContrastAdjustment.normalGeometry()
QWidget.normalGeometry() -> QRect
- ContrastAdjustment.numColors()
QPaintDevice.numColors() -> int
- ContrastAdjustment.objectName()
QObject.objectName() -> QString
- ContrastAdjustment.overrideWindowFlags()
QWidget.overrideWindowFlags(Qt.WindowFlags)
- ContrastAdjustment.overrideWindowState()
QWidget.overrideWindowState(Qt.WindowStates)
- ContrastAdjustment.paintEngine()
QWidget.paintEngine() -> QPaintEngine
- ContrastAdjustment.paintEvent()
QWidget.paintEvent(QPaintEvent)
- ContrastAdjustment.paintingActive()
QPaintDevice.paintingActive() -> bool
- ContrastAdjustment.palette()
QWidget.palette() -> QPalette
- ContrastAdjustment.paletteChange()
QWidget.paletteChange(QPalette)
- ContrastAdjustment.parent()
QObject.parent() -> QObject
- ContrastAdjustment.parentWidget()
QWidget.parentWidget() -> QWidget
- ContrastAdjustment.physicalDpiX()
QPaintDevice.physicalDpiX() -> int
- ContrastAdjustment.physicalDpiY()
QPaintDevice.physicalDpiY() -> int
- ContrastAdjustment.pos()
QWidget.pos() -> QPoint
- ContrastAdjustment.previousInFocusChain()
QWidget.previousInFocusChain() -> QWidget
- ContrastAdjustment.property()
QObject.property(str) -> QVariant
- ContrastAdjustment.pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- ContrastAdjustment.raise_()
QWidget.raise_()
- ContrastAdjustment.rect()
QWidget.rect() -> QRect
- ContrastAdjustment.register_panel(manager)[source]
Register panel to plot manager
- ContrastAdjustment.releaseKeyboard()
QWidget.releaseKeyboard()
- ContrastAdjustment.releaseMouse()
QWidget.releaseMouse()
- ContrastAdjustment.releaseShortcut()
QWidget.releaseShortcut(int)
- ContrastAdjustment.removeAction()
QWidget.removeAction(QAction)
- ContrastAdjustment.removeEventFilter()
QObject.removeEventFilter(QObject)
- ContrastAdjustment.render()
QWidget.render(QPaintDevice, QPoint targetOffset=QPoint(), QRegion sourceRegion=QRegion(), QWidget.RenderFlags flags=QWidget.DrawWindowBackground|QWidget.DrawChildren) QWidget.render(QPainter, QPoint targetOffset=QPoint(), QRegion sourceRegion=QRegion(), QWidget.RenderFlags flags=QWidget.DrawWindowBackground|QWidget.DrawChildren)
- ContrastAdjustment.repaint()
QWidget.repaint() QWidget.repaint(int, int, int, int) QWidget.repaint(QRect) QWidget.repaint(QRegion)
- ContrastAdjustment.resetInputContext()
QWidget.resetInputContext()
- ContrastAdjustment.resize()
QWidget.resize(QSize) QWidget.resize(int, int)
- ContrastAdjustment.resizeEvent()
QWidget.resizeEvent(QResizeEvent)
- ContrastAdjustment.restoreGeometry()
QWidget.restoreGeometry(QByteArray) -> bool
- ContrastAdjustment.saveGeometry()
QWidget.saveGeometry() -> QByteArray
- ContrastAdjustment.scroll()
QWidget.scroll(int, int) QWidget.scroll(int, int, QRect)
- ContrastAdjustment.setAcceptDrops()
QWidget.setAcceptDrops(bool)
- ContrastAdjustment.setAttribute()
QWidget.setAttribute(Qt.WidgetAttribute, bool on=True)
- ContrastAdjustment.setAutoFillBackground()
QWidget.setAutoFillBackground(bool)
- ContrastAdjustment.setBackgroundRole()
QWidget.setBackgroundRole(QPalette.ColorRole)
- ContrastAdjustment.setBaseSize()
QWidget.setBaseSize(int, int) QWidget.setBaseSize(QSize)
- ContrastAdjustment.setContentsMargins()
QWidget.setContentsMargins(int, int, int, int) QWidget.setContentsMargins(QMargins)
- ContrastAdjustment.setContextMenuPolicy()
QWidget.setContextMenuPolicy(Qt.ContextMenuPolicy)
- ContrastAdjustment.setCursor()
QWidget.setCursor(QCursor)
- ContrastAdjustment.setDisabled()
QWidget.setDisabled(bool)
- ContrastAdjustment.setEnabled()
QWidget.setEnabled(bool)
- ContrastAdjustment.setFixedHeight()
QWidget.setFixedHeight(int)
- ContrastAdjustment.setFixedSize()
QWidget.setFixedSize(QSize) QWidget.setFixedSize(int, int)
- ContrastAdjustment.setFixedWidth()
QWidget.setFixedWidth(int)
- ContrastAdjustment.setFocus()
QWidget.setFocus() QWidget.setFocus(Qt.FocusReason)
- ContrastAdjustment.setFocusPolicy()
QWidget.setFocusPolicy(Qt.FocusPolicy)
- ContrastAdjustment.setFocusProxy()
QWidget.setFocusProxy(QWidget)
- ContrastAdjustment.setFont()
QWidget.setFont(QFont)
- ContrastAdjustment.setForegroundRole()
QWidget.setForegroundRole(QPalette.ColorRole)
- ContrastAdjustment.setGeometry()
QWidget.setGeometry(QRect) QWidget.setGeometry(int, int, int, int)
- ContrastAdjustment.setGraphicsEffect()
QWidget.setGraphicsEffect(QGraphicsEffect)
- ContrastAdjustment.setHidden()
QWidget.setHidden(bool)
- ContrastAdjustment.setInputContext()
QWidget.setInputContext(QInputContext)
- ContrastAdjustment.setInputMethodHints()
QWidget.setInputMethodHints(Qt.InputMethodHints)
- ContrastAdjustment.setLayout()
QWidget.setLayout(QLayout)
- ContrastAdjustment.setLayoutDirection()
QWidget.setLayoutDirection(Qt.LayoutDirection)
- ContrastAdjustment.setLocale()
QWidget.setLocale(QLocale)
- ContrastAdjustment.setMask()
QWidget.setMask(QBitmap) QWidget.setMask(QRegion)
- ContrastAdjustment.setMaximumHeight()
QWidget.setMaximumHeight(int)
- ContrastAdjustment.setMaximumSize()
QWidget.setMaximumSize(int, int) QWidget.setMaximumSize(QSize)
- ContrastAdjustment.setMaximumWidth()
QWidget.setMaximumWidth(int)
- ContrastAdjustment.setMinimumHeight()
QWidget.setMinimumHeight(int)
- ContrastAdjustment.setMinimumSize()
QWidget.setMinimumSize(int, int) QWidget.setMinimumSize(QSize)
- ContrastAdjustment.setMinimumWidth()
QWidget.setMinimumWidth(int)
- ContrastAdjustment.setMouseTracking()
QWidget.setMouseTracking(bool)
- ContrastAdjustment.setObjectName()
QObject.setObjectName(QString)
- ContrastAdjustment.setPalette()
QWidget.setPalette(QPalette)
- ContrastAdjustment.setParent()
QWidget.setParent(QWidget) QWidget.setParent(QWidget, Qt.WindowFlags)
- ContrastAdjustment.setProperty()
QObject.setProperty(str, QVariant) -> bool
- ContrastAdjustment.setShortcutAutoRepeat()
QWidget.setShortcutAutoRepeat(int, bool enabled=True)
- ContrastAdjustment.setShortcutEnabled()
QWidget.setShortcutEnabled(int, bool enabled=True)
- ContrastAdjustment.setShown()
QWidget.setShown(bool)
- ContrastAdjustment.setSizeIncrement()
QWidget.setSizeIncrement(int, int) QWidget.setSizeIncrement(QSize)
- ContrastAdjustment.setSizePolicy()
QWidget.setSizePolicy(QSizePolicy) QWidget.setSizePolicy(QSizePolicy.Policy, QSizePolicy.Policy)
- ContrastAdjustment.setStatusTip()
QWidget.setStatusTip(QString)
- ContrastAdjustment.setStyle()
QWidget.setStyle(QStyle)
- ContrastAdjustment.setStyleSheet()
QWidget.setStyleSheet(QString)
- ContrastAdjustment.setTabOrder()
QWidget.setTabOrder(QWidget, QWidget)
- ContrastAdjustment.setToolTip()
QWidget.setToolTip(QString)
- ContrastAdjustment.setUpdatesEnabled()
QWidget.setUpdatesEnabled(bool)
- ContrastAdjustment.setVisible()
QWidget.setVisible(bool)
- ContrastAdjustment.setWhatsThis()
QWidget.setWhatsThis(QString)
- ContrastAdjustment.setWindowFilePath()
QWidget.setWindowFilePath(QString)
- ContrastAdjustment.setWindowFlags()
QWidget.setWindowFlags(Qt.WindowFlags)
- ContrastAdjustment.setWindowIcon()
QWidget.setWindowIcon(QIcon)
- ContrastAdjustment.setWindowIconText()
QWidget.setWindowIconText(QString)
- ContrastAdjustment.setWindowModality()
QWidget.setWindowModality(Qt.WindowModality)
- ContrastAdjustment.setWindowModified()
QWidget.setWindowModified(bool)
- ContrastAdjustment.setWindowOpacity()
QWidget.setWindowOpacity(float)
- ContrastAdjustment.setWindowRole()
QWidget.setWindowRole(QString)
- ContrastAdjustment.setWindowState()
QWidget.setWindowState(Qt.WindowStates)
- ContrastAdjustment.setWindowTitle()
QWidget.setWindowTitle(QString)
- ContrastAdjustment.set_range(_min, _max)[source]
Set contrast panel’s histogram range
- ContrastAdjustment.show()
QWidget.show()
- ContrastAdjustment.showFullScreen()
QWidget.showFullScreen()
- ContrastAdjustment.showMaximized()
QWidget.showMaximized()
- ContrastAdjustment.showMinimized()
QWidget.showMinimized()
- ContrastAdjustment.showNormal()
QWidget.showNormal()
- ContrastAdjustment.signalsBlocked()
QObject.signalsBlocked() -> bool
- ContrastAdjustment.size()
QWidget.size() -> QSize
- ContrastAdjustment.sizeHint()
QWidget.sizeHint() -> QSize
- ContrastAdjustment.sizeIncrement()
QWidget.sizeIncrement() -> QSize
- ContrastAdjustment.sizePolicy()
QWidget.sizePolicy() -> QSizePolicy
- ContrastAdjustment.stackUnder()
QWidget.stackUnder(QWidget)
- ContrastAdjustment.startTimer()
QObject.startTimer(int) -> int
- ContrastAdjustment.statusTip()
QWidget.statusTip() -> QString
- ContrastAdjustment.style()
QWidget.style() -> QStyle
- ContrastAdjustment.styleSheet()
QWidget.styleSheet() -> QString
- ContrastAdjustment.tabletEvent()
QWidget.tabletEvent(QTabletEvent)
- ContrastAdjustment.testAttribute()
QWidget.testAttribute(Qt.WidgetAttribute) -> bool
- ContrastAdjustment.thread()
QObject.thread() -> QThread
- ContrastAdjustment.toolTip()
QWidget.toolTip() -> QString
- ContrastAdjustment.topLevelWidget()
QWidget.topLevelWidget() -> QWidget
- ContrastAdjustment.tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- ContrastAdjustment.trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- ContrastAdjustment.underMouse()
QWidget.underMouse() -> bool
- ContrastAdjustment.ungrabGesture()
QWidget.ungrabGesture(Qt.GestureType)
- ContrastAdjustment.unsetCursor()
QWidget.unsetCursor()
- ContrastAdjustment.unsetLayoutDirection()
QWidget.unsetLayoutDirection()
- ContrastAdjustment.unsetLocale()
QWidget.unsetLocale()
- ContrastAdjustment.update()
QWidget.update() QWidget.update(QRect) QWidget.update(QRegion) QWidget.update(int, int, int, int)
- ContrastAdjustment.updateGeometry()
QWidget.updateGeometry()
- ContrastAdjustment.updateMicroFocus()
QWidget.updateMicroFocus()
- ContrastAdjustment.updatesEnabled()
QWidget.updatesEnabled() -> bool
- ContrastAdjustment.visibility_changed(enable)
DockWidget visibility has changed
- ContrastAdjustment.visibleRegion()
QWidget.visibleRegion() -> QRegion
- ContrastAdjustment.whatsThis()
QWidget.whatsThis() -> QString
- ContrastAdjustment.wheelEvent()
QWidget.wheelEvent(QWheelEvent)
- ContrastAdjustment.width()
QWidget.width() -> int
- ContrastAdjustment.widthMM()
QPaintDevice.widthMM() -> int
- ContrastAdjustment.winEvent()
QWidget.winEvent(MSG) -> (bool, int)
- ContrastAdjustment.winId()
QWidget.winId() -> sip.voidptr
- ContrastAdjustment.window()
QWidget.window() -> QWidget
- ContrastAdjustment.windowActivationChange()
QWidget.windowActivationChange(bool)
- ContrastAdjustment.windowFilePath()
QWidget.windowFilePath() -> QString
- ContrastAdjustment.windowFlags()
QWidget.windowFlags() -> Qt.WindowFlags
- ContrastAdjustment.windowIcon()
QWidget.windowIcon() -> QIcon
- ContrastAdjustment.windowIconText()
QWidget.windowIconText() -> QString
- ContrastAdjustment.windowModality()
QWidget.windowModality() -> Qt.WindowModality
- ContrastAdjustment.windowOpacity()
QWidget.windowOpacity() -> float
- ContrastAdjustment.windowRole()
QWidget.windowRole() -> QString
- ContrastAdjustment.windowState()
QWidget.windowState() -> Qt.WindowStates
- ContrastAdjustment.windowTitle()
QWidget.windowTitle() -> QString
- ContrastAdjustment.windowType()
QWidget.windowType() -> Qt.WindowType
- ContrastAdjustment.x()
QWidget.x() -> int
- ContrastAdjustment.y()
QWidget.y() -> int
- class guiqwt.histogram.LevelsHistogram(parent=None)[source]
Image levels histogram widget
- DEFAULT_ITEM_TYPE
alias of ICurveItemType
- class RenderFlags
QWidget.RenderFlags(QWidget.RenderFlags) QWidget.RenderFlags(int) QWidget.RenderFlags()
- LevelsHistogram.acceptDrops()
QWidget.acceptDrops() -> bool
- LevelsHistogram.actions()
QWidget.actions() -> list-of-QAction
- LevelsHistogram.activateWindow()
QWidget.activateWindow()
- LevelsHistogram.addAction()
QWidget.addAction(QAction)
- LevelsHistogram.addActions()
QWidget.addActions(list-of-QAction)
- LevelsHistogram.add_item(item, z=None)
- Add a plot item instance to this plot widget
- item: QwtPlotItem (PyQt4.Qwt5) object implementing the IBasePlotItem interface (guiqwt.interfaces)
- z: item’s z order (None -> z = max(self.get_items())+1)
- LevelsHistogram.add_item_with_z_offset(item, zoffset)
Add a plot item instance within a specified z range, over zmin
- LevelsHistogram.adjustSize()
QWidget.adjustSize()
- LevelsHistogram.autoFillBackground()
QWidget.autoFillBackground() -> bool
- LevelsHistogram.backgroundRole()
QWidget.backgroundRole() -> QPalette.ColorRole
- LevelsHistogram.baseSize()
QWidget.baseSize() -> QSize
- LevelsHistogram.blockSignals()
QObject.blockSignals(bool) -> bool
- LevelsHistogram.childAt()
QWidget.childAt(QPoint) -> QWidget QWidget.childAt(int, int) -> QWidget
- LevelsHistogram.children()
QObject.children() -> list-of-QObject
- LevelsHistogram.childrenRect()
QWidget.childrenRect() -> QRect
- LevelsHistogram.childrenRegion()
QWidget.childrenRegion() -> QRegion
- LevelsHistogram.clearFocus()
QWidget.clearFocus()
- LevelsHistogram.clearMask()
QWidget.clearMask()
- LevelsHistogram.close()
QWidget.close() -> bool
- LevelsHistogram.colorCount()
QPaintDevice.colorCount() -> int
- LevelsHistogram.connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- LevelsHistogram.contentsMargins()
QWidget.contentsMargins() -> QMargins
- LevelsHistogram.contentsRect()
QWidget.contentsRect() -> QRect
- LevelsHistogram.contextMenuPolicy()
QWidget.contextMenuPolicy() -> Qt.ContextMenuPolicy
- LevelsHistogram.copy_to_clipboard()
Copy widget’s window to clipboard
- LevelsHistogram.cursor()
QWidget.cursor() -> QCursor
- LevelsHistogram.customContextMenuRequested
QWidget.customContextMenuRequested[QPoint] [signal]
- LevelsHistogram.del_all_items(except_grid=True)
Del all items, eventually (default) except grid
- LevelsHistogram.del_item(item)
Remove item from widget Convenience function (see ‘del_items’)
- LevelsHistogram.del_items(items)
Remove item from widget
- LevelsHistogram.deleteLater()
QObject.deleteLater()
- LevelsHistogram.depth()
QPaintDevice.depth() -> int
- LevelsHistogram.deserialize(reader)
- Restore items from HDF5 file:
- reader: guidata.hdf5io.HDF5Reader object
See also guiqwt.baseplot.BasePlot.save_items_to_hdf5()
- LevelsHistogram.destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- LevelsHistogram.devType()
QWidget.devType() -> int
- LevelsHistogram.disable_autoscale()
Re-apply the axis scales so as to disable autoscaling without changing the view
- LevelsHistogram.disable_unused_axes()
Disable unused axes
- LevelsHistogram.disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- LevelsHistogram.do_autoscale(replot=True)
Do autoscale on all axes
- LevelsHistogram.do_pan_view(dx, dy)
Translate the active axes by dx, dy dx, dy are tuples composed of (initial pos, dest pos)
- LevelsHistogram.do_zoom_view(dx, dy, lock_aspect_ratio=False)
Change the scale of the active axes (zoom/dezoom) according to dx, dy dx, dy are tuples composed of (initial pos, dest pos) We try to keep initial pos fixed on the canvas as the scale changes
- LevelsHistogram.dumpObjectInfo()
QObject.dumpObjectInfo()
- LevelsHistogram.dumpObjectTree()
QObject.dumpObjectTree()
- LevelsHistogram.dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- LevelsHistogram.edit_axis_parameters(axis_id)
Edit axis parameters
- LevelsHistogram.edit_plot_parameters(key)
Edit plot parameters
- LevelsHistogram.effectiveWinId()
QWidget.effectiveWinId() -> sip.voidptr
- LevelsHistogram.eliminate_outliers(percent)[source]
Eliminate outliers: eliminate percent/2*N counts on each side of the histogram (where N is the total count number)
- LevelsHistogram.emit()
QObject.emit(SIGNAL(), ...)
- LevelsHistogram.enable_used_axes()
Enable only used axes For now, this is needed only by the pyplot interface
- LevelsHistogram.ensurePolished()
QWidget.ensurePolished()
- LevelsHistogram.eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- LevelsHistogram.find()
QWidget.find(sip.voidptr) -> QWidget
- LevelsHistogram.findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- LevelsHistogram.findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- LevelsHistogram.focusPolicy()
QWidget.focusPolicy() -> Qt.FocusPolicy
- LevelsHistogram.focusProxy()
QWidget.focusProxy() -> QWidget
- LevelsHistogram.focusWidget()
QWidget.focusWidget() -> QWidget
- LevelsHistogram.font()
QWidget.font() -> QFont
- LevelsHistogram.fontInfo()
QWidget.fontInfo() -> QFontInfo
- LevelsHistogram.fontMetrics()
QWidget.fontMetrics() -> QFontMetrics
- LevelsHistogram.foregroundRole()
QWidget.foregroundRole() -> QPalette.ColorRole
- LevelsHistogram.frameGeometry()
QWidget.frameGeometry() -> QRect
- LevelsHistogram.frameRect()
QFrame.frameRect() -> QRect
- LevelsHistogram.frameShadow()
QFrame.frameShadow() -> QFrame.Shadow
- LevelsHistogram.frameShape()
QFrame.frameShape() -> QFrame.Shape
- LevelsHistogram.frameSize()
QWidget.frameSize() -> QSize
- LevelsHistogram.frameStyle()
QFrame.frameStyle() -> int
- LevelsHistogram.frameWidth()
QFrame.frameWidth() -> int
- LevelsHistogram.geometry()
QWidget.geometry() -> QRect
- LevelsHistogram.getContentsMargins()
QWidget.getContentsMargins() -> (int, int, int, int)
- LevelsHistogram.get_active_axes()
Return active axes
- LevelsHistogram.get_active_item(force=False)
Return active item Force item activation if there is no active item
- LevelsHistogram.get_axesparam_class(item)
Return AxesParam dataset class associated to item’s type
- LevelsHistogram.get_axis_color(axis_id)
Get axis color (color name, i.e. string)
- LevelsHistogram.get_axis_direction(axis_id)
- Return axis direction of increasing values
- axis_id: axis id (BasePlot.Y_LEFT, BasePlot.X_BOTTOM, ...) or string: ‘bottom’, ‘left’, ‘top’ or ‘right’
- LevelsHistogram.get_axis_font(axis_id)
Get axis font
- LevelsHistogram.get_axis_id(axis_name)
Return axis ID from axis name If axis ID is passed directly, check the ID
- LevelsHistogram.get_axis_limits(axis_id)
Return axis limits (minimum and maximum values)
- LevelsHistogram.get_axis_scale(axis_id)
Return the name (‘lin’ or ‘log’) of the scale used by axis
- LevelsHistogram.get_axis_title(axis_id)
Get axis title
- LevelsHistogram.get_axis_unit(axis_id)
Get axis unit
Return widget context menu
- LevelsHistogram.get_default_item()
Return default item, depending on plot’s default item type (e.g. for a curve plot, this is a curve item type).
Return nothing if there is more than one item matching the default item type.
- LevelsHistogram.get_items(z_sorted=False, item_type=None)
Return widget’s item list (items are based on IBasePlotItem’s interface)
- LevelsHistogram.get_last_active_item(item_type)
Return last active item corresponding to passed item_type
- LevelsHistogram.get_max_z()
Return maximum z-order for all items registered in plot If there is no item, return 0
- LevelsHistogram.get_nearest_object(pos, close_dist=0)
Return nearest item from position ‘pos’ If close_dist > 0: return the first found item (higher z) which
distance to ‘pos’ is less than close_distelse: return the closest item
- LevelsHistogram.get_nearest_object_in_z(pos)
Return nearest item for which position ‘pos’ is inside of it (iterate over items with respect to their ‘z’ coordinate)
- LevelsHistogram.get_plot_limits(xaxis='bottom', yaxis='left')
Return plot scale limits
- LevelsHistogram.get_private_items(z_sorted=False, item_type=None)
Return widget’s private item list (items are based on IBasePlotItem’s interface)
- LevelsHistogram.get_public_items(z_sorted=False, item_type=None)
Return widget’s public item list (items are based on IBasePlotItem’s interface)
- LevelsHistogram.get_scales()
Return active curve scales
- LevelsHistogram.get_selected_items(z_sorted=False, item_type=None)
Return selected items
- LevelsHistogram.get_title()
Get plot title
- LevelsHistogram.grabGesture()
QWidget.grabGesture(Qt.GestureType, Qt.GestureFlags flags=Qt.GestureFlags(0))
- LevelsHistogram.grabKeyboard()
QWidget.grabKeyboard()
- LevelsHistogram.grabMouse()
QWidget.grabMouse() QWidget.grabMouse(QCursor)
- LevelsHistogram.grabShortcut()
QWidget.grabShortcut(QKeySequence, Qt.ShortcutContext context=Qt.WindowShortcut) -> int
- LevelsHistogram.graphicsEffect()
QWidget.graphicsEffect() -> QGraphicsEffect
- LevelsHistogram.graphicsProxyWidget()
QWidget.graphicsProxyWidget() -> QGraphicsProxyWidget
- LevelsHistogram.hasFocus()
QWidget.hasFocus() -> bool
- LevelsHistogram.hasMouseTracking()
QWidget.hasMouseTracking() -> bool
- LevelsHistogram.height()
QWidget.height() -> int
- LevelsHistogram.heightForWidth()
QWidget.heightForWidth(int) -> int
- LevelsHistogram.heightMM()
QPaintDevice.heightMM() -> int
- LevelsHistogram.hide()
QWidget.hide()
- LevelsHistogram.hide_items(items=None, item_type=None)
Hide items (if items is None, hide all items)
- LevelsHistogram.inherits()
QObject.inherits(str) -> bool
- LevelsHistogram.inputContext()
QWidget.inputContext() -> QInputContext
- LevelsHistogram.inputMethodHints()
QWidget.inputMethodHints() -> Qt.InputMethodHints
- LevelsHistogram.inputMethodQuery()
QWidget.inputMethodQuery(Qt.InputMethodQuery) -> QVariant
- LevelsHistogram.insertAction()
QWidget.insertAction(QAction, QAction)
- LevelsHistogram.insertActions()
QWidget.insertActions(QAction, list-of-QAction)
- LevelsHistogram.installEventFilter()
QObject.installEventFilter(QObject)
- LevelsHistogram.invalidate()
Invalidate paint cache and schedule redraw use instead of replot when only the content of the canvas needs redrawing (axes, shouldn’t change)
- LevelsHistogram.isActiveWindow()
QWidget.isActiveWindow() -> bool
- LevelsHistogram.isAncestorOf()
QWidget.isAncestorOf(QWidget) -> bool
- LevelsHistogram.isEnabled()
QWidget.isEnabled() -> bool
- LevelsHistogram.isEnabledTo()
QWidget.isEnabledTo(QWidget) -> bool
- LevelsHistogram.isEnabledToTLW()
QWidget.isEnabledToTLW() -> bool
- LevelsHistogram.isFullScreen()
QWidget.isFullScreen() -> bool
- LevelsHistogram.isHidden()
QWidget.isHidden() -> bool
- LevelsHistogram.isLeftToRight()
QWidget.isLeftToRight() -> bool
- LevelsHistogram.isMaximized()
QWidget.isMaximized() -> bool
- LevelsHistogram.isMinimized()
QWidget.isMinimized() -> bool
- LevelsHistogram.isModal()
QWidget.isModal() -> bool
- LevelsHistogram.isRightToLeft()
QWidget.isRightToLeft() -> bool
- LevelsHistogram.isTopLevel()
QWidget.isTopLevel() -> bool
- LevelsHistogram.isVisible()
QWidget.isVisible() -> bool
- LevelsHistogram.isVisibleTo()
QWidget.isVisibleTo(QWidget) -> bool
- LevelsHistogram.isWidgetType()
QObject.isWidgetType() -> bool
- LevelsHistogram.isWindow()
QWidget.isWindow() -> bool
- LevelsHistogram.isWindowModified()
QWidget.isWindowModified() -> bool
- LevelsHistogram.keyboardGrabber()
QWidget.keyboardGrabber() -> QWidget
- LevelsHistogram.killTimer()
QObject.killTimer(int)
- LevelsHistogram.layout()
QWidget.layout() -> QLayout
- LevelsHistogram.layoutDirection()
QWidget.layoutDirection() -> Qt.LayoutDirection
- LevelsHistogram.lineWidth()
QFrame.lineWidth() -> int
- LevelsHistogram.locale()
QWidget.locale() -> QLocale
- LevelsHistogram.logicalDpiX()
QPaintDevice.logicalDpiX() -> int
- LevelsHistogram.logicalDpiY()
QPaintDevice.logicalDpiY() -> int
- LevelsHistogram.lower()
QWidget.lower()
- LevelsHistogram.mapFrom()
QWidget.mapFrom(QWidget, QPoint) -> QPoint
- LevelsHistogram.mapFromGlobal()
QWidget.mapFromGlobal(QPoint) -> QPoint
- LevelsHistogram.mapFromParent()
QWidget.mapFromParent(QPoint) -> QPoint
- LevelsHistogram.mapTo()
QWidget.mapTo(QWidget, QPoint) -> QPoint
- LevelsHistogram.mapToGlobal()
QWidget.mapToGlobal(QPoint) -> QPoint
- LevelsHistogram.mapToParent()
QWidget.mapToParent(QPoint) -> QPoint
- LevelsHistogram.mask()
QWidget.mask() -> QRegion
- LevelsHistogram.maximumHeight()
QWidget.maximumHeight() -> int
- LevelsHistogram.maximumSize()
QWidget.maximumSize() -> QSize
- LevelsHistogram.maximumWidth()
QWidget.maximumWidth() -> int
- LevelsHistogram.metaObject()
QObject.metaObject() -> QMetaObject
- LevelsHistogram.midLineWidth()
QFrame.midLineWidth() -> int
- LevelsHistogram.minimumHeight()
QWidget.minimumHeight() -> int
- LevelsHistogram.minimumSize()
QWidget.minimumSize() -> QSize
- LevelsHistogram.minimumWidth()
QWidget.minimumWidth() -> int
- LevelsHistogram.mouseDoubleClickEvent(event)
Reimplement QWidget method
- LevelsHistogram.mouseGrabber()
QWidget.mouseGrabber() -> QWidget
- LevelsHistogram.move()
QWidget.move(QPoint) QWidget.move(int, int)
- LevelsHistogram.moveToThread()
QObject.moveToThread(QThread)
- LevelsHistogram.move_down(item_list)
Move item(s) down, i.e. to the background (swap item with the previous item in z-order)
item: plot item or list of plot items
Return True if items have been moved effectively
- LevelsHistogram.move_up(item_list)
Move item(s) up, i.e. to the foreground (swap item with the next item in z-order)
item: plot item or list of plot items
Return True if items have been moved effectively
- LevelsHistogram.nativeParentWidget()
QWidget.nativeParentWidget() -> QWidget
- LevelsHistogram.nextInFocusChain()
QWidget.nextInFocusChain() -> QWidget
- LevelsHistogram.normalGeometry()
QWidget.normalGeometry() -> QRect
- LevelsHistogram.numColors()
QPaintDevice.numColors() -> int
- LevelsHistogram.objectName()
QObject.objectName() -> QString
- LevelsHistogram.overrideWindowFlags()
QWidget.overrideWindowFlags(Qt.WindowFlags)
- LevelsHistogram.overrideWindowState()
QWidget.overrideWindowState(Qt.WindowStates)
- LevelsHistogram.paintEngine()
QWidget.paintEngine() -> QPaintEngine
- LevelsHistogram.paintingActive()
QPaintDevice.paintingActive() -> bool
- LevelsHistogram.palette()
QWidget.palette() -> QPalette
- LevelsHistogram.parent()
QObject.parent() -> QObject
- LevelsHistogram.parentWidget()
QWidget.parentWidget() -> QWidget
- LevelsHistogram.physicalDpiX()
QPaintDevice.physicalDpiX() -> int
- LevelsHistogram.physicalDpiY()
QPaintDevice.physicalDpiY() -> int
- LevelsHistogram.pos()
QWidget.pos() -> QPoint
- LevelsHistogram.previousInFocusChain()
QWidget.previousInFocusChain() -> QWidget
- LevelsHistogram.property()
QObject.property(str) -> QVariant
- LevelsHistogram.pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- LevelsHistogram.raise_()
QWidget.raise_()
- LevelsHistogram.read_axes_styles(section, options)
Read axes styles from section and options (one option for each axis in the order left, right, bottom, top)
Skip axis if option is None
- LevelsHistogram.rect()
QWidget.rect() -> QRect
- LevelsHistogram.releaseKeyboard()
QWidget.releaseKeyboard()
- LevelsHistogram.releaseMouse()
QWidget.releaseMouse()
- LevelsHistogram.releaseShortcut()
QWidget.releaseShortcut(int)
- LevelsHistogram.removeAction()
QWidget.removeAction(QAction)
- LevelsHistogram.removeEventFilter()
QObject.removeEventFilter(QObject)
- LevelsHistogram.render()
QWidget.render(QPaintDevice, QPoint targetOffset=QPoint(), QRegion sourceRegion=QRegion(), QWidget.RenderFlags flags=QWidget.DrawWindowBackground|QWidget.DrawChildren) QWidget.render(QPainter, QPoint targetOffset=QPoint(), QRegion sourceRegion=QRegion(), QWidget.RenderFlags flags=QWidget.DrawWindowBackground|QWidget.DrawChildren)
- LevelsHistogram.repaint()
QWidget.repaint() QWidget.repaint(int, int, int, int) QWidget.repaint(QRect) QWidget.repaint(QRegion)
- LevelsHistogram.resize()
QWidget.resize(QSize) QWidget.resize(int, int)
- LevelsHistogram.restoreGeometry()
QWidget.restoreGeometry(QByteArray) -> bool
- LevelsHistogram.restore_items(iofile)
- Restore items from file using the pickle protocol
- iofile: file object or filename
- LevelsHistogram.saveGeometry()
QWidget.saveGeometry() -> QByteArray
- LevelsHistogram.save_items(iofile, selected=False)
- Save (serializable) items to file using the pickle protocol
- iofile: file object or filename
- selected=False: if True, will save only selected items
- LevelsHistogram.scroll()
QWidget.scroll(int, int) QWidget.scroll(int, int, QRect)
- LevelsHistogram.select_all()
Select all selectable items
- LevelsHistogram.select_item(item)
Select item
- LevelsHistogram.select_some_items(items)
Select items
- LevelsHistogram.serialize(writer, selected=False)
- Save (serializable) items to HDF5 file:
- writer: guidata.hdf5io.HDF5Writer object
- selected=False: if True, will save only selected items
See also guiqwt.baseplot.BasePlot.restore_items_from_hdf5()
- LevelsHistogram.setAcceptDrops()
QWidget.setAcceptDrops(bool)
- LevelsHistogram.setAttribute()
QWidget.setAttribute(Qt.WidgetAttribute, bool on=True)
- LevelsHistogram.setAutoFillBackground()
QWidget.setAutoFillBackground(bool)
- LevelsHistogram.setBackgroundRole()
QWidget.setBackgroundRole(QPalette.ColorRole)
- LevelsHistogram.setBaseSize()
QWidget.setBaseSize(int, int) QWidget.setBaseSize(QSize)
- LevelsHistogram.setContentsMargins()
QWidget.setContentsMargins(int, int, int, int) QWidget.setContentsMargins(QMargins)
- LevelsHistogram.setContextMenuPolicy()
QWidget.setContextMenuPolicy(Qt.ContextMenuPolicy)
- LevelsHistogram.setCursor()
QWidget.setCursor(QCursor)
- LevelsHistogram.setDisabled()
QWidget.setDisabled(bool)
- LevelsHistogram.setEnabled()
QWidget.setEnabled(bool)
- LevelsHistogram.setFixedHeight()
QWidget.setFixedHeight(int)
- LevelsHistogram.setFixedSize()
QWidget.setFixedSize(QSize) QWidget.setFixedSize(int, int)
- LevelsHistogram.setFixedWidth()
QWidget.setFixedWidth(int)
- LevelsHistogram.setFocus()
QWidget.setFocus() QWidget.setFocus(Qt.FocusReason)
- LevelsHistogram.setFocusPolicy()
QWidget.setFocusPolicy(Qt.FocusPolicy)
- LevelsHistogram.setFocusProxy()
QWidget.setFocusProxy(QWidget)
- LevelsHistogram.setFont()
QWidget.setFont(QFont)
- LevelsHistogram.setForegroundRole()
QWidget.setForegroundRole(QPalette.ColorRole)
- LevelsHistogram.setFrameRect()
QFrame.setFrameRect(QRect)
- LevelsHistogram.setFrameShadow()
QFrame.setFrameShadow(QFrame.Shadow)
- LevelsHistogram.setFrameShape()
QFrame.setFrameShape(QFrame.Shape)
- LevelsHistogram.setFrameStyle()
QFrame.setFrameStyle(int)
- LevelsHistogram.setGeometry()
QWidget.setGeometry(QRect) QWidget.setGeometry(int, int, int, int)
- LevelsHistogram.setGraphicsEffect()
QWidget.setGraphicsEffect(QGraphicsEffect)
- LevelsHistogram.setHidden()
QWidget.setHidden(bool)
- LevelsHistogram.setInputContext()
QWidget.setInputContext(QInputContext)
- LevelsHistogram.setInputMethodHints()
QWidget.setInputMethodHints(Qt.InputMethodHints)
- LevelsHistogram.setLayout()
QWidget.setLayout(QLayout)
- LevelsHistogram.setLayoutDirection()
QWidget.setLayoutDirection(Qt.LayoutDirection)
- LevelsHistogram.setLineWidth()
QFrame.setLineWidth(int)
- LevelsHistogram.setLocale()
QWidget.setLocale(QLocale)
- LevelsHistogram.setMask()
QWidget.setMask(QBitmap) QWidget.setMask(QRegion)
- LevelsHistogram.setMaximumHeight()
QWidget.setMaximumHeight(int)
- LevelsHistogram.setMaximumSize()
QWidget.setMaximumSize(int, int) QWidget.setMaximumSize(QSize)
- LevelsHistogram.setMaximumWidth()
QWidget.setMaximumWidth(int)
- LevelsHistogram.setMidLineWidth()
QFrame.setMidLineWidth(int)
- LevelsHistogram.setMinimumHeight()
QWidget.setMinimumHeight(int)
- LevelsHistogram.setMinimumSize()
QWidget.setMinimumSize(int, int) QWidget.setMinimumSize(QSize)
- LevelsHistogram.setMinimumWidth()
QWidget.setMinimumWidth(int)
- LevelsHistogram.setMouseTracking()
QWidget.setMouseTracking(bool)
- LevelsHistogram.setObjectName()
QObject.setObjectName(QString)
- LevelsHistogram.setPalette()
QWidget.setPalette(QPalette)
- LevelsHistogram.setParent()
QWidget.setParent(QWidget) QWidget.setParent(QWidget, Qt.WindowFlags)
- LevelsHistogram.setProperty()
QObject.setProperty(str, QVariant) -> bool
- LevelsHistogram.setShortcutAutoRepeat()
QWidget.setShortcutAutoRepeat(int, bool enabled=True)
- LevelsHistogram.setShortcutEnabled()
QWidget.setShortcutEnabled(int, bool enabled=True)
- LevelsHistogram.setShown()
QWidget.setShown(bool)
- LevelsHistogram.setSizeIncrement()
QWidget.setSizeIncrement(int, int) QWidget.setSizeIncrement(QSize)
- LevelsHistogram.setSizePolicy()
QWidget.setSizePolicy(QSizePolicy) QWidget.setSizePolicy(QSizePolicy.Policy, QSizePolicy.Policy)
- LevelsHistogram.setStatusTip()
QWidget.setStatusTip(QString)
- LevelsHistogram.setStyle()
QWidget.setStyle(QStyle)
- LevelsHistogram.setStyleSheet()
QWidget.setStyleSheet(QString)
- LevelsHistogram.setTabOrder()
QWidget.setTabOrder(QWidget, QWidget)
- LevelsHistogram.setToolTip()
QWidget.setToolTip(QString)
- LevelsHistogram.setUpdatesEnabled()
QWidget.setUpdatesEnabled(bool)
- LevelsHistogram.setVisible()
QWidget.setVisible(bool)
- LevelsHistogram.setWhatsThis()
