STM32L4R9I-Discovery BSP User Manual
|
stm32l4r9i_discovery_sd.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4r9i_discovery_sd.h 00004 * @author MCD Application Team 00005 * @brief This file contains the common defines and functions prototypes for 00006 * the stm32l4r9i_discovery_sd.c driver. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Define to prevent recursive inclusion -------------------------------------*/ 00038 #ifndef __STM32L4R9I_DISCOVERY_SD_H 00039 #define __STM32L4R9I_DISCOVERY_SD_H 00040 00041 #ifdef __cplusplus 00042 extern "C" { 00043 #endif 00044 00045 /* Includes ------------------------------------------------------------------*/ 00046 #include "stm32l4xx_hal.h" 00047 00048 /** @addtogroup BSP 00049 * @{ 00050 */ 00051 00052 /** @addtogroup STM32L4R9I_DISCOVERY 00053 * @{ 00054 */ 00055 00056 /** @addtogroup STM32L4R9I_DISCOVERY_SD 00057 * @{ 00058 */ 00059 00060 /* Exported types ------------------------------------------------------------*/ 00061 00062 /** @defgroup STM32L4R9I_DISCOVERY_SD_Exported_Types Exported Types 00063 * @{ 00064 */ 00065 00066 /** 00067 * @brief SD Card information structure 00068 */ 00069 #define BSP_SD_CardInfo HAL_SD_CardInfoTypeDef 00070 /** 00071 * @} 00072 */ 00073 00074 /** 00075 * @brief SD status structure definition 00076 */ 00077 #define MSD_OK ((uint8_t)0x00) 00078 #define MSD_ERROR ((uint8_t)0x01) 00079 #define MSD_ERROR_SD_NOT_PRESENT ((uint8_t)0x02) 00080 00081 /** 00082 * @brief SD transfer state definition 00083 */ 00084 #define SD_TRANSFER_OK ((uint8_t)0x00) 00085 #define SD_TRANSFER_BUSY ((uint8_t)0x01) 00086 #define SD_TRANSFER_ERROR ((uint8_t)0x02) 00087 00088 /* Exported constants --------------------------------------------------------*/ 00089 00090 /** @defgroup STM32L4R9I_DISCOVERY_SD_Exported_Constants Exported Constants 00091 * @{ 00092 */ 00093 #define SD_DATATIMEOUT ((uint32_t)100000000) 00094 00095 #define SD_PRESENT ((uint8_t)0x01) 00096 #define SD_NOT_PRESENT ((uint8_t)0x00) 00097 00098 /* SD IRQ handler */ 00099 #define SDMMCx_IRQHandler SDMMC1_IRQHandler 00100 #define SDMMCx_IRQn SDMMC1_IRQn 00101 00102 /** 00103 * @} 00104 */ 00105 00106 /* Exported variables --------------------------------------------------------*/ 00107 00108 /** @defgroup STM32L4R9I_DISCOVERY_SD_Exported_Variables Exported Variables 00109 * @{ 00110 */ 00111 extern SD_HandleTypeDef hsd_discovery; 00112 00113 /** 00114 * @} 00115 */ 00116 00117 /* Exported functions --------------------------------------------------------*/ 00118 00119 /** @defgroup STM32L4R9I_DISCOVERY_SD_Exported_Functions Exported Functions 00120 * @{ 00121 */ 00122 uint8_t BSP_SD_Init(void); 00123 uint8_t BSP_SD_DeInit(void); 00124 uint8_t BSP_SD_ITConfig(void); 00125 uint8_t BSP_SD_ReadBlocks(uint32_t *pData, uint32_t ReadAddr, uint32_t NumOfBlocks, uint32_t Timeout); 00126 uint8_t BSP_SD_WriteBlocks(uint32_t *pData, uint32_t WriteAddr, uint32_t NumOfBlocks, uint32_t Timeout); 00127 uint8_t BSP_SD_ReadBlocks_DMA(uint32_t *pData, uint32_t ReadAddr, uint32_t NumOfBlocks); 00128 uint8_t BSP_SD_WriteBlocks_DMA(uint32_t *pData, uint32_t WriteAddr, uint32_t NumOfBlocks); 00129 uint8_t BSP_SD_Erase(uint32_t StartAddr, uint32_t EndAddr); 00130 uint8_t BSP_SD_GetCardState(void); 00131 void BSP_SD_GetCardInfo(BSP_SD_CardInfo *CardInfo); 00132 uint8_t BSP_SD_IsDetected(void); 00133 00134 /* These __weak functions can be surcharged by application code in case the current settings 00135 (eg. interrupt priority, callbacks implementation) need to be changed for specific application needs */ 00136 void BSP_SD_MspInit(SD_HandleTypeDef *hsd, void *Params); 00137 void BSP_SD_MspDeInit(SD_HandleTypeDef *hsd, void *Params); 00138 void BSP_SD_AbortCallback(void); 00139 void BSP_SD_WriteCpltCallback(void); 00140 void BSP_SD_ReadCpltCallback(void); 00141 00142 /** 00143 * @} 00144 */ 00145 00146 /** 00147 * @} 00148 */ 00149 00150 /** 00151 * @} 00152 */ 00153 00154 /** 00155 * @} 00156 */ 00157 00158 #ifdef __cplusplus 00159 } 00160 #endif 00161 00162 #endif /* __STM32L4R9I_DISCOVERY_SD_H */ 00163 00164 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Oct 13 2017 02:37:42 for STM32L4R9I-Discovery BSP User Manual by
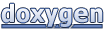