STM32L4R9I-Discovery BSP User Manual
|
stm32l4r9i_discovery_psram.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4r9i_discovery_psram.c 00004 * @author MCD Application Team 00005 * @brief This file includes the PSRAM driver for the IS61WV51216BLL-10MLI memory 00006 * device mounted on STM32L4R9I_DISCOVERY boards. 00007 @verbatim 00008 How To use this driver: 00009 ----------------------- 00010 - This driver is used to drive the IS66WVC2M16ECLL-7010BLI external memory mounted 00011 on STM32L4R9I discovery board. 00012 - This driver does not need a specific component driver for the PSRAM device 00013 to be included with. 00014 00015 Driver description: 00016 ------------------ 00017 + Initialization steps: 00018 o Initialize the PSRAM external memory using the BSP_PSRAM_Init() function. This 00019 function includes the MSP layer hardware resources initialization and the 00020 FMC controller configuration to interface with the external PSRAM memory. 00021 00022 + PSRAM read/write operations 00023 o PSRAM external memory can be accessed with read/write operations once it is 00024 initialized. 00025 Read/write operation can be performed with AHB access using the functions 00026 BSP_PSRAM_ReadData()/BSP_PSRAM_WriteData(), or by DMA transfer using the functions 00027 BSP_PSRAM_ReadData_DMA()/BSP_PSRAM_WriteData_DMA(). 00028 o The AHB access is performed with 16-bit width transaction, the DMA transfer 00029 configuration is fixed at single (no burst) halfword transfer. 00030 o User can implement his own functions for read/write access with his desired 00031 configurations. 00032 o If interrupt mode is used for DMA transfer, the function BSP_PSRAM_DMA_IRQHandler() 00033 is called in IRQ handler file, to serve the generated interrupt once the DMA 00034 transfer is complete. 00035 @endverbatim 00036 ****************************************************************************** 00037 * @attention 00038 * 00039 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00040 * 00041 * Redistribution and use in source and binary forms, with or without modification, 00042 * are permitted provided that the following conditions are met: 00043 * 1. Redistributions of source code must retain the above copyright notice, 00044 * this list of conditions and the following disclaimer. 00045 * 2. Redistributions in binary form must reproduce the above copyright notice, 00046 * this list of conditions and the following disclaimer in the documentation 00047 * and/or other materials provided with the distribution. 00048 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00049 * may be used to endorse or promote products derived from this software 00050 * without specific prior written permission. 00051 * 00052 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00053 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00054 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00055 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00056 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00057 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00058 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00059 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00060 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00061 