QWidget.setWhatsThis(QString)
- LevelsHistogram.setWindowFilePath()
QWidget.setWindowFilePath(QString)
- LevelsHistogram.setWindowFlags()
QWidget.setWindowFlags(Qt.WindowFlags)
- LevelsHistogram.setWindowIcon()
QWidget.setWindowIcon(QIcon)
- LevelsHistogram.setWindowIconText()
QWidget.setWindowIconText(QString)
- LevelsHistogram.setWindowModality()
QWidget.setWindowModality(Qt.WindowModality)
- LevelsHistogram.setWindowModified()
QWidget.setWindowModified(bool)
- LevelsHistogram.setWindowOpacity()
QWidget.setWindowOpacity(float)
- LevelsHistogram.setWindowRole()
QWidget.setWindowRole(QString)
- LevelsHistogram.setWindowState()
QWidget.setWindowState(Qt.WindowStates)
- LevelsHistogram.setWindowTitle()
QWidget.setWindowTitle(QString)
- LevelsHistogram.set_active_item(item)
Override base set_active_item to change the grid’s axes according to the selected item
- LevelsHistogram.set_antialiasing(checked)
Toggle curve antialiasing
- LevelsHistogram.set_axis_color(axis_id, color)
Set axis color color: color name (string) or QColor instance
- LevelsHistogram.set_axis_direction(axis_id, reverse=False)
- Set axis direction of increasing values
axis_id: axis id (BasePlot.Y_LEFT, BasePlot.X_BOTTOM, ...) or string: ‘bottom’, ‘left’, ‘top’ or ‘right’
- reverse: False (default)
- x-axis values increase from left to right
- y-axis values increase from bottom to top
- reverse: True
- x-axis values increase from right to left
- y-axis values increase from top to bottom
- LevelsHistogram.set_axis_font(axis_id, font)
Set axis font
- LevelsHistogram.set_axis_limits(axis_id, vmin, vmax, stepsize=0)
Set axis limits (minimum and maximum values)
- LevelsHistogram.set_axis_scale(axis_id, scale, autoscale=True)
Set axis scale Example: self.set_axis_scale(curve.yAxis(), ‘lin’)
- LevelsHistogram.set_axis_ticks(axis_id, nmajor=None, nminor=None)
Set axis maximum number of major ticks and maximum of minor ticks
- LevelsHistogram.set_axis_title(axis_id, text)
Set axis title
- LevelsHistogram.set_axis_unit(axis_id, text)
Set axis unit
- LevelsHistogram.set_full_range()[source]
Set range bounds to image min/max levels
- LevelsHistogram.set_item_visible(item, state, notify=True, replot=True)
Show/hide item and emit a SIG_ITEMS_CHANGED signal
- LevelsHistogram.set_items(*args)
Utility function used to quickly setup a plot with a set of items
- LevelsHistogram.set_items_readonly(state)
Set all items readonly state to state Default item’s readonly state: False (items may be deleted)
- LevelsHistogram.set_manager(manager, plot_id)
Set the associated guiqwt.plot.PlotManager instance
- LevelsHistogram.set_plot_limits(x0, x1, y0, y1, xaxis='bottom', yaxis='left')
Set plot scale limits
- LevelsHistogram.set_pointer(pointer_type)
Set pointer. Valid values of pointer_type:
- None: disable pointer
- “canvas”: enable canvas pointer
- “curve”: enable on-curve pointer
- LevelsHistogram.set_scales(xscale, yscale)
Set active curve scales Example: self.set_scales(‘lin’, ‘lin’)
- LevelsHistogram.set_title(title)
Set plot title
- LevelsHistogram.set_titles(title=None, xlabel=None, ylabel=None, xunit=None, yunit=None)
- Set plot and axes titles at once
- title: plot title
- xlabel: (bottom axis title, top axis title) or bottom axis title only
- ylabel: (left axis title, right axis title) or left axis title only
- xunit: (bottom axis unit, top axis unit) or bottom axis unit only
- yunit: (left axis unit, right axis unit) or left axis unit only
- LevelsHistogram.show()
QWidget.show()
- LevelsHistogram.showEvent(event)
Reimplement Qwt method
- LevelsHistogram.showFullScreen()
QWidget.showFullScreen()
- LevelsHistogram.showMaximized()
QWidget.showMaximized()
- LevelsHistogram.showMinimized()
QWidget.showMinimized()
- LevelsHistogram.showNormal()
QWidget.showNormal()
- LevelsHistogram.show_items(items=None, item_type=None)
Show items (if items is None, show all items)
- LevelsHistogram.signalsBlocked()
QObject.signalsBlocked() -> bool
- LevelsHistogram.size()
QWidget.size() -> QSize
- LevelsHistogram.sizeHint()
Preferred size
- LevelsHistogram.sizeIncrement()
QWidget.sizeIncrement() -> QSize
- LevelsHistogram.sizePolicy()
QWidget.sizePolicy() -> QSizePolicy
- LevelsHistogram.stackUnder()
QWidget.stackUnder(QWidget)
- LevelsHistogram.startTimer()
QObject.startTimer(int) -> int
- LevelsHistogram.statusTip()
QWidget.statusTip() -> QString
- LevelsHistogram.style()
QWidget.style() -> QStyle
- LevelsHistogram.styleSheet()
QWidget.styleSheet() -> QString
- LevelsHistogram.testAttribute()
QWidget.testAttribute(Qt.WidgetAttribute) -> bool
- LevelsHistogram.thread()
QObject.thread() -> QThread
- LevelsHistogram.toolTip()
QWidget.toolTip() -> QString
- LevelsHistogram.topLevelWidget()
QWidget.topLevelWidget() -> QWidget
- LevelsHistogram.tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- LevelsHistogram.trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- LevelsHistogram.underMouse()
QWidget.underMouse() -> bool
- LevelsHistogram.ungrabGesture()
QWidget.ungrabGesture(Qt.GestureType)
- LevelsHistogram.unselect_all()
Unselect all selected items
- LevelsHistogram.unselect_item(item)
Unselect item
- LevelsHistogram.unsetCursor()
QWidget.unsetCursor()
- LevelsHistogram.unsetLayoutDirection()
QWidget.unsetLayoutDirection()
- LevelsHistogram.unsetLocale()
QWidget.unsetLocale()
- LevelsHistogram.update()
QWidget.update() QWidget.update(QRect) QWidget.update(QRegion) QWidget.update(int, int, int, int)
- LevelsHistogram.updateGeometry()
QWidget.updateGeometry()
- LevelsHistogram.update_all_axes_styles()
Update all axes styles
- LevelsHistogram.update_axis_style(axis_id)
Update axis style
- LevelsHistogram.updatesEnabled()
QWidget.updatesEnabled() -> bool
- LevelsHistogram.visibleRegion()
QWidget.visibleRegion() -> QRegion
- LevelsHistogram.whatsThis()
QWidget.whatsThis() -> QString
- LevelsHistogram.width()
QWidget.width() -> int
- LevelsHistogram.widthMM()
QPaintDevice.widthMM() -> int
- LevelsHistogram.winId()
QWidget.winId() -> sip.voidptr
- LevelsHistogram.window()
QWidget.window() -> QWidget
- LevelsHistogram.windowFilePath()
QWidget.windowFilePath() -> QString
- LevelsHistogram.windowFlags()
QWidget.windowFlags() -> Qt.WindowFlags
- LevelsHistogram.windowIcon()
QWidget.windowIcon() -> QIcon
- LevelsHistogram.windowIconText()
QWidget.windowIconText() -> QString
- LevelsHistogram.windowModality()
QWidget.windowModality() -> Qt.WindowModality
- LevelsHistogram.windowOpacity()
QWidget.windowOpacity() -> float
- LevelsHistogram.windowRole()
QWidget.windowRole() -> QString
- LevelsHistogram.windowState()
QWidget.windowState() -> Qt.WindowStates
- LevelsHistogram.windowTitle()
QWidget.windowTitle() -> QString
- LevelsHistogram.windowType()
QWidget.windowType() -> Qt.WindowType
- LevelsHistogram.x()
QWidget.x() -> int
- LevelsHistogram.y()
QWidget.y() -> int
guiqwt.cross_section
- The cross_section module provides cross section related objects:
- guiqwt.cross_section.XCrossSection: the X-axis cross-section panel
- guiqwt.cross_section.YCrossSection: the Y-axis cross-section panel
- and other related objects which are exclusively used by the cross-section panels
Example
Simple cross-section demo:
# -*- coding: utf-8 -*-
#
# Copyright © 2009-2010 CEA
# Pierre Raybaut
# Licensed under the terms of the CECILL License
# (see guiqwt/__init__.py for details)
"""Renders a cross section chosen by a cross marker"""
SHOW = True # Show test in GUI-based test launcher
import os.path as osp, numpy as np
from guiqwt.plot import ImageDialog
from guiqwt.builder import make
def create_window():
win = ImageDialog(edit=False, toolbar=True, wintitle="Cross sections test",
options=dict(show_xsection=True, show_ysection=True))
win.resize(600, 600)
return win
def test():
"""Test"""
# -- Create QApplication
import guidata
_app = guidata.qapplication()
# --
filename = osp.join(osp.dirname(__file__), "brain.png")
win = create_window()
image = make.image(filename=filename, colormap="bone")
data2 = np.array(image.data.T[200:], copy=True)
image2 = make.image(data2, title="Modified", alpha_mask=True)
plot = win.get_plot()
plot.add_item(image)
plot.add_item(image2, z=1)
win.exec_()
if __name__ == "__main__":
test()
Reference
- class guiqwt.cross_section.XCrossSection(parent=None)[source]
X-axis cross section widget
- CrossSectionPlotKlass
alias of XCrossSectionPlot
- class RenderFlags
QWidget.RenderFlags(QWidget.RenderFlags) QWidget.RenderFlags(int) QWidget.RenderFlags()
- XCrossSection.acceptDrops()
QWidget.acceptDrops() -> bool
- XCrossSection.actionEvent()
QWidget.actionEvent(QActionEvent)
- XCrossSection.actions()
QWidget.actions() -> list-of-QAction
- XCrossSection.activateWindow()
QWidget.activateWindow()
- XCrossSection.addAction()
QWidget.addAction(QAction)
- XCrossSection.addActions()
QWidget.addActions(list-of-QAction)
- XCrossSection.adjustSize()
QWidget.adjustSize()
- XCrossSection.autoFillBackground()
QWidget.autoFillBackground() -> bool
- XCrossSection.backgroundRole()
QWidget.backgroundRole() -> QPalette.ColorRole
- XCrossSection.baseSize()
QWidget.baseSize() -> QSize
- XCrossSection.blockSignals()
QObject.blockSignals(bool) -> bool
- XCrossSection.changeEvent()
QWidget.changeEvent(QEvent)
- XCrossSection.childAt()
QWidget.childAt(QPoint) -> QWidget QWidget.childAt(int, int) -> QWidget
- XCrossSection.children()
QObject.children() -> list-of-QObject
- XCrossSection.childrenRect()
QWidget.childrenRect() -> QRect
- XCrossSection.childrenRegion()
QWidget.childrenRegion() -> QRegion
- XCrossSection.clearFocus()
QWidget.clearFocus()
- XCrossSection.clearMask()
QWidget.clearMask()
- XCrossSection.close()
QWidget.close() -> bool
- XCrossSection.colorCount()
QPaintDevice.colorCount() -> int
- XCrossSection.configure_panel()
Configure panel
- XCrossSection.connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- XCrossSection.contentsMargins()
QWidget.contentsMargins() -> QMargins
- XCrossSection.contentsRect()
QWidget.contentsRect() -> QRect
- XCrossSection.contextMenuEvent()
QWidget.contextMenuEvent(QContextMenuEvent)
- XCrossSection.contextMenuPolicy()
QWidget.contextMenuPolicy() -> Qt.ContextMenuPolicy
- XCrossSection.create()
QWidget.create(sip.voidptr window=None, bool initializeWindow=True, bool destroyOldWindow=True)
- XCrossSection.create_dockwidget(title)
Add to parent QMainWindow as a dock widget
- XCrossSection.cs_curve_has_changed(curve)
Cross section curve has just changed
- XCrossSection.cursor()
QWidget.cursor() -> QCursor
- XCrossSection.customContextMenuRequested
QWidget.customContextMenuRequested[QPoint] [signal]
- XCrossSection.deleteLater()
QObject.deleteLater()
- XCrossSection.depth()
QPaintDevice.depth() -> int
- XCrossSection.destroy()
QWidget.destroy(bool destroyWindow=True, bool destroySubWindows=True)
- XCrossSection.destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- XCrossSection.devType()
QWidget.devType() -> int
- XCrossSection.disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- XCrossSection.dragEnterEvent()
QWidget.dragEnterEvent(QDragEnterEvent)
- XCrossSection.dragLeaveEvent()
QWidget.dragLeaveEvent(QDragLeaveEvent)
- XCrossSection.dragMoveEvent()
QWidget.dragMoveEvent(QDragMoveEvent)
- XCrossSection.dropEvent()
QWidget.dropEvent(QDropEvent)
- XCrossSection.dumpObjectInfo()
QObject.dumpObjectInfo()
- XCrossSection.dumpObjectTree()
QObject.dumpObjectTree()
- XCrossSection.dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- XCrossSection.effectiveWinId()
QWidget.effectiveWinId() -> sip.voidptr
- XCrossSection.emit()
QObject.emit(SIGNAL(), ...)
- XCrossSection.enabledChange()
QWidget.enabledChange(bool)
- XCrossSection.ensurePolished()
QWidget.ensurePolished()
- XCrossSection.enterEvent()
QWidget.enterEvent(QEvent)
- XCrossSection.event()
QWidget.event(QEvent) -> bool
- XCrossSection.eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- XCrossSection.find()
QWidget.find(sip.voidptr) -> QWidget
- XCrossSection.findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- XCrossSection.findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- XCrossSection.focusInEvent()
QWidget.focusInEvent(QFocusEvent)
- XCrossSection.focusNextChild()
QWidget.focusNextChild() -> bool
- XCrossSection.focusNextPrevChild()
QWidget.focusNextPrevChild(bool) -> bool
- XCrossSection.focusOutEvent()
QWidget.focusOutEvent(QFocusEvent)
- XCrossSection.focusPolicy()
QWidget.focusPolicy() -> Qt.FocusPolicy
- XCrossSection.focusPreviousChild()
QWidget.focusPreviousChild() -> bool
- XCrossSection.focusProxy()
QWidget.focusProxy() -> QWidget
- XCrossSection.focusWidget()
QWidget.focusWidget() -> QWidget
- XCrossSection.font()
QWidget.font() -> QFont
- XCrossSection.fontChange()
QWidget.fontChange(QFont)
- XCrossSection.fontInfo()
QWidget.fontInfo() -> QFontInfo
- XCrossSection.fontMetrics()
QWidget.fontMetrics() -> QFontMetrics
- XCrossSection.foregroundRole()
QWidget.foregroundRole() -> QPalette.ColorRole
- XCrossSection.frameGeometry()
QWidget.frameGeometry() -> QRect
- XCrossSection.frameSize()
QWidget.frameSize() -> QSize
- XCrossSection.geometry()
QWidget.geometry() -> QRect
- XCrossSection.getContentsMargins()
QWidget.getContentsMargins() -> (int, int, int, int)
- XCrossSection.grabGesture()
QWidget.grabGesture(Qt.GestureType, Qt.GestureFlags flags=Qt.GestureFlags(0))
- XCrossSection.grabKeyboard()
QWidget.grabKeyboard()
- XCrossSection.grabMouse()
QWidget.grabMouse() QWidget.grabMouse(QCursor)
- XCrossSection.grabShortcut()
QWidget.grabShortcut(QKeySequence, Qt.ShortcutContext context=Qt.WindowShortcut) -> int
- XCrossSection.graphicsEffect()
QWidget.graphicsEffect() -> QGraphicsEffect
- XCrossSection.graphicsProxyWidget()
QWidget.graphicsProxyWidget() -> QGraphicsProxyWidget
- XCrossSection.hasFocus()
QWidget.hasFocus() -> bool
- XCrossSection.hasMouseTracking()
QWidget.hasMouseTracking() -> bool
- XCrossSection.height()
QWidget.height() -> int
- XCrossSection.heightForWidth()
QWidget.heightForWidth(int) -> int
- XCrossSection.heightMM()
QPaintDevice.heightMM() -> int
- XCrossSection.hide()
QWidget.hide()
- XCrossSection.inherits()
QObject.inherits(str) -> bool
- XCrossSection.inputContext()
QWidget.inputContext() -> QInputContext
- XCrossSection.inputMethodEvent()
QWidget.inputMethodEvent(QInputMethodEvent)
- XCrossSection.inputMethodHints()
QWidget.inputMethodHints() -> Qt.InputMethodHints
- XCrossSection.inputMethodQuery()
QWidget.inputMethodQuery(Qt.InputMethodQuery) -> QVariant
- XCrossSection.insertAction()
QWidget.insertAction(QAction, QAction)
- XCrossSection.insertActions()
QWidget.insertActions(QAction, list-of-QAction)
- XCrossSection.installEventFilter()
QObject.installEventFilter(QObject)
- XCrossSection.isActiveWindow()
QWidget.isActiveWindow() -> bool
- XCrossSection.isAncestorOf()
QWidget.isAncestorOf(QWidget) -> bool
- XCrossSection.isEnabled()
QWidget.isEnabled() -> bool
- XCrossSection.isEnabledTo()
QWidget.isEnabledTo(QWidget) -> bool
- XCrossSection.isEnabledToTLW()
QWidget.isEnabledToTLW() -> bool
- XCrossSection.isFullScreen()
QWidget.isFullScreen() -> bool
- XCrossSection.isHidden()
QWidget.isHidden() -> bool
- XCrossSection.isLeftToRight()
QWidget.isLeftToRight() -> bool
- XCrossSection.isMaximized()
QWidget.isMaximized() -> bool
- XCrossSection.isMinimized()
QWidget.isMinimized() -> bool
- XCrossSection.isModal()
QWidget.isModal() -> bool
- XCrossSection.isRightToLeft()
QWidget.isRightToLeft() -> bool
- XCrossSection.isTopLevel()
QWidget.isTopLevel() -> bool
- XCrossSection.isVisible()
QWidget.isVisible() -> bool
- XCrossSection.isVisibleTo()
QWidget.isVisibleTo(QWidget) -> bool
- XCrossSection.isWidgetType()
QObject.isWidgetType() -> bool
- XCrossSection.isWindow()
QWidget.isWindow() -> bool
- XCrossSection.isWindowModified()
QWidget.isWindowModified() -> bool
- XCrossSection.keyPressEvent()
QWidget.keyPressEvent(QKeyEvent)
- XCrossSection.keyReleaseEvent()
QWidget.keyReleaseEvent(QKeyEvent)
- XCrossSection.keyboardGrabber()
QWidget.keyboardGrabber() -> QWidget
- XCrossSection.killTimer()
QObject.killTimer(int)
- XCrossSection.languageChange()
QWidget.languageChange()
- XCrossSection.layout()
QWidget.layout() -> QLayout
- XCrossSection.layoutDirection()
QWidget.layoutDirection() -> Qt.LayoutDirection
- XCrossSection.leaveEvent()
QWidget.leaveEvent(QEvent)
- XCrossSection.locale()
QWidget.locale() -> QLocale
- XCrossSection.logicalDpiX()
QPaintDevice.logicalDpiX() -> int
- XCrossSection.logicalDpiY()
QPaintDevice.logicalDpiY() -> int
- XCrossSection.lower()
QWidget.lower()
- XCrossSection.mapFrom()
QWidget.mapFrom(QWidget, QPoint) -> QPoint
- XCrossSection.mapFromGlobal()
QWidget.mapFromGlobal(QPoint) -> QPoint
- XCrossSection.mapFromParent()
QWidget.mapFromParent(QPoint) -> QPoint
- XCrossSection.mapTo()
QWidget.mapTo(QWidget, QPoint) -> QPoint
- XCrossSection.mapToGlobal()
QWidget.mapToGlobal(QPoint) -> QPoint
- XCrossSection.mapToParent()
QWidget.mapToParent(QPoint) -> QPoint
- XCrossSection.mask()
QWidget.mask() -> QRegion
- XCrossSection.maximumHeight()
QWidget.maximumHeight() -> int
- XCrossSection.maximumSize()
QWidget.maximumSize() -> QSize
- XCrossSection.maximumWidth()
QWidget.maximumWidth() -> int
- XCrossSection.metaObject()
QObject.metaObject() -> QMetaObject
- XCrossSection.metric()
QWidget.metric(QPaintDevice.PaintDeviceMetric) -> int
- XCrossSection.minimumHeight()
QWidget.minimumHeight() -> int
- XCrossSection.minimumSize()
QWidget.minimumSize() -> QSize
- XCrossSection.minimumSizeHint()
QWidget.minimumSizeHint() -> QSize
- XCrossSection.minimumWidth()
QWidget.minimumWidth() -> int
- XCrossSection.mouseDoubleClickEvent()
QWidget.mouseDoubleClickEvent(QMouseEvent)
- XCrossSection.mouseGrabber()
QWidget.mouseGrabber() -> QWidget
- XCrossSection.mouseMoveEvent()
QWidget.mouseMoveEvent(QMouseEvent)
- XCrossSection.mousePressEvent()
QWidget.mousePressEvent(QMouseEvent)
- XCrossSection.mouseReleaseEvent()
QWidget.mouseReleaseEvent(QMouseEvent)
- XCrossSection.move()
QWidget.move(QPoint) QWidget.move(int, int)
- XCrossSection.moveEvent()
QWidget.moveEvent(QMoveEvent)
- XCrossSection.moveToThread()
QObject.moveToThread(QThread)
- XCrossSection.nativeParentWidget()
QWidget.nativeParentWidget() -> QWidget
- XCrossSection.nextInFocusChain()
QWidget.nextInFocusChain() -> QWidget
- XCrossSection.normalGeometry()
QWidget.normalGeometry() -> QRect
- XCrossSection.numColors()
QPaintDevice.numColors() -> int
- XCrossSection.objectName()
QObject.objectName() -> QString
- XCrossSection.overrideWindowFlags()
QWidget.overrideWindowFlags(Qt.WindowFlags)
- XCrossSection.overrideWindowState()
QWidget.overrideWindowState(Qt.WindowStates)
- XCrossSection.paintEngine()
QWidget.paintEngine() -> QPaintEngine
- XCrossSection.paintEvent()
QWidget.paintEvent(QPaintEvent)
- XCrossSection.paintingActive()
QPaintDevice.paintingActive() -> bool
- XCrossSection.palette()
QWidget.palette() -> QPalette
- XCrossSection.paletteChange()
QWidget.paletteChange(QPalette)
- XCrossSection.parent()
QObject.parent() -> QObject
- XCrossSection.parentWidget()
QWidget.parentWidget() -> QWidget
- XCrossSection.physicalDpiX()
QPaintDevice.physicalDpiX() -> int
- XCrossSection.physicalDpiY()
QPaintDevice.physicalDpiY() -> int
- XCrossSection.pos()
QWidget.pos() -> QPoint
- XCrossSection.previousInFocusChain()
QWidget.previousInFocusChain() -> QWidget
- XCrossSection.property()
QObject.property(str) -> QVariant
- XCrossSection.pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- XCrossSection.raise_()
QWidget.raise_()
- XCrossSection.rect()
QWidget.rect() -> QRect
- XCrossSection.register_panel(manager)
Register panel to plot manager
- XCrossSection.releaseKeyboard()
QWidget.releaseKeyboard()
- XCrossSection.releaseMouse()
QWidget.releaseMouse()
- XCrossSection.releaseShortcut()
QWidget.releaseShortcut(int)
- XCrossSection.removeAction()
QWidget.removeAction(QAction)
- XCrossSection.removeEventFilter()
QObject.removeEventFilter(QObject)
- XCrossSection.render()
QWidget.render(QPaintDevice, QPoint targetOffset=QPoint(), QRegion sourceRegion=QRegion(), QWidget.RenderFlags flags=QWidget.DrawWindowBackground|QWidget.DrawChildren) QWidget.render(QPainter, QPoint targetOffset=QPoint(), QRegion sourceRegion=QRegion(), QWidget.RenderFlags flags=QWidget.DrawWindowBackground|QWidget.DrawChildren)
- XCrossSection.repaint()
QWidget.repaint() QWidget.repaint(int, int, int, int) QWidget.repaint(QRect) QWidget.repaint(QRegion)
- XCrossSection.resetInputContext()
QWidget.resetInputContext()
- XCrossSection.resize()
QWidget.resize(QSize) QWidget.resize(int, int)
- XCrossSection.resizeEvent()
QWidget.resizeEvent(QResizeEvent)
- XCrossSection.restoreGeometry()
QWidget.restoreGeometry(QByteArray) -> bool
- XCrossSection.saveGeometry()
QWidget.saveGeometry() -> QByteArray
- XCrossSection.scroll()
QWidget.scroll(int, int) QWidget.scroll(int, int, QRect)
- XCrossSection.setAcceptDrops()
QWidget.setAcceptDrops(bool)
- XCrossSection.setAttribute()
QWidget.setAttribute(Qt.WidgetAttribute, bool on=True)
- XCrossSection.setAutoFillBackground()
QWidget.setAutoFillBackground(bool)
- XCrossSection.setBackgroundRole()
QWidget.setBackgroundRole(QPalette.ColorRole)
- XCrossSection.setBaseSize()
QWidget.setBaseSize(int, int) QWidget.setBaseSize(QSize)
- XCrossSection.setContentsMargins()
QWidget.setContentsMargins(int, int, int, int) QWidget.setContentsMargins(QMargins)
- XCrossSection.setContextMenuPolicy()
QWidget.setContextMenuPolicy(Qt.ContextMenuPolicy)
- XCrossSection.setCursor()
QWidget.setCursor(QCursor)
- XCrossSection.setDisabled()
QWidget.setDisabled(bool)
- XCrossSection.setEnabled()
QWidget.setEnabled(bool)
- XCrossSection.setFixedHeight()
QWidget.setFixedHeight(int)
- XCrossSection.setFixedSize()
QWidget.setFixedSize(QSize) QWidget.setFixedSize(int, int)
- XCrossSection.setFixedWidth()
QWidget.setFixedWidth(int)
- XCrossSection.setFocus()
QWidget.setFocus() QWidget.setFocus(Qt.FocusReason)
- XCrossSection.setFocusPolicy()
QWidget.setFocusPolicy(Qt.FocusPolicy)
- XCrossSection.setFocusProxy()
QWidget.setFocusProxy(QWidget)
- XCrossSection.setFont()
QWidget.setFont(QFont)
- XCrossSection.setForegroundRole()
QWidget.setForegroundRole(QPalette.ColorRole)
- XCrossSection.setGeometry()
QWidget.setGeometry(QRect) QWidget.setGeometry(int, int, int, int)
- XCrossSection.setGraphicsEffect()
QWidget.setGraphicsEffect(QGraphicsEffect)
- XCrossSection.setHidden()
QWidget.setHidden(bool)
- XCrossSection.setInputContext()
QWidget.setInputContext(QInputContext)
- XCrossSection.setInputMethodHints()
QWidget.setInputMethodHints(Qt.InputMethodHints)
- XCrossSection.setLayout()
QWidget.setLayout(QLayout)
- XCrossSection.setLayoutDirection()
QWidget.setLayoutDirection(Qt.LayoutDirection)
- XCrossSection.setLocale()
QWidget.setLocale(QLocale)
- XCrossSection.setMask()
QWidget.setMask(QBitmap) QWidget.setMask(QRegion)
- XCrossSection.setMaximumHeight()
QWidget.setMaximumHeight(int)
- XCrossSection.setMaximumSize()
QWidget.setMaximumSize(int, int) QWidget.setMaximumSize(QSize)
- XCrossSection.setMaximumWidth()
QWidget.setMaximumWidth(int)
- XCrossSection.setMinimumHeight()
QWidget.setMinimumHeight(int)
- XCrossSection.setMinimumSize()
QWidget.setMinimumSize(int, int) QWidget.setMinimumSize(QSize)
- XCrossSection.setMinimumWidth()
QWidget.setMinimumWidth(int)
- XCrossSection.setMouseTracking()
QWidget.setMouseTracking(bool)
- XCrossSection.setObjectName()
QObject.setObjectName(QString)
- XCrossSection.setPalette()
QWidget.setPalette(QPalette)
- XCrossSection.setParent()
QWidget.setParent(QWidget) QWidget.setParent(QWidget, Qt.WindowFlags)
- XCrossSection.setProperty()
QObject.setProperty(str, QVariant) -> bool
- XCrossSection.setShortcutAutoRepeat()
QWidget.setShortcutAutoRepeat(int, bool enabled=True)
- XCrossSection.setShortcutEnabled()
QWidget.setShortcutEnabled(int, bool enabled=True)
- XCrossSection.setShown()
QWidget.setShown(bool)
- XCrossSection.setSizeIncrement()
QWidget.setSizeIncrement(int, int) QWidget.setSizeIncrement(QSize)
- XCrossSection.setSizePolicy()
QWidget.setSizePolicy(QSizePolicy) QWidget.setSizePolicy(QSizePolicy.Policy, QSizePolicy.Policy)
- XCrossSection.setStatusTip()
QWidget.setStatusTip(QString)
- XCrossSection.setStyle()
QWidget.setStyle(QStyle)
- XCrossSection.setStyleSheet()
QWidget.setStyleSheet(QString)
- XCrossSection.setTabOrder()
QWidget.setTabOrder(QWidget, QWidget)
- XCrossSection.setToolTip()
QWidget.setToolTip(QString)
- XCrossSection.setUpdatesEnabled()
QWidget.setUpdatesEnabled(bool)
- XCrossSection.setVisible()
QWidget.setVisible(bool)
- XCrossSection.setWhatsThis()
QWidget.setWhatsThis(QString)
- XCrossSection.setWindowFilePath()
QWidget.setWindowFilePath(QString)
- XCrossSection.setWindowFlags()
QWidget.setWindowFlags(Qt.WindowFlags)
- XCrossSection.setWindowIcon()
QWidget.setWindowIcon(QIcon)
- XCrossSection.setWindowIconText()
QWidget.setWindowIconText(QString)
- XCrossSection.setWindowModality()
QWidget.setWindowModality(Qt.WindowModality)
- XCrossSection.setWindowModified()
QWidget.setWindowModified(bool)
- XCrossSection.setWindowOpacity()
QWidget.setWindowOpacity(float)
- XCrossSection.setWindowRole()
QWidget.setWindowRole(QString)
- XCrossSection.setWindowState()
QWidget.setWindowState(Qt.WindowStates)
- XCrossSection.setWindowTitle()
QWidget.setWindowTitle(QString)
- XCrossSection.show()
QWidget.show()
- XCrossSection.showFullScreen()
QWidget.showFullScreen()
- XCrossSection.showMaximized()
QWidget.showMaximized()
- XCrossSection.showMinimized()
QWidget.showMinimized()
- XCrossSection.showNormal()
QWidget.showNormal()
- XCrossSection.signalsBlocked()
QObject.signalsBlocked() -> bool
- XCrossSection.size()
QWidget.size() -> QSize
- XCrossSection.sizeHint()
QWidget.sizeHint() -> QSize
- XCrossSection.sizeIncrement()
QWidget.sizeIncrement() -> QSize
- XCrossSection.sizePolicy()
QWidget.sizePolicy() -> QSizePolicy
- XCrossSection.stackUnder()
QWidget.stackUnder(QWidget)
- XCrossSection.startTimer()
QObject.startTimer(int) -> int
- XCrossSection.statusTip()
QWidget.statusTip() -> QString
- XCrossSection.style()
QWidget.style() -> QStyle
- XCrossSection.styleSheet()
QWidget.styleSheet() -> QString
- XCrossSection.tabletEvent()
QWidget.tabletEvent(QTabletEvent)
- XCrossSection.testAttribute()
QWidget.testAttribute(Qt.WidgetAttribute) -> bool
- XCrossSection.thread()
QObject.thread() -> QThread
- XCrossSection.toolTip()
QWidget.toolTip() -> QString
- XCrossSection.topLevelWidget()
QWidget.topLevelWidget() -> QWidget
- XCrossSection.tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- XCrossSection.trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- XCrossSection.underMouse()
QWidget.underMouse() -> bool
- XCrossSection.ungrabGesture()
QWidget.ungrabGesture(Qt.GestureType)
- XCrossSection.unsetCursor()
QWidget.unsetCursor()
- XCrossSection.unsetLayoutDirection()
QWidget.unsetLayoutDirection()
- XCrossSection.unsetLocale()
QWidget.unsetLocale()
- XCrossSection.update()
QWidget.update() QWidget.update(QRect) QWidget.update(QRegion) QWidget.update(int, int, int, int)
- XCrossSection.updateGeometry()
QWidget.updateGeometry()
- XCrossSection.updateMicroFocus()
QWidget.updateMicroFocus()
- XCrossSection.update_plot(obj=None)
Update cross section curve(s) associated to object obj
obj may be a marker or a rectangular shape (see guiqwt.tools.CrossSectionTool and guiqwt.tools.AverageCrossSectionTool)
If obj is None, update the cross sections of the last active object
- XCrossSection.updatesEnabled()
QWidget.updatesEnabled() -> bool
- XCrossSection.visibility_changed(enable)
DockWidget visibility has changed
- XCrossSection.visibleRegion()
QWidget.visibleRegion() -> QRegion
- XCrossSection.whatsThis()
QWidget.whatsThis() -> QString
- XCrossSection.wheelEvent()
QWidget.wheelEvent(QWheelEvent)
- XCrossSection.width()
QWidget.width() -> int
- XCrossSection.widthMM()
QPaintDevice.widthMM() -> int
- XCrossSection.winEvent()
QWidget.winEvent(MSG) -> (bool, int)
- XCrossSection.winId()
QWidget.winId() -> sip.voidptr
- XCrossSection.window()
QWidget.window() -> QWidget
- XCrossSection.windowActivationChange()
QWidget.windowActivationChange(bool)
- XCrossSection.windowFilePath()
QWidget.windowFilePath() -> QString
- XCrossSection.windowFlags()
QWidget.windowFlags() -> Qt.WindowFlags
- XCrossSection.windowIcon()
QWidget.windowIcon() -> QIcon
- XCrossSection.windowIconText()
QWidget.windowIconText() -> QString
- XCrossSection.windowModality()
QWidget.windowModality() -> Qt.WindowModality
- XCrossSection.windowOpacity()
QWidget.windowOpacity() -> float
- XCrossSection.windowRole()
QWidget.windowRole() -> QString
- XCrossSection.windowState()
QWidget.windowState() -> Qt.WindowStates
- XCrossSection.windowTitle()
QWidget.windowTitle() -> QString
- XCrossSection.windowType()
QWidget.windowType() -> Qt.WindowType
- XCrossSection.x()
QWidget.x() -> int
- XCrossSection.y()
QWidget.y() -> int
- class guiqwt.cross_section.YCrossSection(parent=None, position='right', xsection_pos='top')[source]
Y-axis cross section widget parent (QWidget): parent widget position (string): “left” or “right”
- CrossSectionPlotKlass
alias of YCrossSectionPlot
- class RenderFlags
QWidget.RenderFlags(QWidget.RenderFlags) QWidget.RenderFlags(int) QWidget.RenderFlags()
- YCrossSection.acceptDrops()
QWidget.acceptDrops() -> bool
- YCrossSection.actionEvent()
QWidget.actionEvent(QActionEvent)
- YCrossSection.actions()
QWidget.actions() -> list-of-QAction
- YCrossSection.activateWindow()
QWidget.activateWindow()
- YCrossSection.addAction()
QWidget.addAction(QAction)
- YCrossSection.addActions()
QWidget.addActions(list-of-QAction)
- YCrossSection.adjustSize()
QWidget.adjustSize()
- YCrossSection.autoFillBackground()
QWidget.autoFillBackground() -> bool
- YCrossSection.backgroundRole()
QWidget.backgroundRole() -> QPalette.ColorRole
- YCrossSection.baseSize()
QWidget.baseSize() -> QSize
- YCrossSection.blockSignals()
QObject.blockSignals(bool) -> bool
- YCrossSection.changeEvent()
QWidget.changeEvent(QEvent)
- YCrossSection.childAt()
QWidget.childAt(QPoint) -> QWidget QWidget.childAt(int, int) -> QWidget
- YCrossSection.children()
QObject.children() -> list-of-QObject
- YCrossSection.childrenRect()
QWidget.childrenRect() -> QRect
- YCrossSection.childrenRegion()
QWidget.childrenRegion() -> QRegion
- YCrossSection.clearFocus()
QWidget.clearFocus()
- YCrossSection.clearMask()
QWidget.clearMask()
- YCrossSection.close()
QWidget.close() -> bool
- YCrossSection.colorCount()
QPaintDevice.colorCount() -> int
- YCrossSection.configure_panel()
Configure panel
- YCrossSection.connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- YCrossSection.contentsMargins()
QWidget.contentsMargins() -> QMargins
- YCrossSection.contentsRect()
QWidget.contentsRect() -> QRect
- YCrossSection.contextMenuEvent()
QWidget.contextMenuEvent(QContextMenuEvent)
- YCrossSection.contextMenuPolicy()
QWidget.contextMenuPolicy() -> Qt.ContextMenuPolicy
- YCrossSection.create()
QWidget.create(sip.voidptr window=None, bool initializeWindow=True, bool destroyOldWindow=True)
- YCrossSection.create_dockwidget(title)
Add to parent QMainWindow as a dock widget
- YCrossSection.cs_curve_has_changed(curve)
Cross section curve has just changed
- YCrossSection.cursor()
QWidget.cursor() -> QCursor
- YCrossSection.customContextMenuRequested
QWidget.customContextMenuRequested[QPoint] [signal]
- YCrossSection.deleteLater()
QObject.deleteLater()
- YCrossSection.depth()
QPaintDevice.depth() -> int
- YCrossSection.destroy()
QWidget.destroy(bool destroyWindow=True, bool destroySubWindows=True)
- YCrossSection.destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- YCrossSection.devType()
QWidget.devType() -> int
- YCrossSection.disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- YCrossSection.dragEnterEvent()
QWidget.dragEnterEvent(QDragEnterEvent)
- YCrossSection.dragLeaveEvent()
QWidget.dragLeaveEvent(QDragLeaveEvent)
- YCrossSection.dragMoveEvent()
QWidget.dragMoveEvent(QDragMoveEvent)
- YCrossSection.dropEvent()
QWidget.dropEvent(QDropEvent)
- YCrossSection.dumpObjectInfo()
QObject.dumpObjectInfo()
- YCrossSection.dumpObjectTree()
QObject.dumpObjectTree()
- YCrossSection.dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- YCrossSection.effectiveWinId()
QWidget.effectiveWinId() -> sip.voidptr
- YCrossSection.emit()
QObject.emit(SIGNAL(), ...)
