STM32L4R9I-Discovery BSP User Manual
|
stm32l4r9i_discovery_io.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4r9i_discovery_io.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of functions needed to manage the IO pins 00006 * on STM32L4R9I_DISCOVERY discovery board. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* File Info : ----------------------------------------------------------------- 00038 User NOTES 00039 1. How To use this driver: 00040 -------------------------- 00041 - This driver is used to drive the IO module of the STM32L4R9I_DISCOVERY 00042 board. 00043 - The MFXSTM32L152 IO expander device component driver must be included with this 00044 driver in order to run the IO functionalities commanded by the IO expander 00045 device mounted on the discovery board. 00046 00047 2. Driver description: 00048 --------------------- 00049 + Initialization steps: 00050 o Initialize the IO module using the BSP_IO_Init() function. This 00051 function includes the MSP layer hardware resources initialization and the 00052 communication layer configuration to start the IO functionalities use. 00053 00054 + IO functionalities use 00055 o The IO pin mode is configured when calling the function BSP_IO_ConfigPin(), you 00056 must specify the desired IO mode by choosing the "IO_ModeTypedef" parameter 00057 predefined value. 00058 o If an IO pin is used in interrupt mode, the function BSP_IO_ITGetStatus() is 00059 needed to get the interrupt status. To clear the IT pending bits, you should 00060 call the function BSP_IO_ITClear() with specifying the IO pending bit to clear. 00061 o The IT is handled using the corresponding external interrupt IRQ handler, 00062 the user IT callback treatment is implemented on the same external interrupt 00063 callback. 00064 o To get/set an IO pin combination state you can use the functions 00065 BSP_IO_ReadPin()/BSP_IO_WritePin() or the function BSP_IO_TogglePin() to toggle the pin 00066 state. 00067 00068 ------------------------------------------------------------------------------*/ 00069 00070 /* Includes ------------------------------------------------------------------*/ 00071 #include "stm32l4r9i_discovery_io.h" 00072 00073 /** @addtogroup BSP 00074 * @{ 00075 */ 00076 00077 /** @addtogroup STM32L4R9I_DISCOVERY 00078 * @{ 00079 */ 00080 00081 /** @defgroup STM32L4R9I_DISCOVERY_IO STM32L4R9I_DISCOVERY IO 00082 * @{ 00083 */ 00084 00085 /* Private variables ---------------------------------------------------------*/ 00086 00087 /** @defgroup STM32L4R9I_DISCOVERY_IO_Private_Variables Private Variables 00088 * @{ 00089 */ 00090 static IO_DrvTypeDef *io_driver; 00091 00092 /** 00093 * @} 00094 */ 00095 00096 /* Exported functions --------------------------------------------------------*/ 00097 00098 /** @addtogroup STM32L4R9I_DISCOVERY_IO_Exported_Functions 00099 * @{ 00100 */ 00101 00102 /** 00103 * @brief Initialize and configure the IO functionalities and configures all 00104 * necessary hardware resources (GPIOs, clocks..). 00105 * @note BSP_IO_Init() is using HAL_Delay() function to ensure that stmpe811 00106 * IO Expander is correctly reset. HAL_Delay() function provides accurate 00107 * delay (in milliseconds) based on variable incremented in SysTick ISR. 00108 * This implies that if BSP_IO_Init() is called from a peripheral ISR process, 00109 * then the SysTick interrupt must have higher priority (numerically lower) 00110 * than the peripheral interrupt. Otherwise the caller ISR process will be blocked. 00111 * @retval IO_OK: if all initializations are OK. Other value if error. 