STM32L4R9I-Discovery BSP User Manual
|
stm32l4r9i_discovery_idd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4r9i_discovery_idd.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of firmware functions to manage the 00006 * Idd measurement driver for STM32L4R9I_DISCOVERY board. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Includes ------------------------------------------------------------------*/ 00038 #include "stm32l4r9i_discovery_idd.h" 00039 #include "stm32l4r9i_discovery_io.h" 00040 00041 /** @addtogroup BSP 00042 * @{ 00043 */ 00044 00045 /** @addtogroup STM32L4R9I_DISCOVERY 00046 * @{ 00047 */ 00048 00049 /** @defgroup STM32L4R9I_DISCOVERY_IDD STM32L4R9I_DISCOVERY IDD 00050 * @brief This file includes the Idd driver for STM32L4R9I_DISCOVERY board. 00051 * It allows user to measure MCU Idd current on board, especially in 00052 * different low power modes. 00053 * @{ 00054 */ 00055 00056 /** @defgroup STM32L4R9I_DISCOVERY_IDD_Private_Variables Private Variables 00057 * @{ 00058 */ 00059 static IDD_DrvTypeDef *IddDrv; 00060 00061 /** 00062 * @} 00063 */ 00064 00065 /** @defgroup STM32L4R9I_DISCOVERY_IDD_Exported_Functions Exported Functions 00066 * @{ 00067 */ 00068 00069 /** 00070 * @brief Configures IDD measurement component. 00071 * @retval IDD_OK if no problem during initialization 00072 */ 00073 uint8_t BSP_IDD_Init(void) 00074 { 00075 IDD_ConfigTypeDef iddconfig = {0}; 00076 uint8_t mfxstm32l152_id = 0; 00077 uint8_t ret = 0; 00078 00079 /* Initialize IO functionalities (MFX) used by control amp */ 00080 BSP_IO_Init(); 00081 00082 /* wake up mfx component in case it went to standby mode */ 00083 mfxstm32l152_idd_drv.WakeUp(IDD_I2C_ADDRESS); 00084 HAL_Delay(5); 00085 00086 /* Read ID and verify if the MFX is ready */ 00087 mfxstm32l152_id = mfxstm32l152_idd_drv.ReadID(IDD_I2C_ADDRESS); 00088 00089 if((mfxstm32l152_id == MFXSTM32L152_ID_1) || (mfxstm32l152_id == MFXSTM32L152_ID_2)) 00090 { 00091 /* Initialize the Idd driver structure */ 00092 IddDrv = &mfxstm32l152_idd_drv; 00093 00094 /* Initialize the Idd driver */ 00095 if(IddDrv->Init != NULL) 00096 { 00097 IddDrv->Init(IDD_I2C_ADDRESS); 00098 } 00099 00100 /* Configure Idd component with default values */ 00101 iddconfig.AmpliGain = DISCOVERY_IDD_AMPLI_GAIN; 00102 iddconfig.VddMin = DISCOVERY_IDD_VDD_MIN; 00103 iddconfig.Shunt0Value = DISCOVERY_IDD_SHUNT0_VALUE; 00104 iddconfig.Shunt1Value = DISCOVERY_IDD_SHUNT1_VALUE; 00105 iddconfig.Shunt2Value = DISCOVERY_IDD_SHUNT2_VALUE; 00106 iddconfig.Shunt3Value = 0; 00107 iddconfig.Shunt4Value = DISCOVERY_IDD_SHUNT4_VALUE; 00108 iddconfig.Shunt0StabDelay = DISCOVERY_IDD_SHUNT0_STABDELAY; 00109 iddconfig.Shunt1StabDelay = DISCOVERY_IDD_SHUNT1_STABDELAY; 00110 iddconfig.Shunt2StabDelay = DISCOVERY_IDD_SHUNT2_STABDELAY; 00111 iddconfig.Shunt3StabDelay = 0; 00112 iddconfig.Shunt4StabDelay = DISCOVERY_IDD_SHUNT4_STABDELAY; 00113 iddconfig.ShuntNbOnBoard = MFXSTM32L152_IDD_SHUNT_NB_4; 00114 iddconfig.ShuntNbUsed = MFXSTM32L152_IDD_SHUNT_NB_4; 00115 iddconfig.VrefMeasurement = MFXSTM32L152_IDD_VREF_AUTO_MEASUREMENT_ENABLE; 00116 iddconfig.Calibration = MFXSTM32L152_IDD_AUTO_CALIBRATION_ENABLE; 00117 iddconfig.PreDelayUnit = MFXSTM32L152_IDD_PREDELAY_20_MS; 00118 iddconfig.PreDelayValue = 0x7F; 00119 iddconfig.MeasureNb = 100; 00120 iddconfig.DeltaDelayUnit= MFXSTM32L152_IDD_DELTADELAY_0_5_MS; 00121 iddconfig.DeltaDelayValue = 10; 00122 BSP_IDD_Config(iddconfig); 00123 00124 ret = IDD_OK; 00125 } 00126 else 00127 { 00128 ret = IDD_ERROR; 00129 } 00130 00131 return ret; 00132 } 00133 00134 /** 00135 * @brief Unconfigures IDD measurement component. 00136 * @retval IDD_OK if no problem during deinitialization 00137 */ 00138 void BSP_IDD_DeInit(void) 00139 { 00140 if(IddDrv->DeInit!= NULL) 00141 { 00142 IddDrv->DeInit(IDD_I2C_ADDRESS); 00143 } 00144 } 00145 00146 /** 00147 * @brief Reset Idd measurement component. 00148 * @retval None 00149 */ 00150 void BSP_IDD_Reset(void) 00151 { 00152 if(IddDrv->Reset != NULL) 00153 { 00154 IddDrv->Reset(IDD_I2C_ADDRESS); 00155 } 00156 } 00157 00158 /** 00159 * @brief Turn Idd measurement component in low power (standby/sleep) mode 00160 * @retval None 00161 */ 00162 void BSP_IDD_LowPower(void) 00163 { 00164 if(IddDrv->LowPower != NULL) 00165 { 00166 IddDrv->LowPower(IDD_I2C_ADDRESS); 00167 } 00168 } 00169 00170 /** 00171 * @brief Start Measurement campaign 00172 * @retval None 00173 */ 00174 void BSP_IDD_StartMeasure(void) 00175 { 00176 00177 /* Activate the OPAMP used ny the MFX to measure the current consumption */ 00178 BSP_IO_ConfigPin(IDD_AMP_CONTROL_PIN, IO_MODE_OUTPUT); 00179 BSP_IO_WritePin(IDD_AMP_CONTROL_PIN, GPIO_PIN_RESET); 00180 00181 if(IddDrv->Start != NULL) 00182 { 00183 IddDrv->Start(IDD_I2C_ADDRESS); 00184 } 00185 } 00186 00187 /** 00188 * @brief Configure Idd component 00189 * @param IddConfig: structure of idd parameters 00190 * @retval None 00191 */ 00192 void BSP_IDD_Config(IDD_ConfigTypeDef IddConfig) 00193 { 00194 if(IddDrv->Config != NULL) 00195 { 00196 IddDrv->Config(IDD_I2C_ADDRESS, IddConfig); 00197 } 00198 } 00199 00200 /** 00201 * @brief Get Idd current value. 00202 * @param IddValue: Pointer on u32 to store Idd. Value unit is 10 nA. 00203 * @retval None 00204 */ 00205 void BSP_IDD_GetValue(uint32_t *IddValue) 00206 { 00207 /* De-activate the OPAMP used ny the MFX to measure the current consumption */ 00208 BSP_IO_ConfigPin(IDD_AMP_CONTROL_PIN, IO_MODE_OUTPUT); 00209 BSP_IO_WritePin(IDD_AMP_CONTROL_PIN, GPIO_PIN_RESET); 00210 00211 if(IddDrv->GetValue != NULL) 00212 { 00213 IddDrv->GetValue(IDD_I2C_ADDRESS, IddValue); 00214 } 00215 } 00216 00217 /** 00218 * @brief Enable Idd interrupt that warn end of measurement 00219 * @retval None 00220 */ 00221 void BSP_IDD_EnableIT(void) 00222 { 00223 if(IddDrv->EnableIT != NULL) 00224 { 00225 IddDrv->EnableIT(IDD_I2C_ADDRESS); 00226 } 00227 } 00228 00229 /** 00230 * @brief Clear Idd interrupt that warn end of measurement 