- YCrossSection.enabledChange()
QWidget.enabledChange(bool)
- YCrossSection.ensurePolished()
QWidget.ensurePolished()
- YCrossSection.enterEvent()
QWidget.enterEvent(QEvent)
- YCrossSection.event()
QWidget.event(QEvent) -> bool
- YCrossSection.eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- YCrossSection.find()
QWidget.find(sip.voidptr) -> QWidget
- YCrossSection.findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- YCrossSection.findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- YCrossSection.focusInEvent()
QWidget.focusInEvent(QFocusEvent)
- YCrossSection.focusNextChild()
QWidget.focusNextChild() -> bool
- YCrossSection.focusNextPrevChild()
QWidget.focusNextPrevChild(bool) -> bool
- YCrossSection.focusOutEvent()
QWidget.focusOutEvent(QFocusEvent)
- YCrossSection.focusPolicy()
QWidget.focusPolicy() -> Qt.FocusPolicy
- YCrossSection.focusPreviousChild()
QWidget.focusPreviousChild() -> bool
- YCrossSection.focusProxy()
QWidget.focusProxy() -> QWidget
- YCrossSection.focusWidget()
QWidget.focusWidget() -> QWidget
- YCrossSection.font()
QWidget.font() -> QFont
- YCrossSection.fontChange()
QWidget.fontChange(QFont)
- YCrossSection.fontInfo()
QWidget.fontInfo() -> QFontInfo
- YCrossSection.fontMetrics()
QWidget.fontMetrics() -> QFontMetrics
- YCrossSection.foregroundRole()
QWidget.foregroundRole() -> QPalette.ColorRole
- YCrossSection.frameGeometry()
QWidget.frameGeometry() -> QRect
- YCrossSection.frameSize()
QWidget.frameSize() -> QSize
- YCrossSection.geometry()
QWidget.geometry() -> QRect
- YCrossSection.getContentsMargins()
QWidget.getContentsMargins() -> (int, int, int, int)
- YCrossSection.grabGesture()
QWidget.grabGesture(Qt.GestureType, Qt.GestureFlags flags=Qt.GestureFlags(0))
- YCrossSection.grabKeyboard()
QWidget.grabKeyboard()
- YCrossSection.grabMouse()
QWidget.grabMouse() QWidget.grabMouse(QCursor)
- YCrossSection.grabShortcut()
QWidget.grabShortcut(QKeySequence, Qt.ShortcutContext context=Qt.WindowShortcut) -> int
- YCrossSection.graphicsEffect()
QWidget.graphicsEffect() -> QGraphicsEffect
- YCrossSection.graphicsProxyWidget()
QWidget.graphicsProxyWidget() -> QGraphicsProxyWidget
- YCrossSection.hasFocus()
QWidget.hasFocus() -> bool
- YCrossSection.hasMouseTracking()
QWidget.hasMouseTracking() -> bool
- YCrossSection.height()
QWidget.height() -> int
- YCrossSection.heightForWidth()
QWidget.heightForWidth(int) -> int
- YCrossSection.heightMM()
QPaintDevice.heightMM() -> int
- YCrossSection.hide()
QWidget.hide()
- YCrossSection.inherits()
QObject.inherits(str) -> bool
- YCrossSection.inputContext()
QWidget.inputContext() -> QInputContext
- YCrossSection.inputMethodEvent()
QWidget.inputMethodEvent(QInputMethodEvent)
- YCrossSection.inputMethodHints()
QWidget.inputMethodHints() -> Qt.InputMethodHints
- YCrossSection.inputMethodQuery()
QWidget.inputMethodQuery(Qt.InputMethodQuery) -> QVariant
- YCrossSection.insertAction()
QWidget.insertAction(QAction, QAction)
- YCrossSection.insertActions()
QWidget.insertActions(QAction, list-of-QAction)
- YCrossSection.installEventFilter()
QObject.installEventFilter(QObject)
- YCrossSection.isActiveWindow()
QWidget.isActiveWindow() -> bool
- YCrossSection.isAncestorOf()
QWidget.isAncestorOf(QWidget) -> bool
- YCrossSection.isEnabled()
QWidget.isEnabled() -> bool
- YCrossSection.isEnabledTo()
QWidget.isEnabledTo(QWidget) -> bool
- YCrossSection.isEnabledToTLW()
QWidget.isEnabledToTLW() -> bool
- YCrossSection.isFullScreen()
QWidget.isFullScreen() -> bool
- YCrossSection.isHidden()
QWidget.isHidden() -> bool
- YCrossSection.isLeftToRight()
QWidget.isLeftToRight() -> bool
- YCrossSection.isMaximized()
QWidget.isMaximized() -> bool
- YCrossSection.isMinimized()
QWidget.isMinimized() -> bool
- YCrossSection.isModal()
QWidget.isModal() -> bool
- YCrossSection.isRightToLeft()
QWidget.isRightToLeft() -> bool
- YCrossSection.isTopLevel()
QWidget.isTopLevel() -> bool
- YCrossSection.isVisible()
QWidget.isVisible() -> bool
- YCrossSection.isVisibleTo()
QWidget.isVisibleTo(QWidget) -> bool
- YCrossSection.isWidgetType()
QObject.isWidgetType() -> bool
- YCrossSection.isWindow()
QWidget.isWindow() -> bool
- YCrossSection.isWindowModified()
QWidget.isWindowModified() -> bool
- YCrossSection.keyPressEvent()
QWidget.keyPressEvent(QKeyEvent)
- YCrossSection.keyReleaseEvent()
QWidget.keyReleaseEvent(QKeyEvent)
- YCrossSection.keyboardGrabber()
QWidget.keyboardGrabber() -> QWidget
- YCrossSection.killTimer()
QObject.killTimer(int)
- YCrossSection.languageChange()
QWidget.languageChange()
- YCrossSection.layout()
QWidget.layout() -> QLayout
- YCrossSection.layoutDirection()
QWidget.layoutDirection() -> Qt.LayoutDirection
- YCrossSection.leaveEvent()
QWidget.leaveEvent(QEvent)
- YCrossSection.locale()
QWidget.locale() -> QLocale
- YCrossSection.logicalDpiX()
QPaintDevice.logicalDpiX() -> int
- YCrossSection.logicalDpiY()
QPaintDevice.logicalDpiY() -> int
- YCrossSection.lower()
QWidget.lower()
- YCrossSection.mapFrom()
QWidget.mapFrom(QWidget, QPoint) -> QPoint
- YCrossSection.mapFromGlobal()
QWidget.mapFromGlobal(QPoint) -> QPoint
- YCrossSection.mapFromParent()
QWidget.mapFromParent(QPoint) -> QPoint
- YCrossSection.mapTo()
QWidget.mapTo(QWidget, QPoint) -> QPoint
- YCrossSection.mapToGlobal()
QWidget.mapToGlobal(QPoint) -> QPoint
- YCrossSection.mapToParent()
QWidget.mapToParent(QPoint) -> QPoint
- YCrossSection.mask()
QWidget.mask() -> QRegion
- YCrossSection.maximumHeight()
QWidget.maximumHeight() -> int
- YCrossSection.maximumSize()
QWidget.maximumSize() -> QSize
- YCrossSection.maximumWidth()
QWidget.maximumWidth() -> int
- YCrossSection.metaObject()
QObject.metaObject() -> QMetaObject
- YCrossSection.metric()
QWidget.metric(QPaintDevice.PaintDeviceMetric) -> int
- YCrossSection.minimumHeight()
QWidget.minimumHeight() -> int
- YCrossSection.minimumSize()
QWidget.minimumSize() -> QSize
- YCrossSection.minimumSizeHint()
QWidget.minimumSizeHint() -> QSize
- YCrossSection.minimumWidth()
QWidget.minimumWidth() -> int
- YCrossSection.mouseDoubleClickEvent()
QWidget.mouseDoubleClickEvent(QMouseEvent)
- YCrossSection.mouseGrabber()
QWidget.mouseGrabber() -> QWidget
- YCrossSection.mouseMoveEvent()
QWidget.mouseMoveEvent(QMouseEvent)
- YCrossSection.mousePressEvent()
QWidget.mousePressEvent(QMouseEvent)
- YCrossSection.mouseReleaseEvent()
QWidget.mouseReleaseEvent(QMouseEvent)
- YCrossSection.move()
QWidget.move(QPoint) QWidget.move(int, int)
- YCrossSection.moveEvent()
QWidget.moveEvent(QMoveEvent)
- YCrossSection.moveToThread()
QObject.moveToThread(QThread)
- YCrossSection.nativeParentWidget()
QWidget.nativeParentWidget() -> QWidget
- YCrossSection.nextInFocusChain()
QWidget.nextInFocusChain() -> QWidget
- YCrossSection.normalGeometry()
QWidget.normalGeometry() -> QRect
- YCrossSection.numColors()
QPaintDevice.numColors() -> int
- YCrossSection.objectName()
QObject.objectName() -> QString
- YCrossSection.overrideWindowFlags()
QWidget.overrideWindowFlags(Qt.WindowFlags)
- YCrossSection.overrideWindowState()
QWidget.overrideWindowState(Qt.WindowStates)
- YCrossSection.paintEngine()
QWidget.paintEngine() -> QPaintEngine
- YCrossSection.paintEvent()
QWidget.paintEvent(QPaintEvent)
- YCrossSection.paintingActive()
QPaintDevice.paintingActive() -> bool
- YCrossSection.palette()
QWidget.palette() -> QPalette
- YCrossSection.paletteChange()
QWidget.paletteChange(QPalette)
- YCrossSection.parent()
QObject.parent() -> QObject
- YCrossSection.parentWidget()
QWidget.parentWidget() -> QWidget
- YCrossSection.physicalDpiX()
QPaintDevice.physicalDpiX() -> int
- YCrossSection.physicalDpiY()
QPaintDevice.physicalDpiY() -> int
- YCrossSection.pos()
QWidget.pos() -> QPoint
- YCrossSection.previousInFocusChain()
QWidget.previousInFocusChain() -> QWidget
- YCrossSection.property()
QObject.property(str) -> QVariant
- YCrossSection.pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- YCrossSection.raise_()
QWidget.raise_()
- YCrossSection.rect()
QWidget.rect() -> QRect
- YCrossSection.register_panel(manager)
Register panel to plot manager
- YCrossSection.releaseKeyboard()
QWidget.releaseKeyboard()
- YCrossSection.releaseMouse()
QWidget.releaseMouse()
- YCrossSection.releaseShortcut()
QWidget.releaseShortcut(int)
- YCrossSection.removeAction()
QWidget.removeAction(QAction)
- YCrossSection.removeEventFilter()
QObject.removeEventFilter(QObject)
- YCrossSection.render()
QWidget.render(QPaintDevice, QPoint targetOffset=QPoint(), QRegion sourceRegion=QRegion(), QWidget.RenderFlags flags=QWidget.DrawWindowBackground|QWidget.DrawChildren) QWidget.render(QPainter, QPoint targetOffset=QPoint(), QRegion sourceRegion=QRegion(), QWidget.RenderFlags flags=QWidget.DrawWindowBackground|QWidget.DrawChildren)
- YCrossSection.repaint()
QWidget.repaint() QWidget.repaint(int, int, int, int) QWidget.repaint(QRect) QWidget.repaint(QRegion)
- YCrossSection.resetInputContext()
QWidget.resetInputContext()
- YCrossSection.resize()
QWidget.resize(QSize) QWidget.resize(int, int)
- YCrossSection.resizeEvent()
QWidget.resizeEvent(QResizeEvent)
- YCrossSection.restoreGeometry()
QWidget.restoreGeometry(QByteArray) -> bool
- YCrossSection.saveGeometry()
QWidget.saveGeometry() -> QByteArray
- YCrossSection.scroll()
QWidget.scroll(int, int) QWidget.scroll(int, int, QRect)
- YCrossSection.setAcceptDrops()
QWidget.setAcceptDrops(bool)
- YCrossSection.setAttribute()
QWidget.setAttribute(Qt.WidgetAttribute, bool on=True)
- YCrossSection.setAutoFillBackground()
QWidget.setAutoFillBackground(bool)
- YCrossSection.setBackgroundRole()
QWidget.setBackgroundRole(QPalette.ColorRole)
- YCrossSection.setBaseSize()
QWidget.setBaseSize(int, int) QWidget.setBaseSize(QSize)
- YCrossSection.setContentsMargins()
QWidget.setContentsMargins(int, int, int, int) QWidget.setContentsMargins(QMargins)
- YCrossSection.setContextMenuPolicy()
QWidget.setContextMenuPolicy(Qt.ContextMenuPolicy)
- YCrossSection.setCursor()
QWidget.setCursor(QCursor)
- YCrossSection.setDisabled()
QWidget.setDisabled(bool)
- YCrossSection.setEnabled()
QWidget.setEnabled(bool)
- YCrossSection.setFixedHeight()
QWidget.setFixedHeight(int)
- YCrossSection.setFixedSize()
QWidget.setFixedSize(QSize) QWidget.setFixedSize(int, int)
- YCrossSection.setFixedWidth()
QWidget.setFixedWidth(int)
- YCrossSection.setFocus()
QWidget.setFocus() QWidget.setFocus(Qt.FocusReason)
- YCrossSection.setFocusPolicy()
QWidget.setFocusPolicy(Qt.FocusPolicy)
- YCrossSection.setFocusProxy()
QWidget.setFocusProxy(QWidget)
- YCrossSection.setFont()
QWidget.setFont(QFont)
- YCrossSection.setForegroundRole()
QWidget.setForegroundRole(QPalette.ColorRole)
- YCrossSection.setGeometry()
QWidget.setGeometry(QRect) QWidget.setGeometry(int, int, int, int)
- YCrossSection.setGraphicsEffect()
QWidget.setGraphicsEffect(QGraphicsEffect)
- YCrossSection.setHidden()
QWidget.setHidden(bool)
- YCrossSection.setInputContext()
QWidget.setInputContext(QInputContext)
- YCrossSection.setInputMethodHints()
QWidget.setInputMethodHints(Qt.InputMethodHints)
- YCrossSection.setLayout()
QWidget.setLayout(QLayout)
- YCrossSection.setLayoutDirection()
QWidget.setLayoutDirection(Qt.LayoutDirection)
- YCrossSection.setLocale()
QWidget.setLocale(QLocale)
- YCrossSection.setMask()
QWidget.setMask(QBitmap) QWidget.setMask(QRegion)
- YCrossSection.setMaximumHeight()
QWidget.setMaximumHeight(int)
- YCrossSection.setMaximumSize()
QWidget.setMaximumSize(int, int) QWidget.setMaximumSize(QSize)
- YCrossSection.setMaximumWidth()
QWidget.setMaximumWidth(int)
- YCrossSection.setMinimumHeight()
QWidget.setMinimumHeight(int)
- YCrossSection.setMinimumSize()
QWidget.setMinimumSize(int, int) QWidget.setMinimumSize(QSize)
- YCrossSection.setMinimumWidth()
QWidget.setMinimumWidth(int)
- YCrossSection.setMouseTracking()
QWidget.setMouseTracking(bool)
- YCrossSection.setObjectName()
QObject.setObjectName(QString)
- YCrossSection.setPalette()
QWidget.setPalette(QPalette)
- YCrossSection.setParent()
QWidget.setParent(QWidget) QWidget.setParent(QWidget, Qt.WindowFlags)
- YCrossSection.setProperty()
QObject.setProperty(str, QVariant) -> bool
- YCrossSection.setShortcutAutoRepeat()
QWidget.setShortcutAutoRepeat(int, bool enabled=True)
- YCrossSection.setShortcutEnabled()
QWidget.setShortcutEnabled(int, bool enabled=True)
- YCrossSection.setShown()
QWidget.setShown(bool)
- YCrossSection.setSizeIncrement()
QWidget.setSizeIncrement(int, int) QWidget.setSizeIncrement(QSize)
- YCrossSection.setSizePolicy()
QWidget.setSizePolicy(QSizePolicy) QWidget.setSizePolicy(QSizePolicy.Policy, QSizePolicy.Policy)
- YCrossSection.setStatusTip()
QWidget.setStatusTip(QString)
- YCrossSection.setStyle()
QWidget.setStyle(QStyle)
- YCrossSection.setStyleSheet()
QWidget.setStyleSheet(QString)
- YCrossSection.setTabOrder()
QWidget.setTabOrder(QWidget, QWidget)
- YCrossSection.setToolTip()
QWidget.setToolTip(QString)
- YCrossSection.setUpdatesEnabled()
QWidget.setUpdatesEnabled(bool)
- YCrossSection.setVisible()
QWidget.setVisible(bool)
- YCrossSection.setWhatsThis()
QWidget.setWhatsThis(QString)
- YCrossSection.setWindowFilePath()
QWidget.setWindowFilePath(QString)
- YCrossSection.setWindowFlags()
QWidget.setWindowFlags(Qt.WindowFlags)
- YCrossSection.setWindowIcon()
QWidget.setWindowIcon(QIcon)
- YCrossSection.setWindowIconText()
QWidget.setWindowIconText(QString)
- YCrossSection.setWindowModality()
QWidget.setWindowModality(Qt.WindowModality)
- YCrossSection.setWindowModified()
QWidget.setWindowModified(bool)
- YCrossSection.setWindowOpacity()
QWidget.setWindowOpacity(float)
- YCrossSection.setWindowRole()
QWidget.setWindowRole(QString)
- YCrossSection.setWindowState()
QWidget.setWindowState(Qt.WindowStates)
- YCrossSection.setWindowTitle()
QWidget.setWindowTitle(QString)
- YCrossSection.show()
QWidget.show()
- YCrossSection.showFullScreen()
QWidget.showFullScreen()
- YCrossSection.showMaximized()
QWidget.showMaximized()
- YCrossSection.showMinimized()
QWidget.showMinimized()
- YCrossSection.showNormal()
QWidget.showNormal()
- YCrossSection.signalsBlocked()
QObject.signalsBlocked() -> bool
- YCrossSection.size()
QWidget.size() -> QSize
- YCrossSection.sizeHint()
QWidget.sizeHint() -> QSize
- YCrossSection.sizeIncrement()
QWidget.sizeIncrement() -> QSize
- YCrossSection.sizePolicy()
QWidget.sizePolicy() -> QSizePolicy
- YCrossSection.stackUnder()
QWidget.stackUnder(QWidget)
- YCrossSection.startTimer()
QObject.startTimer(int) -> int
- YCrossSection.statusTip()
QWidget.statusTip() -> QString
- YCrossSection.style()
QWidget.style() -> QStyle
- YCrossSection.styleSheet()
QWidget.styleSheet() -> QString
- YCrossSection.tabletEvent()
QWidget.tabletEvent(QTabletEvent)
- YCrossSection.testAttribute()
QWidget.testAttribute(Qt.WidgetAttribute) -> bool
- YCrossSection.thread()
QObject.thread() -> QThread
- YCrossSection.toolTip()
QWidget.toolTip() -> QString
- YCrossSection.topLevelWidget()
QWidget.topLevelWidget() -> QWidget
- YCrossSection.tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- YCrossSection.trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- YCrossSection.underMouse()
QWidget.underMouse() -> bool
- YCrossSection.ungrabGesture()
QWidget.ungrabGesture(Qt.GestureType)
- YCrossSection.unsetCursor()
QWidget.unsetCursor()
- YCrossSection.unsetLayoutDirection()
QWidget.unsetLayoutDirection()
- YCrossSection.unsetLocale()
QWidget.unsetLocale()
- YCrossSection.update()
QWidget.update() QWidget.update(QRect) QWidget.update(QRegion) QWidget.update(int, int, int, int)
- YCrossSection.updateGeometry()
QWidget.updateGeometry()
- YCrossSection.updateMicroFocus()
QWidget.updateMicroFocus()
- YCrossSection.update_plot(obj=None)
Update cross section curve(s) associated to object obj
obj may be a marker or a rectangular shape (see guiqwt.tools.CrossSectionTool and guiqwt.tools.AverageCrossSectionTool)
If obj is None, update the cross sections of the last active object
- YCrossSection.updatesEnabled()
QWidget.updatesEnabled() -> bool
- YCrossSection.visibility_changed(enable)
DockWidget visibility has changed
- YCrossSection.visibleRegion()
QWidget.visibleRegion() -> QRegion
- YCrossSection.whatsThis()
QWidget.whatsThis() -> QString
- YCrossSection.wheelEvent()
QWidget.wheelEvent(QWheelEvent)
- YCrossSection.width()
QWidget.width() -> int
- YCrossSection.widthMM()
QPaintDevice.widthMM() -> int
- YCrossSection.winEvent()
QWidget.winEvent(MSG) -> (bool, int)
- YCrossSection.winId()
QWidget.winId() -> sip.voidptr
- YCrossSection.window()
QWidget.window() -> QWidget
- YCrossSection.windowActivationChange()
QWidget.windowActivationChange(bool)
- YCrossSection.windowFilePath()
QWidget.windowFilePath() -> QString
- YCrossSection.windowFlags()
QWidget.windowFlags() -> Qt.WindowFlags
- YCrossSection.windowIcon()
QWidget.windowIcon() -> QIcon
- YCrossSection.windowIconText()
QWidget.windowIconText() -> QString
- YCrossSection.windowModality()
QWidget.windowModality() -> Qt.WindowModality
- YCrossSection.windowOpacity()
QWidget.windowOpacity() -> float
- YCrossSection.windowRole()
QWidget.windowRole() -> QString
- YCrossSection.windowState()
QWidget.windowState() -> Qt.WindowStates
- YCrossSection.windowTitle()
QWidget.windowTitle() -> QString
- YCrossSection.windowType()
QWidget.windowType() -> Qt.WindowType
- YCrossSection.x()
QWidget.x() -> int
- YCrossSection.y()
QWidget.y() -> int
guiqwt.annotations
- The annotations module provides annotated shapes:
An annotated shape is a plot item (derived from QwtPlotItem) that may be displayed on a 2D plotting widget like guiqwt.curve.CurvePlot or guiqwt.image.ImagePlot.
See also
module guiqwt.shapes
Examples
- An annotated shape may be created:
- from the associated plot item class (e.g. AnnotatedCircle to create an annotated circle): the item properties are then assigned by creating the appropriate style parameters object (guiqwt.styles.AnnotationParam)
>>> from guiqwt.annotations import AnnotatedCircle
>>> from guiqwt.styles import AnnotationParam
>>> param = AnnotationParam()
>>> param.title = 'My circle'
>>> circle_item = AnnotatedCircle(0., 2., 4., 0., param)
- or using the plot item builder (see guiqwt.builder.make()):
>>> from guiqwt.builder import make
>>> circle_item = make.annotated_circle(0., 2., 4., 0., title='My circle')
Reference
- class guiqwt.annotations.AnnotatedPoint(x=0, y=0, annotationparam=None)[source]
Construct an annotated point at coordinates (x, y) with properties set with annotationparam (see guiqwt.styles.AnnotationParam)
- create_label()
Return the label object associated to this annotated shape object
- create_shape()[source]
Return the shape object associated to this annotated shape object
- deserialize(reader)
Deserialize object from HDF5 reader
- get_center()
Return shape center coordinates: (xc, yc)
- get_infos()[source]
Return formatted string with informations on current shape
- get_pos()[source]
Return the point coordinates
- get_text()
Return text associated to current shape (see guiqwt.label.ObjectInfo)
- get_tr_center()
Return shape center coordinates after applying transform matrix
- get_tr_center_str()
Return center coordinates as a string (with units)
- get_tr_size()
Return shape size after applying transform matrix
- get_tr_size_str()
Return size as a string (with units)
- is_label_visible()
Return True if associated label is visible
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- move_local_point_to(handle, pos, ctrl=None)
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- serialize(writer)
Serialize object to HDF5 writer
- set_label_position()[source]
Set label position, for instance based on shape position
- set_label_visible(state)
Set the annotated shape’s label visibility
- set_movable(state)
Set item movable state
- set_pos(x, y)[source]
Set the point coordinates to (x, y)
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- unselect()
Unselect item
- update_label()
Update the annotated shape’s label contents
- x_to_str(x)
Convert x (float) to a string (with associated unit and uncertainty)
- y_to_str(y)
Convert y (float) to a string (with associated unit and uncertainty)
- class guiqwt.annotations.AnnotatedSegment(x1=0, y1=0, x2=0, y2=0, annotationparam=None)[source]
Construct an annotated segment between coordinates (x1, y1) and (x2, y2) with properties set with annotationparam (see guiqwt.styles.AnnotationParam)
- create_label()
Return the label object associated to this annotated shape object
- create_shape()
Return the shape object associated to this annotated shape object
- deserialize(reader)
Deserialize object from HDF5 reader
- get_center()
Return shape center coordinates: (xc, yc)
- get_infos()[source]
Return formatted string with informations on current shape
- get_rect()[source]
Return the coordinates of the shape’s top-left and bottom-right corners
- get_text()
Return text associated to current shape (see guiqwt.label.ObjectInfo)
- get_tr_center()
Return shape center coordinates after applying transform matrix
- get_tr_center_str()
Return center coordinates as a string (with units)
- get_tr_length()[source]
Return segment length after applying transform matrix
- get_tr_size()
Return shape size after applying transform matrix
- get_tr_size_str()
Return size as a string (with units)
- is_label_visible()
Return True if associated label is visible
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- move_local_point_to(handle, pos, ctrl=None)
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- serialize(writer)
Serialize object to HDF5 writer
- set_label_position()[source]
Set label position, for instance based on shape position
- set_label_visible(state)
Set the annotated shape’s label visibility
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_rect(x1, y1, x2, y2)[source]
Set the coordinates of the shape’s top-left corner to (x1, y1), and of its bottom-right corner to (x2, y2).