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00062 * 00063 ****************************************************************************** 00064 */ 00065 00066 /* Includes ------------------------------------------------------------------*/ 00067 #include "stm32l4r9i_discovery_psram.h" 00068 #include "stm32l4r9i_discovery_io.h" 00069 00070 /** @addtogroup BSP 00071 * @{ 00072 */ 00073 00074 /** @addtogroup STM32L4R9I_DISCOVERY 00075 * @{ 00076 */ 00077 00078 /** @defgroup STM32L4R9I_DISCOVERY_PSRAM STM32L4R9I_DISCOVERY PSRAM 00079 * @{ 00080 */ 00081 00082 /** @defgroup STM32L4R9I_DISCOVERY_PSRAM_Private_Variables Exported Variables 00083 * @{ 00084 */ 00085 SRAM_HandleTypeDef psramHandle = {0}; 00086 00087 /* LCD/PSRAM initialization status sharing the same power source */ 00088 extern uint32_t bsp_lcd_initialized; 00089 extern uint32_t bsp_psram_initialized; 00090 00091 /** 00092 * @} 00093 */ 00094 00095 /** @defgroup STM32L4R9I_DISCOVERY_PSRAM_Private_Function_Prototypes Private Function Prototypes 00096 * @{ 00097 */ 00098 static void PSRAM_PowerOn(void); 00099 static void PSRAM_PowerOff(void); 00100 /** 00101 * @} 00102 */ 00103 00104 /** @defgroup STM32L4R9I_DISCOVERY_PSRAM_Private_Functions Private Functions 00105 * @{ 00106 */ 00107 00108 /** 00109 * @brief Initializes the PSRAM device. 00110 * @retval PSRAM status 00111 */ 00112 uint8_t BSP_PSRAM_Init(void) 00113 { 00114 static uint8_t psram_status = PSRAM_OK; 00115 00116 if (bsp_psram_initialized == 0) 00117 { 00118 static FMC_NORSRAM_TimingTypeDef Timing; 00119 00120 /* Power on PSRAM */ 00121 PSRAM_PowerOn(); 00122 00123 /* PSRAM device configuration */ 00124 /* Timing configuration derived from system clock (up to 120Mhz) 00125 for 60Mhz as PSRAM clock frequency */ 00126 Timing.AddressSetupTime = 4; 00127 Timing.AddressHoldTime = 2; 00128 Timing.DataSetupTime = 6; 00129 Timing.BusTurnAroundDuration = 1; 00130 Timing.CLKDivision = 2; 00131 Timing.DataLatency = 2; 00132 Timing.AccessMode = FMC_ACCESS_MODE_A; 00133 00134 psramHandle.Init.NSBank = FMC_NORSRAM_BANK1; 00135 psramHandle.Init.DataAddressMux = FMC_DATA_ADDRESS_MUX_DISABLE; 00136 psramHandle.Init.MemoryType = FMC_MEMORY_TYPE_PSRAM; 00137 psramHandle.Init.MemoryDataWidth = FMC_NORSRAM_MEM_BUS_WIDTH_16; 00138 psramHandle.Init.BurstAccessMode = FMC_BURST_ACCESS_MODE_DISABLE; 00139 psramHandle.Init.WaitSignalPolarity = FMC_WAIT_SIGNAL_POLARITY_HIGH; 00140 psramHandle.Init.WaitSignalActive = FMC_WAIT_TIMING_BEFORE_WS; 00141 psramHandle.Init.WriteOperation = FMC_WRITE_OPERATION_ENABLE; 00142 psramHandle.Init.WaitSignal = FMC_WAIT_SIGNAL_DISABLE; 00143 psramHandle.Init.ExtendedMode = FMC_EXTENDED_MODE_DISABLE; 00144 psramHandle.Init.AsynchronousWait = FMC_ASYNCHRONOUS_WAIT_DISABLE; 00145 psramHandle.Init.WriteBurst = FMC_WRITE_BURST_DISABLE; 00146 psramHandle.Init.ContinuousClock = FMC_CONTINUOUS_CLOCK_SYNC_ASYNC; 00147 psramHandle.Init.WriteFifo = FMC_WRITE_FIFO_DISABLE; 00148 psramHandle.Init.NBLSetupTime = 0; 00149 psramHandle.Init.PageSize = FMC_PAGE_SIZE_NONE; 00150 00151 psramHandle.Instance = FMC_NORSRAM_DEVICE; 00152 psramHandle.Extended = FMC_NORSRAM_EXTENDED_DEVICE; 00153 00154 /* PSRAM controller initialization */ 00155 BSP_PSRAM_MspInit(&psramHandle, NULL); /* __weak function can be rewritten by the application */ 00156 if(HAL_SRAM_Init(&psramHandle, &Timing, &Timing) != HAL_OK) 00157 { 00158 psram_status = PSRAM_ERROR; 00159 } 00160 else 00161 { 00162 psram_status = PSRAM_OK; 00163 } 00164 00165 bsp_psram_initialized = 1; 00166 } 00167 00168 return psram_status; 00169 } 00170 00171 /** 00172 * @brief DeInitializes the PSRAM device. 