00112 */ 00113 uint8_t BSP_IO_Init(void) 00114 { 00115 uint8_t ret = IO_OK; 00116 uint8_t mfxstm32l152_id = 0; 00117 00118 if (io_driver == NULL) /* Checks if MFX initialization has been already done */ 00119 { 00120 mfxstm32l152_idd_drv.WakeUp(IO_I2C_ADDRESS); 00121 00122 HAL_Delay(10); 00123 00124 /* Read ID and verify the IO expander is ready */ 00125 mfxstm32l152_id = mfxstm32l152_io_drv.ReadID(IO_I2C_ADDRESS); 00126 00127 if((mfxstm32l152_id == MFXSTM32L152_ID_1) || (mfxstm32l152_id == MFXSTM32L152_ID_2)) 00128 { 00129 /* Initialize the MFX */ 00130 io_driver = &mfxstm32l152_io_drv; 00131 00132 /* Initialize the MFX IO driver structure */ 00133 if(io_driver->Init != NULL) 00134 { 00135 io_driver->Init(IO_I2C_ADDRESS); 00136 io_driver->Start(IO_I2C_ADDRESS, IO_PIN_ALL); 00137 } 00138 else 00139 { 00140 ret = IO_ERROR; 00141 } 00142 } 00143 else 00144 { 00145 ret = IO_ERROR; 00146 } 00147 } 00148 else 00149 { 00150 /* MFX initialization already done : do nothing */ 00151 } 00152 00153 return ret; 00154 } 00155 00156 /** 00157 * @brief DeInitialize the IO to allow Mfx Initialization to be executed again 00158 * @note BSP_IO_Init() has no effect if the io_driver is already initialized 00159 * BSP_IO_DeInit() allows to erase the pointer such to allow init to be effective 00160 * @retval IO_OK 00161 */ 00162 uint8_t BSP_IO_DeInit(void) 00163 { 00164 io_driver = NULL; 00165 return IO_OK; 00166 } 00167 00168 /** 00169 * @brief Get the selected pins IT status. 00170 * @param IO_Pin: Selected pin(s) to check the status. 00171 * This parameter can be any combination of the IO pins. 00172 * @retval Status of the checked IO pin(s). 00173 */ 00174 uint32_t BSP_IO_ITGetStatus(uint32_t IO_Pin) 00175 { 00176 /* Return the IO Pin IT status */ 00177 return (io_driver->ITStatus(IO_I2C_ADDRESS, IO_Pin)); 00178 } 00179 00180 /** 00181 * @brief Clear the selected IO IT pending bit. 00182 * @param IO_Pin: Selected pin(s) to clear the status. 00183 * This parameter can be any combination of the IO pins. 00184 * @retval None 00185 */ 00186 void BSP_IO_ITClear(uint32_t IO_Pin) 00187 { 00188 /* Clear the selected IO IT pending bits */ 00189 io_driver->ClearIT(IO_I2C_ADDRESS, IO_Pin); 00190 } 00191 00192 /** 00193 * @brief Configure the IO pin(s) according to IO mode structure value. 00194 * @param IO_Pin: Output IO pin(s) to be set or reset. 00195 * This parameter can be any combination of the IO pin(s). 00196 * @param IO_Mode: IO pin mode to configure 00197 * This parameter can be one of the following values: 00198 * @arg IO_MODE_INPUT 00199 * @arg IO_MODE_OUTPUT 00200 * @arg IO_MODE_IT_RISING_EDGE 00201 * @arg IO_MODE_IT_FALLING_EDGE 00202 * @arg IO_MODE_IT_LOW_LEVEL 00203 * @arg IO_MODE_IT_HIGH_LEVEL 00204 * @retval IO_OK: if all initializations are OK. Other value if error. 00205 */ 00206 uint8_t BSP_IO_ConfigPin(uint32_t IO_Pin, IO_ModeTypedef IO_Mode) 00207 { 00208 /* Configure the selected IO pin(s) mode */ 00209 io_driver->Config(IO_I2C_ADDRESS, IO_Pin, IO_Mode); 00210 00211 return IO_OK; 00212 } 00213 00214 /** 00215 * @brief Set the selected IO pin(s) state. 00216 * @param IO_Pin: Selected IO pin(s) to write. 00217 * This parameter can be any combination of the IO pin(s). 00218 * @param PinState: New pin state to write 00219 * @retval None 00220 */ 00221 void BSP_IO_WritePin(uint32_t IO_Pin, uint8_t PinState) 00222 { 00223 /* Set the IO pin(s) state */ 00224 io_driver->WritePin(IO_I2C_ADDRESS, IO_Pin, PinState); 00225 } 00226 00227 /** 00228 * @brief Get the selected IO pin(s) current state. 00229 * @param IO_Pin: Selected pin(s) to read. 00230 * This parameter can be any combination of the IO pin(s). 00231 * @retval The current pins state 00232 */ 00233 uint32_t BSP_IO_ReadPin(uint32_t IO_Pin) 00234 { 00235 return(io_driver->ReadPin(IO_I2C_ADDRESS, IO_Pin)); 00236 } 00237 00238 /** 00239 * @brief Toggle the selected IO pin(s) state 00240 * @param IO_Pin: Selected IO pin(s) to toggle. 00241 * This parameter can be any combination of the IO pin(s). 00242 * @retval None 00243 */ 00244 void BSP_IO_TogglePin(uint32_t IO_Pin) 00245 { 00246 /* Toggle the IO selected pin(s) state */ 00247 if(io_driver->ReadPin(IO_I2C_ADDRESS, IO_Pin) != 0) /* Set */ 00248 { 00249 io_driver->WritePin(IO_I2C_ADDRESS, IO_Pin, 0); /* Reset */ 00250 } 00251 else 00252 { 00253 io_driver->WritePin(IO_I2C_ADDRESS, IO_Pin, 1); /* Set */ 00254 } 00255 } 00256 00257 /** 00258 * @} 00259 */ 00260 00261 /** 00262 * @} 00263 */ 00264 00265 /** 00266 * @} 00267 */ 00268 00269 /** 00270 * @} 00271 */ 00272 00273 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Oct 13 2017 02:37:42 for STM32L4R9I-Discovery BSP User Manual by
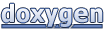