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- unselect()
Unselect item
- update_label()
Update the annotated shape’s label contents
- x_to_str(x)
Convert x (float) to a string (with associated unit and uncertainty)
- y_to_str(y)
Convert y (float) to a string (with associated unit and uncertainty)
- class guiqwt.annotations.AnnotatedRectangle(x1=0, y1=0, x2=0, y2=0, annotationparam=None)[source]
Construct an annotated rectangle between coordinates (x1, y1) and (x2, y2) with properties set with annotationparam (see guiqwt.styles.AnnotationParam)
- create_label()
Return the label object associated to this annotated shape object
- create_shape()
Return the shape object associated to this annotated shape object
- deserialize(reader)
Deserialize object from HDF5 reader
- get_center()
Return shape center coordinates: (xc, yc)
- get_computations_text()[source]
Return formatted string with informations on current shape
- get_infos()[source]
Return formatted string with informations on current shape
- get_rect()[source]
Return the coordinates of the shape’s top-left and bottom-right corners
- get_text()
Return text associated to current shape (see guiqwt.label.ObjectInfo)
- get_tr_center()[source]
Return shape center coordinates after applying transform matrix
- get_tr_center_str()
Return center coordinates as a string (with units)
- get_tr_size()[source]
Return shape size after applying transform matrix
- get_tr_size_str()
Return size as a string (with units)
- is_label_visible()
Return True if associated label is visible
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- move_local_point_to(handle, pos, ctrl=None)
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- serialize(writer)
Serialize object to HDF5 writer
- set_label_position()[source]
Set label position, for instance based on shape position
- set_label_visible(state)
Set the annotated shape’s label visibility
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_rect(x1, y1, x2, y2)[source]
Set the coordinates of the shape’s top-left corner to (x1, y1), and of its bottom-right corner to (x2, y2).
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- unselect()
Unselect item
- update_label()
Update the annotated shape’s label contents
- x_to_str(x)
Convert x (float) to a string (with associated unit and uncertainty)
- y_to_str(y)
Convert y (float) to a string (with associated unit and uncertainty)
- class guiqwt.annotations.AnnotatedObliqueRectangle(x0=0, y0=0, x1=0, y1=0, x2=0, y2=0, x3=0, y3=0, annotationparam=None)[source]
Construct an annotated oblique rectangle between coordinates (x0, y0), (x1, y1), (x2, y2) and (x3, y3) with properties set with annotationparam (see guiqwt.styles.AnnotationParam)
- create_label()
Return the label object associated to this annotated shape object
- create_shape()[source]
Return the shape object associated to this annotated shape object
- deserialize(reader)
Deserialize object from HDF5 reader
- get_bounding_rect_coords()[source]
Return bounding rectangle coordinates (in plot coordinates)
- get_center()
Return shape center coordinates: (xc, yc)
- get_computations_text()
Return formatted string with informations on current shape
- get_infos()[source]
Return formatted string with informations on current shape
- get_rect()
Return the coordinates of the shape’s top-left and bottom-right corners
- get_text()
Return text associated to current shape (see guiqwt.label.ObjectInfo)
- get_tr_angle()[source]
Return X-diameter angle with horizontal direction, after applying transform matrix
- get_tr_center()
Return shape center coordinates after applying transform matrix
- get_tr_center_str()
Return center coordinates as a string (with units)
- get_tr_size()[source]
Return shape size after applying transform matrix
- get_tr_size_str()
Return size as a string (with units)
- is_label_visible()
Return True if associated label is visible
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- move_local_point_to(handle, pos, ctrl=None)
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- serialize(writer)
Serialize object to HDF5 writer
- set_label_position()[source]
Set label position, for instance based on shape position
- set_label_visible(state)
Set the annotated shape’s label visibility
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_rect(x0, y0, x1, y1, x2, y2, x3, y3)[source]
- Set the rectangle corners coordinates:
(x0, y0): top-left corner (x1, y1): top-right corner (x2, y2): bottom-right corner (x3, y3): bottom-left corner
x: additionnal points
- (x0, y0)——>(x1, y1)
- ↑ | | | x x | | | ↓
(x3, y3)<——(x2, y2)
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- unselect()
Unselect item
- update_label()
Update the annotated shape’s label contents
- x_to_str(x)
Convert x (float) to a string (with associated unit and uncertainty)
- y_to_str(y)
Convert y (float) to a string (with associated unit and uncertainty)
- class guiqwt.annotations.AnnotatedEllipse(x1=0, y1=0, x2=0, y2=0, annotationparam=None)[source]
Construct an annotated ellipse with X-axis diameter between coordinates (x1, y1) and (x2, y2) and properties set with annotationparam (see guiqwt.styles.AnnotationParam)
- create_label()
Return the label object associated to this annotated shape object
- create_shape()
Return the shape object associated to this annotated shape object
- deserialize(reader)
Deserialize object from HDF5 reader
- get_center()
Return shape center coordinates: (xc, yc)
- get_infos()[source]
Return formatted string with informations on current shape
- get_text()
Return text associated to current shape (see guiqwt.label.ObjectInfo)
- get_tr_angle()[source]
Return X-diameter angle with horizontal direction, after applying transform matrix
- get_tr_center()[source]
Return center coordinates: (xc, yc)
- get_tr_center_str()
Return center coordinates as a string (with units)
- get_tr_size()[source]
Return shape size after applying transform matrix
- get_tr_size_str()
Return size as a string (with units)
- get_xdiameter()[source]
Return the coordinates of the ellipse’s X-axis diameter Warning: transform matrix is not applied here
- get_ydiameter()[source]
Return the coordinates of the ellipse’s Y-axis diameter Warning: transform matrix is not applied here
- is_label_visible()
Return True if associated label is visible
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- move_local_point_to(handle, pos, ctrl=None)
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- serialize(writer)
Serialize object to HDF5 writer
- set_label_position()[source]
Set label position, for instance based on shape position
- set_label_visible(state)
Set the annotated shape’s label visibility
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- set_xdiameter(x0, y0, x1, y1)[source]
Set the coordinates of the ellipse’s X-axis diameter Warning: transform matrix is not applied here
- set_ydiameter(x2, y2, x3, y3)[source]
Set the coordinates of the ellipse’s Y-axis diameter Warning: transform matrix is not applied here
- unselect()
Unselect item
- update_label()
Update the annotated shape’s label contents
- x_to_str(x)
Convert x (float) to a string (with associated unit and uncertainty)
- y_to_str(y)
Convert y (float) to a string (with associated unit and uncertainty)
- class guiqwt.annotations.AnnotatedCircle(x1=0, y1=0, x2=0, y2=0, annotationparam=None)[source]
Construct an annotated circle with diameter between coordinates (x1, y1) and (x2, y2) and properties set with annotationparam (see guiqwt.styles.AnnotationParam)
- create_label()
Return the label object associated to this annotated shape object
- create_shape()
Return the shape object associated to this annotated shape object
- deserialize(reader)
Deserialize object from HDF5 reader
- get_center()
Return shape center coordinates: (xc, yc)
- get_infos()[source]
Return formatted string with informations on current shape
- get_text()
Return text associated to current shape (see guiqwt.label.ObjectInfo)
- get_tr_angle()
Return X-diameter angle with horizontal direction, after applying transform matrix
- get_tr_center()
Return center coordinates: (xc, yc)
- get_tr_center_str()
Return center coordinates as a string (with units)
- get_tr_diameter()[source]
Return circle diameter after applying transform matrix
- get_tr_size()
Return shape size after applying transform matrix
- get_tr_size_str()
Return size as a string (with units)
- get_xdiameter()
Return the coordinates of the ellipse’s X-axis diameter Warning: transform matrix is not applied here
- get_ydiameter()
Return the coordinates of the ellipse’s Y-axis diameter Warning: transform matrix is not applied here
- is_label_visible()
Return True if associated label is visible
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- move_local_point_to(handle, pos, ctrl=None)
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- serialize(writer)
Serialize object to HDF5 writer
- set_label_position()
Set label position, for instance based on shape position
- set_label_visible(state)
Set the annotated shape’s label visibility
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- set_xdiameter(x0, y0, x1, y1)
Set the coordinates of the ellipse’s X-axis diameter Warning: transform matrix is not applied here
- set_ydiameter(x2, y2, x3, y3)
Set the coordinates of the ellipse’s Y-axis diameter Warning: transform matrix is not applied here
- unselect()
Unselect item
- update_label()
Update the annotated shape’s label contents
- x_to_str(x)
Convert x (float) to a string (with associated unit and uncertainty)
- y_to_str(y)
Convert y (float) to a string (with associated unit and uncertainty)
guiqwt.shapes
- The shapes module provides geometrical shapes:
A shape is a plot item (derived from QwtPlotItem) that may be displayed on a 2D plotting widget like guiqwt.curve.CurvePlot or guiqwt.image.ImagePlot.
See also
module guiqwt.annotations
Examples
- A shape may be created:
- from the associated plot item class (e.g. RectangleShape to create a rectangle): the item properties are then assigned by creating the appropriate style parameters object (guiqwt.styles.ShapeParam)
>>> from guiqwt.shapes import RectangleShape
>>> from guiqwt.styles import ShapeParam
>>> param = ShapeParam()
>>> param.title = 'My rectangle'
>>> rect_item = RectangleShape(0., 2., 4., 0., param)
- or using the plot item builder (see guiqwt.builder.make()):
>>> from guiqwt.builder import make
>>> rect_item = make.rectangle(0., 2., 4., 0., title='My rectangle')
Reference
- class guiqwt.shapes.PolygonShape(points=None, closed=None, shapeparam=None)[source]
- deserialize(reader)[source]
Deserialize object from HDF5 reader
- get_bounding_rect_coords()[source]
Return bounding rectangle coordinates (in plot coordinates)
- get_points()[source]
Return polygon points
- hit_test(pos)[source]
return (dist, handle, inside)
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- move_local_point_to(handle, pos, ctrl=None)
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_local_shape(old_pos, new_pos)
Translate the shape such that old_pos becomes new_pos in canvas coordinates
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- serialize(writer)[source]
Serialize object to HDF5 writer
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- unselect()
Unselect item
- class guiqwt.shapes.RectangleShape(x1=0, y1=0, x2=0, y2=0, shapeparam=None)[source]
- deserialize(reader)
Deserialize object from HDF5 reader
- get_bounding_rect_coords()
Return bounding rectangle coordinates (in plot coordinates)
- get_center()[source]
Return center coordinates: (xc, yc)
- get_points()
Return polygon points
- hit_test(pos)
return (dist, handle, inside)
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- move_local_point_to(handle, pos, ctrl=None)
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_local_shape(old_pos, new_pos)
Translate the shape such that old_pos becomes new_pos in canvas coordinates
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- serialize(writer)
Serialize object to HDF5 writer
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_rect(x1, y1, x2, y2)[source]
Set the coordinates of the rectangle’s top-left corner to (x1, y1), and of its bottom-right corner to (x2, y2).
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- unselect()
Unselect item
- class guiqwt.shapes.ObliqueRectangleShape(x0=0, y0=0, x1=0, y1=0, x2=0, y2=0, x3=0, y3=0, shapeparam=None)[source]
- deserialize(reader)
Deserialize object from HDF5 reader
- get_bounding_rect_coords()
Return bounding rectangle coordinates (in plot coordinates)
- get_center()[source]
Return center coordinates: (xc, yc)
- get_points()
Return polygon points
- hit_test(pos)
return (dist, handle, inside)
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- move_local_point_to(handle, pos, ctrl=None)
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_local_shape(old_pos, new_pos)
Translate the shape such that old_pos becomes new_pos in canvas coordinates
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- serialize(writer)
Serialize object to HDF5 writer
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_rect(x0, y0, x1, y1, x2, y2, x3, y3)[source]
- Set the rectangle corners coordinates:
(x0, y0): top-left corner (x1, y1): top-right corner (x2, y2): bottom-right corner (x3, y3): bottom-left corner
x: additionnal points (handles used for rotation – other handles being used for rectangle resizing)
- (x0, y0)——>(x1, y1)
- ↑ | | | x x | | | ↓
(x3, y3)<——(x2, y2)
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- unselect()
Unselect item
- class guiqwt.shapes.PointShape(x=0, y=0, shapeparam=None)[source]
- deserialize(reader)
Deserialize object from HDF5 reader
- get_bounding_rect_coords()
Return bounding rectangle coordinates (in plot coordinates)
- get_points()
Return polygon points
- get_pos()[source]
Return the point coordinates
- hit_test(pos)
return (dist, handle, inside)
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- move_local_point_to(handle, pos, ctrl=None)
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_local_shape(old_pos, new_pos)
Translate the shape such that old_pos becomes new_pos in canvas coordinates
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- serialize(writer)
Serialize object to HDF5 writer
- set_movable(state)
Set item movable state
- set_pos(x, y)[source]
Set the point coordinates to (x, y)
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- unselect()
Unselect item
- class guiqwt.shapes.SegmentShape(x1=0, y1=0, x2=0, y2=0, shapeparam=None)[source]
- deserialize(reader)
Deserialize object from HDF5 reader
- get_bounding_rect_coords()
Return bounding rectangle coordinates (in plot coordinates)
- get_points()
Return polygon points
- hit_test(pos)
return (dist, handle, inside)
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- move_local_point_to(handle, pos, ctrl=None)
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_local_shape(old_pos, new_pos)
Translate the shape such that old_pos becomes new_pos in canvas coordinates
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- serialize(writer)
Serialize object to HDF5 writer
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_rect(x1, y1, x2, y2)[source]
Set the start point of this segment to (x1, y1) and the end point of this line to (x2, y2)
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- unselect()
Unselect item
- class guiqwt.shapes.EllipseShape(x1=0, y1=0, x2=0, y2=0, shapeparam=None)[source]
- compute_elements(xMap, yMap)[source]
Return points, lines and ellipse rect
- deserialize(reader)
Deserialize object from HDF5 reader
- get_bounding_rect_coords()
Return bounding rectangle coordinates (in plot coordinates)
- get_center()[source]
Return center coordinates: (xc, yc)
- get_points()
Return polygon points
- get_rect()[source]
Circle only!
- get_xdiameter()[source]
Return the coordinates of the ellipse’s X-axis diameter
- get_ydiameter()[source]
Return the coordinates of the ellipse’s Y-axis diameter
- hit_test(pos)[source]
return (dist, handle, inside)
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- move_local_point_to(handle, pos, ctrl=None)
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_local_shape(old_pos, new_pos)
Translate the shape such that old_pos becomes new_pos in canvas coordinates
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- serialize(writer)
Serialize object to HDF5 writer
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_rect(x0, y0, x1, y1)[source]
Circle only!
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- set_xdiameter(x0, y0, x1, y1)[source]
Set the coordinates of the ellipse’s X-axis diameter
- set_ydiameter(x2, y2, x3, y3)[source]
Set the coordinates of the ellipse’s Y-axis diameter
- unselect()
Unselect item
- class guiqwt.shapes.Axes(p0=(0, 0), p1=(0, 0), p2=(0, 0), axesparam=None, shapeparam=None)[source]
Axes( (0,1), (1,1), (0,0) )
- deserialize(reader)[source]
Deserialize object from HDF5 reader
- get_bounding_rect_coords()
Return bounding rectangle coordinates (in plot coordinates)
- get_points()
Return polygon points
- hit_test(pos)
return (dist, handle, inside)
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- move_local_point_to(handle, pos, ctrl=None)
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_local_shape(old_pos, new_pos)
Translate the shape such that old_pos becomes new_pos in canvas coordinates
- move_shape(old_pos, new_pos)[source]
Overriden to emit the axes_changed signal
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- serialize(writer)[source]
Serialize object to HDF5 writer
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- unselect()
Unselect item
- class guiqwt.shapes.XRangeSelection(_min, _max, shapeparam=None)[source]
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- move_local_point_to(handle, pos, ctrl=None)[source]
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_local_shape(old_pos, new_pos)
Translate the shape such that old_pos becomes new_pos in canvas coordinates
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- types()
Returns a group or category for this item this should be a class object inheriting from IItemType
- unselect()
Unselect item
guiqwt.label
- The labels module provides plot items related to labels and legends:
- guiqwt.shapes.LabelItem
- guiqwt.shapes.LegendBoxItem
- guiqwt.shapes.SelectedLegendBoxItem
- guiqwt.shapes.RangeComputation
- guiqwt.shapes.RangeComputation2d
- guiqwt.shapes.DataInfoLabel
A label or a legend is a plot item (derived from QwtPlotItem) that may be displayed on a 2D plotting widget like guiqwt.curve.CurvePlot or guiqwt.image.ImagePlot.
Reference
- class guiqwt.label.LabelItem(text=None, labelparam=None)[source]
- deserialize(reader)[source]
Deserialize object from HDF5 reader
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- move_local_point_to(handle, pos, ctrl=None)
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_local_shape(old_pos, new_pos)
Translate the shape such that old_pos becomes new_pos in canvas coordinates
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- serialize(writer)[source]
Serialize object to HDF5 writer
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- unselect()
Unselect item
- class guiqwt.label.LegendBoxItem(labelparam=None)[source]
- deserialize(reader)
Deserialize object from HDF5 reader
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- move_local_point_to(handle, pos, ctrl=None)
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_local_shape(old_pos, new_pos)
Translate the shape such that old_pos becomes new_pos in canvas coordinates
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- serialize(writer)
Serialize object to HDF5 writer
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- unselect()
Unselect item
- class guiqwt.label.SelectedLegendBoxItem(dataset=None, itemlist=None)[source]
- deserialize(reader)
Deserialize object from HDF5 reader
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- move_local_point_to(handle, pos, ctrl=None)
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_local_shape(old_pos, new_pos)
Translate the shape such that old_pos becomes new_pos in canvas coordinates
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- serialize(writer)
Serialize object to HDF5 writer
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- unselect()
Unselect item
- class guiqwt.label.RangeComputation(label, curve, xrangeselection, function=None)[source]
ObjectInfo showing curve computations relative to a XRangeSelection shape.
label: formatted string curve: CurveItem object xrangeselection: XRangeSelection object function: input arguments are x, y arrays (extraction of arrays corresponding to the xrangeselection X-axis range)
- class guiqwt.label.RangeComputation2d(label, image, rect, function)[source]
- class guiqwt.label.DataInfoLabel(labelparam=None, infos=None)[source]
- deserialize(reader)
Deserialize object from HDF5 reader
- is_private()
Return True if object is private
- is_readonly()
Return object readonly state
- move_local_point_to(handle, pos, ctrl=None)
Move a handle as returned by hit_test to the new position pos ctrl: True if <Ctrl> button is being pressed, False otherwise
- move_local_shape(old_pos, new_pos)
Translate the shape such that old_pos becomes new_pos in canvas coordinates
- move_with_selection(delta_x, delta_y)
Translate the shape together with other selected items delta_x, delta_y: translation in plot coordinates
- select()
Select item
- serialize(writer)
Serialize object to HDF5 writer
- set_movable(state)
Set item movable state
- set_private(state)
Set object as private
- set_readonly(state)
Set object readonly state
- set_resizable(state)
Set item resizable state (or any action triggered when moving an handle, e.g. rotation)
- set_rotatable(state)
Set item rotatable state
- set_selectable(state)
Set item selectable state
- unselect()
Unselect item
guiqwt.tools
- The tools module provides a collection of plot tools :
- guiqwt.tools.RectZoomTool
- guiqwt.tools.SelectTool
- guiqwt.tools.SelectPointTool
- guiqwt.tools.MultiLineTool
- guiqwt.tools.FreeFormTool
- guiqwt.tools.LabelTool
- guiqwt.tools.RectangleTool
- guiqwt.tools.PointTool
- guiqwt.tools.SegmentTool
- guiqwt.tools.CircleTool
- guiqwt.tools.EllipseTool
- guiqwt.tools.PlaceAxesTool
- guiqwt.tools.AnnotatedRectangleTool
- guiqwt.tools.AnnotatedCircleTool
- guiqwt.tools.AnnotatedEllipseTool
- guiqwt.tools.AnnotatedPointTool
- guiqwt.tools.AnnotatedSegmentTool
- guiqwt.tools.HRangeTool
- guiqwt.tools.DummySeparatorTool
- guiqwt.tools.AntiAliasingTool
- guiqwt.tools.DisplayCoordsTool
- guiqwt.tools.ReverseYAxisTool
- guiqwt.tools.AspectRatioTool
- guiqwt.tools.PanelTool
- guiqwt.tools.ItemListPanelTool
- guiqwt.tools.ContrastPanelTool
- guiqwt.tools.ColormapTool
- guiqwt.tools.XCSPanelTool
- guiqwt.tools.YCSPanelTool
- guiqwt.tools.CrossSectionTool
- guiqwt.tools.AverageCrossSectionTool
- guiqwt.tools.SaveAsTool
- guiqwt.tools.CopyToClipboardTool
- guiqwt.tools.OpenFileTool
- guiqwt.tools.OpenImageTool
- guiqwt.tools.SnapshotTool
- guiqwt.tools.PrintTool
- guiqwt.tools.SaveItemsTool
- guiqwt.tools.LoadItemsTool
- guiqwt.tools.AxisScaleTool
- guiqwt.tools.HelpTool
- guiqwt.tools.ExportItemDataTool
- guiqwt.tools.EditItemDataTool
- guiqwt.tools.ItemCenterTool
- guiqwt.tools.DeleteItemTool
A plot tool is an object providing various features to a plotting widget (guiqwt.curve.CurvePlot or guiqwt.image.ImagePlot): buttons, menus, selection tools, image I/O tools, etc. To make it work, a tool has to be registered to the plotting widget’s manager, i.e. an instance of the guiqwt.plot.PlotManager class (see the guiqwt.plot module for more details on the procedure).
The CurvePlot and ImagePlot widgets do not provide any PlotManager: the manager has to be created separately. On the contrary, the ready-to-use widgets guiqwt.plot.CurveWidget and guiqwt.plot.ImageWidget are higher-level plotting widgets with integrated manager, tools and panels.
See also
- Module guiqwt.plot
- Module providing ready-to-use curve and image plotting widgets and dialog boxes
- Module guiqwt.curve
- Module providing curve-related plot items and plotting widgets
- Module guiqwt.image
- Module providing image-related plot items and plotting widgets
Example
The following example add all the existing tools to an ImageWidget object for testing purpose:
import os.path as osp
from guiqwt.plot import ImageDialog
from guiqwt.tools import (RectangleTool, EllipseTool, HRangeTool, PlaceAxesTool,
MultiLineTool, FreeFormTool, SegmentTool, CircleTool,
AnnotatedRectangleTool, AnnotatedEllipseTool,
AnnotatedSegmentTool, AnnotatedCircleTool, LabelTool,
AnnotatedPointTool,
VCursorTool, HCursorTool, XCursorTool,
ObliqueRectangleTool, AnnotatedObliqueRectangleTool)
from guiqwt.builder import make
def create_window():
win = ImageDialog(edit=False, toolbar=True,
wintitle="All image and plot tools test")
for toolklass in (LabelTool, HRangeTool,
VCursorTool, HCursorTool, XCursorTool,
SegmentTool, RectangleTool, ObliqueRectangleTool,
CircleTool, EllipseTool,
MultiLineTool, FreeFormTool, PlaceAxesTool,
AnnotatedRectangleTool, AnnotatedObliqueRectangleTool,
AnnotatedCircleTool, AnnotatedEllipseTool,
AnnotatedSegmentTool, AnnotatedPointTool):
win.add_tool(toolklass)
return win
def test():
"""Test"""
# -- Create QApplication
import guidata
_app = guidata.qapplication()
# --
filename = osp.join(osp.dirname(__file__), "brain.png")
win = create_window()
image = make.image(filename=filename, colormap="bone")
plot = win.get_plot()
plot.add_item(image)
win.exec_()
if __name__ == "__main__":
test()
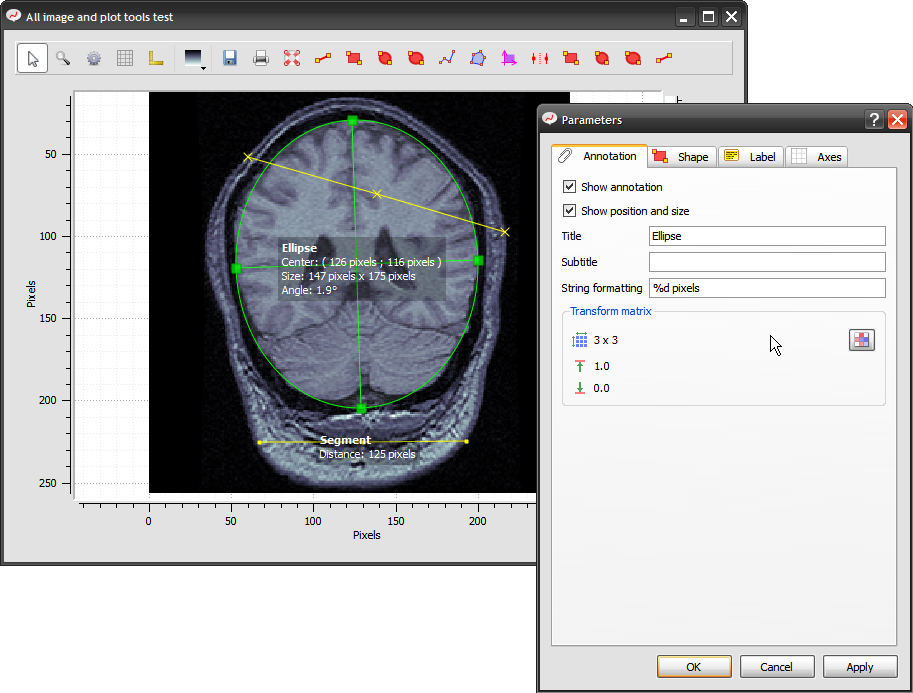
Reference
- class guiqwt.tools.RectZoomTool(manager, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]
- activate()
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- cursor()
Return tool mouse cursor
- customEvent()
QObject.customEvent(QEvent)
- deactivate()
Deactivate tool
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.SelectTool(manager, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]
Graphical Object Selection Tool
- activate()
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- cursor()
Return tool mouse cursor
- customEvent()
QObject.customEvent(QEvent)
- deactivate()
Deactivate tool
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.SelectPointTool(manager, mode='reuse', on_active_item=False, title=None, icon=None, tip=None, end_callback=None, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>, marker_style=None, switch_to_default_tool=None)[source]
- activate()
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- cursor()
Return tool mouse cursor
- customEvent()
QObject.customEvent(QEvent)
- deactivate()
Deactivate tool
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.MultiLineTool(manager, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]
- activate()
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- cursor()
Return tool mouse cursor
- customEvent()
QObject.customEvent(QEvent)
- deactivate()
Deactivate tool
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- mouse_press(filter, event)[source]
We create a new shape if it’s the first point otherwise we add a new point
- mouse_release(filter, event)[source]
Releasing the mouse button validate the last point position
- move(filter, event)[source]
moving while holding the button down lets the user position the last created point
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.FreeFormTool(manager, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]
- activate()
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- cancel_point(filter, event)[source]
Reimplement base class method
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- cursor()
Return tool mouse cursor
- customEvent()
QObject.customEvent(QEvent)
- deactivate()
Deactivate tool
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- mouse_press(filter, event)[source]
Reimplement base class method
- mouse_release(filter, event)
Releasing the mouse button validate the last point position
- move(filter, event)
moving while holding the button down lets the user position the last created point
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.LabelTool(manager, handle_label_cb=None, label_style=None, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]
- activate()
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- cursor()
Return tool mouse cursor
- customEvent()
QObject.customEvent(QEvent)
- deactivate()
Deactivate tool
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.RectangleTool(manager, setup_shape_cb=None, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]
- activate()
Activate tool
- add_shape_to_plot(plot, p0, p1)
Method called when shape’s rectangular area has just been drawn on screen. Adding the final shape to plot and returning it.
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- cursor()
Return tool mouse cursor
- customEvent()
QObject.customEvent(QEvent)
- deactivate()
Deactivate tool
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- get_shape()
Reimplemented RectangularActionTool method
- handle_final_shape(shape)
To be reimplemented
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
- setup_shape(shape)
To be reimplemented
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.PointTool(manager, setup_shape_cb=None, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]
- activate()
Activate tool
- add_shape_to_plot(plot, p0, p1)
Method called when shape’s rectangular area has just been drawn on screen. Adding the final shape to plot and returning it.
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- cursor()
Return tool mouse cursor
- customEvent()
QObject.customEvent(QEvent)
- deactivate()
Deactivate tool
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- get_shape()
Reimplemented RectangularActionTool method
- handle_final_shape(shape)
To be reimplemented
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
- setup_shape(shape)
To be reimplemented
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.SegmentTool(manager, setup_shape_cb=None, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]
- activate()
Activate tool
- add_shape_to_plot(plot, p0, p1)
Method called when shape’s rectangular area has just been drawn on screen. Adding the final shape to plot and returning it.
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- cursor()
Return tool mouse cursor
- customEvent()
QObject.customEvent(QEvent)
- deactivate()
Deactivate tool
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- get_shape()
Reimplemented RectangularActionTool method
- handle_final_shape(shape)
To be reimplemented
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
- setup_shape(shape)
To be reimplemented
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.CircleTool(manager, setup_shape_cb=None, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]
- activate()
Activate tool
- add_shape_to_plot(plot, p0, p1)
Method called when shape’s rectangular area has just been drawn on screen. Adding the final shape to plot and returning it.
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- cursor()
Return tool mouse cursor
- customEvent()
QObject.customEvent(QEvent)
- deactivate()
Deactivate tool
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- get_shape()
Reimplemented RectangularActionTool method
- handle_final_shape(shape)
To be reimplemented
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
- setup_shape(shape)
To be reimplemented
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.EllipseTool(manager, setup_shape_cb=None, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]
- activate()
Activate tool
- add_shape_to_plot(plot, p0, p1)
Method called when shape’s rectangular area has just been drawn on screen. Adding the final shape to plot and returning it.
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- cursor()
Return tool mouse cursor
- customEvent()
QObject.customEvent(QEvent)
- deactivate()
Deactivate tool
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- get_shape()
Reimplemented RectangularActionTool method
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
- setup_shape(shape)
To be reimplemented
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.PlaceAxesTool(manager, setup_shape_cb=None, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]
- activate()
Activate tool
- add_shape_to_plot(plot, p0, p1)
Method called when shape’s rectangular area has just been drawn on screen. Adding the final shape to plot and returning it.
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- cursor()
Return tool mouse cursor
- customEvent()
QObject.customEvent(QEvent)
- deactivate()
Deactivate tool
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- get_shape()
Reimplemented RectangularActionTool method
- handle_final_shape(shape)
To be reimplemented
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
- setup_shape(shape)
To be reimplemented
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.AnnotatedRectangleTool(manager, setup_shape_cb=None, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]
- activate()
Activate tool
- add_shape_to_plot(plot, p0, p1)
Method called when shape’s rectangular area has just been drawn on screen. Adding the final shape to plot and returning it.
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- cursor()
Return tool mouse cursor
- customEvent()
QObject.customEvent(QEvent)
- deactivate()
Deactivate tool
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- get_shape()
Reimplemented RectangularActionTool method
- handle_final_shape(shape)
To be reimplemented
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
- setup_shape(shape)
To be reimplemented
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.AnnotatedCircleTool(manager, setup_shape_cb=None, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]
- activate()
Activate tool
- add_shape_to_plot(plot, p0, p1)
Method called when shape’s rectangular area has just been drawn on screen. Adding the final shape to plot and returning it.
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- cursor()
Return tool mouse cursor
- customEvent()
QObject.customEvent(QEvent)
- deactivate()
Deactivate tool
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- get_shape()
Reimplemented RectangularActionTool method
- handle_final_shape(shape)
To be reimplemented
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
- setup_shape(shape)
To be reimplemented
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.AnnotatedEllipseTool(manager, setup_shape_cb=None, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]
- activate()
Activate tool
- add_shape_to_plot(plot, p0, p1)
Method called when shape’s rectangular area has just been drawn on screen. Adding the final shape to plot and returning it.
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- cursor()
Return tool mouse cursor
- customEvent()
QObject.customEvent(QEvent)
- deactivate()
Deactivate tool
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- get_shape()
Reimplemented RectangularActionTool method
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
- setup_shape(shape)
To be reimplemented
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.AnnotatedPointTool(manager, setup_shape_cb=None, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]
- activate()
Activate tool
- add_shape_to_plot(plot, p0, p1)
Method called when shape’s rectangular area has just been drawn on screen. Adding the final shape to plot and returning it.
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- cursor()
Return tool mouse cursor
- customEvent()
QObject.customEvent(QEvent)
- deactivate()
Deactivate tool
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- get_shape()
Reimplemented RectangularActionTool method
- handle_final_shape(shape)
To be reimplemented
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
- setup_shape(shape)
To be reimplemented
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.AnnotatedSegmentTool(manager, setup_shape_cb=None, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]
- activate()
Activate tool
- add_shape_to_plot(plot, p0, p1)
Method called when shape’s rectangular area has just been drawn on screen. Adding the final shape to plot and returning it.