00173 * @retval PSRAM status 00174 */ 00175 uint8_t BSP_PSRAM_DeInit(void) 00176 { 00177 static uint8_t psram_status = PSRAM_OK; 00178 00179 if (bsp_psram_initialized == 1) 00180 { 00181 /* PSRAM device de-initialization */ 00182 psramHandle.Instance = FMC_NORSRAM_DEVICE; 00183 psramHandle.Extended = FMC_NORSRAM_EXTENDED_DEVICE; 00184 00185 if(HAL_SRAM_DeInit(&psramHandle) != HAL_OK) 00186 { 00187 psram_status = PSRAM_ERROR; 00188 } 00189 else 00190 { 00191 psram_status = PSRAM_OK; 00192 } 00193 00194 /* PSRAM controller de-initialization */ 00195 BSP_PSRAM_MspDeInit(&psramHandle, NULL); 00196 00197 /* Power off PSRAM */ 00198 PSRAM_PowerOff(); 00199 00200 bsp_psram_initialized = 0; 00201 } 00202 00203 return psram_status; 00204 } 00205 00206 /** 00207 * @brief Reads an amount of data from the PSRAM device in polling mode. 00208 * @param uwStartAddress: Read start address 00209 * @param pData: Pointer to data to be read 00210 * @param uwDataSize: Size of read data from the memory 00211 * @retval PSRAM status 00212 */ 00213 uint8_t BSP_PSRAM_ReadData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00214 { 00215 if(HAL_SRAM_Read_16b(&psramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00216 { 00217 return PSRAM_ERROR; 00218 } 00219 else 00220 { 00221 return PSRAM_OK; 00222 } 00223 } 00224 00225 /** 00226 * @brief Reads an amount of data from the PSRAM device in DMA mode. 00227 * @param uwStartAddress: Read start address 00228 * @param pData: Pointer to data to be read 00229 * @param uwDataSize: Size of read data from the memory 00230 * @retval PSRAM status 00231 */ 00232 uint8_t BSP_PSRAM_ReadData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00233 { 00234 if(HAL_SRAM_Read_DMA(&psramHandle, (uint32_t *)uwStartAddress, (uint32_t *)pData, uwDataSize) != HAL_OK) 00235 { 00236 return PSRAM_ERROR; 00237 } 00238 else 00239 { 00240 return PSRAM_OK; 00241 } 00242 } 00243 00244 /** 00245 * @brief Writes an amount of data from the PSRAM device in polling mode. 00246 * @param uwStartAddress: Write start address 00247 * @param pData: Pointer to data to be written 00248 * @param uwDataSize: Size of written data from the memory 00249 * @retval PSRAM status 00250 */ 00251 uint8_t BSP_PSRAM_WriteData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00252 { 00253 if(HAL_SRAM_Write_16b(&psramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00254 { 00255 return PSRAM_ERROR; 00256 } 00257 else 00258 { 00259 return PSRAM_OK; 00260 } 00261 } 00262 00263 /** 00264 * @brief Writes an amount of data from the PSRAM device in DMA mode. 00265 * @param uwStartAddress: Write start address 00266 * @param pData: Pointer to data to be written 00267 * @param uwDataSize: Size of written data from the memory 00268 * @retval PSRAM status 00269 */ 00270 uint8_t BSP_PSRAM_WriteData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00271 { 00272 if(HAL_SRAM_Write_DMA(&psramHandle, (uint32_t *)uwStartAddress, (uint32_t *)pData, uwDataSize) != HAL_OK) 00273 { 00274 return PSRAM_ERROR; 00275 } 00276 else 00277 { 00278 return PSRAM_OK; 00279 } 00280 } 00281 00282 /** 00283 * @brief Initializes PSRAM MSP. 00284 * @param hsram: PSRAM handle 00285 * @param Params 00286 * @retval None 00287 */ 00288 __weak void BSP_PSRAM_MspInit(SRAM_HandleTypeDef *hsram, void *Params) 00289 { 00290 static DMA_HandleTypeDef dma_handle; 00291 GPIO_InitTypeDef GPIO_Init_Structure; 00292 00293 /* Enable DMAx clock */ 00294 __PSRAM_DMAx_CLK_ENABLE(); 00295 __HAL_RCC_DMAMUX1_CLK_ENABLE(); 00296 00297 /* Enable FMC clock */ 00298 __HAL_RCC_FMC_CLK_ENABLE(); 00299 00300 /* Enable GPIOs clock */ 00301 __HAL_RCC_GPIOB_CLK_ENABLE(); 00302 __HAL_RCC_GPIOD_CLK_ENABLE(); 00303 __HAL_RCC_GPIOE_CLK_ENABLE(); 00304 __HAL_RCC_GPIOF_CLK_ENABLE(); 00305 __HAL_RCC_GPIOG_CLK_ENABLE(); 00306 /* IOSV bit MUST be set to access GPIO port G[2:15] */ 00307 __HAL_RCC_PWR_CLK_ENABLE(); 00308 SET_BIT(PWR->CR2, PWR_CR2_IOSV); 00309 00310 /* Common GPIO configuration */ 00311 GPIO_Init_Structure.Mode = GPIO_MODE_AF_PP; 00312 GPIO_Init_Structure.Pull = GPIO_NOPULL; 00313 GPIO_Init_Structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00314 GPIO_Init_Structure.Alternate = GPIO_AF12_FMC; 00315 00316 /*## Data Bus #######*/ 00317 /* GPIOD configuration */ 00318 GPIO_Init_Structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_8 | GPIO_PIN_9 | 00319 GPIO_PIN_10 | GPIO_PIN_14 | GPIO_PIN_15; 00320 HAL_GPIO_Init(GPIOD, &GPIO_Init_Structure); 00321 00322 /* GPIOE configuration */ 00323 GPIO_Init_Structure.Pin = GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | 00324 GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | 00325 GPIO_PIN_14 | GPIO_PIN_15; 00326 HAL_GPIO_Init(GPIOE, &GPIO_Init_Structure); 00327 00328 00329 /*## Address Bus #######*/ 00330 /* GPIOF configuration */ 00331 GPIO_Init_Structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | 00332 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_12 | GPIO_PIN_13 | 00333 GPIO_PIN_14 | GPIO_PIN_15; 00334 HAL_GPIO_Init(GPIOF, &GPIO_Init_Structure); 00335 00336 00337 /* GPIOG configuration */ 00338 GPIO_Init_Structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | 00339 GPIO_PIN_3 | GPIO_PIN_4 | GPIO_PIN_5; 00340 HAL_GPIO_Init(GPIOG, &GPIO_Init_Structure); 00341 00342 /* GPIOD configuration */ 00343 GPIO_Init_Structure.Pin = GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13; 00344 HAL_GPIO_Init(GPIOD, &GPIO_Init_Structure); 00345 00346 /* GPIOE configuration */ 00347 GPIO_Init_Structure.Pin = GPIO_PIN_3 | GPIO_PIN_4; 00348 HAL_GPIO_Init(GPIOE, &GPIO_Init_Structure); 00349 GPIO_Init_Structure.Pull = GPIO_PULLUP; 00350 00351 00352 /*## NOE and NWE configuration #######*/ 00353 GPIO_Init_Structure.Pin = GPIO_PIN_4 |GPIO_PIN_5; 00354 HAL_GPIO_Init(GPIOD, &GPIO_Init_Structure); 00355 00356 /* Chip select configuration */ 00357 /*## NE1 configuration #######*/ 00358 GPIO_Init_Structure.Pin = GPIO_PIN_7; 00359 HAL_GPIO_Init(GPIOD, &GPIO_Init_Structure); 00360 00361 /*## NE2, NE3, NE4 configuration #######*/ 00362 GPIO_Init_Structure.Pin = GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_12; 