00231 * @retval None 00232 */ 00233 void BSP_IDD_ClearIT(void) 00234 { 00235 if(IddDrv->ClearIT != NULL) 00236 { 00237 IddDrv->ClearIT(IDD_I2C_ADDRESS); 00238 } 00239 } 00240 00241 /** 00242 * @brief Get Idd interrupt status 00243 * @retval status 00244 */ 00245 uint8_t BSP_IDD_GetITStatus(void) 00246 { 00247 if(IddDrv->GetITStatus != NULL) 00248 { 00249 return (IddDrv->GetITStatus(IDD_I2C_ADDRESS)); 00250 } 00251 else 00252 { 00253 return IDD_ERROR; 00254 } 00255 } 00256 00257 /** 00258 * @brief Disable Idd interrupt that warn end of measurement 00259 * @retval None 00260 */ 00261 void BSP_IDD_DisableIT(void) 00262 { 00263 if(IddDrv->DisableIT != NULL) 00264 { 00265 IddDrv->DisableIT(IDD_I2C_ADDRESS); 00266 } 00267 } 00268 00269 /** 00270 * @brief Get Error Code . 00271 * @retval Error code or error status 00272 */ 00273 uint8_t BSP_IDD_ErrorGetCode(void) 00274 { 00275 if(IddDrv->ErrorGetSrc != NULL) 00276 { 00277 if((IddDrv->ErrorGetSrc(IDD_I2C_ADDRESS) & MFXSTM32L152_IDD_ERROR_SRC) != RESET) 00278 { 00279 if(IddDrv->ErrorGetCode != NULL) 00280 { 00281 return IddDrv->ErrorGetCode(IDD_I2C_ADDRESS); 00282 } 00283 else 00284 { 00285 return IDD_ERROR; 00286 } 00287 } 00288 else 00289 { 00290 return IDD_ERROR; 00291 } 00292 } 00293 else 00294 { 00295 return IDD_ERROR; 00296 } 00297 } 00298 00299 00300 /** 00301 * @brief Enable error interrupt that warn end of measurement 00302 * @retval None 00303 */ 00304 void BSP_IDD_ErrorEnableIT(void) 00305 { 00306 if(IddDrv->ErrorEnableIT != NULL) 00307 { 00308 IddDrv->ErrorEnableIT(IDD_I2C_ADDRESS); 00309 } 00310 } 00311 00312 /** 00313 * @brief Clear Error interrupt that warn end of measurement 00314 * @retval None 00315 */ 00316 void BSP_IDD_ErrorClearIT(void) 00317 { 00318 if(IddDrv->ErrorClearIT != NULL) 00319 { 00320 IddDrv->ErrorClearIT(IDD_I2C_ADDRESS); 00321 } 00322 } 00323 00324 /** 00325 * @brief Get Error interrupt status 00326 * @retval Status 00327 */ 00328 uint8_t BSP_IDD_ErrorGetITStatus(void) 00329 { 00330 if(IddDrv->ErrorGetITStatus != NULL) 00331 { 00332 return (IddDrv->ErrorGetITStatus(IDD_I2C_ADDRESS)); 00333 } 00334 else 00335 { 00336 return 0; 00337 } 00338 } 00339 00340 /** 00341 * @brief Disable Error interrupt 00342 * @retval None 00343 */ 00344 void BSP_IDD_ErrorDisableIT(void) 00345 { 00346 if(IddDrv->ErrorDisableIT != NULL) 00347 { 00348 IddDrv->ErrorDisableIT(IDD_I2C_ADDRESS); 00349 } 00350 } 00351 00352 /** 00353 * @brief Wake up Idd measurement component. 00354 * @retval None 00355 */ 00356 void BSP_IDD_WakeUp(void) 00357 { 00358 if(IddDrv->WakeUp != NULL) 00359 { 00360 IddDrv->WakeUp(IDD_I2C_ADDRESS); 00361 } 00362 } 00363 00364 /** 00365 * @} 00366 */ 00367 00368 /** 00369 * @} 00370 */ 00371 00372 /** 00373 * @} 00374 */ 00375 00376 /** 00377 * @} 00378 */ 00379 00380 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00381
Generated on Fri Oct 13 2017 02:37:42 for STM32L4R9I-Discovery BSP User Manual by
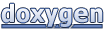