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- cursor()
Return tool mouse cursor
- customEvent()
QObject.customEvent(QEvent)
- deactivate()
Deactivate tool
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- get_shape()
Reimplemented RectangularActionTool method
- handle_final_shape(shape)
To be reimplemented
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
- setup_shape(shape)
To be reimplemented
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.HRangeTool(manager, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]
- activate()
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- cursor()
Return tool mouse cursor
- customEvent()
QObject.customEvent(QEvent)
- deactivate()
Deactivate tool
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.DummySeparatorTool(manager, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>)[source]
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- customEvent()
QObject.customEvent(QEvent)
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- register_plot(baseplot)
Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
- setup_toolbar(toolbar)[source]
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.AntiAliasingTool(manager)[source]
- activate_command(plot, checked)[source]
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- customEvent()
QObject.customEvent(QEvent)
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- register_plot(baseplot)
Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- class guiqwt.tools.DisplayCoordsTool(manager)[source]
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- customEvent()
QObject.customEvent(QEvent)
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- register_plot(baseplot)
Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- class guiqwt.tools.ReverseYAxisTool(manager)[source]
- activate_command(plot, checked)[source]
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- customEvent()
QObject.customEvent(QEvent)
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- register_plot(baseplot)
Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- class guiqwt.tools.AspectRatioTool(manager)[source]
- activate_command(plot, checked)[source]
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- customEvent()
QObject.customEvent(QEvent)
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- lock_aspect_ratio(checked)[source]
Lock aspect ratio
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- register_plot(baseplot)
Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- class guiqwt.tools.PanelTool(manager)[source]
- activate_command(plot, checked)[source]
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- customEvent()
QObject.customEvent(QEvent)
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- register_plot(baseplot)
Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- class guiqwt.tools.ItemListPanelTool(manager)[source]
- activate_command(plot, checked)
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- customEvent()
QObject.customEvent(QEvent)
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- register_plot(baseplot)
Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- class guiqwt.tools.ContrastPanelTool(manager)[source]
- activate_command(plot, checked)
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- customEvent()
QObject.customEvent(QEvent)
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- register_plot(baseplot)
Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- class guiqwt.tools.ColormapTool(manager, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>)[source]
- activate_command(plot, checked)[source]
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- customEvent()
QObject.customEvent(QEvent)
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- register_plot(baseplot)
Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- class guiqwt.tools.XCSPanelTool(manager)[source]
- activate_command(plot, checked)
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- customEvent()
QObject.customEvent(QEvent)
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- register_plot(baseplot)
Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- class guiqwt.tools.YCSPanelTool(manager)[source]
- activate_command(plot, checked)
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- customEvent()
QObject.customEvent(QEvent)
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- register_plot(baseplot)
Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- class guiqwt.tools.CrossSectionTool(manager, setup_shape_cb=None, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]
- activate()[source]
Activate tool
- add_shape_to_plot(plot, p0, p1)
Method called when shape’s rectangular area has just been drawn on screen. Adding the final shape to plot and returning it.
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- cursor()
Return tool mouse cursor
- customEvent()
QObject.customEvent(QEvent)
- deactivate()
Deactivate tool
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- get_shape()
Reimplemented RectangularActionTool method
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.AverageCrossSectionTool(manager, setup_shape_cb=None, handle_final_shape_cb=None, shape_style=None, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>, title=None, icon=None, tip=None, switch_to_default_tool=None)[source]
- activate()
Activate tool
- add_shape_to_plot(plot, p0, p1)
Method called when shape’s rectangular area has just been drawn on screen. Adding the final shape to plot and returning it.
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- cursor()
Return tool mouse cursor
- customEvent()
QObject.customEvent(QEvent)
- deactivate()
Deactivate tool
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- get_shape()
Reimplemented RectangularActionTool method
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.SaveAsTool(manager, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>)[source]
- activate_command(plot, checked)[source]
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- customEvent()
QObject.customEvent(QEvent)
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- register_plot(baseplot)
Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.CopyToClipboardTool(manager, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>)[source]
- activate_command(plot, checked)[source]
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- customEvent()
QObject.customEvent(QEvent)
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- register_plot(baseplot)
Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.OpenFileTool(manager, title=u'Ouvrir...', formats='*.*', toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>)[source]
- activate_command(plot, checked)[source]
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- customEvent()
QObject.customEvent(QEvent)
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- register_plot(baseplot)
Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.OpenImageTool(manager, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>)[source]
- activate_command(plot, checked)
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- customEvent()
QObject.customEvent(QEvent)
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- register_plot(baseplot)
Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.SnapshotTool(manager, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>)[source]
- activate()
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- cursor()
Return tool mouse cursor
- customEvent()
QObject.customEvent(QEvent)
- deactivate()
Deactivate tool
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- get_shape()
Reimplemented RectangularActionTool method
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
If the tool supports it, this method should install an action in the context menu
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.PrintTool(manager, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>)[source]
- activate_command(plot, checked)[source]
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- customEvent()
QObject.customEvent(QEvent)
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- register_plot(baseplot)
Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.SaveItemsTool(manager, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>)[source]
- activate_command(plot, checked)[source]
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- customEvent()
QObject.customEvent(QEvent)
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- register_plot(baseplot)
Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.LoadItemsTool(manager, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>)[source]
- activate_command(plot, checked)[source]
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- customEvent()
QObject.customEvent(QEvent)
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- register_plot(baseplot)
Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.AxisScaleTool(manager)[source]
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- customEvent()
QObject.customEvent(QEvent)
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- register_plot(baseplot)
Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- class guiqwt.tools.HelpTool(manager, toolbar_id=<class guiqwt.tools.DefaultToolbarID at 0x06007880>)[source]
- activate_command(plot, checked)[source]
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- customEvent()
QObject.customEvent(QEvent)
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- register_plot(baseplot)
Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- update_status(plot)
called by to allow derived classes to update the states of actions based on the currently active BasePlot
can also be called after an action modifying the BasePlot (e.g. in order to update action states when an item is deselected)
- class guiqwt.tools.ExportItemDataTool(manager, toolbar_id=None)[source]
- activate_command(plot, checked)
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- customEvent()
QObject.customEvent(QEvent)
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- register_plot(baseplot)
Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- class guiqwt.tools.EditItemDataTool(manager, toolbar_id=None)[source]
Edit item data (requires spyderlib)
- activate_command(plot, checked)
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- customEvent()
QObject.customEvent(QEvent)
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- register_plot(baseplot)
Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- class guiqwt.tools.ItemCenterTool(manager, toolbar_id=None)[source]
- activate_command(plot, checked)[source]
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- customEvent()
QObject.customEvent(QEvent)
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- register_plot(baseplot)
Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- class guiqwt.tools.DeleteItemTool(manager, toolbar_id=None)[source]
- activate_command(plot, checked)[source]
Activate tool
- blockSignals()
QObject.blockSignals(bool) -> bool
- childEvent()
QObject.childEvent(QChildEvent)
- children()
QObject.children() -> list-of-QObject
- connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- connectNotify()
QObject.connectNotify(SIGNAL())
- create_action(manager)
Create and return tool’s action
Create and return menu for the tool’s action
- customEvent()
QObject.customEvent(QEvent)
- deleteLater()
QObject.deleteLater()
- destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- disconnectNotify()
QObject.disconnectNotify(SIGNAL())
- dumpObjectInfo()
QObject.dumpObjectInfo()
- dumpObjectTree()
QObject.dumpObjectTree()
- dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- emit()
QObject.emit(SIGNAL(), ...)
- event()
QObject.event(QEvent) -> bool
- eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- inherits()
QObject.inherits(str) -> bool
- installEventFilter()
QObject.installEventFilter(QObject)
- isWidgetType()
QObject.isWidgetType() -> bool
- killTimer()
QObject.killTimer(int)
- metaObject()
QObject.metaObject() -> QMetaObject
- moveToThread()
QObject.moveToThread(QThread)
- objectName()
QObject.objectName() -> QString
- parent()
QObject.parent() -> QObject
- property()
QObject.property(str) -> QVariant
- pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- receivers()
QObject.receivers(SIGNAL()) -> int
- register_plot(baseplot)
Every BasePlot using this tool should call register_plot to notify the tool about this widget using it
- removeEventFilter()
QObject.removeEventFilter(QObject)
- sender()
QObject.sender() -> QObject
- setObjectName()
QObject.setObjectName(QString)
- setParent()
QObject.setParent(QObject)
- setProperty()
QObject.setProperty(str, QVariant) -> bool
- set_parent_tool(tool)
Used to organize tools automatically in menu items
- setup_toolbar(toolbar)
Setup tool’s toolbar
- signalsBlocked()
QObject.signalsBlocked() -> bool
- startTimer()
QObject.startTimer(int) -> int
- thread()
QObject.thread() -> QThread
- timerEvent()
QObject.timerEvent(QTimerEvent)
- tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
guiqwt.styles
The styles module provides set of parameters (DataSet classes) to configure plot items and plot tools.
See also
- Module guiqwt.plot
- Module providing ready-to-use curve and image plotting widgets and dialog boxes
- Module guiqwt.curve
- Module providing curve-related plot items and plotting widgets
- Module guiqwt.image
- Module providing image-related plot items and plotting widgets
- Module guiqwt.tools
- Module providing the plot tools
Reference
- class guiqwt.styles.CurveParam(title=None, comment=None, icon='')[source]
- accept(vis)
helper function that passes the visitor to the accept methods of all the items in this dataset
- baseline
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- check()
Check the dataset item values
- curvestyle
- Construct a data item for a list of choices with images
- label [string]: name
- choices [list, tuple or function]: (label, image) list or (key, label, image) list function of two arguments (item, value) returning a list of tuples (key, label, image) where image is an icon path, a QIcon instance or a function of one argument (key) returning a QIcon instance
- default [-]: default label or default key (optional)
- help [string]: text shown in tooltip (optional)
- curvetype
- Construct a data item for a list of choices with images
- label [string]: name
- choices [list, tuple or function]: (label, image) list or (key, label, image) list function of two arguments (item, value) returning a list of tuples (key, label, image) where image is an icon path, a QIcon instance or a function of one argument (key) returning a QIcon instance
- default [-]: default label or default key (optional)
- help [string]: text shown in tooltip (optional)
- edit(parent=None, apply=None, size=None)
- Open a dialog box to edit data set
- parent: parent widget (default is None, meaning no parent)
- apply: apply callback (default is None)
- size: dialog size (QSize object or integer tuple (width, height))
- fitted
- Construct a boolean data item
- text [string]: form’s field name (optional)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- get_comment()
Return data set comment
- get_icon()
Return data set icon
- get_title()
Return data set title
- label
- Construct a string data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- line
Item holding a LineStyleParam
- set_defaults()
Set default values
- shade
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- symbol
Item holding a SymbolParam
- text_edit()
Edit data set with text input only
- to_string(debug=False, indent=None, align=False)
Return readable string representation of the data set If debug is True, add more details on data items
- view(parent=None, size=None)
- Open a dialog box to view data set
- parent: parent widget (default is None, meaning no parent)
- size: dialog size (QSize object or integer tuple (width, height))
- class guiqwt.styles.ErrorBarParam(title=None, comment=None, icon='')[source]
- accept(vis)
helper function that passes the visitor to the accept methods of all the items in this dataset
- alpha
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- cap
- Construct an integer data item
- label [string]: name
- default [int]: default value (optional)
- min [int]: minimum value (optional)
- max [int]: maximum value (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- even [bool]: if True, even values are valid, if False, odd values are valid if None (default), ignored (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- help [string]: text shown in tooltip (optional)
- check()
Check the dataset item values
- color
- Construct a color data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
Color values are encoded as hexadecimal strings or Qt color names
- edit(parent=None, apply=None, size=None)
- Open a dialog box to edit data set
- parent: parent widget (default is None, meaning no parent)
- apply: apply callback (default is None)
- size: dialog size (QSize object or integer tuple (width, height))
- get_comment()
Return data set comment
- get_icon()
Return data set icon
- get_title()
Return data set title
- mode
- Construct a data item for a list of choices.
- label [string]: name
- choices [list, tuple or function]: string list or (key, label) list function of two arguments (item, value) returning a list of tuples (key, label, image) where image is an icon path, a QIcon instance or a function of one argument (key) returning a QIcon instance
- default [-]: default label or default key (optional)
- help [string]: text shown in tooltip (optional)
- ontop
- Construct a boolean data item
- text [string]: form’s field name (optional)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- set_defaults()
Set default values
- text_edit()
Edit data set with text input only
- to_string(debug=False, indent=None, align=False)
Return readable string representation of the data set If debug is True, add more details on data items
- view(parent=None, size=None)
- Open a dialog box to view data set
- parent: parent widget (default is None, meaning no parent)
- size: dialog size (QSize object or integer tuple (width, height))
- width
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- class guiqwt.styles.GridParam(title=None, comment=None, icon='')[source]
- accept(vis)
helper function that passes the visitor to the accept methods of all the items in this dataset
- background
- Construct a color data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
Color values are encoded as hexadecimal strings or Qt color names
- check()
Check the dataset item values
- edit(parent=None, apply=None, size=None)
- Open a dialog box to edit data set
- parent: parent widget (default is None, meaning no parent)
- apply: apply callback (default is None)
- size: dialog size (QSize object or integer tuple (width, height))
- get_comment()
Return data set comment
- get_icon()
Return data set icon
- get_title()
Return data set title
- maj
Data item which does not represent anything but a begin flag to define a data set group
- maj_line
Item holding a LineStyleParam
- maj_xenabled
- Construct a boolean data item
- text [string]: form’s field name (optional)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- maj_yenabled
- Construct a boolean data item
- text [string]: form’s field name (optional)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- min
Data item which does not represent anything but a begin flag to define a data set group
- min_line
Item holding a LineStyleParam
- min_xenabled
- Construct a boolean data item
- text [string]: form’s field name (optional)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- min_yenabled
- Construct a boolean data item
- text [string]: form’s field name (optional)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- set_defaults()
Set default values
- text_edit()
Edit data set with text input only
- to_string(debug=False, indent=None, align=False)
Return readable string representation of the data set If debug is True, add more details on data items
- view(parent=None, size=None)
- Open a dialog box to view data set
- parent: parent widget (default is None, meaning no parent)
- size: dialog size (QSize object or integer tuple (width, height))
- class guiqwt.styles.ImageParam(title=None, comment=None, icon='')[source]
- accept(vis)
helper function that passes the visitor to the accept methods of all the items in this dataset
- alpha
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- alpha_mask
- Construct a boolean data item
- text [string]: form’s field name (optional)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- background
- Construct a color data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
Color values are encoded as hexadecimal strings or Qt color names
- check()
Check the dataset item values
- colormap
- Construct a data item for a list of choices with images
- label [string]: name
- choices [list, tuple or function]: (label, image) list or (key, label, image) list function of two arguments (item, value) returning a list of tuples (key, label, image) where image is an icon path, a QIcon instance or a function of one argument (key) returning a QIcon instance
- default [-]: default label or default key (optional)
- help [string]: text shown in tooltip (optional)
- edit(parent=None, apply=None, size=None)
- Open a dialog box to edit data set
- parent: parent widget (default is None, meaning no parent)
- apply: apply callback (default is None)
- size: dialog size (QSize object or integer tuple (width, height))
- get_comment()
Return data set comment
- get_icon()
Return data set icon
- get_title()
Return data set title
- interpolation
- Construct a data item for a list of choices.
- label [string]: name
- choices [list, tuple or function]: string list or (key, label) list function of two arguments (item, value) returning a list of tuples (key, label, image) where image is an icon path, a QIcon instance or a function of one argument (key) returning a QIcon instance
- default [-]: default label or default key (optional)
- help [string]: text shown in tooltip (optional)
- label
- Construct a string data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- set_defaults()
Set default values
- text_edit()
Edit data set with text input only
- to_string(debug=False, indent=None, align=False)
Return readable string representation of the data set If debug is True, add more details on data items
- view(parent=None, size=None)
- Open a dialog box to view data set
- parent: parent widget (default is None, meaning no parent)
- size: dialog size (QSize object or integer tuple (width, height))
- xformat
- Construct a string data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- xmax
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- xmin
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- yformat
- Construct a string data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- ymax
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- ymin
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- zformat
- Construct a string data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- class guiqwt.styles.TrImageParam(title=None, comment=None, icon='')[source]
- accept(vis)
helper function that passes the visitor to the accept methods of all the items in this dataset
- alpha
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- alpha_mask
- Construct a boolean data item
- text [string]: form’s field name (optional)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- background
- Construct a color data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
Color values are encoded as hexadecimal strings or Qt color names
- check()
Check the dataset item values
- colormap
- Construct a data item for a list of choices with images
- label [string]: name
- choices [list, tuple or function]: (label, image) list or (key, label, image) list function of two arguments (item, value) returning a list of tuples (key, label, image) where image is an icon path, a QIcon instance or a function of one argument (key) returning a QIcon instance
- default [-]: default label or default key (optional)
- help [string]: text shown in tooltip (optional)
- crop_bottom
- Construct an integer data item
- label [string]: name
- default [int]: default value (optional)
- min [int]: minimum value (optional)
- max [int]: maximum value (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- even [bool]: if True, even values are valid, if False, odd values are valid if None (default), ignored (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- help [string]: text shown in tooltip (optional)
- crop_left
- Construct an integer data item
- label [string]: name
- default [int]: default value (optional)
- min [int]: minimum value (optional)
- max [int]: maximum value (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- even [bool]: if True, even values are valid, if False, odd values are valid if None (default), ignored (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- help [string]: text shown in tooltip (optional)
- crop_right
- Construct an integer data item
- label [string]: name
- default [int]: default value (optional)
- min [int]: minimum value (optional)
- max [int]: maximum value (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- even [bool]: if True, even values are valid, if False, odd values are valid if None (default), ignored (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- help [string]: text shown in tooltip (optional)
- crop_top
- Construct an integer data item
- label [string]: name
- default [int]: default value (optional)
- min [int]: minimum value (optional)
- max [int]: maximum value (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- even [bool]: if True, even values are valid, if False, odd values are valid if None (default), ignored (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- help [string]: text shown in tooltip (optional)
- dx
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- dy
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- edit(parent=None, apply=None, size=None)
- Open a dialog box to edit data set
- parent: parent widget (default is None, meaning no parent)
- apply: apply callback (default is None)
- size: dialog size (QSize object or integer tuple (width, height))
- get_comment()
Return data set comment
- get_icon()
Return data set icon
- get_title()
Return data set title
- hflip
- Construct a boolean data item
- text [string]: form’s field name (optional)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- interpolation
- Construct a data item for a list of choices.
- label [string]: name
- choices [list, tuple or function]: string list or (key, label) list function of two arguments (item, value) returning a list of tuples (key, label, image) where image is an icon path, a QIcon instance or a function of one argument (key) returning a QIcon instance
- default [-]: default label or default key (optional)
- help [string]: text shown in tooltip (optional)
- label
- Construct a string data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- pos_angle
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- pos_x0
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- pos_y0
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- set_defaults()
Set default values
- text_edit()
Edit data set with text input only
- to_string(debug=False, indent=None, align=False)
Return readable string representation of the data set If debug is True, add more details on data items
- vflip
- Construct a boolean data item
- text [string]: form’s field name (optional)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- view(parent=None, size=None)
- Open a dialog box to view data set
- parent: parent widget (default is None, meaning no parent)
- size: dialog size (QSize object or integer tuple (width, height))
- xformat
- Construct a string data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- yformat
- Construct a string data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- zformat
- Construct a string data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- class guiqwt.styles.ImageFilterParam(title=None, comment=None, icon='')[source]
- accept(vis)
helper function that passes the visitor to the accept methods of all the items in this dataset
- alpha
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- alpha_mask
- Construct a boolean data item
- text [string]: form’s field name (optional)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- check()
Check the dataset item values
- colormap
- Construct a data item for a list of choices with images
- label [string]: name
- choices [list, tuple or function]: (label, image) list or (key, label, image) list function of two arguments (item, value) returning a list of tuples (key, label, image) where image is an icon path, a QIcon instance or a function of one argument (key) returning a QIcon instance
- default [-]: default label or default key (optional)
- help [string]: text shown in tooltip (optional)
- edit(parent=None, apply=None, size=None)
- Open a dialog box to edit data set
- parent: parent widget (default is None, meaning no parent)
- apply: apply callback (default is None)
- size: dialog size (QSize object or integer tuple (width, height))
- g1
Data item which does not represent anything but a begin flag to define a data set group
- get_comment()
Return data set comment
- get_icon()
Return data set icon
- get_title()
Return data set title
- interpolation
- Construct a data item for a list of choices.
- label [string]: name
- choices [list, tuple or function]: string list or (key, label) list function of two arguments (item, value) returning a list of tuples (key, label, image) where image is an icon path, a QIcon instance or a function of one argument (key) returning a QIcon instance
- default [-]: default label or default key (optional)
- help [string]: text shown in tooltip (optional)
- label
- Construct a string data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- set_defaults()
Set default values
- text_edit()
Edit data set with text input only
- to_string(debug=False, indent=None, align=False)
Return readable string representation of the data set If debug is True, add more details on data items
- use_source_cmap
- Construct a boolean data item
- text [string]: form’s field name (optional)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- view(parent=None, size=None)
- Open a dialog box to view data set
- parent: parent widget (default is None, meaning no parent)
- size: dialog size (QSize object or integer tuple (width, height))
- xformat
- Construct a string data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- xmax
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- xmin
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- yformat
- Construct a string data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- ymax
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- ymin
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- zformat
- Construct a string data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- class guiqwt.styles.HistogramParam(title=None, comment=None, icon='')[source]
- accept(vis)
helper function that passes the visitor to the accept methods of all the items in this dataset
- check()
Check the dataset item values
- edit(parent=None, apply=None, size=None)
- Open a dialog box to edit data set
- parent: parent widget (default is None, meaning no parent)
- apply: apply callback (default is None)
- size: dialog size (QSize object or integer tuple (width, height))
- get_comment()
Return data set comment
- get_icon()
Return data set icon
- get_title()
Return data set title
- logscale
- Construct a boolean data item
- text [string]: form’s field name (optional)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- n_bins
- Construct an integer data item
- label [string]: name
- default [int]: default value (optional)
- min [int]: minimum value (optional)
- max [int]: maximum value (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- even [bool]: if True, even values are valid, if False, odd values are valid if None (default), ignored (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- help [string]: text shown in tooltip (optional)
- set_defaults()
Set default values
- text_edit()
Edit data set with text input only
- to_string(debug=False, indent=None, align=False)
Return readable string representation of the data set If debug is True, add more details on data items
- view(parent=None, size=None)
- Open a dialog box to view data set
- parent: parent widget (default is None, meaning no parent)
- size: dialog size (QSize object or integer tuple (width, height))
- class guiqwt.styles.Histogram2DParam(title=None, comment=None, icon='')[source]
Histogram
- accept(vis)
helper function that passes the visitor to the accept methods of all the items in this dataset
- alpha
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- alpha_mask
- Construct a boolean data item
- text [string]: form’s field name (optional)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- auto_lut
- Construct a boolean data item
- text [string]: form’s field name (optional)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- background
- Construct a color data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
Color values are encoded as hexadecimal strings or Qt color names
- check()
Check the dataset item values
- colormap
- Construct a data item for a list of choices with images
- label [string]: name
- choices [list, tuple or function]: (label, image) list or (key, label, image) list function of two arguments (item, value) returning a list of tuples (key, label, image) where image is an icon path, a QIcon instance or a function of one argument (key) returning a QIcon instance
- default [-]: default label or default key (optional)
- help [string]: text shown in tooltip (optional)
- computation
- Construct a data item for a list of choices.
- label [string]: name
- choices [list, tuple or function]: string list or (key, label) list function of two arguments (item, value) returning a list of tuples (key, label, image) where image is an icon path, a QIcon instance or a function of one argument (key) returning a QIcon instance
- default [-]: default label or default key (optional)
- help [string]: text shown in tooltip (optional)
- edit(parent=None, apply=None, size=None)
- Open a dialog box to edit data set
- parent: parent widget (default is None, meaning no parent)
- apply: apply callback (default is None)
- size: dialog size (QSize object or integer tuple (width, height))
- get_comment()
Return data set comment
- get_icon()
Return data set icon
- get_title()
Return data set title
- interpolation
- Construct a data item for a list of choices.
- label [string]: name
- choices [list, tuple or function]: string list or (key, label) list function of two arguments (item, value) returning a list of tuples (key, label, image) where image is an icon path, a QIcon instance or a function of one argument (key) returning a QIcon instance
- default [-]: default label or default key (optional)
- help [string]: text shown in tooltip (optional)
- label
- Construct a string data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- logscale
- Construct a boolean data item
- text [string]: form’s field name (optional)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- nx_bins
- Construct an integer data item
- label [string]: name
- default [int]: default value (optional)
- min [int]: minimum value (optional)
- max [int]: maximum value (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- even [bool]: if True, even values are valid, if False, odd values are valid if None (default), ignored (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- help [string]: text shown in tooltip (optional)
- ny_bins
- Construct an integer data item
- label [string]: name
- default [int]: default value (optional)
- min [int]: minimum value (optional)
- max [int]: maximum value (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- even [bool]: if True, even values are valid, if False, odd values are valid if None (default), ignored (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- help [string]: text shown in tooltip (optional)
- set_defaults()
Set default values
- text_edit()
Edit data set with text input only
- to_string(debug=False, indent=None, align=False)
Return readable string representation of the data set If debug is True, add more details on data items
- view(parent=None, size=None)
- Open a dialog box to view data set
- parent: parent widget (default is None, meaning no parent)
- size: dialog size (QSize object or integer tuple (width, height))
- xformat
- Construct a string data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- yformat
- Construct a string data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- zformat
- Construct a string data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- class guiqwt.styles.AxesParam(title=None, comment=None, icon='')[source]
- accept(vis)
helper function that passes the visitor to the accept methods of all the items in this dataset
- check()
Check the dataset item values
- edit(parent=None, apply=None, size=None)
- Open a dialog box to edit data set
- parent: parent widget (default is None, meaning no parent)
- apply: apply callback (default is None)
- size: dialog size (QSize object or integer tuple (width, height))
- get_comment()
Return data set comment
- get_icon()
Return data set icon
- get_title()
Return data set title
- set_defaults()
Set default values
- text_edit()
Edit data set with text input only
- to_string(debug=False, indent=None, align=False)
Return readable string representation of the data set If debug is True, add more details on data items
- view(parent=None, size=None)
- Open a dialog box to view data set
- parent: parent widget (default is None, meaning no parent)
- size: dialog size (QSize object or integer tuple (width, height))
- xaxis_id
- Construct a data item for a list of choices.
- label [string]: name
- choices [list, tuple or function]: string list or (key, label) list function of two arguments (item, value) returning a list of tuples (key, label, image) where image is an icon path, a QIcon instance or a function of one argument (key) returning a QIcon instance
- default [-]: default label or default key (optional)
- help [string]: text shown in tooltip (optional)
- yaxis_id
- Construct a data item for a list of choices.
- label [string]: name
- choices [list, tuple or function]: string list or (key, label) list function of two arguments (item, value) returning a list of tuples (key, label, image) where image is an icon path, a QIcon instance or a function of one argument (key) returning a QIcon instance
- default [-]: default label or default key (optional)
- help [string]: text shown in tooltip (optional)
- class guiqwt.styles.ImageAxesParam(title=None, comment=None, icon='')[source]
- accept(vis)
helper function that passes the visitor to the accept methods of all the items in this dataset
- check()
Check the dataset item values
- edit(parent=None, apply=None, size=None)
- Open a dialog box to edit data set
- parent: parent widget (default is None, meaning no parent)
- apply: apply callback (default is None)
- size: dialog size (QSize object or integer tuple (width, height))
- get_comment()
Return data set comment
- get_icon()
Return data set icon
- get_title()
Return data set title
- set_defaults()
Set default values
- text_edit()
Edit data set with text input only
- to_string(debug=False, indent=None, align=False)
Return readable string representation of the data set If debug is True, add more details on data items
- view(parent=None, size=None)
- Open a dialog box to view data set
- parent: parent widget (default is None, meaning no parent)
- size: dialog size (QSize object or integer tuple (width, height))
- xmax
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- xmin
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- xparams
Data item which does not represent anything but a begin flag to define a data set group
- ymax
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- ymin
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- yparams
Data item which does not represent anything but a begin flag to define a data set group
- zmax
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- zmin
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- zparams
Data item which does not represent anything but a begin flag to define a data set group
- class guiqwt.styles.LabelParam(title=None, comment=None, icon='')[source]
- absg
- Construct a data item for a list of choices.
- label [string]: name
- choices [list, tuple or function]: string list or (key, label) list function of two arguments (item, value) returning a list of tuples (key, label, image) where image is an icon path, a QIcon instance or a function of one argument (key) returning a QIcon instance
- default [-]: default label or default key (optional)
- help [string]: text shown in tooltip (optional)
- abspos
- Construct a boolean data item
- text [string]: form’s field name (optional)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- accept(vis)
helper function that passes the visitor to the accept methods of all the items in this dataset
- anchor
- Construct a data item for a list of choices.
- label [string]: name
- choices [list, tuple or function]: string list or (key, label) list function of two arguments (item, value) returning a list of tuples (key, label, image) where image is an icon path, a QIcon instance or a function of one argument (key) returning a QIcon instance
- default [-]: default label or default key (optional)
- help [string]: text shown in tooltip (optional)
- bgalpha
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- bgcolor
- Construct a color data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
Color values are encoded as hexadecimal strings or Qt color names
- border
Item holding a LineStyleParam
- check()
Check the dataset item values
- color
- Construct a color data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
Color values are encoded as hexadecimal strings or Qt color names
- contents
- Construct a text data item (multiline string)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- edit(parent=None, apply=None, size=None)
- Open a dialog box to edit data set
- parent: parent widget (default is None, meaning no parent)
- apply: apply callback (default is None)
- size: dialog size (QSize object or integer tuple (width, height))
- font
Item holding a LineStyleParam
- get_comment()
Return data set comment
- get_icon()
Return data set icon
- get_title()
Return data set title
- label
- Construct a string data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- move_anchor
- Construct a data item for a list of choices.
- label [string]: name
- choices [list, tuple or function]: string list or (key, label) list function of two arguments (item, value) returning a list of tuples (key, label, image) where image is an icon path, a QIcon instance or a function of one argument (key) returning a QIcon instance
- default [-]: default label or default key (optional)
- help [string]: text shown in tooltip (optional)
- set_defaults()
Set default values
- symbol
Item holding a SymbolParam
- text_edit()
Edit data set with text input only
- to_string(debug=False, indent=None, align=False)
Return readable string representation of the data set If debug is True, add more details on data items
- view(parent=None, size=None)
- Open a dialog box to view data set
- parent: parent widget (default is None, meaning no parent)
- size: dialog size (QSize object or integer tuple (width, height))
- xc
- Construct an integer data item
- label [string]: name
- default [int]: default value (optional)
- min [int]: minimum value (optional)
- max [int]: maximum value (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- even [bool]: if True, even values are valid, if False, odd values are valid if None (default), ignored (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- help [string]: text shown in tooltip (optional)
- xg
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- yc
- Construct an integer data item
- label [string]: name
- default [int]: default value (optional)
- min [int]: minimum value (optional)
- max [int]: maximum value (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- even [bool]: if True, even values are valid, if False, odd values are valid if None (default), ignored (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- help [string]: text shown in tooltip (optional)
- yg
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- class guiqwt.styles.LegendParam(title=None, comment=None, icon='')[source]
- absg
- Construct a data item for a list of choices.
- label [string]: name
- choices [list, tuple or function]: string list or (key, label) list function of two arguments (item, value) returning a list of tuples (key, label, image) where image is an icon path, a QIcon instance or a function of one argument (key) returning a QIcon instance
- default [-]: default label or default key (optional)
- help [string]: text shown in tooltip (optional)
- abspos
- Construct a boolean data item
- text [string]: form’s field name (optional)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- accept(vis)
helper function that passes the visitor to the accept methods of all the items in this dataset
- anchor
- Construct a data item for a list of choices.
- label [string]: name
- choices [list, tuple or function]: string list or (key, label) list function of two arguments (item, value) returning a list of tuples (key, label, image) where image is an icon path, a QIcon instance or a function of one argument (key) returning a QIcon instance
- default [-]: default label or default key (optional)
- help [string]: text shown in tooltip (optional)
- bgalpha
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- bgcolor
- Construct a color data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
Color values are encoded as hexadecimal strings or Qt color names
- border
Item holding a LineStyleParam
- check()
Check the dataset item values
- color
- Construct a color data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
Color values are encoded as hexadecimal strings or Qt color names
- contents
- Construct a text data item (multiline string)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- edit(parent=None, apply=None, size=None)
- Open a dialog box to edit data set
- parent: parent widget (default is None, meaning no parent)
- apply: apply callback (default is None)
- size: dialog size (QSize object or integer tuple (width, height))
- font
Item holding a LineStyleParam
- get_comment()
Return data set comment
- get_icon()
Return data set icon
- get_title()
Return data set title
- label
- Construct a string data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- move_anchor
- Construct a data item for a list of choices.
