STM32L073Z_EVAL BSP User Manual
|
stm32l073z_eval_glass_lcd.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file STM32L073Z_eval_glass_lcd.h 00004 * @author MCD Application Team 00005 * @brief Header file for STM32L073Z_eval_glass_lcd.c module. 00006 ****************************************************************************** 00007 * @attention 00008 * 00009 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00010 * 00011 * Redistribution and use in source and binary forms, with or without modification, 00012 * are permitted provided that the following conditions are met: 00013 * 1. Redistributions of source code must retain the above copyright notice, 00014 * this list of conditions and the following disclaimer. 00015 * 2. Redistributions in binary form must reproduce the above copyright notice, 00016 * this list of conditions and the following disclaimer in the documentation 00017 * and/or other materials provided with the distribution. 00018 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00019 * may be used to endorse or promote products derived from this software 00020 * without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00023 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00024 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00025 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00026 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00027 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00028 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00029 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00030 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00031 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00032 * 00033 ****************************************************************************** 00034 */ 00035 00036 /** @addtogroup BSP 00037 * @{ 00038 */ 00039 00040 /** @addtogroup STM32L073Z_EVAL 00041 * @{ 00042 */ 00043 00044 /* Define to prevent recursive inclusion -------------------------------------*/ 00045 #ifndef __STM32L073Z_EVAL_GLASS_LCD_H 00046 #define __STM32L073Z_EVAL_GLASS_LCD_H 00047 00048 #ifdef __cplusplus 00049 extern "C" { 00050 #endif 00051 00052 /* Includes ------------------------------------------------------------------*/ 00053 #include "stm32l073z_eval.h" 00054 00055 /** @addtogroup STM32L073Z_EVAL_GLASS_LCD 00056 * @{ 00057 */ 00058 00059 /** @defgroup STM32L073Z_EVAL_GLASS_LCD_Exported_Types Exported Types 00060 * @{ 00061 */ 00062 00063 /** 00064 * @brief LCD Glass point 00065 * Warning: element values correspond to LCD Glass point. 00066 */ 00067 00068 typedef enum 00069 { 00070 POINT_OFF = 0, 00071 POINT_ON = 1 00072 }Point_Typedef; 00073 00074 /** 00075 * @brief LCD Glass Double point 00076 * Warning: element values correspond to LCD Glass Double point. 00077 */ 00078 typedef enum 00079 { 00080 DOUBLEPOINT_OFF = 0, 00081 DOUBLEPOINT_ON = 1 00082 }DoublePoint_Typedef; 00083 00084 /** 00085 * @brief LCD Glass Battery Level 00086 * Warning: element values correspond to LCD Glass Battery Level. 00087 */ 00088 00089 typedef enum 00090 { 00091 BATTERYLEVEL_OFF = 0, 00092 BATTERYLEVEL_1_4 = 1, 00093 BATTERYLEVEL_1_2 = 2, 00094 BATTERYLEVEL_3_4 = 3, 00095 BATTERYLEVEL_FULL = 4 00096 }BatteryLevel_TypeDef; 00097 00098 /** 00099 * @brief LCD Glass Temperature Level 00100 * Warning: element values correspond to LCD Glass Temperature Level. 00101 */ 00102 00103 typedef enum 00104 { 00105 TEMPERATURELEVEL_OFF = 0, 00106 TEMPERATURELEVEL_1 = 1, 00107 TEMPERATURELEVEL_2 = 2, 00108 TEMPERATURELEVEL_3 = 3, 00109 TEMPERATURELEVEL_4 = 4, 00110 TEMPERATURELEVEL_5 = 5, 00111 TEMPERATURELEVEL_6 = 6 00112 }TemperatureLevel_TypeDef; 00113 00114 /** 00115 * @brief LCD Glass Arrow Direction 00116 * Warning: element values correspond to LCD Glass Arrow Direction. 