00363 HAL_GPIO_Init(GPIOG, &GPIO_Init_Structure); 00364 00365 /*## NBL0, NBL1 configuration #######*/ 00366 GPIO_Init_Structure.Pin = GPIO_PIN_0 | GPIO_PIN_1; 00367 GPIO_Init_Structure.Pull = GPIO_PULLUP; 00368 HAL_GPIO_Init(GPIOE, &GPIO_Init_Structure); 00369 00370 00371 GPIO_Init_Structure.Pull = GPIO_PULLDOWN; 00372 /*## CLK and NWAIT configuration #######*/ 00373 GPIO_Init_Structure.Pin = GPIO_PIN_3 | GPIO_PIN_6; 00374 HAL_GPIO_Init(GPIOD, &GPIO_Init_Structure); 00375 00376 /*## ADVn configuration #######*/ 00377 GPIO_Init_Structure.Pin = GPIO_PIN_7; 00378 HAL_GPIO_Init(GPIOB, &GPIO_Init_Structure); 00379 00380 /* Configure common DMA parameters */ 00381 dma_handle.Init.Direction = DMA_MEMORY_TO_MEMORY; 00382 dma_handle.Init.PeriphInc = DMA_PINC_ENABLE; 00383 dma_handle.Init.MemInc = DMA_MINC_ENABLE; 00384 dma_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_HALFWORD; 00385 dma_handle.Init.MemDataAlignment = DMA_MDATAALIGN_HALFWORD; 00386 dma_handle.Init.Mode = DMA_NORMAL; 00387 dma_handle.Init.Priority = DMA_PRIORITY_HIGH; 00388 00389 dma_handle.Instance = PSRAM_DMAx_INSTANCE; 00390 00391 /* Deinitialize the Channel for new transfer */ 00392 HAL_DMA_DeInit(&dma_handle); 00393 00394 /* Configure the DMA Channel */ 00395 HAL_DMA_Init(&dma_handle); 00396 00397 /* Associate the DMA handle to the FMC SRAM one */ 00398 hsram->hdma = &dma_handle; 00399 00400 HAL_NVIC_SetPriorityGrouping(NVIC_PRIORITYGROUP_0); 00401 00402 /* NVIC configuration for DMA transfer complete interrupt */ 00403 HAL_NVIC_SetPriority(PSRAM_DMAx_IRQn, 0, 0); 00404 HAL_NVIC_EnableIRQ(PSRAM_DMAx_IRQn); 00405 } 00406 00407 00408 /** 00409 * @brief DeInitializes SRAM MSP. 00410 * @param hsram: SRAM handle 00411 * @param Params 00412 * @retval None 00413 */ 00414 __weak void BSP_PSRAM_MspDeInit(SRAM_HandleTypeDef *hsram, void *Params) 00415 { 00416 static DMA_HandleTypeDef dma_handle; 00417 00418 /* Disable NVIC configuration for DMA interrupt */ 00419 HAL_NVIC_DisableIRQ(PSRAM_DMAx_IRQn); 00420 00421 /* Deinitialize the stream for new transfer */ 00422 dma_handle.Instance = PSRAM_DMAx_INSTANCE; 00423 HAL_DMA_DeInit(&dma_handle); 00424 00425 /* Deinitialize GPIOs */ 00426 HAL_GPIO_DeInit(GPIOB, GPIO_PIN_7); 00427 HAL_GPIO_DeInit(GPIOD, GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_3 | GPIO_PIN_4 | 00428 GPIO_PIN_5 | GPIO_PIN_6 | GPIO_PIN_7 | GPIO_PIN_8 | 00429 GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 | 00430 GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15); 00431 HAL_GPIO_DeInit(GPIOE, GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_3 | GPIO_PIN_4 | 00432 GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | 00433 GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | 00434 GPIO_PIN_15); 00435 HAL_GPIO_DeInit(GPIOF, GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | 00436 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_12 | GPIO_PIN_13 | 00437 GPIO_PIN_14 | GPIO_PIN_15); 00438 HAL_GPIO_DeInit(GPIOG, GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | 00439 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_9 | GPIO_PIN_10 | 00440 GPIO_PIN_12); 00441 00442 /* GPIO pins clock, FMC clock and DMA clock can be shut down in the applications 00443 by surcharging this __weak function */ 00444 } 00445 00446 /** 00447 * @brief PSRAM power on 00448 * Power on PSRAM. 