STM32L4R9I-Discovery BSP User Manual
|
stm32l4r9i_discovery_idd.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4r9i_discovery_idd.h 00004 * @author MCD Application Team 00005 * @brief Header file for stm32l4r9i_discovery_idd.c module. 00006 ****************************************************************************** 00007 * @attention 00008 * 00009 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00010 * 00011 * Redistribution and use in source and binary forms, with or without modification, 00012 * are permitted provided that the following conditions are met: 00013 * 1. Redistributions of source code must retain the above copyright notice, 00014 * this list of conditions and the following disclaimer. 00015 * 2. Redistributions in binary form must reproduce the above copyright notice, 00016 * this list of conditions and the following disclaimer in the documentation 00017 * and/or other materials provided with the distribution. 00018 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00019 * may be used to endorse or promote products derived from this software 00020 * without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00023 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00024 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00025 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00026 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00027 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00028 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00029 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00030 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00031 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00032 * 00033 ****************************************************************************** 00034 */ 00035 00036 /* Define to prevent recursive inclusion -------------------------------------*/ 00037 #ifndef __STM32L4R9I_DISCOVERY_IDD_H 00038 #define __STM32L4R9I_DISCOVERY_IDD_H 00039 00040 #ifdef __cplusplus 00041 extern "C" { 00042 #endif 00043 00044 /* Includes ------------------------------------------------------------------*/ 00045 #include "stm32l4r9i_discovery.h" 00046 /* Include Idd measurement component driver */ 00047 #include "../Components/mfxstm32l152/mfxstm32l152.h" 00048 00049 /** @addtogroup BSP 00050 * @{ 00051 */ 00052 00053 /** @addtogroup STM32L4R9I_DISCOVERY 00054 * @{ 00055 */ 00056 00057 /** @addtogroup STM32L4R9I_DISCOVERY_IDD 00058 * @{ 00059 */ 00060 00061 /** @defgroup STM32L4R9I_DISCOVERY_IDD_Exported_Types Exported Types 00062 * @{ 00063 */ 00064 00065 /** @defgroup IDD_Config IDD Config 00066 * @{ 00067 */ 00068 typedef enum 00069 { 00070 IDD_OK = 0, 00071 IDD_TIMEOUT = 1, 00072 IDD_ZERO_VALUE = 2, 00073 IDD_ERROR = 0xFF 00074 } 00075 IDD_StatusTypeDef; 00076 /** 00077 * @} 00078 */ 00079 00080 /** 00081 * @} 00082 */ 00083 00084 /** @defgroup STM32L4R9I_DISCOVERY_IDD_Exported_Defines Exported Defines 00085 * @{ 00086 */ 00087 /** 00088 * @brief Shunt values on discovery in milli ohms 00089 */ 00090 #define DISCOVERY_IDD_SHUNT0_VALUE ((uint16_t) 1000) /*!< value in milliohm */ 00091 #define DISCOVERY_IDD_SHUNT1_VALUE ((uint16_t) 24) /*!< value in ohm */ 00092 #define DISCOVERY_IDD_SHUNT2_VALUE ((uint16_t) 620) /*!< value in ohm */ 00093 #define DISCOVERY_IDD_SHUNT4_VALUE ((uint16_t) 10000) /*!< value in ohm */ 00094 00095 /** 00096 * @brief Shunt stabilization delay on discovery in milli ohms 00097 */ 00098 #define DISCOVERY_IDD_SHUNT0_STABDELAY ((uint8_t) 149) /*!< value in millisec */ 00099 #define DISCOVERY_IDD_SHUNT1_STABDELAY ((uint8_t) 149) /*!< value in millisec */ 00100 #define DISCOVERY_IDD_SHUNT2_STABDELAY ((uint8_t) 149) /*!< value in millisec */ 00101 #define DISCOVERY_IDD_SHUNT4_STABDELAY ((uint8_t) 255) /*!< value in millisec */ 00102 00103 /** 00104 * @brief IDD Ampli Gain on discovery 00105 */ 00106 #define DISCOVERY_IDD_AMPLI_GAIN ((uint16_t) 4967) /*!< value is gain * 100 */ 00107 00108 /** 00109 * @brief IDD Vdd Min on discovery 00110 */ 00111 #define DISCOVERY_IDD_VDD_MIN ((uint16_t) 1200) /*!< value in millivolt */ 00112 00113 /** 00114 * @} 00115 */ 00116 00117 /* Exported functions --------------------------------------------------------*/ 00118 /** @defgroup STM32L4R9I_DISCOVERY_IDD_Exported_Functions Exported Functions 00119 * @{ 00120 */ 00121 uint8_t BSP_IDD_Init(void); 00122 void BSP_IDD_DeInit(void); 00123 void BSP_IDD_Reset(void); 00124 void BSP_IDD_LowPower(void); 00125 void BSP_IDD_WakeUp(void); 00126 void BSP_IDD_StartMeasure(void); 00127 void BSP_IDD_Config(IDD_ConfigTypeDef IddConfig); 00128 void BSP_IDD_GetValue(uint32_t *IddValue); 00129 void BSP_IDD_EnableIT(void); 00130 void BSP_IDD_ClearIT(void); 00131 uint8_t BSP_IDD_GetITStatus(void); 00132 void BSP_IDD_DisableIT(void); 00133 uint8_t BSP_IDD_ErrorGetCode(void); 00134 void BSP_IDD_ErrorEnableIT(void); 00135 void BSP_IDD_ErrorClearIT(void); 00136 uint8_t BSP_IDD_ErrorGetITStatus(void); 00137 void BSP_IDD_ErrorDisableIT(void); 00138 00139 /** 00140 * @} 00141 */ 00142 00143 /** 00144 * @} 00145 */ 00146 00147 /** 00148 * @} 00149 */ 00150 00151 /** 00152 * @} 00153 */ 00154 00155 #ifdef __cplusplus 00156 } 00157 #endif 00158 00159 #endif /* __STM32L4R9I_DISCOVERY_IDD_H */ 00160 00161 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Oct 13 2017 02:37:42 for STM32L4R9I-Discovery BSP User Manual by
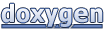