- label [string]: name
- choices [list, tuple or function]: string list or (key, label) list function of two arguments (item, value) returning a list of tuples (key, label, image) where image is an icon path, a QIcon instance or a function of one argument (key) returning a QIcon instance
- default [-]: default label or default key (optional)
- help [string]: text shown in tooltip (optional)
- set_defaults()
Set default values
- symbol
Item holding a SymbolParam
- text_edit()
Edit data set with text input only
- to_string(debug=False, indent=None, align=False)
Return readable string representation of the data set If debug is True, add more details on data items
- view(parent=None, size=None)
- Open a dialog box to view data set
- parent: parent widget (default is None, meaning no parent)
- size: dialog size (QSize object or integer tuple (width, height))
- xc
- Construct an integer data item
- label [string]: name
- default [int]: default value (optional)
- min [int]: minimum value (optional)
- max [int]: maximum value (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- even [bool]: if True, even values are valid, if False, odd values are valid if None (default), ignored (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- help [string]: text shown in tooltip (optional)
- xg
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- yc
- Construct an integer data item
- label [string]: name
- default [int]: default value (optional)
- min [int]: minimum value (optional)
- max [int]: maximum value (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- even [bool]: if True, even values are valid, if False, odd values are valid if None (default), ignored (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- help [string]: text shown in tooltip (optional)
- yg
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- class guiqwt.styles.ShapeParam(title=None, comment=None, icon='')[source]
- accept(vis)
helper function that passes the visitor to the accept methods of all the items in this dataset
- check()
Check the dataset item values
- edit(parent=None, apply=None, size=None)
- Open a dialog box to edit data set
- parent: parent widget (default is None, meaning no parent)
- apply: apply callback (default is None)
- size: dialog size (QSize object or integer tuple (width, height))
- fill
Item holding a LineStyleParam
- get_comment()
Return data set comment
- get_icon()
Return data set icon
- get_title()
Return data set title
- label
- Construct a string data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- line
Item holding a LineStyleParam
- private
- Construct a boolean data item
- text [string]: form’s field name (optional)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- readonly
- Construct a boolean data item
- text [string]: form’s field name (optional)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- sel_fill
Item holding a LineStyleParam
- sel_line
Item holding a LineStyleParam
- sel_symbol
Item holding a SymbolParam
- set_defaults()
Set default values
- symbol
Item holding a SymbolParam
- text_edit()
Edit data set with text input only
- to_string(debug=False, indent=None, align=False)
Return readable string representation of the data set If debug is True, add more details on data items
- view(parent=None, size=None)
- Open a dialog box to view data set
- parent: parent widget (default is None, meaning no parent)
- size: dialog size (QSize object or integer tuple (width, height))
- class guiqwt.styles.AnnotationParam(title=None, comment=None, icon='')[source]
- accept(vis)
helper function that passes the visitor to the accept methods of all the items in this dataset
- check()
Check the dataset item values
- edit(parent=None, apply=None, size=None)
- Open a dialog box to edit data set
- parent: parent widget (default is None, meaning no parent)
- apply: apply callback (default is None)
- size: dialog size (QSize object or integer tuple (width, height))
- format
- Construct a string data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- get_comment()
Return data set comment
- get_icon()
Return data set icon
- get_title()
Return data set title
- private
- Construct a boolean data item
- text [string]: form’s field name (optional)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- readonly
- Construct a boolean data item
- text [string]: form’s field name (optional)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- set_defaults()
Set default values
- show_computations
- Construct a boolean data item
- text [string]: form’s field name (optional)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- show_label
- Construct a boolean data item
- text [string]: form’s field name (optional)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- subtitle
- Construct a string data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- text_edit()
Edit data set with text input only
- title
- Construct a string data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- to_string(debug=False, indent=None, align=False)
Return readable string representation of the data set If debug is True, add more details on data items
- transform_matrix
- Construct a float array data item
- label [string]: name
- default [numpy.ndarray]: default value (optional)
- help [string]: text shown in tooltip (optional)
- format [string]: formatting string (example: ‘%.3f’) (optional)
- transpose [bool]: transpose matrix (display only)
- large [bool]: view all float of the array
- minmax [string]: “all” (default), “columns”, “rows”
- uncertainty
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- view(parent=None, size=None)
- Open a dialog box to view data set
- parent: parent widget (default is None, meaning no parent)
- size: dialog size (QSize object or integer tuple (width, height))
- class guiqwt.styles.AxesShapeParam(title=None, comment=None, icon='')[source]
- accept(vis)
helper function that passes the visitor to the accept methods of all the items in this dataset
- arrow_angle
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- arrow_size
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- check()
Check the dataset item values
- edit(parent=None, apply=None, size=None)
- Open a dialog box to edit data set
- parent: parent widget (default is None, meaning no parent)
- apply: apply callback (default is None)
- size: dialog size (QSize object or integer tuple (width, height))
- get_comment()
Return data set comment
- get_icon()
Return data set icon
- get_title()
Return data set title
- set_defaults()
Set default values
- text_edit()
Edit data set with text input only
- to_string(debug=False, indent=None, align=False)
Return readable string representation of the data set If debug is True, add more details on data items
- view(parent=None, size=None)
- Open a dialog box to view data set
- parent: parent widget (default is None, meaning no parent)
- size: dialog size (QSize object or integer tuple (width, height))
- xarrow_brush
Item holding a LineStyleParam
- xarrow_pen
Item holding a LineStyleParam
- yarrow_brush
Item holding a LineStyleParam
- yarrow_pen
Item holding a LineStyleParam
- class guiqwt.styles.RangeShapeParam(title=None, comment=None, icon='')[source]
- accept(vis)
helper function that passes the visitor to the accept methods of all the items in this dataset
- check()
Check the dataset item values
- edit(parent=None, apply=None, size=None)
- Open a dialog box to edit data set
- parent: parent widget (default is None, meaning no parent)
- apply: apply callback (default is None)
- size: dialog size (QSize object or integer tuple (width, height))
- fill
- Construct a color data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
Color values are encoded as hexadecimal strings or Qt color names
- get_comment()
Return data set comment
- get_icon()
Return data set icon
- get_title()
Return data set title
- line
Item holding a LineStyleParam
- sel_line
Item holding a LineStyleParam
- sel_symbol
Item holding a SymbolParam
- set_defaults()
Set default values
- shade
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- symbol
Item holding a SymbolParam
- text_edit()
Edit data set with text input only
- to_string(debug=False, indent=None, align=False)
Return readable string representation of the data set If debug is True, add more details on data items
- view(parent=None, size=None)
- Open a dialog box to view data set
- parent: parent widget (default is None, meaning no parent)
- size: dialog size (QSize object or integer tuple (width, height))
- class guiqwt.styles.MarkerParam(title=None, comment=None, icon='')[source]
- accept(vis)
helper function that passes the visitor to the accept methods of all the items in this dataset
- check()
Check the dataset item values
- edit(parent=None, apply=None, size=None)
- Open a dialog box to edit data set
- parent: parent widget (default is None, meaning no parent)
- apply: apply callback (default is None)
- size: dialog size (QSize object or integer tuple (width, height))
- get_comment()
Return data set comment
- get_icon()
Return data set icon
- get_title()
Return data set title
- line
Item holding a LineStyleParam
- markerstyle
- Construct a data item for a list of choices with images
- label [string]: name
- choices [list, tuple or function]: (label, image) list or (key, label, image) list function of two arguments (item, value) returning a list of tuples (key, label, image) where image is an icon path, a QIcon instance or a function of one argument (key) returning a QIcon instance
- default [-]: default label or default key (optional)
- help [string]: text shown in tooltip (optional)
- sel_line
Item holding a LineStyleParam
- sel_symbol
Item holding a SymbolParam
- sel_text
Item holding a TextStyleParam
- set_defaults()
Set default values
- set_markerstyle(style)[source]
Set marker line style style:
- convenient values: ‘+’, ‘-‘, ‘|’ or None
- QwtPlotMarker.NoLine, QwtPlotMarker.Vertical, ...
- spacing
- Construct an integer data item
- label [string]: name
- default [int]: default value (optional)
- min [int]: minimum value (optional)
- max [int]: maximum value (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- even [bool]: if True, even values are valid, if False, odd values are valid if None (default), ignored (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- help [string]: text shown in tooltip (optional)
- symbol
Item holding a SymbolParam
- text
Item holding a TextStyleParam
- text_edit()
Edit data set with text input only
- to_string(debug=False, indent=None, align=False)
Return readable string representation of the data set If debug is True, add more details on data items
- view(parent=None, size=None)
- Open a dialog box to view data set
- parent: parent widget (default is None, meaning no parent)
- size: dialog size (QSize object or integer tuple (width, height))
- class guiqwt.styles.FontParam(title=None, comment=None, icon='')[source]
- accept(vis)
helper function that passes the visitor to the accept methods of all the items in this dataset
- bold
- Construct a boolean data item
- text [string]: form’s field name (optional)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- check()
Check the dataset item values
- edit(parent=None, apply=None, size=None)
- Open a dialog box to edit data set
- parent: parent widget (default is None, meaning no parent)
- apply: apply callback (default is None)
- size: dialog size (QSize object or integer tuple (width, height))
- family
- Construct a string data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- notempty [bool]: if True, empty string is not a valid value (opt.)
- wordwrap [bool]: toggle word wrapping (optional)
- get_comment()
Return data set comment
- get_icon()
Return data set icon
- get_title()
Return data set title
- italic
- Construct a boolean data item
- text [string]: form’s field name (optional)
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
- set_defaults()
Set default values
- size
- Construct an integer data item
- label [string]: name
- default [int]: default value (optional)
- min [int]: minimum value (optional)
- max [int]: maximum value (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- even [bool]: if True, even values are valid, if False, odd values are valid if None (default), ignored (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- help [string]: text shown in tooltip (optional)
- text_edit()
Edit data set with text input only
- to_string(debug=False, indent=None, align=False)
Return readable string representation of the data set If debug is True, add more details on data items
- view(parent=None, size=None)
- Open a dialog box to view data set
- parent: parent widget (default is None, meaning no parent)
- size: dialog size (QSize object or integer tuple (width, height))
- class guiqwt.styles.SymbolParam(title=None, comment=None, icon='')[source]
- accept(vis)
helper function that passes the visitor to the accept methods of all the items in this dataset
- alpha
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- check()
Check the dataset item values
- edgecolor
- Construct a color data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
Color values are encoded as hexadecimal strings or Qt color names
- edit(parent=None, apply=None, size=None)
- Open a dialog box to edit data set
- parent: parent widget (default is None, meaning no parent)
- apply: apply callback (default is None)
- size: dialog size (QSize object or integer tuple (width, height))
- facecolor
- Construct a color data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
Color values are encoded as hexadecimal strings or Qt color names
- get_comment()
Return data set comment
- get_icon()
Return data set icon
- get_title()
Return data set title
- marker
- Construct a data item for a list of choices with images
- label [string]: name
- choices [list, tuple or function]: (label, image) list or (key, label, image) list function of two arguments (item, value) returning a list of tuples (key, label, image) where image is an icon path, a QIcon instance or a function of one argument (key) returning a QIcon instance
- default [-]: default label or default key (optional)
- help [string]: text shown in tooltip (optional)
- set_defaults()
Set default values
- size
- Construct an integer data item
- label [string]: name
- default [int]: default value (optional)
- min [int]: minimum value (optional)
- max [int]: maximum value (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- even [bool]: if True, even values are valid, if False, odd values are valid if None (default), ignored (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- help [string]: text shown in tooltip (optional)
- text_edit()
Edit data set with text input only
- to_string(debug=False, indent=None, align=False)
Return readable string representation of the data set If debug is True, add more details on data items
- view(parent=None, size=None)
- Open a dialog box to view data set
- parent: parent widget (default is None, meaning no parent)
- size: dialog size (QSize object or integer tuple (width, height))
- class guiqwt.styles.LineStyleParam(title=None, comment=None, icon='')[source]
- accept(vis)
helper function that passes the visitor to the accept methods of all the items in this dataset
- check()
Check the dataset item values
- color
- Construct a color data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
Color values are encoded as hexadecimal strings or Qt color names
- edit(parent=None, apply=None, size=None)
- Open a dialog box to edit data set
- parent: parent widget (default is None, meaning no parent)
- apply: apply callback (default is None)
- size: dialog size (QSize object or integer tuple (width, height))
- get_comment()
Return data set comment
- get_icon()
Return data set icon
- get_title()
Return data set title
- set_defaults()
Set default values
- set_style_from_matlab(linestyle)[source]
Eventually convert MATLAB-like linestyle into Qt linestyle
- style
- Construct a data item for a list of choices with images
- label [string]: name
- choices [list, tuple or function]: (label, image) list or (key, label, image) list function of two arguments (item, value) returning a list of tuples (key, label, image) where image is an icon path, a QIcon instance or a function of one argument (key) returning a QIcon instance
- default [-]: default label or default key (optional)
- help [string]: text shown in tooltip (optional)
- text_edit()
Edit data set with text input only
- to_string(debug=False, indent=None, align=False)
Return readable string representation of the data set If debug is True, add more details on data items
- view(parent=None, size=None)
- Open a dialog box to view data set
- parent: parent widget (default is None, meaning no parent)
- size: dialog size (QSize object or integer tuple (width, height))
- width
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- class guiqwt.styles.BrushStyleParam(title=None, comment=None, icon='')[source]
- accept(vis)
helper function that passes the visitor to the accept methods of all the items in this dataset
- alpha
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- angle
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- check()
Check the dataset item values
- color
- Construct a color data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
Color values are encoded as hexadecimal strings or Qt color names
- edit(parent=None, apply=None, size=None)
- Open a dialog box to edit data set
- parent: parent widget (default is None, meaning no parent)
- apply: apply callback (default is None)
- size: dialog size (QSize object or integer tuple (width, height))
- get_comment()
Return data set comment
- get_icon()
Return data set icon
- get_title()
Return data set title
- set_defaults()
Set default values
- style
- Construct a data item for a list of choices with images
- label [string]: name
- choices [list, tuple or function]: (label, image) list or (key, label, image) list function of two arguments (item, value) returning a list of tuples (key, label, image) where image is an icon path, a QIcon instance or a function of one argument (key) returning a QIcon instance
- default [-]: default label or default key (optional)
- help [string]: text shown in tooltip (optional)
- sx
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- sy
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- text_edit()
Edit data set with text input only
- to_string(debug=False, indent=None, align=False)
Return readable string representation of the data set If debug is True, add more details on data items
- view(parent=None, size=None)
- Open a dialog box to view data set
- parent: parent widget (default is None, meaning no parent)
- size: dialog size (QSize object or integer tuple (width, height))
- class guiqwt.styles.TextStyleParam(title=None, comment=None, icon='')[source]
- accept(vis)
helper function that passes the visitor to the accept methods of all the items in this dataset
- background_alpha
- Construct a float data item
- label [string]: name
- default [float]: default value (optional)
- min [float]: minimum value (optional)
- max [float]: maximum value (optional)
- slider [bool]: if True, shows a slider widget right after the line edit widget (default is False)
- step [float]: step between tick values with a slider widget (optional)
- nonzero [bool]: if True, zero is not a valid value (optional)
- unit [string]: physical unit (optional)
- help [string]: text shown in tooltip (optional)
- background_color
- Construct a color data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
Color values are encoded as hexadecimal strings or Qt color names
- check()
Check the dataset item values
- edit(parent=None, apply=None, size=None)
- Open a dialog box to edit data set
- parent: parent widget (default is None, meaning no parent)
- apply: apply callback (default is None)
- size: dialog size (QSize object or integer tuple (width, height))
- font
Item holding a LineStyleParam
- get_comment()
Return data set comment
- get_icon()
Return data set icon
- get_title()
Return data set title
- set_defaults()
Set default values
- text_edit()
Edit data set with text input only
- textcolor
- Construct a color data item
- label [string]: name
- default [string]: default value (optional)
- help [string]: text shown in tooltip (optional)
Color values are encoded as hexadecimal strings or Qt color names
- to_string(debug=False, indent=None, align=False)
Return readable string representation of the data set If debug is True, add more details on data items
- update_param(obj)[source]
obj: QwtText instance
- update_text(obj)[source]
obj: QwtText instance
- view(parent=None, size=None)
- Open a dialog box to view data set
- parent: parent widget (default is None, meaning no parent)
- size: dialog size (QSize object or integer tuple (width, height))
guiqwt.io
- The io module provides input/output helper functions:
- guiqwt.io.imread(): load an image (.png, .tiff, .dicom, etc.) and return its data as a NumPy array
- guiqwt.io.imwrite(): save an array to an image file
- guiqwt.io.load_items(): load plot items from HDF5
- guiqwt.io.save_items(): save plot items to HDF5
Reference
- guiqwt.io.imread(fname, ext=None, to_grayscale=False)[source]
Return a NumPy array from an image filename fname.
If to_grayscale is True, convert RGB images to grayscale The ext (optional) argument is a string that specifies the file extension which defines the input format: when not specified, the input format is guessed from filename.
- guiqwt.io.imwrite(fname, arr, ext=None, dtype=None, max_range=None, **kwargs)[source]
Save a NumPy array to an image filename fname.
If to_grayscale is True, convert RGB images to grayscale The ext (optional) argument is a string that specifies the file extension which defines the input format: when not specified, the input format is guessed from filename. If max_range is True, array data is scaled to fit the dtype (or data type itself if dtype is None) dynamic range Warning: option max_range changes data in place
- guiqwt.io.load_items(reader)[source]
Load items from HDF5 file: * reader: guidata.hdf5io.HDF5Reader object
- guiqwt.io.save_items(writer, items)[source]
Save items to HDF5 file: * writer: guidata.hdf5io.HDF5Writer object * items: serializable plot items
resizedialog
The resizedialog module provides a dialog box providing essential GUI for entering parameters needed to resize an image: guiqwt.widgets.resizedialog.ResizeDialog.
Reference
- class guiqwt.widgets.resizedialog.ResizeDialog(parent, new_size, old_size, text='')[source]
- class RenderFlags
QWidget.RenderFlags(QWidget.RenderFlags) QWidget.RenderFlags(int) QWidget.RenderFlags()
- ResizeDialog.accept()
QDialog.accept()
- ResizeDialog.acceptDrops()
QWidget.acceptDrops() -> bool
- ResizeDialog.accepted
QDialog.accepted[] [signal]
- ResizeDialog.actions()
QWidget.actions() -> list-of-QAction
- ResizeDialog.activateWindow()
QWidget.activateWindow()
- ResizeDialog.addAction()
QWidget.addAction(QAction)
- ResizeDialog.addActions()
QWidget.addActions(list-of-QAction)
- ResizeDialog.adjustSize()
QWidget.adjustSize()
- ResizeDialog.autoFillBackground()
QWidget.autoFillBackground() -> bool
- ResizeDialog.backgroundRole()
QWidget.backgroundRole() -> QPalette.ColorRole
- ResizeDialog.baseSize()
QWidget.baseSize() -> QSize
- ResizeDialog.blockSignals()
QObject.blockSignals(bool) -> bool
- ResizeDialog.childAt()
QWidget.childAt(QPoint) -> QWidget QWidget.childAt(int, int) -> QWidget
- ResizeDialog.children()
QObject.children() -> list-of-QObject
- ResizeDialog.childrenRect()
QWidget.childrenRect() -> QRect
- ResizeDialog.childrenRegion()
QWidget.childrenRegion() -> QRegion
- ResizeDialog.clearFocus()
QWidget.clearFocus()
- ResizeDialog.clearMask()
QWidget.clearMask()
- ResizeDialog.close()
QWidget.close() -> bool
- ResizeDialog.closeEvent()
QDialog.closeEvent(QCloseEvent)
- ResizeDialog.colorCount()
QPaintDevice.colorCount() -> int
- ResizeDialog.connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- ResizeDialog.contentsMargins()
QWidget.contentsMargins() -> QMargins
- ResizeDialog.contentsRect()
QWidget.contentsRect() -> QRect
- ResizeDialog.contextMenuEvent()
QDialog.contextMenuEvent(QContextMenuEvent)
- ResizeDialog.contextMenuPolicy()
QWidget.contextMenuPolicy() -> Qt.ContextMenuPolicy
- ResizeDialog.cursor()
QWidget.cursor() -> QCursor
- ResizeDialog.customContextMenuRequested
QWidget.customContextMenuRequested[QPoint] [signal]
- ResizeDialog.deleteLater()
QObject.deleteLater()
- ResizeDialog.depth()
QPaintDevice.depth() -> int
- ResizeDialog.destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- ResizeDialog.devType()
QWidget.devType() -> int
- ResizeDialog.disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- ResizeDialog.done()
QDialog.done(int)
- ResizeDialog.dumpObjectInfo()
QObject.dumpObjectInfo()
- ResizeDialog.dumpObjectTree()
QObject.dumpObjectTree()
- ResizeDialog.dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- ResizeDialog.effectiveWinId()
QWidget.effectiveWinId() -> sip.voidptr
- ResizeDialog.emit()
QObject.emit(SIGNAL(), ...)
- ResizeDialog.ensurePolished()
QWidget.ensurePolished()
- ResizeDialog.eventFilter()
QDialog.eventFilter(QObject, QEvent) -> bool
- ResizeDialog.exec_()
QDialog.exec_() -> int
- ResizeDialog.extension()
QDialog.extension() -> QWidget
- ResizeDialog.find()
QWidget.find(sip.voidptr) -> QWidget
- ResizeDialog.findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- ResizeDialog.findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- ResizeDialog.finished
QDialog.finished[int] [signal]
- ResizeDialog.focusPolicy()
QWidget.focusPolicy() -> Qt.FocusPolicy
- ResizeDialog.focusProxy()
QWidget.focusProxy() -> QWidget
- ResizeDialog.focusWidget()
QWidget.focusWidget() -> QWidget
- ResizeDialog.font()
QWidget.font() -> QFont
- ResizeDialog.fontInfo()
QWidget.fontInfo() -> QFontInfo
- ResizeDialog.fontMetrics()
QWidget.fontMetrics() -> QFontMetrics
- ResizeDialog.foregroundRole()
QWidget.foregroundRole() -> QPalette.ColorRole
- ResizeDialog.frameGeometry()
QWidget.frameGeometry() -> QRect
- ResizeDialog.frameSize()
QWidget.frameSize() -> QSize
- ResizeDialog.geometry()
QWidget.geometry() -> QRect
- ResizeDialog.getContentsMargins()
QWidget.getContentsMargins() -> (int, int, int, int)
- ResizeDialog.grabGesture()
QWidget.grabGesture(Qt.GestureType, Qt.GestureFlags flags=Qt.GestureFlags(0))
- ResizeDialog.grabKeyboard()
QWidget.grabKeyboard()
- ResizeDialog.grabMouse()
QWidget.grabMouse() QWidget.grabMouse(QCursor)
- ResizeDialog.grabShortcut()
QWidget.grabShortcut(QKeySequence, Qt.ShortcutContext context=Qt.WindowShortcut) -> int
- ResizeDialog.graphicsEffect()
QWidget.graphicsEffect() -> QGraphicsEffect
- ResizeDialog.graphicsProxyWidget()
QWidget.graphicsProxyWidget() -> QGraphicsProxyWidget
- ResizeDialog.hasFocus()
QWidget.hasFocus() -> bool
- ResizeDialog.hasMouseTracking()
QWidget.hasMouseTracking() -> bool
- ResizeDialog.height()
QWidget.height() -> int
- ResizeDialog.heightForWidth()
QWidget.heightForWidth(int) -> int
- ResizeDialog.heightMM()
QPaintDevice.heightMM() -> int
- ResizeDialog.hide()
QWidget.hide()
- ResizeDialog.inherits()
QObject.inherits(str) -> bool
- ResizeDialog.inputContext()
QWidget.inputContext() -> QInputContext
- ResizeDialog.inputMethodHints()
QWidget.inputMethodHints() -> Qt.InputMethodHints
- ResizeDialog.inputMethodQuery()
QWidget.inputMethodQuery(Qt.InputMethodQuery) -> QVariant
- ResizeDialog.insertAction()
QWidget.insertAction(QAction, QAction)
- ResizeDialog.insertActions()
QWidget.insertActions(QAction, list-of-QAction)
- ResizeDialog.installEventFilter()
QObject.installEventFilter(QObject)
- ResizeDialog.isActiveWindow()
QWidget.isActiveWindow() -> bool
- ResizeDialog.isAncestorOf()
QWidget.isAncestorOf(QWidget) -> bool
- ResizeDialog.isEnabled()
QWidget.isEnabled() -> bool
- ResizeDialog.isEnabledTo()
QWidget.isEnabledTo(QWidget) -> bool
- ResizeDialog.isEnabledToTLW()
QWidget.isEnabledToTLW() -> bool
- ResizeDialog.isFullScreen()
QWidget.isFullScreen() -> bool
- ResizeDialog.isHidden()
QWidget.isHidden() -> bool
- ResizeDialog.isLeftToRight()
QWidget.isLeftToRight() -> bool
- ResizeDialog.isMaximized()
QWidget.isMaximized() -> bool
- ResizeDialog.isMinimized()
QWidget.isMinimized() -> bool
- ResizeDialog.isModal()
QWidget.isModal() -> bool
- ResizeDialog.isRightToLeft()
QWidget.isRightToLeft() -> bool
- ResizeDialog.isSizeGripEnabled()
QDialog.isSizeGripEnabled() -> bool
- ResizeDialog.isTopLevel()
QWidget.isTopLevel() -> bool
- ResizeDialog.isVisible()
QWidget.isVisible() -> bool
- ResizeDialog.isVisibleTo()
QWidget.isVisibleTo(QWidget) -> bool
- ResizeDialog.isWidgetType()
QObject.isWidgetType() -> bool
- ResizeDialog.isWindow()
QWidget.isWindow() -> bool
- ResizeDialog.isWindowModified()
QWidget.isWindowModified() -> bool
- ResizeDialog.keyPressEvent()
QDialog.keyPressEvent(QKeyEvent)
- ResizeDialog.keyboardGrabber()
QWidget.keyboardGrabber() -> QWidget
- ResizeDialog.killTimer()
QObject.killTimer(int)
- ResizeDialog.layout()
QWidget.layout() -> QLayout
- ResizeDialog.layoutDirection()
QWidget.layoutDirection() -> Qt.LayoutDirection
- ResizeDialog.locale()
QWidget.locale() -> QLocale
- ResizeDialog.logicalDpiX()
QPaintDevice.logicalDpiX() -> int
- ResizeDialog.logicalDpiY()
QPaintDevice.logicalDpiY() -> int
- ResizeDialog.lower()
QWidget.lower()
- ResizeDialog.mapFrom()
QWidget.mapFrom(QWidget, QPoint) -> QPoint
- ResizeDialog.mapFromGlobal()
QWidget.mapFromGlobal(QPoint) -> QPoint
- ResizeDialog.mapFromParent()
QWidget.mapFromParent(QPoint) -> QPoint
- ResizeDialog.mapTo()
QWidget.mapTo(QWidget, QPoint) -> QPoint
- ResizeDialog.mapToGlobal()
QWidget.mapToGlobal(QPoint) -> QPoint
- ResizeDialog.mapToParent()
QWidget.mapToParent(QPoint) -> QPoint
- ResizeDialog.mask()
QWidget.mask() -> QRegion
- ResizeDialog.maximumHeight()
QWidget.maximumHeight() -> int
- ResizeDialog.maximumSize()
QWidget.maximumSize() -> QSize
- ResizeDialog.maximumWidth()
QWidget.maximumWidth() -> int
- ResizeDialog.metaObject()
QObject.metaObject() -> QMetaObject
- ResizeDialog.minimumHeight()
QWidget.minimumHeight() -> int
- ResizeDialog.minimumSize()
QWidget.minimumSize() -> QSize
- ResizeDialog.minimumSizeHint()
QDialog.minimumSizeHint() -> QSize
- ResizeDialog.minimumWidth()
QWidget.minimumWidth() -> int
- ResizeDialog.mouseGrabber()
QWidget.mouseGrabber() -> QWidget
- ResizeDialog.move()
QWidget.move(QPoint) QWidget.move(int, int)
- ResizeDialog.moveToThread()
QObject.moveToThread(QThread)
- ResizeDialog.nativeParentWidget()
QWidget.nativeParentWidget() -> QWidget
- ResizeDialog.nextInFocusChain()
QWidget.nextInFocusChain() -> QWidget
- ResizeDialog.normalGeometry()
QWidget.normalGeometry() -> QRect
- ResizeDialog.numColors()
QPaintDevice.numColors() -> int
- ResizeDialog.objectName()
QObject.objectName() -> QString
- ResizeDialog.open()
QDialog.open()
- ResizeDialog.orientation()
QDialog.orientation() -> Qt.Orientation
- ResizeDialog.overrideWindowFlags()
QWidget.overrideWindowFlags(Qt.WindowFlags)
- ResizeDialog.overrideWindowState()
QWidget.overrideWindowState(Qt.WindowStates)
- ResizeDialog.paintEngine()
QWidget.paintEngine() -> QPaintEngine
- ResizeDialog.paintingActive()
QPaintDevice.paintingActive() -> bool
- ResizeDialog.palette()
QWidget.palette() -> QPalette
- ResizeDialog.parent()
QObject.parent() -> QObject
- ResizeDialog.parentWidget()
QWidget.parentWidget() -> QWidget
- ResizeDialog.physicalDpiX()
QPaintDevice.physicalDpiX() -> int
- ResizeDialog.physicalDpiY()
QPaintDevice.physicalDpiY() -> int
- ResizeDialog.pos()
QWidget.pos() -> QPoint
- ResizeDialog.previousInFocusChain()
QWidget.previousInFocusChain() -> QWidget
- ResizeDialog.property()
QObject.property(str) -> QVariant
- ResizeDialog.pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- ResizeDialog.raise_()
QWidget.raise_()
- ResizeDialog.rect()
QWidget.rect() -> QRect
- ResizeDialog.reject()
QDialog.reject()
- ResizeDialog.rejected
QDialog.rejected[] [signal]
- ResizeDialog.releaseKeyboard()
QWidget.releaseKeyboard()
- ResizeDialog.releaseMouse()
QWidget.releaseMouse()
- ResizeDialog.releaseShortcut()
QWidget.releaseShortcut(int)
- ResizeDialog.removeAction()
QWidget.removeAction(QAction)
- ResizeDialog.removeEventFilter()
QObject.removeEventFilter(QObject)
- ResizeDialog.render()
QWidget.render(QPaintDevice, QPoint targetOffset=QPoint(), QRegion sourceRegion=QRegion(), QWidget.RenderFlags flags=QWidget.DrawWindowBackground|QWidget.DrawChildren) QWidget.render(QPainter, QPoint targetOffset=QPoint(), QRegion sourceRegion=QRegion(), QWidget.RenderFlags flags=QWidget.DrawWindowBackground|QWidget.DrawChildren)
- ResizeDialog.repaint()
QWidget.repaint() QWidget.repaint(int, int, int, int) QWidget.repaint(QRect) QWidget.repaint(QRegion)
- ResizeDialog.resize()
QWidget.resize(QSize) QWidget.resize(int, int)
- ResizeDialog.resizeEvent()
QDialog.resizeEvent(QResizeEvent)
- ResizeDialog.restoreGeometry()
QWidget.restoreGeometry(QByteArray) -> bool
- ResizeDialog.result()
QDialog.result() -> int
- ResizeDialog.saveGeometry()
QWidget.saveGeometry() -> QByteArray
- ResizeDialog.scroll()
QWidget.scroll(int, int) QWidget.scroll(int, int, QRect)
- ResizeDialog.setAcceptDrops()
QWidget.setAcceptDrops(bool)
- ResizeDialog.setAttribute()
QWidget.setAttribute(Qt.WidgetAttribute, bool on=True)
- ResizeDialog.setAutoFillBackground()
QWidget.setAutoFillBackground(bool)
- ResizeDialog.setBackgroundRole()
QWidget.setBackgroundRole(QPalette.ColorRole)
- ResizeDialog.setBaseSize()
QWidget.setBaseSize(int, int) QWidget.setBaseSize(QSize)
- ResizeDialog.setContentsMargins()
QWidget.setContentsMargins(int, int, int, int) QWidget.setContentsMargins(QMargins)
- ResizeDialog.setContextMenuPolicy()
QWidget.setContextMenuPolicy(Qt.ContextMenuPolicy)
- ResizeDialog.setCursor()
QWidget.setCursor(QCursor)
- ResizeDialog.setDisabled()
QWidget.setDisabled(bool)
- ResizeDialog.setEnabled()
QWidget.setEnabled(bool)
- ResizeDialog.setExtension()
QDialog.setExtension(QWidget)
- ResizeDialog.setFixedHeight()
QWidget.setFixedHeight(int)
- ResizeDialog.setFixedSize()
QWidget.setFixedSize(QSize) QWidget.setFixedSize(int, int)
- ResizeDialog.setFixedWidth()
QWidget.setFixedWidth(int)
- ResizeDialog.setFocus()
QWidget.setFocus() QWidget.setFocus(Qt.FocusReason)
- ResizeDialog.setFocusPolicy()
QWidget.setFocusPolicy(Qt.FocusPolicy)
- ResizeDialog.setFocusProxy()
QWidget.setFocusProxy(QWidget)
- ResizeDialog.setFont()
QWidget.setFont(QFont)
- ResizeDialog.setForegroundRole()
QWidget.setForegroundRole(QPalette.ColorRole)
- ResizeDialog.setGeometry()
QWidget.setGeometry(QRect) QWidget.setGeometry(int, int, int, int)
- ResizeDialog.setGraphicsEffect()
QWidget.setGraphicsEffect(QGraphicsEffect)
- ResizeDialog.setHidden()
QWidget.setHidden(bool)
- ResizeDialog.setInputContext()
QWidget.setInputContext(QInputContext)
- ResizeDialog.setInputMethodHints()
QWidget.setInputMethodHints(Qt.InputMethodHints)
- ResizeDialog.setLayout()
QWidget.setLayout(QLayout)
- ResizeDialog.setLayoutDirection()
QWidget.setLayoutDirection(Qt.LayoutDirection)
- ResizeDialog.setLocale()
QWidget.setLocale(QLocale)
- ResizeDialog.setMask()
QWidget.setMask(QBitmap) QWidget.setMask(QRegion)
- ResizeDialog.setMaximumHeight()
QWidget.setMaximumHeight(int)
- ResizeDialog.setMaximumSize()
QWidget.setMaximumSize(int, int) QWidget.setMaximumSize(QSize)
- ResizeDialog.setMaximumWidth()
QWidget.setMaximumWidth(int)
- ResizeDialog.setMinimumHeight()
QWidget.setMinimumHeight(int)
- ResizeDialog.setMinimumSize()
QWidget.setMinimumSize(int, int) QWidget.setMinimumSize(QSize)
- ResizeDialog.setMinimumWidth()
QWidget.setMinimumWidth(int)
- ResizeDialog.setModal()
QDialog.setModal(bool)
- ResizeDialog.setMouseTracking()
QWidget.setMouseTracking(bool)
- ResizeDialog.setObjectName()
QObject.setObjectName(QString)
- ResizeDialog.setOrientation()
QDialog.setOrientation(Qt.Orientation)
- ResizeDialog.setPalette()
QWidget.setPalette(QPalette)
- ResizeDialog.setParent()
QWidget.setParent(QWidget) QWidget.setParent(QWidget, Qt.WindowFlags)
- ResizeDialog.setProperty()
QObject.setProperty(str, QVariant) -> bool
- ResizeDialog.setResult()
QDialog.setResult(int)
- ResizeDialog.setShortcutAutoRepeat()
QWidget.setShortcutAutoRepeat(int, bool enabled=True)
- ResizeDialog.setShortcutEnabled()
QWidget.setShortcutEnabled(int, bool enabled=True)
- ResizeDialog.setShown()
QWidget.setShown(bool)
- ResizeDialog.setSizeGripEnabled()
QDialog.setSizeGripEnabled(bool)
- ResizeDialog.setSizeIncrement()
QWidget.setSizeIncrement(int, int) QWidget.setSizeIncrement(QSize)
- ResizeDialog.setSizePolicy()
QWidget.setSizePolicy(QSizePolicy) QWidget.setSizePolicy(QSizePolicy.Policy, QSizePolicy.Policy)
- ResizeDialog.setStatusTip()
QWidget.setStatusTip(QString)
- ResizeDialog.setStyle()
QWidget.setStyle(QStyle)
- ResizeDialog.setStyleSheet()
QWidget.setStyleSheet(QString)
- ResizeDialog.setTabOrder()
QWidget.setTabOrder(QWidget, QWidget)
- ResizeDialog.setToolTip()
QWidget.setToolTip(QString)
- ResizeDialog.setUpdatesEnabled()
QWidget.setUpdatesEnabled(bool)
- ResizeDialog.setVisible()
QDialog.setVisible(bool)
- ResizeDialog.setWhatsThis()
QWidget.setWhatsThis(QString)
- ResizeDialog.setWindowFilePath()
QWidget.setWindowFilePath(QString)
- ResizeDialog.setWindowFlags()
QWidget.setWindowFlags(Qt.WindowFlags)
- ResizeDialog.setWindowIcon()
QWidget.setWindowIcon(QIcon)
- ResizeDialog.setWindowIconText()
QWidget.setWindowIconText(QString)
- ResizeDialog.setWindowModality()
QWidget.setWindowModality(Qt.WindowModality)
- ResizeDialog.setWindowModified()
QWidget.setWindowModified(bool)
- ResizeDialog.setWindowOpacity()
QWidget.setWindowOpacity(float)
- ResizeDialog.setWindowRole()
QWidget.setWindowRole(QString)
- ResizeDialog.setWindowState()
QWidget.setWindowState(Qt.WindowStates)
- ResizeDialog.setWindowTitle()
QWidget.setWindowTitle(QString)
- ResizeDialog.show()
QWidget.show()
- ResizeDialog.showEvent()
QDialog.showEvent(QShowEvent)
- ResizeDialog.showExtension()
QDialog.showExtension(bool)
- ResizeDialog.showFullScreen()
QWidget.showFullScreen()
- ResizeDialog.showMaximized()
QWidget.showMaximized()
- ResizeDialog.showMinimized()
QWidget.showMinimized()
- ResizeDialog.showNormal()
QWidget.showNormal()
- ResizeDialog.signalsBlocked()
QObject.signalsBlocked() -> bool
- ResizeDialog.size()
QWidget.size() -> QSize
- ResizeDialog.sizeHint()
QDialog.sizeHint() -> QSize
- ResizeDialog.sizeIncrement()
QWidget.sizeIncrement() -> QSize
- ResizeDialog.sizePolicy()
QWidget.sizePolicy() -> QSizePolicy
- ResizeDialog.stackUnder()
QWidget.stackUnder(QWidget)
- ResizeDialog.startTimer()
QObject.startTimer(int) -> int
- ResizeDialog.statusTip()
QWidget.statusTip() -> QString
- ResizeDialog.style()
QWidget.style() -> QStyle
- ResizeDialog.styleSheet()
QWidget.styleSheet() -> QString
- ResizeDialog.testAttribute()
QWidget.testAttribute(Qt.WidgetAttribute) -> bool
- ResizeDialog.thread()
QObject.thread() -> QThread
- ResizeDialog.toolTip()
QWidget.toolTip() -> QString
- ResizeDialog.topLevelWidget()
QWidget.topLevelWidget() -> QWidget
- ResizeDialog.tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- ResizeDialog.trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- ResizeDialog.underMouse()
QWidget.underMouse() -> bool
- ResizeDialog.ungrabGesture()
QWidget.ungrabGesture(Qt.GestureType)
- ResizeDialog.unsetCursor()
QWidget.unsetCursor()
- ResizeDialog.unsetLayoutDirection()
QWidget.unsetLayoutDirection()
- ResizeDialog.unsetLocale()
QWidget.unsetLocale()
- ResizeDialog.update()
QWidget.update() QWidget.update(QRect) QWidget.update(QRegion) QWidget.update(int, int, int, int)
- ResizeDialog.updateGeometry()
QWidget.updateGeometry()
- ResizeDialog.updatesEnabled()
QWidget.updatesEnabled() -> bool
- ResizeDialog.visibleRegion()
QWidget.visibleRegion() -> QRegion
- ResizeDialog.whatsThis()
QWidget.whatsThis() -> QString
- ResizeDialog.width()
QWidget.width() -> int
- ResizeDialog.widthMM()
QPaintDevice.widthMM() -> int
- ResizeDialog.winId()
QWidget.winId() -> sip.voidptr
- ResizeDialog.window()
QWidget.window() -> QWidget
- ResizeDialog.windowFilePath()
QWidget.windowFilePath() -> QString
- ResizeDialog.windowFlags()
QWidget.windowFlags() -> Qt.WindowFlags
- ResizeDialog.windowIcon()
QWidget.windowIcon() -> QIcon
- ResizeDialog.windowIconText()
QWidget.windowIconText() -> QString
- ResizeDialog.windowModality()
QWidget.windowModality() -> Qt.WindowModality
- ResizeDialog.windowOpacity()
QWidget.windowOpacity() -> float
- ResizeDialog.windowRole()
QWidget.windowRole() -> QString
- ResizeDialog.windowState()
QWidget.windowState() -> Qt.WindowStates
- ResizeDialog.windowTitle()
QWidget.windowTitle() -> QString
- ResizeDialog.windowType()
QWidget.windowType() -> Qt.WindowType
- ResizeDialog.x()
QWidget.x() -> int
- ResizeDialog.y()
QWidget.y() -> int
rotatecrop
The rotatecrop module provides a dialog box providing essential GUI elements for rotating (arbitrary angle) and cropping an image:
- guiqwt.widgets.rotatecrop.RotateCropDialog: dialog box
- guiqwt.widgets.rotatecrop.RotateCropWidget: equivalent widget
Reference
- class guiqwt.widgets.rotatecrop.RotateCropDialog(parent, wintitle=None, options=None, resize_to=None)[source]
Rotate & Crop Dialog
Rotate and crop a guiqwt.image.TrImageItem plot item
- class RenderFlags
QWidget.RenderFlags(QWidget.RenderFlags) QWidget.RenderFlags(int) QWidget.RenderFlags()
- RotateCropDialog.accept()
QDialog.accept()
- RotateCropDialog.acceptDrops()
QWidget.acceptDrops() -> bool
- RotateCropDialog.accept_changes()
Computed rotated/cropped array and apply changes to item
- RotateCropDialog.accepted
QDialog.accepted[] [signal]
- RotateCropDialog.actions()
QWidget.actions() -> list-of-QAction
- RotateCropDialog.activateWindow()
QWidget.activateWindow()
- RotateCropDialog.activate_default_tool()
Activate default tool
- RotateCropDialog.addAction()
QWidget.addAction(QAction)
- RotateCropDialog.addActions()
QWidget.addActions(list-of-QAction)
Add the standard apply button
Add tool buttons to layout
- RotateCropDialog.add_panel(panel)
Register a panel to the plot manager
- Plot manager’s registration sequence is the following:
- add plots
- add panels
- add tools
- RotateCropDialog.add_plot(plot, plot_id=<class 'guiqwt.plot.DefaultPlotID'>)
- Register a plot to the plot manager:
- plot: guiqwt.curve.CurvePlot or guiqwt.image.ImagePlot object
- plot_id (default id is the plot object’s id: id(plot)): unique ID identifying the plot (any Python object), this ID will be asked by the manager to access this plot later.