00117 */ 00118 00119 typedef enum 00120 { 00121 ARROWDIRECTION_OFF = 0, 00122 ARROWDIRECTION_UP = 1, 00123 ARROWDIRECTION_DOWN = 2, 00124 ARROWDIRECTION_LEFT = 3, 00125 ARROWDIRECTION_RIGHT = 4 00126 }ArrowDirection_TypeDef; 00127 00128 /** 00129 * @brief LCD Glass Value Unit 00130 * Warning: element values correspond to LCD Glass Value Unit. 00131 */ 00132 typedef enum 00133 { 00134 VALUEUNIT_OFF = 0, 00135 VALUEUNIT_MILLIAMPERE = 1, 00136 VALUEUNIT_MICROAMPERE = 2, 00137 VALUEUNIT_NANOAMPERE = 3 00138 }ValueUnit_TypeDef; 00139 00140 /** 00141 * @brief LCD Glass Sign 00142 * Warning: element values correspond to LCD Glass Sign. 00143 */ 00144 typedef enum 00145 { 00146 SIGN_POSITIVE = 0, 00147 SIGN_NEGATIVE = 1 00148 }Sign_TypeDef; 00149 00150 /** 00151 * @brief LCD Glass Pixel Row 00152 * Warning: element values correspond to LCD Glass Pixel Row. 00153 */ 00154 typedef enum 00155 { 00156 PIXELROW_1 = 1, 00157 PIXELROW_2 = 2, 00158 PIXELROW_3 = 3, 00159 PIXELROW_4 = 4, 00160 PIXELROW_5 = 5, 00161 PIXELROW_6 = 6, 00162 PIXELROW_7 = 7, 00163 PIXELROW_8 = 8, 00164 PIXELROW_9 = 9, 00165 PIXELROW_10 = 10 00166 }PixelRow_TypeDef; 00167 00168 /** 00169 * @brief LCD Glass Pixel Column 00170 * Warning: element values correspond to LCD Glass Pixel Column. 00171 */ 00172 typedef enum 00173 { 00174 PIXELCOLUMN_1 = 1, 00175 PIXELCOLUMN_2 = 2, 00176 PIXELCOLUMN_3 = 3, 00177 PIXELCOLUMN_4 = 4, 00178 PIXELCOLUMN_5 = 5, 00179 PIXELCOLUMN_6 = 6, 00180 PIXELCOLUMN_7 = 7, 00181 PIXELCOLUMN_8 = 8, 00182 PIXELCOLUMN_9 = 9, 00183 PIXELCOLUMN_10 = 10, 00184 PIXELCOLUMN_11 = 11, 00185 PIXELCOLUMN_12 = 12, 00186 PIXELCOLUMN_13 = 13, 00187 PIXELCOLUMN_14 = 14, 00188 PIXELCOLUMN_15 = 15, 00189 PIXELCOLUMN_16 = 16, 00190 PIXELCOLUMN_17 = 17, 00191 PIXELCOLUMN_18 = 18, 00192 PIXELCOLUMN_19 = 19 00193 }PixelColumn_TypeDef; 00194 00195 /** 00196 * @} 00197 */ 00198 00199 /** @defgroup STM32L073Z_EVAL_GLASS_LCD_Exported_Constants Exported_Constants 00200 * @{ 00201 */ 00202 00203 /* Define for scrolling sentences*/ 00204 #define SCROLL_SPEED 200 00205 #define SCROLL_SPEED_L 400 00206 #define SCROLL_NUM 1 00207 00208 #define DOT 0x8000 /* for add decimal point in string */ 00209 #define DOUBLE_DOT 0x4000 /* for add decimal point in string */ 00210 00211 /** 00212 * @} 00213 */ 00214 00215 00216 /** @addtogroup STM32L073Z_EVAL_GLASS_LCD_Exported_Functions Exported Functions 00217 * @{ 00218 */ 00219 void BSP_LCD_GLASS_Init(void); 00220 void BSP_LCD_GLASS_Contrast(uint32_t Contrast); 00221 void BSP_LCD_GLASS_DisplayChar(uint8_t* Ch, Point_Typedef Point, DoublePoint_Typedef DoublePoint, uint8_t Position); 00222 void BSP_LCD_GLASS_DisplayString(uint8_t* ptr); 00223 void BSP_LCD_GLASS_WriteChar(uint8_t* ch, uint8_t Point, uint8_t Column, uint8_t Position); 00224 void BSP_LCD_GLASS_ClearChar(uint8_t position); 00225 void BSP_LCD_GLASS_DisplayStrDeci(uint16_t* ptr); 00226 void BSP_LCD_GLASS_Clear(void); 00227 00228 void BSP_LCD_GLASS_ClearTextZone(void); 00229 void BSP_LCD_GLASS_DisplayLogo(FunctionalState NewState); 00230 void BSP_LCD_GLASS_ArrowConfig(ArrowDirection_TypeDef ArrowDirection); 00231 void BSP_LCD_GLASS_TemperatureConfig(TemperatureLevel_TypeDef TemperatureLevel); 00232 void BSP_LCD_GLASS_ValueUnitConfig(ValueUnit_TypeDef ValueUnit); 00233 void BSP_LCD_GLASS_SignCmd(Sign_TypeDef Sign, FunctionalState NewState); 00234 00235 void BSP_LCD_GLASS_WriteMatrixPixel(PixelRow_TypeDef PixelRow, PixelColumn_TypeDef PixelColumn); 00236 void BSP_LCD_GLASS_ClearMatrixPixel(PixelRow_TypeDef PixelRow, PixelColumn_TypeDef PixelColumn); 00237 00238 void BSP_LCD_GLASS_ScrollSentence(uint8_t* ptr, uint16_t nScroll, uint16_t ScrollSpeed); 00239 void BSP_LCD_GLASS_BarLevelConfig(uint8_t BarLevel); 00240 00241 /** 00242 * @} 00243 */ 00244 00245 /** 00246 * @} 00247 */ 00248 00249 #ifdef __cplusplus 00250 } 00251 #endif 00252 00253 #endif /* __STM32L073Z_EVAL_GLASS_LCD_H */ 00254 00255 /** 00256 * @} 00257 */ 00258 00259 /** 00260 * @} 00261 */ 00262 00263 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Mon Aug 28 2017 14:54:25 for STM32L073Z_EVAL BSP User Manual by
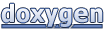