![]() |
WINC1500 IoT Software APIs
19.5.2
WINC Software API Reference Manual
|
Functions | |
NMI_API sint8 | m2m_wifi_request_scan (uint8 ch) |
NMI_API sint8 | m2m_wifi_request_scan_passive (uint8 ch, uint16 scan_time) |
NMI_API sint8 | m2m_wifi_request_scan_ssid_list (uint8 ch, uint8 *u8Ssidlist) |
Detailed Description
Asynchronous Wi-FI scan request on the given channel. The scan status is delivered in the wifi event callback and then the application is supposed to read the scan results sequentially. The number of APs found (N) is returned in event M2M_WIFI_RESP_SCAN_DONE with the number of found APs. The application reads the list of APs by calling the function m2m_wifi_req_scan_result N times.
Same as m2m_wifi_request_scan but perform passive scanning while the other one perform active scanning.
Asynchronous wi-fi scan request on the given channel and the hidden scan list. The scan status is delivered in the wi-fi event callback and then the application is to read the scan results sequentially. The number of APs found (N) is returned in event M2M_WIFI_RESP_SCAN_DONE with the number of found APs. The application could read the list of APs by calling the function m2m_wifi_req_scan_result N times.
Function Documentation
◆ m2m_wifi_request_scan()
- Parameters
-
[in] ch RF Channel ID for SCAN operation. It should be set according to tenuM2mScanCh. With a value of M2M_WIFI_CH_ALL(255)), means to scan all channels.
- Warning
- This function is not allowed in P2P or AP modes. It works only for STA mode (both connected or disconnected states).
- Precondition
- A Wi-Fi notification callback of type tpfAppWifiCb MUST be implemented and registered at initialization. Registering the callback is done through passing it to the m2m_wifi_init.
- The events M2M_WIFI_RESP_SCAN_DONE and M2M_WIFI_RESP_SCAN_RESULT. must be handled in the callback.
- The m2m_wifi_handle_events function MUST be called to receive the responses in the callback.
- See also
- M2M_WIFI_RESP_SCAN_DONE M2M_WIFI_RESP_SCAN_RESULT tpfAppWifiCb tstrM2mWifiscanResult tenuM2mScanCh m2m_wifi_init m2m_wifi_handle_events m2m_wifi_req_scan_result
- Returns
- The function returns M2M_SUCCESS for successful operations and a negative value otherwise.
Example
The code snippet demonstrates an example of how the scan request is called from the application's main function and the handling of the events received in response.
◆ m2m_wifi_request_scan_passive()
- Parameters
-
[in] ch RF Channel ID for SCAN operation. It should be set according to tenuM2mScanCh. With a value of M2M_WIFI_CH_ALL(255)), means to scan all channels. [in] scan_time The time in ms that passive scan is listening to beacons on each channel per one slot, enter 0 for deafult setting.
- Warning
- This function is not allowed in P2P or AP modes. It works only for STA mode (both connected or disconnected states).
- Precondition
- A Wi-Fi notification callback of type tpfAppWifiCb MUST be implemented and registered at initialization. Registering the callback is done through passing it to the m2m_wifi_init.
- The events M2M_WIFI_RESP_SCAN_DONE and M2M_WIFI_RESP_SCAN_RESULT. must be handled in the callback.
- The m2m_wifi_handle_events function MUST be called to receive the responses in the callback.
- See also
- m2m_wifi_request_scan M2M_WIFI_RESP_SCAN_DONE M2M_WIFI_RESP_SCAN_RESULT tpfAppWifiCb tstrM2mWifiscanResult tenuM2mScanCh m2m_wifi_init m2m_wifi_handle_events m2m_wifi_req_scan_result
- Returns
- The function returns M2M_SUCCESS for successful operations and a negative value otherwise.
◆ m2m_wifi_request_scan_ssid_list()
- Parameters
-
[in] ch RF Channel ID for SCAN operation. It should be set according to tenuM2mScanCh. With a value of M2M_WIFI_CH_ALL(255)), means to scan all channels. [in] u8SsidList u8SsidList is a buffer containing a list of hidden SSIDs to include during the scan. The first byte in the buffer, u8SsidList[0], is the number of SSIDs encoded in the string. The number of hidden SSIDs cannot exceed MAX_HIDDEN_SITES. All SSIDs are concatenated in the following bytes and each SSID is prefixed with a one-byte header containing its length. The total number of bytes in u8SsidList buffer, including length byte, cannot exceed 133 bytes (MAX_HIDDEN_SITES SSIDs x 32 bytes each, which is max SSID length). For instance, encoding the two hidden SSIDs "DEMO_AP" and "TEST" results in the following buffer content: uint8 u8SsidList[14];u8SsidList[0] = 2; // Number of SSIDs is 2u8SsidList[1] = 7; // Length of the string "DEMO_AP" without NULL terminationmemcpy(&u8SsidList[2], "DEMO_AP", 7); // Bytes index 2-9 containing the string DEMO_APu8SsidList[9] = 4; // Length of the string "TEST" without NULL terminationmemcpy(&u8SsidList[10], "TEST", 4); // Bytes index 10-13 containing the string TEST
- Warning
- This function is not allowed in P2P. It works only for STA/AP mode (connected or disconnected).
- Precondition
- A Wi-Fi notification callback of type tpfAppWifiCb MUST be implemented and registered at initialization. Registering the callback is done through passing it to the m2m_wifi_init.
- The events M2M_WIFI_RESP_SCAN_DONE and M2M_WIFI_RESP_SCAN_RESULT. must be handled in the callback.
- The m2m_wifi_handle_events function MUST be called to receive the responses in the callback.
- See also
- M2M_WIFI_RESP_SCAN_DONE M2M_WIFI_RESP_SCAN_RESULT tpfAppWifiCb tstrM2mWifiscanResult tenuM2mScanCh m2m_wifi_init m2m_wifi_handle_events m2m_wifi_req_scan_result
- Returns
- The function returns M2M_SUCCESS for successful operations and a negative value otherwise.
Example
The code snippet demonstrates an example of how the scan request is called from the application's main function and the handling of the events received in response.
Generated on Thu Jan 26 2017 22:15:21 for WINC1500 IoT Software APIs by
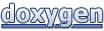