- Plot manager’s registration sequence is the following:
- add plots
- add panels
- add tools
Add the standard reset button
- RotateCropDialog.add_separator_tool(toolbar_id=None)
Register a separator tool to the plot manager: the separator tool is just a tool which insert a separator in the plot context menu
- RotateCropDialog.add_tool(ToolKlass, *args, **kwargs)
- Register a tool to the manager
- ToolKlass: tool’s class (guiqwt builtin tools are defined in module guiqwt.tools)
- *args: arguments sent to the tool’s class
- **kwargs: keyword arguments sent to the tool’s class
- Plot manager’s registration sequence is the following:
- add plots
- add panels
- add tools
- RotateCropDialog.add_toolbar(toolbar, toolbar_id='default')
- Add toolbar to the plot manager
- toolbar: a QToolBar object toolbar_id: toolbar’s id (default id is string “default”)
- RotateCropDialog.adjustSize()
QWidget.adjustSize()
- RotateCropDialog.apply_transformation()
Apply transformation, e.g. crop or rotate
- RotateCropDialog.autoFillBackground()
QWidget.autoFillBackground() -> bool
- RotateCropDialog.backgroundRole()
QWidget.backgroundRole() -> QPalette.ColorRole
- RotateCropDialog.baseSize()
QWidget.baseSize() -> QSize
- RotateCropDialog.blockSignals()
QObject.blockSignals(bool) -> bool
- RotateCropDialog.childAt()
QWidget.childAt(QPoint) -> QWidget QWidget.childAt(int, int) -> QWidget
- RotateCropDialog.children()
QObject.children() -> list-of-QObject
- RotateCropDialog.childrenRect()
QWidget.childrenRect() -> QRect
- RotateCropDialog.childrenRegion()
QWidget.childrenRegion() -> QRegion
- RotateCropDialog.clearFocus()
QWidget.clearFocus()
- RotateCropDialog.clearMask()
QWidget.clearMask()
- RotateCropDialog.close()
QWidget.close() -> bool
- RotateCropDialog.closeEvent()
QDialog.closeEvent(QCloseEvent)
- RotateCropDialog.colorCount()
QPaintDevice.colorCount() -> int
- RotateCropDialog.compute_transformation()
Compute transformation, return compute output array
- RotateCropDialog.configure_panels()
Call all the registred panels ‘configure_panel’ methods to finalize the object construction (this allows to use tools registered to the same plot manager as the panel itself with breaking the registration sequence: “add plots, then panels, then tools”)
- RotateCropDialog.connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- RotateCropDialog.contentsMargins()
QWidget.contentsMargins() -> QMargins
- RotateCropDialog.contentsRect()
QWidget.contentsRect() -> QRect
- RotateCropDialog.contextMenuEvent()
QDialog.contextMenuEvent(QContextMenuEvent)
- RotateCropDialog.contextMenuPolicy()
QWidget.contextMenuPolicy() -> Qt.ContextMenuPolicy
- RotateCropDialog.create_plot(options, row=0, column=0, rowspan=1, columnspan=1)
Create the plotting widget (which is an instance of class guiqwt.plot.BaseImageWidget), add it to the dialog box main layout (guiqwt.plot.CurveDialog.plot_layout) and then add the item list, contrast adjustment and X/Y axes cross section panels.
May be overriden to customize the plot layout (guiqwt.plot.CurveDialog.plot_layout)
- RotateCropDialog.cursor()
QWidget.cursor() -> QCursor
- RotateCropDialog.customContextMenuRequested
QWidget.customContextMenuRequested[QPoint] [signal]
- RotateCropDialog.deleteLater()
QObject.deleteLater()
- RotateCropDialog.depth()
QPaintDevice.depth() -> int
- RotateCropDialog.destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- RotateCropDialog.devType()
QWidget.devType() -> int
- RotateCropDialog.disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- RotateCropDialog.done()
QDialog.done(int)
- RotateCropDialog.dumpObjectInfo()
QObject.dumpObjectInfo()
- RotateCropDialog.dumpObjectTree()
QObject.dumpObjectTree()
- RotateCropDialog.dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- RotateCropDialog.effectiveWinId()
QWidget.effectiveWinId() -> sip.voidptr
- RotateCropDialog.emit()
QObject.emit(SIGNAL(), ...)
- RotateCropDialog.ensurePolished()
QWidget.ensurePolished()
- RotateCropDialog.eventFilter()
QDialog.eventFilter(QObject, QEvent) -> bool
- RotateCropDialog.exec_()
QDialog.exec_() -> int
- RotateCropDialog.extension()
QDialog.extension() -> QWidget
- RotateCropDialog.find()
QWidget.find(sip.voidptr) -> QWidget
- RotateCropDialog.findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- RotateCropDialog.findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- RotateCropDialog.finished
QDialog.finished[int] [signal]
- RotateCropDialog.focusPolicy()
QWidget.focusPolicy() -> Qt.FocusPolicy
- RotateCropDialog.focusProxy()
QWidget.focusProxy() -> QWidget
- RotateCropDialog.focusWidget()
QWidget.focusWidget() -> QWidget
- RotateCropDialog.font()
QWidget.font() -> QFont
- RotateCropDialog.fontInfo()
QWidget.fontInfo() -> QFontInfo
- RotateCropDialog.fontMetrics()
QWidget.fontMetrics() -> QFontMetrics
- RotateCropDialog.foregroundRole()
QWidget.foregroundRole() -> QPalette.ColorRole
- RotateCropDialog.frameGeometry()
QWidget.frameGeometry() -> QRect
- RotateCropDialog.frameSize()
QWidget.frameSize() -> QSize
- RotateCropDialog.geometry()
QWidget.geometry() -> QRect
- RotateCropDialog.getContentsMargins()
QWidget.getContentsMargins() -> (int, int, int, int)
- RotateCropDialog.get_active_plot()
Return the active plot
The active plot is the plot whose canvas has the focus otherwise it’s the “default” plot
- RotateCropDialog.get_active_tool()
Return active tool
Return widget context menu – built using active tools
- RotateCropDialog.get_contrast_panel()
Convenience function to get the contrast adjustment panel
Return None if the contrast adjustment panel has not been added to this manager
- RotateCropDialog.get_default_plot()
Return default plot
The default plot is the plot on which tools and panels will act.
- RotateCropDialog.get_default_tool()
Get default tool
- RotateCropDialog.get_default_toolbar()
Return default toolbar
- RotateCropDialog.get_itemlist_panel()
Convenience function to get the item list panel
Return None if the item list panel has not been added to this manager
- RotateCropDialog.get_main()
Return the main (parent) widget
Note that for py:class:guiqwt.plot.CurveWidget or guiqwt.plot.ImageWidget objects, this method will return the widget itself because the plot manager is integrated to it.
- RotateCropDialog.get_panel(panel_id)
Return panel from its ID Panel IDs are listed in module guiqwt.panels
- RotateCropDialog.get_plot(plot_id=<class 'guiqwt.plot.DefaultPlotID'>)
Return plot associated to plot_id (if method is called without specifying the plot_id parameter, return the default plot)
- RotateCropDialog.get_plots()
Return all registered plots
- RotateCropDialog.get_tool(ToolKlass)
Return tool instance from its class
- RotateCropDialog.get_toolbar(toolbar_id='default')
- Return toolbar from its ID
- toolbar_id: toolbar’s id (default id is string “default”)
- RotateCropDialog.get_xcs_panel()
Convenience function to get the X-axis cross section panel
Return None if the X-axis cross section panel has not been added to this manager
- RotateCropDialog.get_ycs_panel()
Convenience function to get the Y-axis cross section panel
Return None if the Y-axis cross section panel has not been added to this manager
- RotateCropDialog.grabGesture()
QWidget.grabGesture(Qt.GestureType, Qt.GestureFlags flags=Qt.GestureFlags(0))
- RotateCropDialog.grabKeyboard()
QWidget.grabKeyboard()
- RotateCropDialog.grabMouse()
QWidget.grabMouse() QWidget.grabMouse(QCursor)
- RotateCropDialog.grabShortcut()
QWidget.grabShortcut(QKeySequence, Qt.ShortcutContext context=Qt.WindowShortcut) -> int
- RotateCropDialog.graphicsEffect()
QWidget.graphicsEffect() -> QGraphicsEffect
- RotateCropDialog.graphicsProxyWidget()
QWidget.graphicsProxyWidget() -> QGraphicsProxyWidget
- RotateCropDialog.hasFocus()
QWidget.hasFocus() -> bool
- RotateCropDialog.hasMouseTracking()
QWidget.hasMouseTracking() -> bool
- RotateCropDialog.height()
QWidget.height() -> int
- RotateCropDialog.heightForWidth()
QWidget.heightForWidth(int) -> int
- RotateCropDialog.heightMM()
QPaintDevice.heightMM() -> int
- RotateCropDialog.hide()
QWidget.hide()
- RotateCropDialog.inherits()
QObject.inherits(str) -> bool
- RotateCropDialog.inputContext()
QWidget.inputContext() -> QInputContext
- RotateCropDialog.inputMethodHints()
QWidget.inputMethodHints() -> Qt.InputMethodHints
- RotateCropDialog.inputMethodQuery()
QWidget.inputMethodQuery(Qt.InputMethodQuery) -> QVariant
- RotateCropDialog.insertAction()
QWidget.insertAction(QAction, QAction)
- RotateCropDialog.insertActions()
QWidget.insertActions(QAction, list-of-QAction)
- RotateCropDialog.installEventFilter()
QObject.installEventFilter(QObject)
Reimplemented ImageDialog method
- RotateCropDialog.isActiveWindow()
QWidget.isActiveWindow() -> bool
- RotateCropDialog.isAncestorOf()
QWidget.isAncestorOf(QWidget) -> bool
- RotateCropDialog.isEnabled()
QWidget.isEnabled() -> bool
- RotateCropDialog.isEnabledTo()
QWidget.isEnabledTo(QWidget) -> bool
- RotateCropDialog.isEnabledToTLW()
QWidget.isEnabledToTLW() -> bool
- RotateCropDialog.isFullScreen()
QWidget.isFullScreen() -> bool
- RotateCropDialog.isHidden()
QWidget.isHidden() -> bool
- RotateCropDialog.isLeftToRight()
QWidget.isLeftToRight() -> bool
- RotateCropDialog.isMaximized()
QWidget.isMaximized() -> bool
- RotateCropDialog.isMinimized()
QWidget.isMinimized() -> bool
- RotateCropDialog.isModal()
QWidget.isModal() -> bool
- RotateCropDialog.isRightToLeft()
QWidget.isRightToLeft() -> bool
- RotateCropDialog.isSizeGripEnabled()
QDialog.isSizeGripEnabled() -> bool
- RotateCropDialog.isTopLevel()
QWidget.isTopLevel() -> bool
- RotateCropDialog.isVisible()
QWidget.isVisible() -> bool
- RotateCropDialog.isVisibleTo()
QWidget.isVisibleTo(QWidget) -> bool
- RotateCropDialog.isWidgetType()
QObject.isWidgetType() -> bool
- RotateCropDialog.isWindow()
QWidget.isWindow() -> bool
- RotateCropDialog.isWindowModified()
QWidget.isWindowModified() -> bool
- RotateCropDialog.keyPressEvent()
QDialog.keyPressEvent(QKeyEvent)
- RotateCropDialog.keyboardGrabber()
QWidget.keyboardGrabber() -> QWidget
- RotateCropDialog.killTimer()
QObject.killTimer(int)
- RotateCropDialog.layout()
QWidget.layout() -> QLayout
- RotateCropDialog.layoutDirection()
QWidget.layoutDirection() -> Qt.LayoutDirection
- RotateCropDialog.locale()
QWidget.locale() -> QLocale
- RotateCropDialog.logicalDpiX()
QPaintDevice.logicalDpiX() -> int
- RotateCropDialog.logicalDpiY()
QPaintDevice.logicalDpiY() -> int
- RotateCropDialog.lower()
QWidget.lower()
- RotateCropDialog.mapFrom()
QWidget.mapFrom(QWidget, QPoint) -> QPoint
- RotateCropDialog.mapFromGlobal()
QWidget.mapFromGlobal(QPoint) -> QPoint
- RotateCropDialog.mapFromParent()
QWidget.mapFromParent(QPoint) -> QPoint
- RotateCropDialog.mapTo()
QWidget.mapTo(QWidget, QPoint) -> QPoint
- RotateCropDialog.mapToGlobal()
QWidget.mapToGlobal(QPoint) -> QPoint
- RotateCropDialog.mapToParent()
QWidget.mapToParent(QPoint) -> QPoint
- RotateCropDialog.mask()
QWidget.mask() -> QRegion
- RotateCropDialog.maximumHeight()
QWidget.maximumHeight() -> int
- RotateCropDialog.maximumSize()
QWidget.maximumSize() -> QSize
- RotateCropDialog.maximumWidth()
QWidget.maximumWidth() -> int
- RotateCropDialog.metaObject()
QObject.metaObject() -> QMetaObject
- RotateCropDialog.minimumHeight()
QWidget.minimumHeight() -> int
- RotateCropDialog.minimumSize()
QWidget.minimumSize() -> QSize
- RotateCropDialog.minimumSizeHint()
QDialog.minimumSizeHint() -> QSize
- RotateCropDialog.minimumWidth()
QWidget.minimumWidth() -> int
- RotateCropDialog.mouseGrabber()
QWidget.mouseGrabber() -> QWidget
- RotateCropDialog.move()
QWidget.move(QPoint) QWidget.move(int, int)
- RotateCropDialog.moveToThread()
QObject.moveToThread(QThread)
- RotateCropDialog.nativeParentWidget()
QWidget.nativeParentWidget() -> QWidget
- RotateCropDialog.nextInFocusChain()
QWidget.nextInFocusChain() -> QWidget
- RotateCropDialog.normalGeometry()
QWidget.normalGeometry() -> QRect
- RotateCropDialog.numColors()
QPaintDevice.numColors() -> int
- RotateCropDialog.objectName()
QObject.objectName() -> QString
- RotateCropDialog.open()
QDialog.open()
- RotateCropDialog.orientation()
QDialog.orientation() -> Qt.Orientation
- RotateCropDialog.overrideWindowFlags()
QWidget.overrideWindowFlags(Qt.WindowFlags)
- RotateCropDialog.overrideWindowState()
QWidget.overrideWindowState(Qt.WindowStates)
- RotateCropDialog.paintEngine()
QWidget.paintEngine() -> QPaintEngine
- RotateCropDialog.paintingActive()
QPaintDevice.paintingActive() -> bool
- RotateCropDialog.palette()
QWidget.palette() -> QPalette
- RotateCropDialog.parent()
QObject.parent() -> QObject
- RotateCropDialog.parentWidget()
QWidget.parentWidget() -> QWidget
- RotateCropDialog.physicalDpiX()
QPaintDevice.physicalDpiX() -> int
- RotateCropDialog.physicalDpiY()
QPaintDevice.physicalDpiY() -> int
- RotateCropDialog.pos()
QWidget.pos() -> QPoint
- RotateCropDialog.previousInFocusChain()
QWidget.previousInFocusChain() -> QWidget
- RotateCropDialog.property()
QObject.property(str) -> QVariant
- RotateCropDialog.pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- RotateCropDialog.raise_()
QWidget.raise_()
- RotateCropDialog.rect()
QWidget.rect() -> QRect
- RotateCropDialog.register_all_curve_tools()
Register standard, curve-related and other tools
See also
methods
guiqwt.plot.PlotManager.add_tool() guiqwt.plot.PlotManager.register_standard_tools() guiqwt.plot.PlotManager.register_other_tools() guiqwt.plot.PlotManager.register_curve_tools() guiqwt.plot.PlotManager.register_image_tools() guiqwt.plot.PlotManager.register_all_image_tools()
- RotateCropDialog.register_all_image_tools()
Register standard, image-related and other tools
See also
methods
guiqwt.plot.PlotManager.add_tool() guiqwt.plot.PlotManager.register_standard_tools() guiqwt.plot.PlotManager.register_other_tools() guiqwt.plot.PlotManager.register_curve_tools() guiqwt.plot.PlotManager.register_image_tools() guiqwt.plot.PlotManager.register_all_curve_tools()
- RotateCropDialog.register_curve_tools()
Register only curve-related tools
See also
methods
guiqwt.plot.PlotManager.add_tool() guiqwt.plot.PlotManager.register_standard_tools() guiqwt.plot.PlotManager.register_other_tools() guiqwt.plot.PlotManager.register_image_tools()
- RotateCropDialog.register_image_tools()
Register only image-related tools
See also
methods
guiqwt.plot.PlotManager.add_tool() guiqwt.plot.PlotManager.register_standard_tools() guiqwt.plot.PlotManager.register_other_tools() guiqwt.plot.PlotManager.register_curve_tools()
- RotateCropDialog.register_other_tools()
Register other common tools
See also
methods
guiqwt.plot.PlotManager.add_tool() guiqwt.plot.PlotManager.register_standard_tools() guiqwt.plot.PlotManager.register_curve_tools() guiqwt.plot.PlotManager.register_image_tools()
- RotateCropDialog.register_standard_tools()
Registering basic tools for standard plot dialog –> top of the context-menu
- RotateCropDialog.register_tools()
Register the plotting dialog box tools: the base implementation provides standard, image-related and other tools - i.e. calling this method is exactly the same as calling guiqwt.plot.CurveDialog.register_all_image_tools()
This method may be overriden to provide a fully customized set of tools
- RotateCropDialog.reject()
QDialog.reject()
- RotateCropDialog.reject_changes()
Restore item original transform settings
- RotateCropDialog.rejected
QDialog.rejected[] [signal]
- RotateCropDialog.releaseKeyboard()
QWidget.releaseKeyboard()
- RotateCropDialog.releaseMouse()
QWidget.releaseMouse()
- RotateCropDialog.releaseShortcut()
QWidget.releaseShortcut(int)
- RotateCropDialog.removeAction()
QWidget.removeAction(QAction)
- RotateCropDialog.removeEventFilter()
QObject.removeEventFilter(QObject)
- RotateCropDialog.render()
QWidget.render(QPaintDevice, QPoint targetOffset=QPoint(), QRegion sourceRegion=QRegion(), QWidget.RenderFlags flags=QWidget.DrawWindowBackground|QWidget.DrawChildren) QWidget.render(QPainter, QPoint targetOffset=QPoint(), QRegion sourceRegion=QRegion(), QWidget.RenderFlags flags=QWidget.DrawWindowBackground|QWidget.DrawChildren)
- RotateCropDialog.repaint()
QWidget.repaint() QWidget.repaint(int, int, int, int) QWidget.repaint(QRect) QWidget.repaint(QRegion)
- RotateCropDialog.reset()
Reset crop/transform image settings
- RotateCropDialog.reset_transformation()
Reset transformation
- RotateCropDialog.resize()
QWidget.resize(QSize) QWidget.resize(int, int)
- RotateCropDialog.resizeEvent()
QDialog.resizeEvent(QResizeEvent)
- RotateCropDialog.restoreGeometry()
QWidget.restoreGeometry(QByteArray) -> bool
- RotateCropDialog.restore_original_state()
Restore item original state
- RotateCropDialog.result()
QDialog.result() -> int
- RotateCropDialog.saveGeometry()
QWidget.saveGeometry() -> QByteArray
- RotateCropDialog.scroll()
QWidget.scroll(int, int) QWidget.scroll(int, int, QRect)
- RotateCropDialog.setAcceptDrops()
QWidget.setAcceptDrops(bool)
- RotateCropDialog.setAttribute()
QWidget.setAttribute(Qt.WidgetAttribute, bool on=True)
- RotateCropDialog.setAutoFillBackground()
QWidget.setAutoFillBackground(bool)
- RotateCropDialog.setBackgroundRole()
QWidget.setBackgroundRole(QPalette.ColorRole)
- RotateCropDialog.setBaseSize()
QWidget.setBaseSize(int, int) QWidget.setBaseSize(QSize)
- RotateCropDialog.setContentsMargins()
QWidget.setContentsMargins(int, int, int, int) QWidget.setContentsMargins(QMargins)
- RotateCropDialog.setContextMenuPolicy()
QWidget.setContextMenuPolicy(Qt.ContextMenuPolicy)
- RotateCropDialog.setCursor()
QWidget.setCursor(QCursor)
- RotateCropDialog.setDisabled()
QWidget.setDisabled(bool)
- RotateCropDialog.setEnabled()
QWidget.setEnabled(bool)
- RotateCropDialog.setExtension()
QDialog.setExtension(QWidget)
- RotateCropDialog.setFixedHeight()
QWidget.setFixedHeight(int)
- RotateCropDialog.setFixedSize()
QWidget.setFixedSize(QSize) QWidget.setFixedSize(int, int)
- RotateCropDialog.setFixedWidth()
QWidget.setFixedWidth(int)
- RotateCropDialog.setFocus()
QWidget.setFocus() QWidget.setFocus(Qt.FocusReason)
- RotateCropDialog.setFocusPolicy()
QWidget.setFocusPolicy(Qt.FocusPolicy)
- RotateCropDialog.setFocusProxy()
QWidget.setFocusProxy(QWidget)
- RotateCropDialog.setFont()
QWidget.setFont(QFont)
- RotateCropDialog.setForegroundRole()
QWidget.setForegroundRole(QPalette.ColorRole)
- RotateCropDialog.setGeometry()
QWidget.setGeometry(QRect) QWidget.setGeometry(int, int, int, int)
- RotateCropDialog.setGraphicsEffect()
QWidget.setGraphicsEffect(QGraphicsEffect)
- RotateCropDialog.setHidden()
QWidget.setHidden(bool)
- RotateCropDialog.setInputContext()
QWidget.setInputContext(QInputContext)
- RotateCropDialog.setInputMethodHints()
QWidget.setInputMethodHints(Qt.InputMethodHints)
- RotateCropDialog.setLayout()
QWidget.setLayout(QLayout)
- RotateCropDialog.setLayoutDirection()
QWidget.setLayoutDirection(Qt.LayoutDirection)
- RotateCropDialog.setLocale()
QWidget.setLocale(QLocale)
- RotateCropDialog.setMask()
QWidget.setMask(QBitmap) QWidget.setMask(QRegion)
- RotateCropDialog.setMaximumHeight()
QWidget.setMaximumHeight(int)
- RotateCropDialog.setMaximumSize()
QWidget.setMaximumSize(int, int) QWidget.setMaximumSize(QSize)
- RotateCropDialog.setMaximumWidth()
QWidget.setMaximumWidth(int)
- RotateCropDialog.setMinimumHeight()
QWidget.setMinimumHeight(int)
- RotateCropDialog.setMinimumSize()
QWidget.setMinimumSize(int, int) QWidget.setMinimumSize(QSize)
- RotateCropDialog.setMinimumWidth()
QWidget.setMinimumWidth(int)
- RotateCropDialog.setModal()
QDialog.setModal(bool)
- RotateCropDialog.setMouseTracking()
QWidget.setMouseTracking(bool)
- RotateCropDialog.setObjectName()
QObject.setObjectName(QString)
- RotateCropDialog.setOrientation()
QDialog.setOrientation(Qt.Orientation)
- RotateCropDialog.setPalette()
QWidget.setPalette(QPalette)
- RotateCropDialog.setParent()
QWidget.setParent(QWidget) QWidget.setParent(QWidget, Qt.WindowFlags)
- RotateCropDialog.setProperty()
QObject.setProperty(str, QVariant) -> bool
- RotateCropDialog.setResult()
QDialog.setResult(int)
- RotateCropDialog.setShortcutAutoRepeat()
QWidget.setShortcutAutoRepeat(int, bool enabled=True)
- RotateCropDialog.setShortcutEnabled()
QWidget.setShortcutEnabled(int, bool enabled=True)
- RotateCropDialog.setShown()
QWidget.setShown(bool)
- RotateCropDialog.setSizeGripEnabled()
QDialog.setSizeGripEnabled(bool)
- RotateCropDialog.setSizeIncrement()
QWidget.setSizeIncrement(int, int) QWidget.setSizeIncrement(QSize)
- RotateCropDialog.setSizePolicy()
QWidget.setSizePolicy(QSizePolicy) QWidget.setSizePolicy(QSizePolicy.Policy, QSizePolicy.Policy)
- RotateCropDialog.setStatusTip()
QWidget.setStatusTip(QString)
- RotateCropDialog.setStyle()
QWidget.setStyle(QStyle)
- RotateCropDialog.setStyleSheet()
QWidget.setStyleSheet(QString)
- RotateCropDialog.setTabOrder()
QWidget.setTabOrder(QWidget, QWidget)
- RotateCropDialog.setToolTip()
QWidget.setToolTip(QString)
- RotateCropDialog.setUpdatesEnabled()
QWidget.setUpdatesEnabled(bool)
- RotateCropDialog.setVisible()
QDialog.setVisible(bool)
- RotateCropDialog.setWhatsThis()
QWidget.setWhatsThis(QString)
- RotateCropDialog.setWindowFilePath()
QWidget.setWindowFilePath(QString)
- RotateCropDialog.setWindowFlags()
QWidget.setWindowFlags(Qt.WindowFlags)
- RotateCropDialog.setWindowIcon()
QWidget.setWindowIcon(QIcon)
- RotateCropDialog.setWindowIconText()
QWidget.setWindowIconText(QString)
- RotateCropDialog.setWindowModality()
QWidget.setWindowModality(Qt.WindowModality)
- RotateCropDialog.setWindowModified()
QWidget.setWindowModified(bool)
- RotateCropDialog.setWindowOpacity()
QWidget.setWindowOpacity(float)
- RotateCropDialog.setWindowRole()
QWidget.setWindowRole(QString)
- RotateCropDialog.setWindowState()
QWidget.setWindowState(Qt.WindowStates)
- RotateCropDialog.setWindowTitle()
QWidget.setWindowTitle(QString)
- RotateCropDialog.set_active_tool(tool=None)
Set active tool (if tool argument is None, the active tool will be the default tool)
- RotateCropDialog.set_contrast_range(zmin, zmax)
Convenience function to set the contrast adjustment panel range
This is strictly equivalent to the following:
# Here, *widget* is for example a CurveWidget instance # (the same apply for CurvePlot, ImageWidget, ImagePlot or any # class deriving from PlotManager) widget.get_contrast_panel().set_range(zmin, zmax)
- RotateCropDialog.set_default_plot(plot)
Set default plot
The default plot is the plot on which tools and panels will act.