00449 */ 00450 static void PSRAM_PowerOn(void) 00451 { 00452 /* Configure DSI_RESET and DSI_POWER_ON only if lcd is not currently used */ 00453 if(bsp_lcd_initialized == 0) 00454 { 00455 BSP_IO_Init(); 00456 00457 #if defined(USE_STM32L4R9I_DISCO_REVA) || defined(USE_STM32L4R9I_DISCO_REVB) 00458 /* Set DSI_POWER_ON to input floating to avoid I2C issue during input PD configuration */ 00459 BSP_IO_ConfigPin(IO_PIN_8, IO_MODE_INPUT); 00460 00461 /* Configure the GPIO connected to DSI_RESET signal */ 00462 BSP_IO_ConfigPin(IO_PIN_10, IO_MODE_OUTPUT); 00463 00464 /* Activate DSI_RESET (active low) */ 00465 BSP_IO_WritePin(IO_PIN_10, GPIO_PIN_RESET); 00466 00467 /* Configure the GPIO connected to DSI_POWER_ON signal as input pull down */ 00468 /* to activate 3V3_LCD. VDD_LCD is also activated if VDD = 3,3V */ 00469 BSP_IO_ConfigPin(IO_PIN_8, IO_MODE_INPUT_PD); 00470 00471 /* Wait at least 1ms before enabling 1V8_LCD */ 00472 HAL_Delay(1); 00473 00474 /* Configure the GPIO connected to DSI_POWER_ON signal as output low */ 00475 /* to activate 1V8_LCD. VDD_LCD is also activated if VDD = 1,8V */ 00476 BSP_IO_WritePin(IO_PIN_8, GPIO_PIN_RESET); 00477 BSP_IO_ConfigPin(IO_PIN_8, IO_MODE_OUTPUT); 00478 #else /* USE_STM32L4R9I_DISCO_REVA || USE_STM32L4R9I_DISCO_REVB */ 00479 /* Configure the GPIO connected to DSI_3V3_POWERON signal as output low */ 00480 /* to activate 3V3_LCD. VDD_LCD is also activated if VDD = 3,3V */ 00481 BSP_IO_WritePin(IO_PIN_8, GPIO_PIN_RESET); 00482 BSP_IO_ConfigPin(IO_PIN_8, IO_MODE_OUTPUT); 00483 00484 /* Wait at least 1ms before enabling 1V8_LCD */ 00485 HAL_Delay(1); 00486 00487 /* Configure the GPIO connected to DSI_1V8_POWERON signal as output low */ 00488 /* to activate 1V8_LCD. VDD_LCD is also activated if VDD = 1,8V */ 00489 BSP_IO_WritePin(AGPIO_PIN_2, GPIO_PIN_RESET); 00490 BSP_IO_ConfigPin(AGPIO_PIN_2, IO_MODE_OUTPUT); 00491 #endif /* USE_STM32L4R9I_DISCO_REVA || USE_STM32L4R9I_DISCO_REVB */ 00492 00493 /* Wait at least 15 ms (minimum reset low width is 10ms and add margin for 1V8_LCD ramp-up) */ 00494 HAL_Delay(15); 00495 } 00496 } 00497 00498 /** 00499 * @brief PSRAM power off 00500 * Power off PSRAM. 00501 */ 00502 static void PSRAM_PowerOff(void) 00503 { 00504 /* Set DSI_POWER_ON to analog mode only if lcd is not currently used */ 00505 if(bsp_lcd_initialized == 0) 00506 { 00507 #if defined(USE_STM32L4R9I_DISCO_REVA) || defined(USE_STM32L4R9I_DISCO_REVB) 00508 BSP_IO_ConfigPin(IO_PIN_8, IO_MODE_ANALOG); 00509 #else /* USE_STM32L4R9I_DISCO_REVA || USE_STM32L4R9I_DISCO_REVB */ 00510 /* Disable first DSI_1V8_PWRON then DSI_3V3_PWRON */ 00511 BSP_IO_ConfigPin(AGPIO_PIN_2, IO_MODE_ANALOG); 00512 BSP_IO_ConfigPin(IO_PIN_8, IO_MODE_ANALOG); 00513 #endif /* USE_STM32L4R9I_DISCO_REVA || USE_STM32L4R9I_DISCO_REVB */ 00514 } 00515 } 00516 00517 /** 00518 * @} 00519 */ 00520 00521 /** 00522 * @} 00523 */ 00524 00525 /** 00526 * @} 00527 */ 00528 00529 /** 00530 * @} 00531 */ 00532 00533 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Oct 13 2017 02:37:42 for STM32L4R9I-Discovery BSP User Manual by
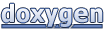