- RotateCropDialog.set_default_tool(tool)
Set default tool
- RotateCropDialog.set_default_toolbar(toolbar)
Set default toolbar
- RotateCropDialog.set_item(item)
Set associated item – must be a TrImageItem object
- RotateCropDialog.show()
QWidget.show()
- RotateCropDialog.showEvent()
QDialog.showEvent(QShowEvent)
- RotateCropDialog.showExtension()
QDialog.showExtension(bool)
- RotateCropDialog.showFullScreen()
QWidget.showFullScreen()
- RotateCropDialog.showMaximized()
QWidget.showMaximized()
- RotateCropDialog.showMinimized()
QWidget.showMinimized()
- RotateCropDialog.showNormal()
QWidget.showNormal()
- RotateCropDialog.show_crop_rect(state)
Show/hide cropping rectangle shape
- RotateCropDialog.signalsBlocked()
QObject.signalsBlocked() -> bool
- RotateCropDialog.size()
QWidget.size() -> QSize
- RotateCropDialog.sizeHint()
QDialog.sizeHint() -> QSize
- RotateCropDialog.sizeIncrement()
QWidget.sizeIncrement() -> QSize
- RotateCropDialog.sizePolicy()
QWidget.sizePolicy() -> QSizePolicy
- RotateCropDialog.stackUnder()
QWidget.stackUnder(QWidget)
- RotateCropDialog.startTimer()
QObject.startTimer(int) -> int
- RotateCropDialog.statusTip()
QWidget.statusTip() -> QString
- RotateCropDialog.style()
QWidget.style() -> QStyle
- RotateCropDialog.styleSheet()
QWidget.styleSheet() -> QString
- RotateCropDialog.testAttribute()
QWidget.testAttribute(Qt.WidgetAttribute) -> bool
- RotateCropDialog.thread()
QObject.thread() -> QThread
- RotateCropDialog.toolTip()
QWidget.toolTip() -> QString
- RotateCropDialog.topLevelWidget()
QWidget.topLevelWidget() -> QWidget
- RotateCropDialog.tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- RotateCropDialog.trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- RotateCropDialog.underMouse()
QWidget.underMouse() -> bool
- RotateCropDialog.ungrabGesture()
QWidget.ungrabGesture(Qt.GestureType)
- RotateCropDialog.unsetCursor()
QWidget.unsetCursor()
- RotateCropDialog.unsetLayoutDirection()
QWidget.unsetLayoutDirection()
- RotateCropDialog.unsetLocale()
QWidget.unsetLocale()
- RotateCropDialog.unset_item()
Unset the associated item, freeing memory
- RotateCropDialog.update()
QWidget.update() QWidget.update(QRect) QWidget.update(QRegion) QWidget.update(int, int, int, int)
- RotateCropDialog.updateGeometry()
QWidget.updateGeometry()
- RotateCropDialog.update_cross_sections()
Convenience function to update the cross section panels at once
This is strictly equivalent to the following:
# Here, *widget* is for example a CurveWidget instance # (the same apply for CurvePlot, ImageWidget, ImagePlot or any # class deriving from PlotManager) widget.get_xcs_panel().update_plot() widget.get_ycs_panel().update_plot()
- RotateCropDialog.update_tools_status(plot=None)
Update tools for current plot
- RotateCropDialog.updatesEnabled()
QWidget.updatesEnabled() -> bool
- RotateCropDialog.visibleRegion()
QWidget.visibleRegion() -> QRegion
- RotateCropDialog.whatsThis()
QWidget.whatsThis() -> QString
- RotateCropDialog.width()
QWidget.width() -> int
- RotateCropDialog.widthMM()
QPaintDevice.widthMM() -> int
- RotateCropDialog.winId()
QWidget.winId() -> sip.voidptr
- RotateCropDialog.window()
QWidget.window() -> QWidget
- RotateCropDialog.windowFilePath()
QWidget.windowFilePath() -> QString
- RotateCropDialog.windowFlags()
QWidget.windowFlags() -> Qt.WindowFlags
- RotateCropDialog.windowIcon()
QWidget.windowIcon() -> QIcon
- RotateCropDialog.windowIconText()
QWidget.windowIconText() -> QString
- RotateCropDialog.windowModality()
QWidget.windowModality() -> Qt.WindowModality
- RotateCropDialog.windowOpacity()
QWidget.windowOpacity() -> float
- RotateCropDialog.windowRole()
QWidget.windowRole() -> QString
- RotateCropDialog.windowState()
QWidget.windowState() -> Qt.WindowStates
- RotateCropDialog.windowTitle()
QWidget.windowTitle() -> QString
- RotateCropDialog.windowType()
QWidget.windowType() -> Qt.WindowType
- RotateCropDialog.x()
QWidget.x() -> int
- RotateCropDialog.y()
QWidget.y() -> int
- class guiqwt.widgets.rotatecrop.RotateCropWidget(parent, options=None)[source]
Rotate & Crop Widget
Rotate and crop a guiqwt.image.TrImageItem plot item
- class RenderFlags
QWidget.RenderFlags(QWidget.RenderFlags) QWidget.RenderFlags(int) QWidget.RenderFlags()
- RotateCropWidget.acceptDrops()
QWidget.acceptDrops() -> bool
- RotateCropWidget.accept_changes()
Computed rotated/cropped array and apply changes to item
- RotateCropWidget.actionEvent()
QWidget.actionEvent(QActionEvent)
- RotateCropWidget.actions()
QWidget.actions() -> list-of-QAction
- RotateCropWidget.activateWindow()
QWidget.activateWindow()
- RotateCropWidget.addAction()
QWidget.addAction(QAction)
- RotateCropWidget.addActions()
QWidget.addActions(list-of-QAction)
Add the standard apply button
Add tool buttons to layout
Add the standard reset button
- RotateCropWidget.adjustSize()
QWidget.adjustSize()
- RotateCropWidget.apply_transformation()
Apply transformation, e.g. crop or rotate
- RotateCropWidget.autoFillBackground()
QWidget.autoFillBackground() -> bool
- RotateCropWidget.backgroundRole()
QWidget.backgroundRole() -> QPalette.ColorRole
- RotateCropWidget.baseSize()
QWidget.baseSize() -> QSize
- RotateCropWidget.blockSignals()
QObject.blockSignals(bool) -> bool
- RotateCropWidget.changeEvent()
QWidget.changeEvent(QEvent)
- RotateCropWidget.childAt()
QWidget.childAt(QPoint) -> QWidget QWidget.childAt(int, int) -> QWidget
- RotateCropWidget.children()
QObject.children() -> list-of-QObject
- RotateCropWidget.childrenRect()
QWidget.childrenRect() -> QRect
- RotateCropWidget.childrenRegion()
QWidget.childrenRegion() -> QRegion
- RotateCropWidget.clearFocus()
QWidget.clearFocus()
- RotateCropWidget.clearMask()
QWidget.clearMask()
- RotateCropWidget.close()
QWidget.close() -> bool
- RotateCropWidget.closeEvent()
QWidget.closeEvent(QCloseEvent)
- RotateCropWidget.colorCount()
QPaintDevice.colorCount() -> int
- RotateCropWidget.compute_transformation()
Compute transformation, return compute output array
- RotateCropWidget.connect()
QObject.connect(QObject, SIGNAL(), QObject, SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), callable, Qt.ConnectionType=Qt.AutoConnection) -> bool QObject.connect(QObject, SIGNAL(), SLOT(), Qt.ConnectionType=Qt.AutoConnection) -> bool
- RotateCropWidget.contentsMargins()
QWidget.contentsMargins() -> QMargins
- RotateCropWidget.contentsRect()
QWidget.contentsRect() -> QRect
- RotateCropWidget.contextMenuEvent()
QWidget.contextMenuEvent(QContextMenuEvent)
- RotateCropWidget.contextMenuPolicy()
QWidget.contextMenuPolicy() -> Qt.ContextMenuPolicy
- RotateCropWidget.create()
QWidget.create(sip.voidptr window=None, bool initializeWindow=True, bool destroyOldWindow=True)
- RotateCropWidget.cursor()
QWidget.cursor() -> QCursor
- RotateCropWidget.customContextMenuRequested
QWidget.customContextMenuRequested[QPoint] [signal]
- RotateCropWidget.deleteLater()
QObject.deleteLater()
- RotateCropWidget.depth()
QPaintDevice.depth() -> int
- RotateCropWidget.destroy()
QWidget.destroy(bool destroyWindow=True, bool destroySubWindows=True)
- RotateCropWidget.destroyed
QObject.destroyed[QObject] [signal] QObject.destroyed[] [signal]
- RotateCropWidget.devType()
QWidget.devType() -> int
- RotateCropWidget.disconnect()
QObject.disconnect(QObject, SIGNAL(), QObject, SLOT()) -> bool QObject.disconnect(QObject, SIGNAL(), callable) -> bool
- RotateCropWidget.dragEnterEvent()
QWidget.dragEnterEvent(QDragEnterEvent)
- RotateCropWidget.dragLeaveEvent()
QWidget.dragLeaveEvent(QDragLeaveEvent)
- RotateCropWidget.dragMoveEvent()
QWidget.dragMoveEvent(QDragMoveEvent)
- RotateCropWidget.dropEvent()
QWidget.dropEvent(QDropEvent)
- RotateCropWidget.dumpObjectInfo()
QObject.dumpObjectInfo()
- RotateCropWidget.dumpObjectTree()
QObject.dumpObjectTree()
- RotateCropWidget.dynamicPropertyNames()
QObject.dynamicPropertyNames() -> list-of-QByteArray
- RotateCropWidget.effectiveWinId()
QWidget.effectiveWinId() -> sip.voidptr
- RotateCropWidget.emit()
QObject.emit(SIGNAL(), ...)
- RotateCropWidget.enabledChange()
QWidget.enabledChange(bool)
- RotateCropWidget.ensurePolished()
QWidget.ensurePolished()
- RotateCropWidget.enterEvent()
QWidget.enterEvent(QEvent)
- RotateCropWidget.event()
QWidget.event(QEvent) -> bool
- RotateCropWidget.eventFilter()
QObject.eventFilter(QObject, QEvent) -> bool
- RotateCropWidget.find()
QWidget.find(sip.voidptr) -> QWidget
- RotateCropWidget.findChild()
QObject.findChild(type, QString name=QString()) -> QObject QObject.findChild(tuple, QString name=QString()) -> QObject
- RotateCropWidget.findChildren()
QObject.findChildren(type, QString name=QString()) -> list-of-QObject QObject.findChildren(tuple, QString name=QString()) -> list-of-QObject QObject.findChildren(type, QRegExp) -> list-of-QObject QObject.findChildren(tuple, QRegExp) -> list-of-QObject
- RotateCropWidget.focusInEvent()
QWidget.focusInEvent(QFocusEvent)
- RotateCropWidget.focusNextChild()
QWidget.focusNextChild() -> bool
- RotateCropWidget.focusNextPrevChild()
QWidget.focusNextPrevChild(bool) -> bool
- RotateCropWidget.focusOutEvent()
QWidget.focusOutEvent(QFocusEvent)
- RotateCropWidget.focusPolicy()
QWidget.focusPolicy() -> Qt.FocusPolicy
- RotateCropWidget.focusPreviousChild()
QWidget.focusPreviousChild() -> bool
- RotateCropWidget.focusProxy()
QWidget.focusProxy() -> QWidget
- RotateCropWidget.focusWidget()
QWidget.focusWidget() -> QWidget
- RotateCropWidget.font()
QWidget.font() -> QFont
- RotateCropWidget.fontChange()
QWidget.fontChange(QFont)
- RotateCropWidget.fontInfo()
QWidget.fontInfo() -> QFontInfo
- RotateCropWidget.fontMetrics()
QWidget.fontMetrics() -> QFontMetrics
- RotateCropWidget.foregroundRole()
QWidget.foregroundRole() -> QPalette.ColorRole
- RotateCropWidget.frameGeometry()
QWidget.frameGeometry() -> QRect
- RotateCropWidget.frameSize()
QWidget.frameSize() -> QSize
- RotateCropWidget.geometry()
QWidget.geometry() -> QRect
- RotateCropWidget.getContentsMargins()
QWidget.getContentsMargins() -> (int, int, int, int)
- RotateCropWidget.get_plot()
Required for BaseTransformMixin
- RotateCropWidget.grabGesture()
QWidget.grabGesture(Qt.GestureType, Qt.GestureFlags flags=Qt.GestureFlags(0))
- RotateCropWidget.grabKeyboard()
QWidget.grabKeyboard()
- RotateCropWidget.grabMouse()
QWidget.grabMouse() QWidget.grabMouse(QCursor)
- RotateCropWidget.grabShortcut()
QWidget.grabShortcut(QKeySequence, Qt.ShortcutContext context=Qt.WindowShortcut) -> int
- RotateCropWidget.graphicsEffect()
QWidget.graphicsEffect() -> QGraphicsEffect
- RotateCropWidget.graphicsProxyWidget()
QWidget.graphicsProxyWidget() -> QGraphicsProxyWidget
- RotateCropWidget.hasFocus()
QWidget.hasFocus() -> bool
- RotateCropWidget.hasMouseTracking()
QWidget.hasMouseTracking() -> bool
- RotateCropWidget.height()
QWidget.height() -> int
- RotateCropWidget.heightForWidth()
QWidget.heightForWidth(int) -> int
- RotateCropWidget.heightMM()
QPaintDevice.heightMM() -> int
- RotateCropWidget.hide()
QWidget.hide()
- RotateCropWidget.hideEvent()
QWidget.hideEvent(QHideEvent)
- RotateCropWidget.inherits()
QObject.inherits(str) -> bool
- RotateCropWidget.inputContext()
QWidget.inputContext() -> QInputContext
- RotateCropWidget.inputMethodEvent()
QWidget.inputMethodEvent(QInputMethodEvent)
- RotateCropWidget.inputMethodHints()
QWidget.inputMethodHints() -> Qt.InputMethodHints
- RotateCropWidget.inputMethodQuery()
QWidget.inputMethodQuery(Qt.InputMethodQuery) -> QVariant
- RotateCropWidget.insertAction()
QWidget.insertAction(QAction, QAction)
- RotateCropWidget.insertActions()
QWidget.insertActions(QAction, list-of-QAction)
- RotateCropWidget.installEventFilter()
QObject.installEventFilter(QObject)
- RotateCropWidget.isActiveWindow()
QWidget.isActiveWindow() -> bool
- RotateCropWidget.isAncestorOf()
QWidget.isAncestorOf(QWidget) -> bool
- RotateCropWidget.isEnabled()
QWidget.isEnabled() -> bool
- RotateCropWidget.isEnabledTo()
QWidget.isEnabledTo(QWidget) -> bool
- RotateCropWidget.isEnabledToTLW()
QWidget.isEnabledToTLW() -> bool
- RotateCropWidget.isFullScreen()
QWidget.isFullScreen() -> bool
- RotateCropWidget.isHidden()
QWidget.isHidden() -> bool
- RotateCropWidget.isLeftToRight()
QWidget.isLeftToRight() -> bool
- RotateCropWidget.isMaximized()
QWidget.isMaximized() -> bool
- RotateCropWidget.isMinimized()
QWidget.isMinimized() -> bool
- RotateCropWidget.isModal()
QWidget.isModal() -> bool
- RotateCropWidget.isRightToLeft()
QWidget.isRightToLeft() -> bool
- RotateCropWidget.isTopLevel()
QWidget.isTopLevel() -> bool
- RotateCropWidget.isVisible()
QWidget.isVisible() -> bool
- RotateCropWidget.isVisibleTo()
QWidget.isVisibleTo(QWidget) -> bool
- RotateCropWidget.isWidgetType()
QObject.isWidgetType() -> bool
- RotateCropWidget.isWindow()
QWidget.isWindow() -> bool
- RotateCropWidget.isWindowModified()
QWidget.isWindowModified() -> bool
- RotateCropWidget.keyPressEvent()
QWidget.keyPressEvent(QKeyEvent)
- RotateCropWidget.keyReleaseEvent()
QWidget.keyReleaseEvent(QKeyEvent)
- RotateCropWidget.keyboardGrabber()
QWidget.keyboardGrabber() -> QWidget
- RotateCropWidget.killTimer()
QObject.killTimer(int)
- RotateCropWidget.languageChange()
QWidget.languageChange()
- RotateCropWidget.layout()
QWidget.layout() -> QLayout
- RotateCropWidget.layoutDirection()
QWidget.layoutDirection() -> Qt.LayoutDirection
- RotateCropWidget.leaveEvent()
QWidget.leaveEvent(QEvent)
- RotateCropWidget.locale()
QWidget.locale() -> QLocale
- RotateCropWidget.logicalDpiX()
QPaintDevice.logicalDpiX() -> int
- RotateCropWidget.logicalDpiY()
QPaintDevice.logicalDpiY() -> int
- RotateCropWidget.lower()
QWidget.lower()
- RotateCropWidget.mapFrom()
QWidget.mapFrom(QWidget, QPoint) -> QPoint
- RotateCropWidget.mapFromGlobal()
QWidget.mapFromGlobal(QPoint) -> QPoint
- RotateCropWidget.mapFromParent()
QWidget.mapFromParent(QPoint) -> QPoint
- RotateCropWidget.mapTo()
QWidget.mapTo(QWidget, QPoint) -> QPoint
- RotateCropWidget.mapToGlobal()
QWidget.mapToGlobal(QPoint) -> QPoint
- RotateCropWidget.mapToParent()
QWidget.mapToParent(QPoint) -> QPoint
- RotateCropWidget.mask()
QWidget.mask() -> QRegion
- RotateCropWidget.maximumHeight()
QWidget.maximumHeight() -> int
- RotateCropWidget.maximumSize()
QWidget.maximumSize() -> QSize
- RotateCropWidget.maximumWidth()
QWidget.maximumWidth() -> int
- RotateCropWidget.metaObject()
QObject.metaObject() -> QMetaObject
- RotateCropWidget.metric()
QWidget.metric(QPaintDevice.PaintDeviceMetric) -> int
- RotateCropWidget.minimumHeight()
QWidget.minimumHeight() -> int
- RotateCropWidget.minimumSize()
QWidget.minimumSize() -> QSize
- RotateCropWidget.minimumSizeHint()
QWidget.minimumSizeHint() -> QSize
- RotateCropWidget.minimumWidth()
QWidget.minimumWidth() -> int
- RotateCropWidget.mouseDoubleClickEvent()
QWidget.mouseDoubleClickEvent(QMouseEvent)
- RotateCropWidget.mouseGrabber()
QWidget.mouseGrabber() -> QWidget
- RotateCropWidget.mouseMoveEvent()
QWidget.mouseMoveEvent(QMouseEvent)
- RotateCropWidget.mousePressEvent()
QWidget.mousePressEvent(QMouseEvent)
- RotateCropWidget.mouseReleaseEvent()
QWidget.mouseReleaseEvent(QMouseEvent)
- RotateCropWidget.move()
QWidget.move(QPoint) QWidget.move(int, int)
- RotateCropWidget.moveEvent()
QWidget.moveEvent(QMoveEvent)
- RotateCropWidget.moveToThread()
QObject.moveToThread(QThread)
- RotateCropWidget.nativeParentWidget()
QWidget.nativeParentWidget() -> QWidget
- RotateCropWidget.nextInFocusChain()
QWidget.nextInFocusChain() -> QWidget
- RotateCropWidget.normalGeometry()
QWidget.normalGeometry() -> QRect
- RotateCropWidget.numColors()
QPaintDevice.numColors() -> int
- RotateCropWidget.objectName()
QObject.objectName() -> QString
- RotateCropWidget.overrideWindowFlags()
QWidget.overrideWindowFlags(Qt.WindowFlags)
- RotateCropWidget.overrideWindowState()
QWidget.overrideWindowState(Qt.WindowStates)
- RotateCropWidget.paintEngine()
QWidget.paintEngine() -> QPaintEngine
- RotateCropWidget.paintEvent()
QWidget.paintEvent(QPaintEvent)
- RotateCropWidget.paintingActive()
QPaintDevice.paintingActive() -> bool
- RotateCropWidget.palette()
QWidget.palette() -> QPalette
- RotateCropWidget.paletteChange()
QWidget.paletteChange(QPalette)
- RotateCropWidget.parent()
QObject.parent() -> QObject
- RotateCropWidget.parentWidget()
QWidget.parentWidget() -> QWidget
- RotateCropWidget.physicalDpiX()
QPaintDevice.physicalDpiX() -> int
- RotateCropWidget.physicalDpiY()
QPaintDevice.physicalDpiY() -> int
- RotateCropWidget.pos()
QWidget.pos() -> QPoint
- RotateCropWidget.previousInFocusChain()
QWidget.previousInFocusChain() -> QWidget
- RotateCropWidget.property()
QObject.property(str) -> QVariant
- RotateCropWidget.pyqtConfigure()
QObject.pyqtConfigure(...)
Each keyword argument is either the name of a Qt property or a Qt signal. For properties the property is set to the given value which should be of an appropriate type. For signals the signal is connected to the given value which should be a callable.
- RotateCropWidget.raise_()
QWidget.raise_()
- RotateCropWidget.rect()
QWidget.rect() -> QRect
- RotateCropWidget.reject_changes()
Restore item original transform settings
- RotateCropWidget.releaseKeyboard()
QWidget.releaseKeyboard()
- RotateCropWidget.releaseMouse()
QWidget.releaseMouse()
- RotateCropWidget.releaseShortcut()
QWidget.releaseShortcut(int)
- RotateCropWidget.removeAction()
QWidget.removeAction(QAction)
- RotateCropWidget.removeEventFilter()
QObject.removeEventFilter(QObject)
- RotateCropWidget.render()
QWidget.render(QPaintDevice, QPoint targetOffset=QPoint(), QRegion sourceRegion=QRegion(), QWidget.RenderFlags flags=QWidget.DrawWindowBackground|QWidget.DrawChildren) QWidget.render(QPainter, QPoint targetOffset=QPoint(), QRegion sourceRegion=QRegion(), QWidget.RenderFlags flags=QWidget.DrawWindowBackground|QWidget.DrawChildren)
- RotateCropWidget.repaint()
QWidget.repaint() QWidget.repaint(int, int, int, int) QWidget.repaint(QRect) QWidget.repaint(QRegion)
- RotateCropWidget.reset()
Reset crop/transform image settings
- RotateCropWidget.resetInputContext()
QWidget.resetInputContext()
- RotateCropWidget.reset_transformation()
Reset transformation
- RotateCropWidget.resize()
QWidget.resize(QSize) QWidget.resize(int, int)
- RotateCropWidget.resizeEvent()
QWidget.resizeEvent(QResizeEvent)
- RotateCropWidget.restoreGeometry()
QWidget.restoreGeometry(QByteArray) -> bool
- RotateCropWidget.restore_original_state()
Restore item original state
- RotateCropWidget.saveGeometry()
QWidget.saveGeometry() -> QByteArray
- RotateCropWidget.scroll()
QWidget.scroll(int, int) QWidget.scroll(int, int, QRect)
- RotateCropWidget.setAcceptDrops()
QWidget.setAcceptDrops(bool)
- RotateCropWidget.setAttribute()
QWidget.setAttribute(Qt.WidgetAttribute, bool on=True)
- RotateCropWidget.setAutoFillBackground()
QWidget.setAutoFillBackground(bool)
- RotateCropWidget.setBackgroundRole()
QWidget.setBackgroundRole(QPalette.ColorRole)
- RotateCropWidget.setBaseSize()
QWidget.setBaseSize(int, int) QWidget.setBaseSize(QSize)
- RotateCropWidget.setContentsMargins()
QWidget.setContentsMargins(int, int, int, int) QWidget.setContentsMargins(QMargins)
- RotateCropWidget.setContextMenuPolicy()
QWidget.setContextMenuPolicy(Qt.ContextMenuPolicy)
- RotateCropWidget.setCursor()
QWidget.setCursor(QCursor)
- RotateCropWidget.setDisabled()
QWidget.setDisabled(bool)
- RotateCropWidget.setEnabled()
QWidget.setEnabled(bool)
- RotateCropWidget.setFixedHeight()
QWidget.setFixedHeight(int)
- RotateCropWidget.setFixedSize()
QWidget.setFixedSize(QSize) QWidget.setFixedSize(int, int)
- RotateCropWidget.setFixedWidth()
QWidget.setFixedWidth(int)
- RotateCropWidget.setFocus()
QWidget.setFocus() QWidget.setFocus(Qt.FocusReason)
- RotateCropWidget.setFocusPolicy()
QWidget.setFocusPolicy(Qt.FocusPolicy)
- RotateCropWidget.setFocusProxy()
QWidget.setFocusProxy(QWidget)
- RotateCropWidget.setFont()
QWidget.setFont(QFont)
- RotateCropWidget.setForegroundRole()
QWidget.setForegroundRole(QPalette.ColorRole)
- RotateCropWidget.setGeometry()
QWidget.setGeometry(QRect) QWidget.setGeometry(int, int, int, int)
- RotateCropWidget.setGraphicsEffect()
QWidget.setGraphicsEffect(QGraphicsEffect)
- RotateCropWidget.setHidden()
QWidget.setHidden(bool)
- RotateCropWidget.setInputContext()
QWidget.setInputContext(QInputContext)
- RotateCropWidget.setInputMethodHints()
QWidget.setInputMethodHints(Qt.InputMethodHints)
- RotateCropWidget.setLayout()
QWidget.setLayout(QLayout)
- RotateCropWidget.setLayoutDirection()
QWidget.setLayoutDirection(Qt.LayoutDirection)
- RotateCropWidget.setLocale()
QWidget.setLocale(QLocale)
- RotateCropWidget.setMask()
QWidget.setMask(QBitmap) QWidget.setMask(QRegion)
- RotateCropWidget.setMaximumHeight()
QWidget.setMaximumHeight(int)
- RotateCropWidget.setMaximumSize()
QWidget.setMaximumSize(int, int) QWidget.setMaximumSize(QSize)
- RotateCropWidget.setMaximumWidth()
QWidget.setMaximumWidth(int)
- RotateCropWidget.setMinimumHeight()
QWidget.setMinimumHeight(int)
- RotateCropWidget.setMinimumSize()
QWidget.setMinimumSize(int, int) QWidget.setMinimumSize(QSize)
- RotateCropWidget.setMinimumWidth()
QWidget.setMinimumWidth(int)
- RotateCropWidget.setMouseTracking()
QWidget.setMouseTracking(bool)
- RotateCropWidget.setObjectName()
QObject.setObjectName(QString)
- RotateCropWidget.setPalette()
QWidget.setPalette(QPalette)
- RotateCropWidget.setParent()
QWidget.setParent(QWidget) QWidget.setParent(QWidget, Qt.WindowFlags)
- RotateCropWidget.setProperty()
QObject.setProperty(str, QVariant) -> bool
- RotateCropWidget.setShortcutAutoRepeat()
QWidget.setShortcutAutoRepeat(int, bool enabled=True)
- RotateCropWidget.setShortcutEnabled()
QWidget.setShortcutEnabled(int, bool enabled=True)
- RotateCropWidget.setShown()
QWidget.setShown(bool)
- RotateCropWidget.setSizeIncrement()
QWidget.setSizeIncrement(int, int) QWidget.setSizeIncrement(QSize)
- RotateCropWidget.setSizePolicy()
QWidget.setSizePolicy(QSizePolicy) QWidget.setSizePolicy(QSizePolicy.Policy, QSizePolicy.Policy)
- RotateCropWidget.setStatusTip()
QWidget.setStatusTip(QString)
- RotateCropWidget.setStyle()
QWidget.setStyle(QStyle)
- RotateCropWidget.setStyleSheet()
QWidget.setStyleSheet(QString)
- RotateCropWidget.setTabOrder()
QWidget.setTabOrder(QWidget, QWidget)
- RotateCropWidget.setToolTip()
QWidget.setToolTip(QString)
- RotateCropWidget.setUpdatesEnabled()
QWidget.setUpdatesEnabled(bool)
- RotateCropWidget.setVisible()
QWidget.setVisible(bool)
- RotateCropWidget.setWhatsThis()
QWidget.setWhatsThis(QString)
- RotateCropWidget.setWindowFilePath()
QWidget.setWindowFilePath(QString)
- RotateCropWidget.setWindowFlags()
QWidget.setWindowFlags(Qt.WindowFlags)
- RotateCropWidget.setWindowIcon()
QWidget.setWindowIcon(QIcon)
- RotateCropWidget.setWindowIconText()
QWidget.setWindowIconText(QString)
- RotateCropWidget.setWindowModality()
QWidget.setWindowModality(Qt.WindowModality)
- RotateCropWidget.setWindowModified()
QWidget.setWindowModified(bool)
- RotateCropWidget.setWindowOpacity()
QWidget.setWindowOpacity(float)
- RotateCropWidget.setWindowRole()
QWidget.setWindowRole(QString)
- RotateCropWidget.setWindowState()
QWidget.setWindowState(Qt.WindowStates)
- RotateCropWidget.setWindowTitle()
QWidget.setWindowTitle(QString)
- RotateCropWidget.set_item(item)
Set associated item – must be a TrImageItem object
- RotateCropWidget.show()
QWidget.show()
- RotateCropWidget.showEvent()
QWidget.showEvent(QShowEvent)
- RotateCropWidget.showFullScreen()
QWidget.showFullScreen()
- RotateCropWidget.showMaximized()
QWidget.showMaximized()
- RotateCropWidget.showMinimized()
QWidget.showMinimized()
- RotateCropWidget.showNormal()
QWidget.showNormal()
- RotateCropWidget.show_crop_rect(state)
Show/hide cropping rectangle shape
- RotateCropWidget.signalsBlocked()
QObject.signalsBlocked() -> bool
- RotateCropWidget.size()
QWidget.size() -> QSize
- RotateCropWidget.sizeHint()
QWidget.sizeHint() -> QSize
- RotateCropWidget.sizeIncrement()
QWidget.sizeIncrement() -> QSize
- RotateCropWidget.sizePolicy()
QWidget.sizePolicy() -> QSizePolicy
- RotateCropWidget.stackUnder()
QWidget.stackUnder(QWidget)
- RotateCropWidget.startTimer()
QObject.startTimer(int) -> int
- RotateCropWidget.statusTip()
QWidget.statusTip() -> QString
- RotateCropWidget.style()
QWidget.style() -> QStyle
- RotateCropWidget.styleSheet()
QWidget.styleSheet() -> QString
- RotateCropWidget.tabletEvent()
QWidget.tabletEvent(QTabletEvent)
- RotateCropWidget.testAttribute()
QWidget.testAttribute(Qt.WidgetAttribute) -> bool
- RotateCropWidget.thread()
QObject.thread() -> QThread
- RotateCropWidget.toolTip()
QWidget.toolTip() -> QString
- RotateCropWidget.topLevelWidget()
QWidget.topLevelWidget() -> QWidget
- RotateCropWidget.tr()
QObject.tr(str, str disambiguation=None, int n=-1) -> QString
- RotateCropWidget.trUtf8()
QObject.trUtf8(str, str disambiguation=None, int n=-1) -> QString
- RotateCropWidget.underMouse()
QWidget.underMouse() -> bool
- RotateCropWidget.ungrabGesture()
QWidget.ungrabGesture(Qt.GestureType)
- RotateCropWidget.unsetCursor()
QWidget.unsetCursor()
- RotateCropWidget.unsetLayoutDirection()
QWidget.unsetLayoutDirection()
- RotateCropWidget.unsetLocale()
QWidget.unsetLocale()
- RotateCropWidget.unset_item()
Unset the associated item, freeing memory
- RotateCropWidget.update()
QWidget.update() QWidget.update(QRect) QWidget.update(QRegion) QWidget.update(int, int, int, int)
- RotateCropWidget.updateGeometry()
QWidget.updateGeometry()
- RotateCropWidget.updateMicroFocus()
QWidget.updateMicroFocus()
- RotateCropWidget.updatesEnabled()
QWidget.updatesEnabled() -> bool
- RotateCropWidget.visibleRegion()
QWidget.visibleRegion() -> QRegion
- RotateCropWidget.whatsThis()
QWidget.whatsThis() -> QString
- RotateCropWidget.wheelEvent()
QWidget.wheelEvent(QWheelEvent)
- RotateCropWidget.width()
QWidget.width() -> int
- RotateCropWidget.widthMM()
QPaintDevice.widthMM() -> int
- RotateCropWidget.winEvent()
QWidget.winEvent(MSG) -> (bool, int)
- RotateCropWidget.winId()
QWidget.winId() -> sip.voidptr
- RotateCropWidget.window()
QWidget.window() -> QWidget
- RotateCropWidget.windowActivationChange()
QWidget.windowActivationChange(bool)
- RotateCropWidget.windowFilePath()
QWidget.windowFilePath() -> QString
- RotateCropWidget.windowFlags()
QWidget.windowFlags() -> Qt.WindowFlags
- RotateCropWidget.windowIcon()
QWidget.windowIcon() -> QIcon
- RotateCropWidget.windowIconText()
QWidget.windowIconText() -> QString
- RotateCropWidget.windowModality()
QWidget.windowModality() -> Qt.WindowModality
- RotateCropWidget.windowOpacity()
QWidget.windowOpacity() -> float
- RotateCropWidget.windowRole()
QWidget.windowRole() -> QString
- RotateCropWidget.windowState()
QWidget.windowState() -> Qt.WindowStates
- RotateCropWidget.windowTitle()
QWidget.windowTitle() -> QString
- RotateCropWidget.windowType()
QWidget.windowType() -> Qt.WindowType
- RotateCropWidget.x()
QWidget.x() -> int
- RotateCropWidget.y()
QWidget.y() -> int